To perform periodic processing in JavaScript, you can use setInterval. This article explains the basic usage of setInterval and points to note.
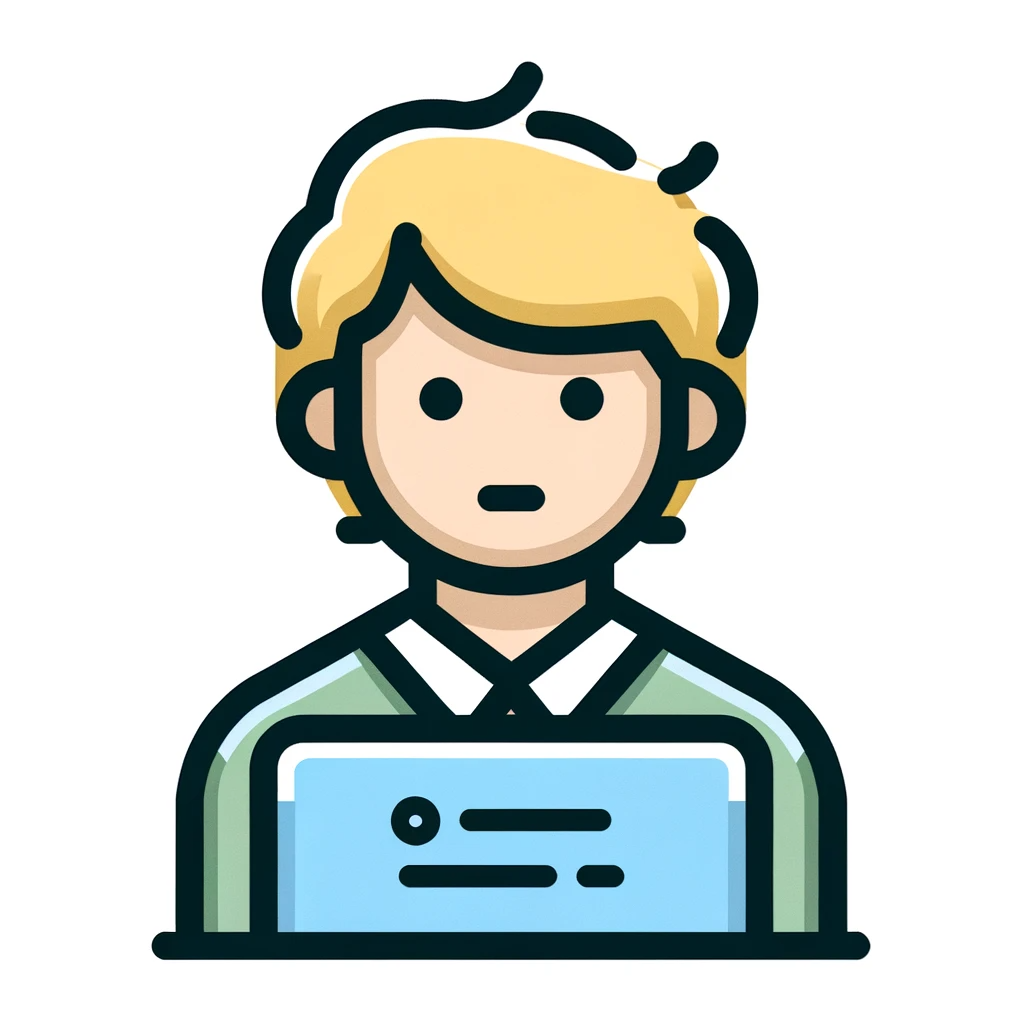
Is there a way to perform periodic processing with JavaScript?
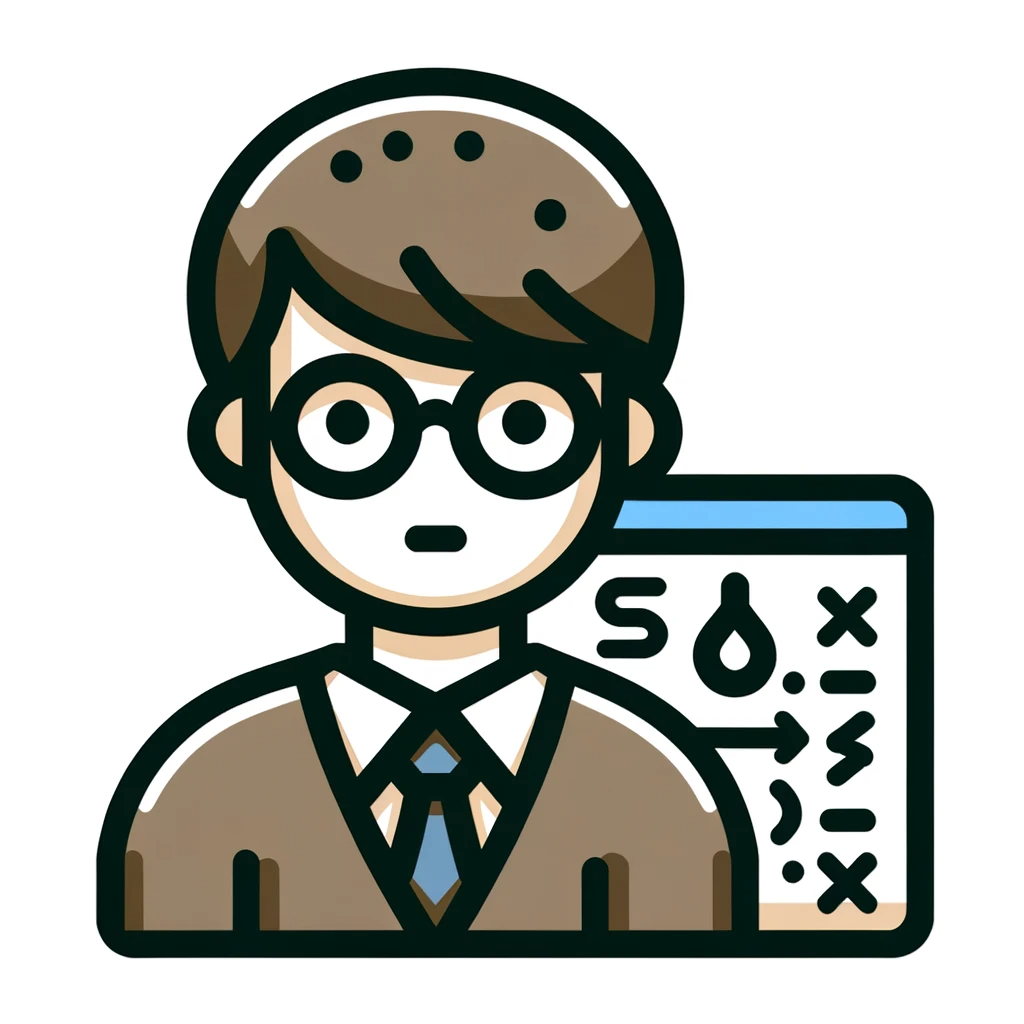
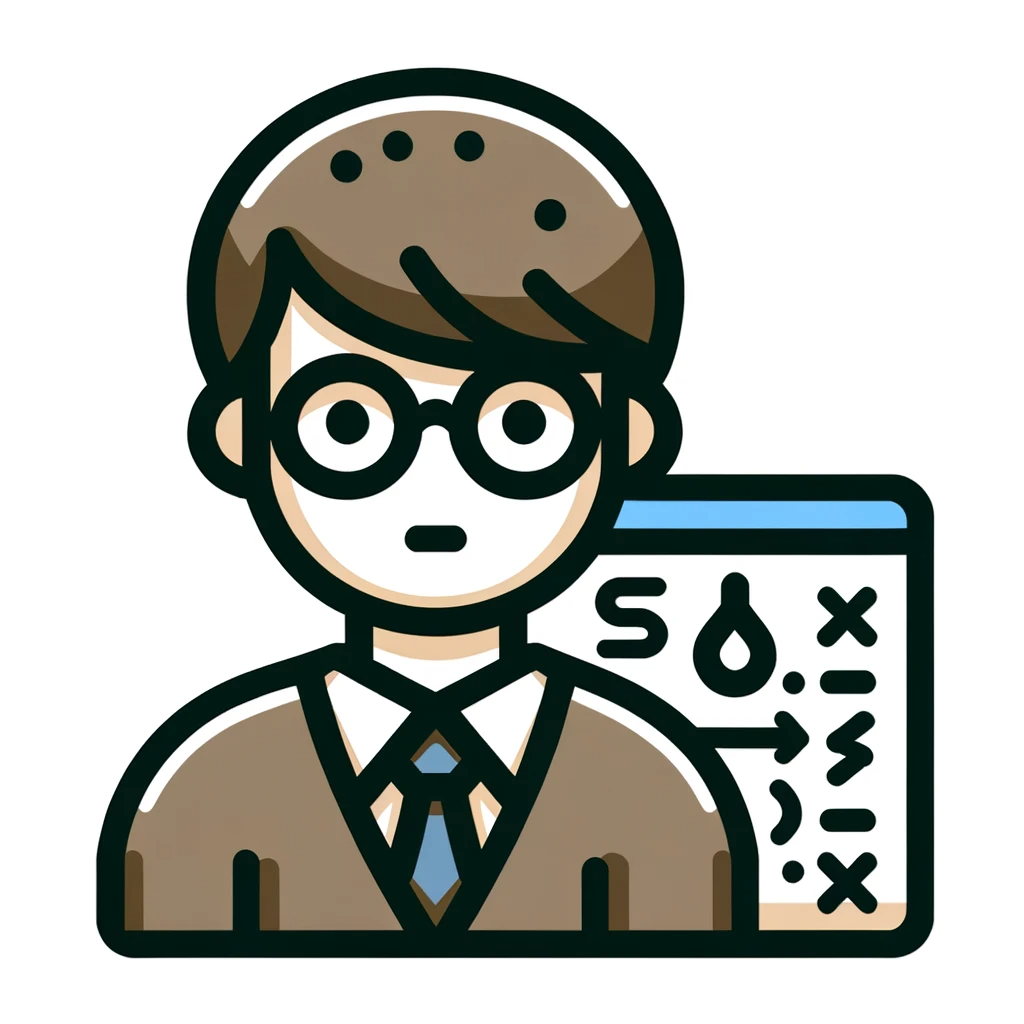
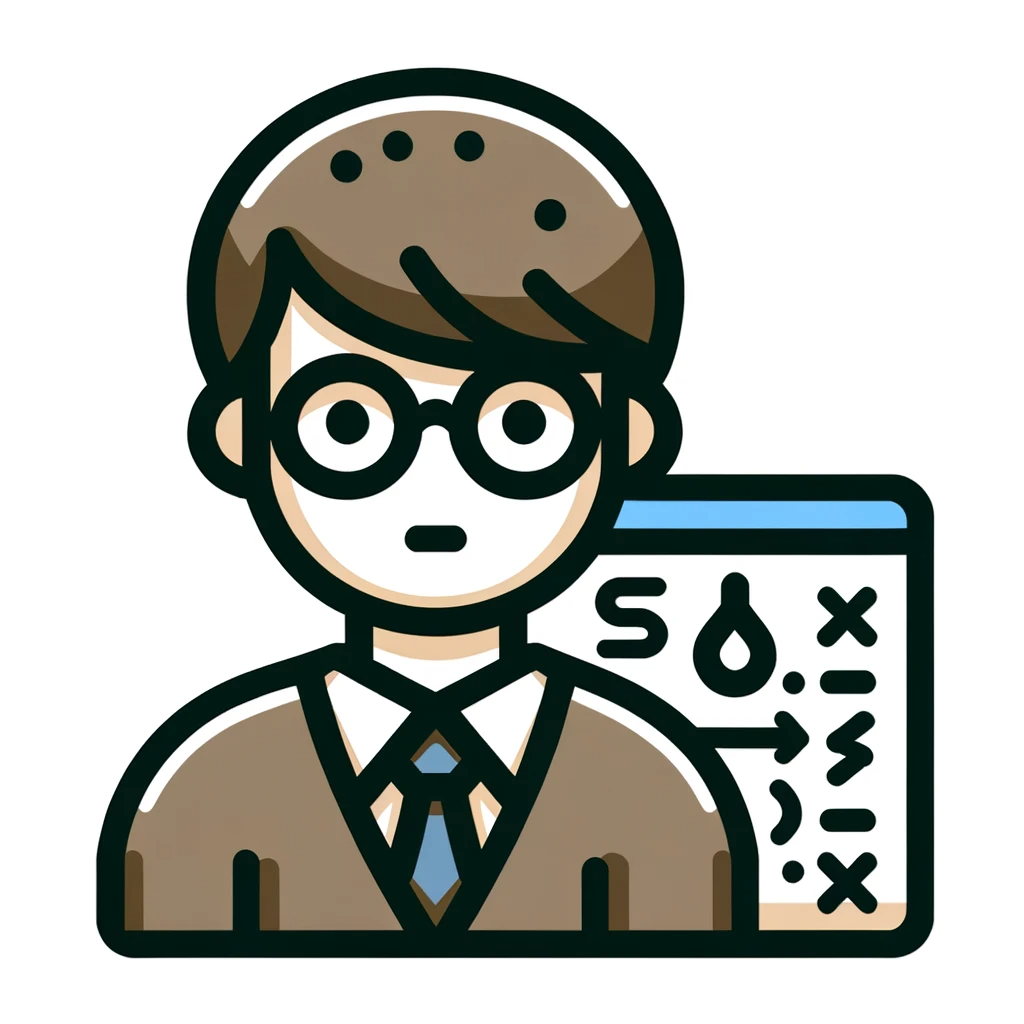
By using setInterval, you can execute the process at regular intervals.
How to use setInterval and basic syntax
setInterval is a method to repeatedly execute a specified function at regular intervals . The basic syntax is as follows.
setInterval(Function, Time Interval);
In this way, setInterval specifies the function to be executed and the time interval .
For example, you can execute a process that outputs logs periodically as shown below.
setInterval(function() {
console.log("Hello, world!");
}, 1000);
In this case, “Hello, world!” will be output to the log every 1000 milliseconds (=1 second). The time interval can be specified in milliseconds. Also, if you want to pass arguments to the function to be executed, you can write it as follows.
setInterval(function(arg1, arg2) {
console.log(arg1 + arg2);
}, 1000, "Hello, ", "world!");
In this case, “Hello, world!” will be output to the log every second. The arguments to be passed to the function are specified in the third and subsequent arguments of setInterval.
Explanation using a sample program
Below is a sample countdown program using setInterval. Displays the remaining time every second from the specified number of seconds until it reaches 0.
function countDown(seconds) {
var intervalId = setInterval(function() {
seconds--;
console.log(seconds);
if (seconds === 0) {
console.log("Countdown ends!");
clearInterval(intervalId);
}
}, 1000);
}
countDown(10);
This program starts the countdown from the specified number of seconds by passing the number of seconds as an argument to the countDown function. The remaining time is displayed every second using setInterval, and the countdown ends when it reaches 0.
summary
We have explained the basic usage and precautions for setInterval.
- setInterval is a method that repeatedly executes a specified function at regular intervals.
- setInterval specifies the function to be executed and the time interval
- By using clearInterval, you can cancel the process registered with setInterval.
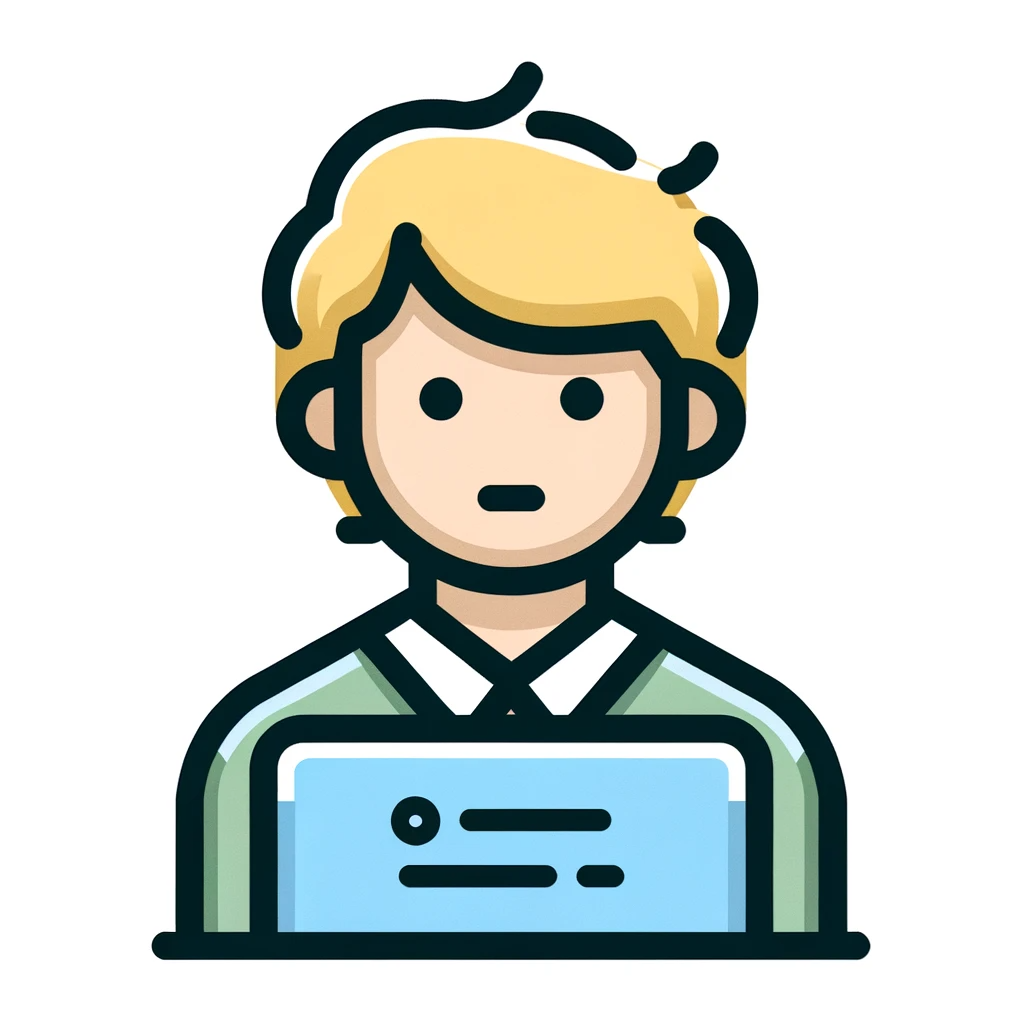
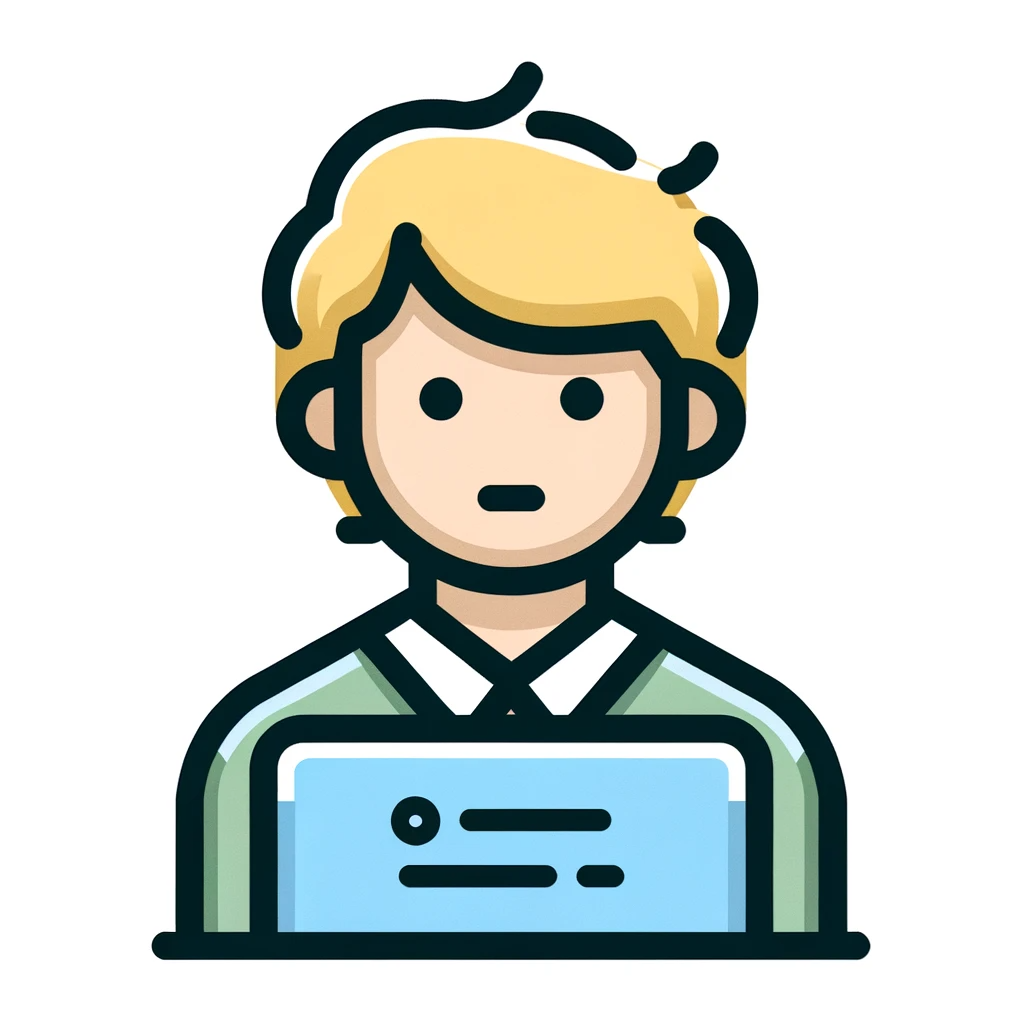
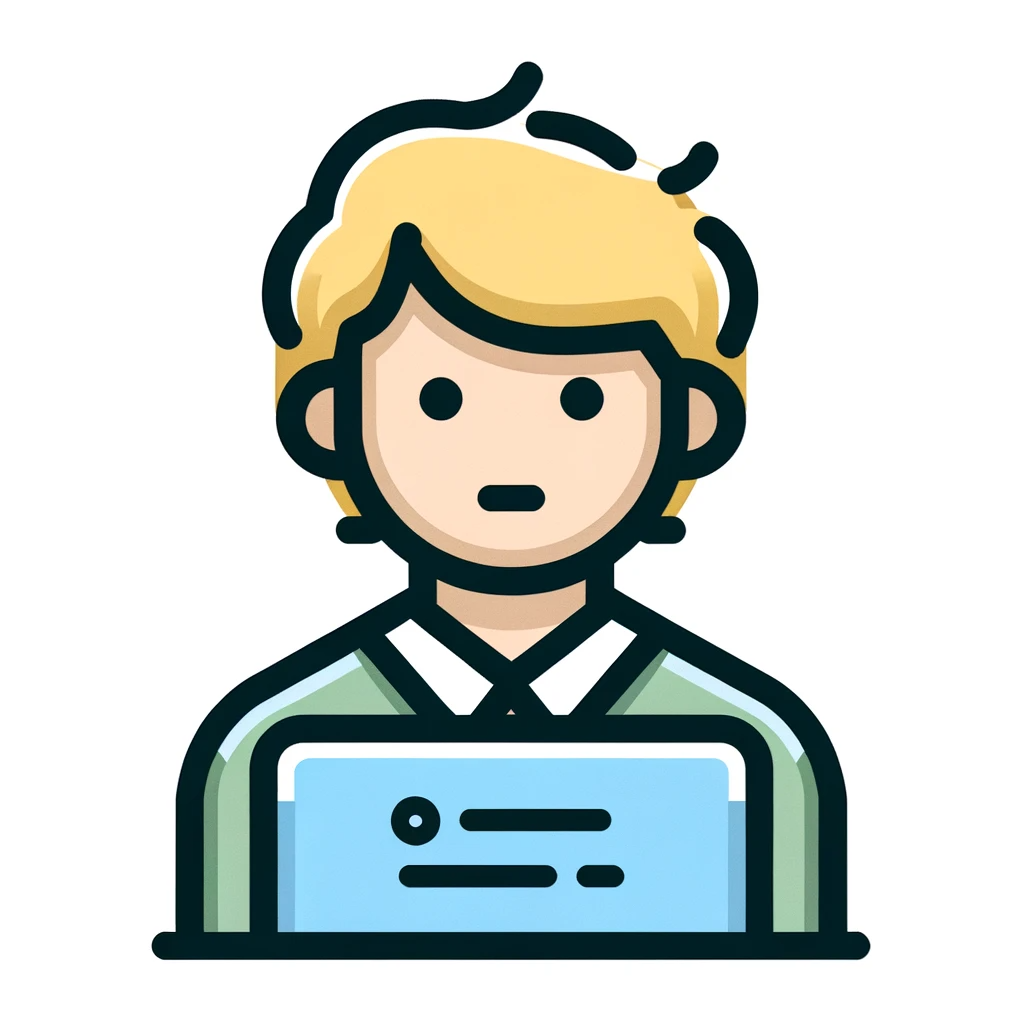
By using setInterval, you can easily execute periodic processing!
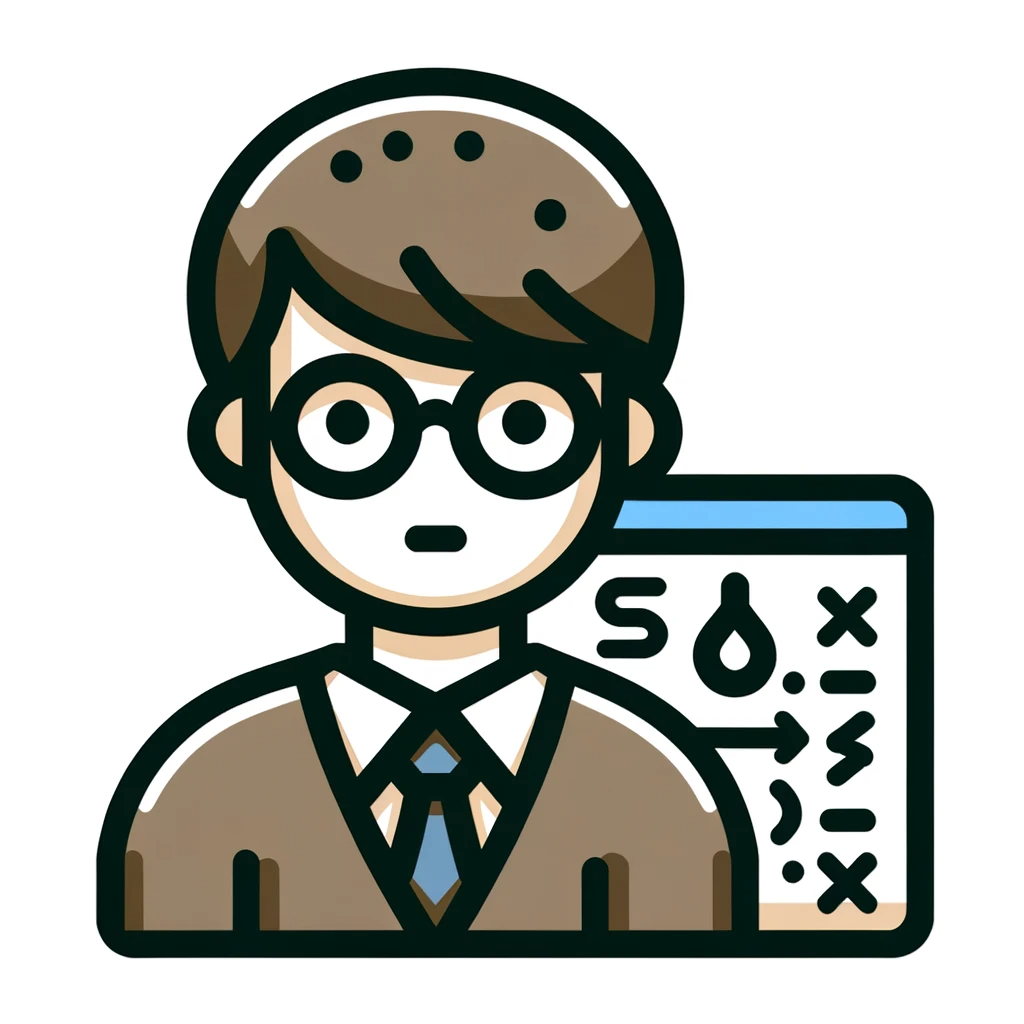
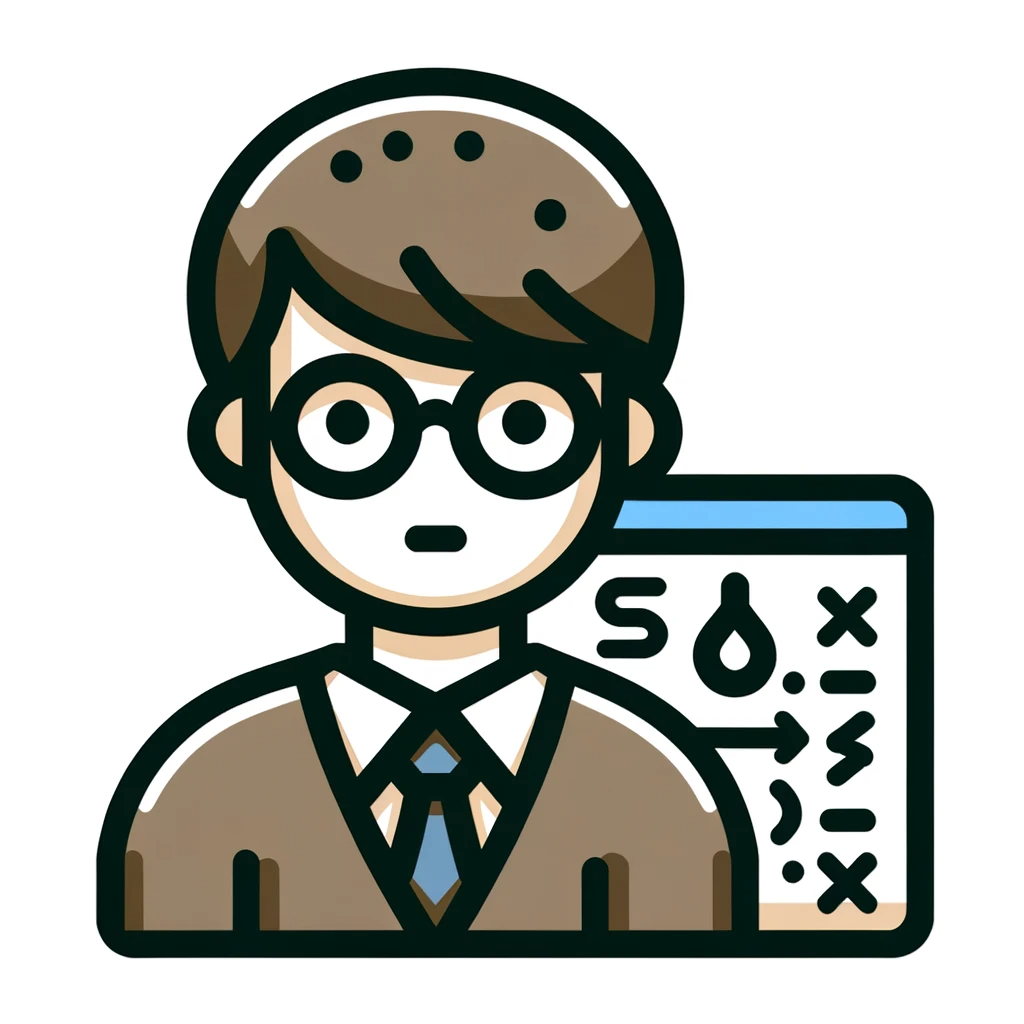
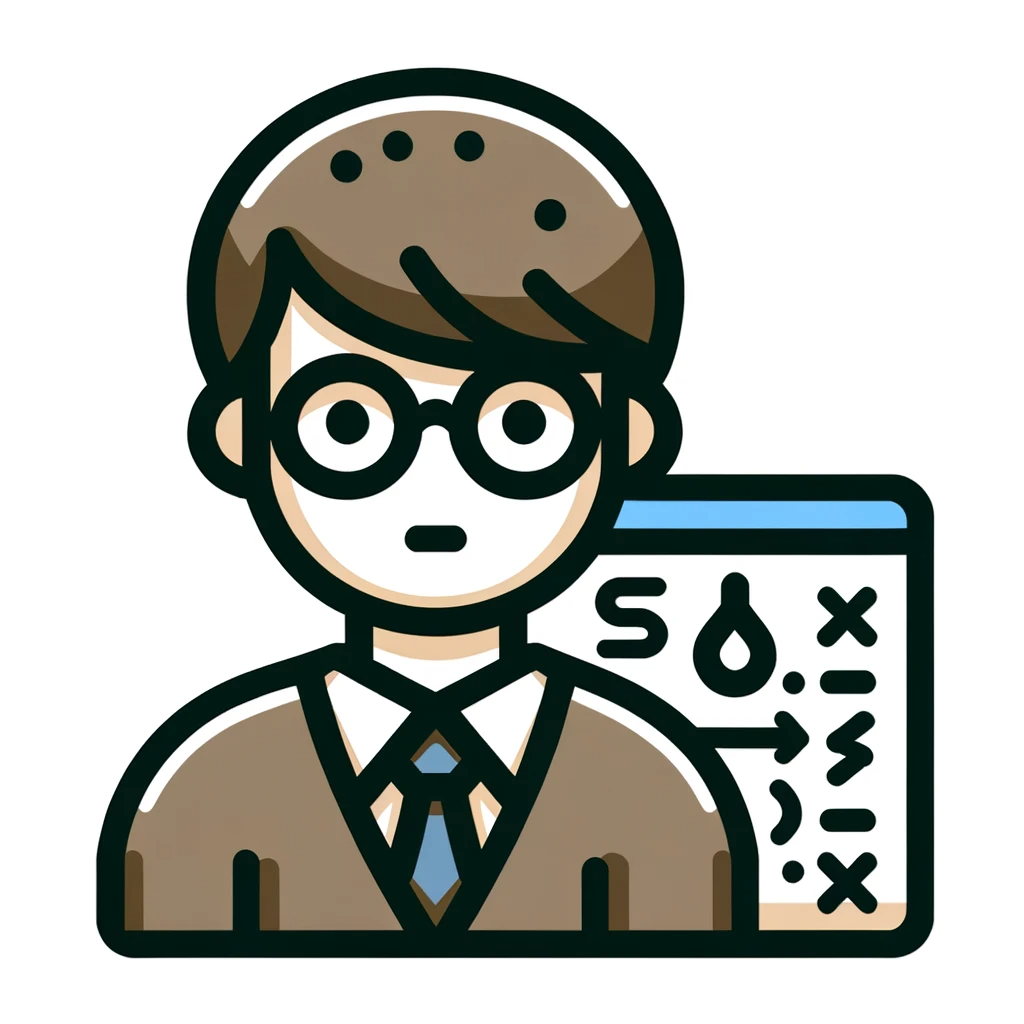
When using setInterval, it is important to be careful to clear it after completing regularly executed processing.
Also, if the setInterval interval is short, the load on the browser may increase and performance may be affected, so be sure to set an appropriate interval.
Comments