This article explains how to use JavaScript to periodically acquire user location information and display it in real time.
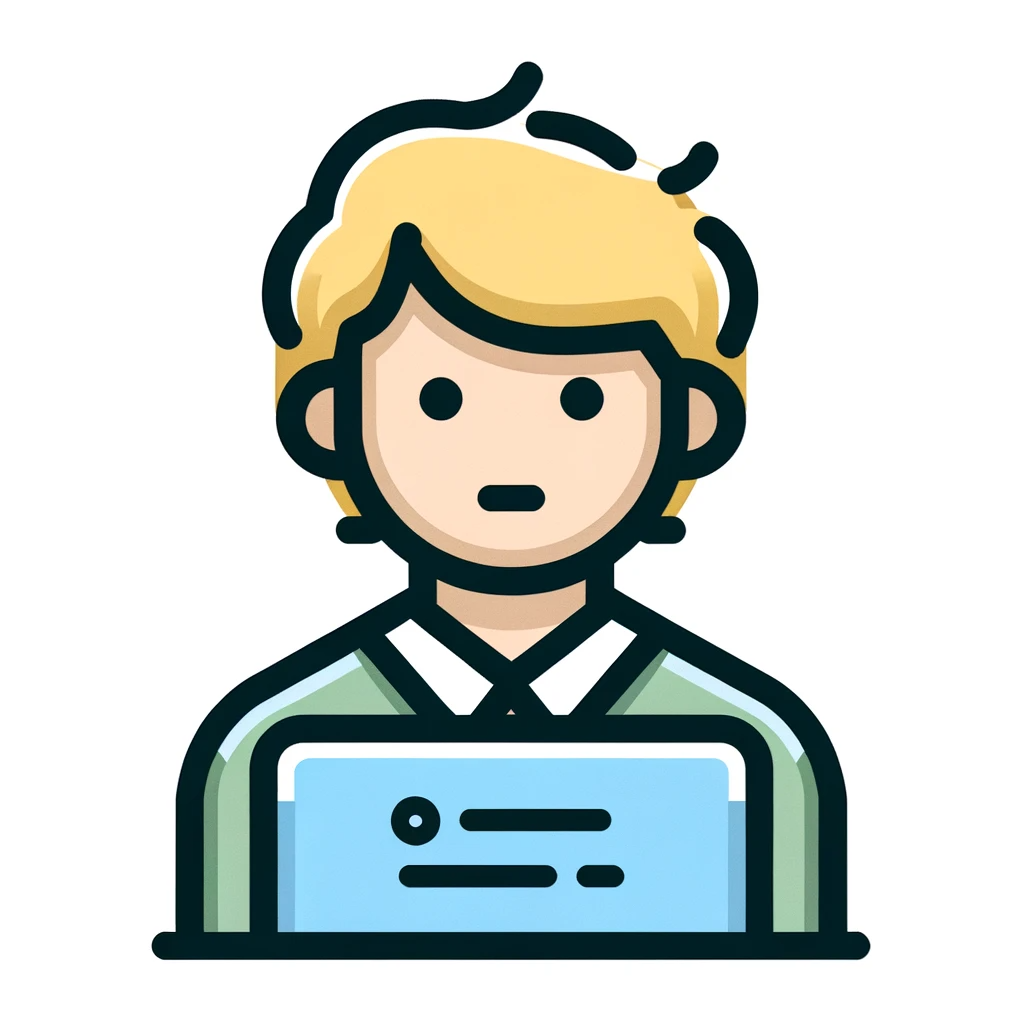
How can I obtain location information periodically?
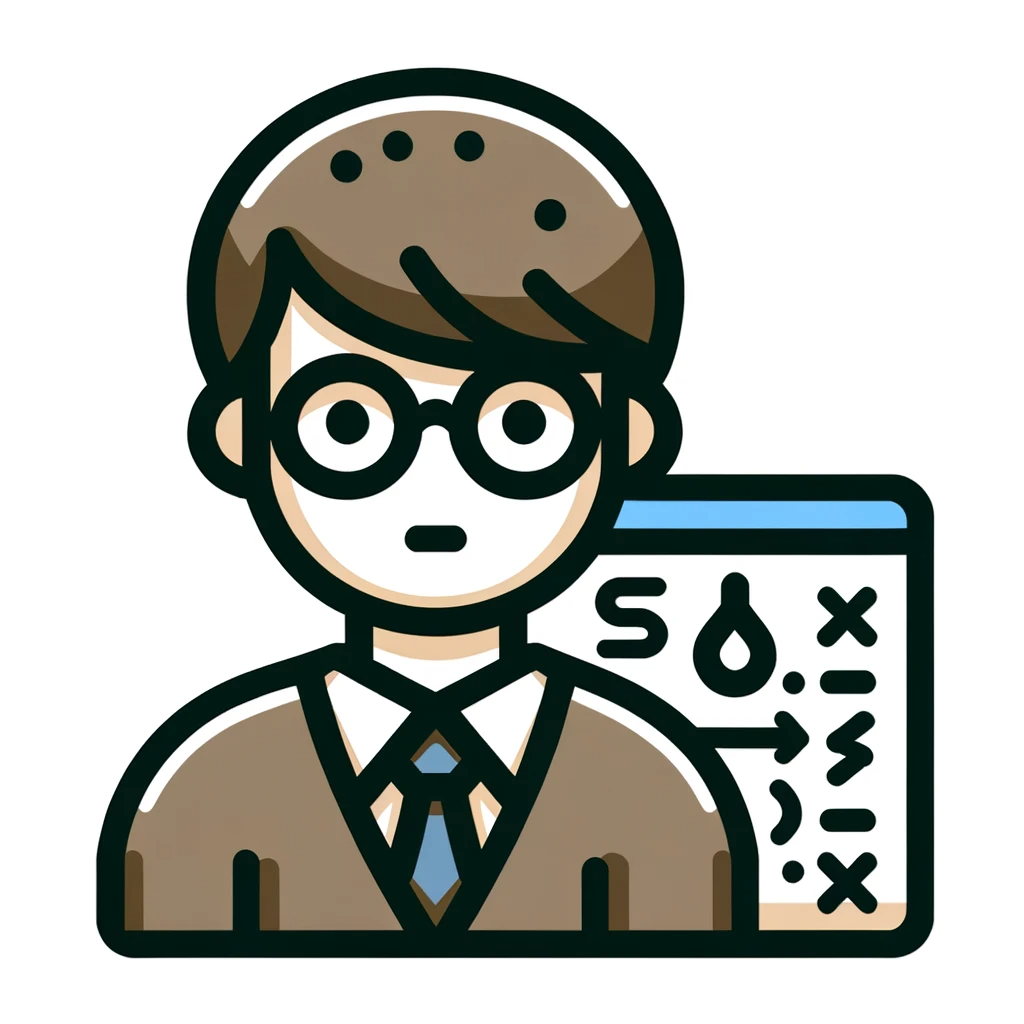
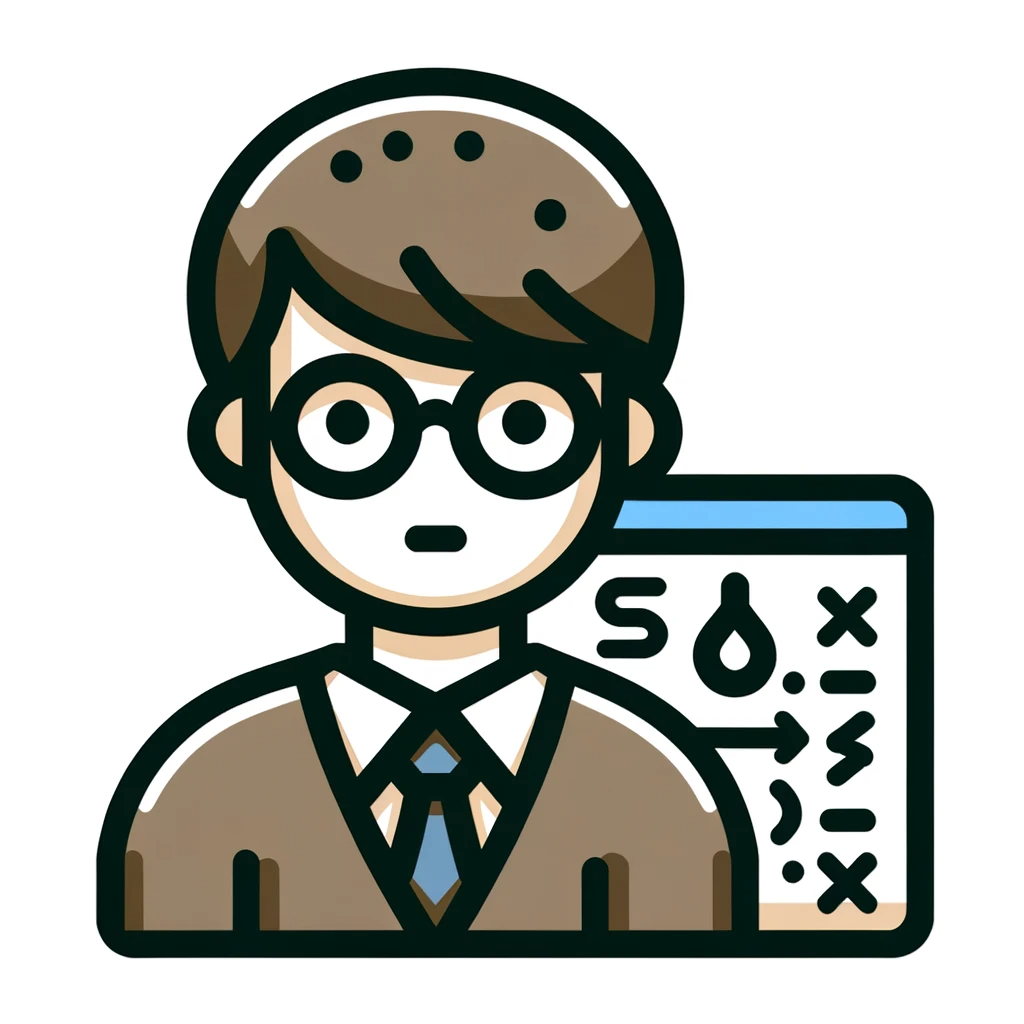
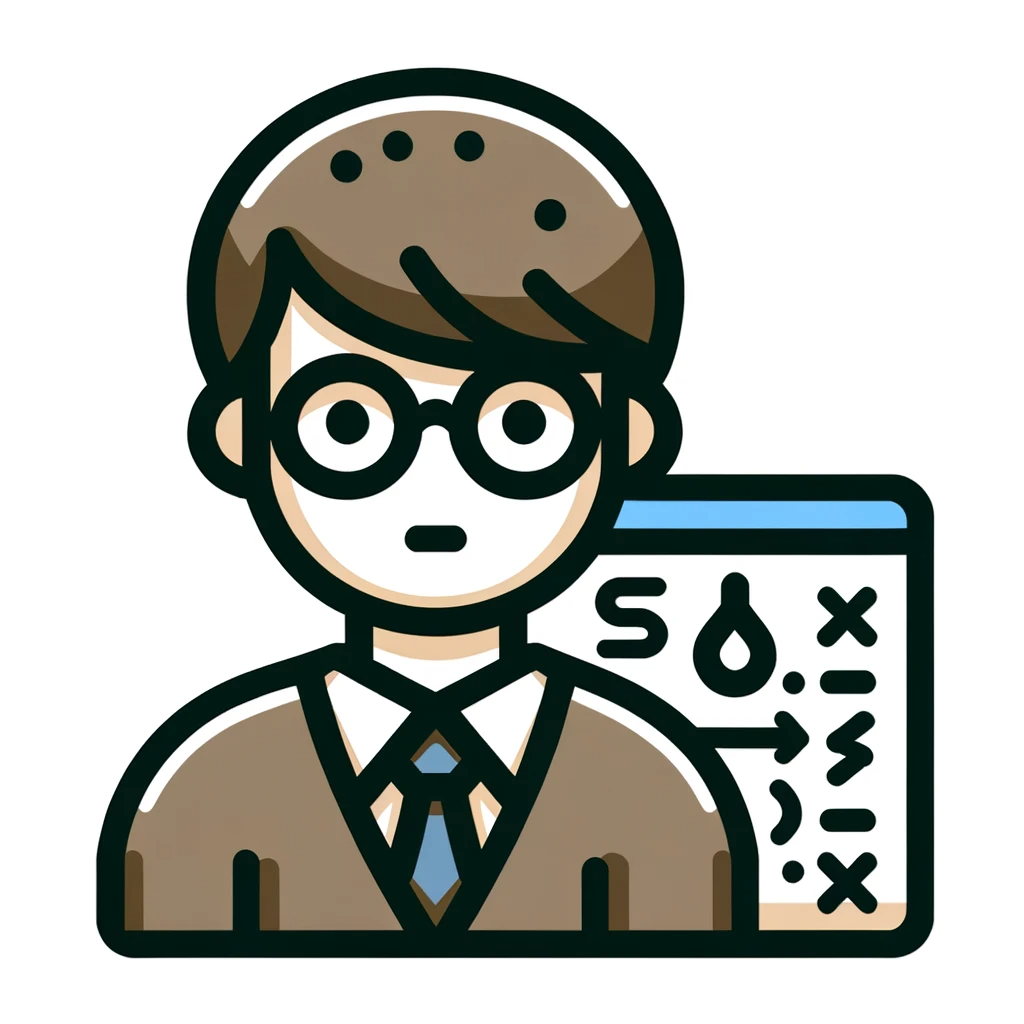
To periodically obtain position information, use the watchPosition() method. watchPosition() method periodically obtains position information, not just once.
How to obtain location information
Location information can be obtained by using JavaScript’s navigator.geolocation object. navigator.geolocation object provides an API that provides information about location information.
To obtain location information, use the getCurrentPosition() method. On success, this method returns a Position object containing the location information to the function passed as the callback function. On failure, it returns a PositionError object containing error information.
The following is sample code that uses the getCurrentPosition() method to obtain position information.
navigator.geolocation.getCurrentPosition(function(position) {
console.log(position.coords.latitude);
console.log(position.coords.longitude);
});
In this sample code, the getCurrentPosition() method is passed a callback function that receives a Position object containing position information. Within the callback function, the latitude and longitude are output to the console using the coords.latitude and coords.longitude properties.
The getCurrentPosition() method can also optionally specify a timeout period for getting position information and a minimum precision for getting position information.
How to periodically obtain location information
To periodically retrieve position information, use the watchPosition() method, which, like the getCurrentPosition() method, provides an API for providing position information, but it does so periodically rather than once. However, it does not acquire position information only once, but periodically.
The watchPosition() method calls the specified callback function each time the position information is updated. The callback function is also called when the position information is changed.
The following is a sample code that uses the watchPosition() method to periodically obtain position information.
var options = {
enableHighAccuracy: true,
timeout: 5000,
maximumAge: 0
};
var watchId = navigator.geolocation.watchPosition(successCallback, errorCallback, options);
function successCallback(position) {
console.log(position.coords.latitude);
console.log(position.coords.longitude);
}
function errorCallback(error) {
console.log(error);
}
In this sample code, enableHighAccuracy is set to true as an option for the watchPosition() method to obtain location information with high accuracy. Also, timeout time is set to timeout to acquire position information. maximumAge is the expiration time of the cached position information.
The watchPosition() method returns an ID named watchId when it starts to acquire location information. This ID can also be used to abort the acquisition of location information using the clearWatch() method.
summary
We explained how to periodically acquire user location information and display it in real time.
- You can use JavaScript’s watchPosition() method to periodically obtain location information.
- The watchPosition() method calls the specified callback function every time the position information is updated, and also calls the callback function when the position information changes.
- As options for the watchPosition() method, you can set the accuracy of location information, timeout period, expiration date for cached location information, etc.
- When the watchPosition() method starts acquiring location information, it returns an ID called watchId, which can be used to stop acquiring location information.
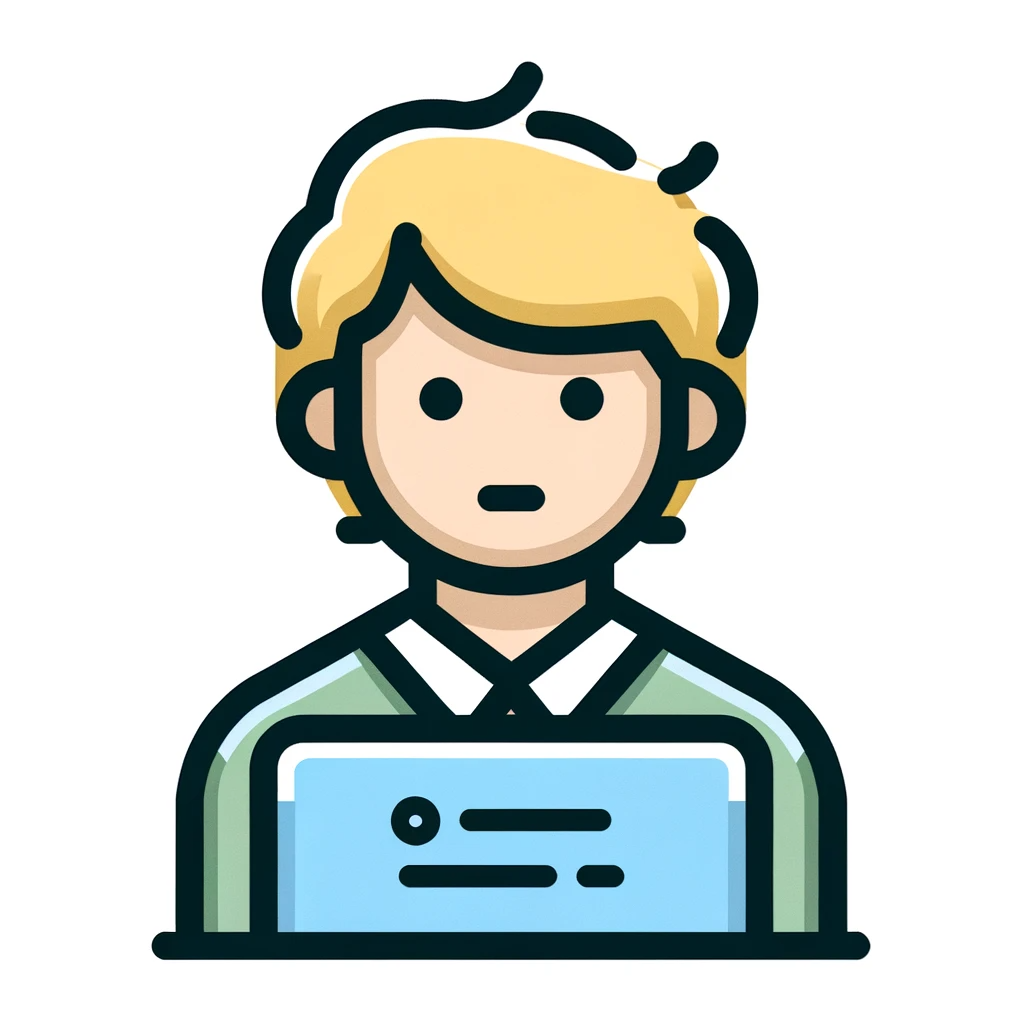
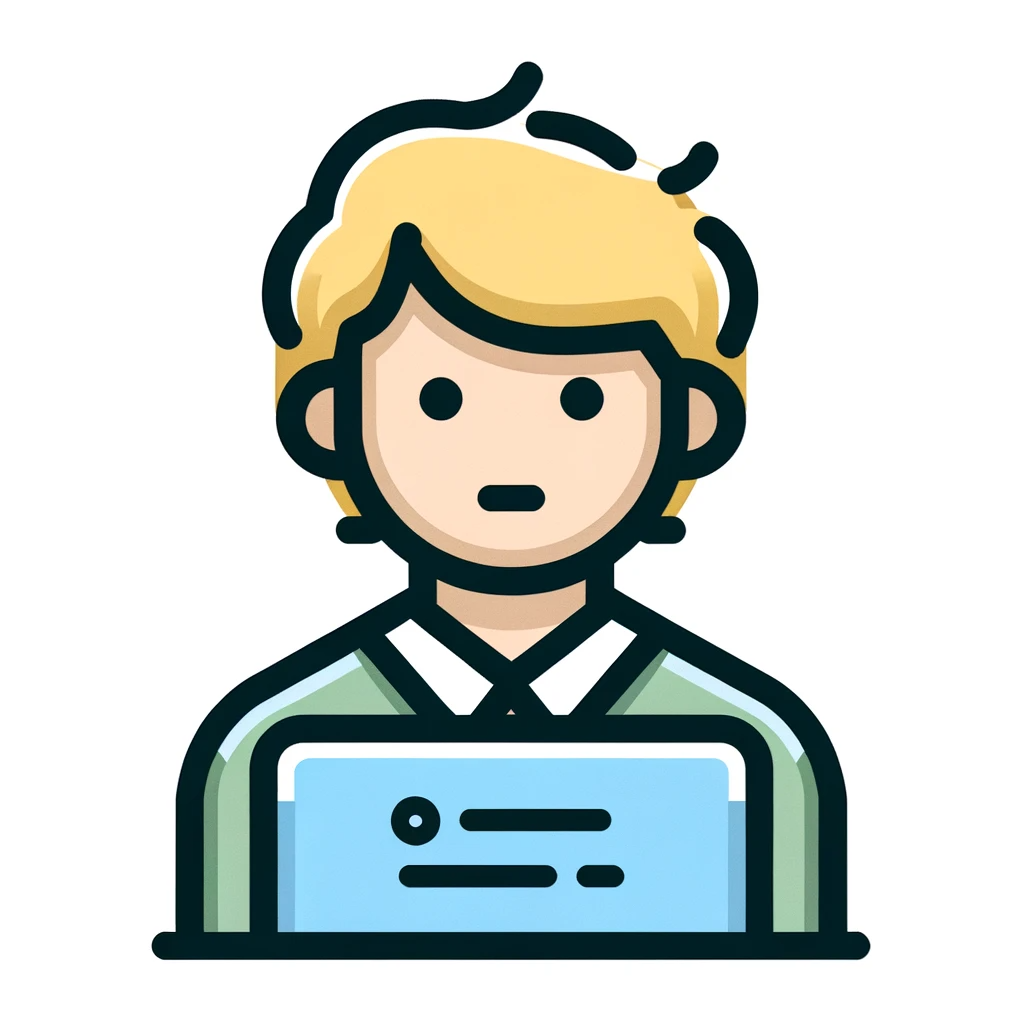
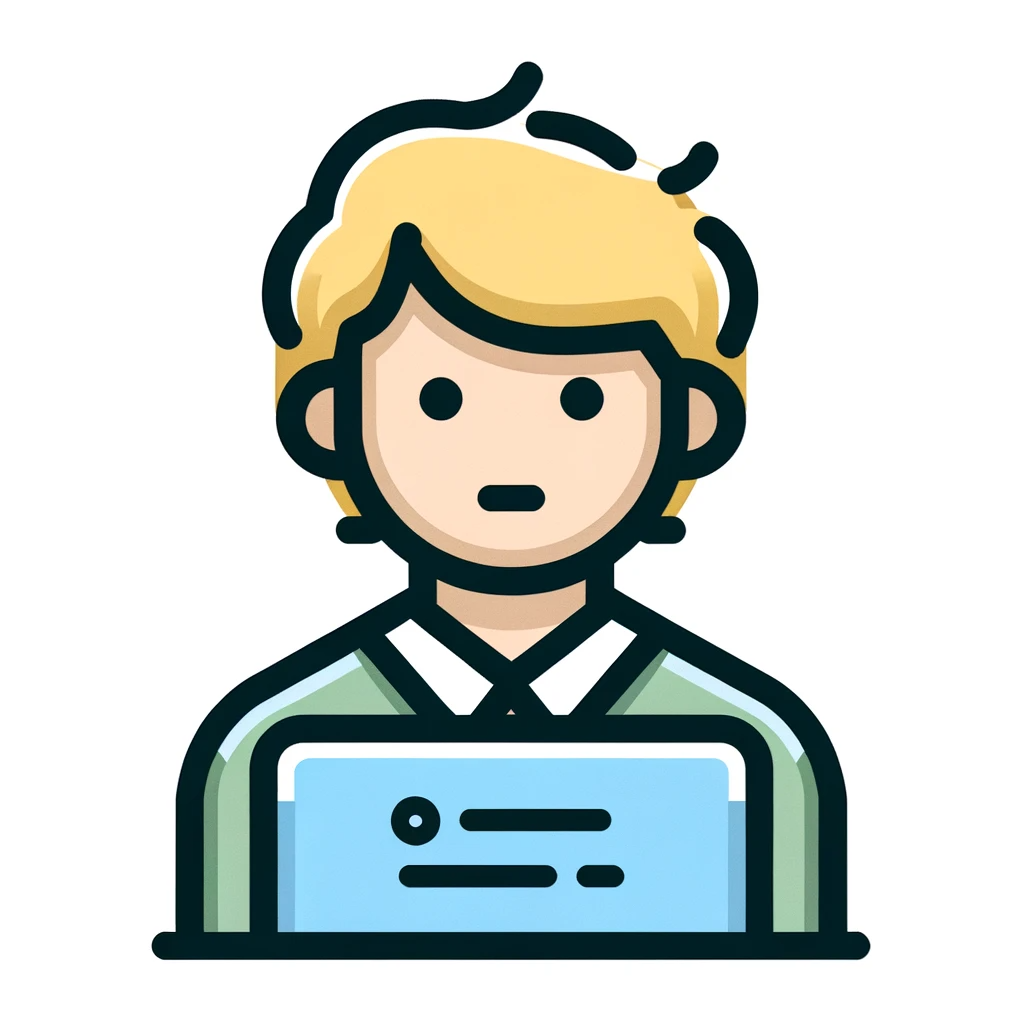
I now have a good understanding of how to handle location information in JavaScript.
I would like to apply this method to develop a service that uses location information.
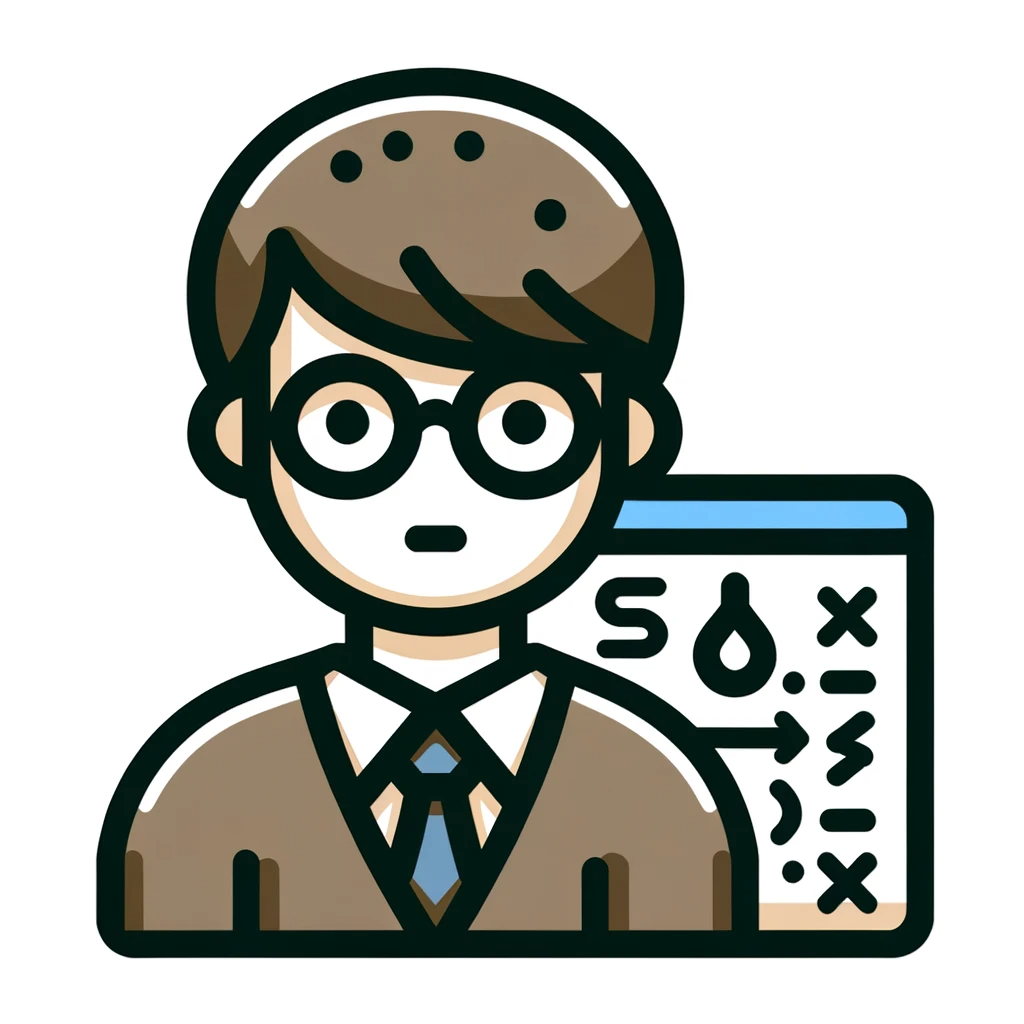
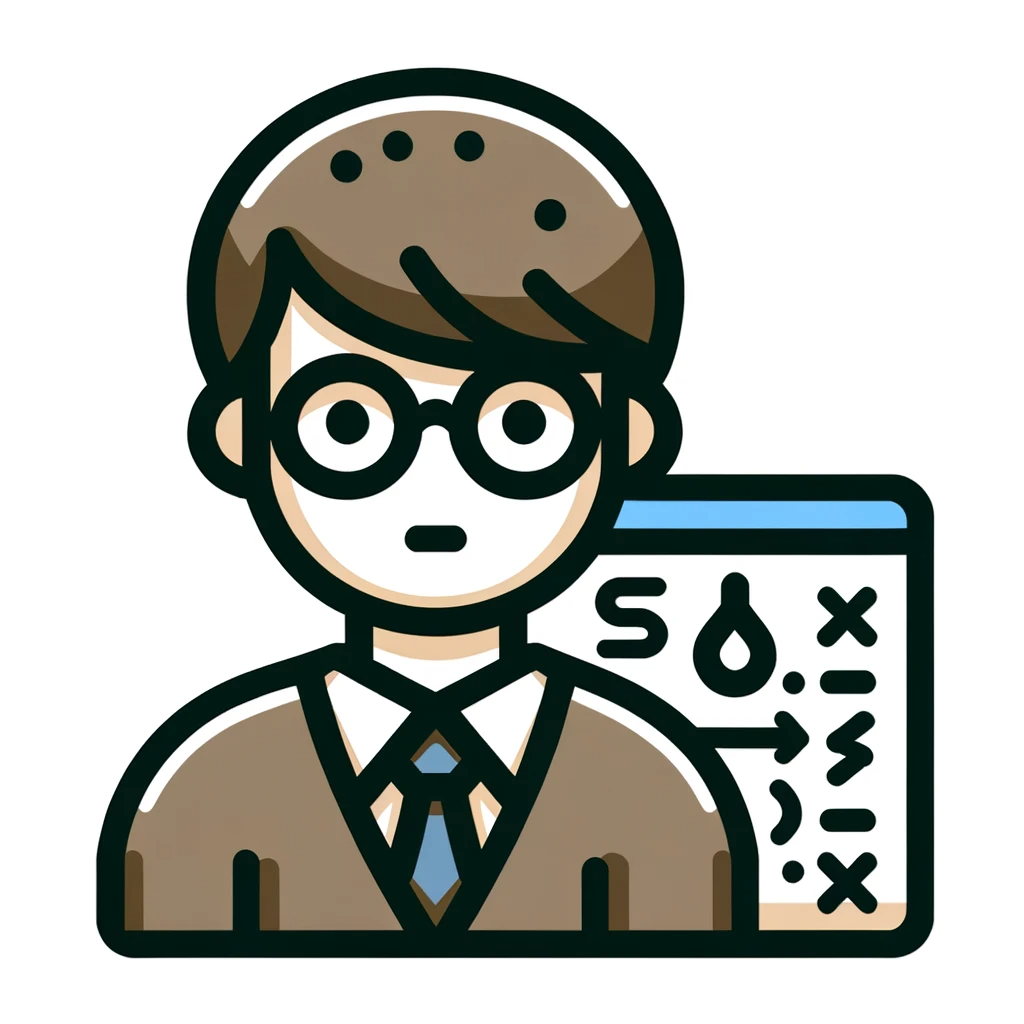
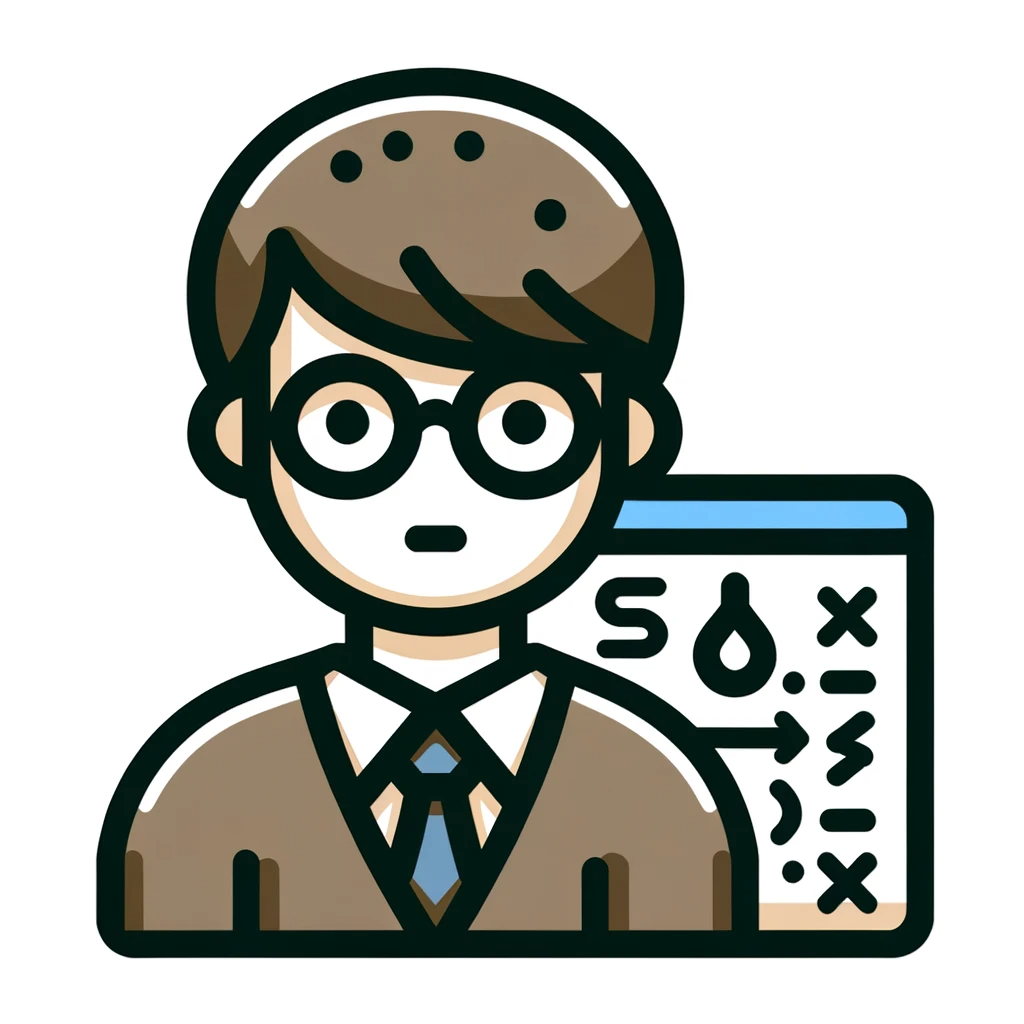
Using JavaScript, you can periodically retrieve location information using the watchPosition() method.
This is very useful for developing map apps and location-based services.
However, you need to be careful as obtaining location information requires the user’s permission and there are privacy issues.
It is important to ask users for permission and implement with appropriate privacy considerations.
Comments