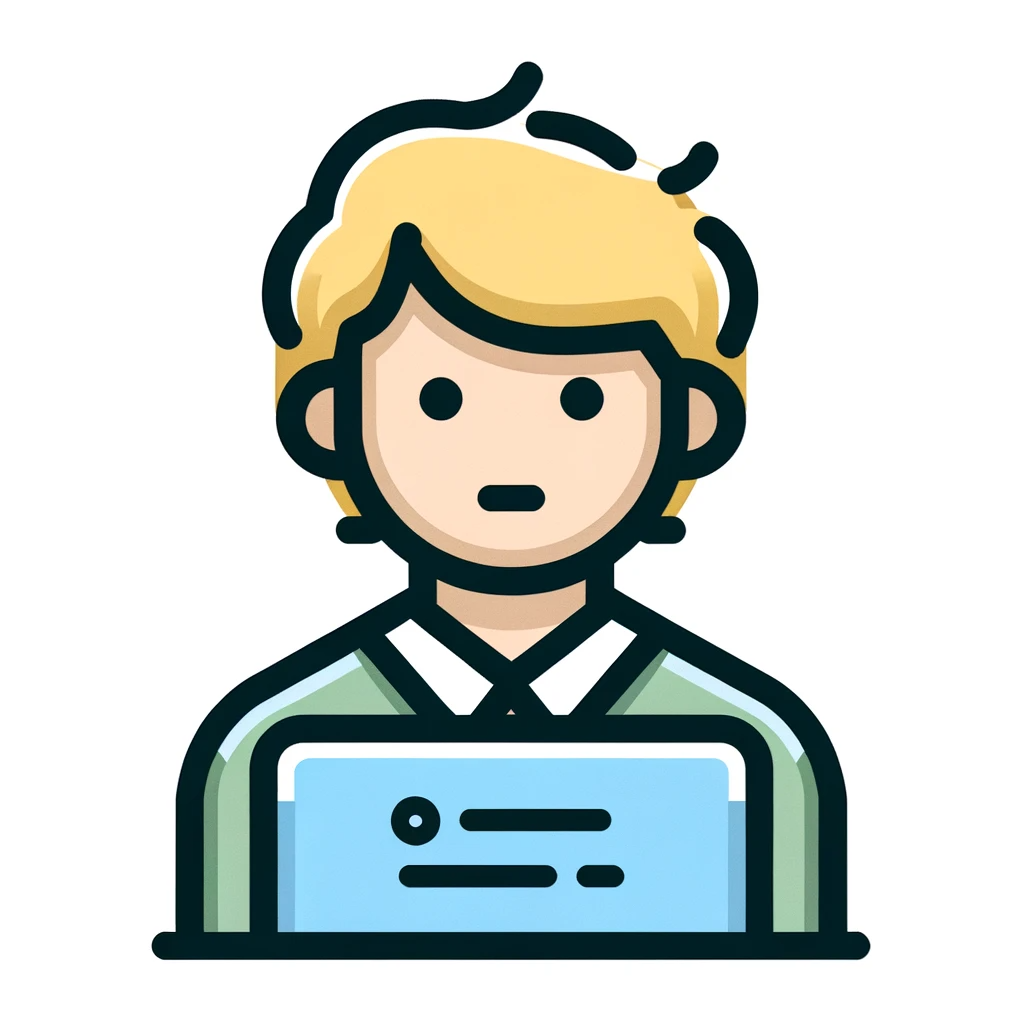
How can I output a string from a script?
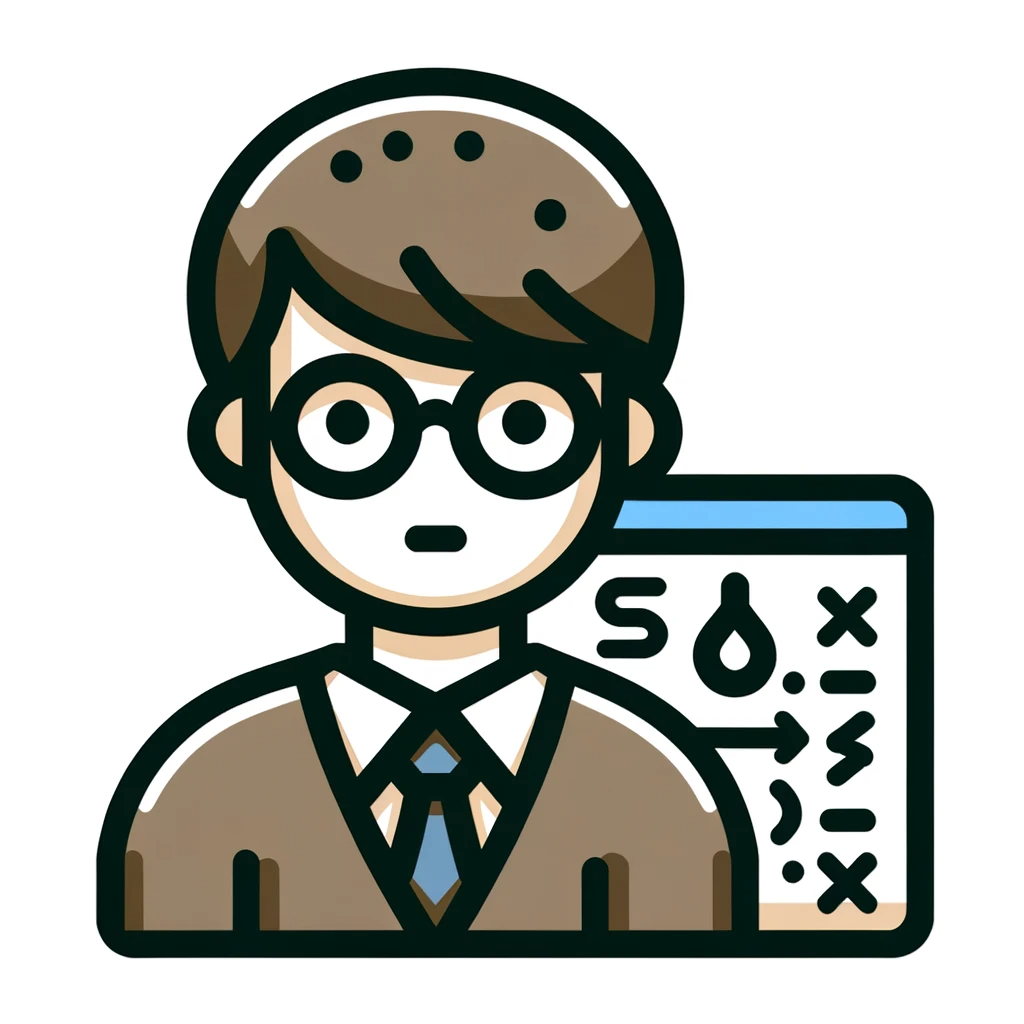
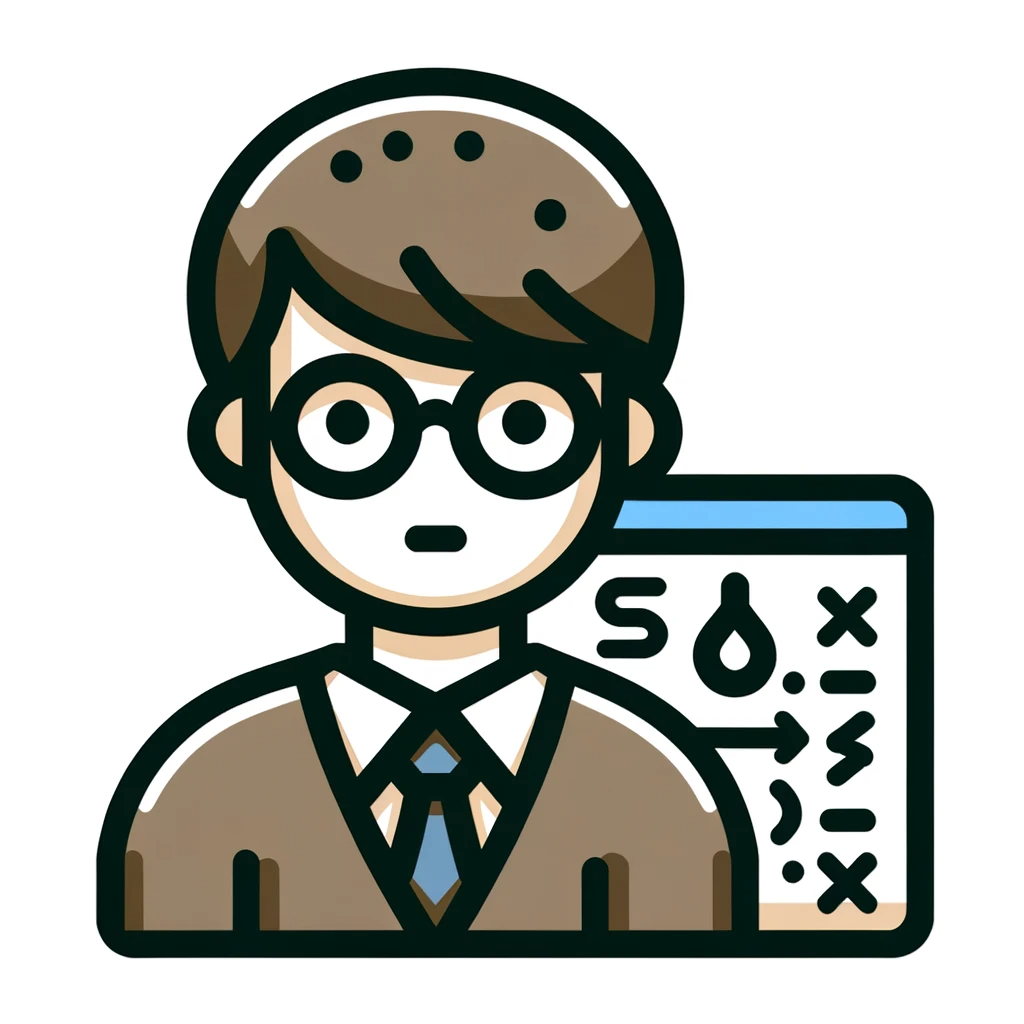
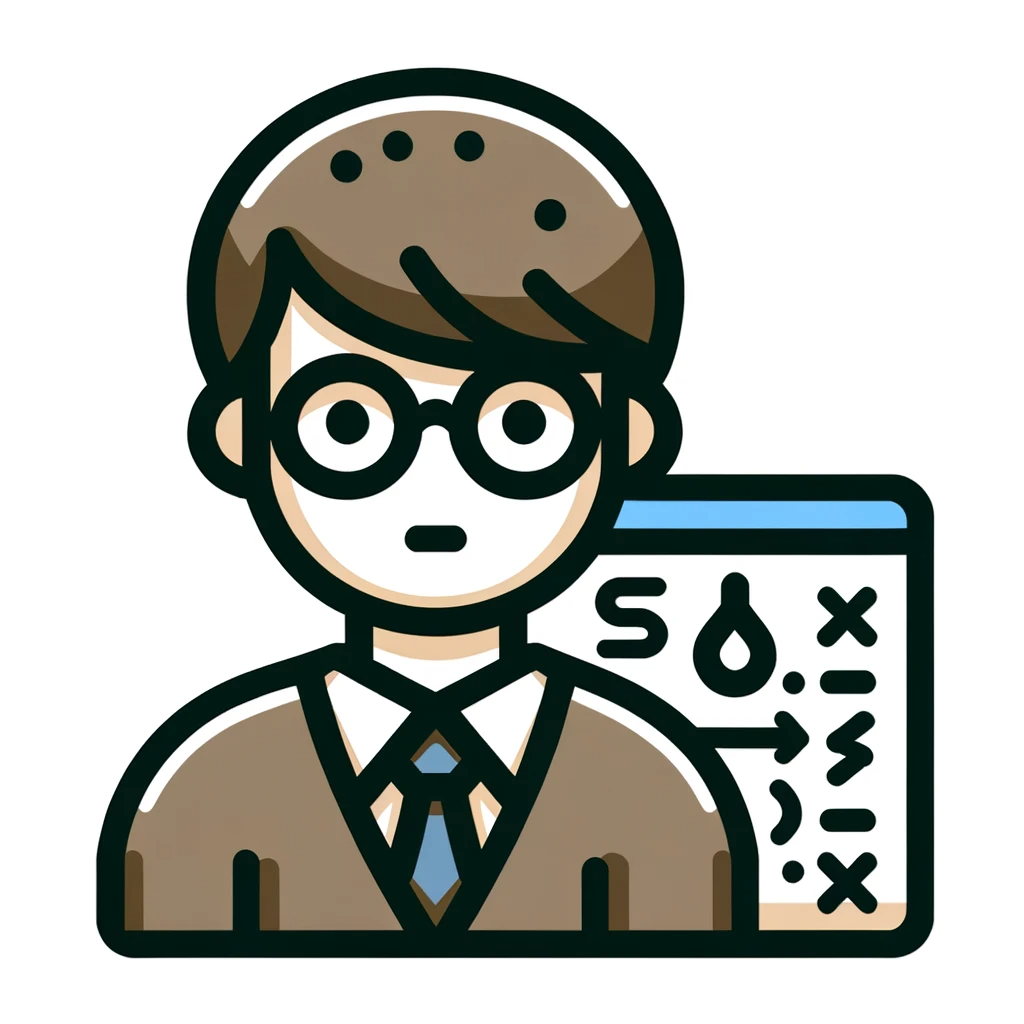
By using the textContent property , you can output a string while also specifying the location.
Output a string using textContent
To output strings within an HTML document using JavaScript, you textContent
can use properties of HTML elements.
textContent
Properties are used to get and set the text content of an HTML element.
document.getElementById(specified ID).textContent = string;
getElementById is a JavaScript function used to retrieve an element with the specified id attribute in an HTML document.
In the following sample, the id attribute is set on the HTML p element, and the id attribute is used to manipulate the textContent property in JavaScript.
<!DOCTYPE html>
<html>
<body>
<h1>Output strings using JavaScript</h1>
<p id="demo">The string is output here.</p>
<script>
document.getElementById("demo").textContent = "Hello, World!";
</script>
</body>
</html>
In the above example, the p element in the HTML document is retrieved and its textContent property is set to a string; when the HTML page is loaded, the string “Hello, World!” is output to the p element.
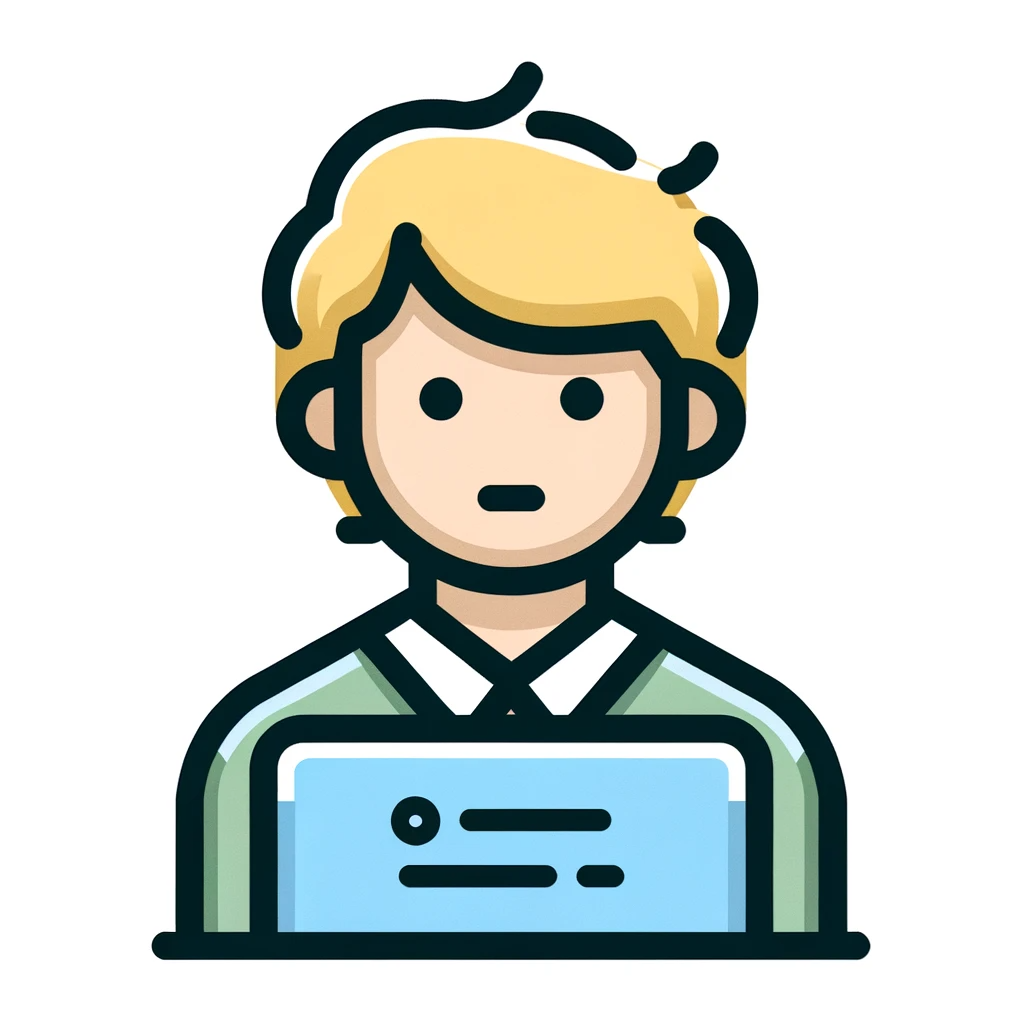
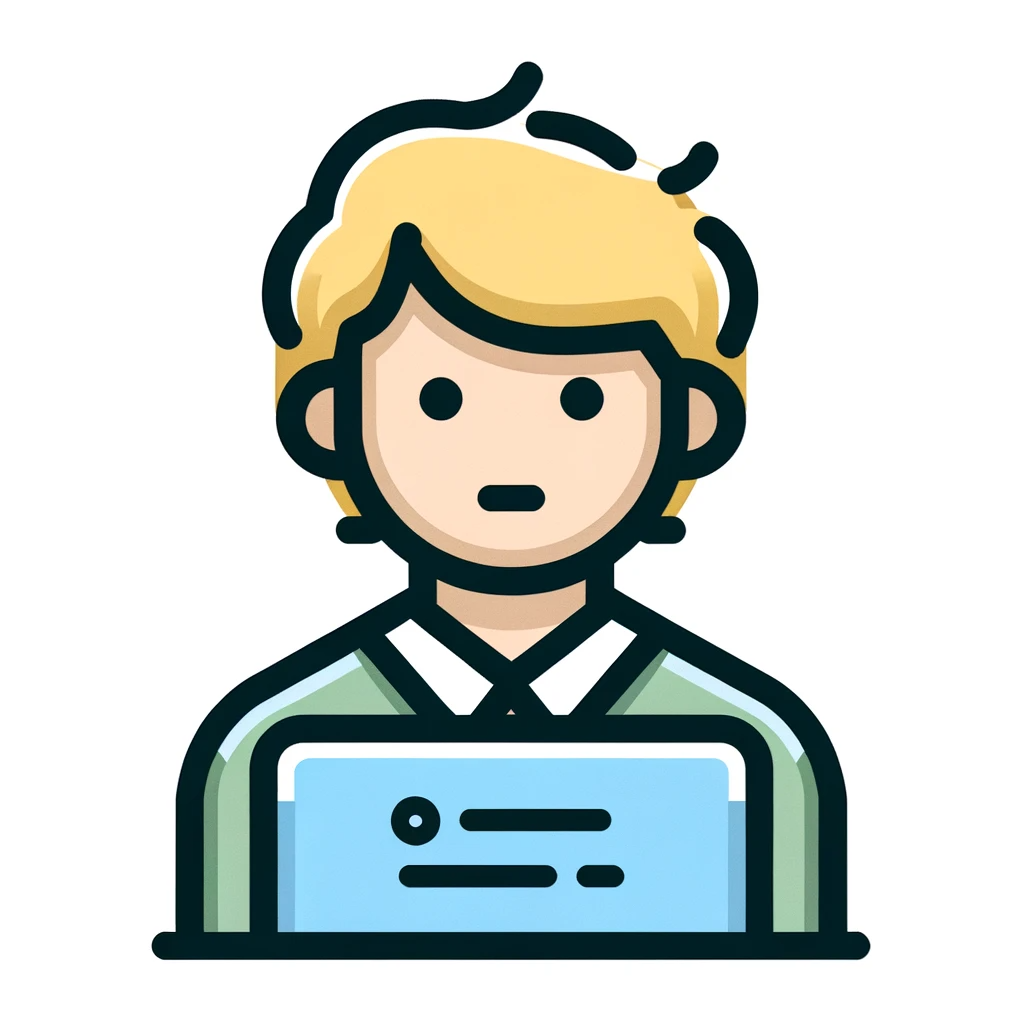
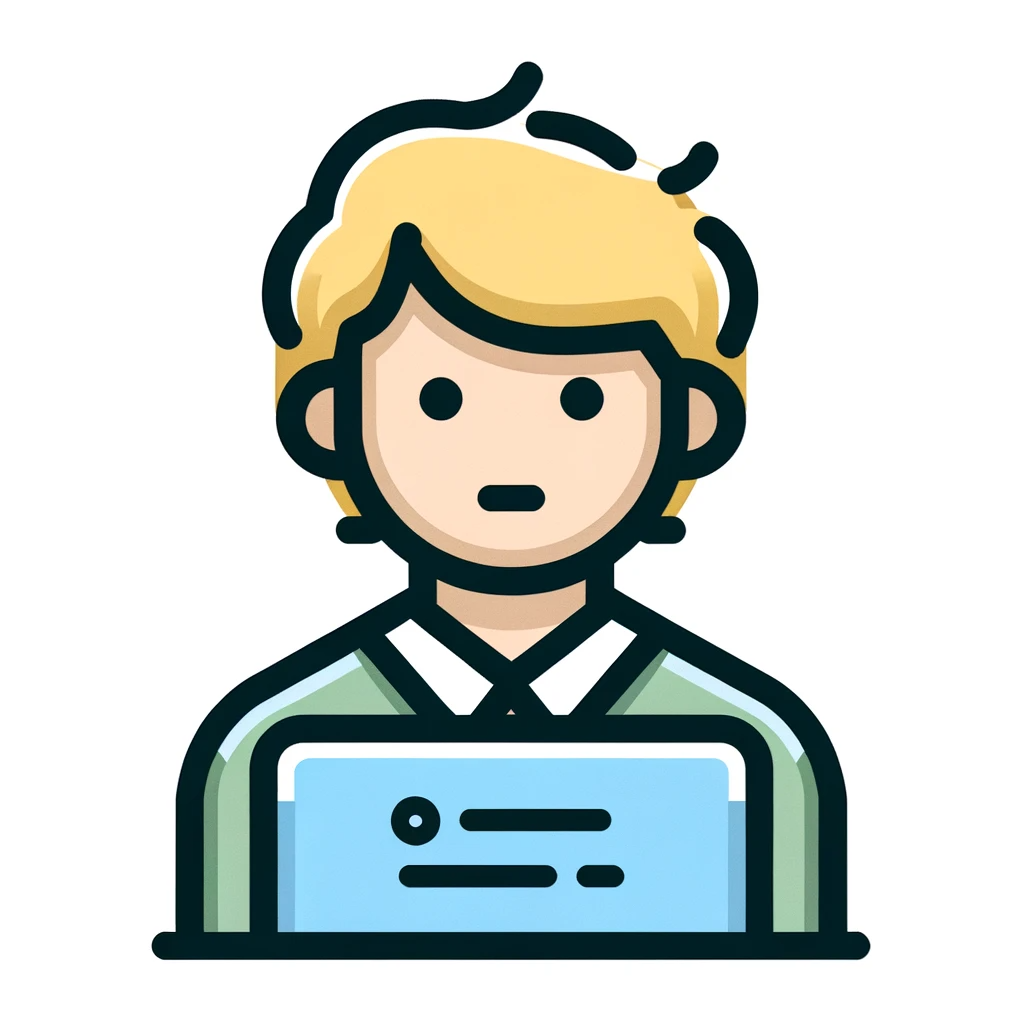
Since you can rewrite the specified ID string, you can change the display position!
textContent
Properties can also be used to retrieve the text content of an HTML element. You can get the text content of an HTML element as follows:
var text = document.getElementById(“demo”).textContent;
Output string in alert
You can use the window.alert method to display a string in a dialog box.
window.alert(string);
<!DOCTYPE html>
<html>
<body>
<h1>Output string using JavaScript</h1>
<script>
window.alert("Hello, World!");
</script>
</body>
</html>
print string to console
If you want to output strings for debugging, you can also output them to the console.
To output strings to the JavaScript console, use the console.log() function.
This way, you can see the strings in the console tab of the web browser’s developer tools.
<!DOCTYPE html>
<html>
<body>
<h1>Output string using JavaScript</h1>
<script>
console.log("Hello, World!");
</script>
</body>
</html>
Summary of “How to output strings
This is a summary of “How to output strings” in JavaScript.
- You can set and retrieve strings to HTML elements by setting the textContent property of the HTML element.
- The alert() function allows you to write a script in an HTML document to display a string in a dialog box.
- The console.log() function can be used to output a string to the JavaScript console.
The HTML element’s textContent property and the alert() and console.log() functions can be used to output strings in HTML documents.
Comments