This article explains how to retrieve a specific range of elements in an array using JavaScript.
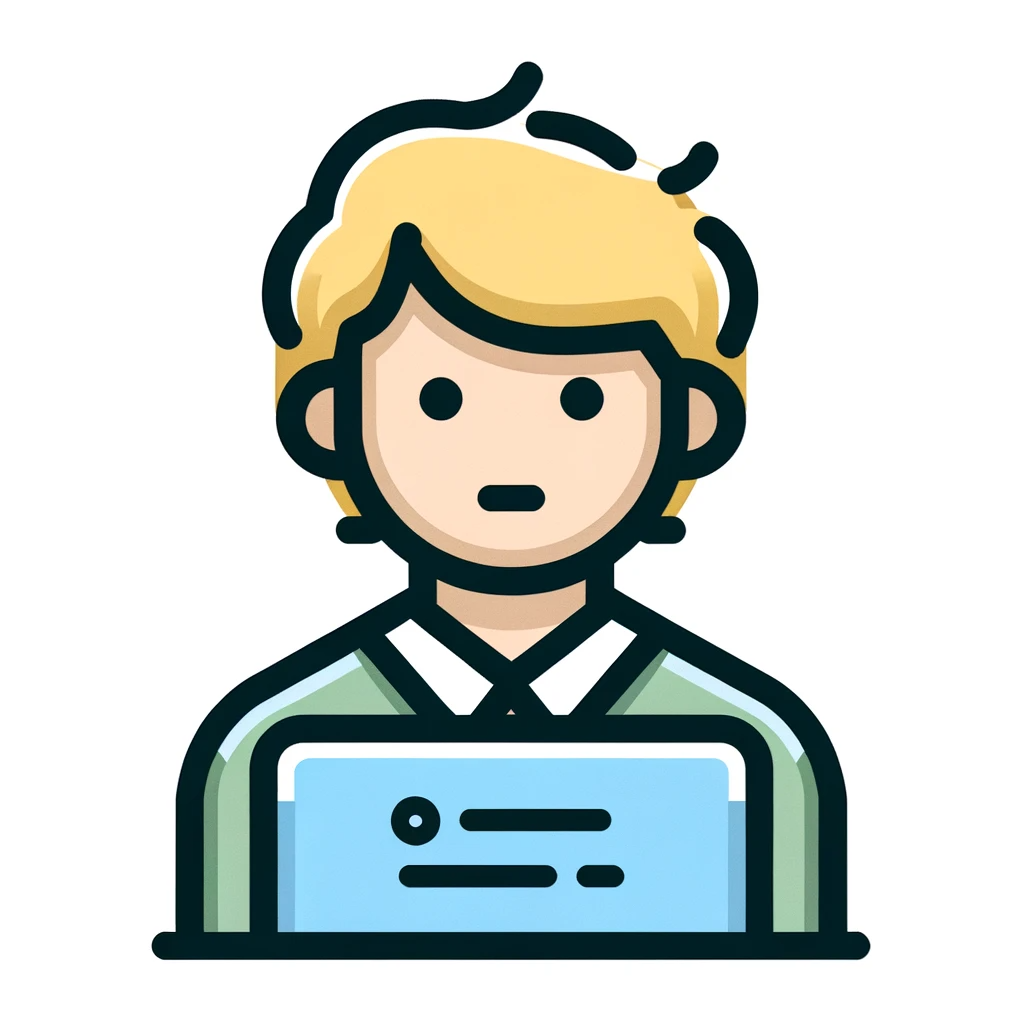
How can I get a specific range of elements in an array?
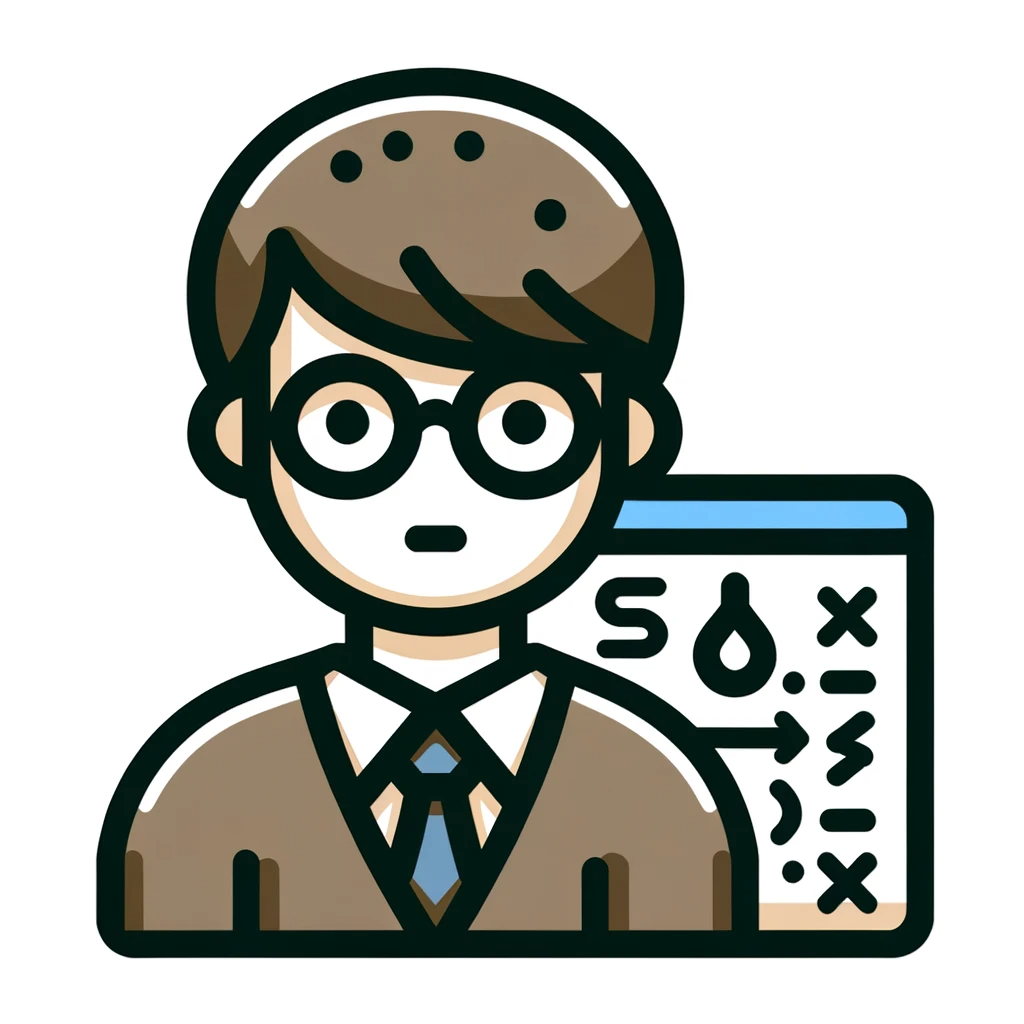
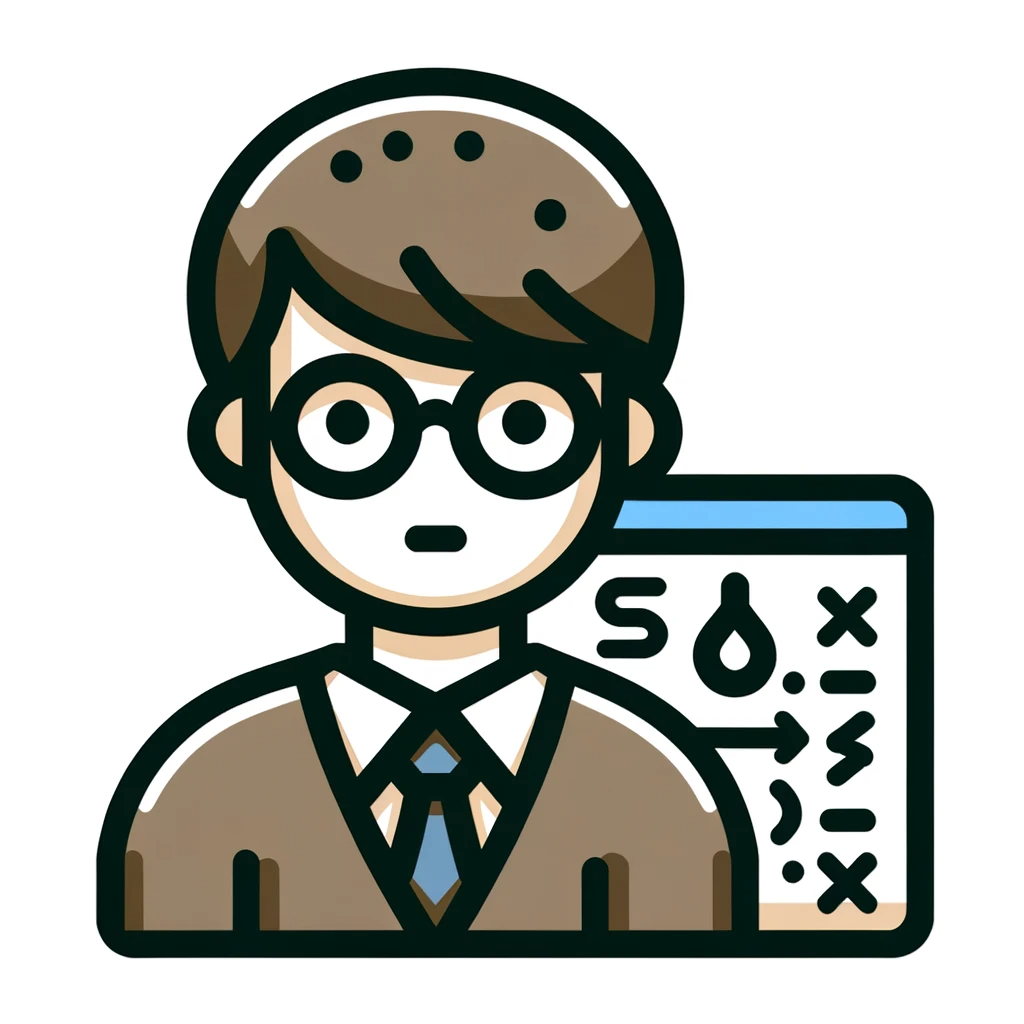
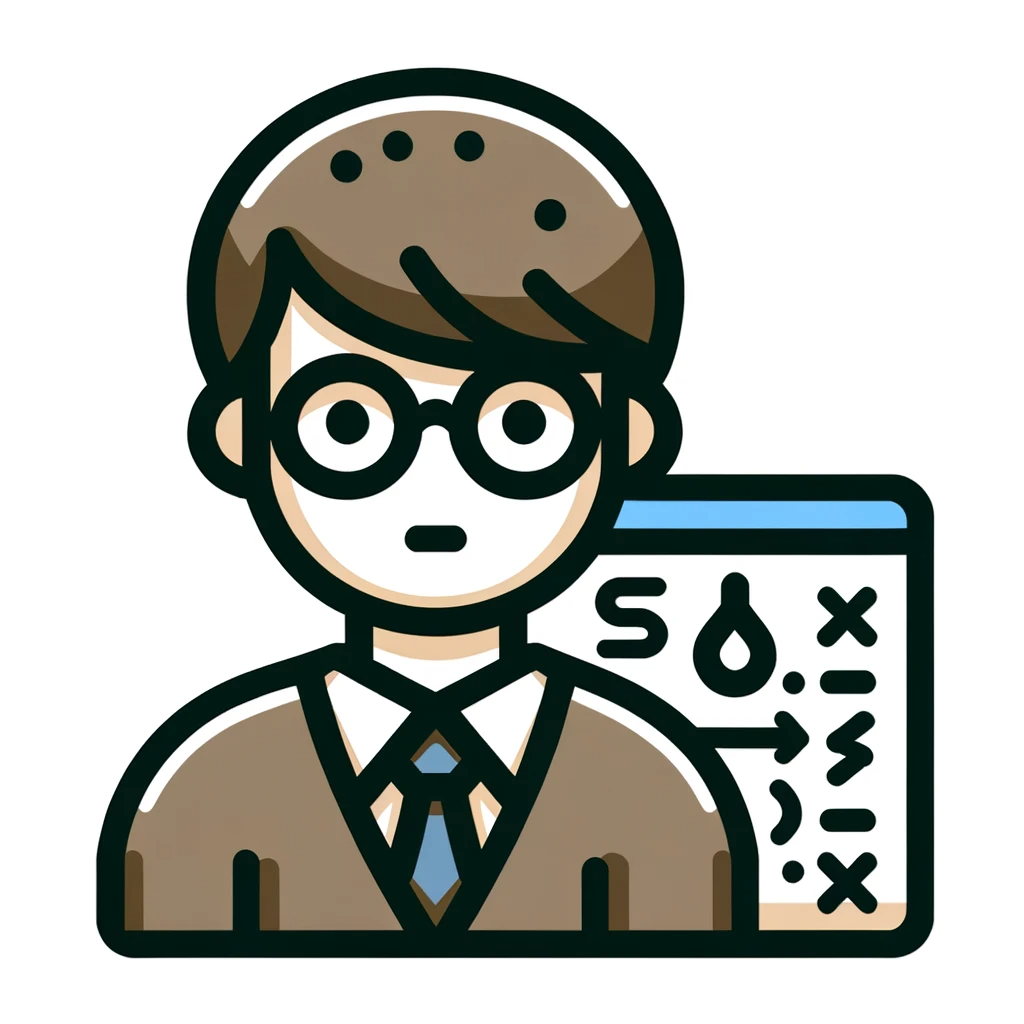
To get a specific range of elements in an array, you can use the slice method.
Obtaining a specific range of elements in an array using the slice method
To get a specific range of elements from an array, you can use the slice method. The slice method extracts a specific range of elements from an array and creates a new array.
The slice() method is used by specifying the array index number. The first argument specifies the starting position of the element you want to extract, and the second argument specifies the ending position of the element you want to extract.
array.slice(start position [, end position])
let fruits = ["apple", "banana", "orange", "mango"];
let selectedFruits = fruits.slice(1, 3);
console.log(selectedFruits); // ["banana", "orange"]
In the above example, the elements in the range of index numbers 1 to 3 are extracted from the array fruits and a new array selectedFruits is created.
Get the element from the position counting from the back
You can also specify a position counted from the back by specifying a negative number as the first argument.
For example, to extract the first element from the end from the third element from the end, specify slice(-3,-1). Also, if the second argument is omitted, it will extract up to the end of the array.
let fruits = ["apple", "banana", "orange", "mango"];
let selectedFruits = fruits.slice(-2);
console.log(selectedFruits); // ["orange", "mango"]
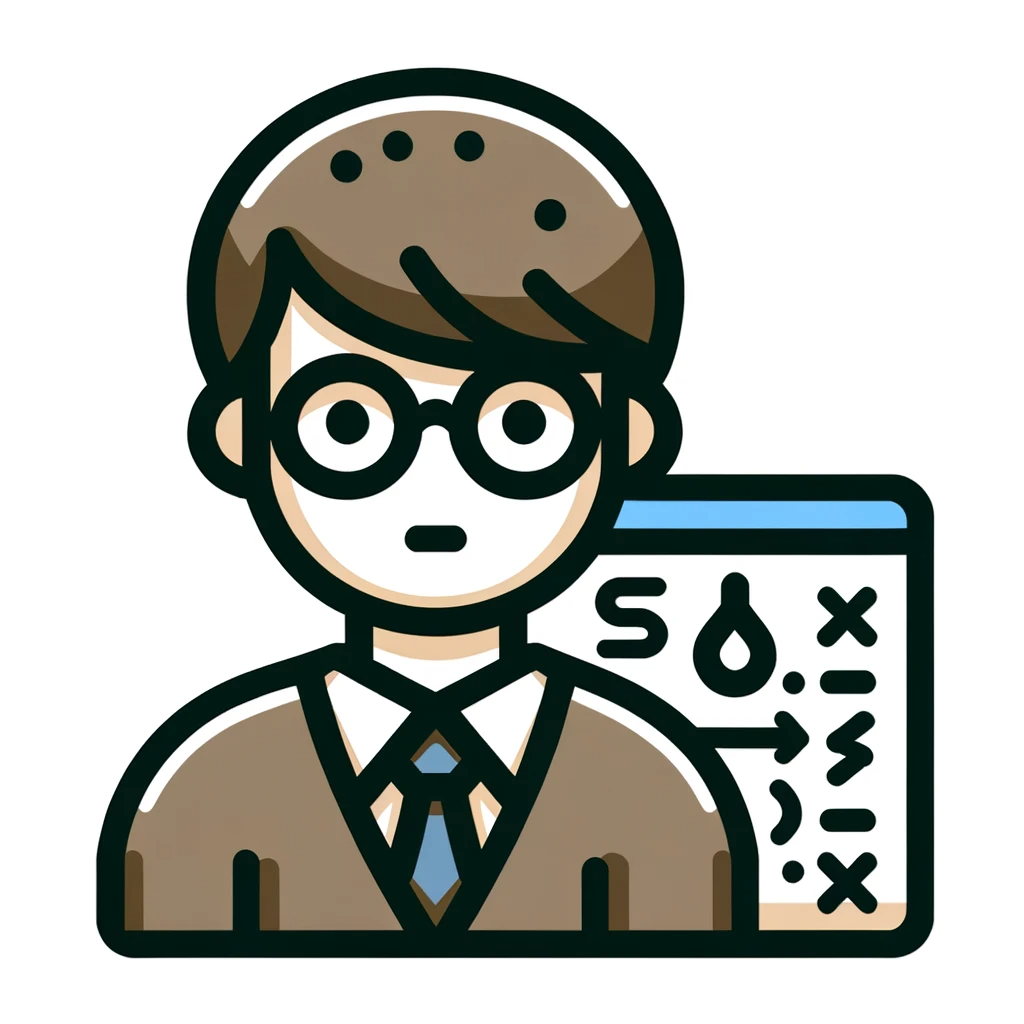
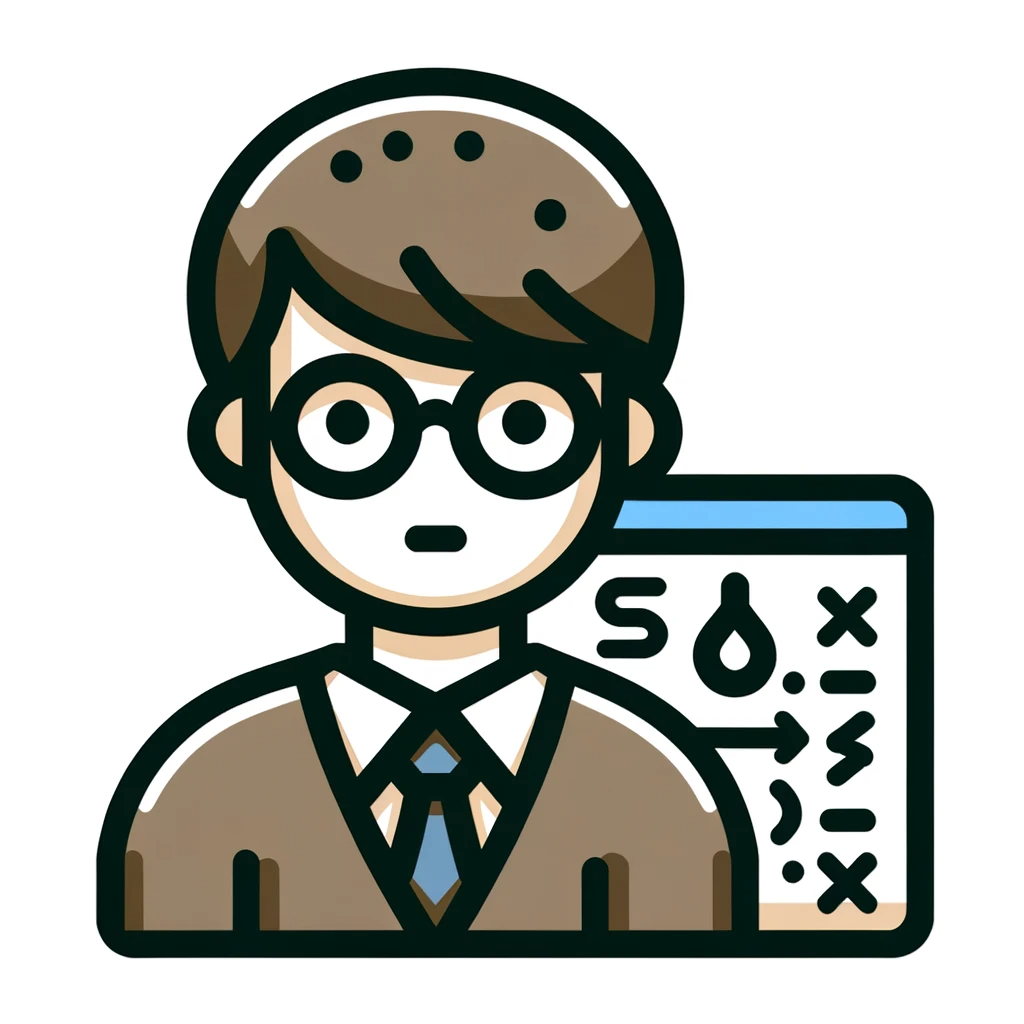
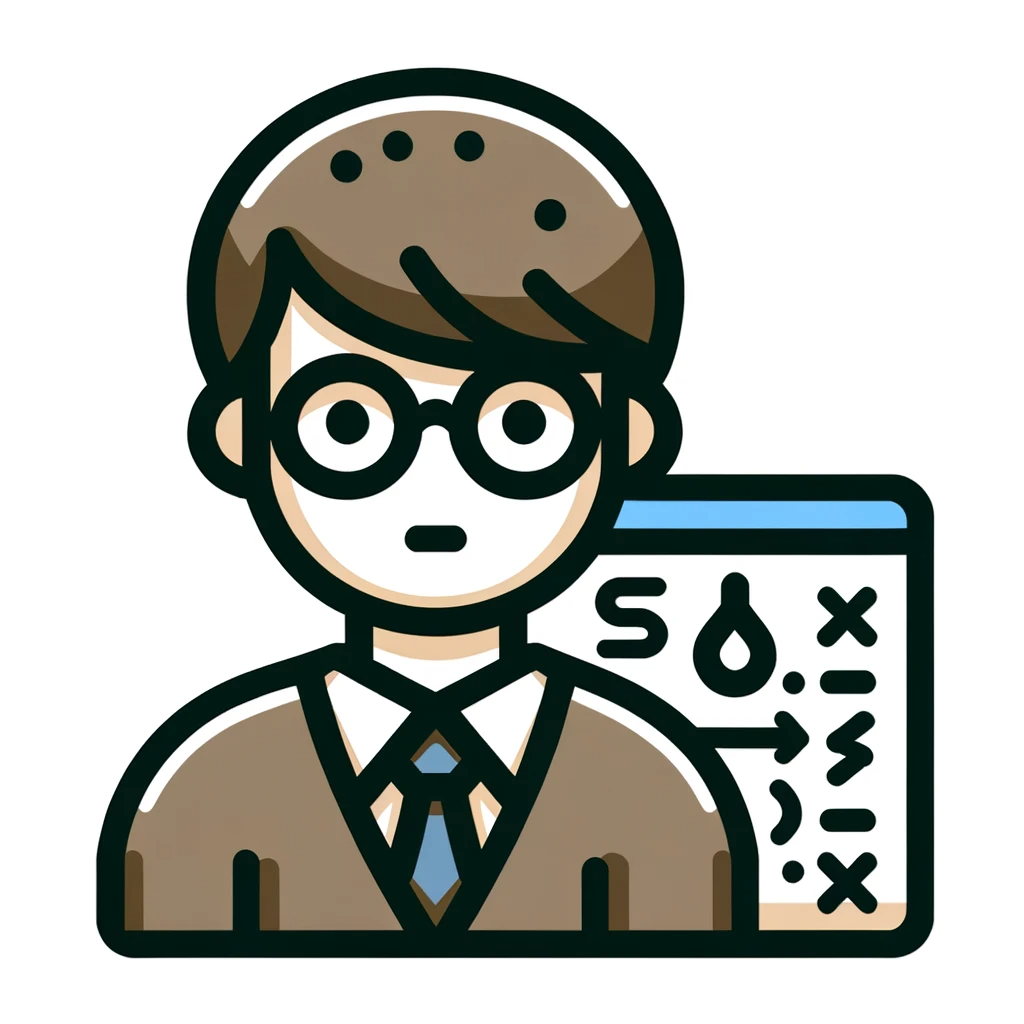
The slice method does not directly modify the array.
In order to use the retrieved elements, we need to create a new array.
Summary
We explained how to retrieve a specific range of elements in an array.
- To get a specific range of elements in an array in JavaScript, use the slice method.
- The slice method extracts a specific range of elements from an array and creates a new array.
- Use the slice method by specifying the index number of the array. The first argument specifies the starting position of the element you want to extract, and the second argument specifies the ending position of the element you want to extract.
- If the second argument is omitted, the last part of the array will be retrieved.
- By specifying a negative number as the first argument, you can also specify the position counting from the back.
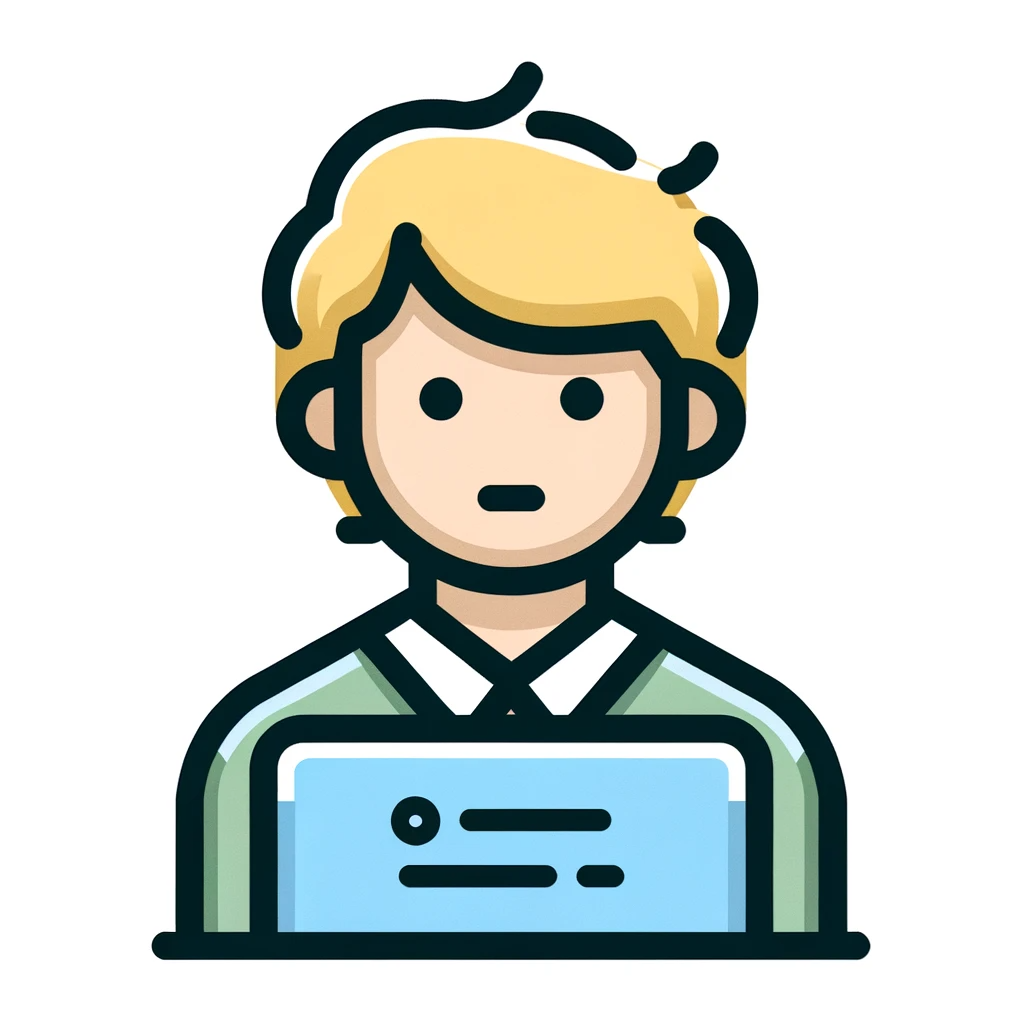
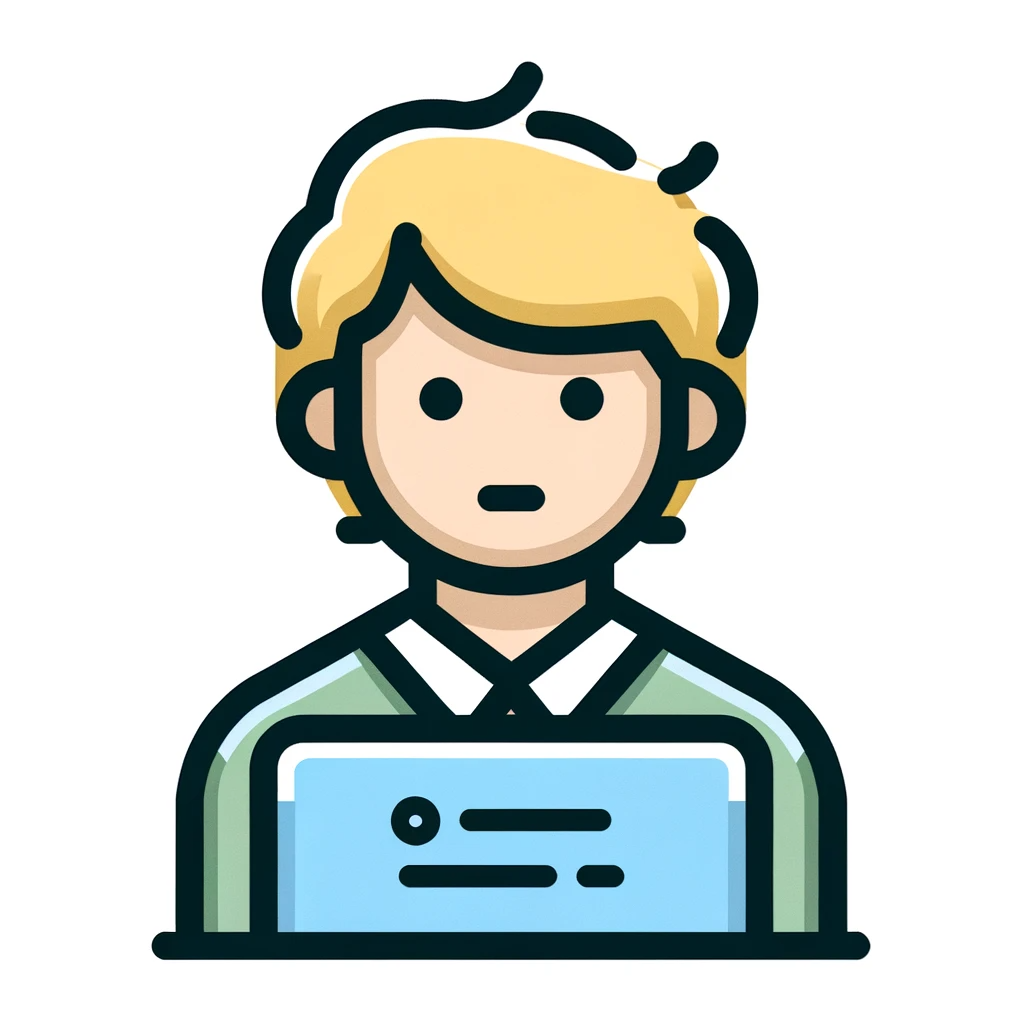
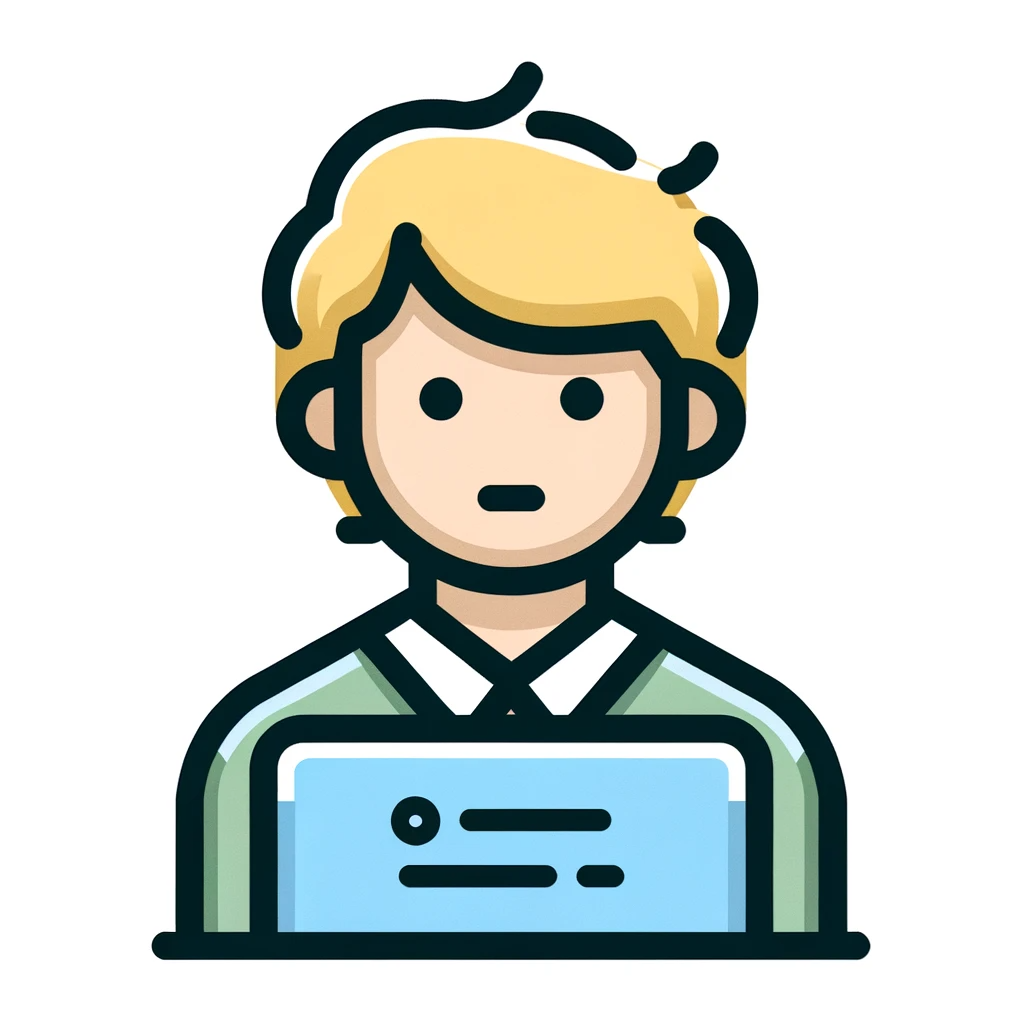
You can easily get a specific range of elements from an array using the slice method!
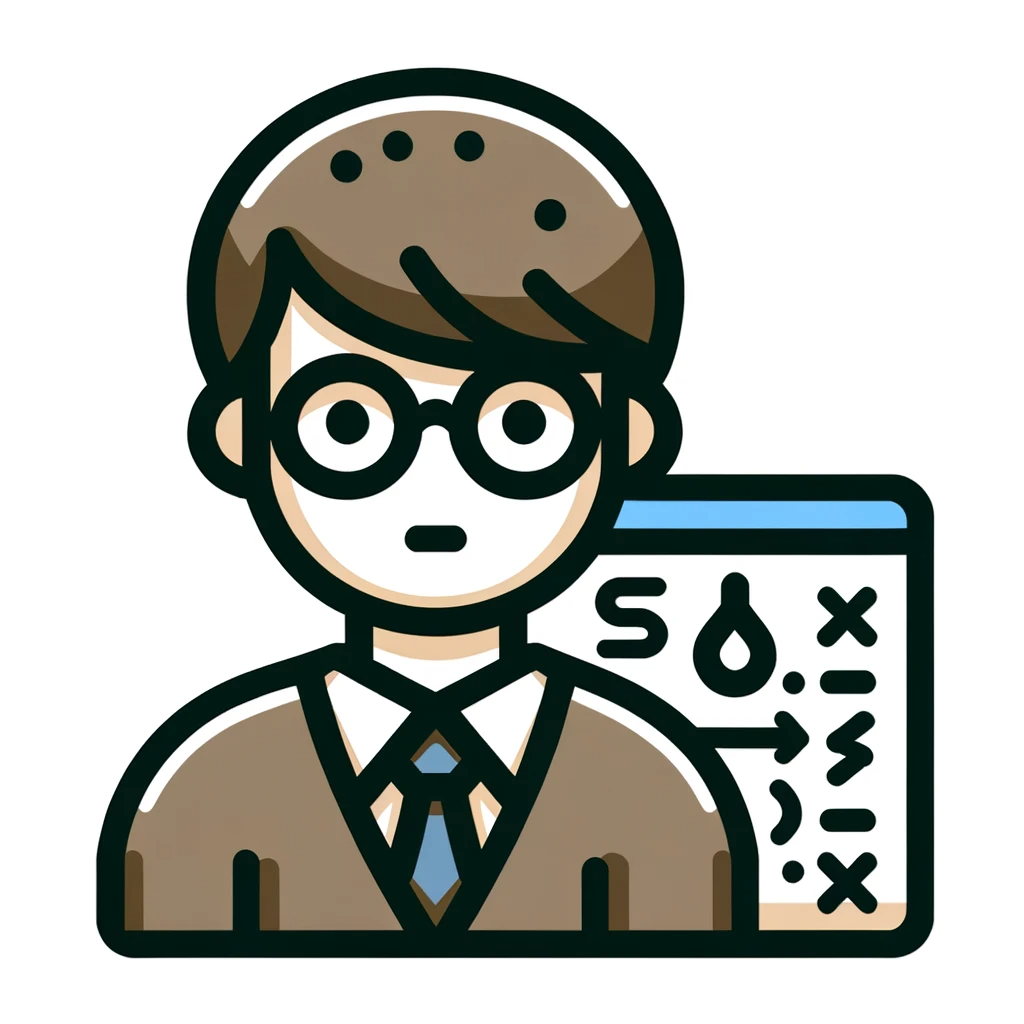
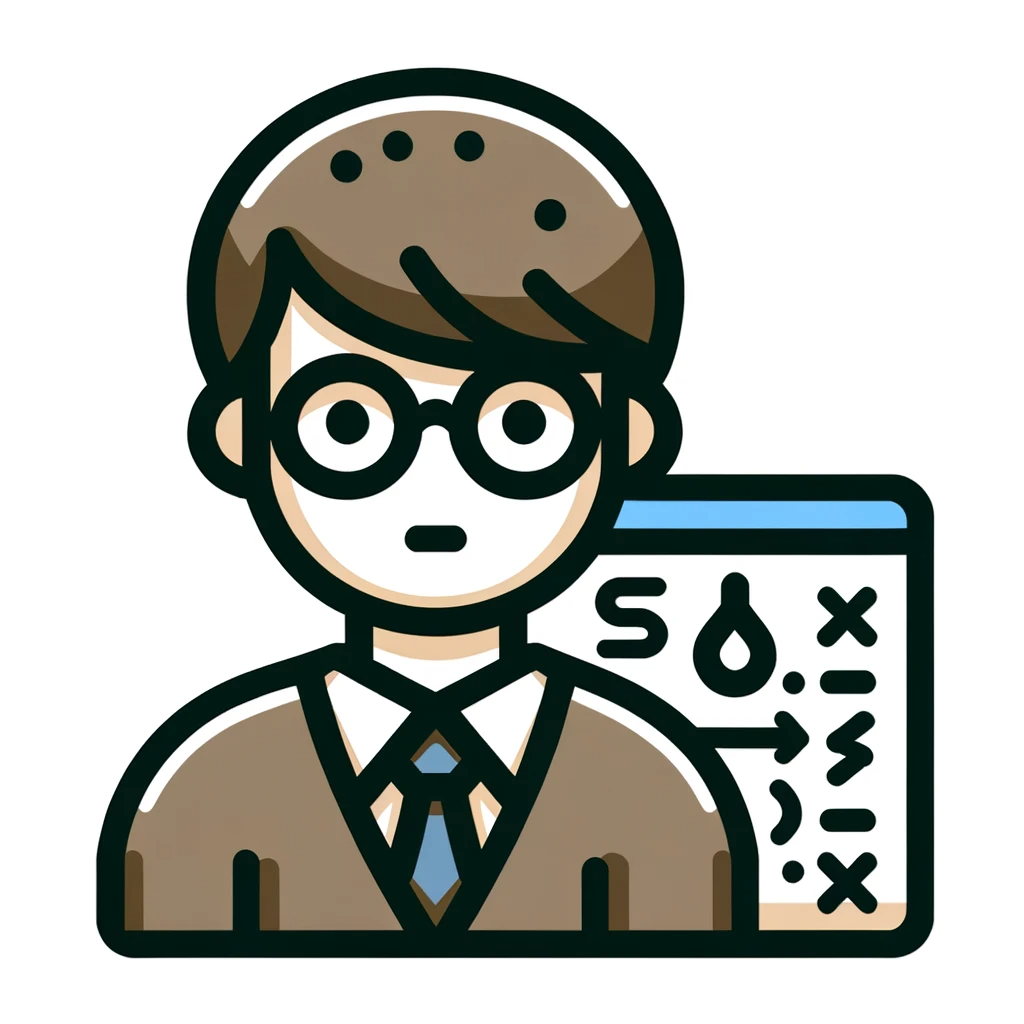
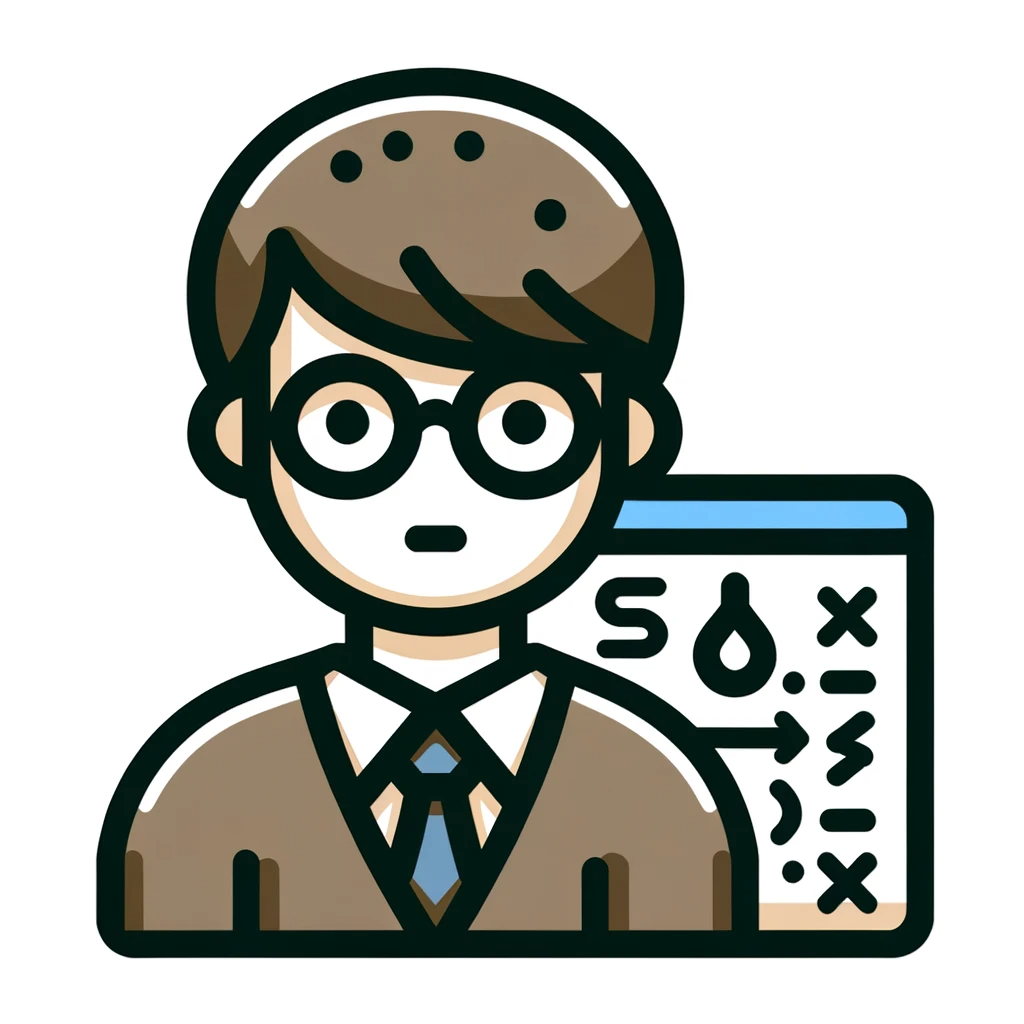
The slice method is a very useful method in JavaScript for retrieving a specific range of elements from an array.
Learn how to use it and utilize it for array operations.
Comments