Explains how to convert a string to uppercase or lowercase in JavaScript.
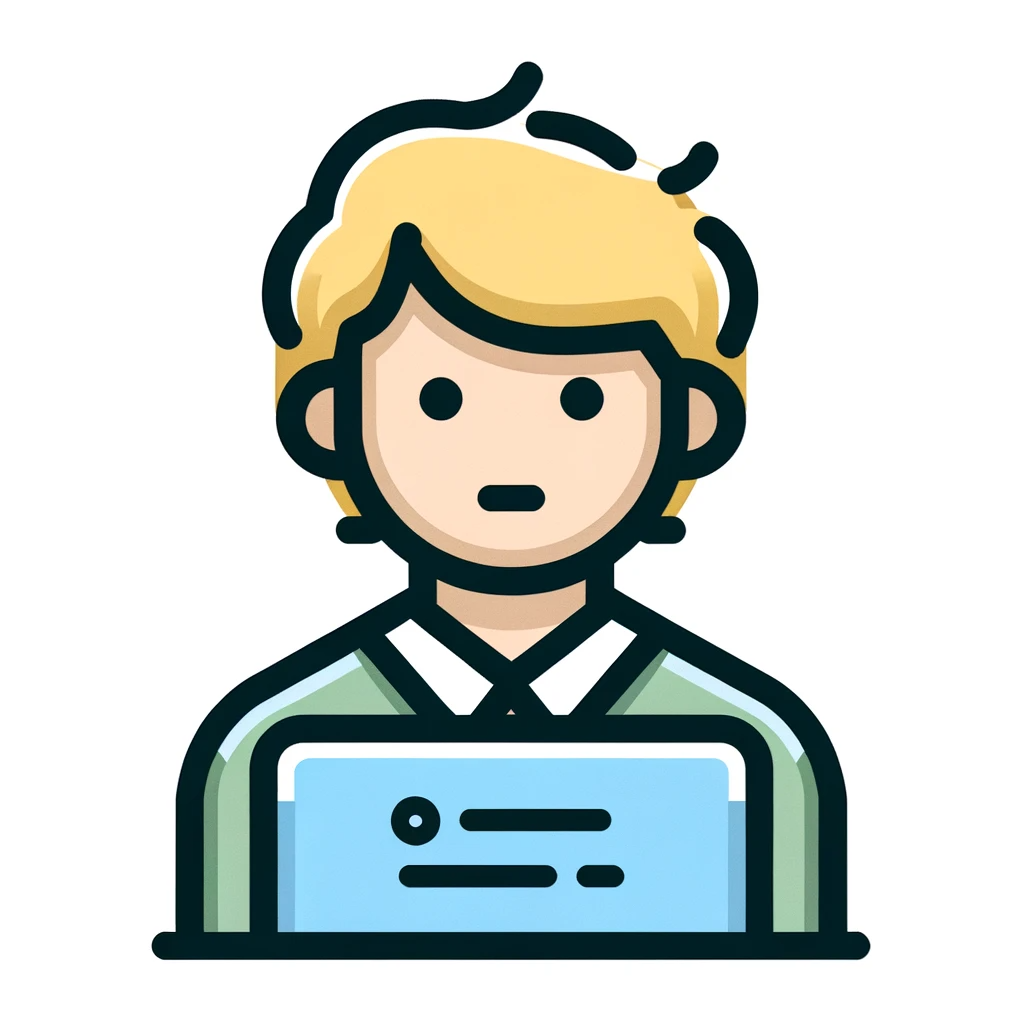
How can I convert a string to uppercase or lowercase?
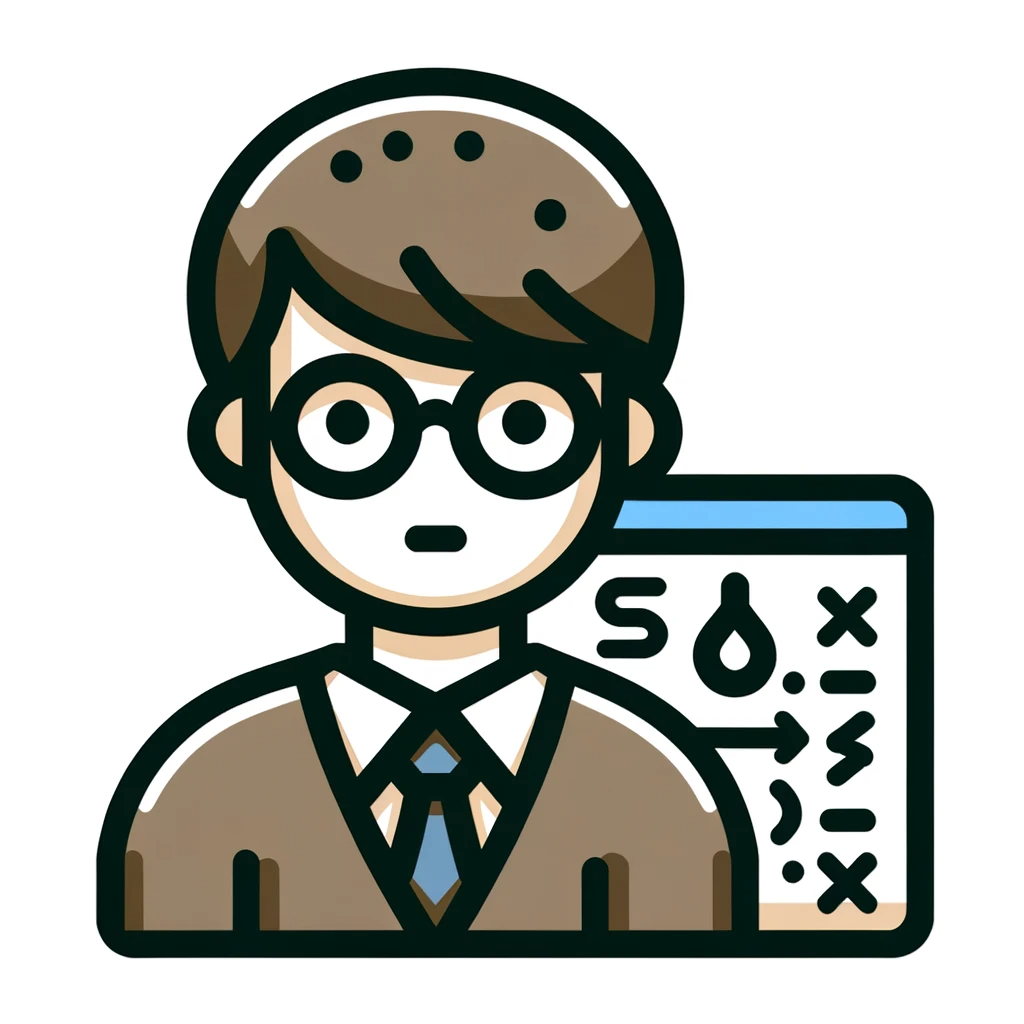
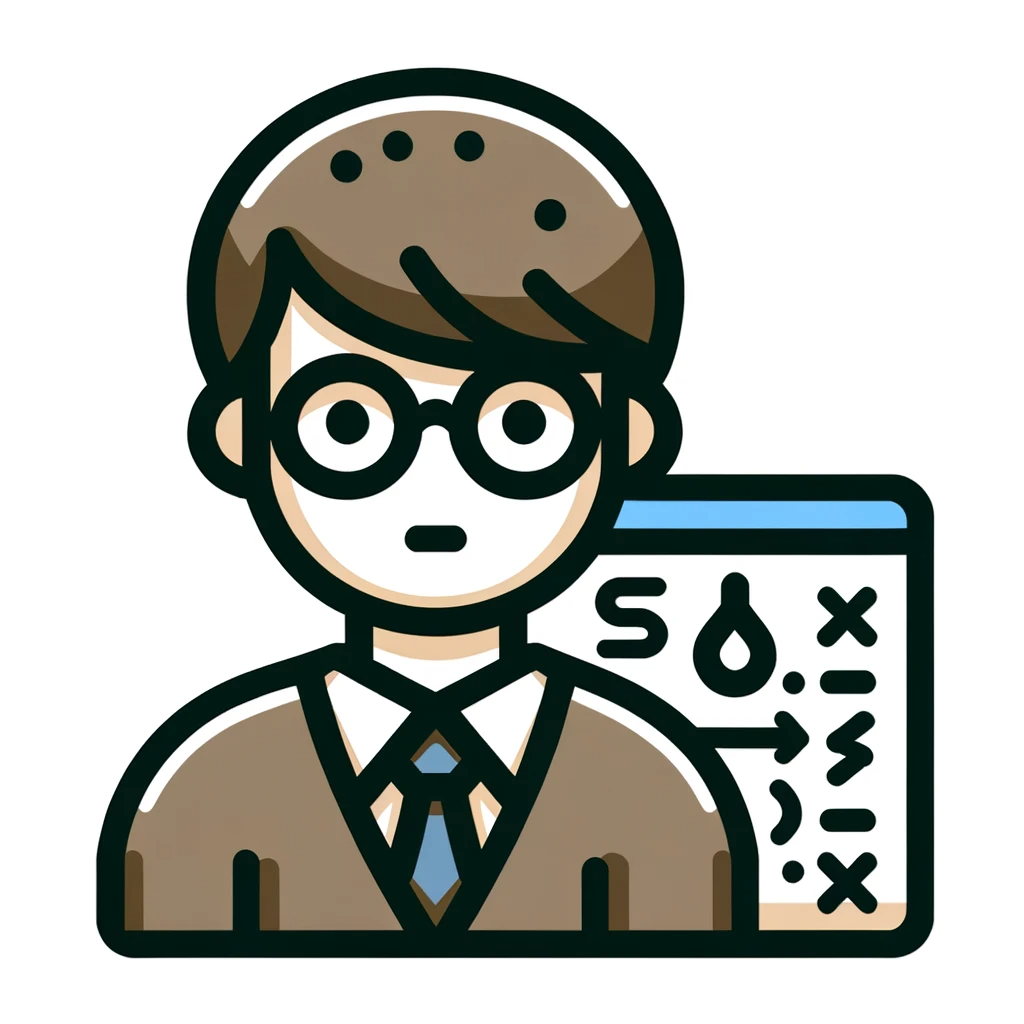
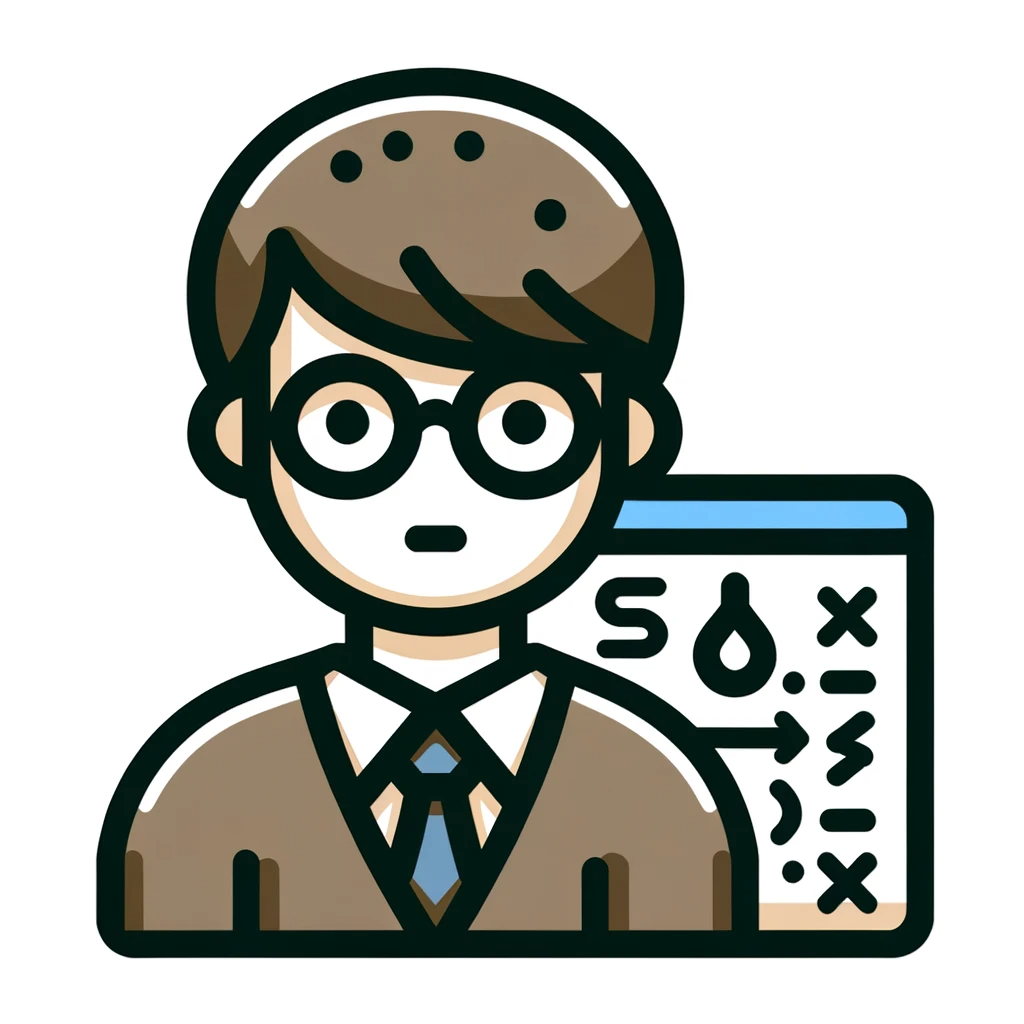
There are toUpperCase and toLowerCase methods.
These can be used to convert strings to uppercase or lowercase respectively.
Convert string to uppercase or lowercase
toUpperCase converts a string to uppercase
To convert a string to uppercase, use the toUpperCase method.
string.toUpperCase();
This method converts all characters in a string to uppercase.
For example, to convert the string “javascript” to uppercase:
let str = "javascript";
let result = str.toUpperCase();
console.log(result); // "JAVASCRIPT"
toLowerCase converts a string to lowercase
To convert a string to lowercase, use the toLowerCase method.
string.toLowerCase();
This method converts all characters in a string to lowercase.
For example, to convert the string “JAVASCRIPT” to lowercase:
let str = "JAVASCRIPT";
let result = str.toLowerCase();
console.log(result); // "javascript"
The toLocaleUpperCase and toLocaleLowerCase methods can be used to convert the case of localized strings.
Summary of “How to convert letters to uppercase and lowercase”
The following is a summary of how to convert characters to uppercase and lowercase.
- To convert a string to uppercase, use the toUpperCase method.
- To convert a string to lowercase, use the toLowerCase method.
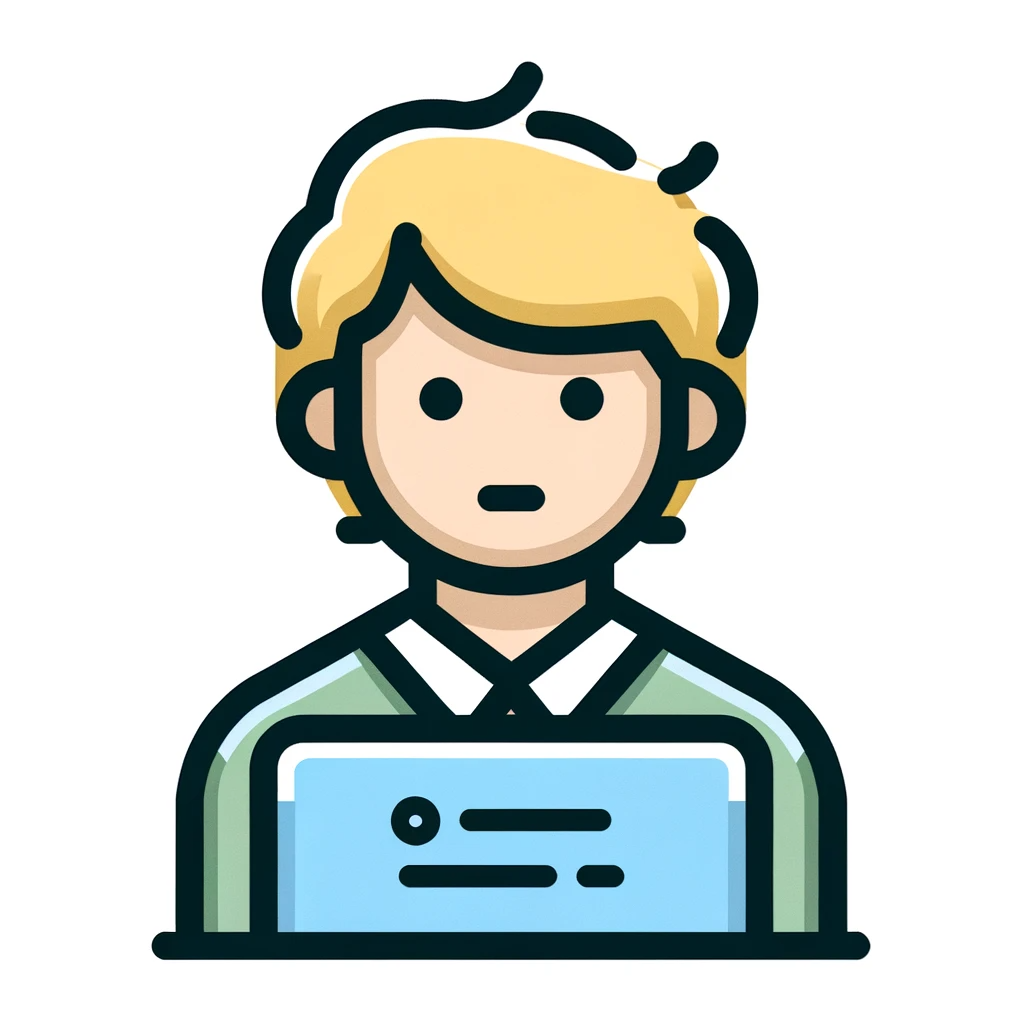
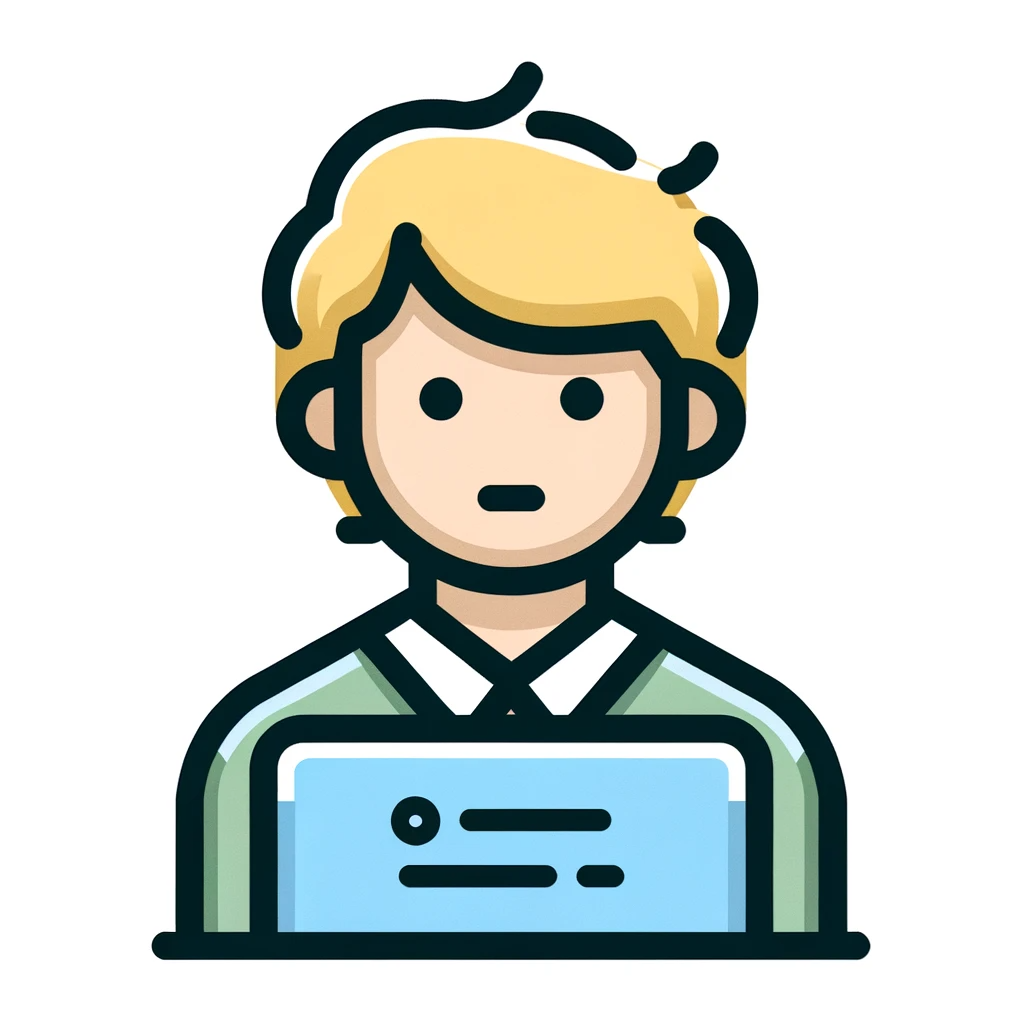
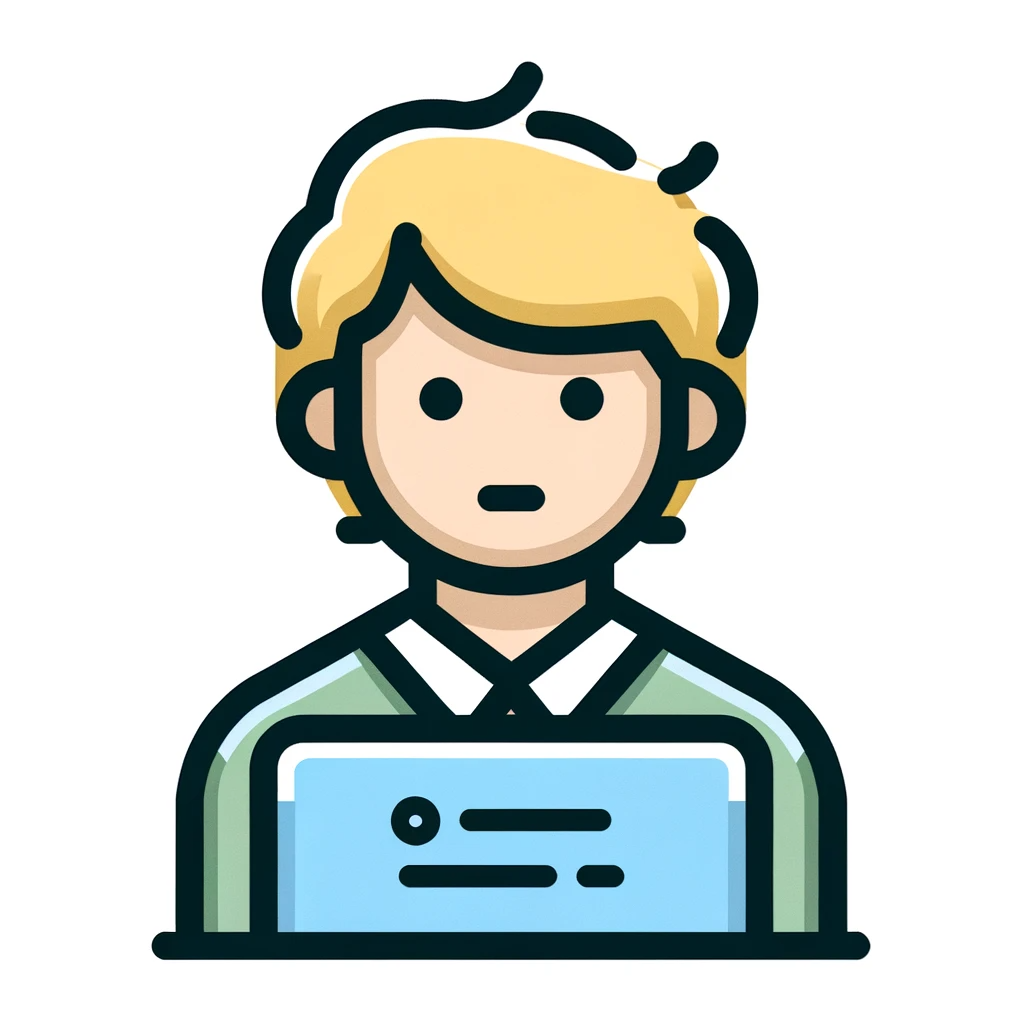
Now you know how to convert using toUpperCase and toLowerCase!
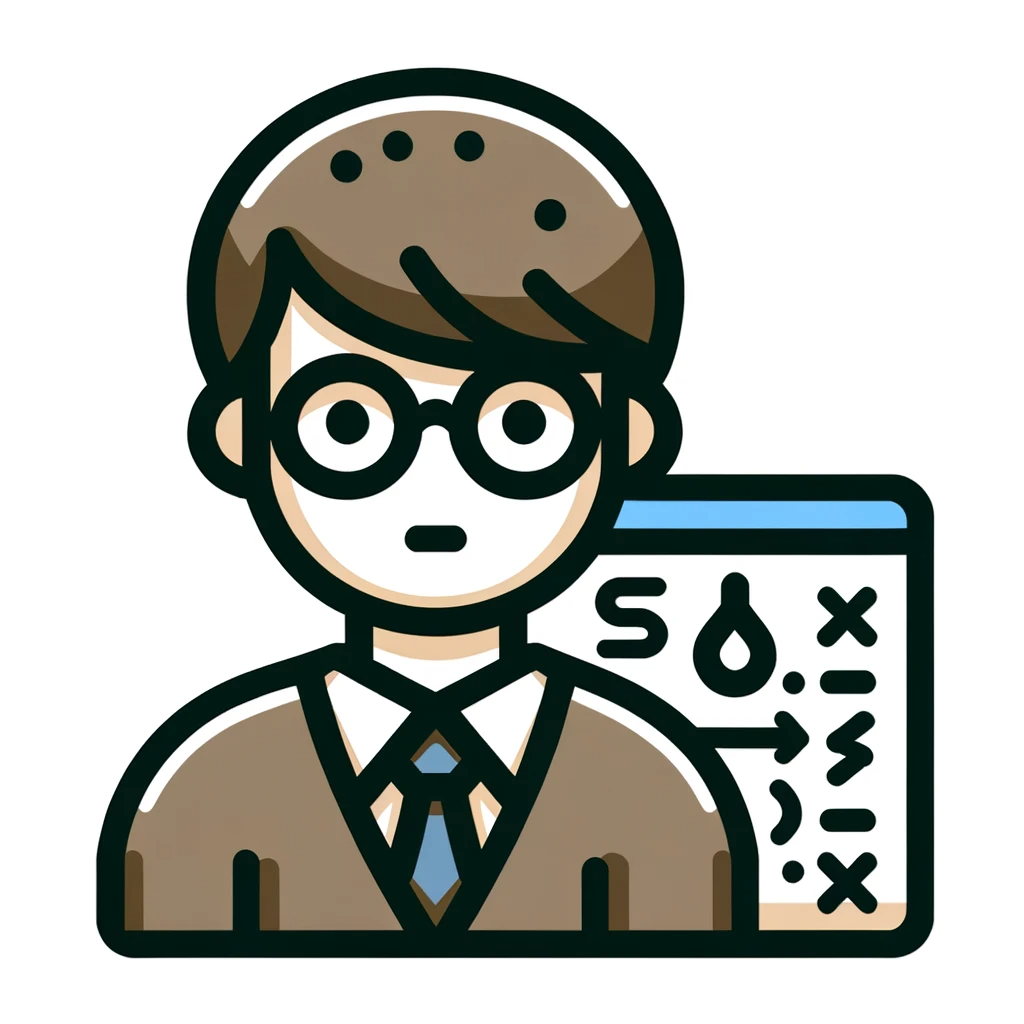
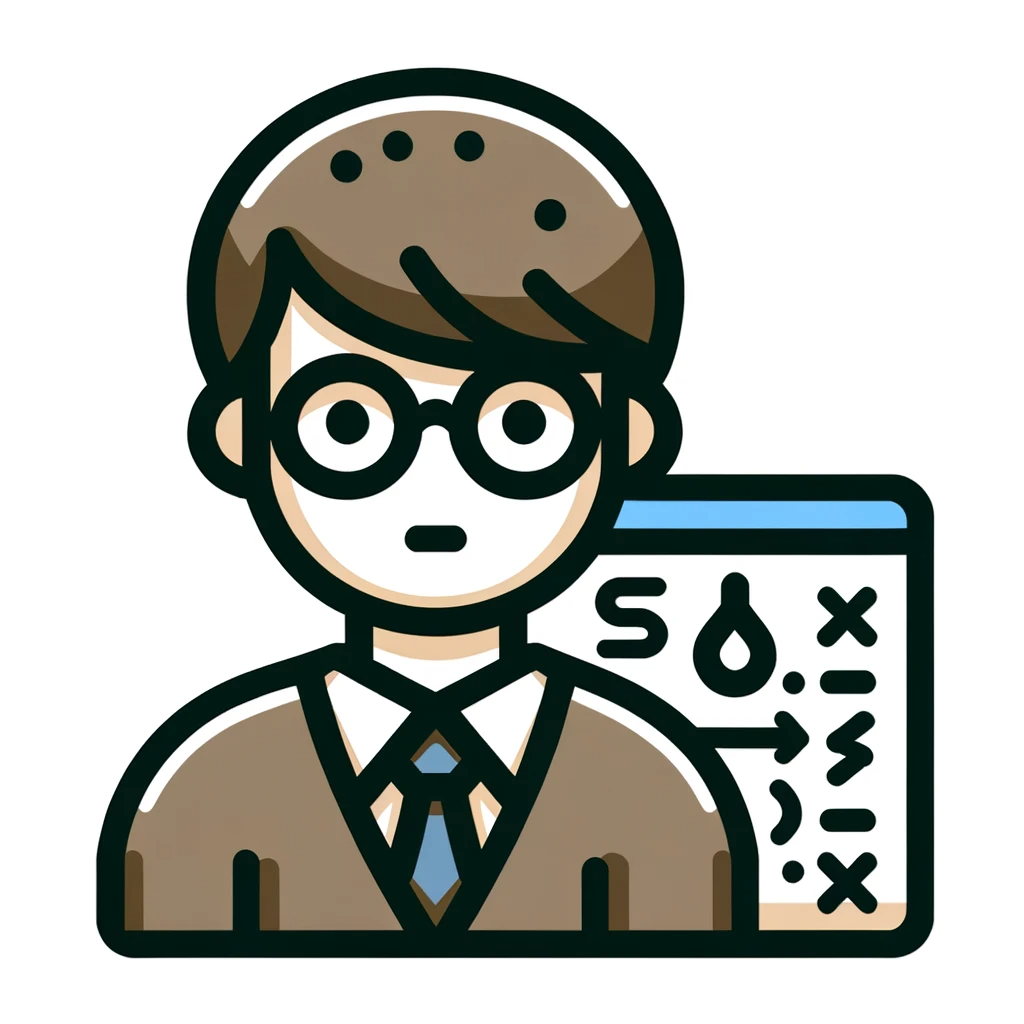
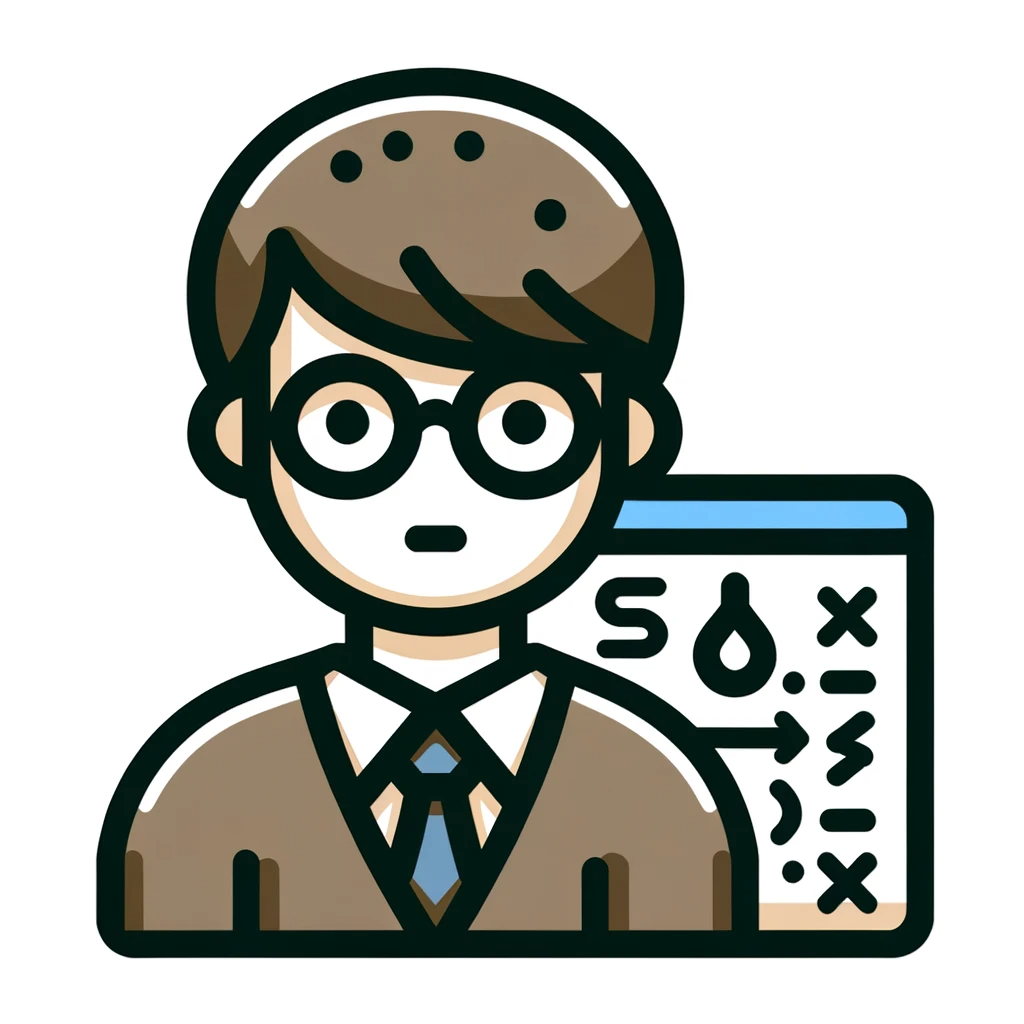
Before converting a string, it is a good idea to perform a null check to avoid errors if the string is null or undefined.
Comments