This article explains how to replace elements in an array using JavaScript.
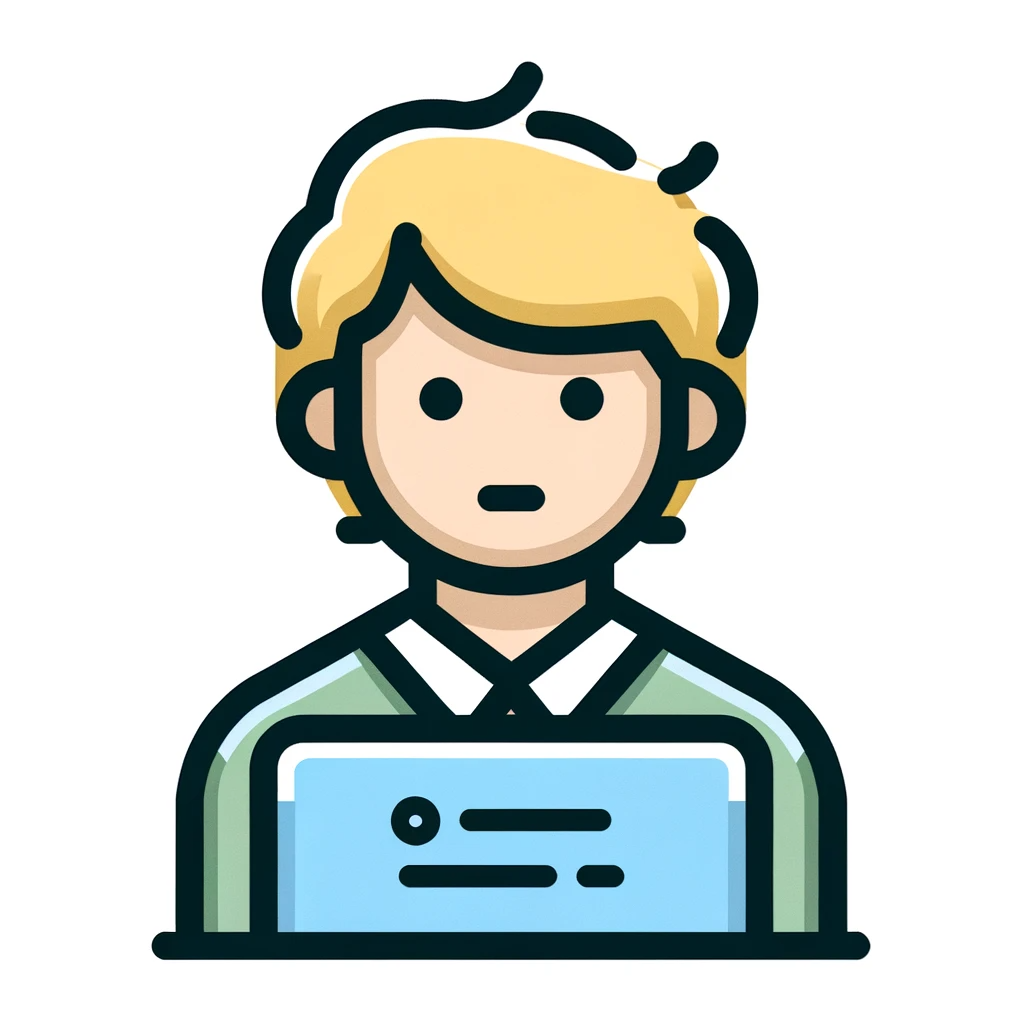
Is there a way to replace elements in an array in JavaScript?
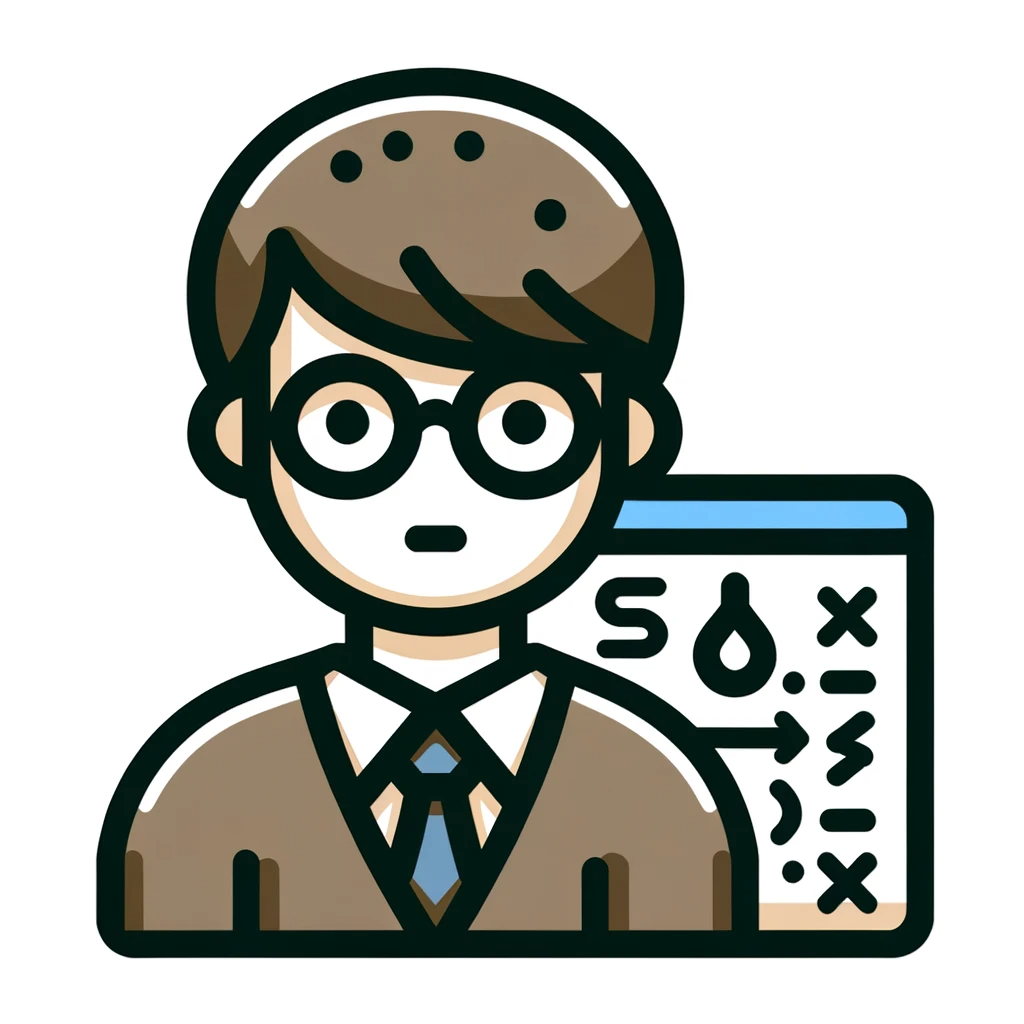
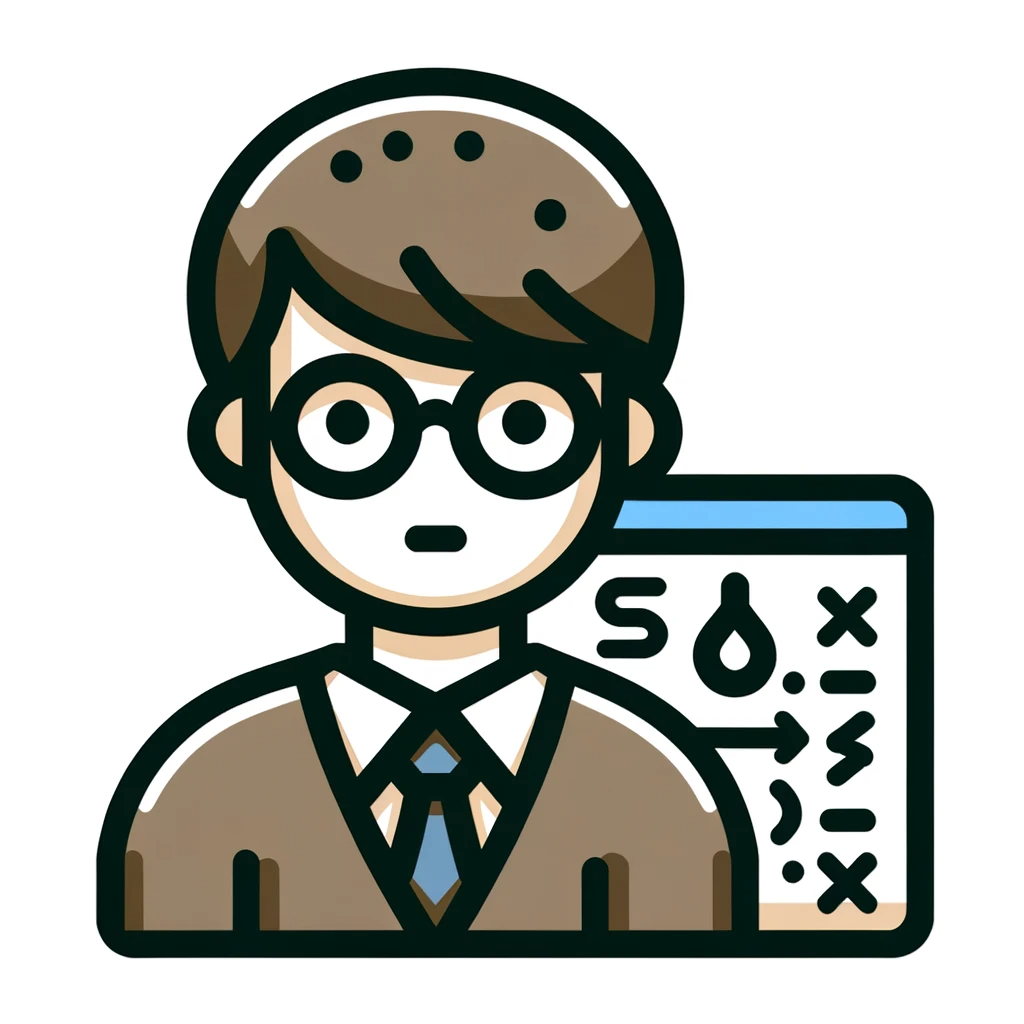
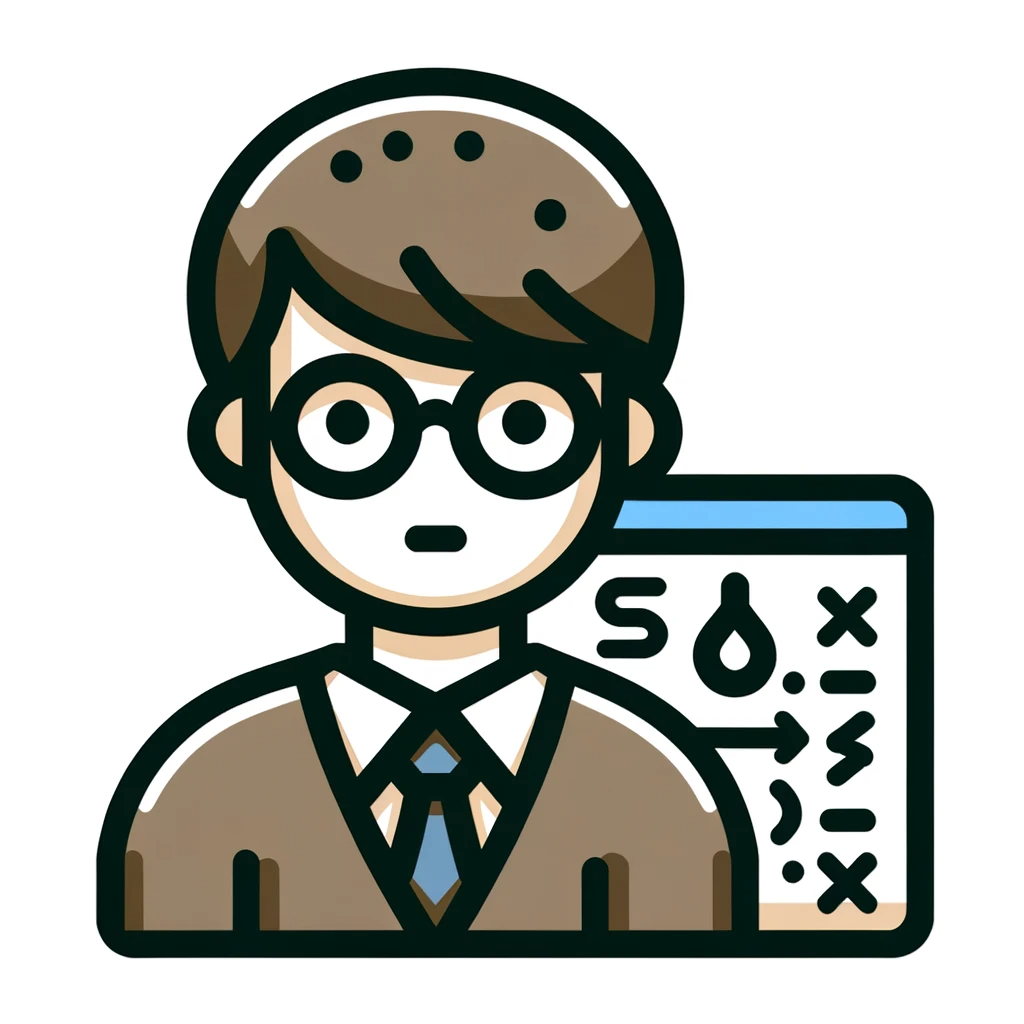
You can easily do this using the splice method.
How to replace elements in an array using the splice method
The easiest way to replace elements in an array in JavaScript is to use the splice method .
The splice method can remove an element from any position in an array and insert a new element at that position.
array.splice(starting position, number of elements [, element after replacement, …])
For example, if you want to replace the third element of the array “[1, 2, 3, 4, 5]” with “6”, write as follows.
var array = [1, 2, 3, 4, 5];
array.splice(2, 1, 6);
console.log(array); // [1, 2, 6, 4, 5]
In the above example, splice(2, 1, 6) is written. We are removing 1 from the second element and inserting 6.
How to replace elements of an array using the spread operator
In addition to the splice method, you can also use the spread operator to replace elements in an array.
For example, if you want to replace the third element of the array “[1, 2, 3, 4, 5]” with “6”, write as follows.
var array = [1, 2, 3, 4, 5];
array = [...array.slice(0, 2), 6, ...array.slice(3)];
console.log(array); // [1, 2, 6, 4, 5]
In the above example, we use the spread operator to split the array and insert new elements.
This performs a DeepCopy of the array rather than the splice method, so a method using the spread operator may be recommended.
Summary of this article
We explained how to replace an array element with a specified element.
- You can use the splice method to replace elements in an array.
- The first argument of the splice method specifies the index of the element to be replaced.
- The second argument of the splice method specifies the number of elements to replace.
- Specify the new element to be replaced after the third argument of the splice method.
- The splice method returns the replaced elements.
- The spread operator allows you to replace elements while duplicating an array.
The splice method is a very useful method, so be sure to remember it when working with arrays.
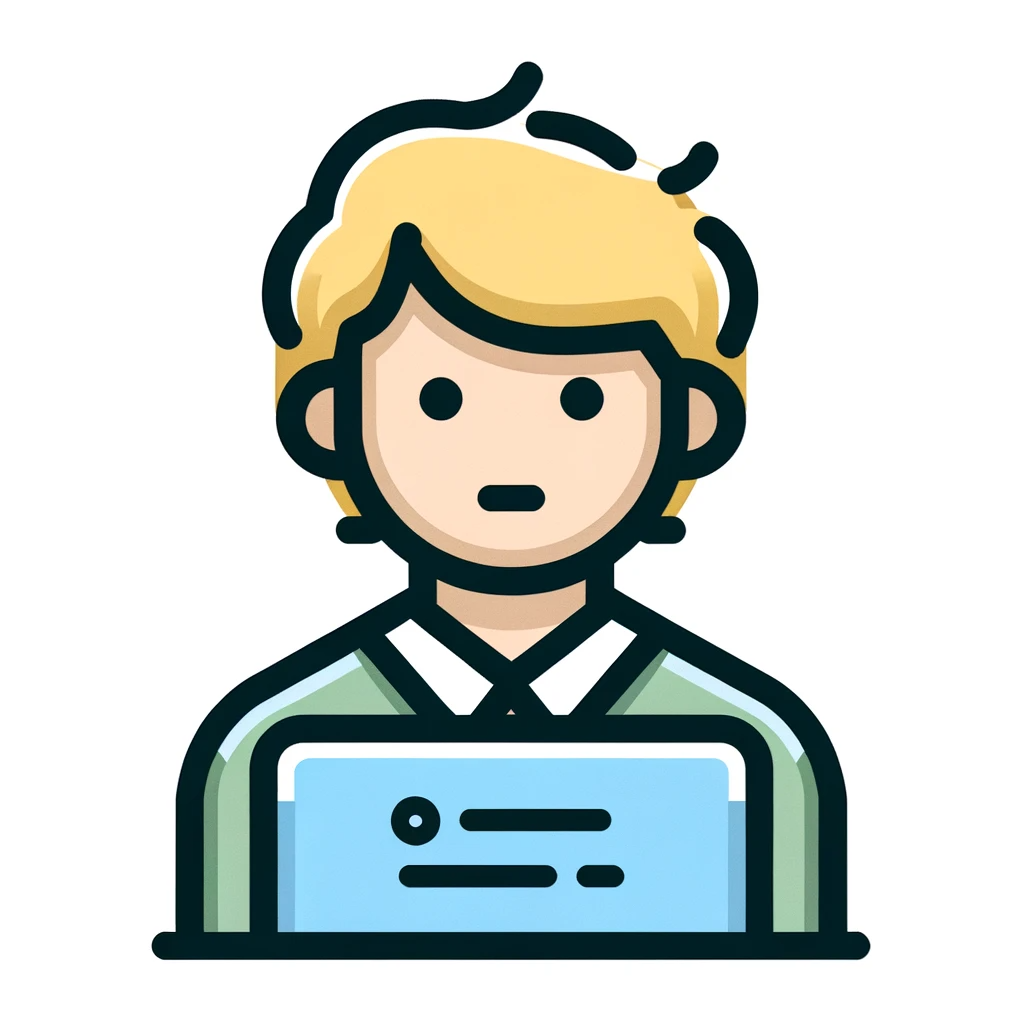
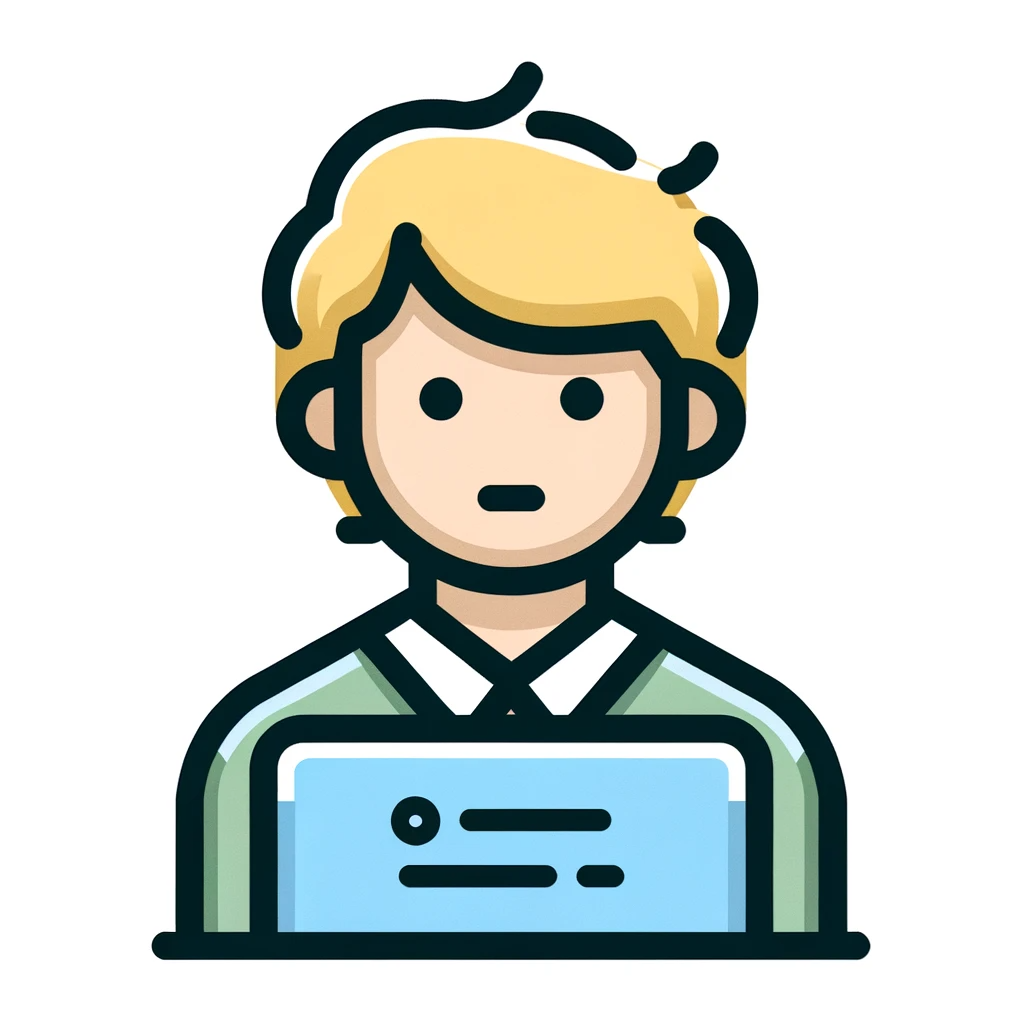
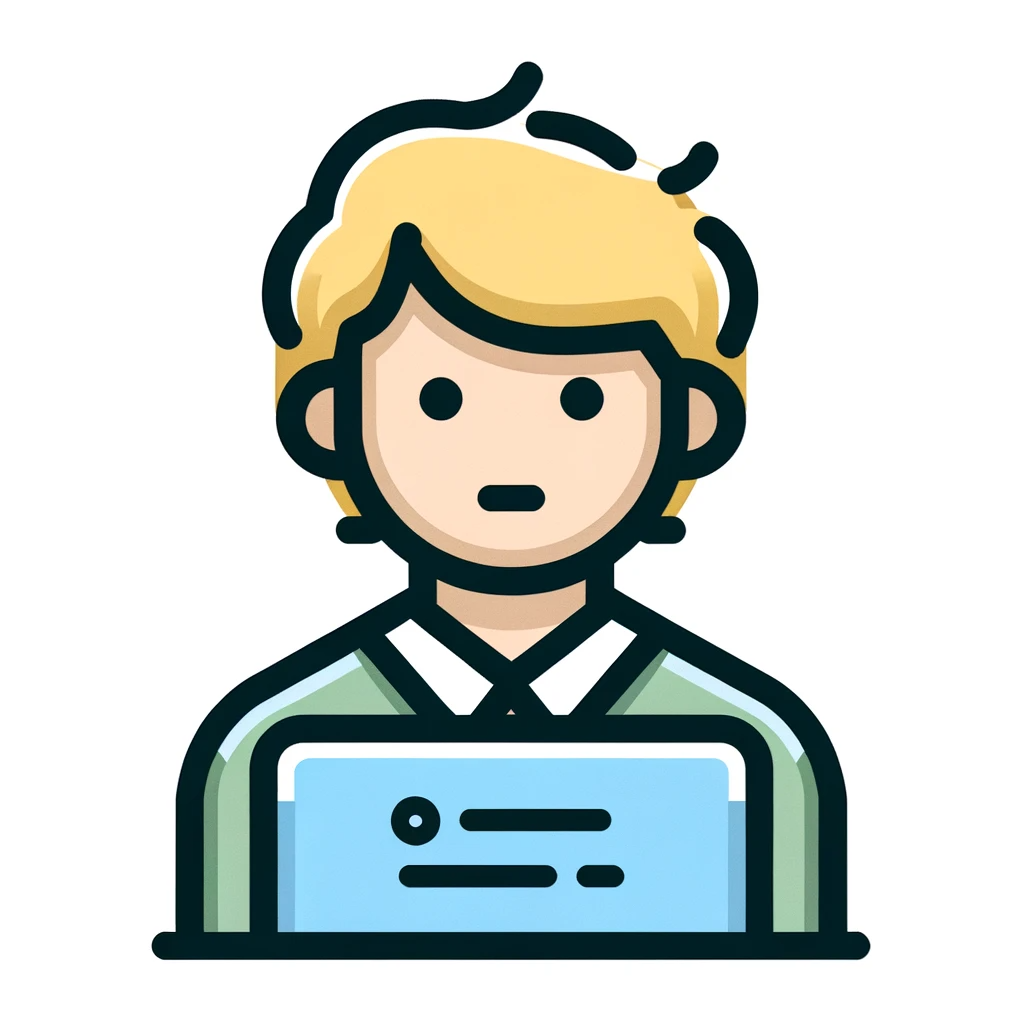
The splice method is easy to use and returns replaced elements, which is very convenient!
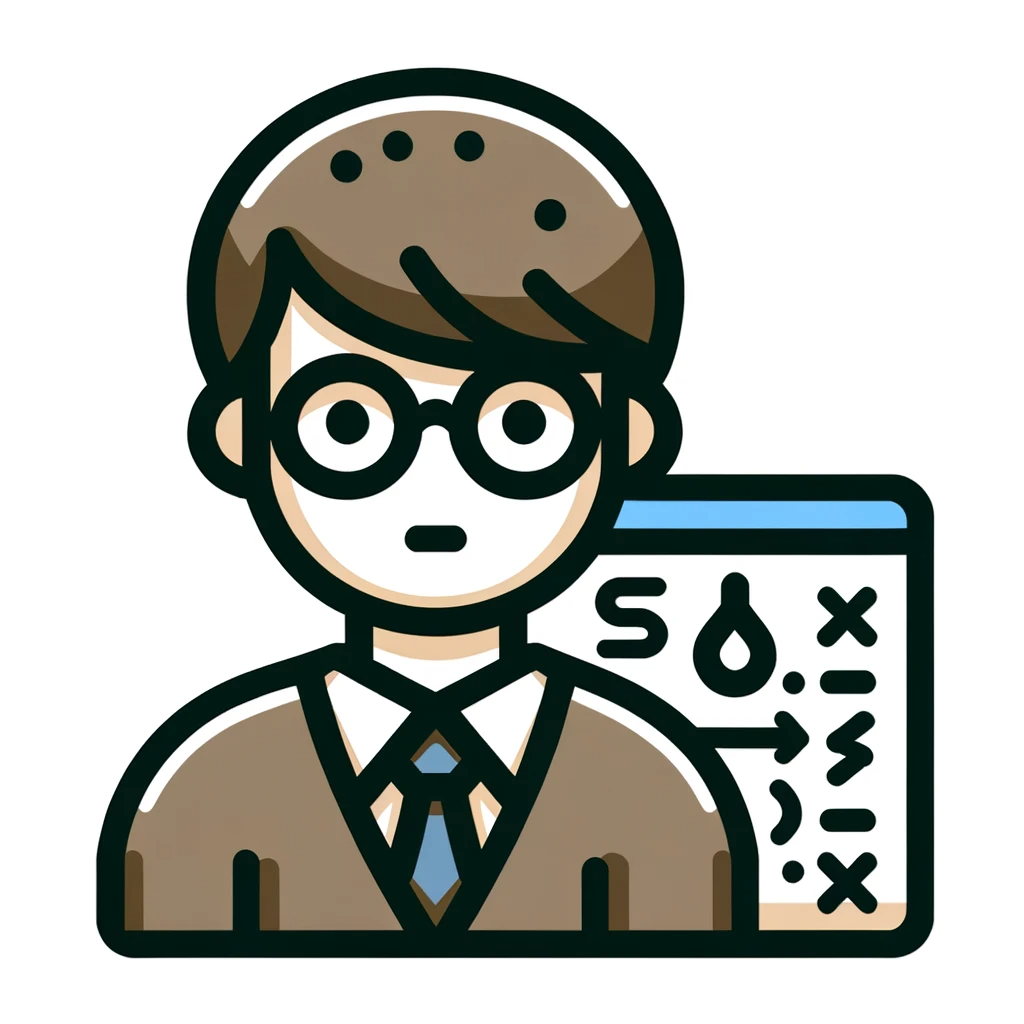
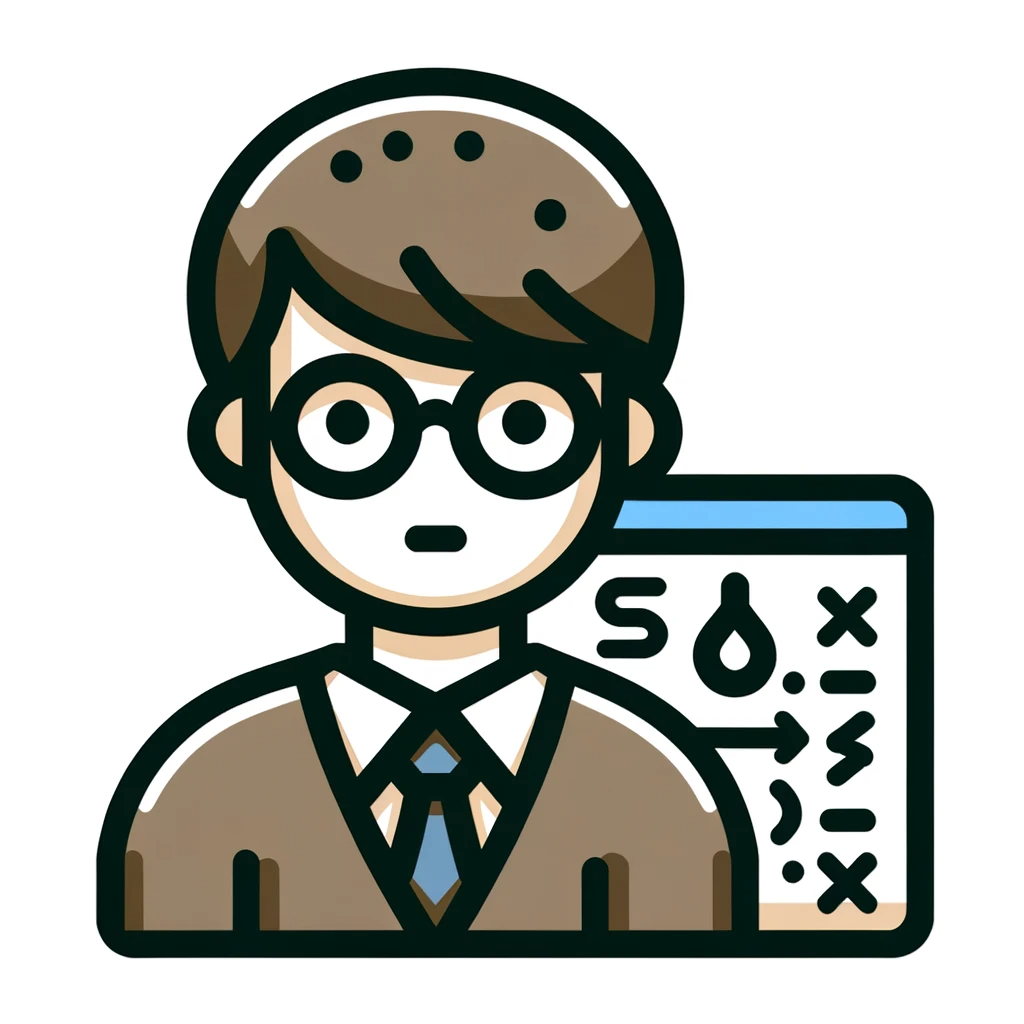
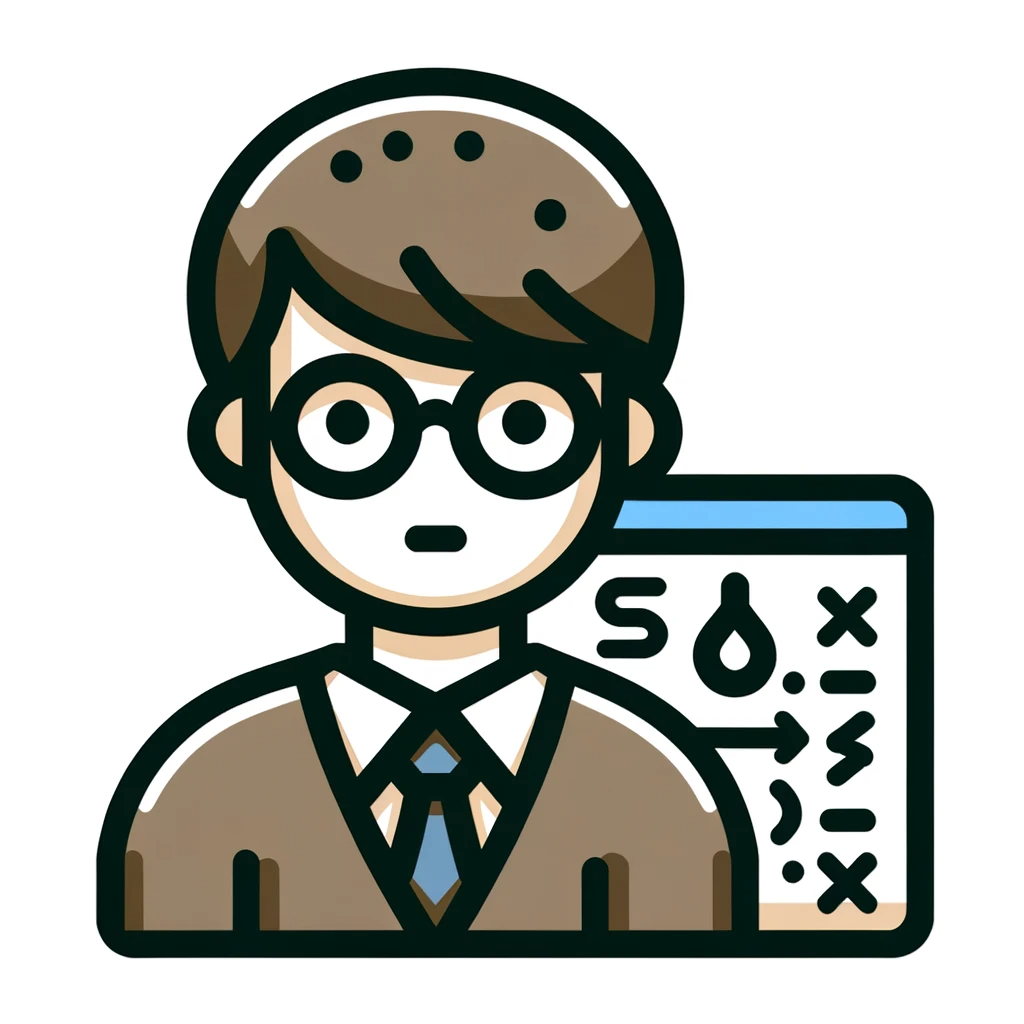
When working with arrays, always remember to check the preservation and duplication of the original array.
Comments