This article explains how to remove duplicates from an array using JavaScript.
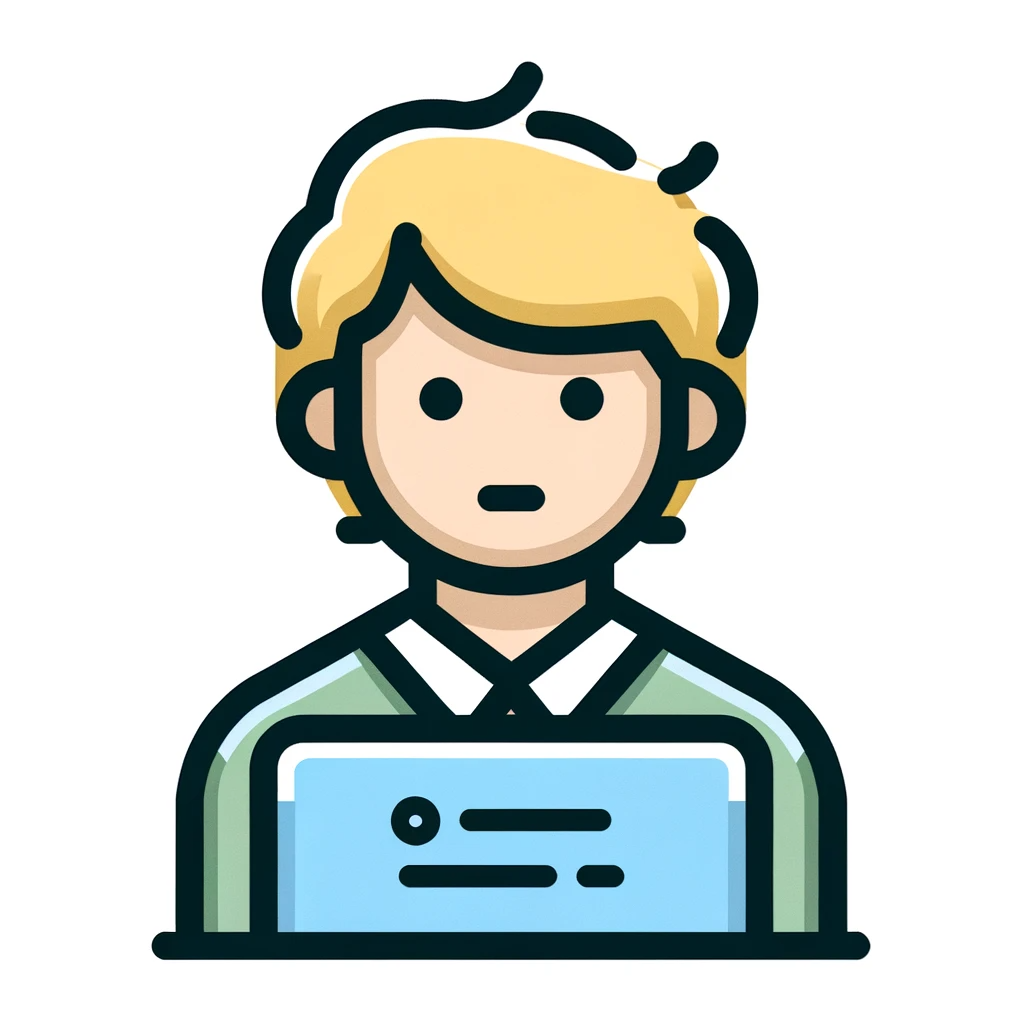
How can I remove duplicates from an array?
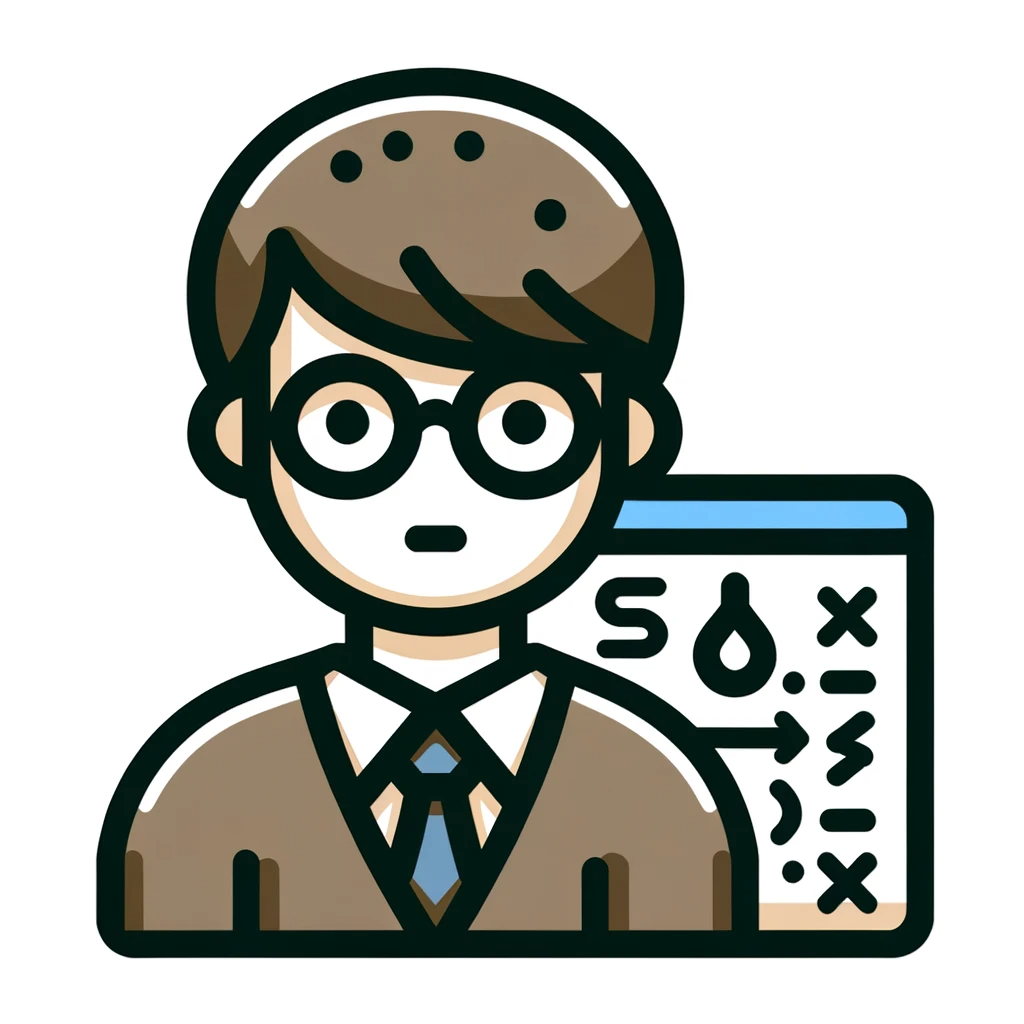
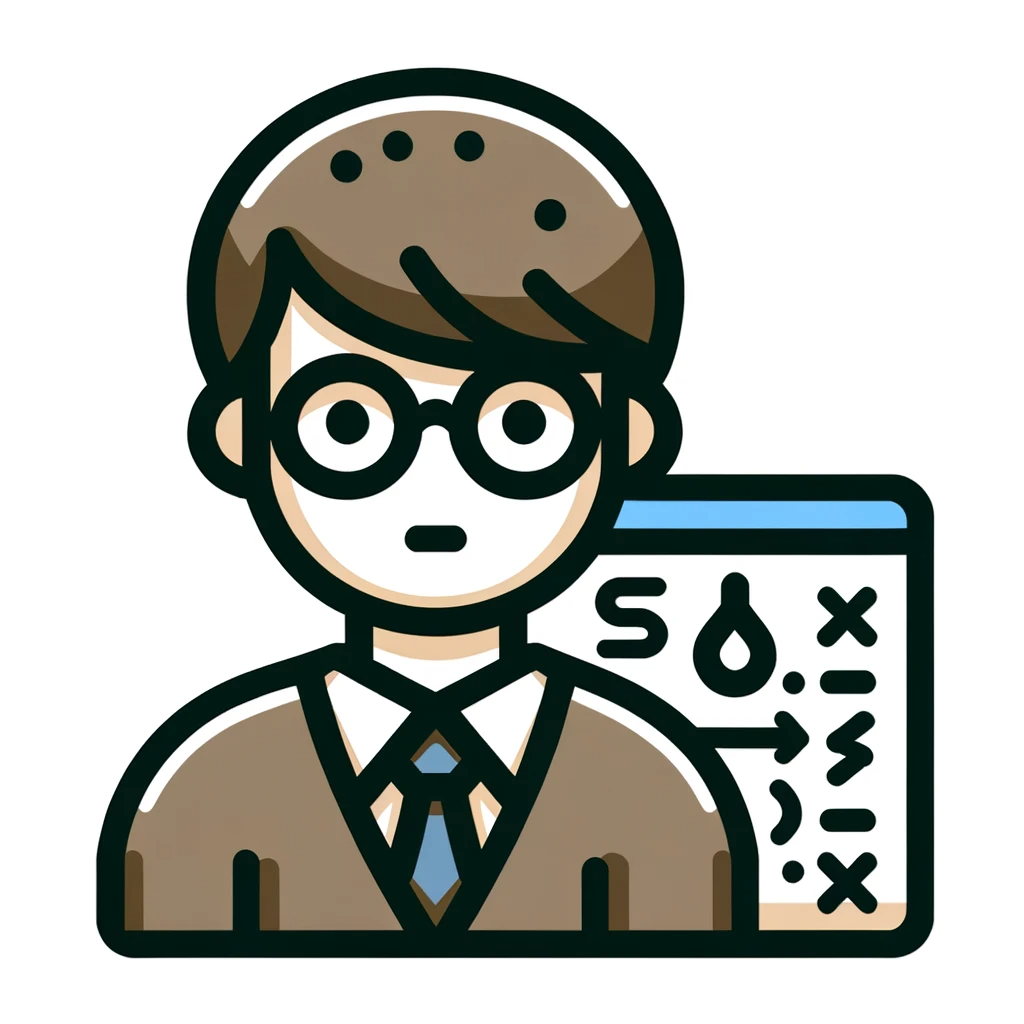
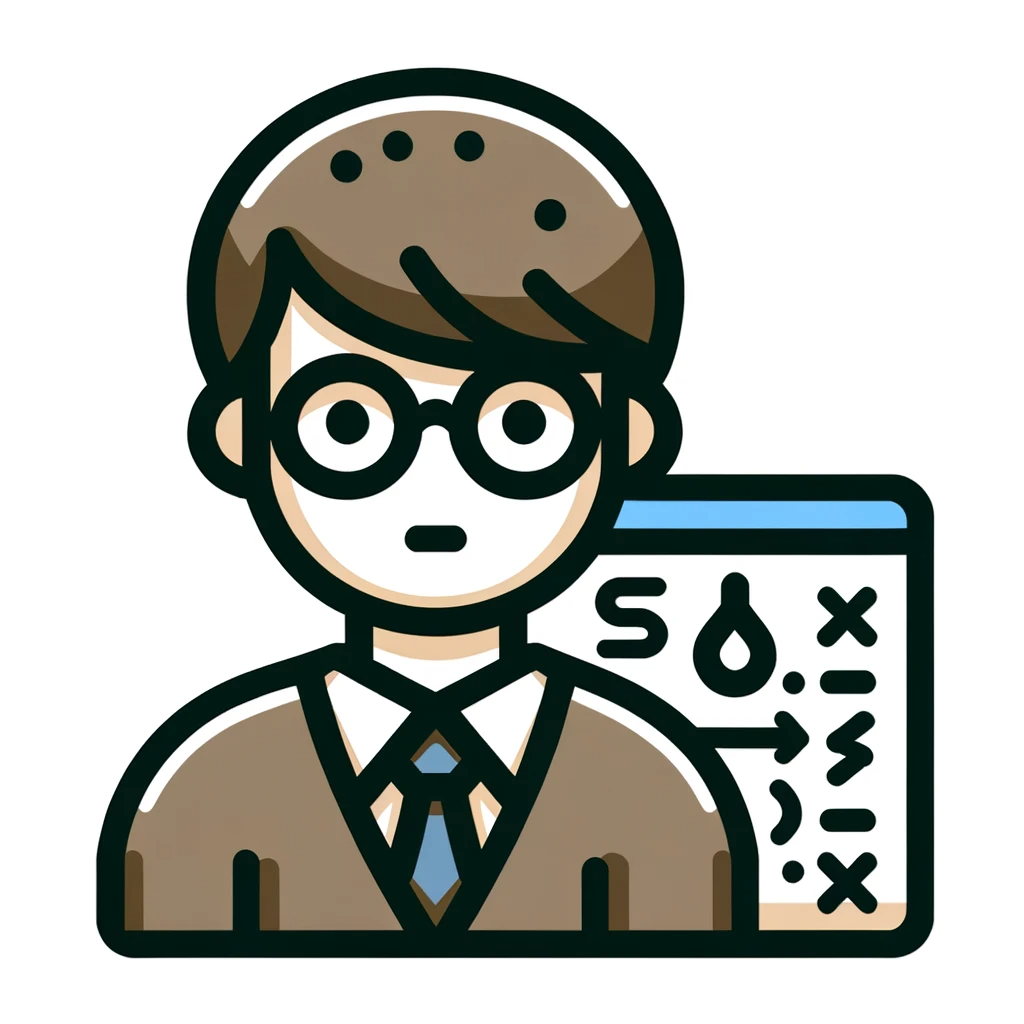
The easiest way is to use a Set object.
How to delete duplicates using Set object
A Set object is a type of collection that does not store duplicate elements.
This makes it very useful for removing duplicates.
Below is a sample code that uses a Set object to remove duplicates from an array.
let numbers = [1, 2, 3, 4, 1, 2, 5];
let uniqueNumbers = Array.from(new Set(numbers));
console.log(uniqueNumbers);
// Output: [1, 2, 3, 4, 5]
This method removes duplicates by converting the array to a Set object and then converting it back to an array.
You can also remove duplicates from an array by combining the Set object and the Spread operator .
Below is a sample code that uses Set and Spread operators to remove duplicates from an array.
let numbers = [1, 2, 3, 4, 1, 2, 5];
let uniqueNumbers = [...new Set(numbers)];
console.log(uniqueNumbers);
// Output: [1, 2, 3, 4, 5]
This method also removes duplicates by converting the array to a Set object and then converting it to an array using the Spread operator.
Summary of this article
We explained how to remove duplicates from arrays.
- A Set object is a type of collection that does not store duplicate elements.
- To remove duplicates from an array, you can use the Set object to remove duplicates.
- You can also remove duplicates from an array by combining the Set object and the Spread operator.
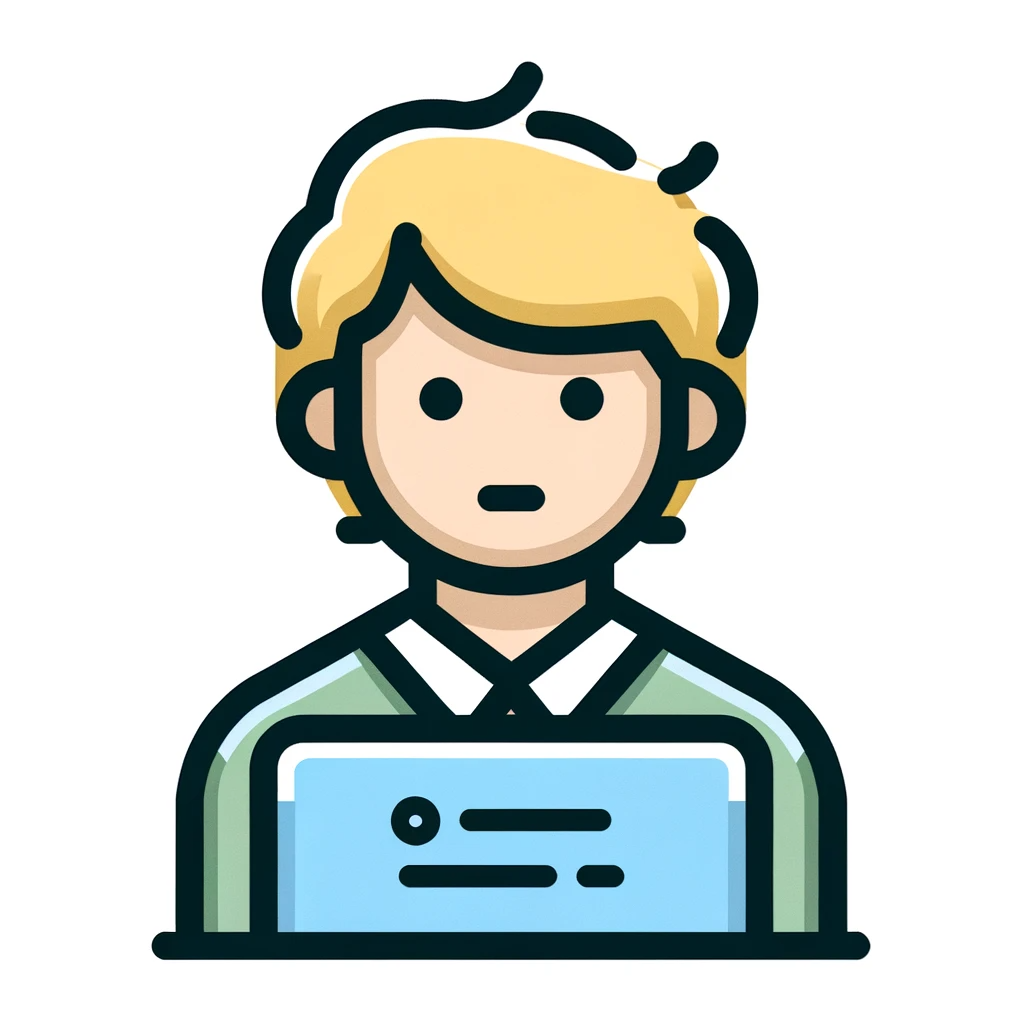
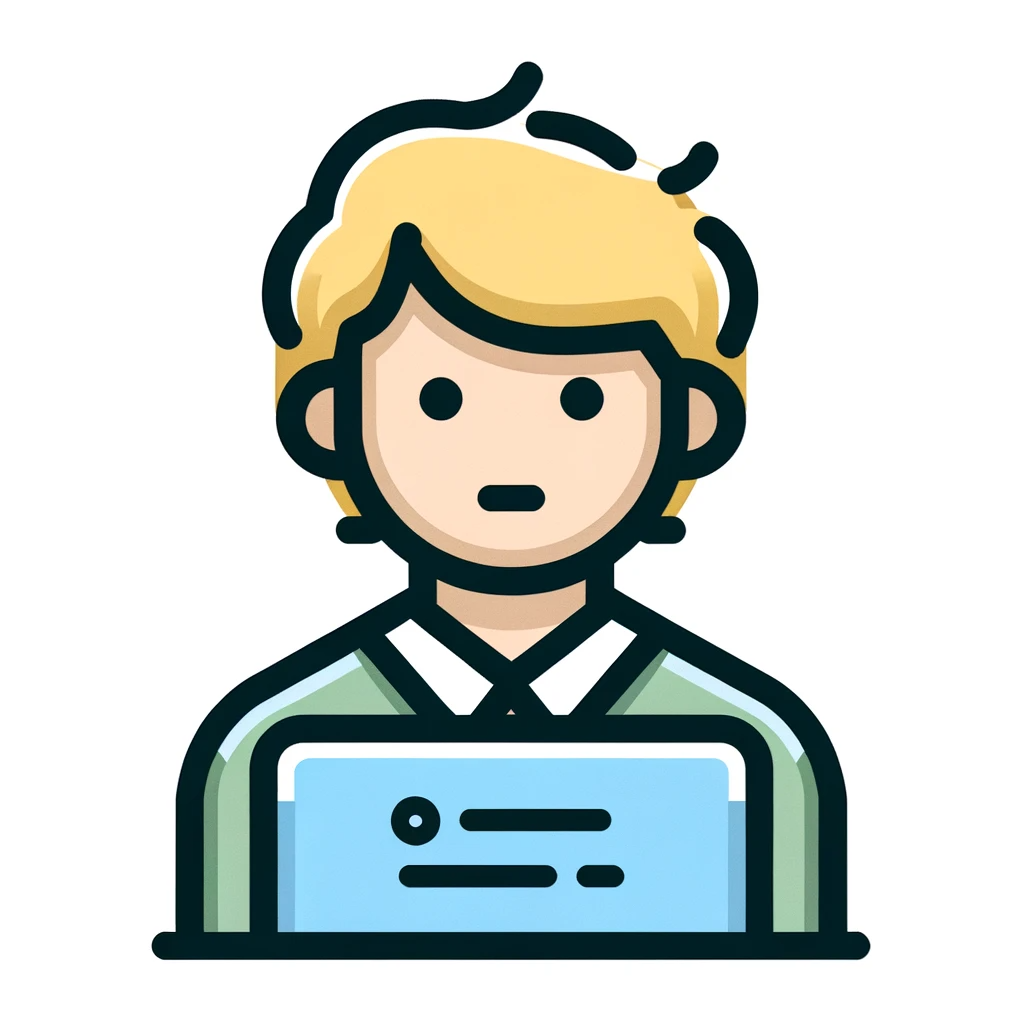
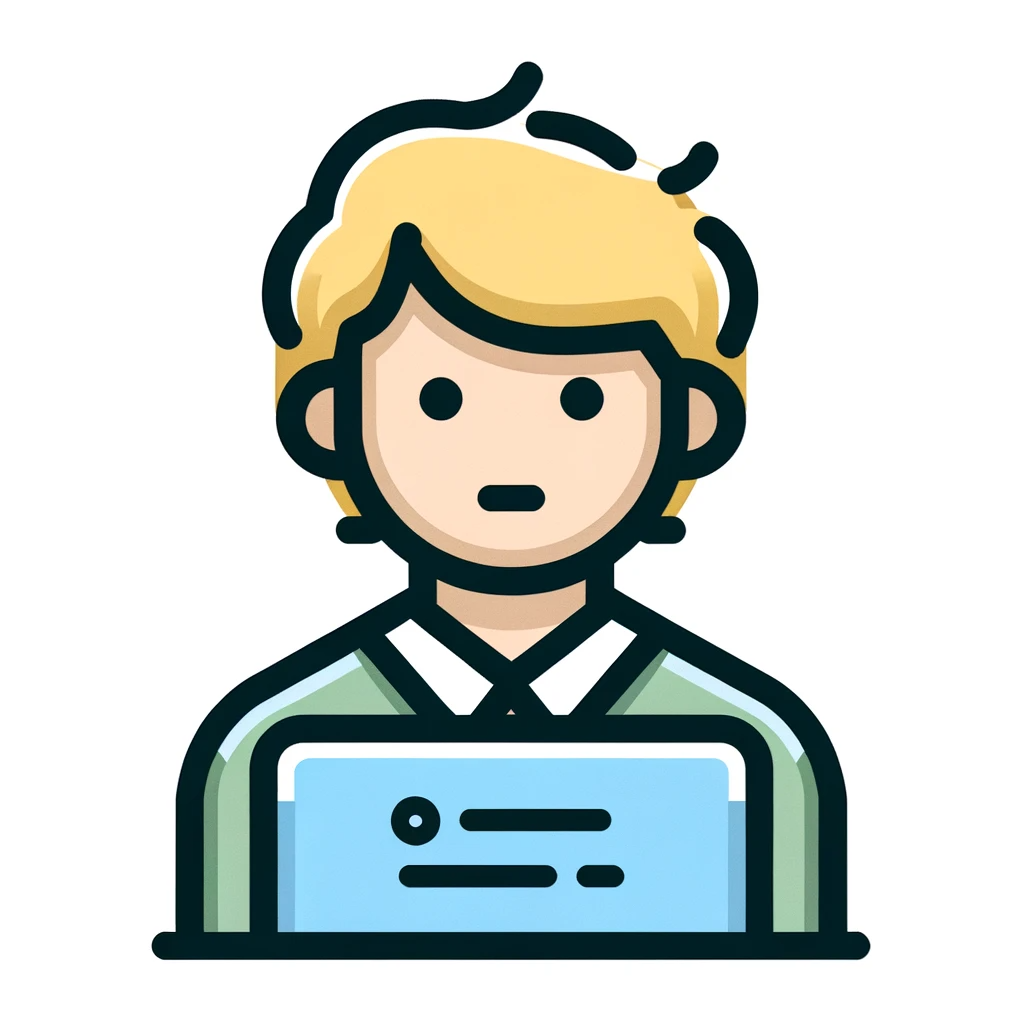
You can easily remove duplicates from an array!
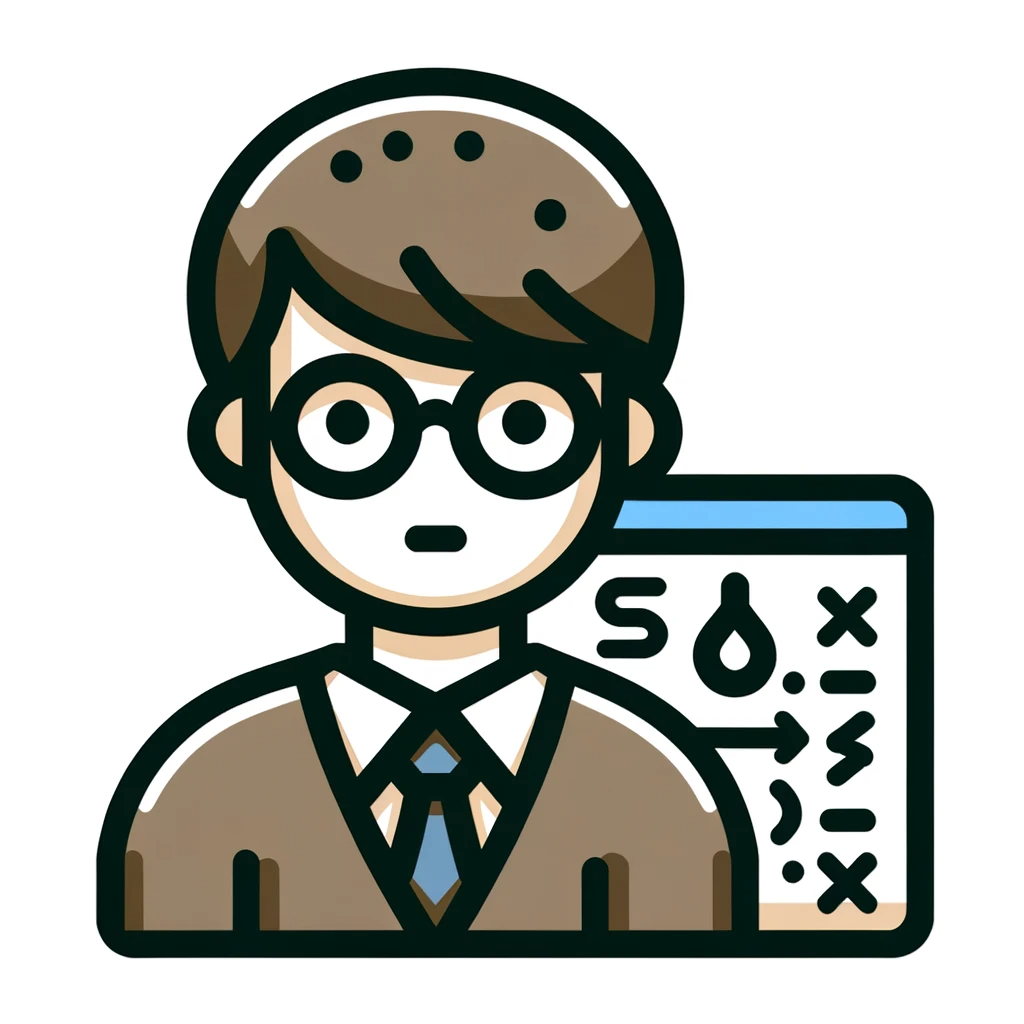
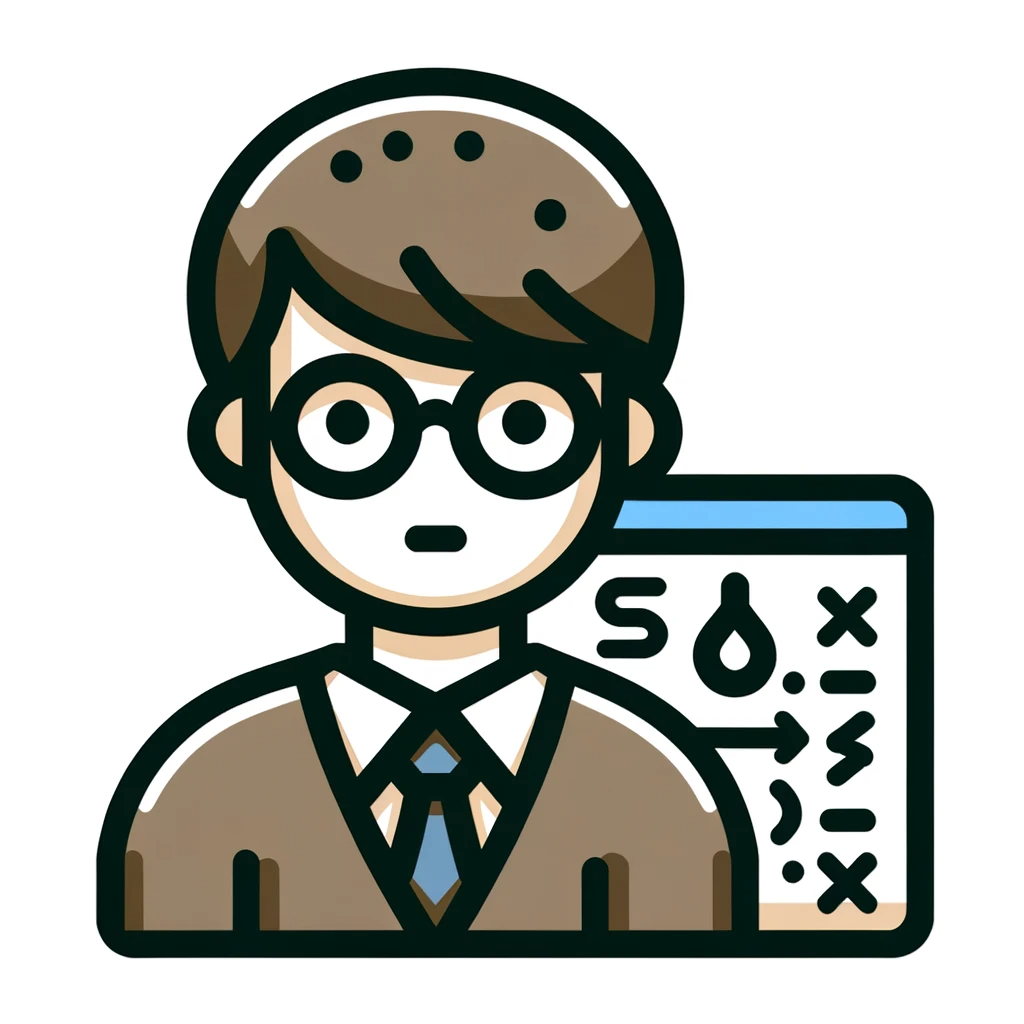
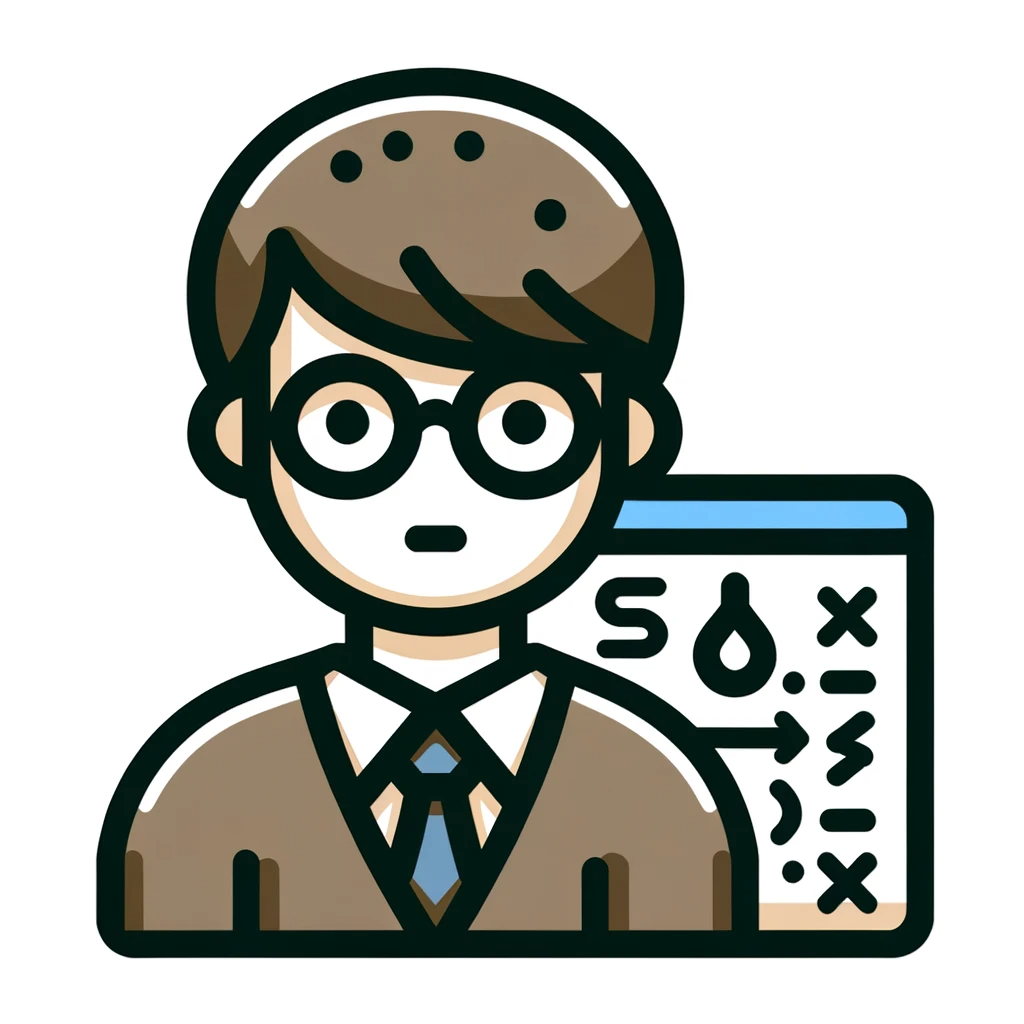
There are several ways to remove duplicates from an array.
Choosing the right method will help you write efficient and readable code.
Comments