Learn in detail how to browse all the data stored in browser storage using JavaScript.
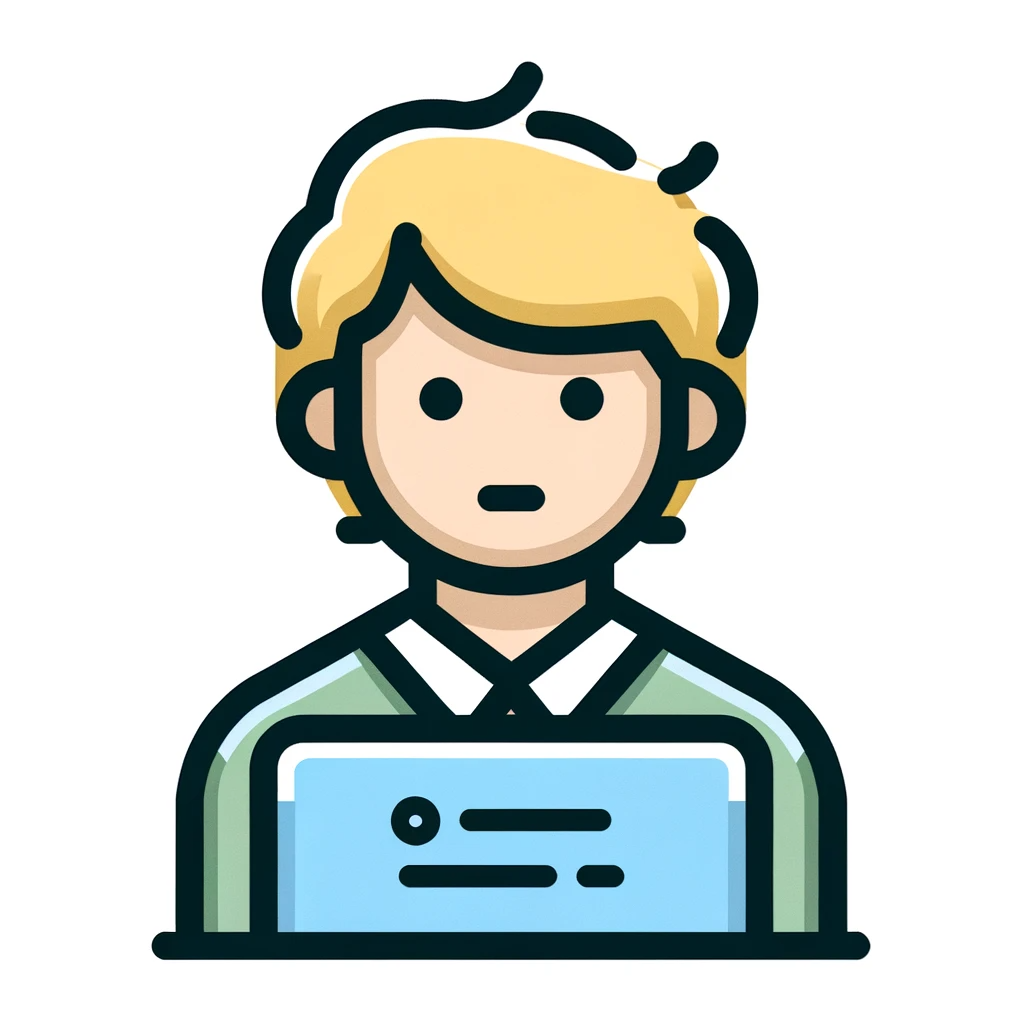
How can I retrieve all data stored in browser storage in JavaScript?
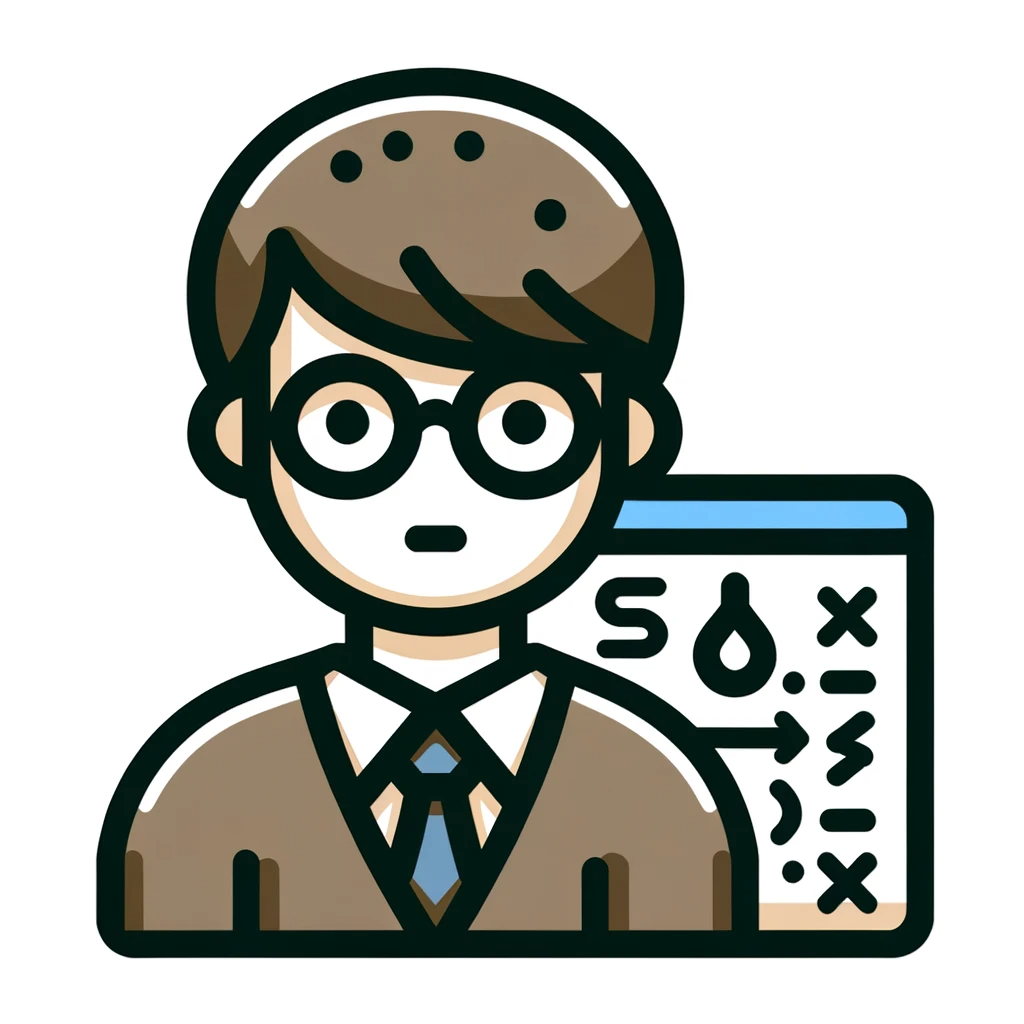
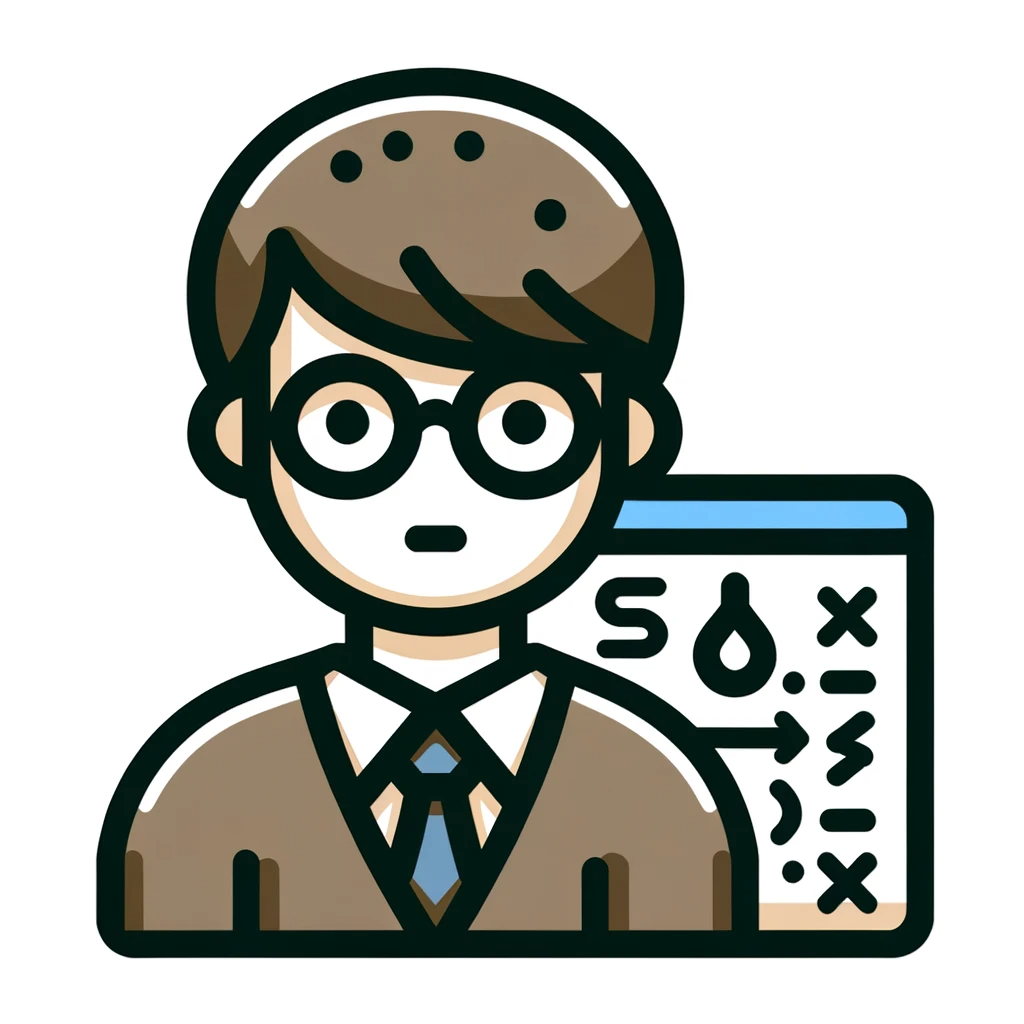
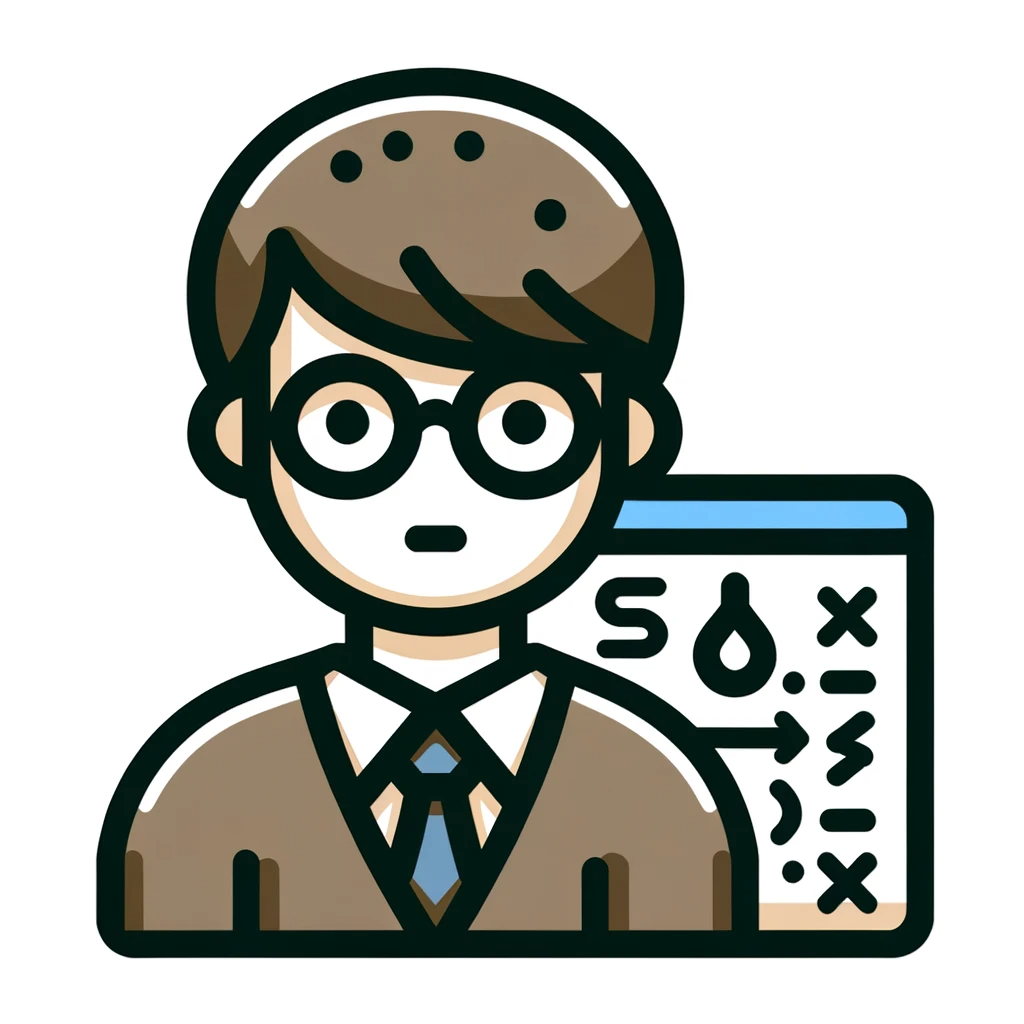
JavaScript supports three types of browser storage: local storage, session storage, and cookies.
Let’s explain how to obtain each.
Storage types and acquisition methods
JavaScript supports three types of browser storage: local storage, session storage, and cookies. The method for acquiring each storage is explained below.
How to retrieve using local storage
Local storage is a storage for saving web application data in the browser, and the data storage period is unlimited. Your data remains even when you close your browser. Access local storage as follows:
// Save data to local storage
localStorage.setItem('key', 'value');
// Retrieve data from local storage
let value = localStorage.getItem('key');
How to retrieve using session storage
Session storage is a storage for saving web application data in the browser, and the data is saved until the session ends. When you close your browser, your session ends and your data is lost. Access session storage as follows:
// Store data in session storage
sessionStorage.setItem('key', 'value');
// Retrieve data from session storage
let value = sessionStorage.getItem('key');
How to obtain using cookies
Cookies are a mechanism for storing web application data in your browser. Cookies have an expiration date, and the data remains until that expiration date. Even if you close your browser, the data will remain if the expiration date remains. Cookies are accessed as follows:
// Storing data in cookies
document.cookie = 'key=value';
// Retrieving data from cookies
let cookies = document.cookie.split(';');
for (let i = 0; i < cookies.length; i++) {
let cookie = cookies[i].trim().split('=');
if (cookie[0] === 'key') {
let value = cookie[1];
}
}
The above is an explanation of the types of browser storage and how to obtain them. Choosing the right storage and retrieval method will help you process your web application’s data smoothly and securely.
Explanation using a sample program
Below, we will explain how to retrieve data from browser storage using a sample program.
Sample program to retrieve data from local storage
The sample program below can display multiple keys and values stored in local storage in a table format.
function showLocalStorage() {
let table = document.createElement('table');
let header = table.insertRow(0);
let keyHeader = header.insertCell(0);
let valueHeader = header.insertCell(1);
keyHeader.innerHTML = "<b>Key</b>";
valueHeader.innerHTML = "<b>Value</b>";
for (let i = 0; i < localStorage.length; i++) {
let key = localStorage.key(i);
let value = localStorage.getItem(key);
let row = table.insertRow(i + 1);
let keyCell = row.insertCell(0);
let valueCell = row.insertCell(1);
keyCell.innerHTML = key;
valueCell.innerHTML = value;
}
document.body.appendChild(table);
}
In this sample program, you can display all keys and values stored in local storage in a table format by calling the showLocalStorage() function. I’m using a localStorage object to retrieve key-value pairs and display each value in a table cell.
Sample program to retrieve data from session storage
The sample program below allows you to display multiple keys and values stored in session storage in table format.
function showSessionStorage() {
let table = document.createElement('table');
let header = table.insertRow(0);
let keyHeader = header.insertCell(0);
let valueHeader = header.insertCell(1);
keyHeader.innerHTML = "<b>Key</b>";
valueHeader.innerHTML = "<b>Value</b>";
for (let i = 0; i < sessionStorage.length; i++) {
let key = sessionStorage.key(i);
let value = sessionStorage.getItem(key);
let row = table.insertRow(i + 1);
let keyCell = row.insertCell(0);
let valueCell = row.insertCell(1);
keyCell.innerHTML = key;
valueCell.innerHTML = value;
}
document.body.appendChild(table);
}
In this sample program, you can display all keys and values stored in session storage in table format by calling the showSessionStorage() function. I’m using a sessionStorage object to retrieve key-value pairs and display each value in a table cell.
Sample program to retrieve data from cookies
The sample program below can display the cookie values stored in the browser in table format.
function showCookie() {
let table = document.createElement('table');
let header = table.insertRow(0);
let nameHeader = header.insertCell(0);
let valueHeader = header.insertCell(1);
nameHeader.innerHTML = "<b>Name</b>";
valueHeader.innerHTML = "<b>Value</b>";
let cookies = document.cookie.split(';');
for (let i = 0; i < cookies.length; i++) {
let cookie = cookies[i].trim().split('=');
let row = table.insertRow(i + 1);
let nameCell = row.insertCell(0);
let valueCell = row.insertCell(1);
nameCell.innerHTML = cookie[0];
valueCell.innerHTML = cookie[1];
}
document.body.appendChild(table);
}
In this sample program, you can display the values of all cookies stored in the browser in table format by calling the showCookie() function. I am using document.cookie to retrieve the cookie values and display each value in a table cell.
summary
We explained how to refer to all data saved in browser storage.
- There are three types of browser storage: local storage, session storage, and cookies, and each needs to be used appropriately.
- Local storage allows you to store and retrieve key-value pairs using the localStorage object.
- For session storage, you can use the sessionStorage object to store and retrieve key-value pairs.
- Cookies can be used to store and retrieve key-value pairs using document.cookie.
- Choosing the right storage and retrieval method will help you process your web application’s data smoothly and securely.
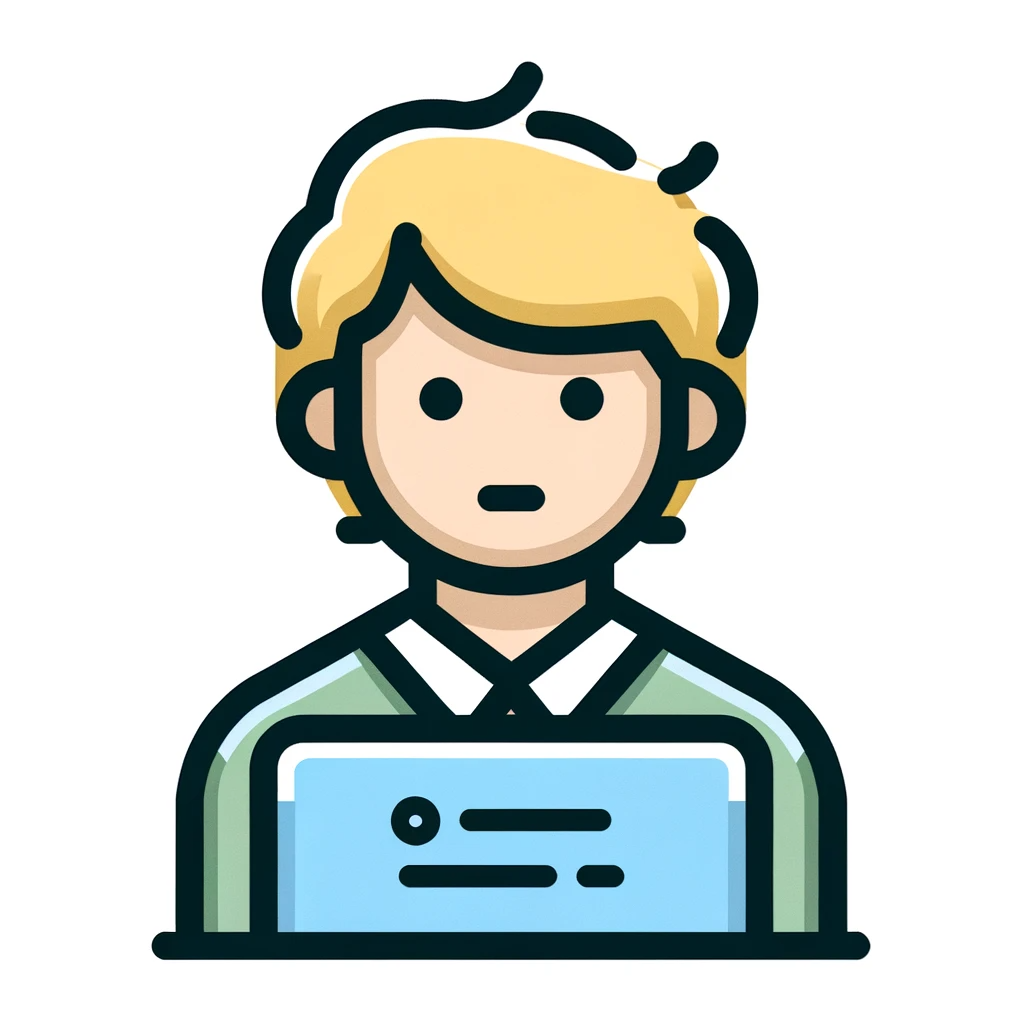
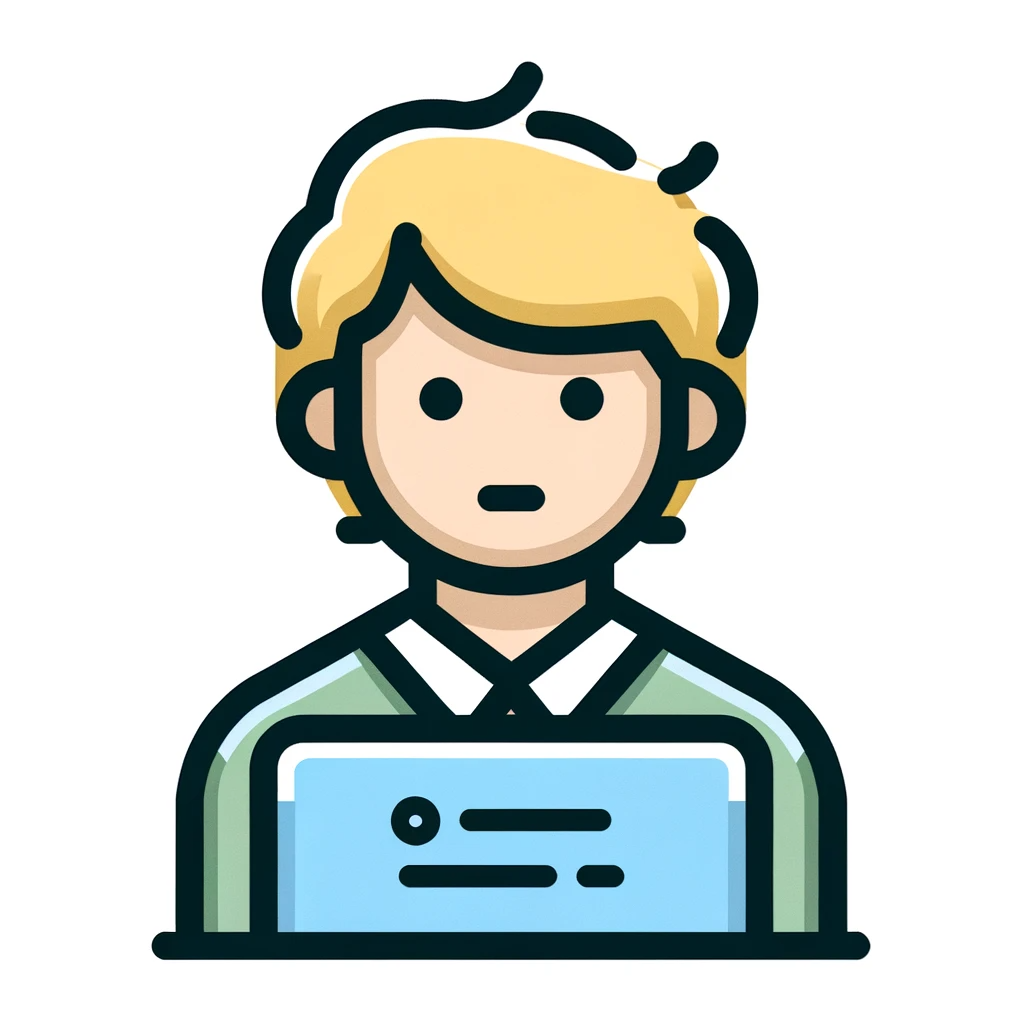
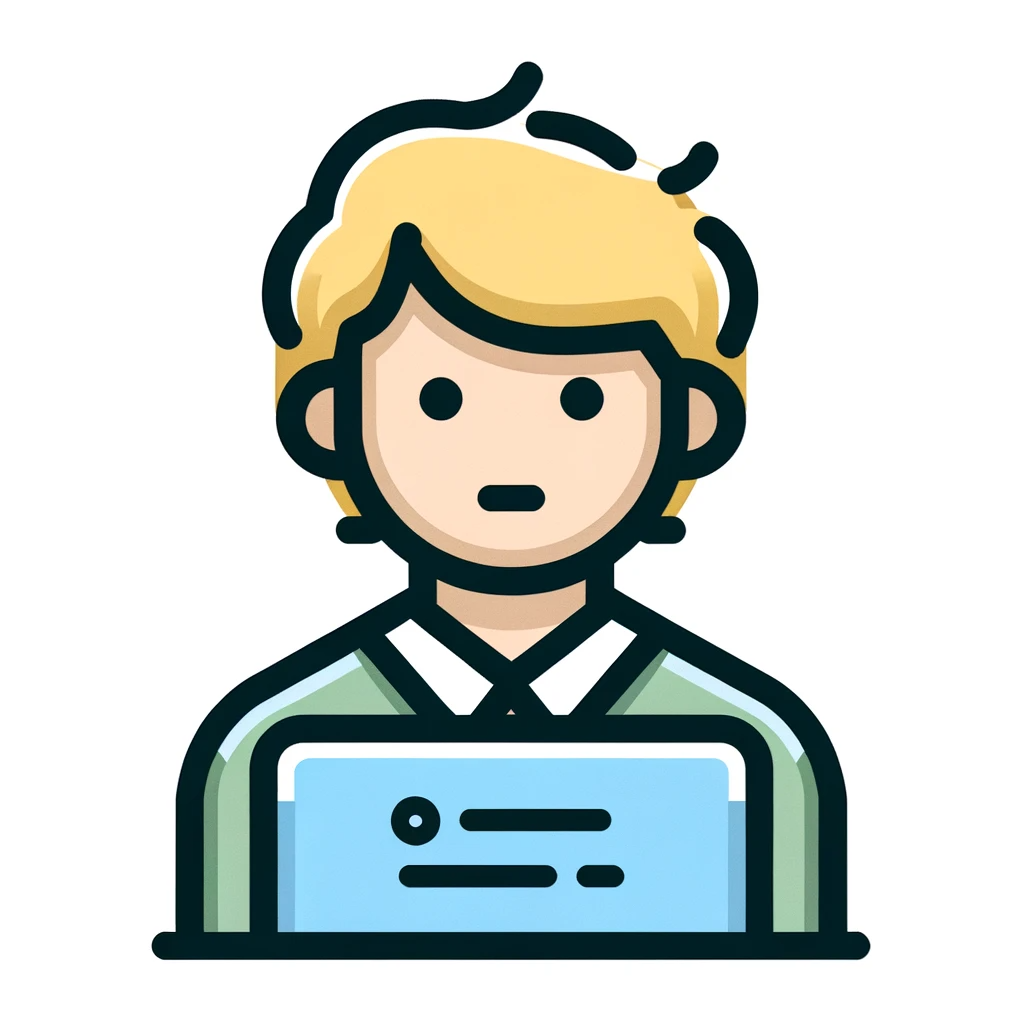
Now you know how to retrieve data from browser storage!
It was very helpful to understand the types of browser storage and how to obtain each type.
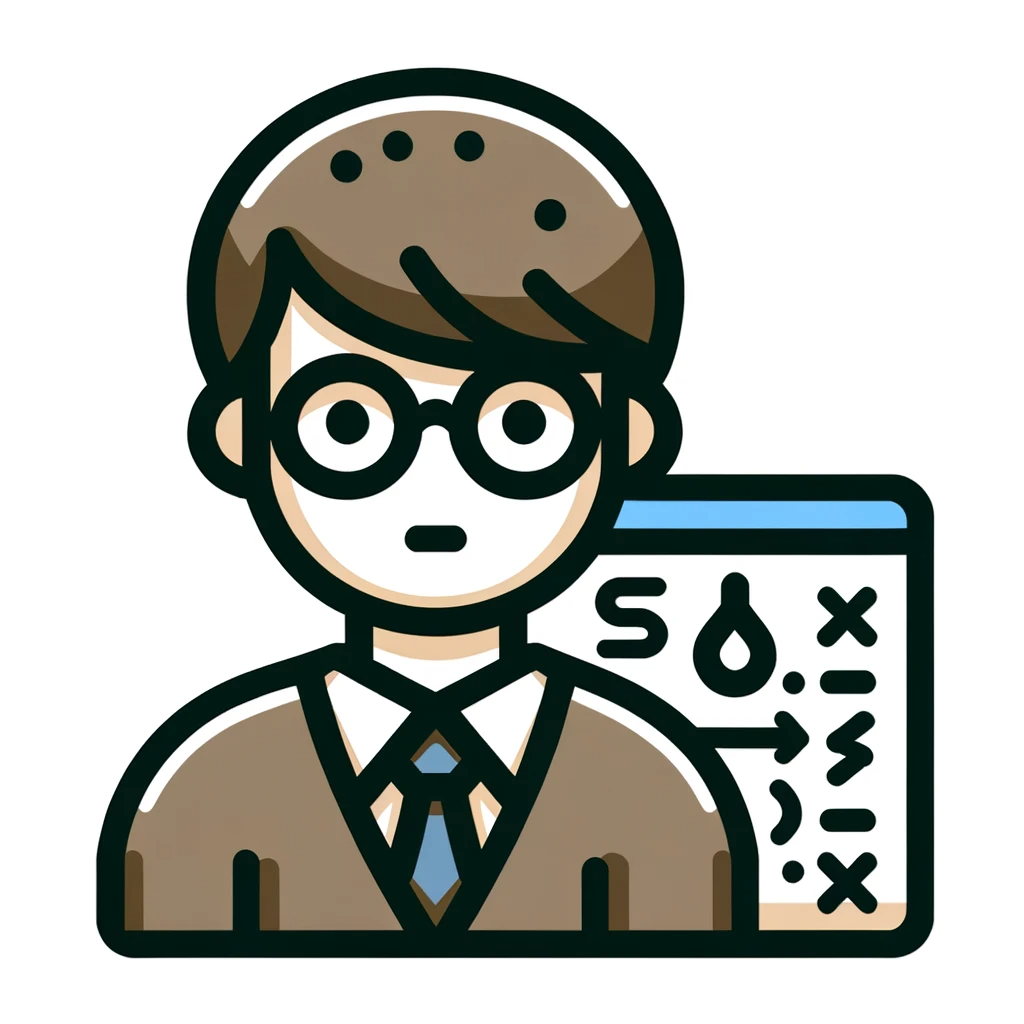
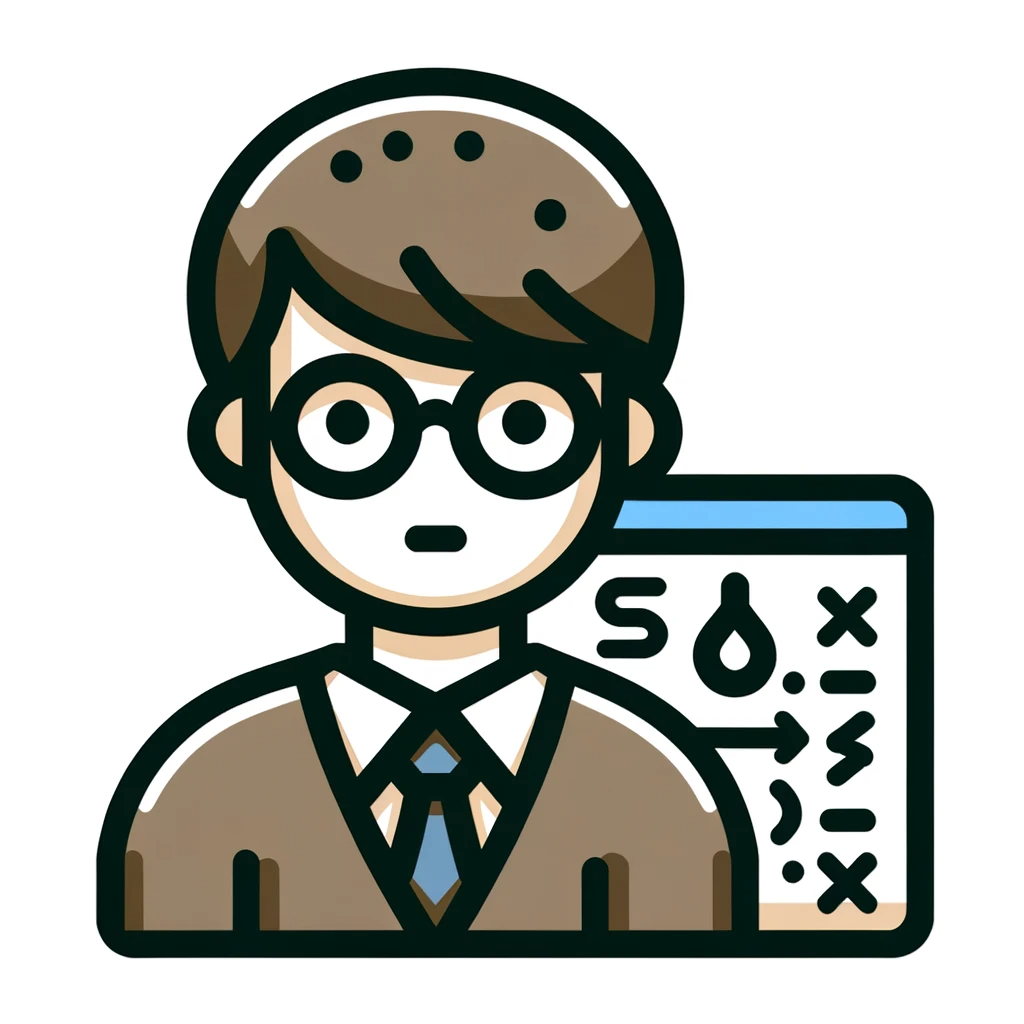
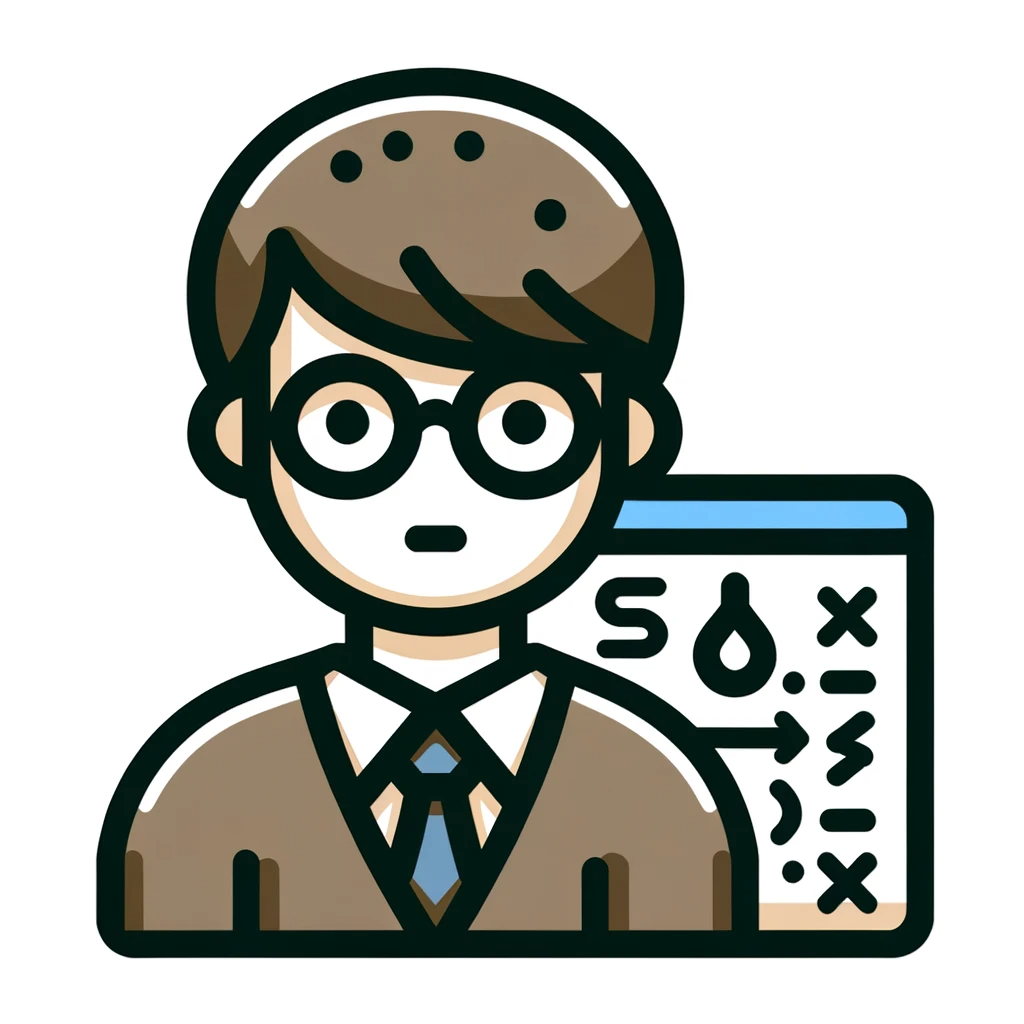
Browser storage allows you to store user data on the browser and improve the performance of your application.
However, the data stored in storage is stored within the user’s browser, so you should also be aware of the security risks.
Please be sure to properly process the information you save in storage.
Comments