We will explain in detail how to set attributes of HTML elements using JavaScript’s setAttribute .
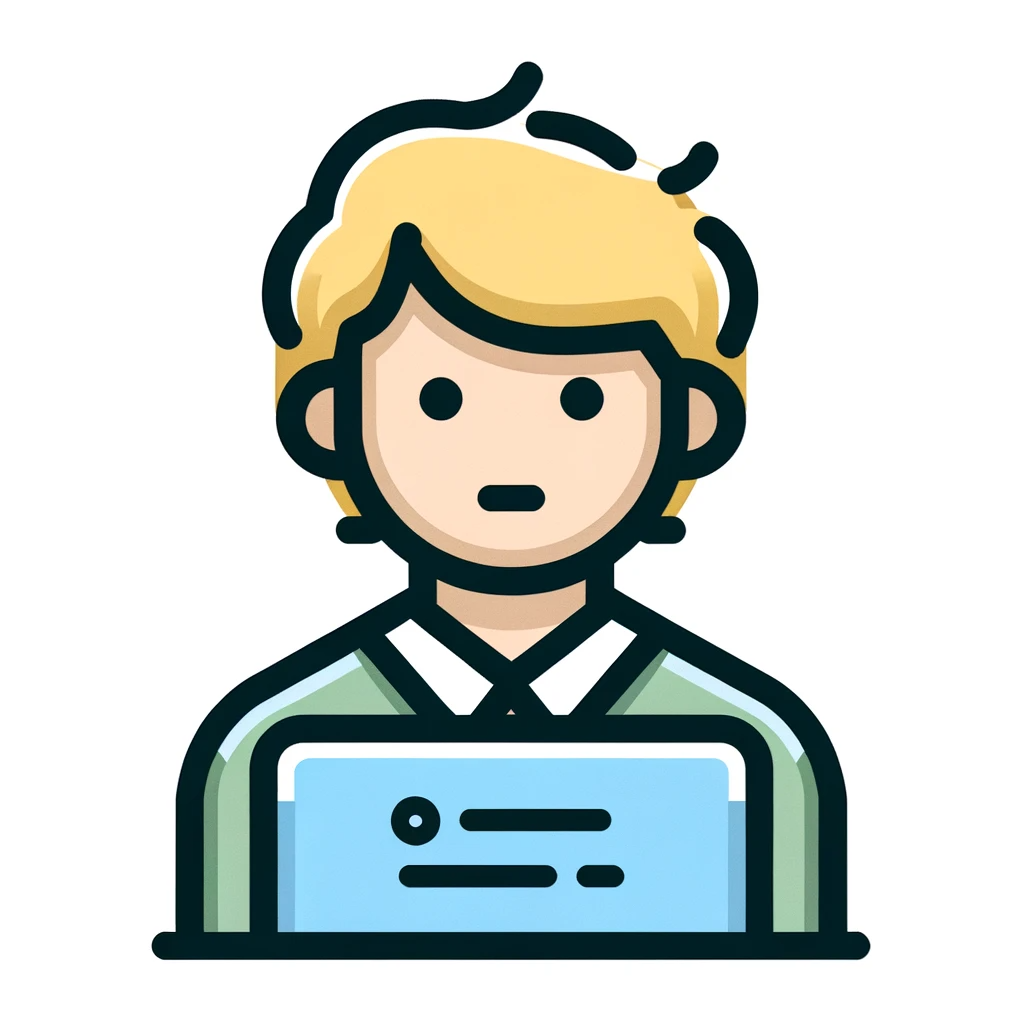
How do I set attributes of an HTML element?
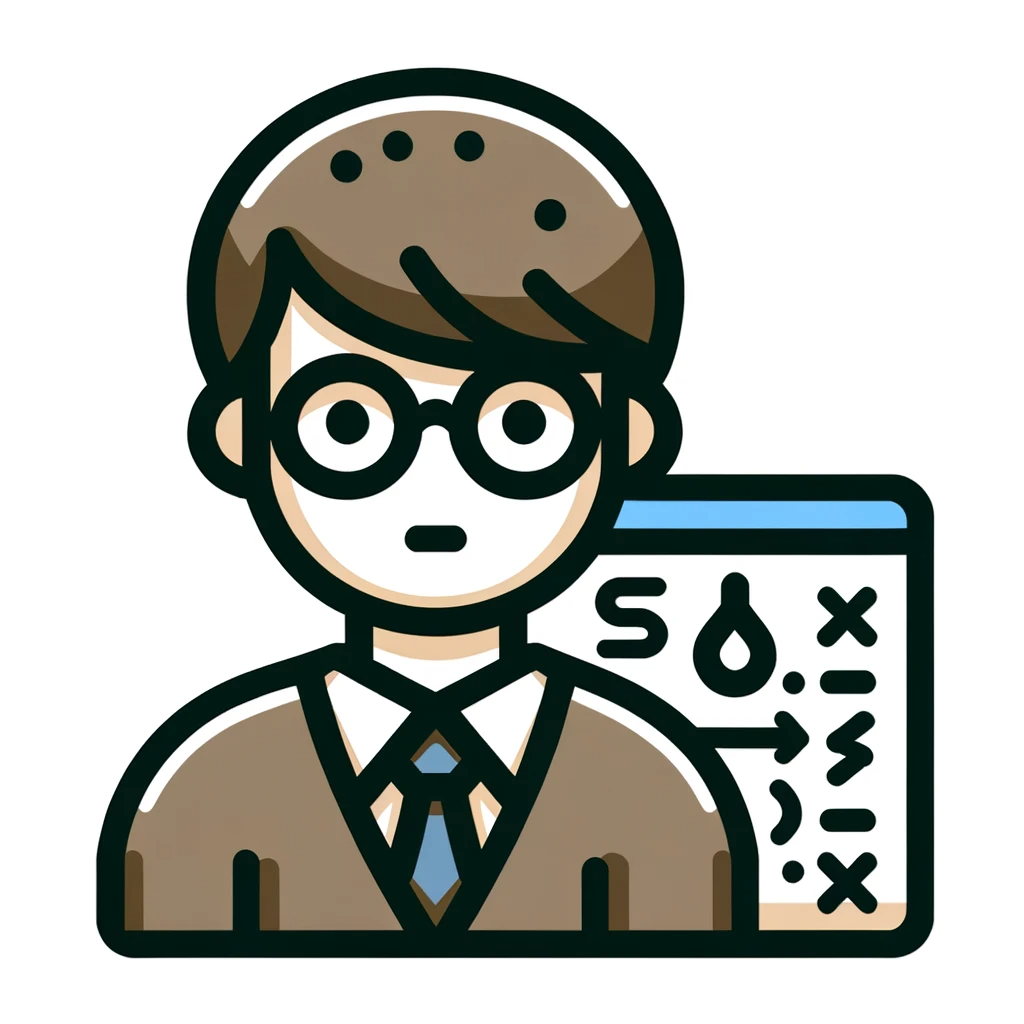
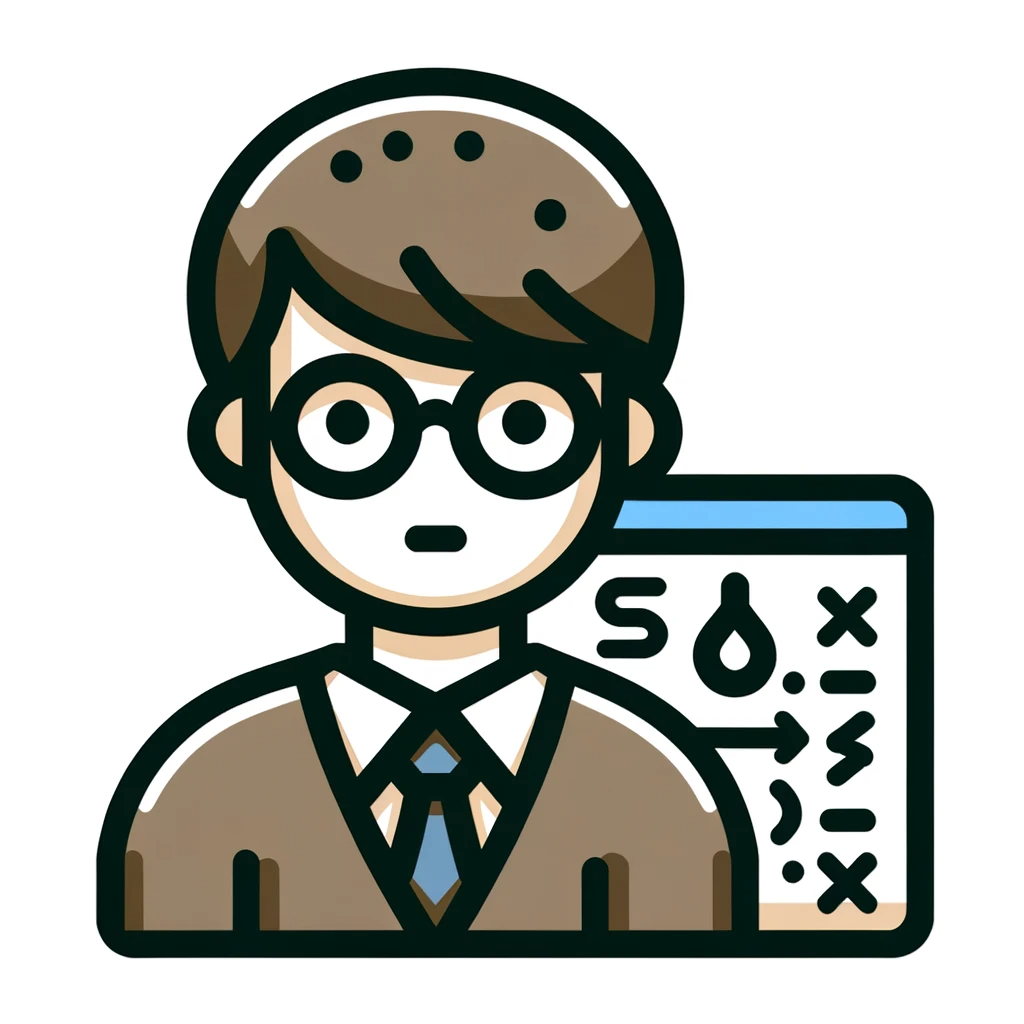
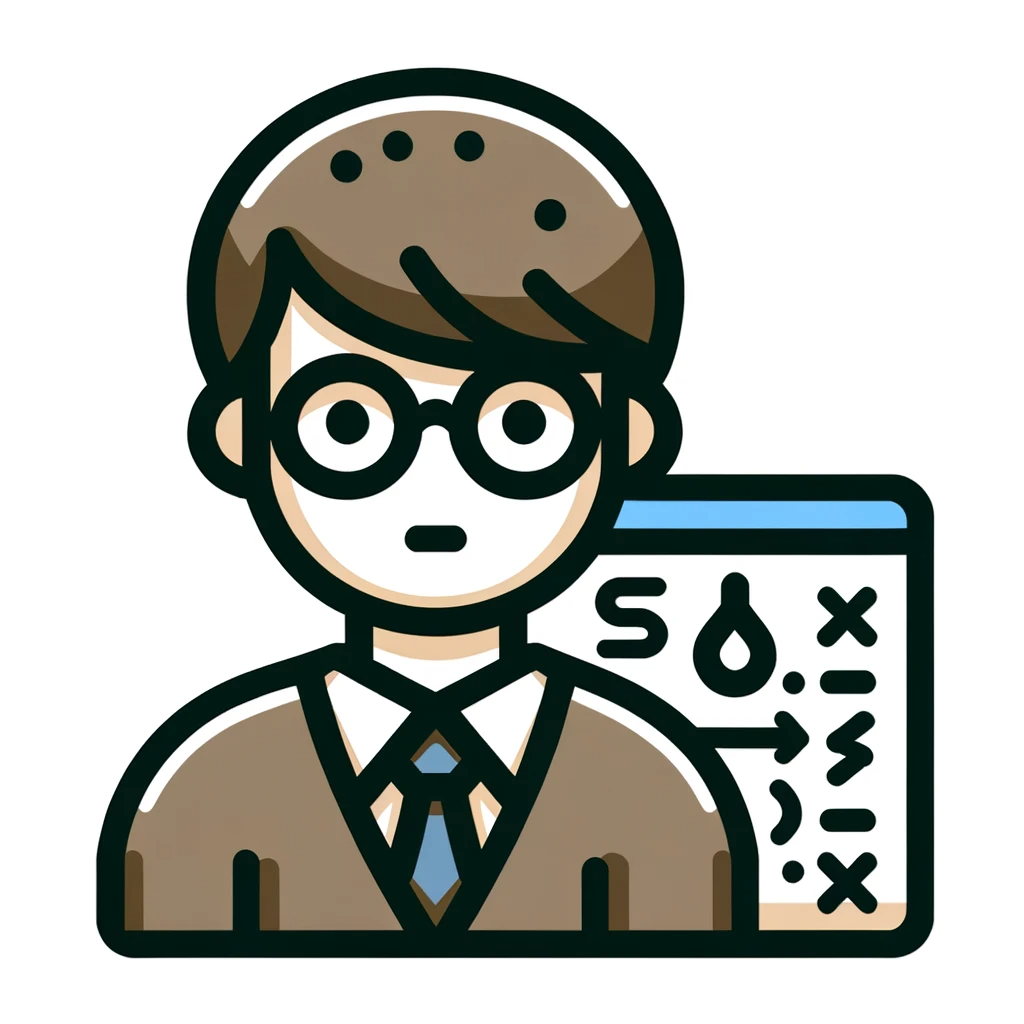
You can set attributes of HTML elements using the setAttribute method.
What is setAttribute?
setAttribute is a method for setting attributes of HTML elements using JavaScript . By using setAttribute, you can change the display and behavior of HTML elements.
For example, to set the class attribute to an element, you can write element.setAttribute(“class”, “attribute value”). This allows you to dynamically change attributes of HTML elements using JavaScript.
How to use setAttribute method
For example, to set the class attribute on an element, you can use setAttribute as follows:
element.setAttribute(“attribute name”, “attribute value”)
For example, if you want to set the class attribute on an element, you can write it like this:
var element = document.getElementById("Element ID");
element.setAttribute("class", "attribute value");
In this way, you can set attributes of HTML elements using setAttribute.
Sample program using setAttribute
A sample program for setAttribute looks like this:
In this sample, you can display images by setting the src attribute of the HTML element.
<img id="myImage">
<button id="changeImage">Change Image</button>
<script>
var image = document.getElementById("myImage");
var button = document.getElementById("changeImage");
button.addEventListener("click", function(){
image.setAttribute("src", "Image URL");
});
</script>
In this way, you can use setAttribute to dynamically change the attributes of an HTML element. In this example, the image is changed by clicking a button, but in reality the HTML element can change depending on various factors such as user actions and timers.
Summary of this article
We explained how to use setAttribute to set attributes of HTML elements.
- By using setAttribute, you can change the behavior and appearance of an element.
- To use setAttribute, simply write it in the format “element.setAttribute(“attribute name”, “attribute value”)”.
- You can improve the user experience of your web pages by using setAttribute.
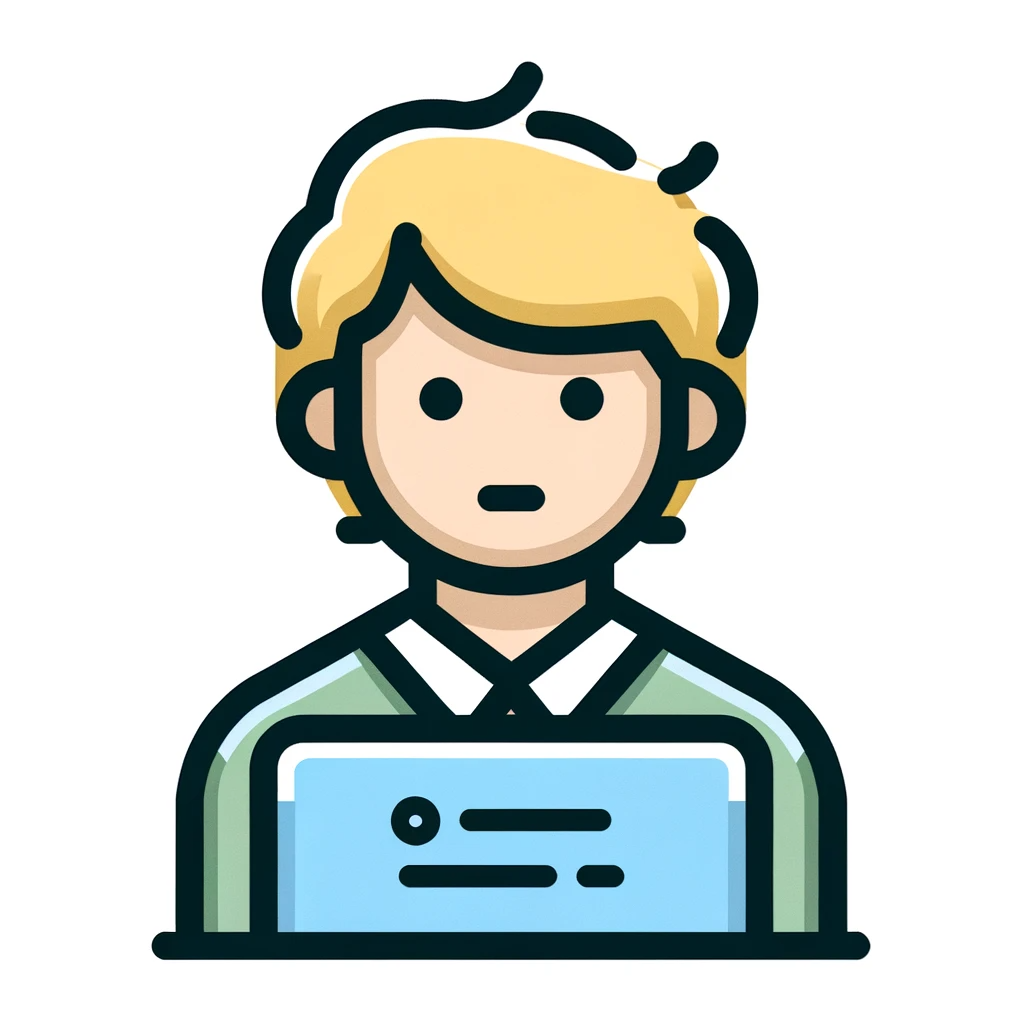
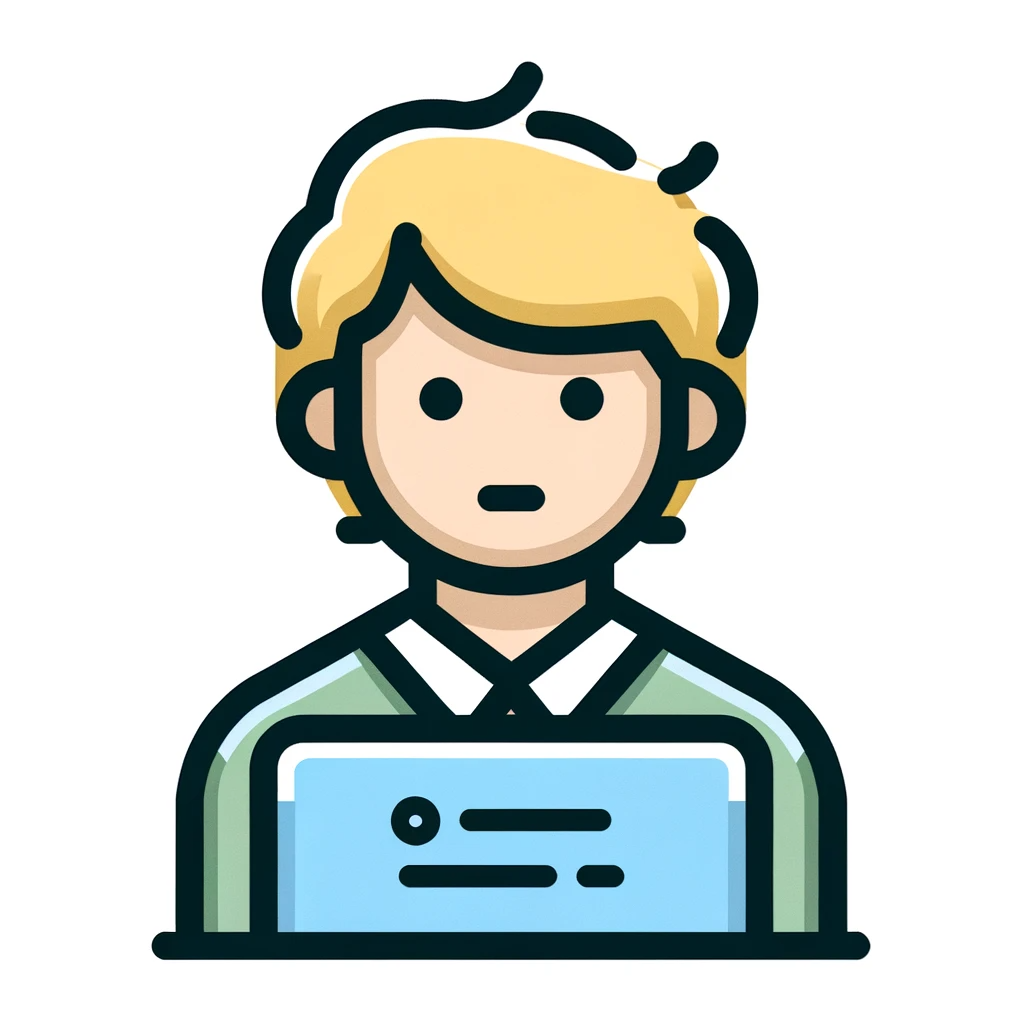
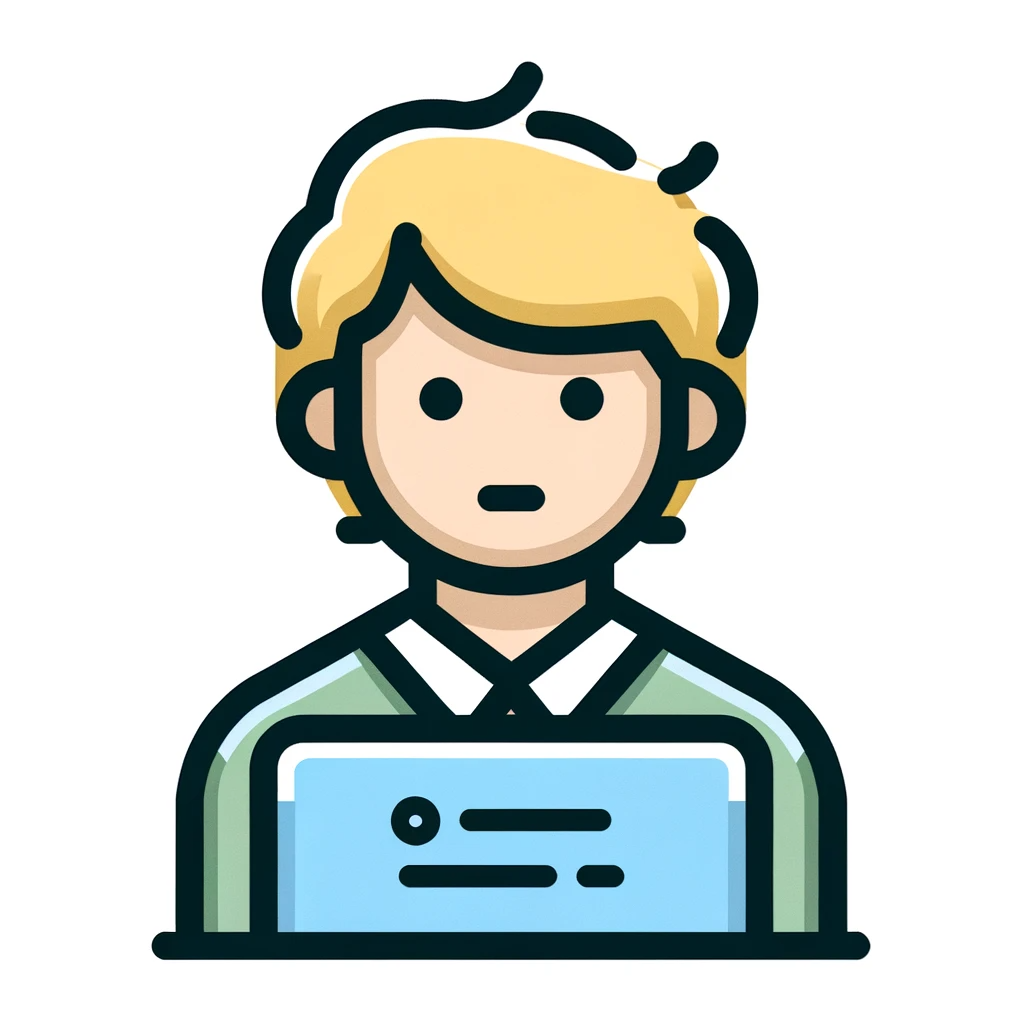
It was easy to understand how to use setAttribute, and I was able to easily set attributes!
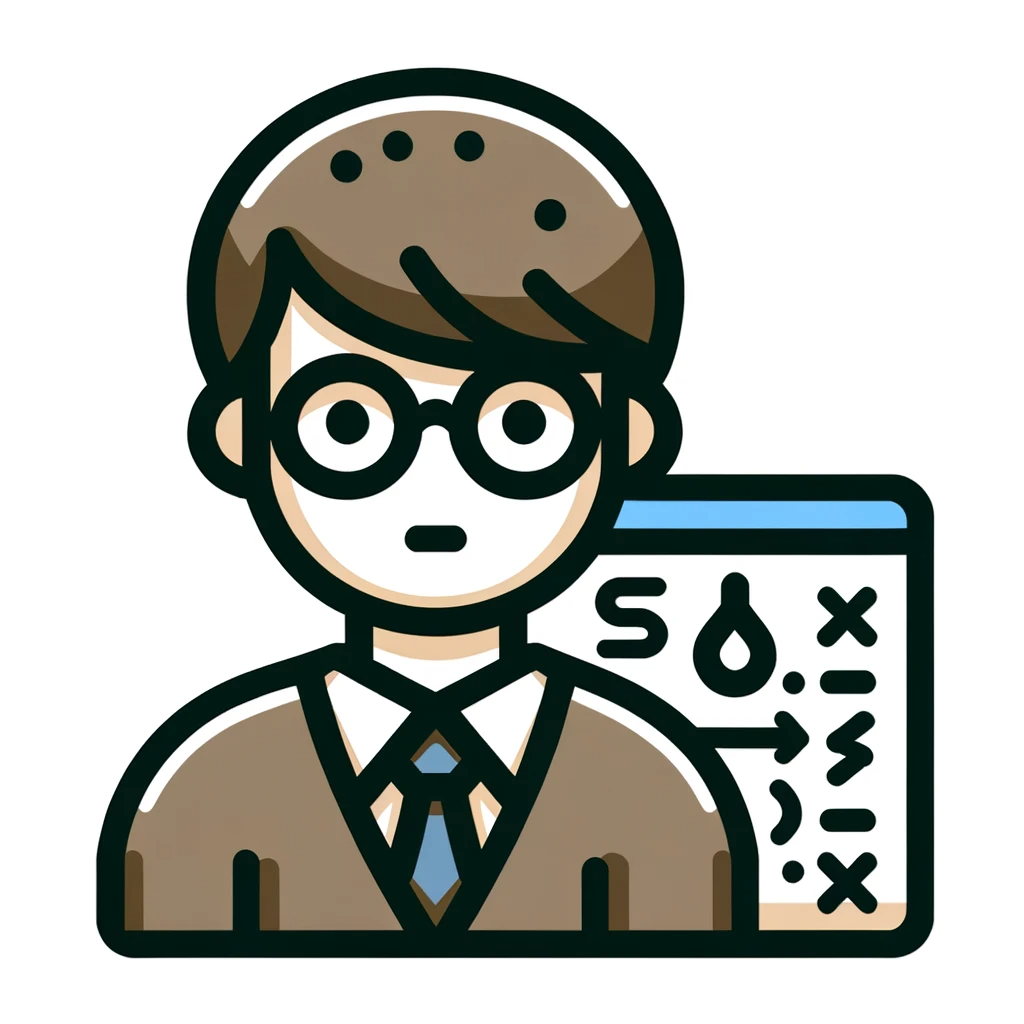
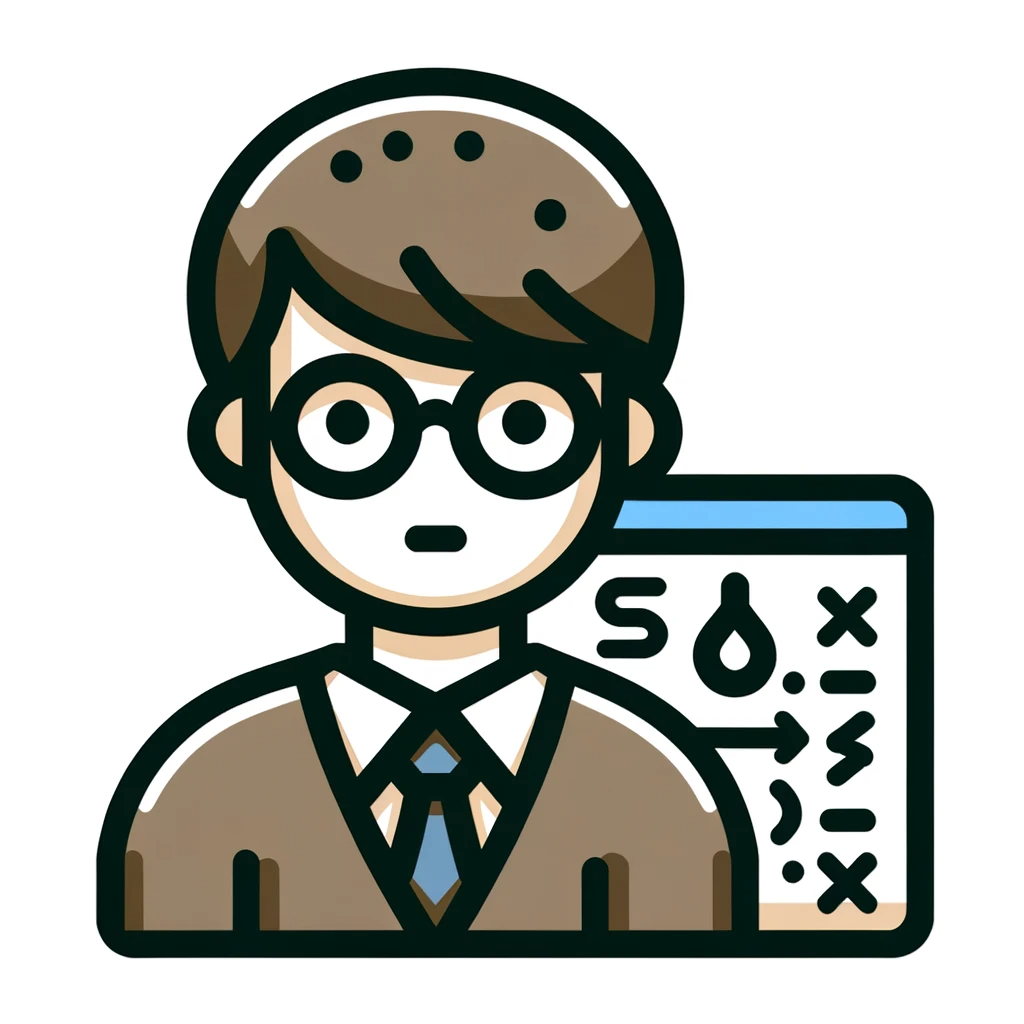
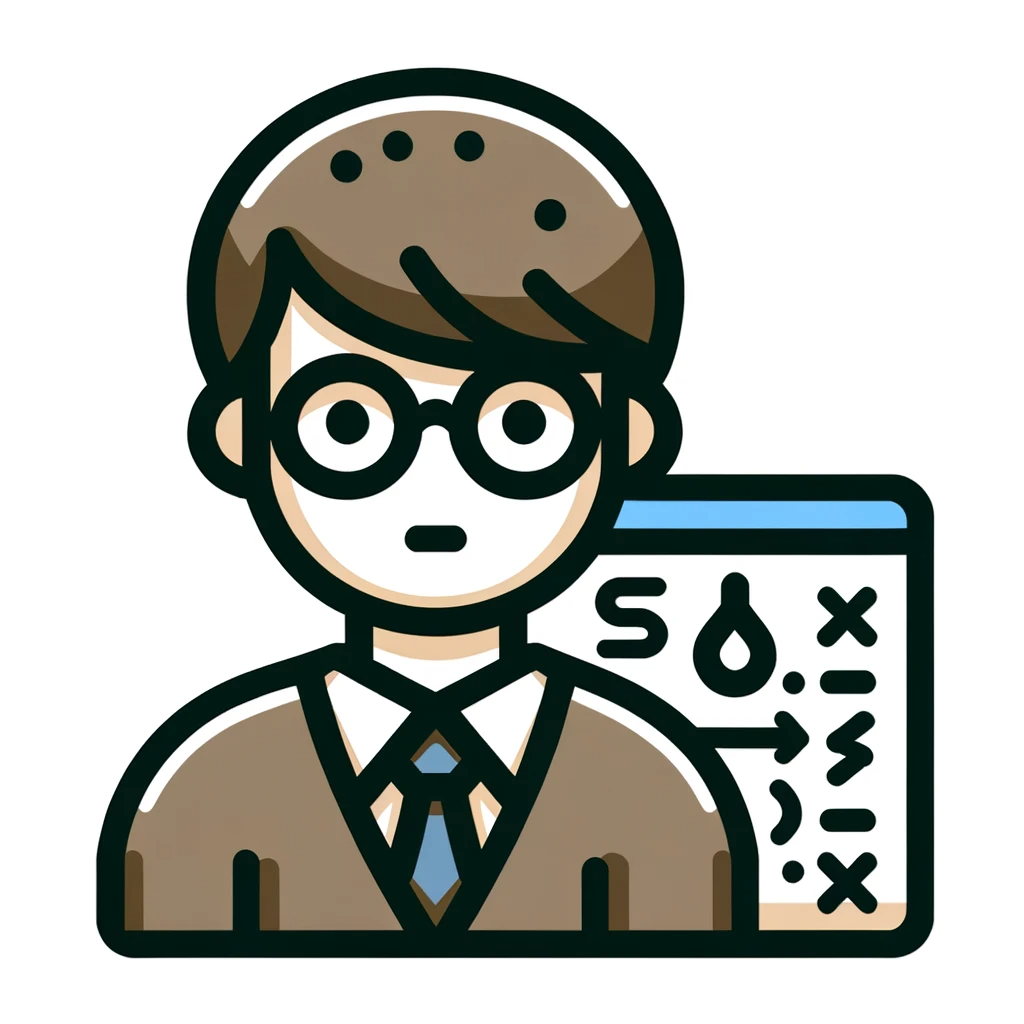
setAttribute is an essential function in web page development using JavaScript.
You can dynamically change the attributes of HTML elements to improve the user experience of your web pages.
Please try using setAttribute to improve your web pages.
Comments