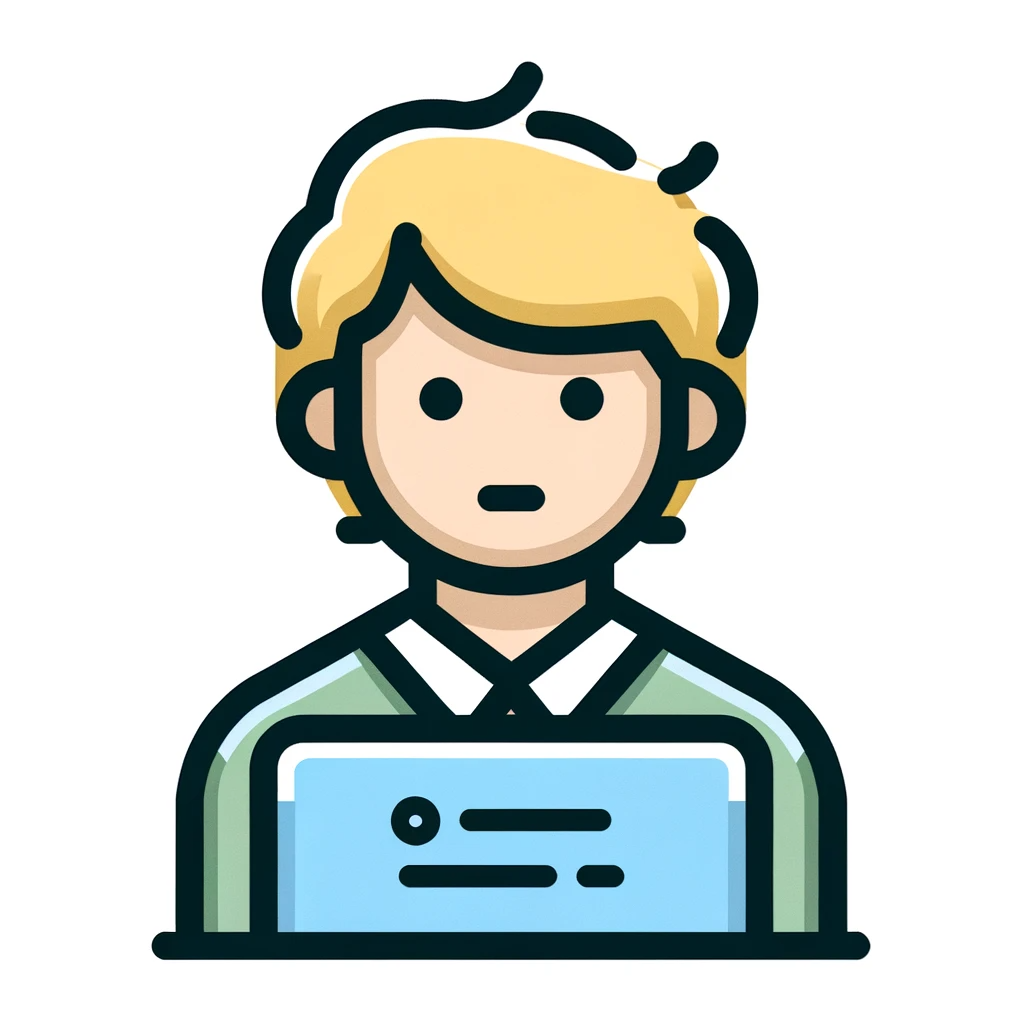
How can I convert numbers to characters?
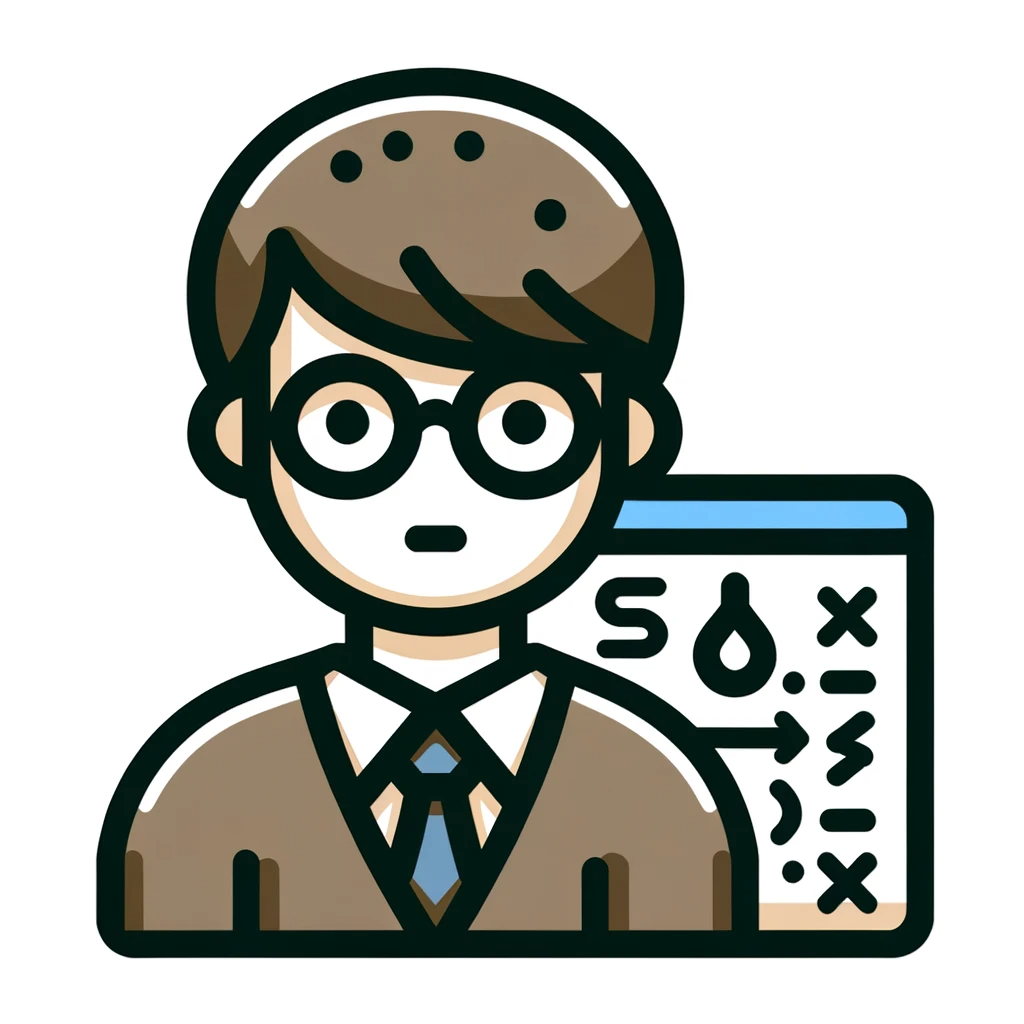
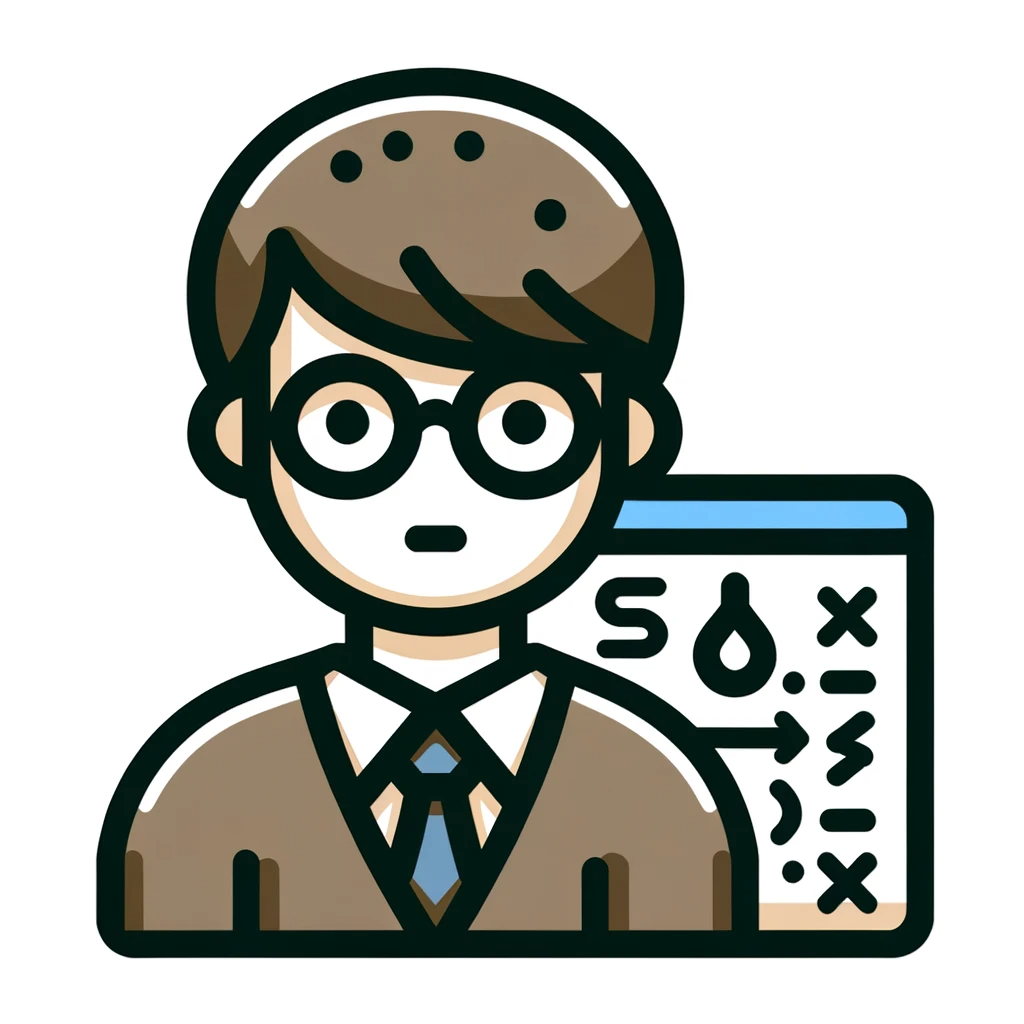
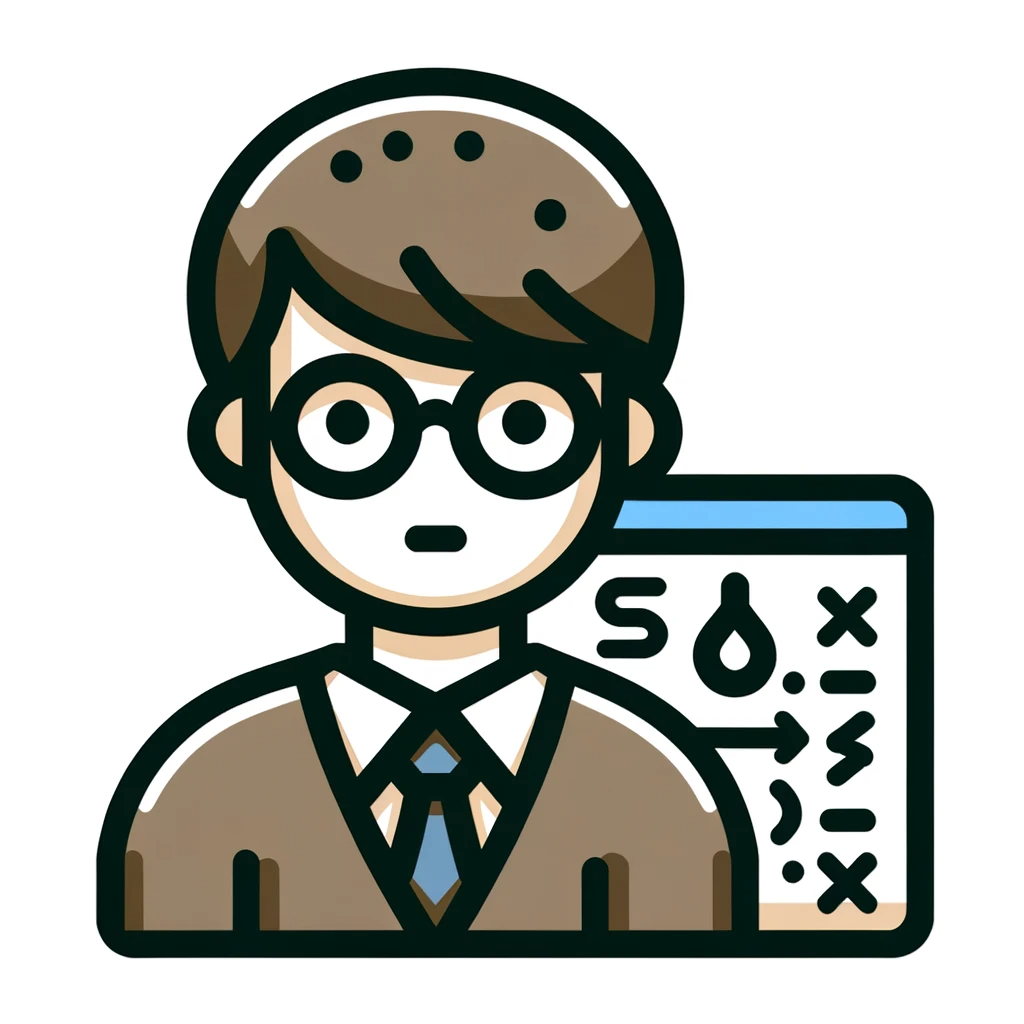
Convenient methods are the toString method and the String constructor.
Convert number to string using toString method
To convert a number to a string, toString
use the methods of the Number object.
variable.toString();
In the example below, the variable num stores the number 123. toString
By using the method, num is converted to a string and stored in a variable called str. str will be the string “123”.
let num = 123;
let str = num.toString();
Convert numbers to strings using the String function
Another way to convert numbers to strings is to use the String function .
String(variable);
The String function specifies the string to use when creating the string. Also, if you specify a number or object, it will convert them to a string and return them.
In the example below, the variable num stores the number 123. By using the String constructor, num is converted to a string and stored in a variable called str. str will be the string “123”.
let num = 123;
let str = String(num);
How to convert numbers to logical type
Another way to convert a number to a logical type is to use the Boolean function .
Boolean(variable);
A Boolean function is a function that converts a specified value to a logical type and returns it. The Boolean function specifies the value to be converted to a logical type. The Boolean function converts a value to a logical type and returns it according to the following rules:
- If 0 or NaN is specified, false is returned.
- If any other number is specified, true is returned.
let num1 = 0;
let bool1 = Boolean(num1); // bool1はfalse
let num2 = 123;
let bool2 = Boolean(num2); // bool2はtrue
let num3 = NaN;
let bool3 = Boolean(num3); // bool3はfalse
In the above example, num1, num2, and num3 store 0, 123, and NaN, respectively. By using the Boolean function, the values converted to logical type are stored in the variables bool1, bool2, and bool3.
Summary of “How to convert numbers to characters and logical types”
The following is a summary of how to convert numbers to characters and logical types .
- You can convert a number to a string by using the toString method.
- You can convert a number to a string by using the String function.
- You can convert numbers to logical types using the Boolean function.
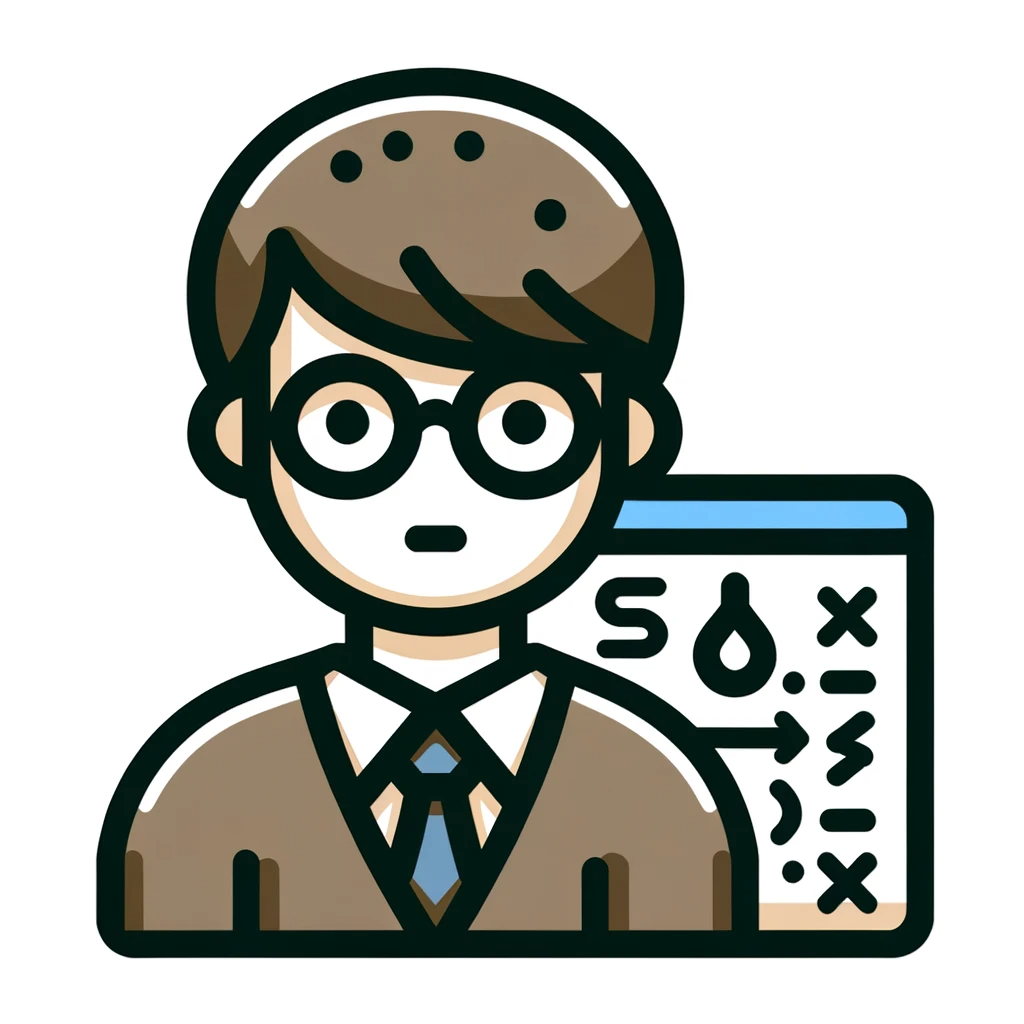
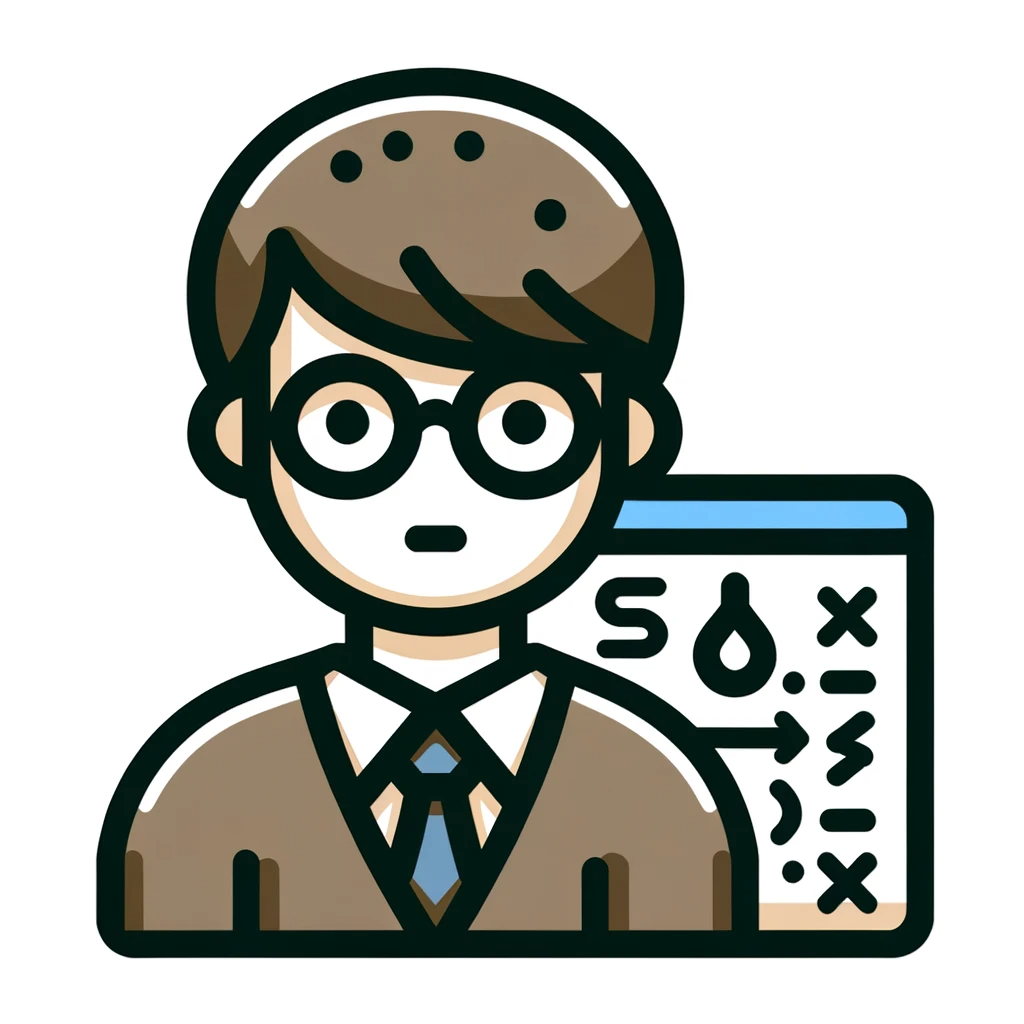
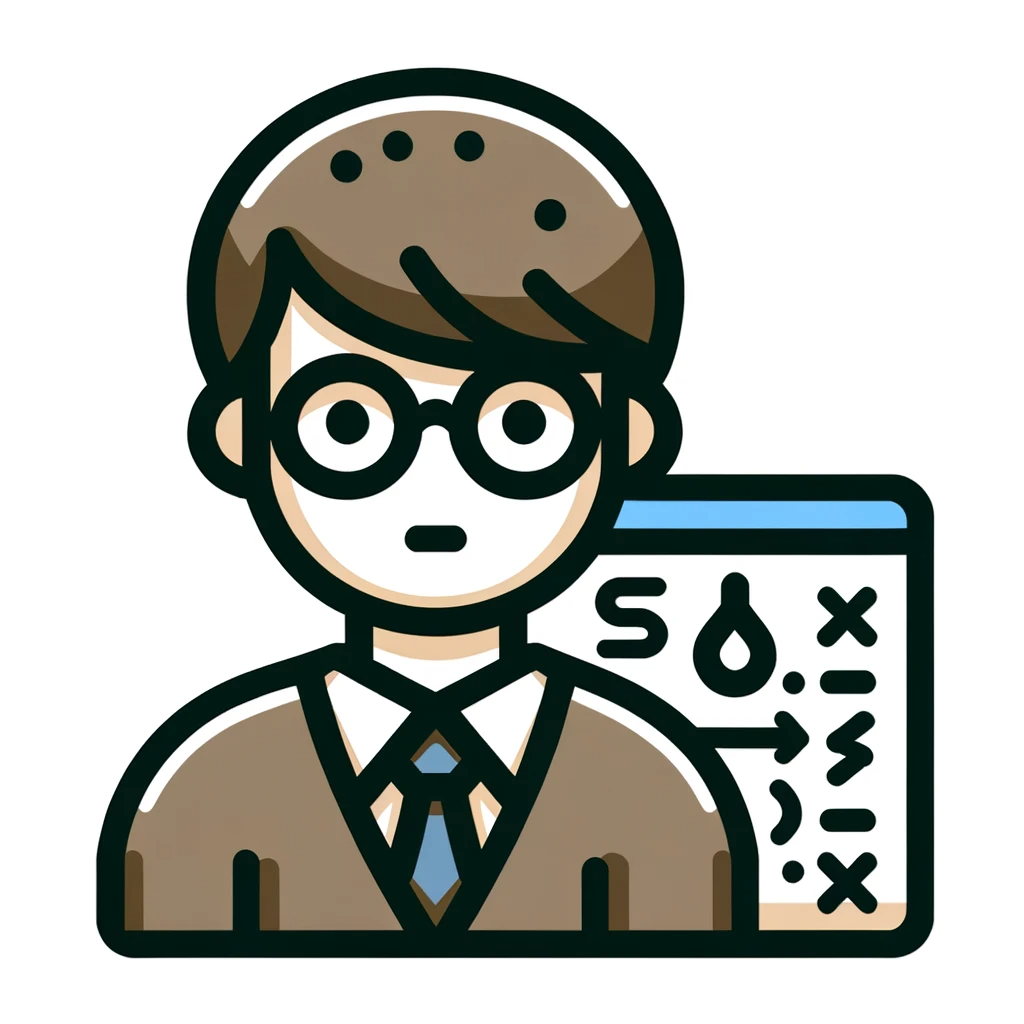
Which method you use depends on the occasion and your preferences.
The key is to use data types wisely.
Comments