This article explains how to change the style of an element using JavaScript’s style property.
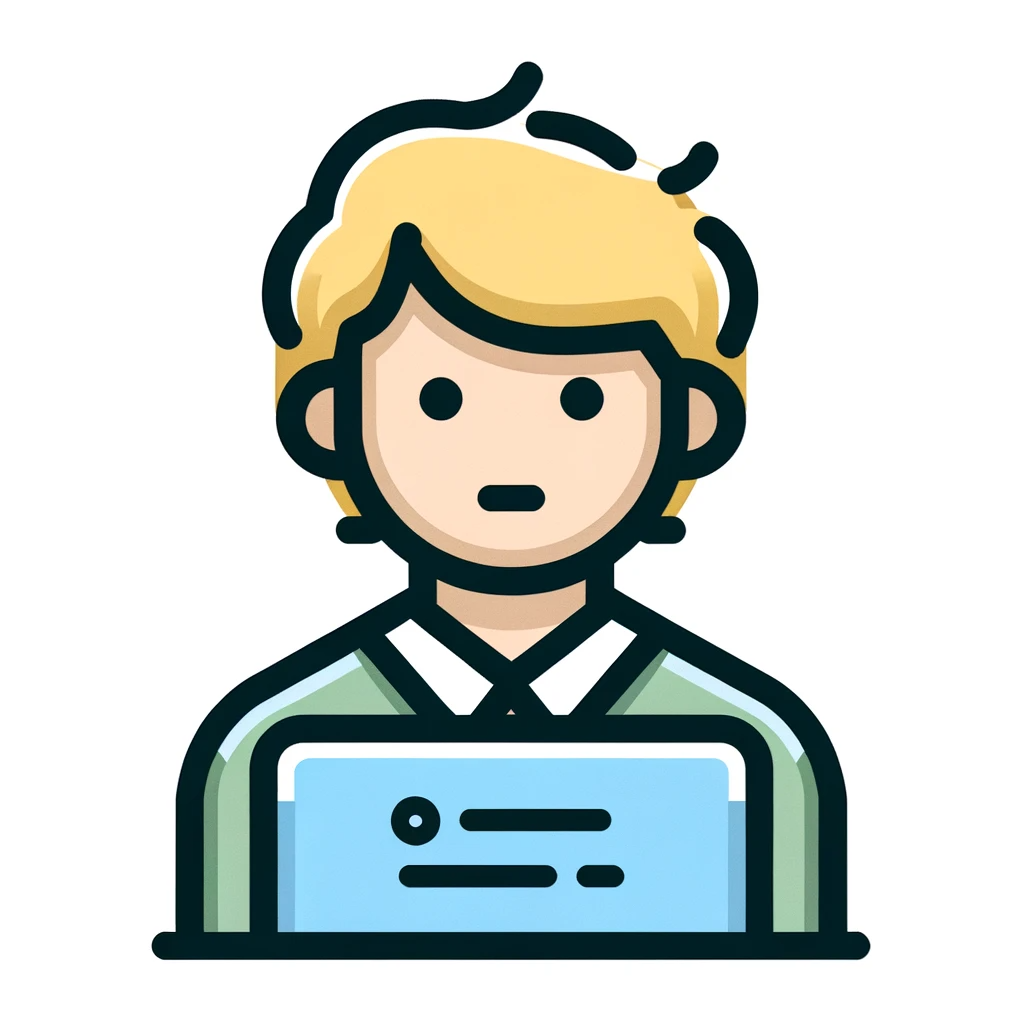
How can I change the style of an element in JavaScript?
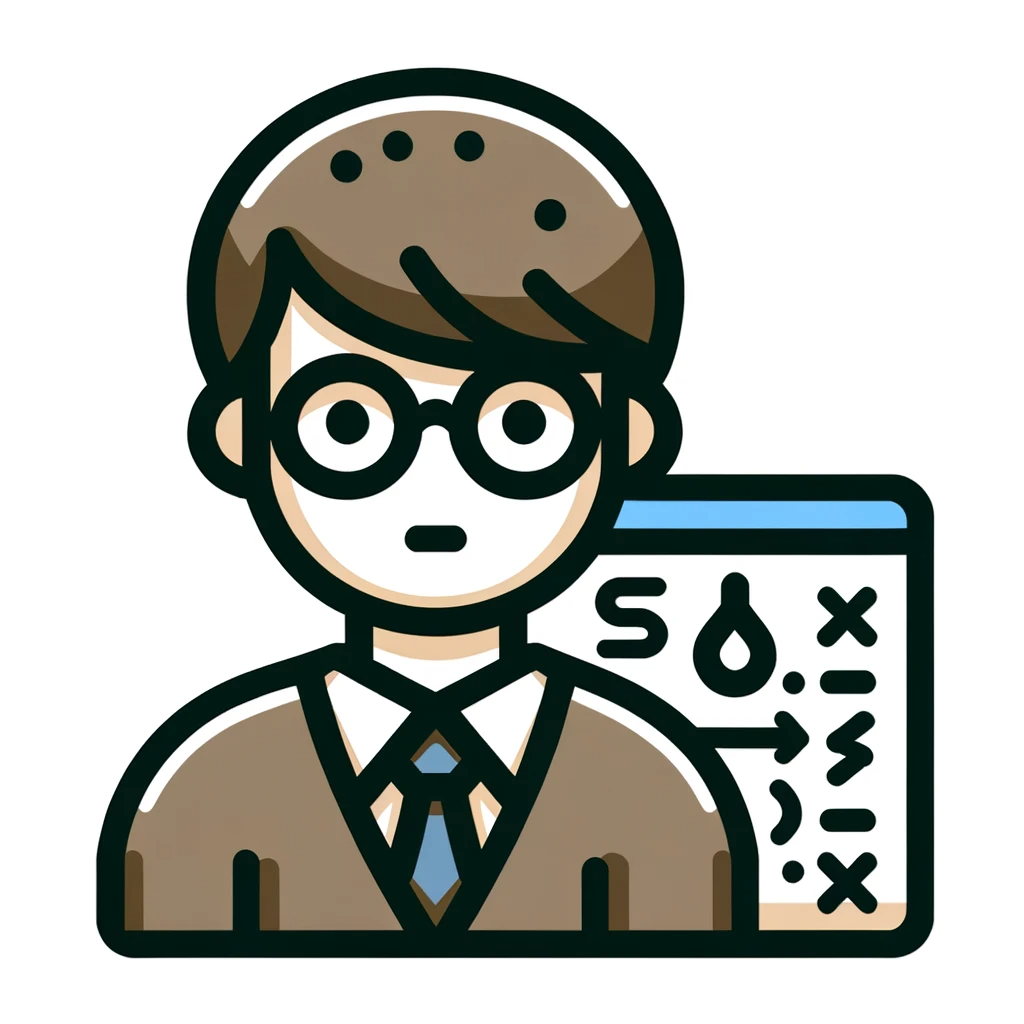
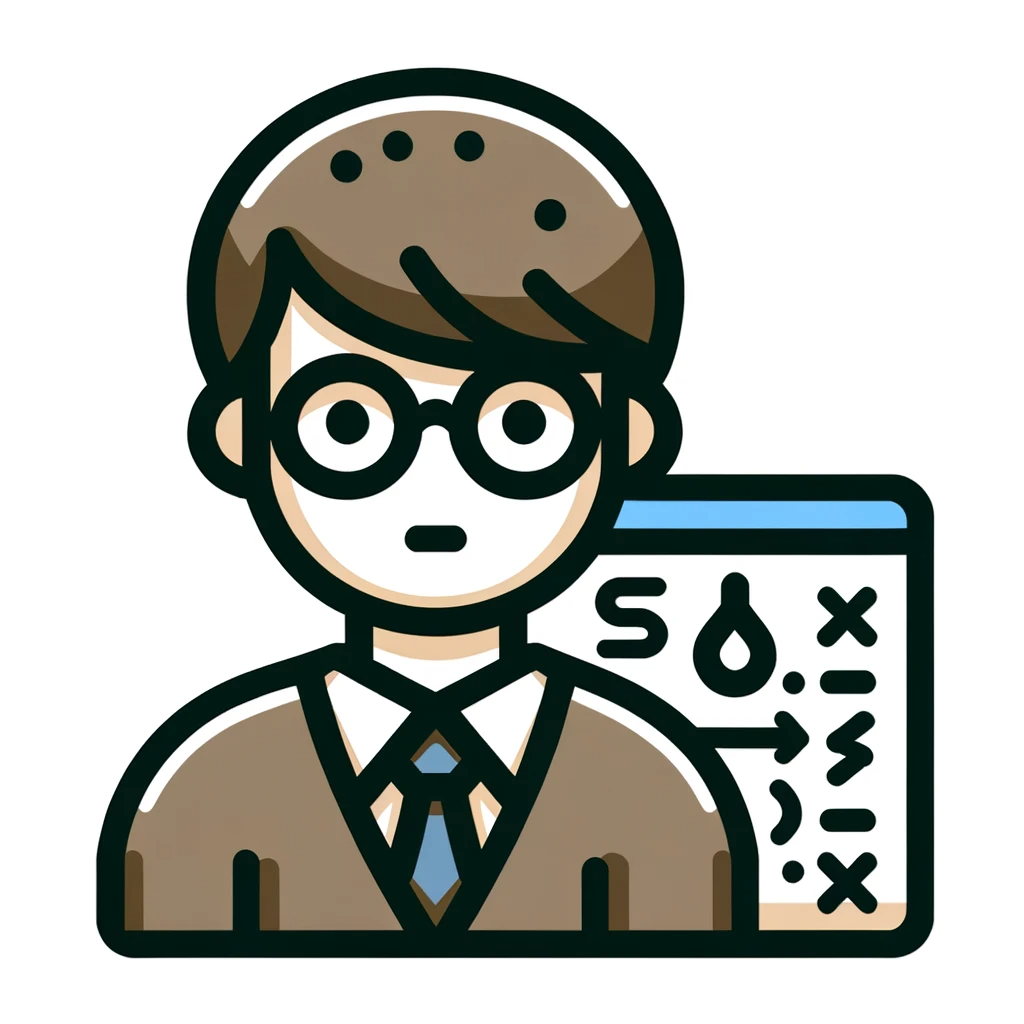
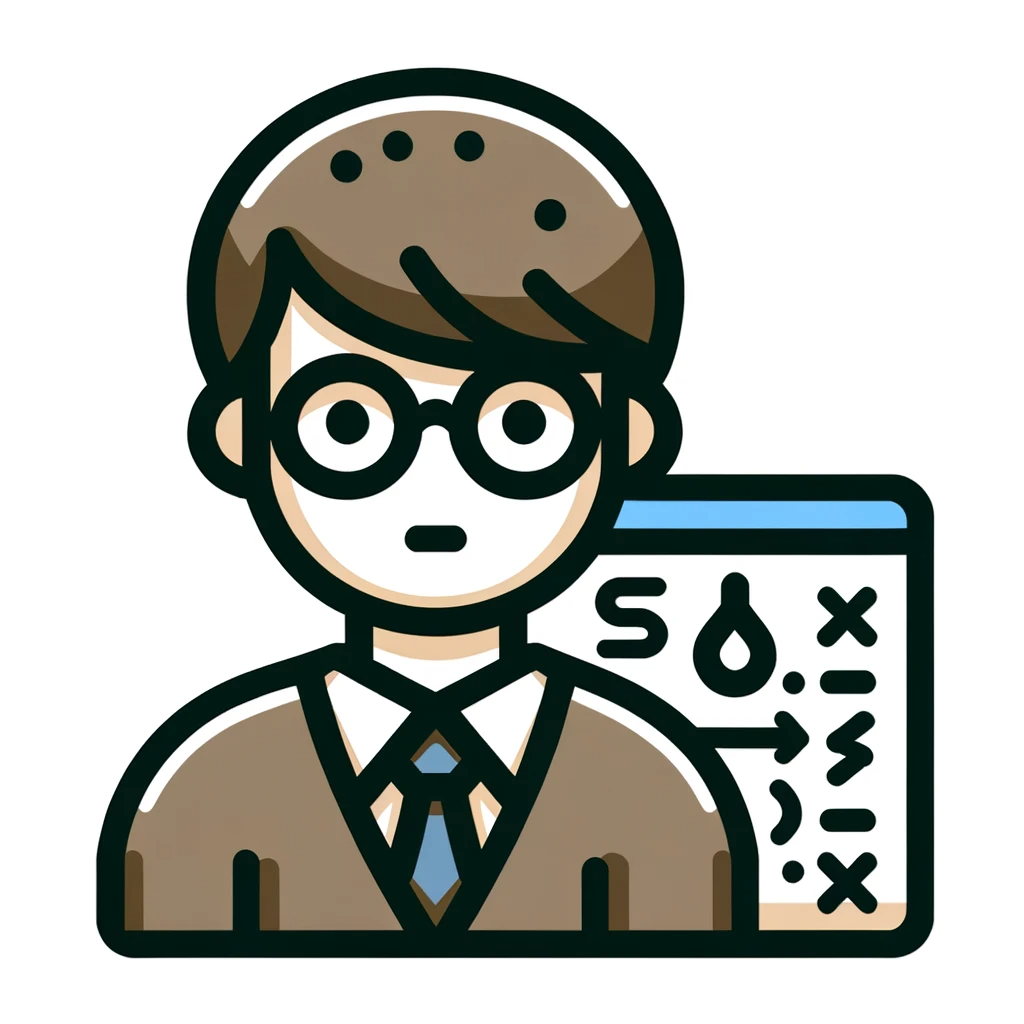
You can change the style of an element using the style property.
What is the style property?
The style property is a property that allows you to change the style (appearance) of an HTML element using JavaScript . This property allows you to change the style of an element in the same way as CSS properties.
The style property is defined as a property of the HTML element object, and is written as follows.
element.style.property = value;
element:HTML element object
property: CSS property
value: value to be set to property
For example, if you want to change the background color of an element, write as follows.
element.style.backgroundColor = 'red';
You can also specify multiple properties at once for the style property. List the properties by separating them with “;” like in CSS, as shown below.
element.style.cssText = 'background-color: blue; color: white; font-size: 20px;';
By using the style property like this, you can dynamically change the style of an HTML element.
How to use the style property: Explanation using a sample program
Below is a sample program that changes the style using the style property.
// Get HTML Elements
const element = document.getElementById('example');
// Change background color
element.style.backgroundColor = 'red';
// Change font size
element.style.fontSize = '16px';
// Specify multiple properties at once
element.style.cssText = 'background-color: blue; color: white; font-size: 20px;';
In the above program, the getElementById() method is used to retrieve an HTML element whose id is “example”. Next, the style property is used to change the style of the element. This changes the background color of the element to red and the font size to 16 pixels.
Also, to specify multiple properties for the style property at once, use the cssText property. As shown below, multiple style properties can be specified for the cssText property, separated by semicolons. This will change the background color of the element to blue, the text color to white, and the font size to 20 pixels.
In this way, you can easily change the style of an HTML element using JavaScript’s style property.
How to initialize the style property
It can be initialized by setting the style property to null.
element.style.property = null;
Changing style with setAttribute method is not recommended
When changing the style of an HTML element, there is a way to directly change the style attribute using the setAttribute method, but this method is not recommended .
This is because if you directly change the style attribute using the setAttribute method, it will overwrite the style that has already been set, so the style set on the element may be completely lost.
For example, suppose you want to set the background color of an element to red as shown below.
document.getElementById('myDiv').setAttribute('style', 'background-color: red;');
If you directly change the style attribute using the setAttribute method like this, the style set on the element will be completely lost. Also, among the styles already set on the element, only the background-color property may be overwritten, and other styles may not be preserved, which may cause the element’s style to change unexpectedly.
Therefore, when changing the style of an element, it is recommended to change the style property directly instead of using the setAttribute method. By using the style property, you can change part of the style while retaining the style set for the element.
document.getElementById('myDiv').style.backgroundColor = 'red';
In this way, by using the style property, you can change the style while retaining the style set for the element.
summary
We explained how to change the style of an element using the style property.
- You can change the style of an element using JavaScript’s style property.
- There are ways to directly rewrite the style property and methods to use the setAttribute() method.
- Style settings may require units.
- You can also specify multiple properties at once in the style property.
- Use null when initializing the style property.
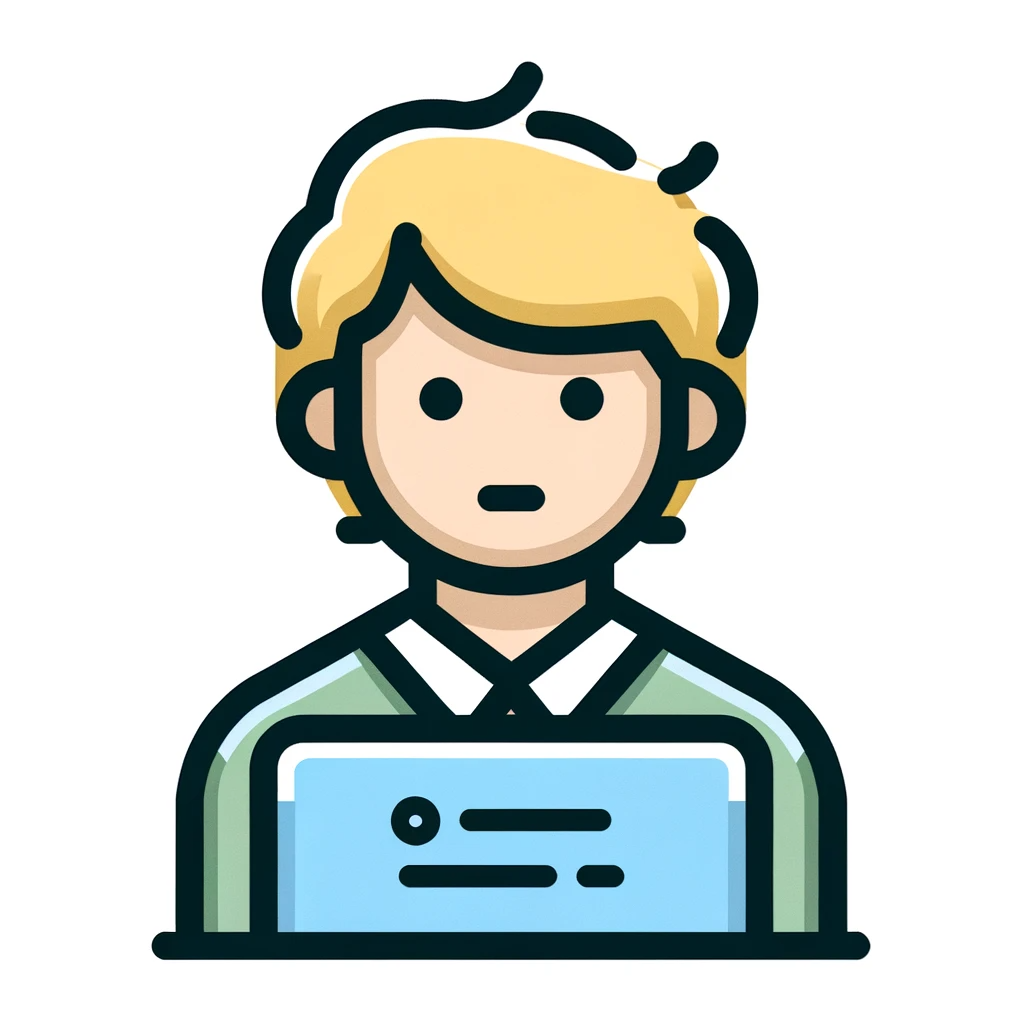
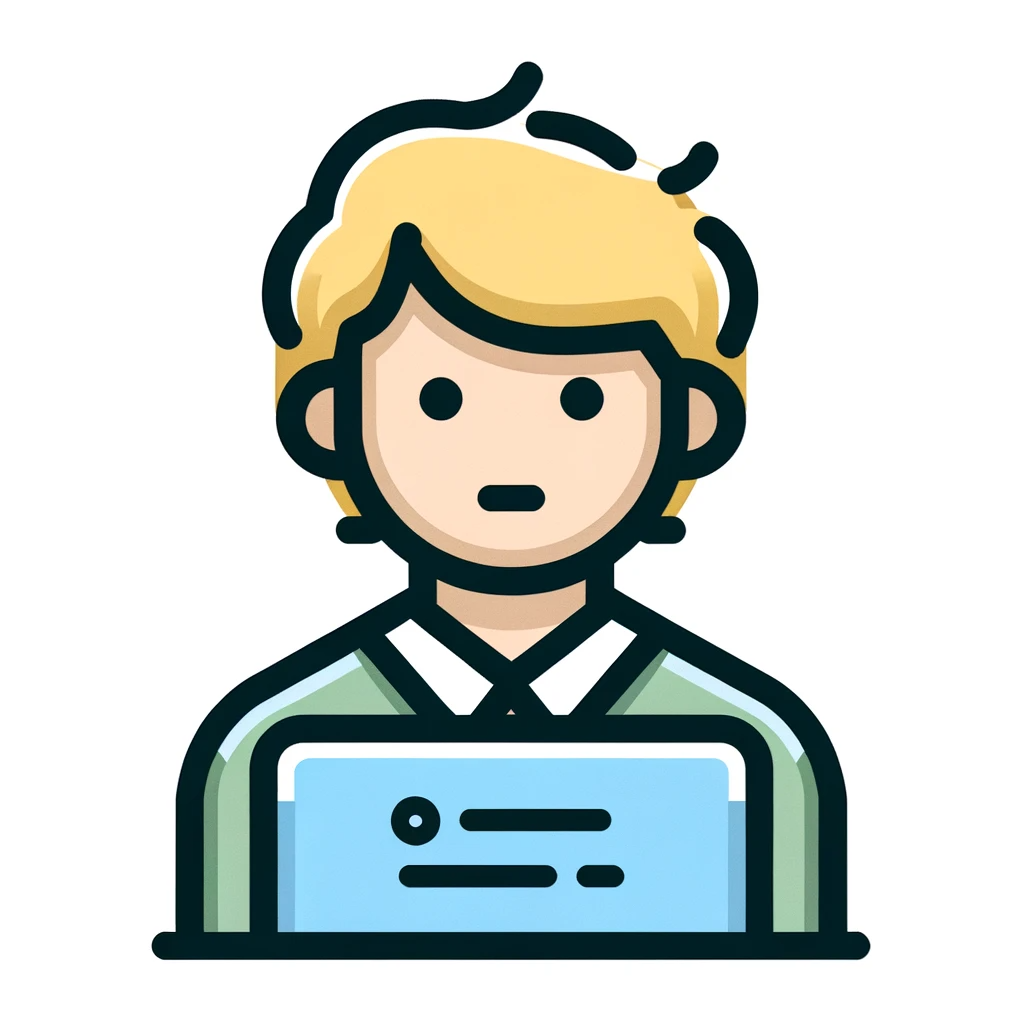
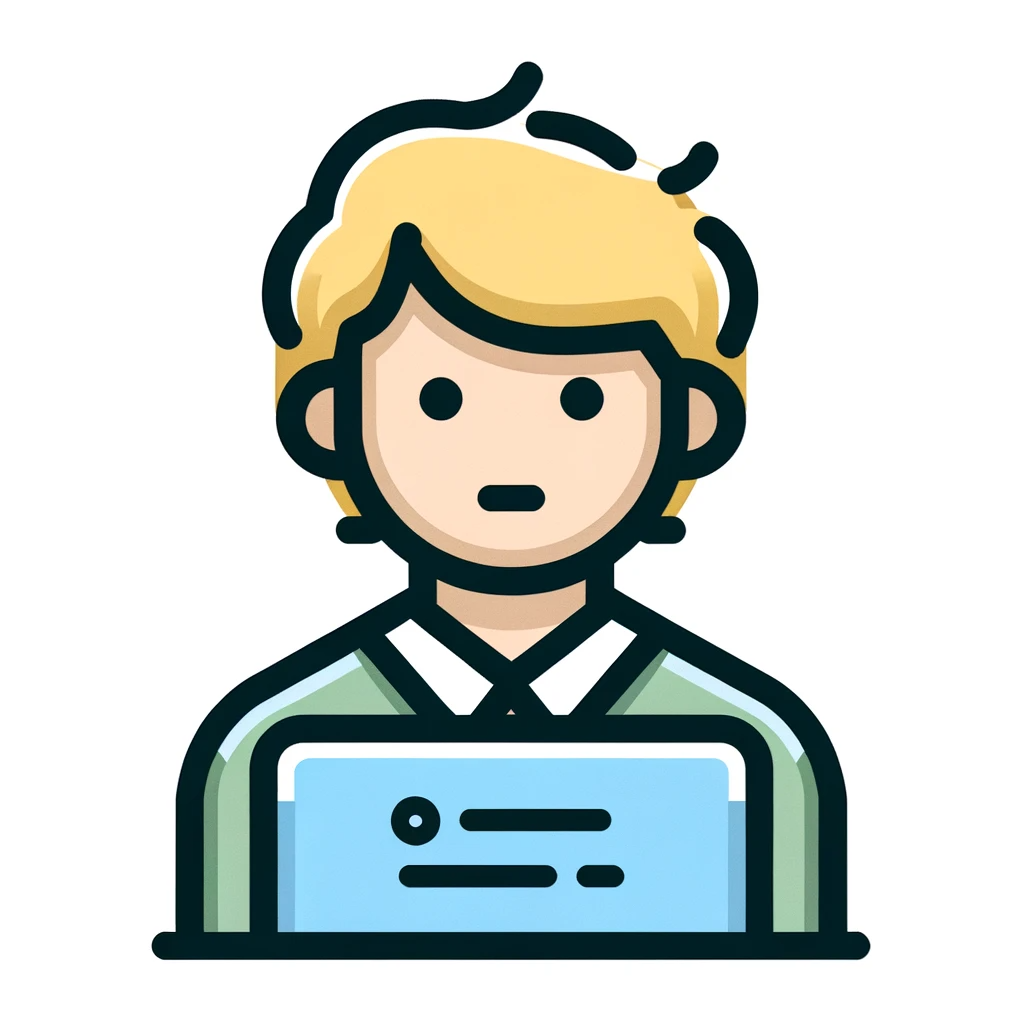
You can now change the appearance of elements using JavaScript!
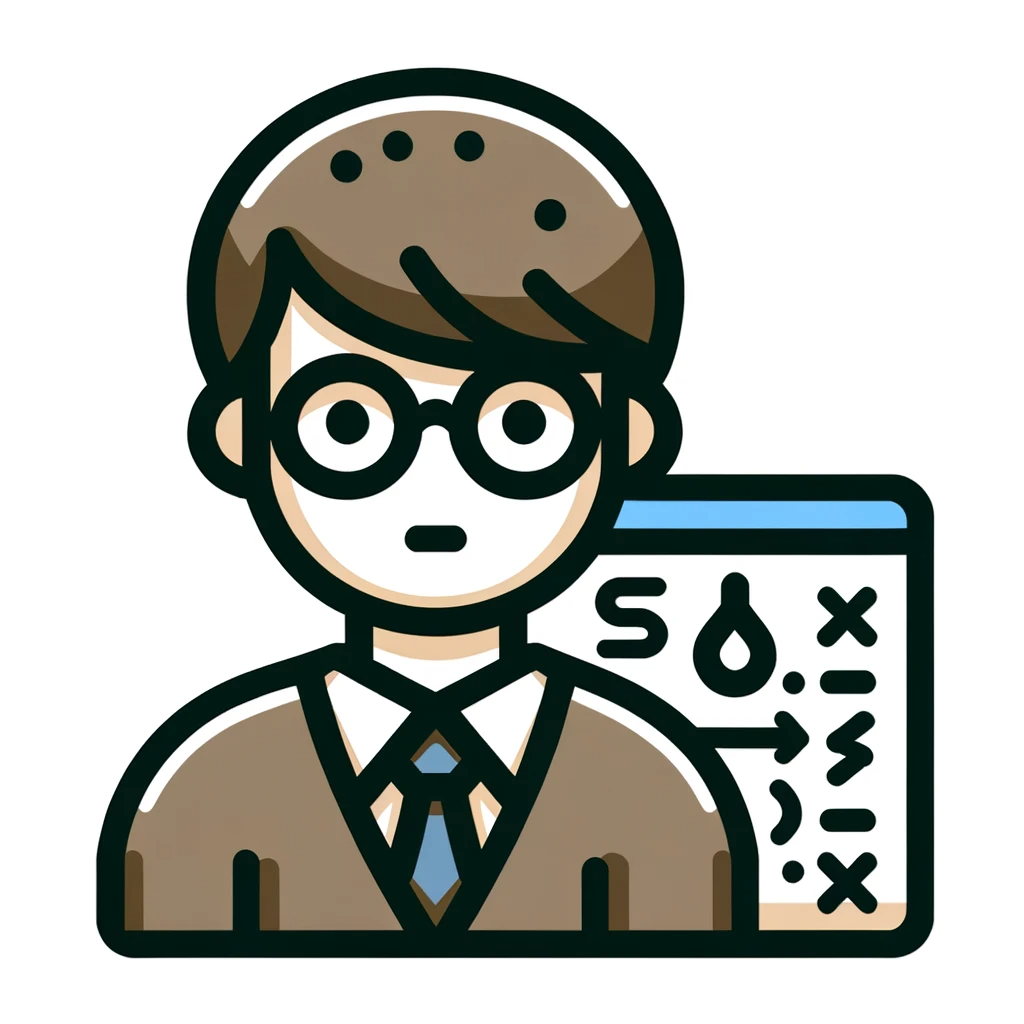
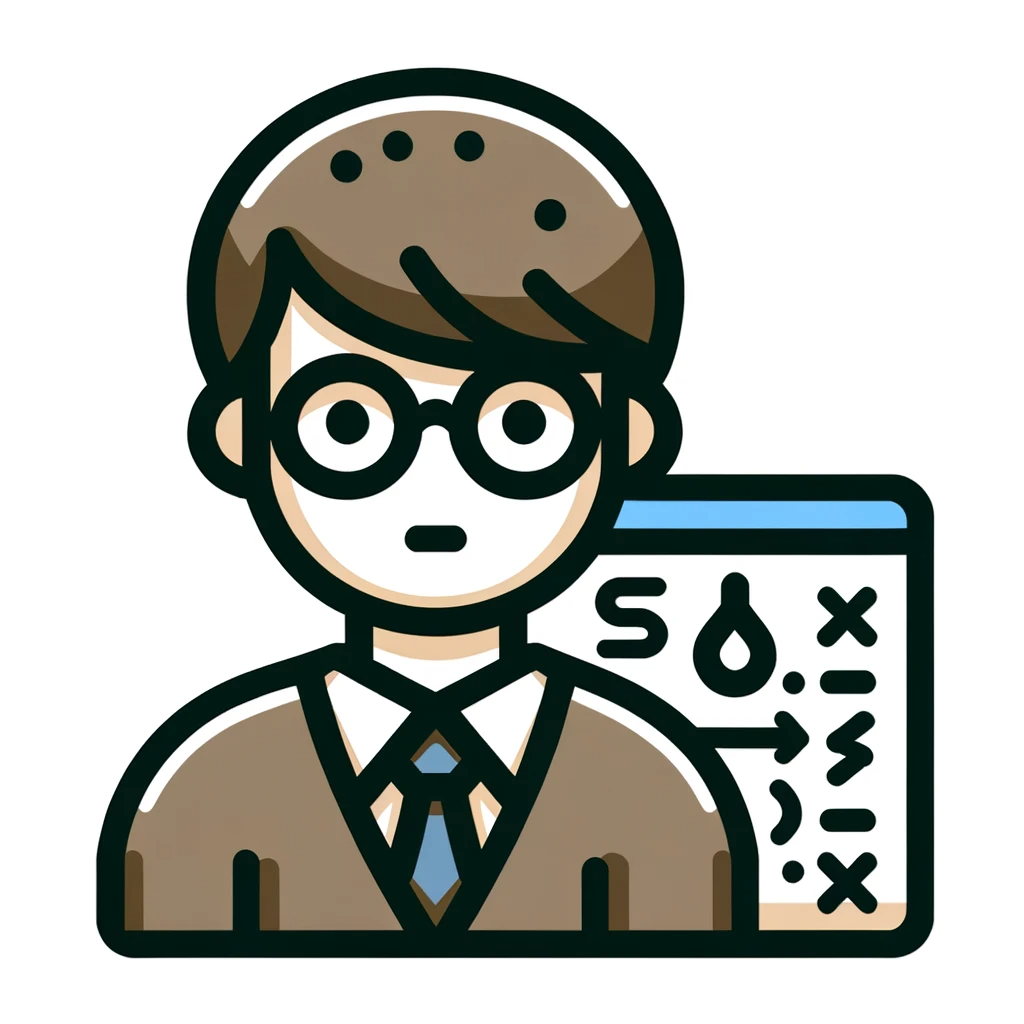
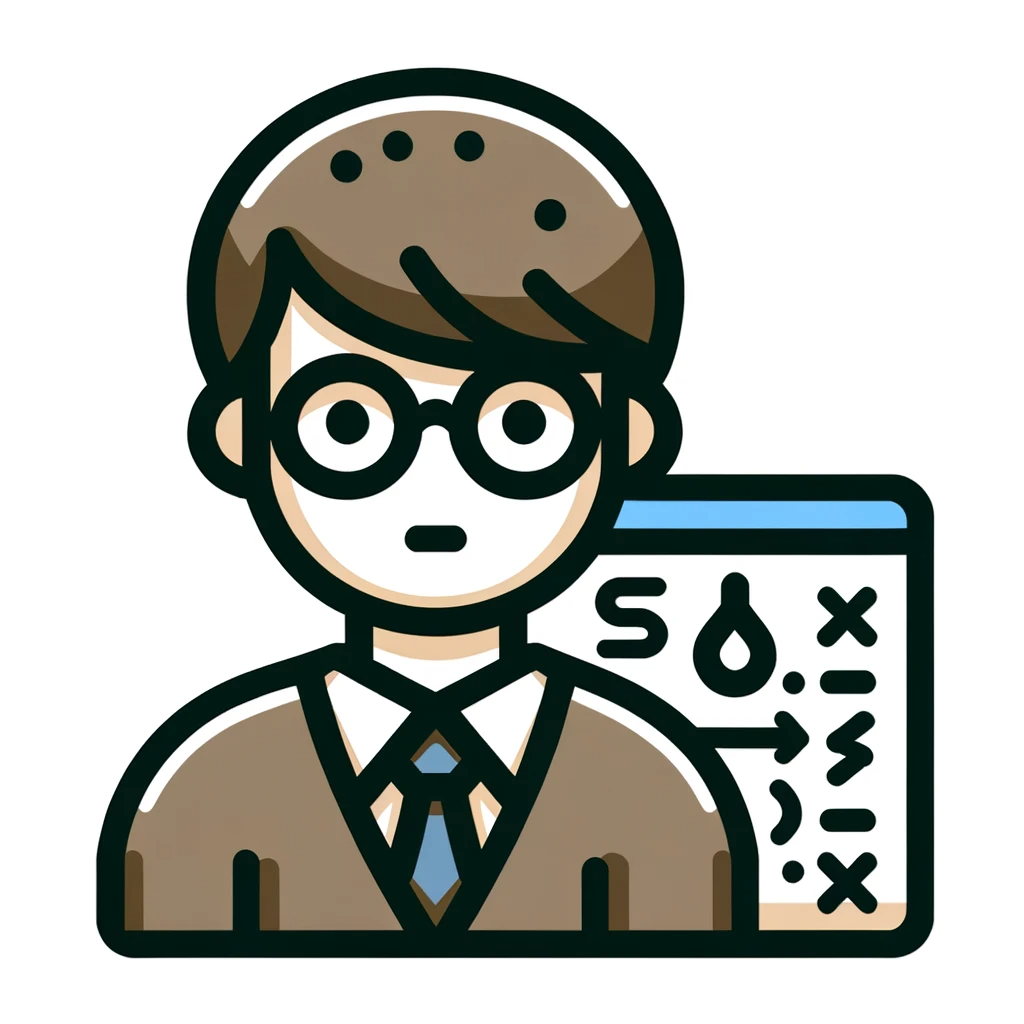
By using JavaScript’s style property, you can dynamically change the appearance of an element.
However, be careful as excessive style changes can have a negative impact on SEO.
Comments