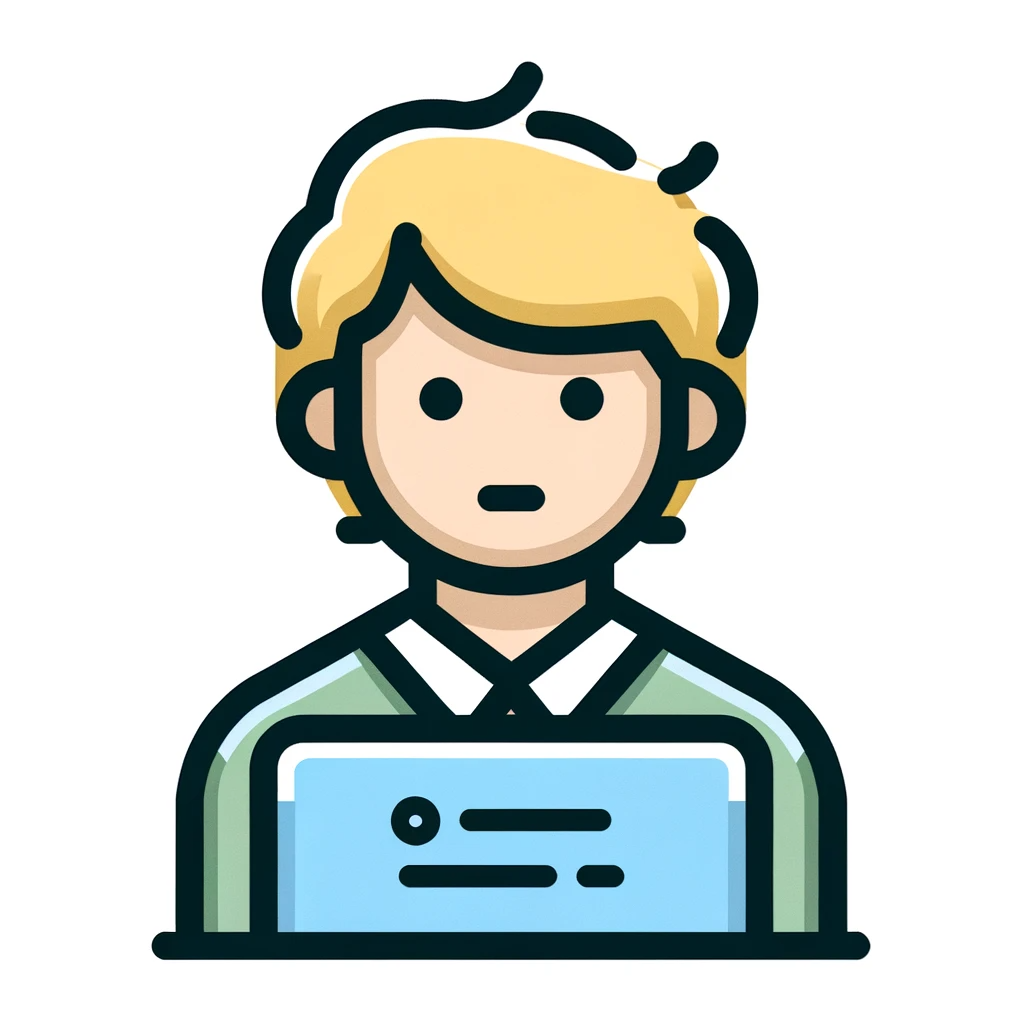
Please tell me how to determine the data type of a variable in JavaScript.
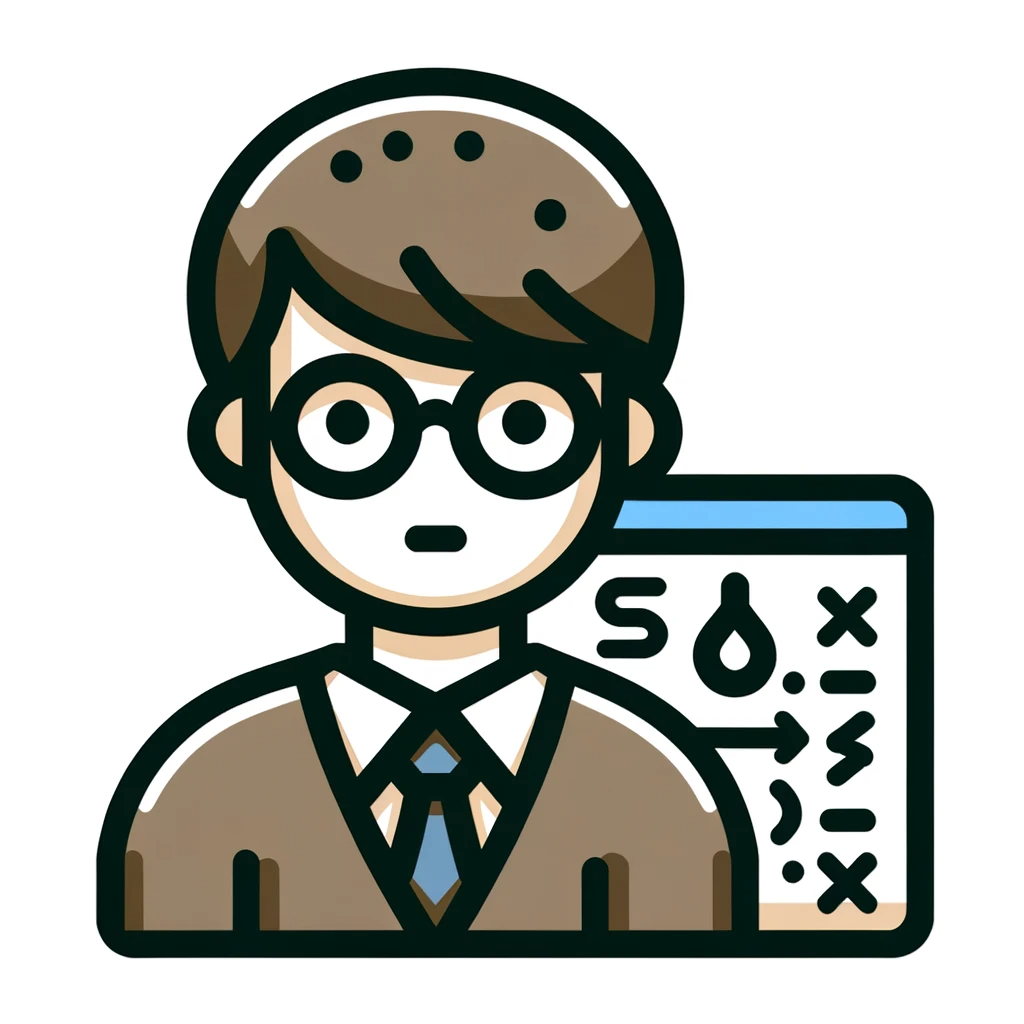
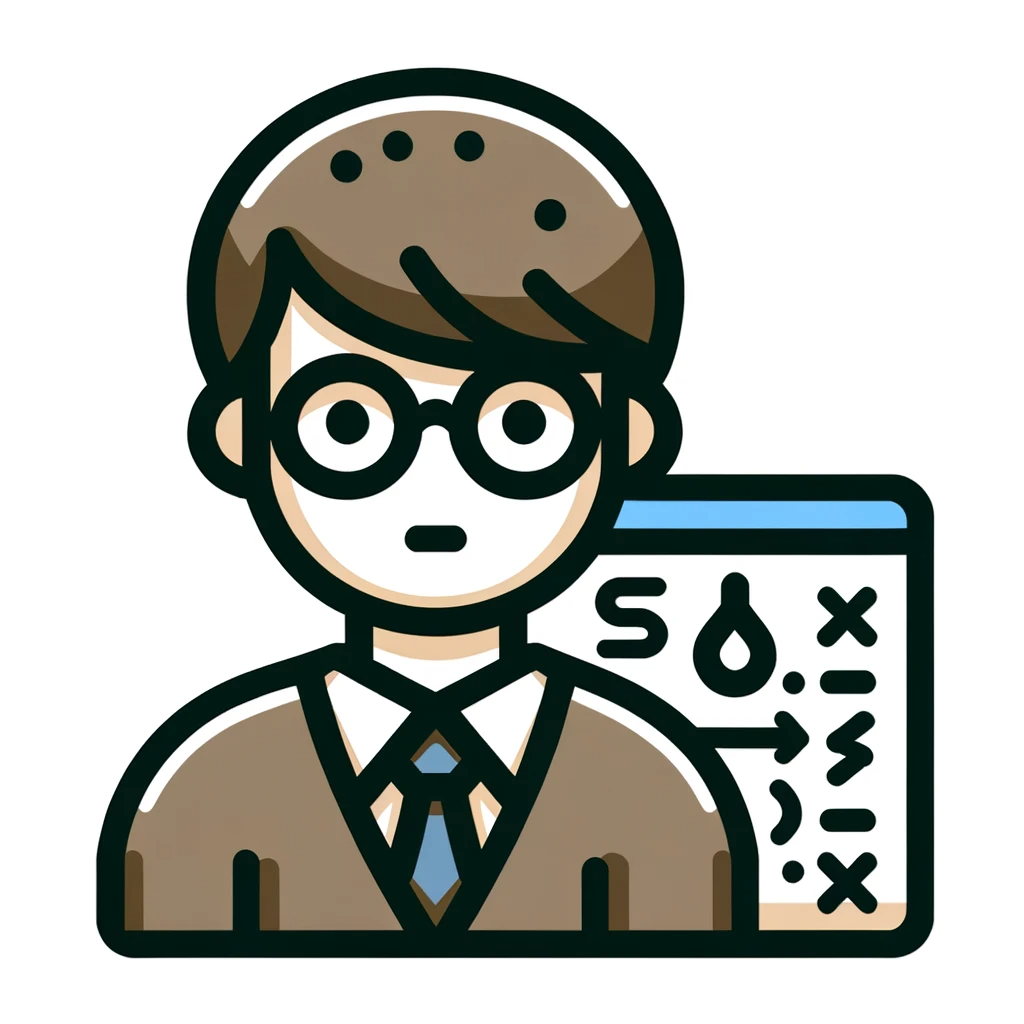
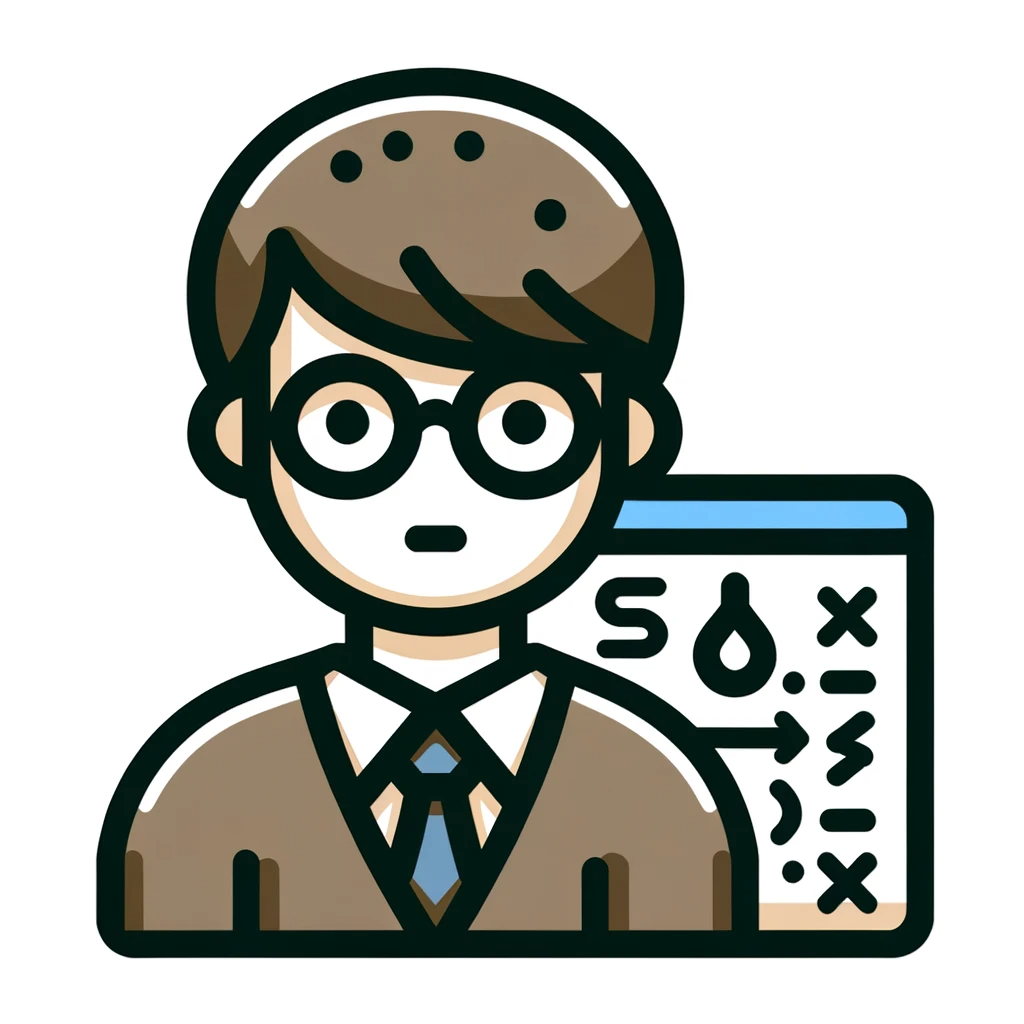
typeof
Use operators
to determine the data type of a variable .
You can also isInteger
isNaN
isArray
make judgments tailored to your needs by using etc.
How to determine data type using typeof operator
typeof
The operator returns a string indicating what data type the variable is.
typeof variable;
The usage is as follows.
let variable = 'hello';
console.log(typeof variable); // 'string'
typeof
Operators can determine data types such as:
string
: string typenumber
: Numeric typeboolean
: Boolean typefunction
: Functional typeobject
: object typeundefined
: undefined type
typeof
Be careful when using operators to judge arrays, dates, regular expressions, null, undefined, etc. as they will be returned as object type.
Determining data type with “isInteger”, “isNaN”, and “isArray”
isInteger(value)
: Method to determine whether it is an integer .
Returns true if it is an integer, false otherwise.
isNaN(value)
: A global function that determines whether it is a number .
Returns false if it is a number, true otherwise.
Array.isArray(value)
: Method to determine whether it is an array .
Returns true if it is an array, false otherwise.
How to use each is as follows.
console.log(Number.isInteger(10)); // true
console.log(Number.isInteger(10.5)); // false
console.log(isNaN(10)); // false
console.log(isNaN('hello')); // true
console.log(Array.isArray([1, 2, 3])); // true
console.log(Array.isArray({})); // false
Summary of “How to determine the data type of a variable”
” How to determine the data type of a variable ” is summarized below.
typeof
Operators can be used to determine data types: string, numeric, boolean, function, object, and undefined.isInteger
returns true if it is an integer, false otherwiseisNaN
returns false if it is a number, true otherwise.isArray
returns true if it is an array, false otherwise.
By accurately determining the data type, you can avoid program errors, so be sure to use it with this in mind.
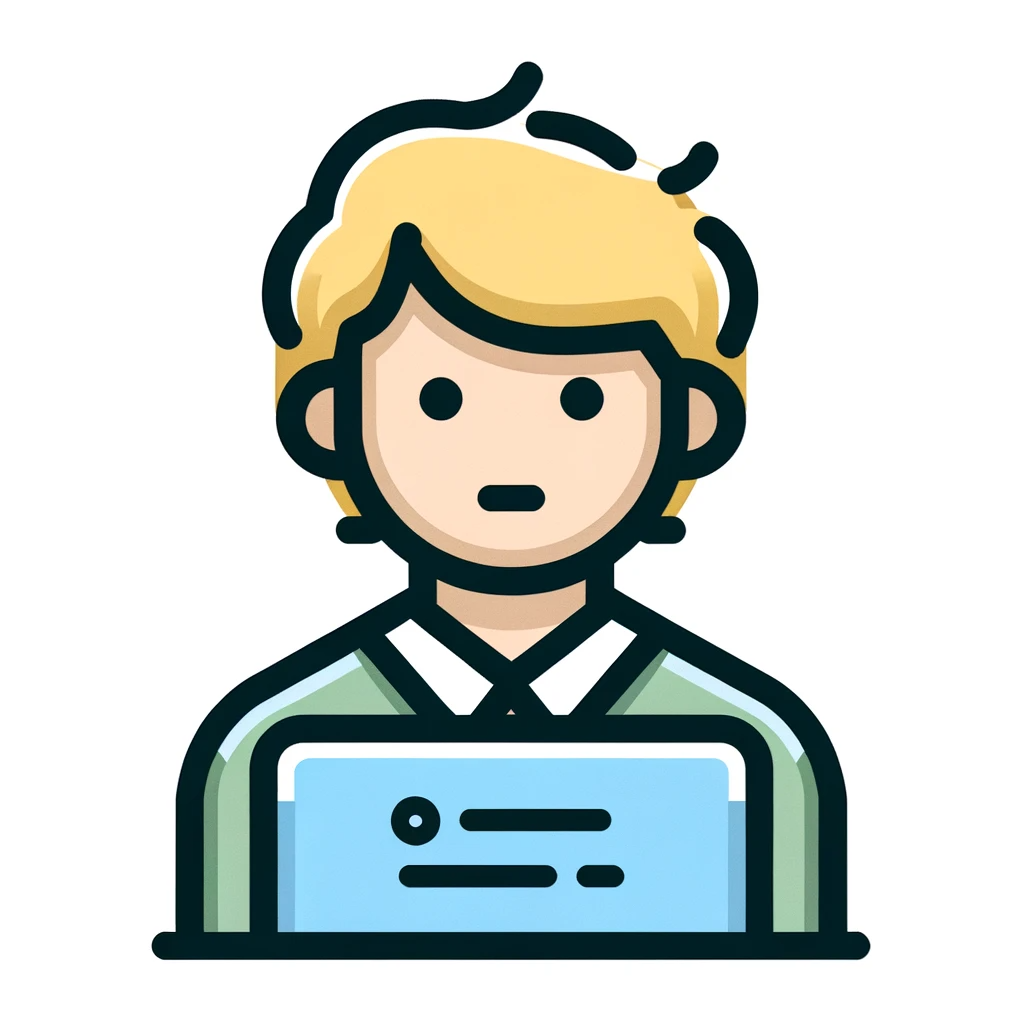
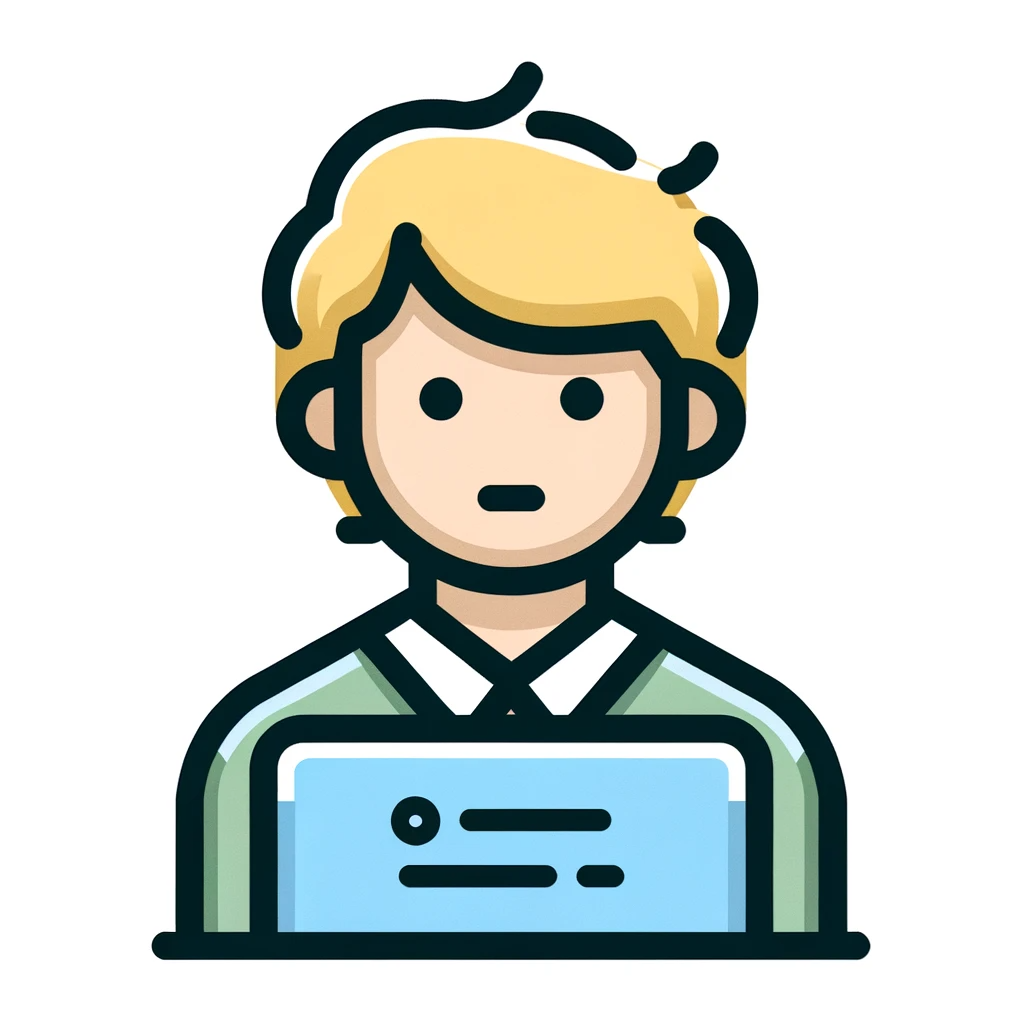
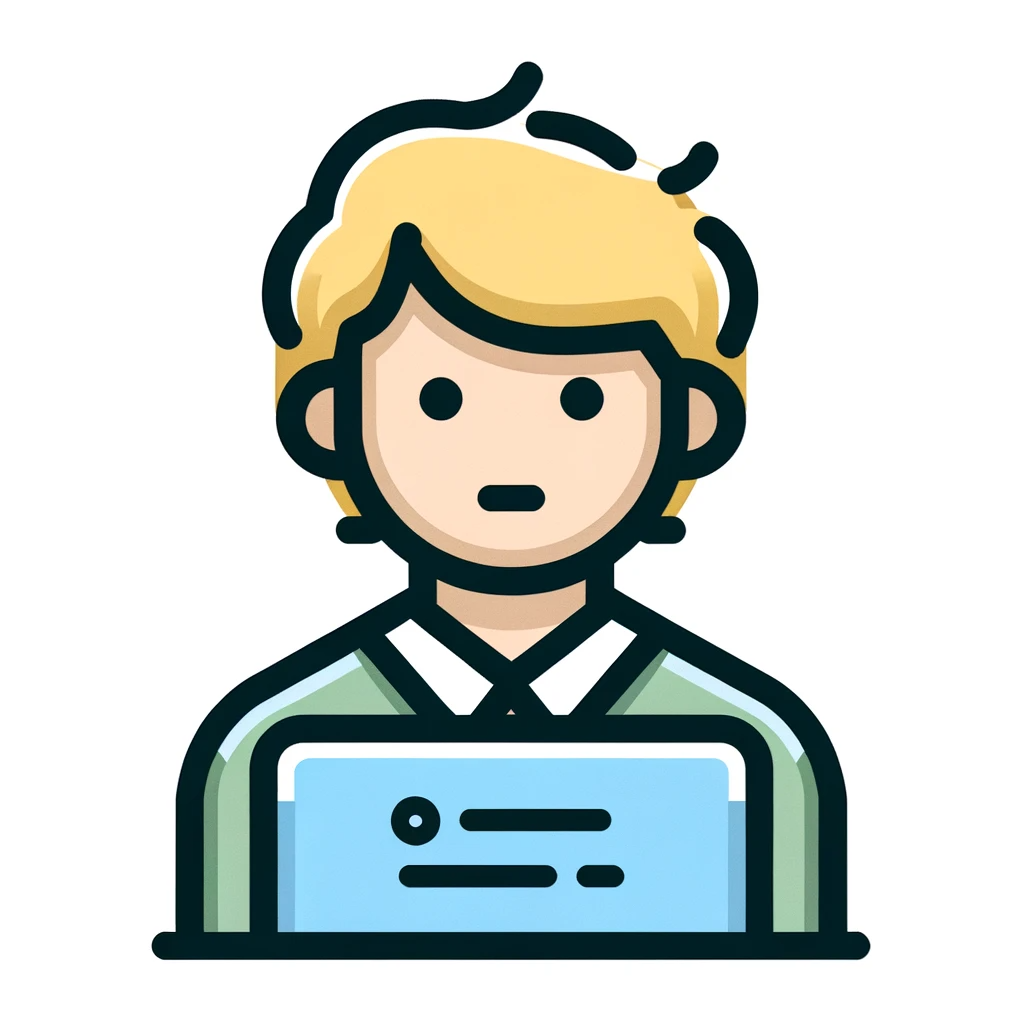
The typeof operator seems easy to use.
Also, isInteger, isNaN, and isArray seem useful.
In particular, isNaN is useful because you can use it like isNaN(parseInt()) to determine whether a string becomes NaN when converting it to a number.
Comments