This article explains how to automatically execute processing after a certain amount of time using JavaScript.
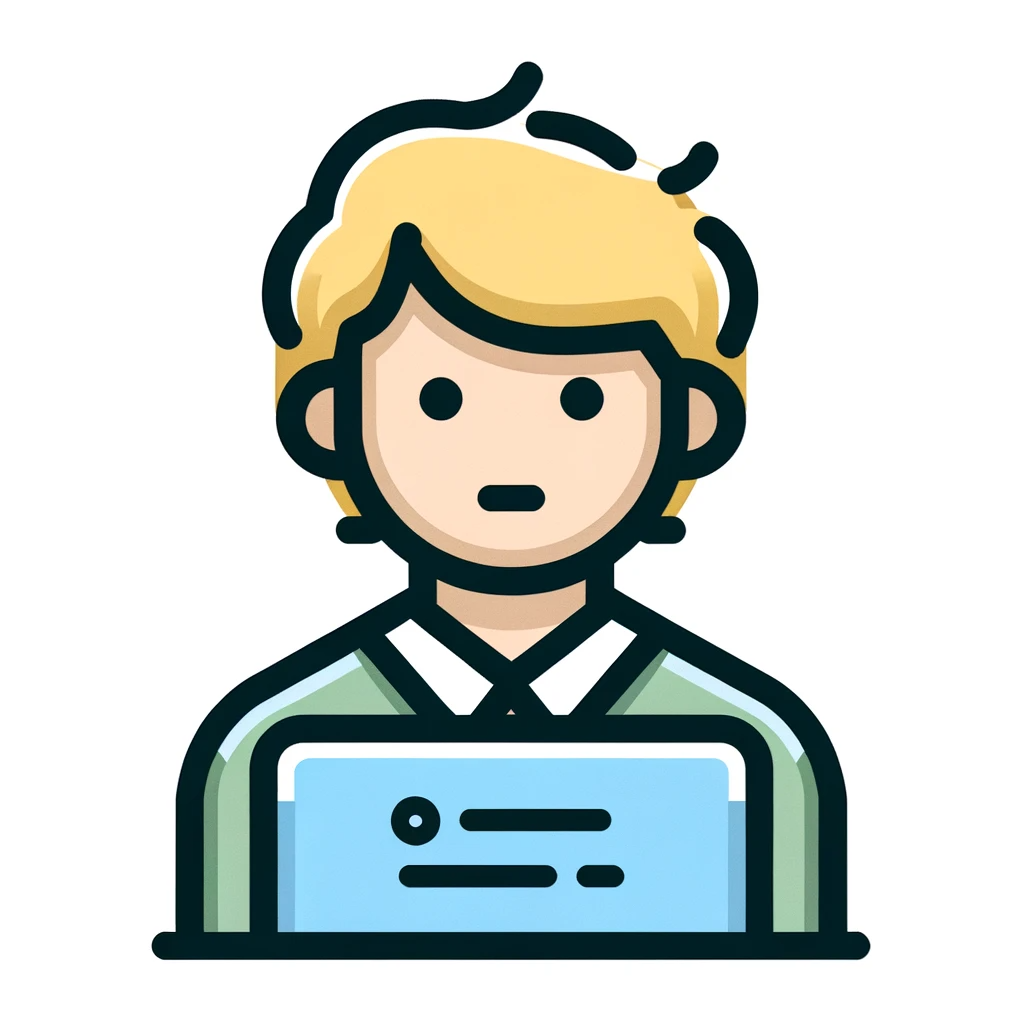
I would like to know how to execute a process after a certain amount of time has passed.
What should I do?
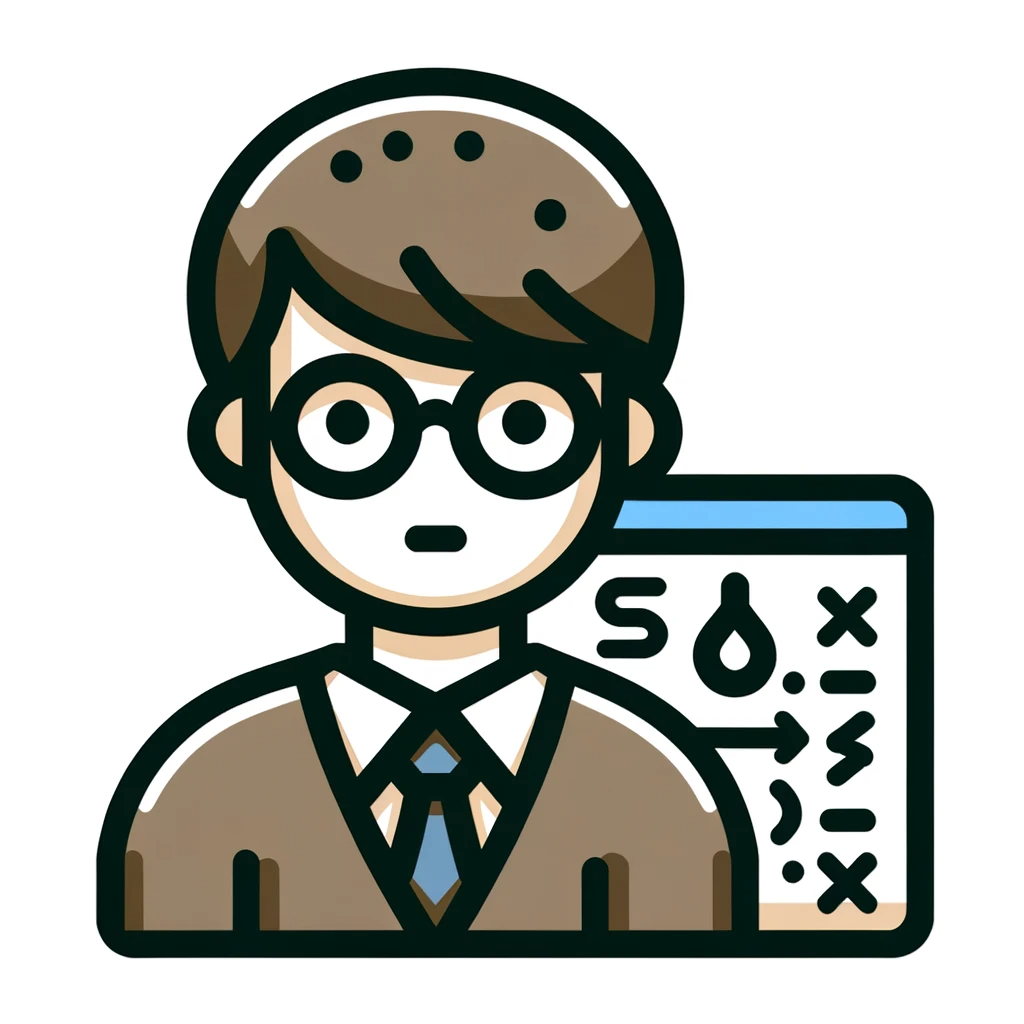
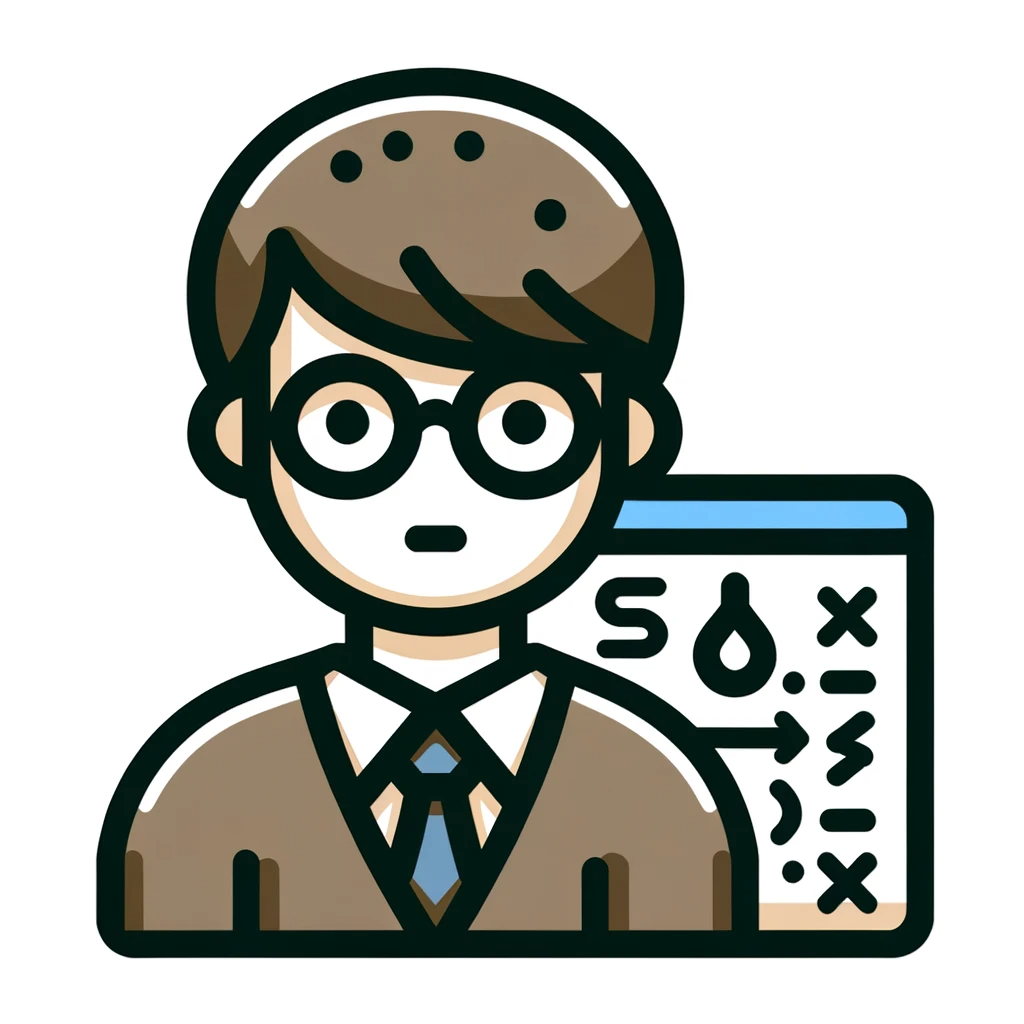
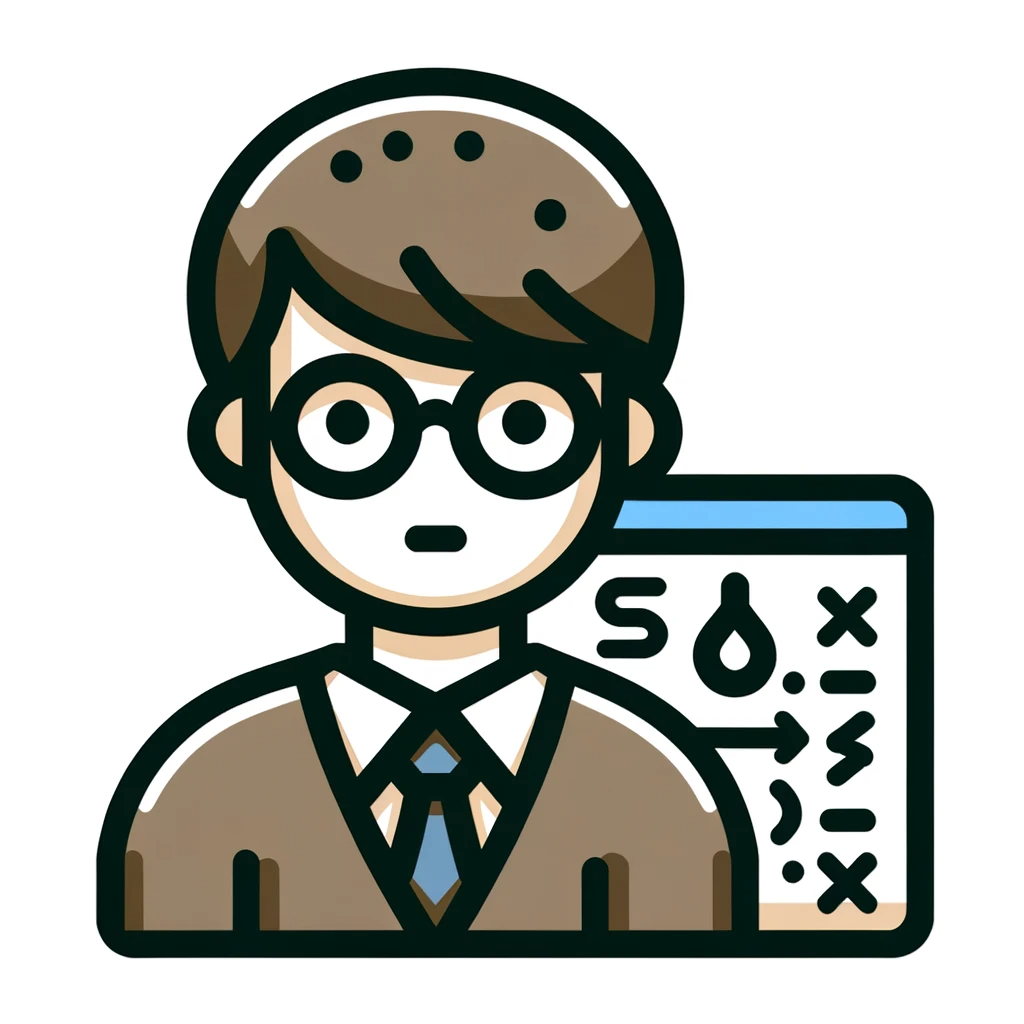
One way to execute a process after a certain amount of time is to use the setTimeout() function.
Method using setTimeout() function
The setTimeout() function is a function that executes a specified function after a certain period of time . Executes only once after the specified time has elapsed.
The setTimeout() function is used as follows.
setTimeout(function, delay);
The first argument specifies the function you want to execute , and the second argument specifies the time (in milliseconds) until the function is executed .
The setTimeout() function returns a numeric ID for stopping execution . You can use this ID to stop the setTimeout() function.
For example, you can stop the setTimeout() function as follows.
var timeoutId = setTimeout(myFunction, 1000);
clearTimeout(timeoutId);
The setTimeout() function executes a function after a specified amount of time has elapsed, so it is useful when you want to execute an operation after a certain amount of time. For example, it is used to display pop-up messages or implement timers.
When using the setTimeout() function, you need to be careful about the interval and execution time.
Explanation using a sample program
Below is a sample program using the setTimeout() function.
function delayedAlert() {
setTimeout(function(){ alert("Hello"); }, 3000);
}
delayedAlert();
In this program, the alert message “Hello” will be displayed 3 seconds after the delayedAlert() function is executed. An anonymous function is specified as the first argument of the setTimeout() function. This anonymous function runs after the specified amount of time has elapsed. The second argument specifies the time (in milliseconds) until execution.
When you run this program, the delayedAlert() function will be called and the alert message “Hello” will be displayed after 3 seconds.
You can also stop processing using the return value of the setTimeout() function, as shown below.
var timeoutId = setTimeout(function(){ alert("Hello"); }, 3000);
clearTimeout(timeoutId);
In this program, the setTimeout() function returns an ID, and the clearTimeout() function stops processing.
In this way, by using the setTimeout() function, you can execute the process after a certain period of time.
summary
We explained how to automatically execute a process after a certain amount of time has passed.
- The setTimeout() function is a function that executes a specified function after a certain period of time.
- Specify the function you want to execute in the first argument and the time (in milliseconds) until the function is executed in the second argument.
- Since it is executed only once after execution, unlike the setInterval() function, it is not suitable for periodic processing.
- The setTimeout() function also returns a numeric ID for stopping execution.
- When using the setTimeout() function, you can also use the clearTimeout() function to stop processing.
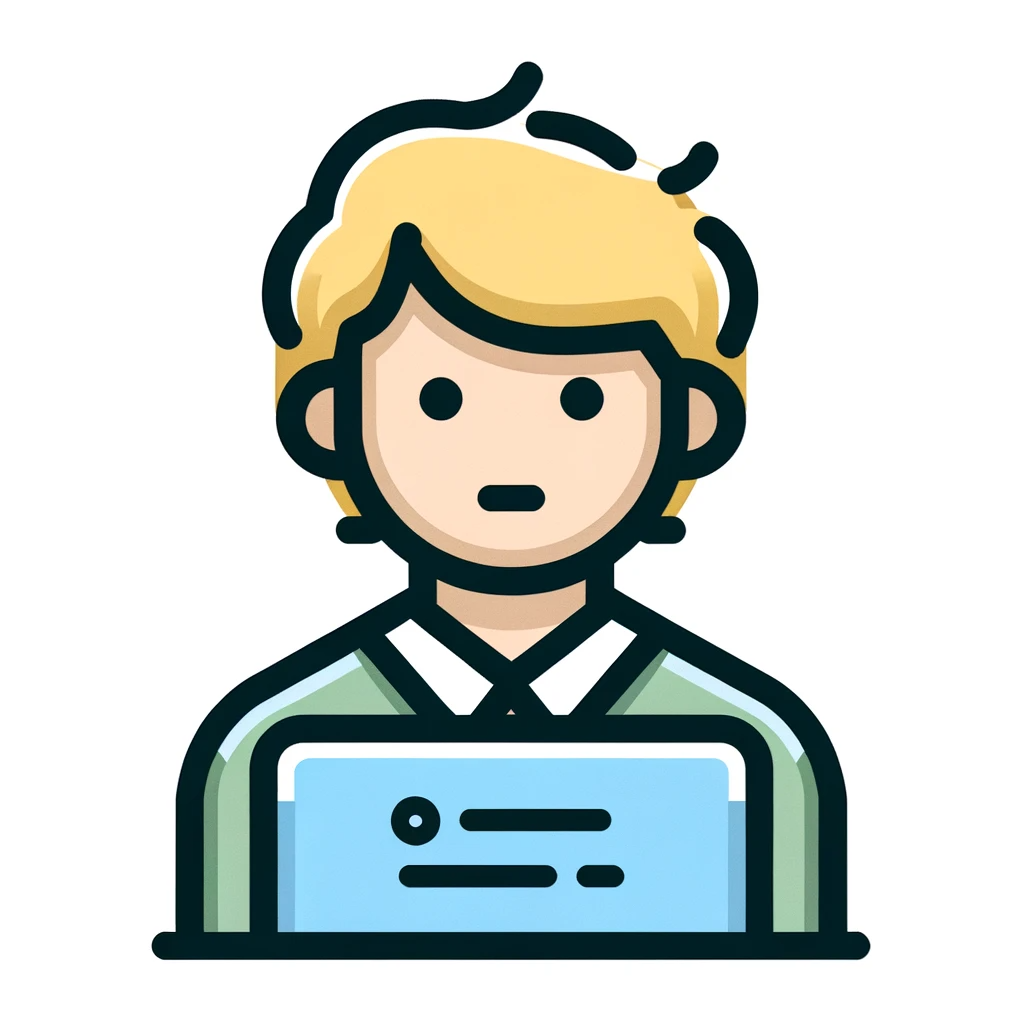
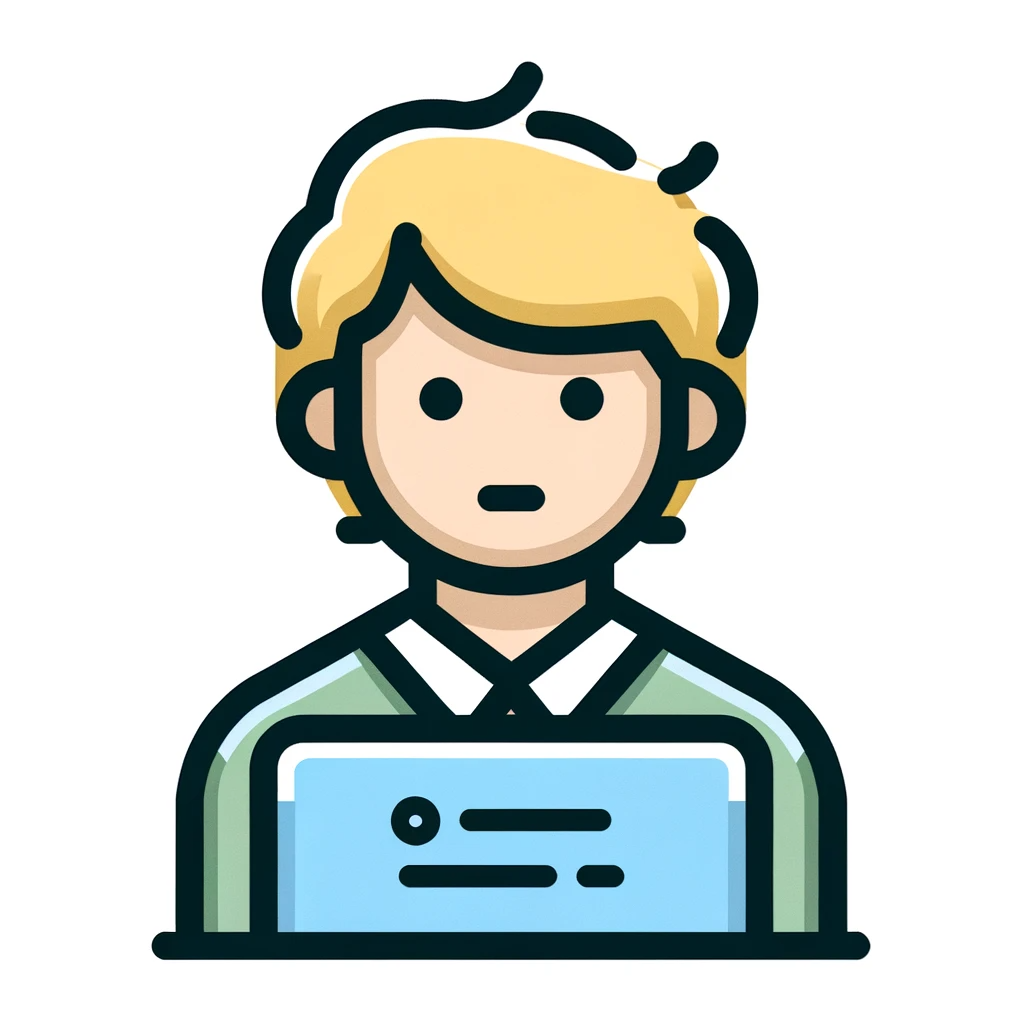
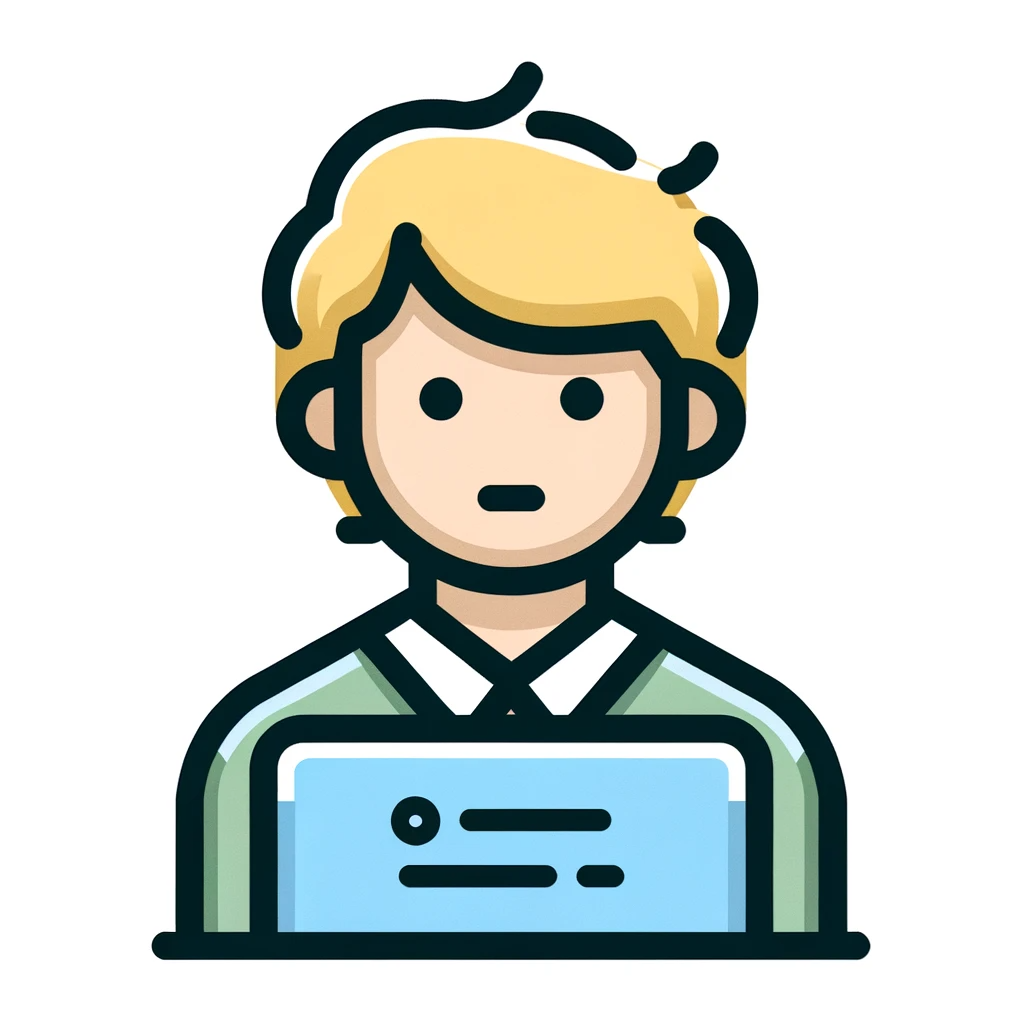
I was able to process it only once using the setTimeout function.
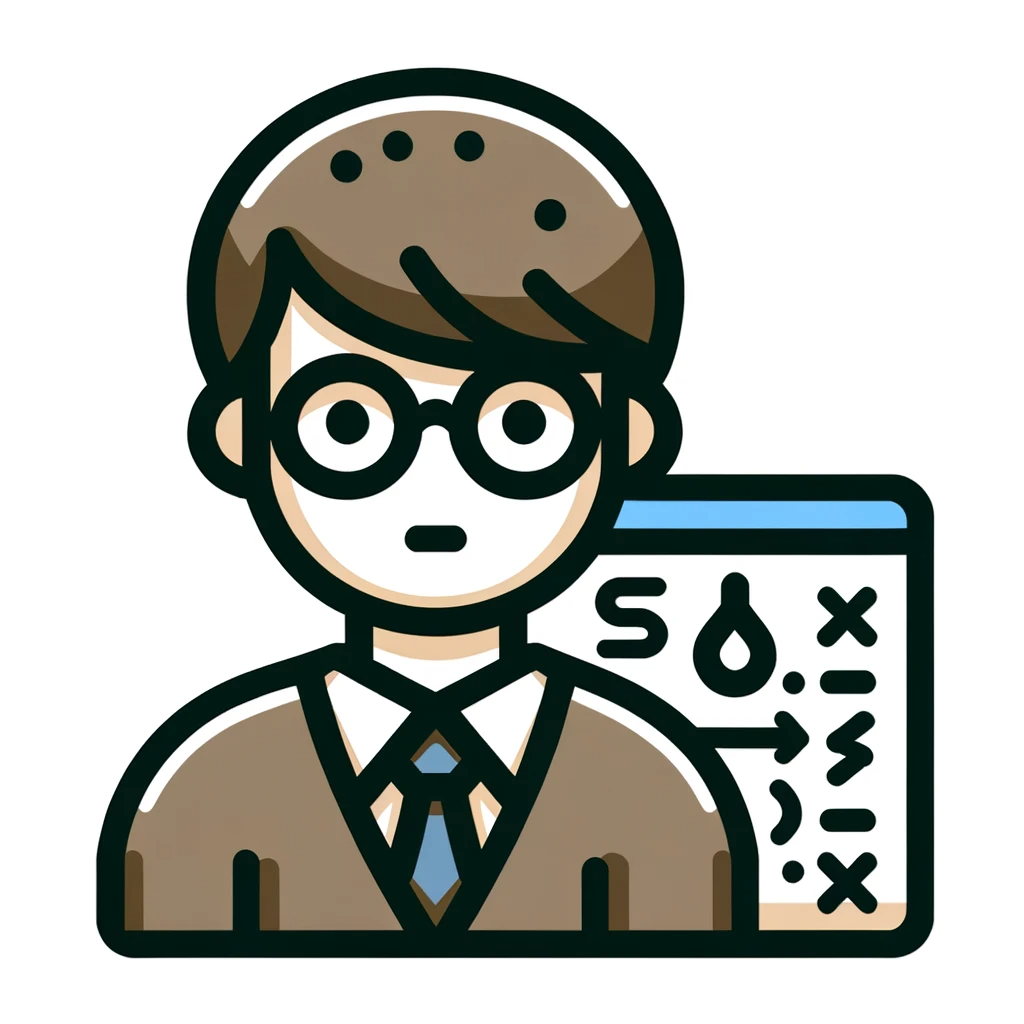
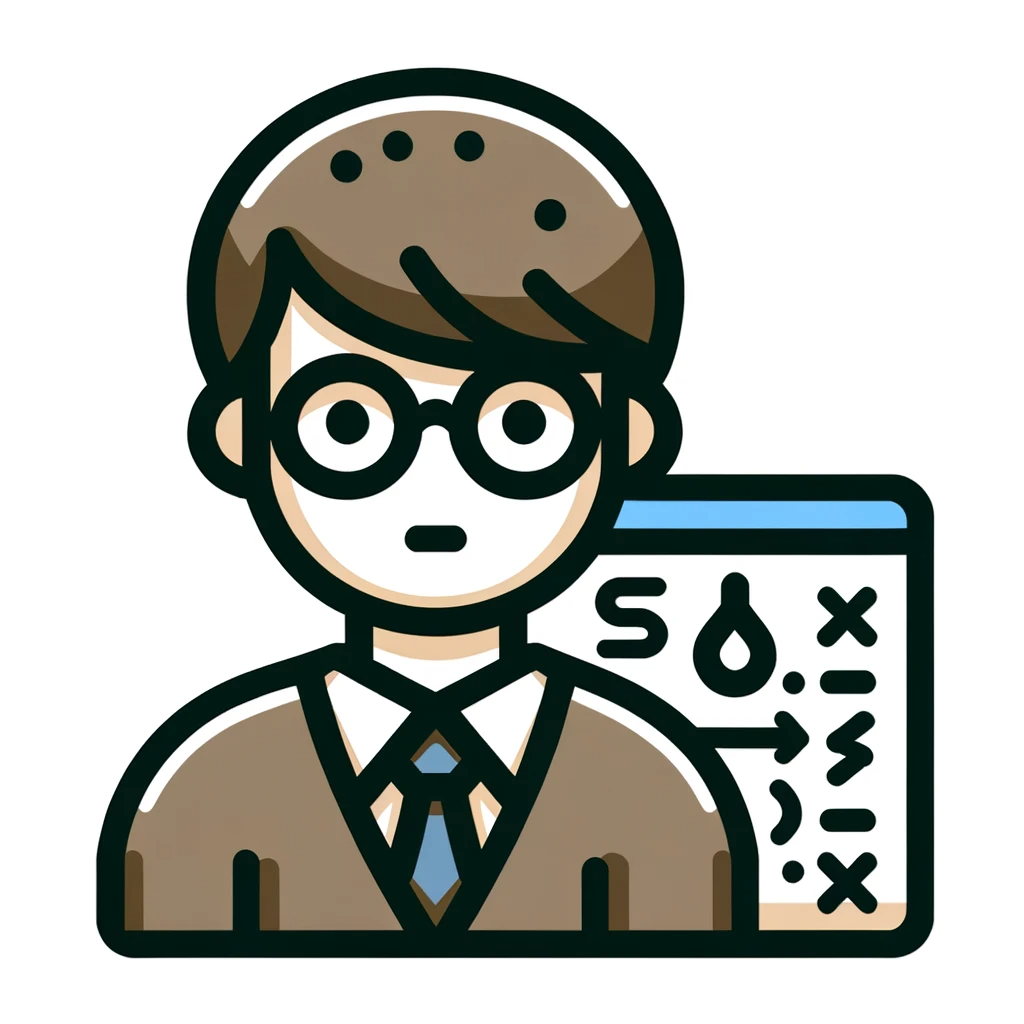
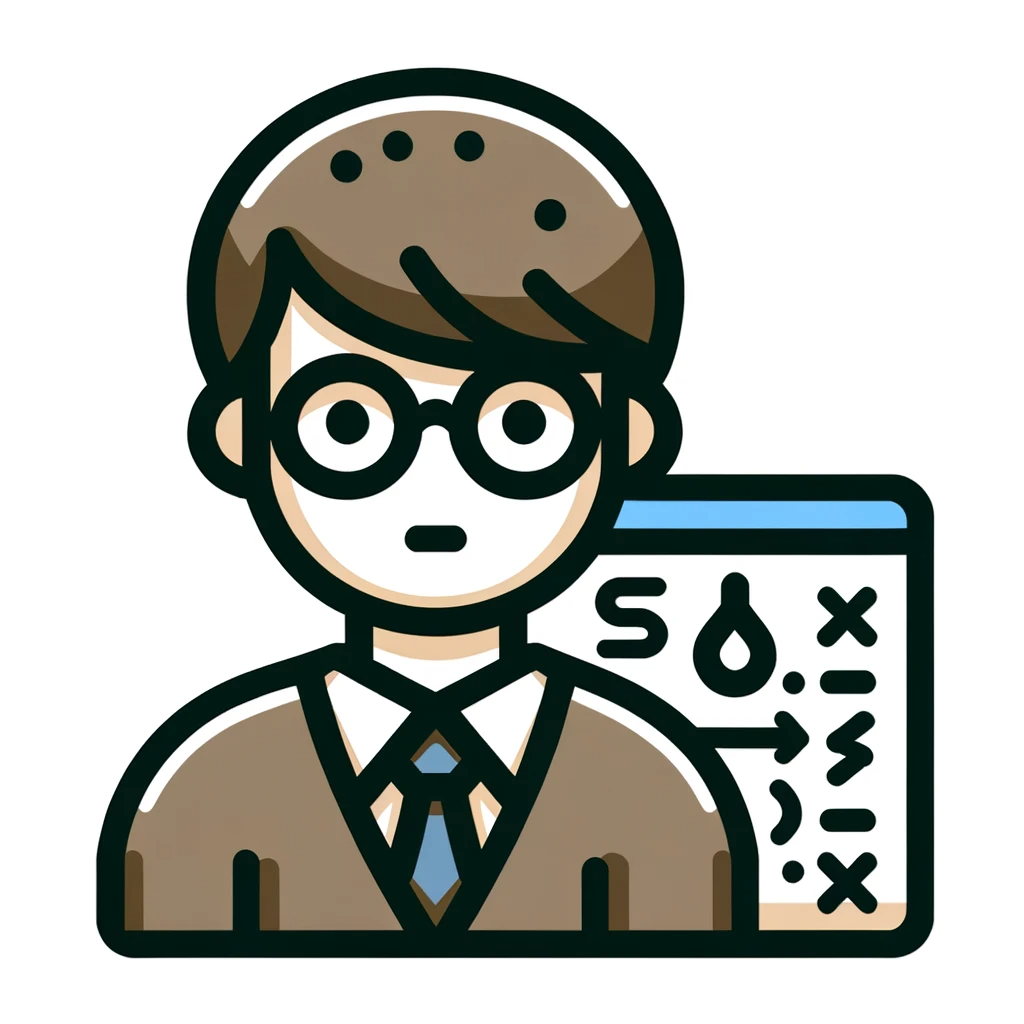
The setTimeout() function is a very useful function that allows you to perform processing that you want to execute after a certain amount of time.
You need to be careful about the interval and execution time, but it is executed only once, so it is ideal if you want to execute processing at a specific time.
Comments