Learn how to monitor changes in browser storage (local storage, session storage, cookies) using JavaScript.
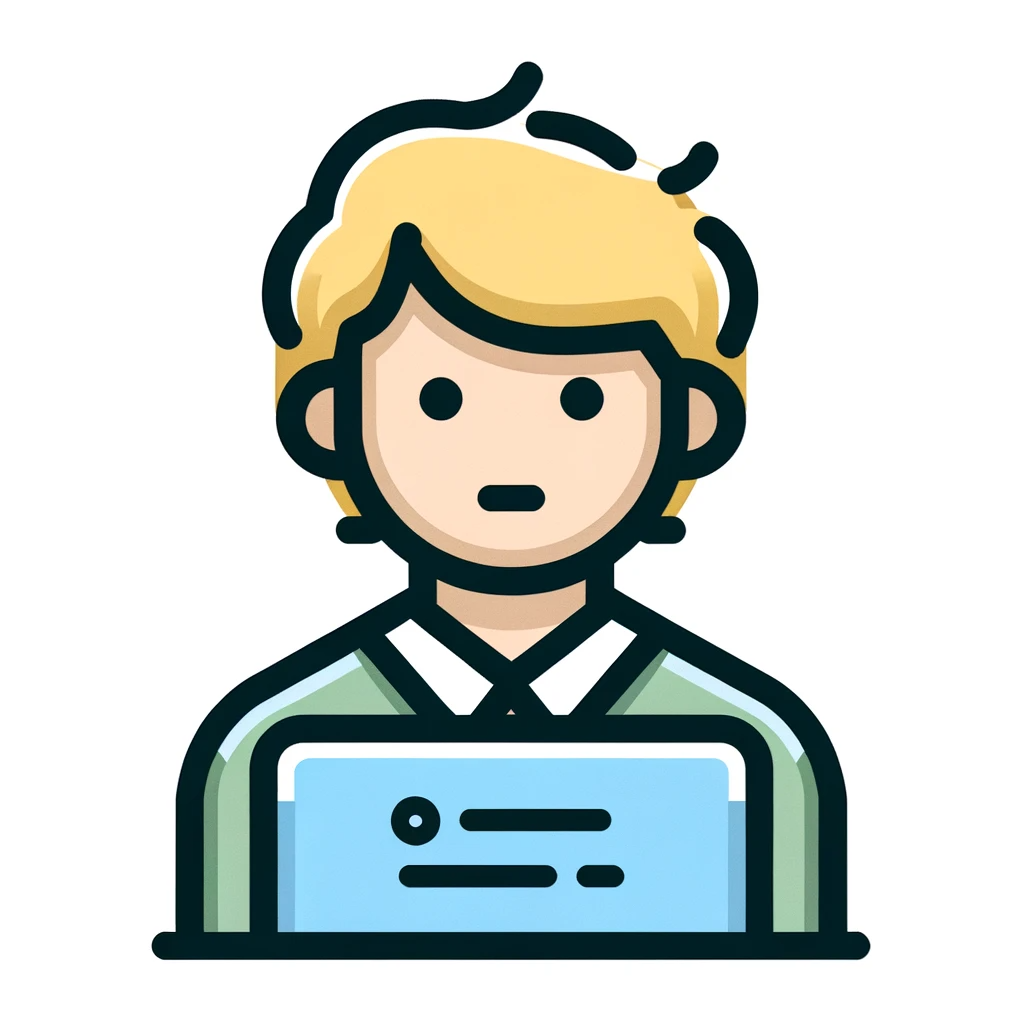
How can I detect storage changes using JavaScript?
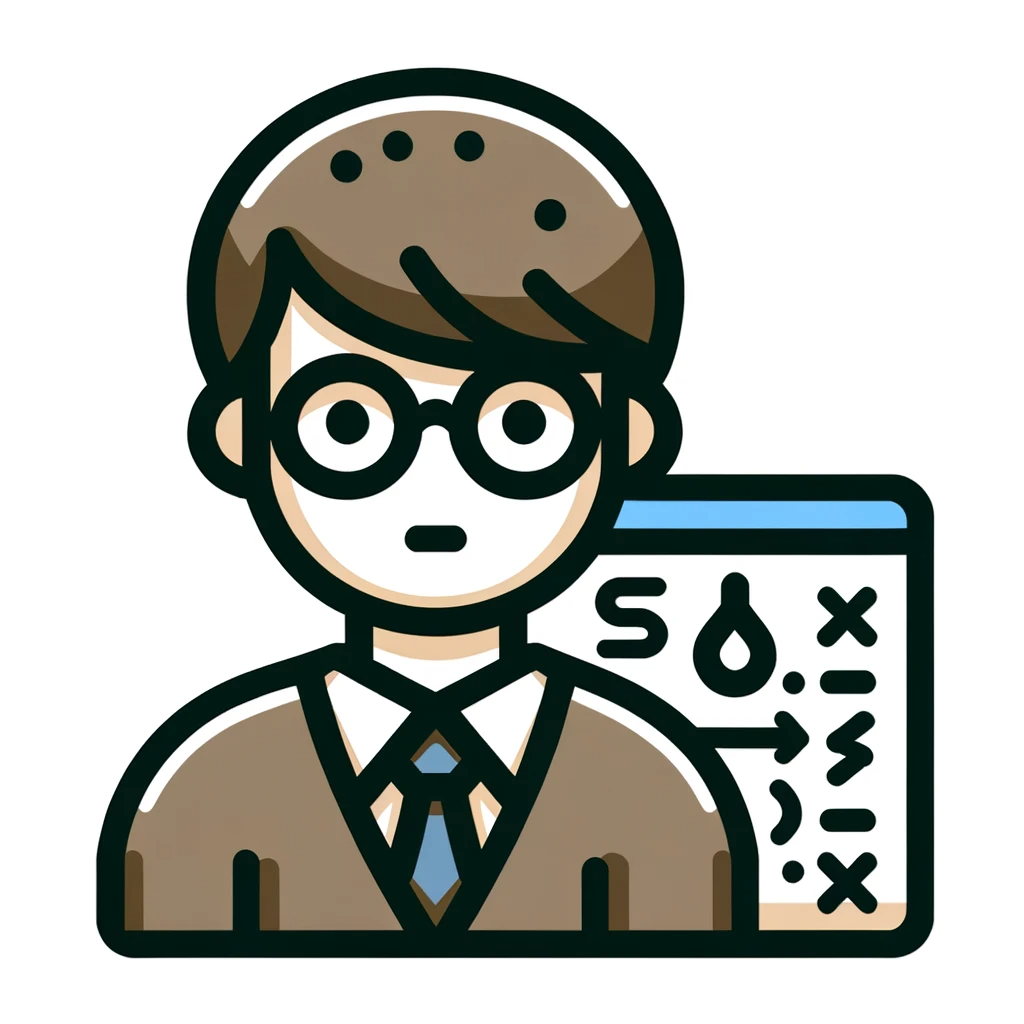
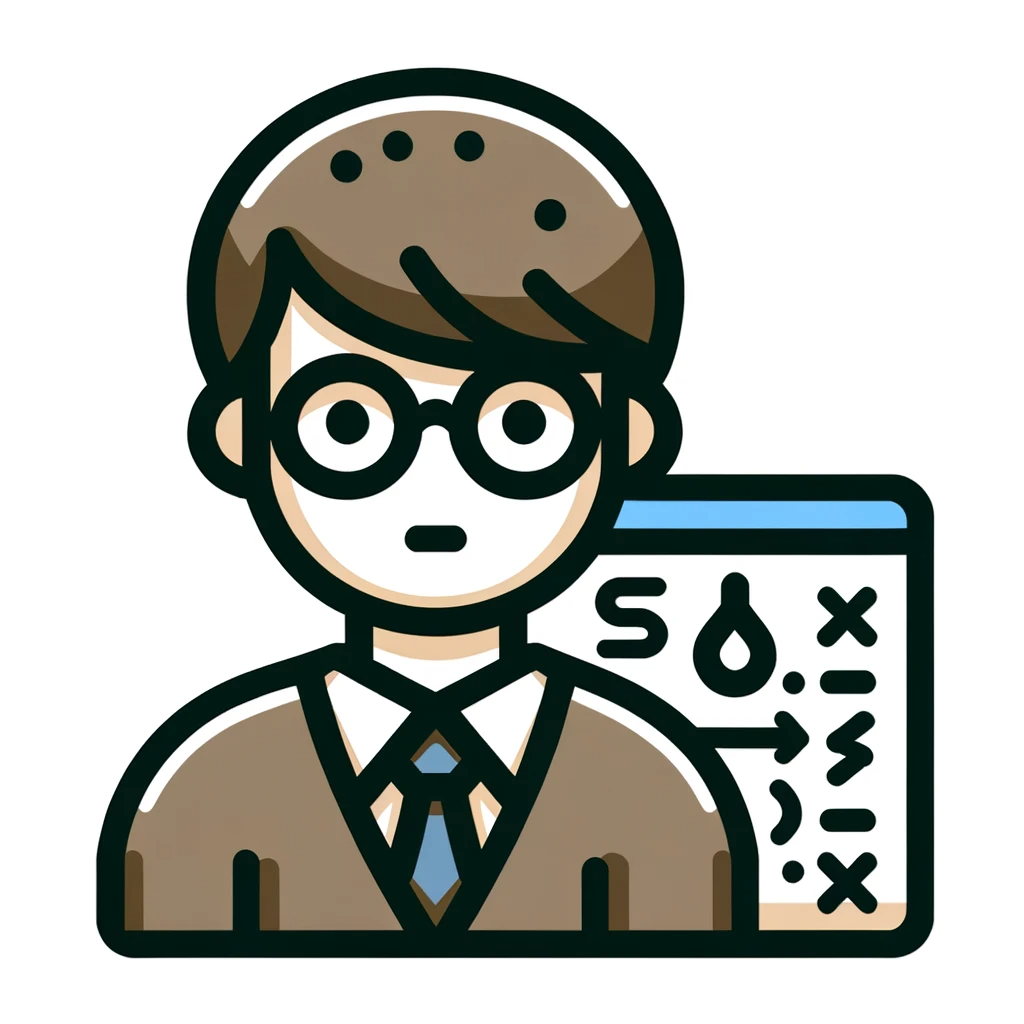
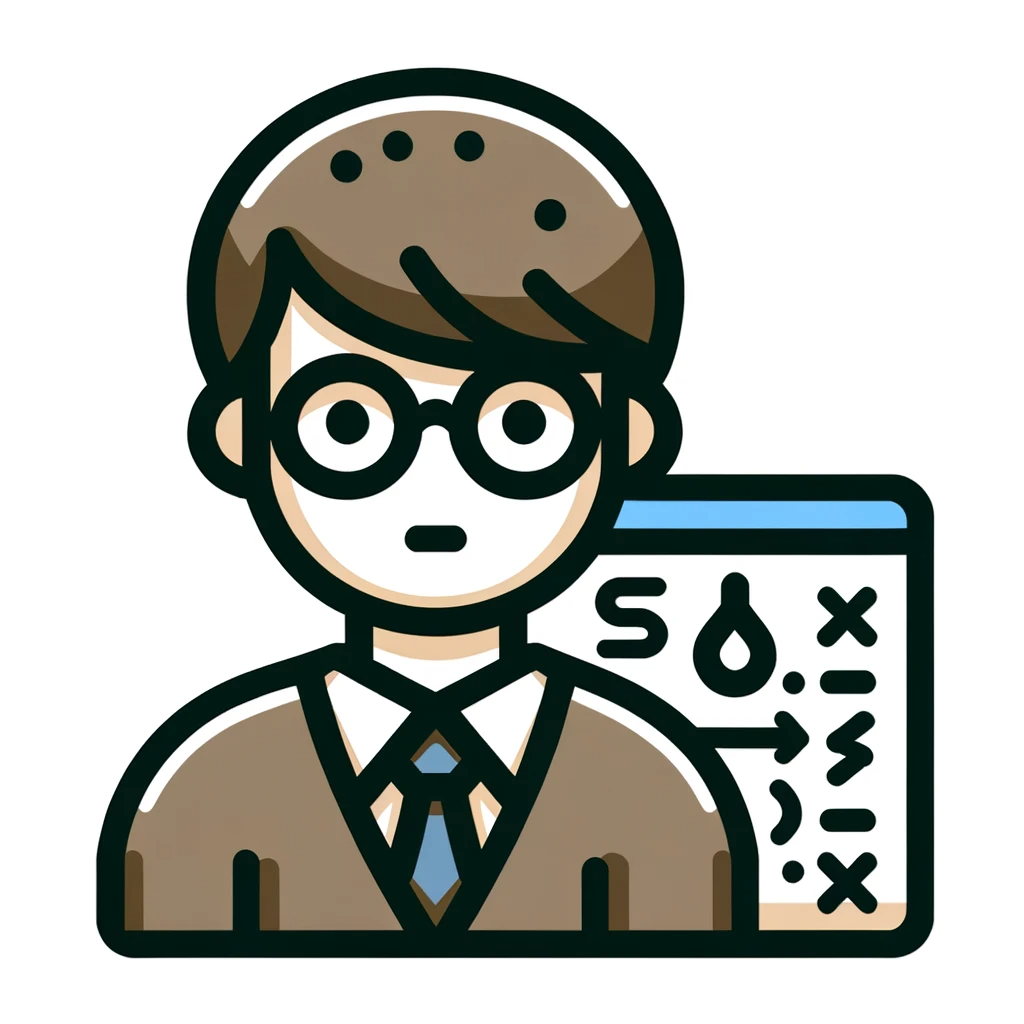
There are two ways to detect storage changes with JavaScript: using Storage events and periodically checking using setInterval.
How to use Storage events
Storage events are events that occur when values change in localStorage, sessionStorage, and cookies .
You can use this event to detect storage changes.
Use the addEventListener method to monitor Storage events. The following is an example of outputting logs to the console when the value of the key myKey in localStorage changes.
window.addEventListener('storage', function(e) {
if(e.key === 'myKey') {
console.log('myKeyの値が変更されました:', e.newValue);
}
});
In this way, you can monitor Storage events and retrieve changed values by the addEventListener method.
How to check periodically using setInterval
Since some browsers do not fire the Storage event, there is a way to periodically check the storage value using setInterval .
This method compares the current value with a previously saved value and takes action if there is a change.
The following is an example of outputting logs to the console when the value of the key myKey in localStorage changes.
let myKey = localStorage.getItem('myKey');
setInterval(function() {
if(localStorage.getItem('myKey') !== myKey) {
console.log('The value of myKey has been changed: myKey', localStorage.getItem('myKey'));
myKey = localStorage.getItem('myKey');
}
}, 1000);
In this way, you can periodically check the storage value using the setInterval method and retrieve the changed value.
Explanation using a sample program
The program below was implemented using two methods to detect storage changes: Storage events and setInterval.
// When using Storage events
window.addEventListener('storage', function(e) {
if(e.key === 'myKey') {
console.log('myKey value has been changed:', e.newValue);
}
});
// To check periodically using setInterval
let myKey = localStorage.getItem('myKey');
setInterval(function() {
if(localStorage.getItem('myKey') !== myKey) {
console.log('myKey value has been changed:', localStorage.getItem('myKey'));
myKey = localStorage.getItem('myKey');
}
}, 1000);
The first part shows how to monitor storage changes using Storage events. I am registering a storage event on the window object using the addEventListener method. When this event occurs, the function passed in the argument is executed and the changed storage key and value can be retrieved. This example will print logs to the console when myKey changes.
The second part shows how to use setInterval to periodically check the storage value. First, we are getting the initial value of myKey from localStorage. Then, it retrieves the value of myKey from localStorage every second, and if it is different from the previous value, it means there has been a change, so it outputs a log to the console. It also updates the value of myKey to the current value.
When you run this program, you will notice that it is monitoring storage changes. Both methods print logs to the console when storage values change. However, Storage events are not supported by all browsers, so using setInterval together can improve browser compatibility.
summary
We explained how to monitor changes in browser storage (local storage, session storage, cookies).
- There are two ways to detect storage changes using JavaScript:
- How to use Storage events
- Events fired when values change in localStorage, sessionStorage, cookies
- Monitor using addEventListener method
- How to check periodically using setInterval
- Check the value of localStorage regularly
- Perform processing if there is a change compared to the previous value
- Please note that it is only valid within the same origin.
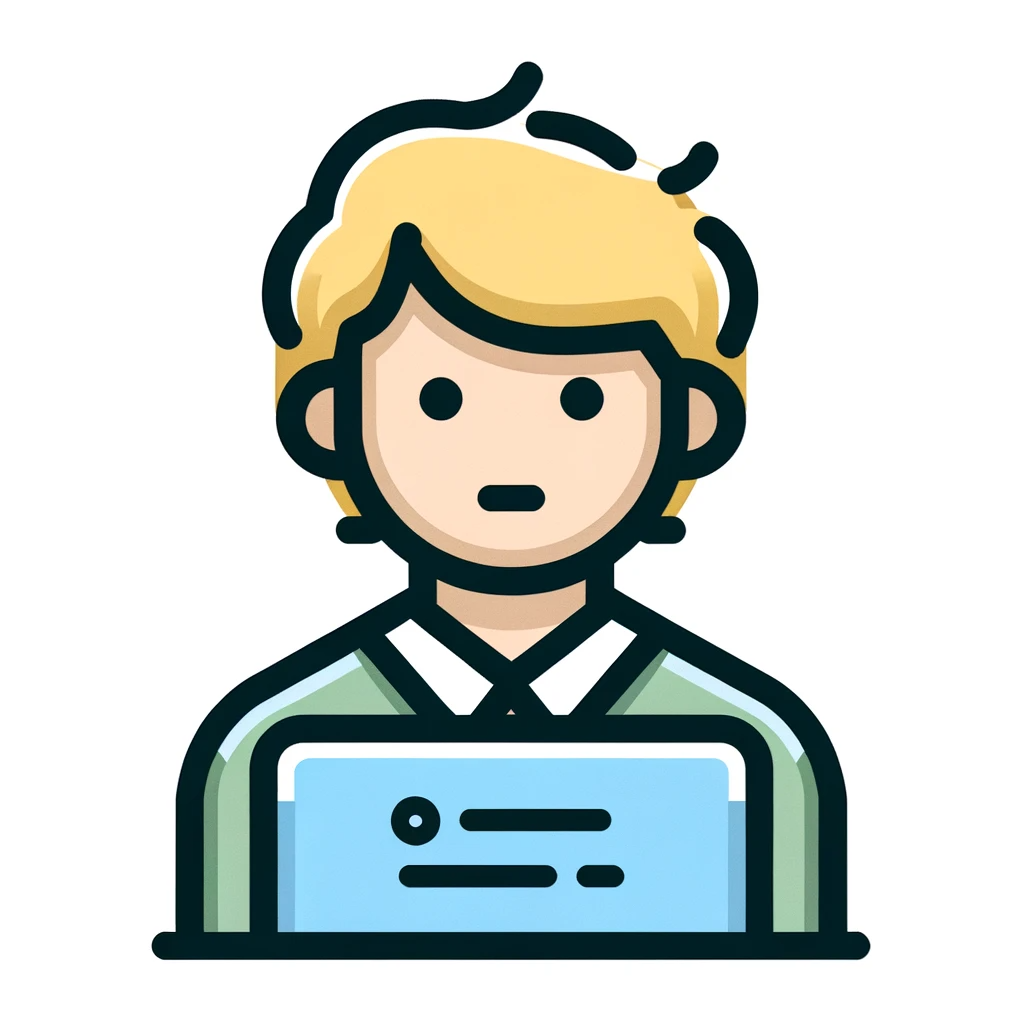
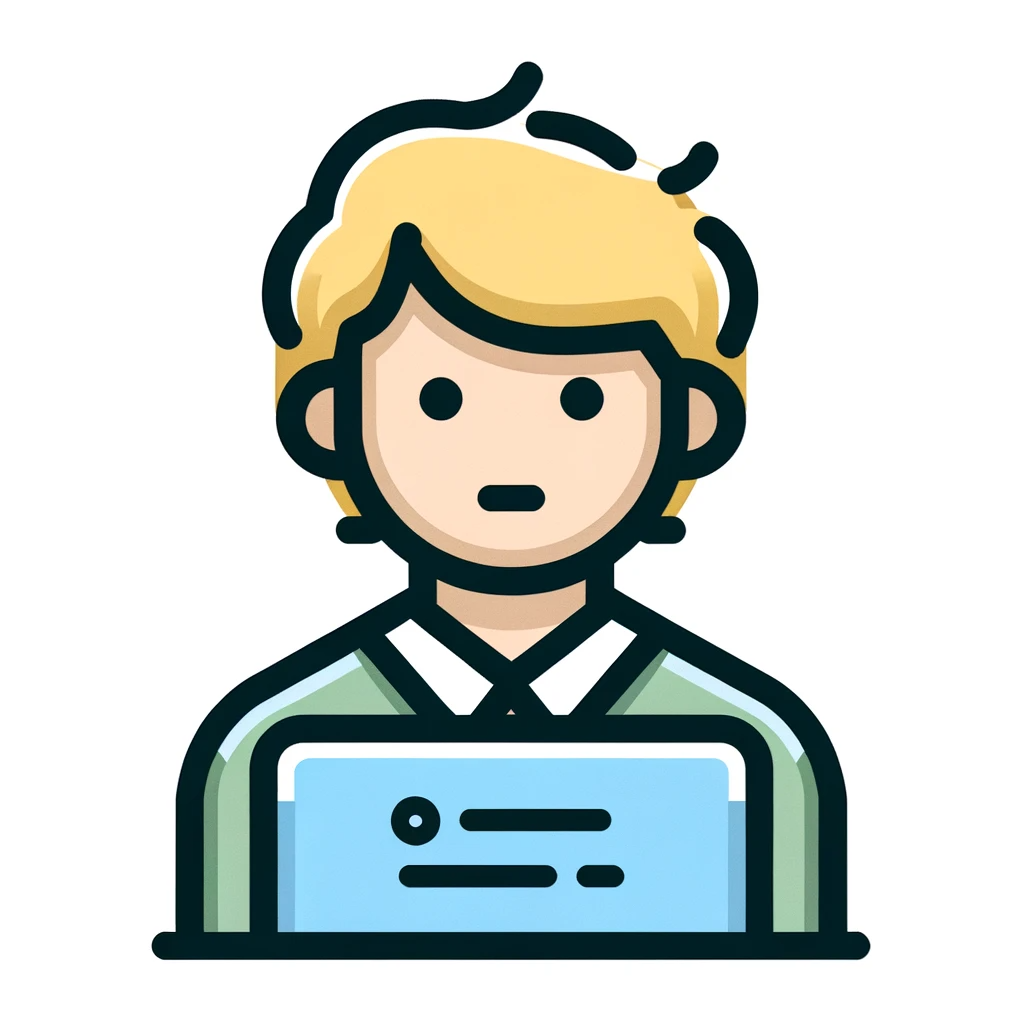
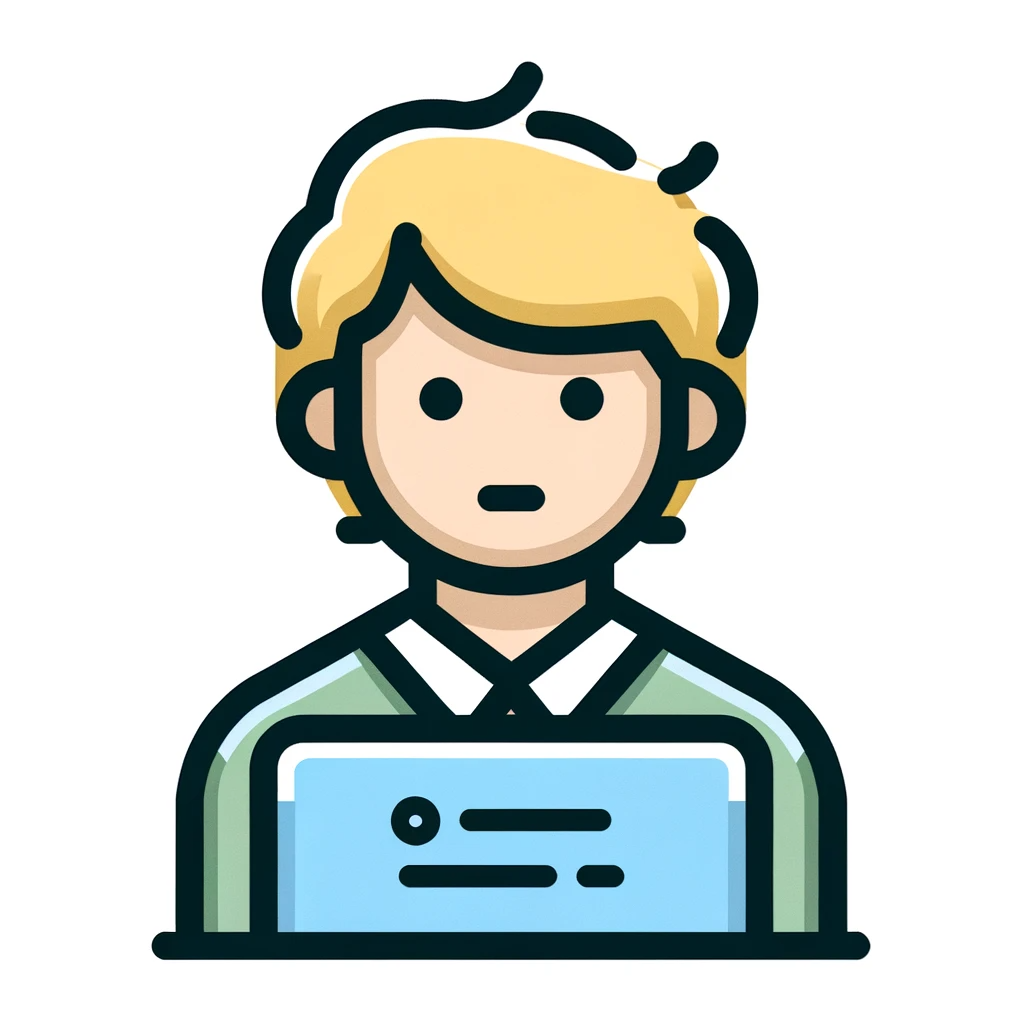
You now have a good understanding of how to monitor storage changes using Storage events and setInterval!
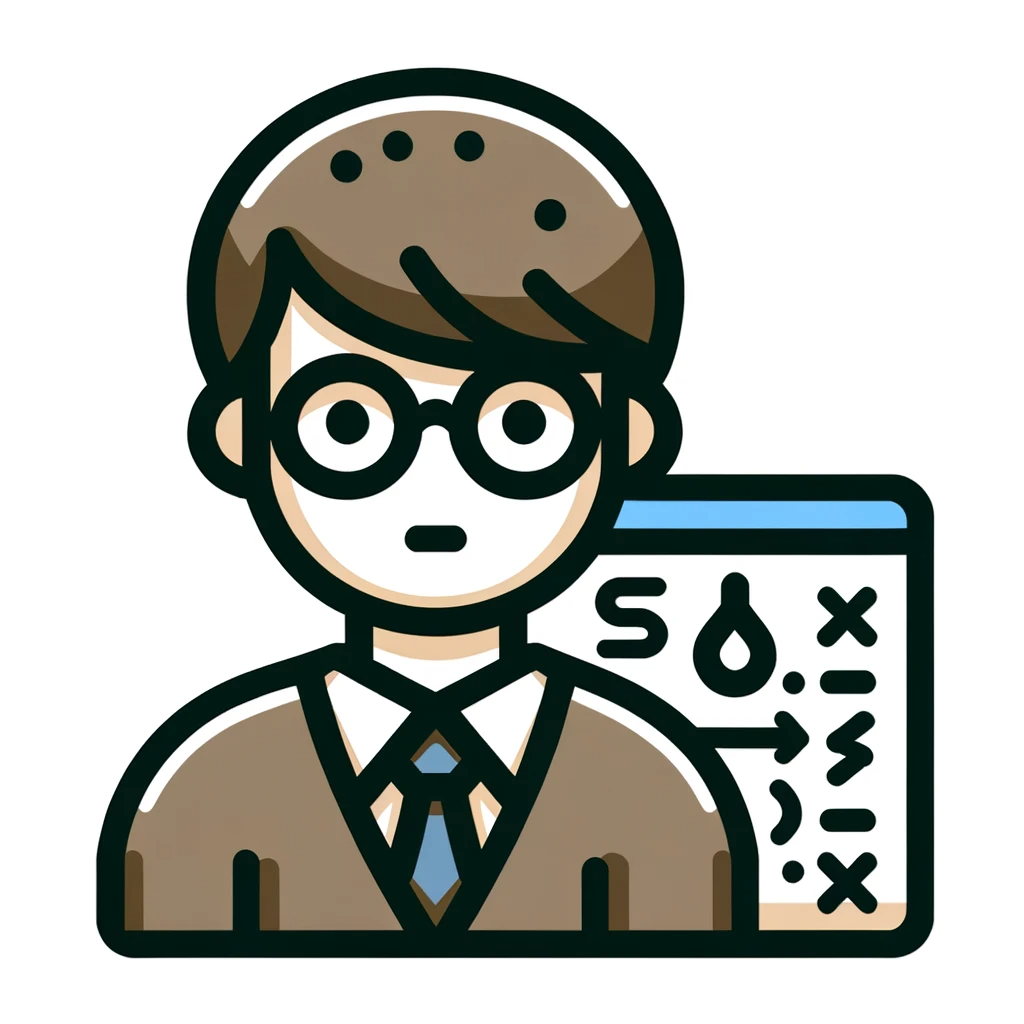
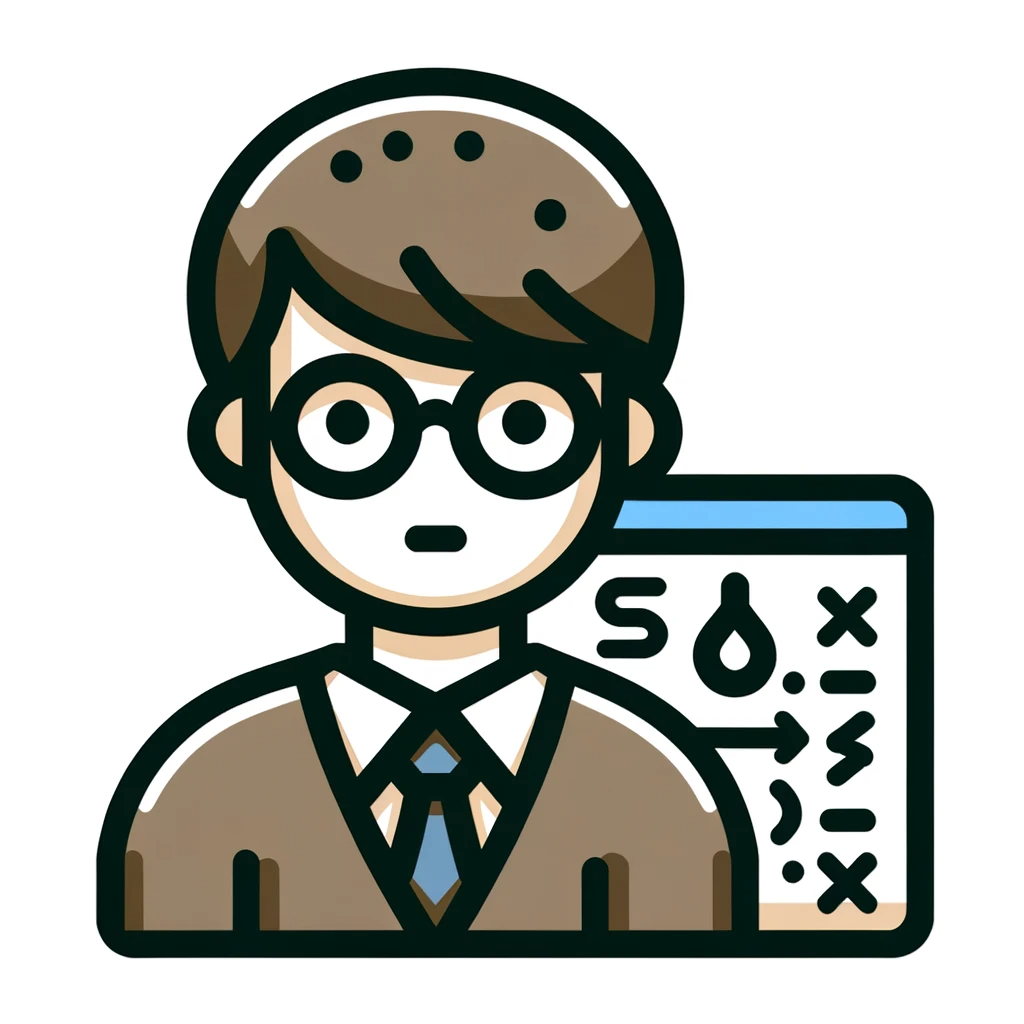
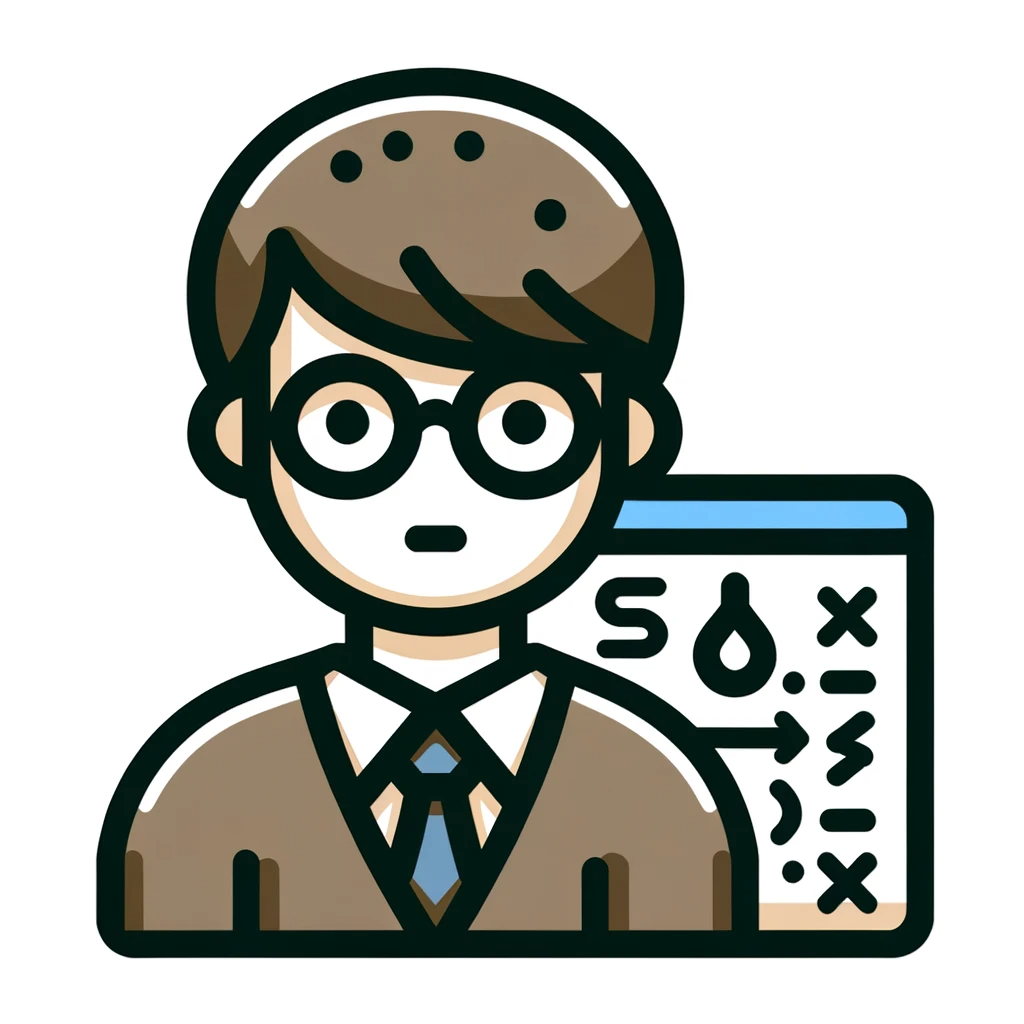
When it comes to detecting storage changes, it is important to consider browser compatibility and choose an appropriate method depending on the purpose.
Also, note that storage change monitoring is only valid within the same origin.
Comments