In this article, we will explain how to easily store large amounts of data in the browser using JavaScript and how to use Web Storage.
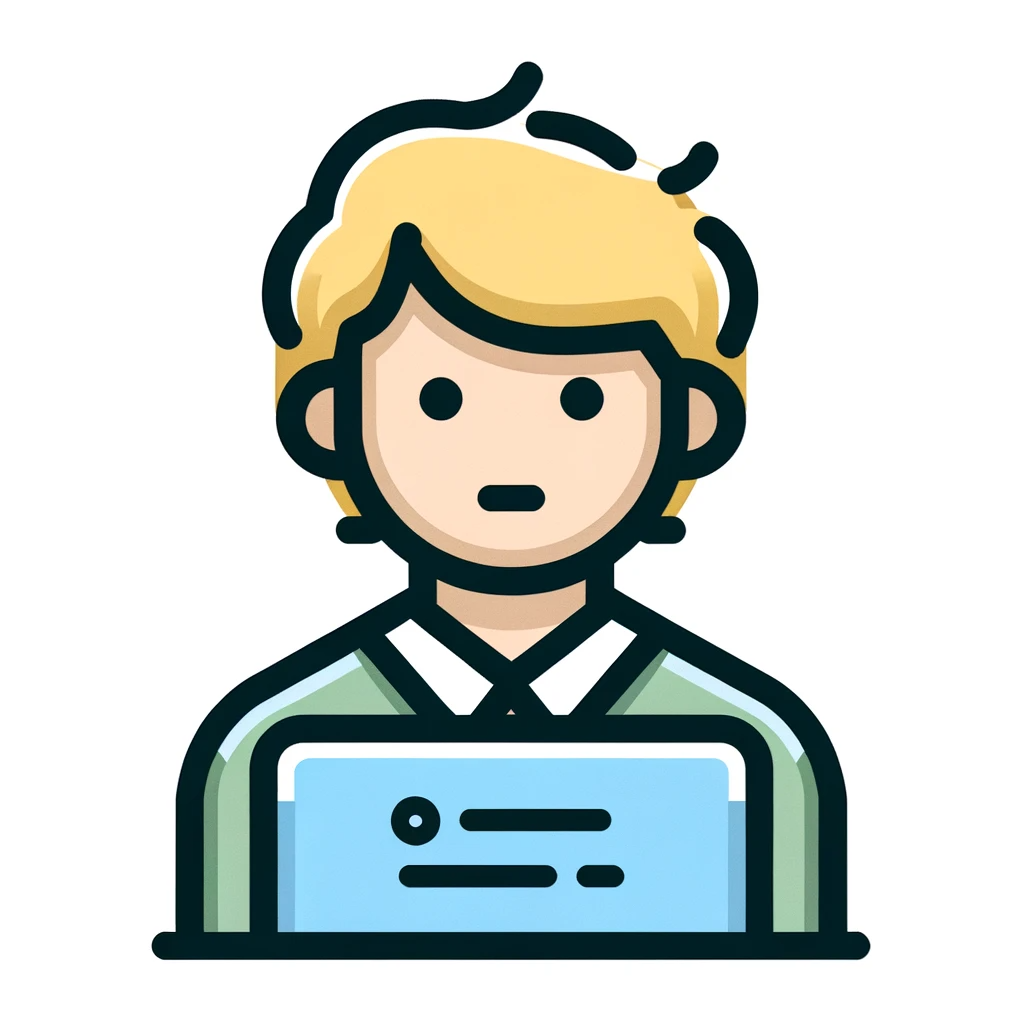
Please tell me how to save large data on the browser using Web Storage.
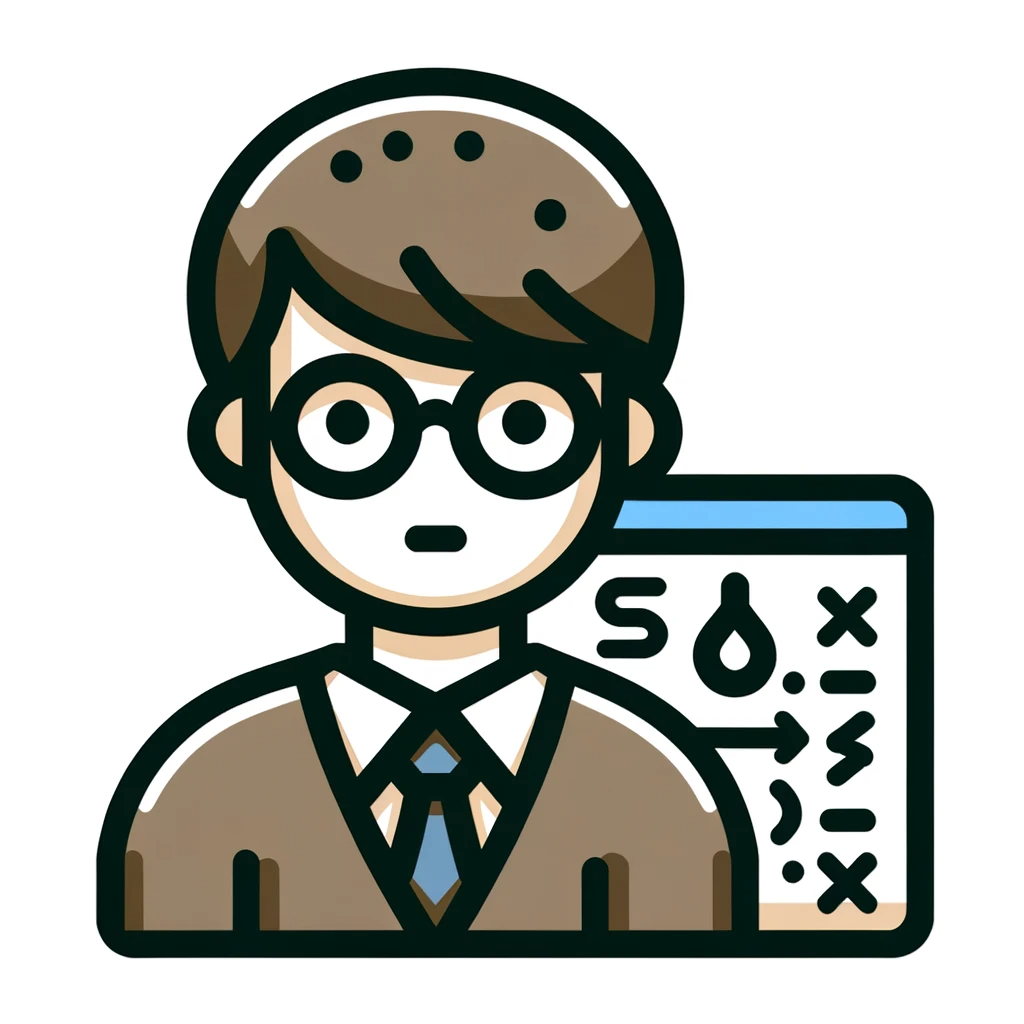
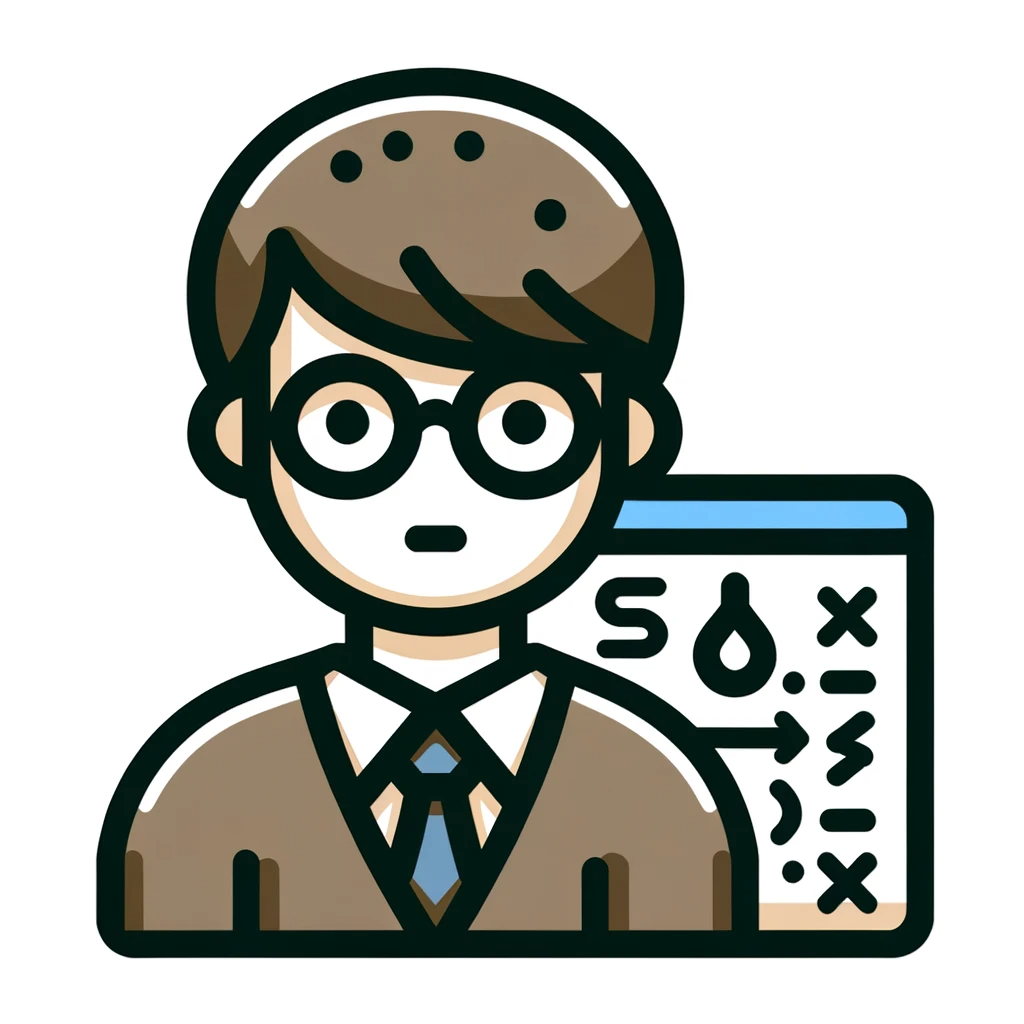
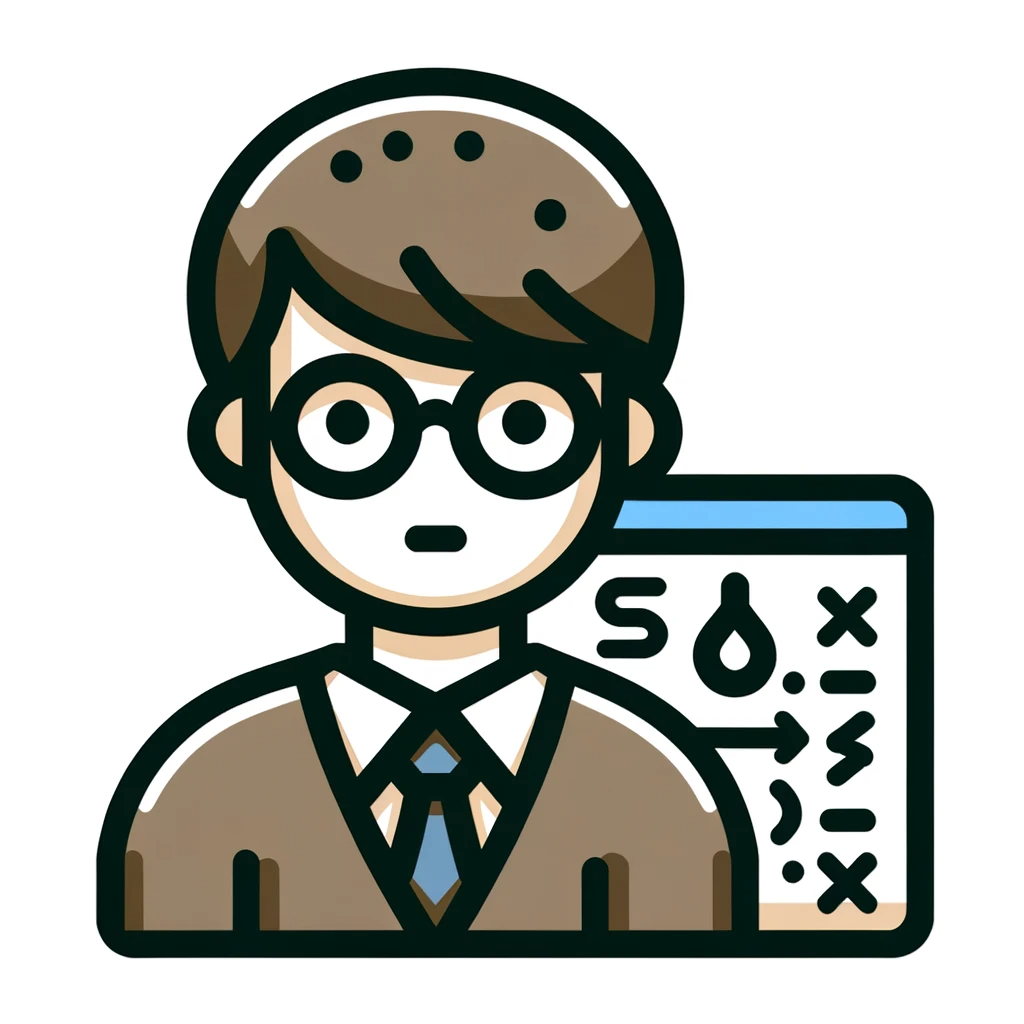
Web Storage is a technology that allows you to easily and efficiently store data on your browser.
This time, I will introduce how to use the two types of Web Storage, localStorage and sessionStorage, and introduce a sample program.
What is Web Storage?
Web Storage is an HTML5 API that provides functionality for storing data on a web browser . Web Storage can handle much larger amounts of data than cookies, and can efficiently store and retrieve data on the client side.
There are two main types of Web Storage, each used for different purposes.
- localStorage:
- This storage can store data permanently. Your data remains even if you close your browser. Suitable for data that needs to be stored for a long time, such as user settings and themes.
- sessionStorage:
- This storage can temporarily store data. The data disappears when you close your browser. Suitable for data that is valid only during the session, such as form input information.
Web Storage can be manipulated using methods such as setItem(), getItem(), and removeItem(), respectively.
Data is stored as key-value pairs and is in text format, so if you want to store numbers or objects, you need to convert them to the appropriate format.
Additionally, due to data capacity limitations, you may need to consider other storage technologies such as IndexedDB when handling large amounts of data.
How to use localStorage
localStorage is a type of Web Storage that allows you to permanently store data in your web browser . Data is stored as key-value pairs. Your data will not disappear when you close your browser and will remain accessible even when you reopen your browser.
The basic usage of localStorage is explained below.
Save data: Use the setItem() method to store key/value pairs.
localStorage.setItem('key', 'value');
Retrieve data:Use the getItem() method to retrieve the value corresponding to a key.
const value = localStorage.getItem('key');
Delete data:Use the removeItem() method to remove data corresponding to a key.
localStorage.removeItem('key');
Delete all data:Use the clear() method to delete all data stored in localStorage.
localStorage.clear();
Get the number of data:Use the length property to get the number of data stored in localStorage.
const dataCount = localStorage.length;
Get Key:Use the key() method to retrieve the key at a given index.
const keyName = localStorage.key(0);
Note that all data saved in localStorage is in string format, so if you want to save numbers or objects, you need to convert them to the appropriate format before saving them, and then convert them to the appropriate format after retrieving them. there is.
You can convert objects to strings and vice versa by using functions such as JSON.stringify() and JSON.parse().
// Convert object to string and save
const obj = {name: 'John', age: 30};
localStorage.setItem('user', JSON.stringify(obj));
// Converts a string to an object and obtains it.
const user = JSON.parse(localStorage.getItem('user'));
How to use sessionStorage
sessionStorage is a type of Web Storage that allows you to temporarily store data in your web browser . Data is stored as key-value pairs. Since the data disappears when you close the browser, it is suitable for storing data that is valid only for the duration of the session.
The basic usage of sessionStorage is explained below.
Save data: Use the setItem() method to store key/value pairs.
sessionStorage.setItem('key', 'value');
Retrieve data:Use the getItem() method to retrieve the value corresponding to a key.
const value = sessionStorage.getItem('key');
Delete data:Use the removeItem() method to remove data corresponding to a key.
sessionStorage.removeItem('key');
Delete all data: Use the clear() method to delete all data stored in sessionStorage.
sessionStorage.clear();
Get the number of data: Use the length property to get the number of data stored in sessionStorage.
const dataCount = sessionStorage.length;
Retrieving keys: Use the key() method to retrieve the key at a given index.
const keyName = sessionStorage.key(0);
Similarly, all data stored in sessionStorage is in string format, so when storing numbers or objects, they must be converted to the appropriate format before storage and after retrieval.
Functions such as JSON.stringify() and JSON.parse() can be used to convert objects to strings and from strings to objects.
// Convert object to string and save
const obj = {name: 'Jane', age: 28};
sessionStorage.setItem('user', JSON.stringify(obj));
// Converts a string to an object and obtains it.
const user = JSON.parse(sessionStorage.getItem('user'));
Explanation using a sample program
The sample program below shows basic usage of localStorage and sessionStorage.
// Store data in localStorage
localStorage.setItem('username', 'Alice');
// Get data from localStorage
const username = localStorage.getItem('username');
console.log('localStorage username:', username);
// Store data in sessionStorage
sessionStorage.setItem('temporary', 'Temp data');
// Get data from sessionStorage
const tempData = sessionStorage.getItem('temporary');
console.log('sessionStorage temporary:', tempData);
Explanation
By running this sample program, you can understand how to use localStorage and sessionStorage and verify that you can save and retrieve data.
- Save data to localStorage:
- The setItem() method is used to store the key ‘username’ and value ‘Alice’ pairs in localStorage.
- Get data from localStorage:
- The getItem() method is used to retrieve the value corresponding to the key ‘username’ and store it in the variable username. Then console.log() outputs the retrieved value.
- Save data to sessionStorage:
- The setItem() method is used to store the key ‘temporary’ and value ‘Temp data’ pairs in sessionStorage.
- Get data from sessionStorage:
- The getItem() method is used to retrieve the value corresponding to the key ‘temporary’ and store it in the variable tempData. Then console.log() outputs the retrieved value.
In actual applications, more complex data structures and error handling should be considered. Also, keep in mind that data is stored in string format, so be sure to perform appropriate data conversion.
summary
We explained how to easily save large data on a browser and how to use Web Storage.
- Web Storage is an HTML5 API that provides the ability to save data on a web browser.
- localStorage permanently stores data and does not disappear even when the browser is closed.
- sessionStorage temporarily stores data and disappears when the browser is closed.
- Data can be manipulated using methods such as setItem(), getItem(), and removeItem().
- Since the data is stored in string format, it must be converted to the appropriate format using JSON.stringify() or JSON.parse().
- For security reasons, avoid storing confidential information.
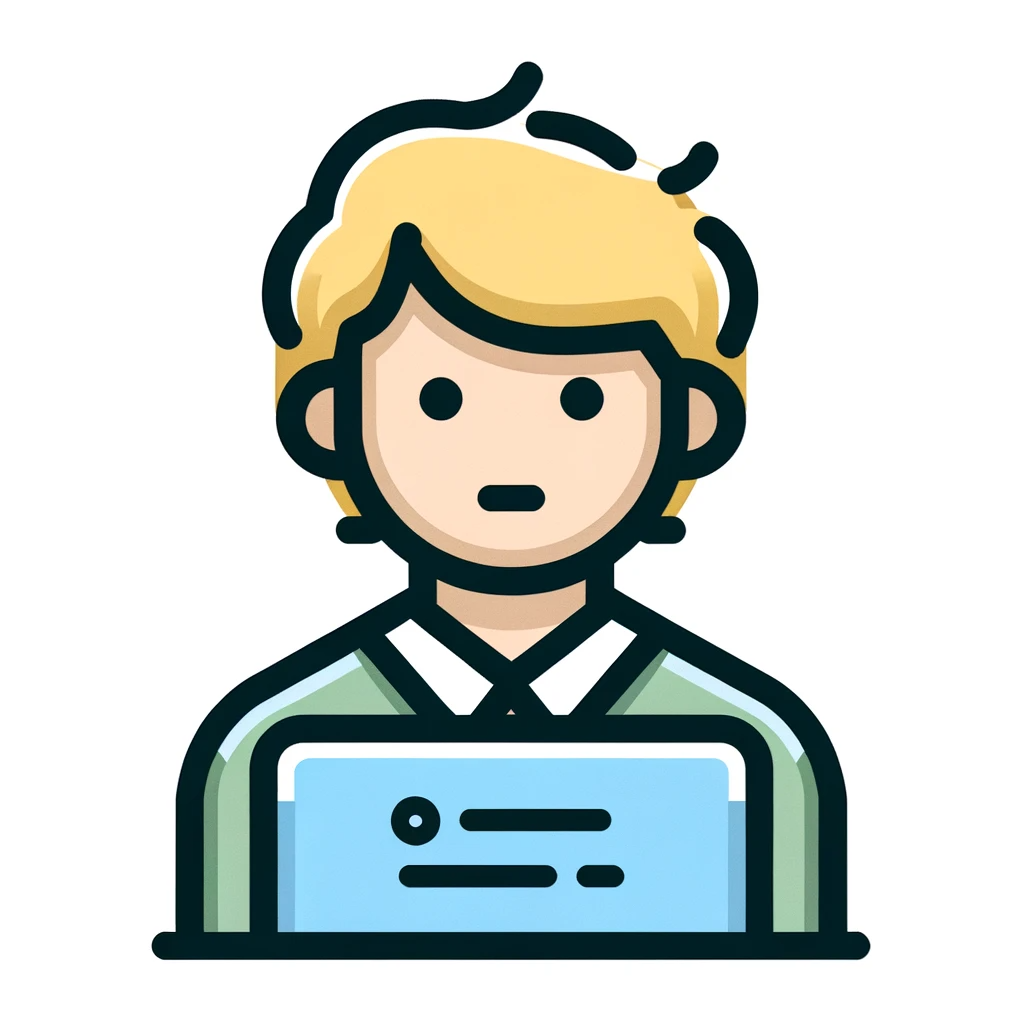
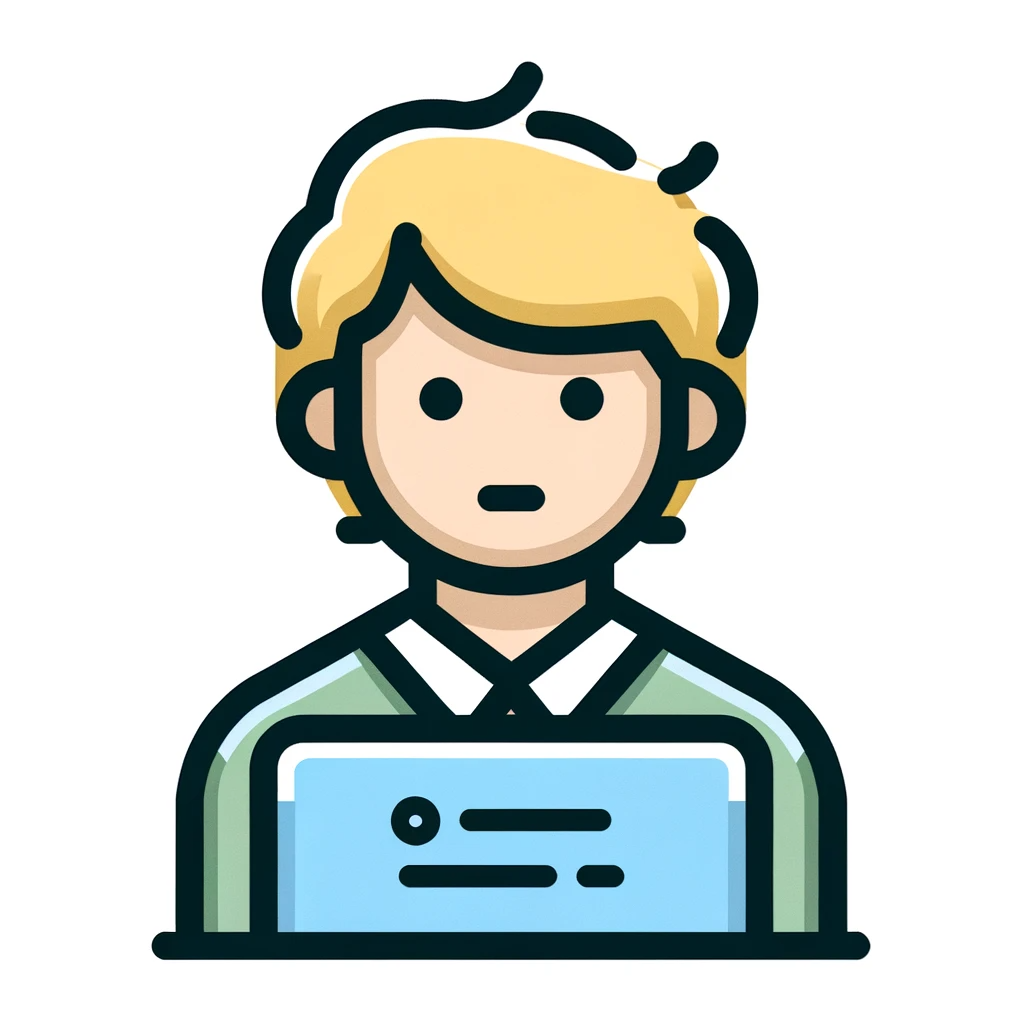
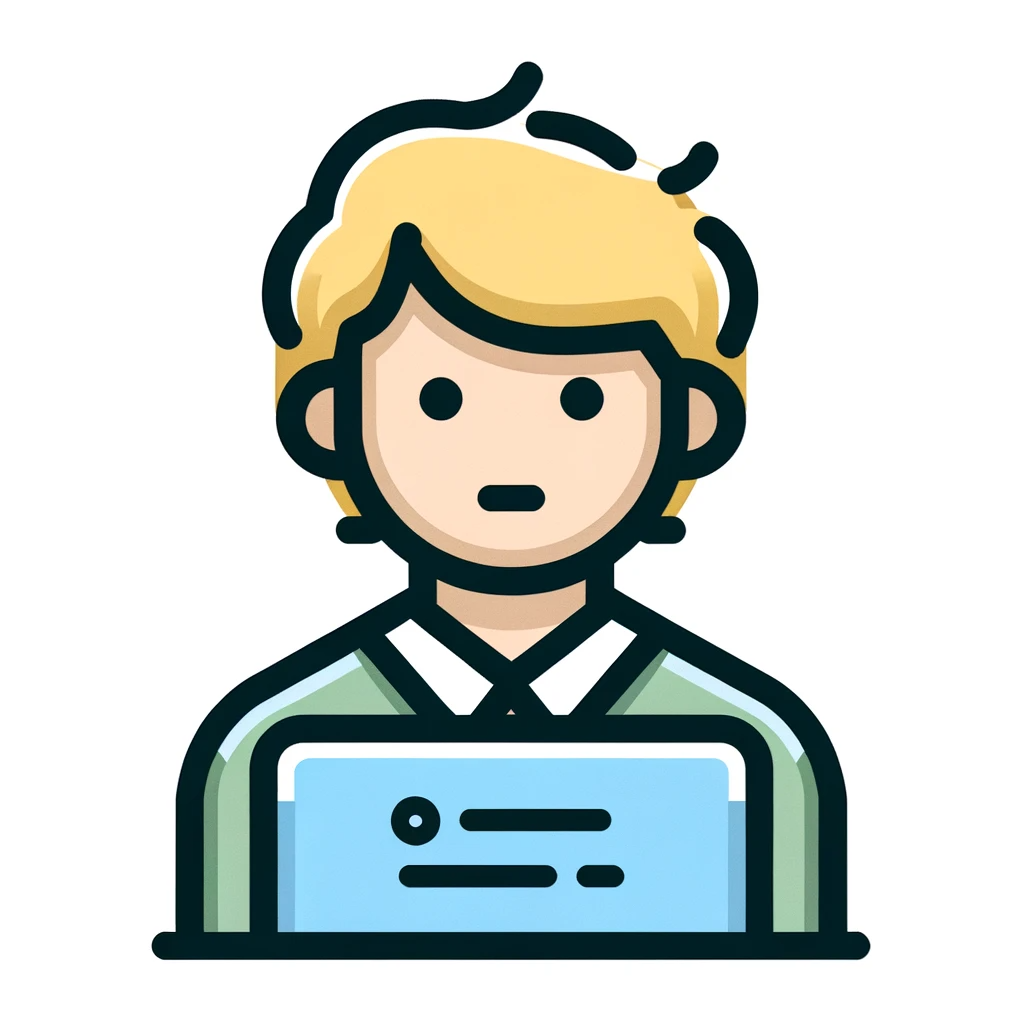
It was easy to understand how to use Web Storage.
You now have a good understanding of the difference between localStorage and sessionStorage and how to actually manipulate data.
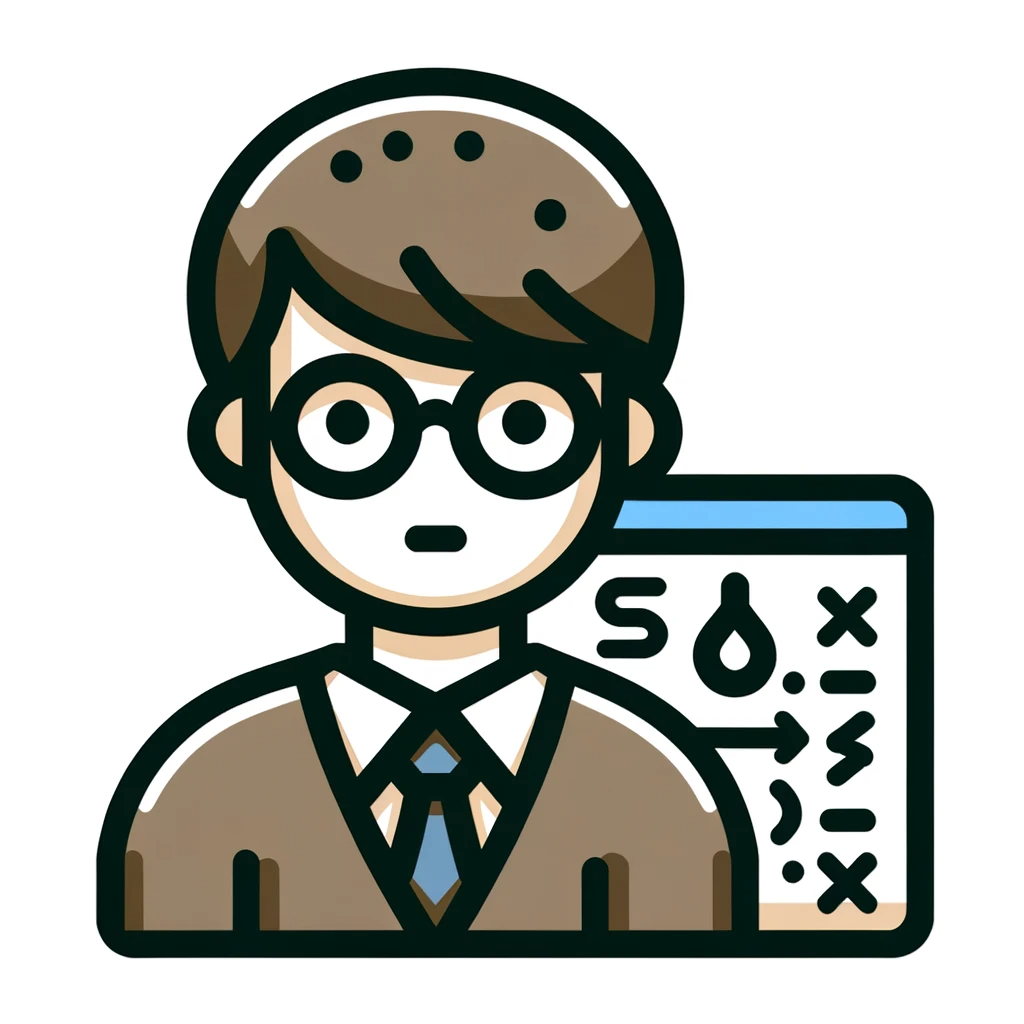
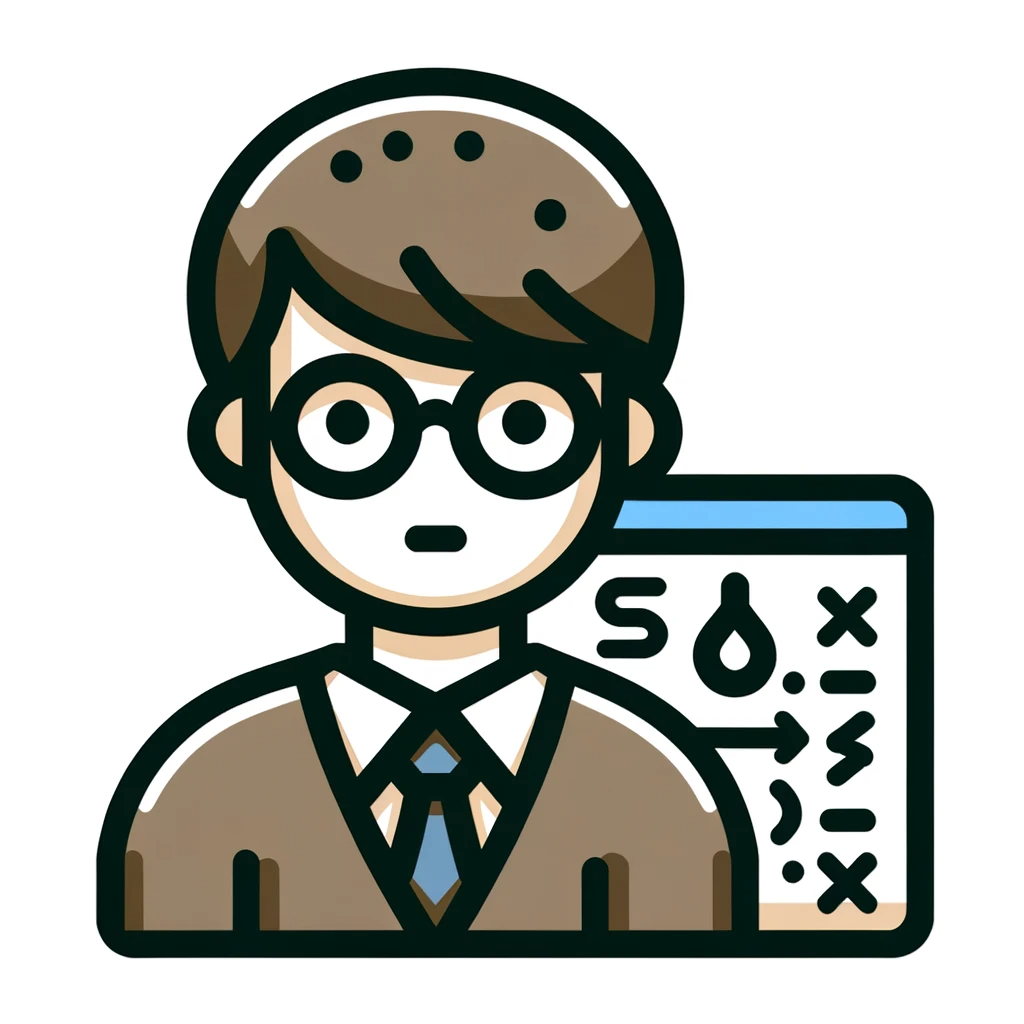
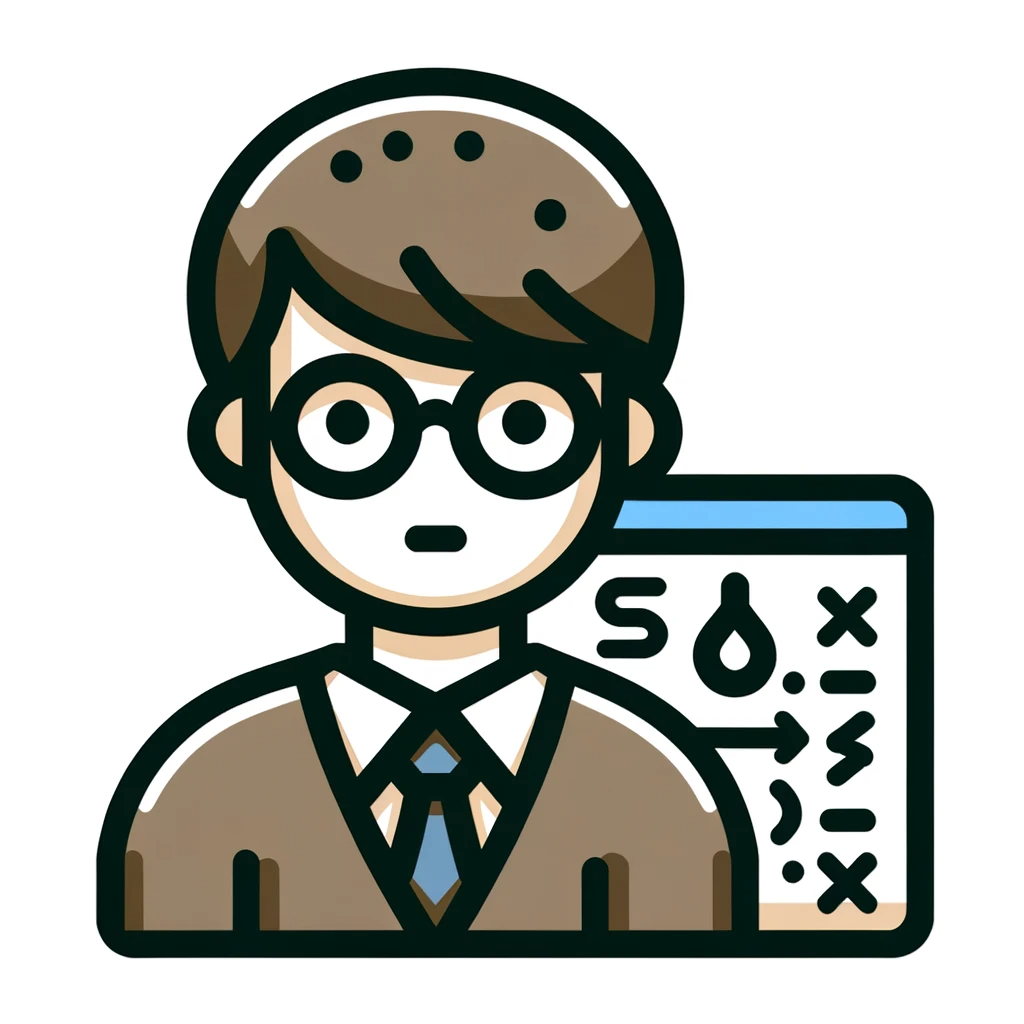
You can now efficiently save and retrieve data using Web Storage.
It is important to use localStorage and sessionStorage differently depending on the application requirements.
Also, pay attention to data storage formats and security aspects to ensure safe and effective data management.
Comments