We will explain how to replace different elements using JavaScript’s ” replaceChild method ” and ” replaceWith method ” and the differences between them.
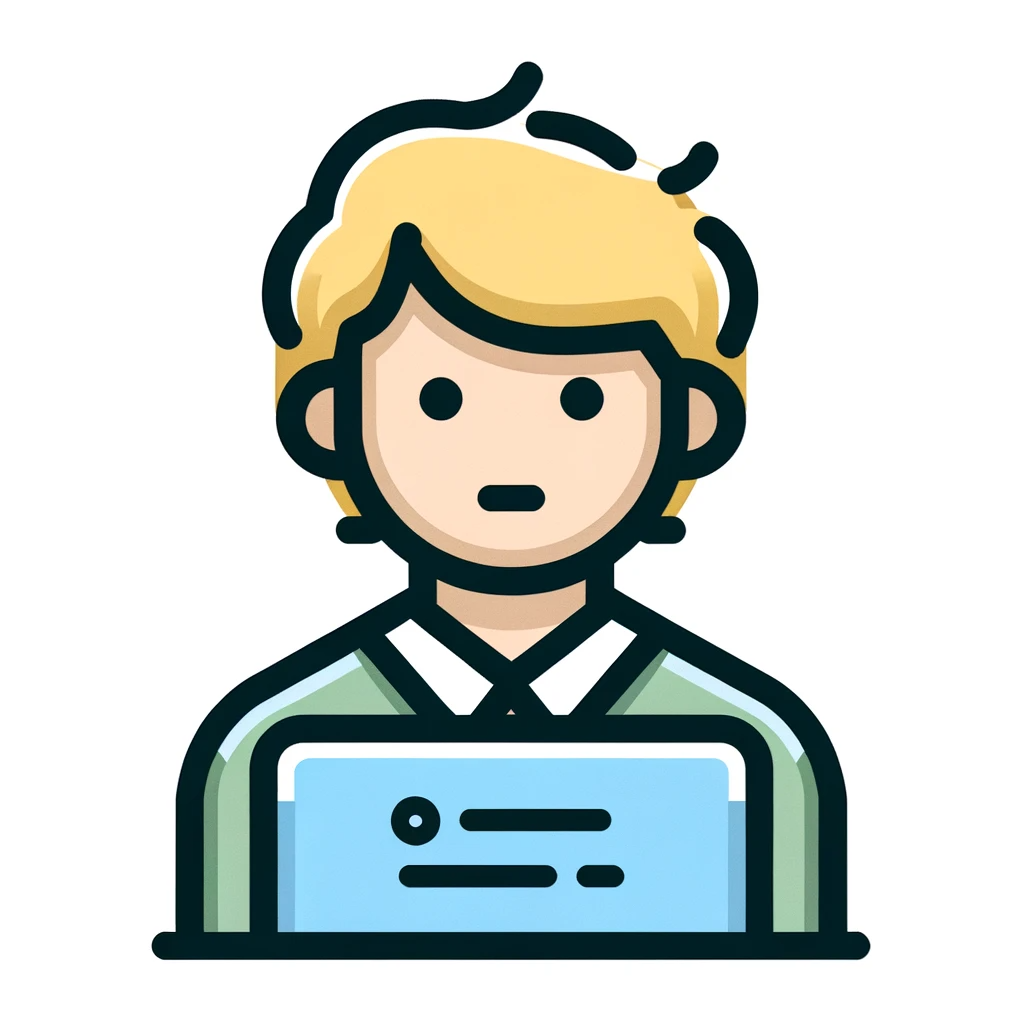
Please tell me how to replace different elements using the replaceChild method.
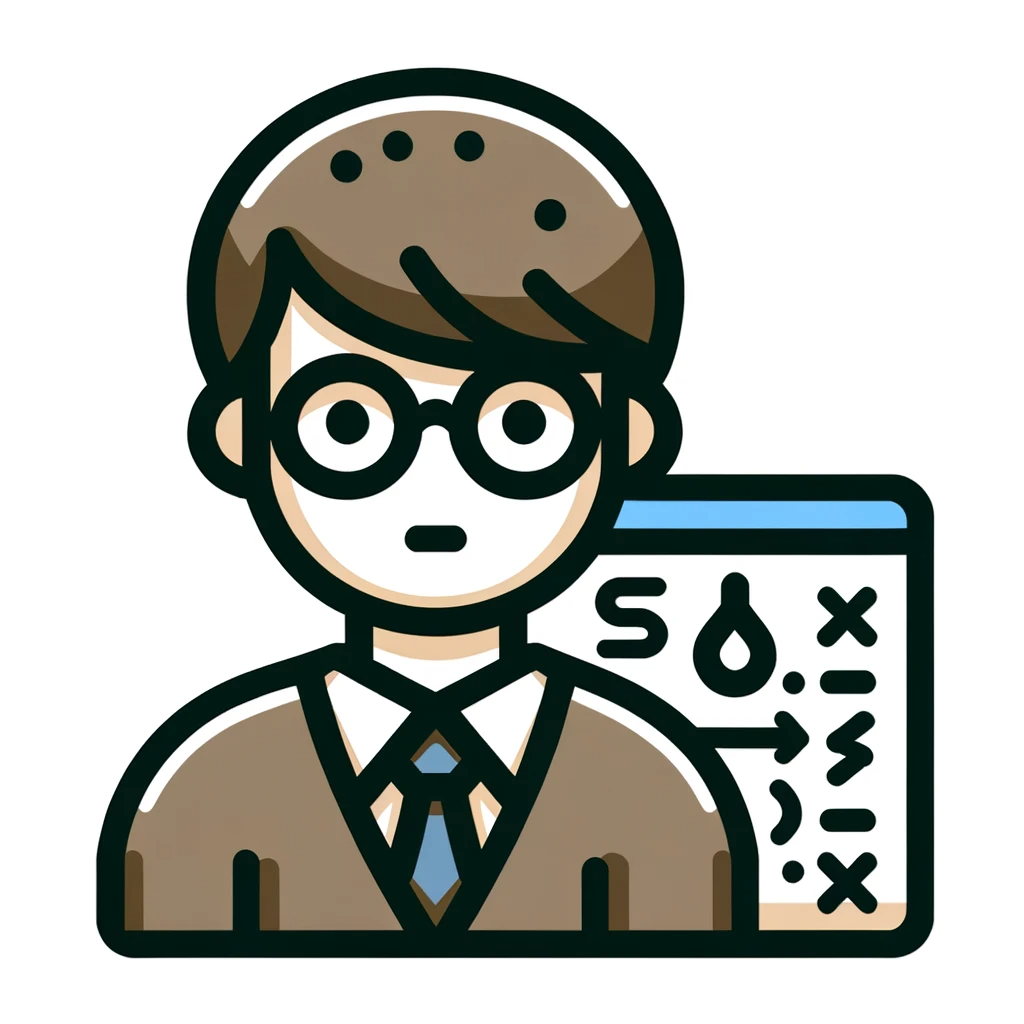
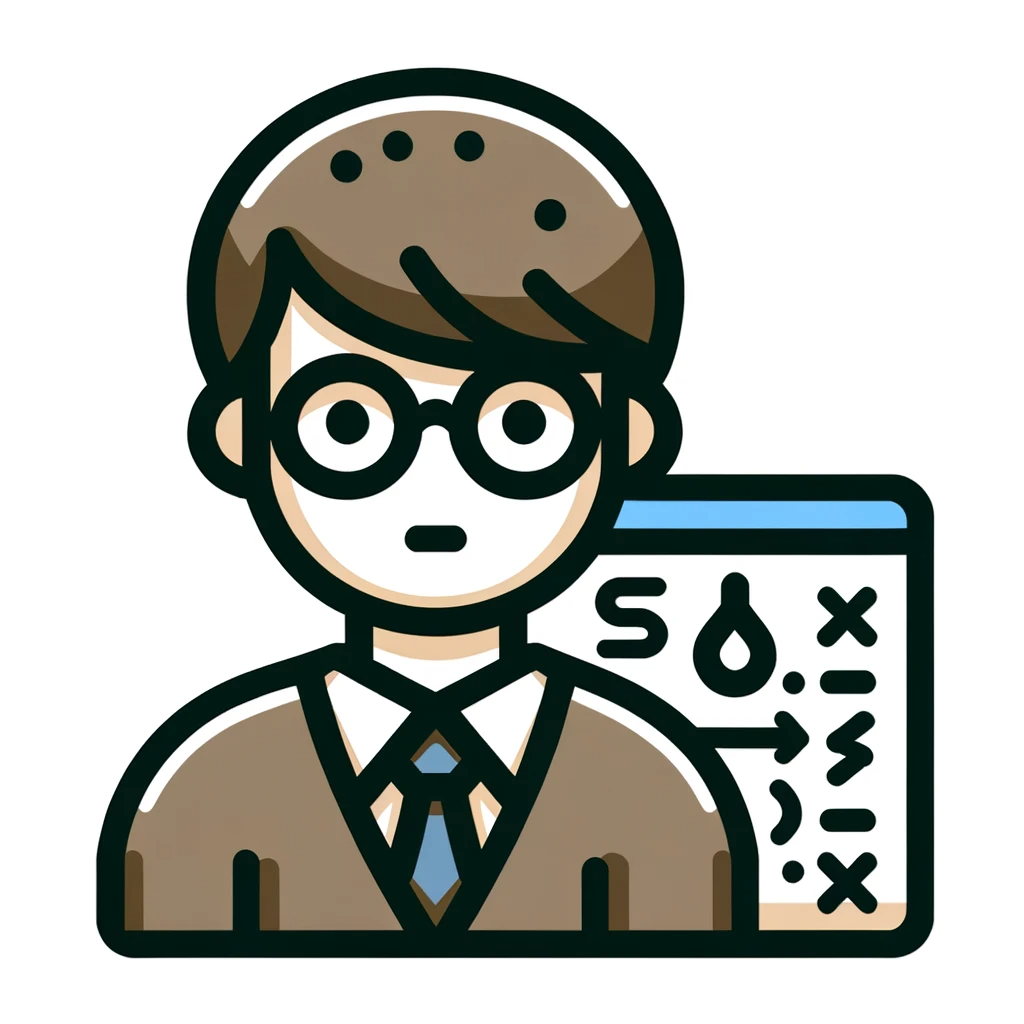
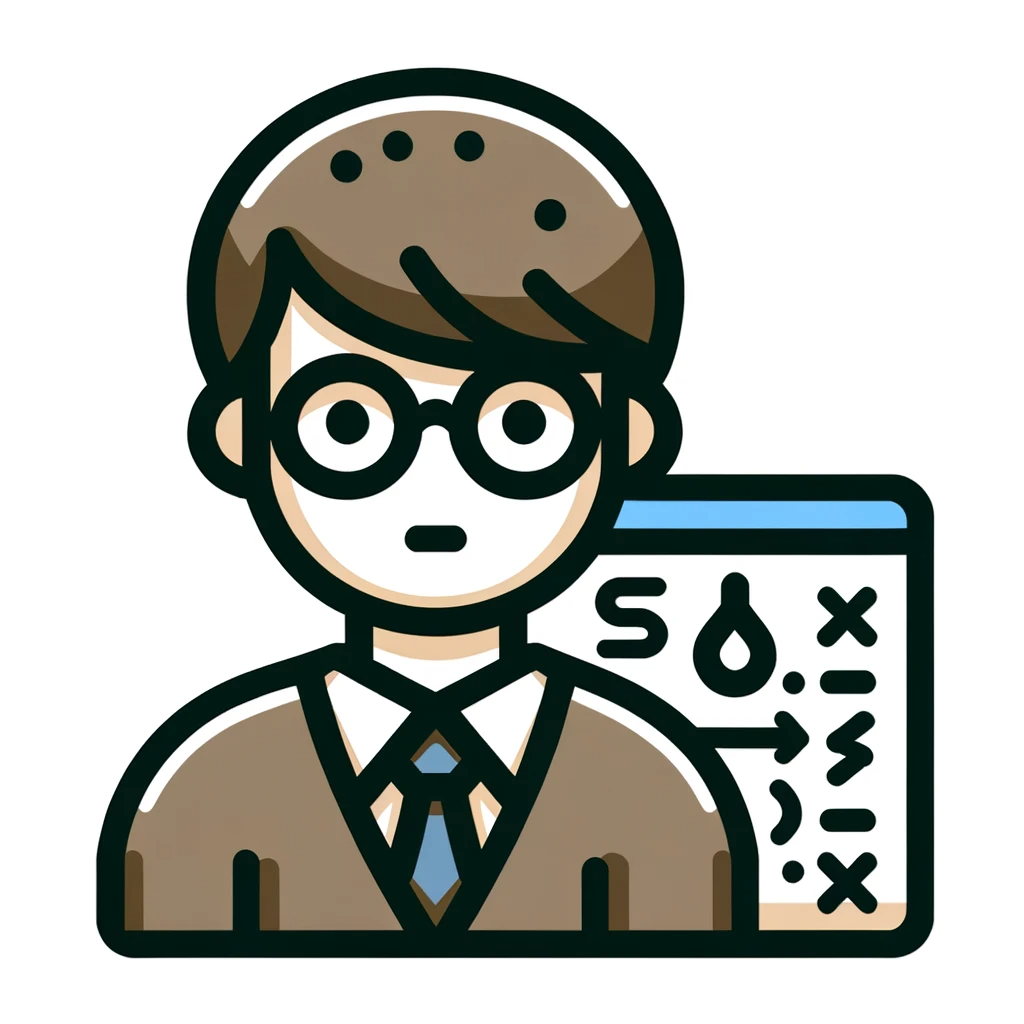
The replaceChild method is a method that allows you to replace a specified existing child element within a parent element with a new child element.
You can use this to swap different elements.
What is the replaceChild method?
The replaceChild method replaces the child element of the specified parent element with another element.
This method allows you to replace an element by specifying the element to be replaced and the element to replace from among the child elements under the specified parent element in the DOM tree.
How to use replaceChild method
Use the replaceChild method by specifying the parent element, the element before replacement, and the element after replacement .
parentelement.replaceChild(new child element, existing child element to replace)
- Parent element: Specify the parent element to which the new child element will be added.
- New child element: Specify the new element to add.
- Existing child element to replace: Specify the element to replace.
Below is an example of basic usage of the replaceChild method.
const parentElement = document.querySelector('#parent');
const oldElement = document.querySelector('#old');
const newElement = document.createElement('div');
newElement.textContent = 'New Element';
parentElement.replaceChild(newElement, oldElement);
This example replaces the child element with id “old” under the parent element with id “parent” with a new div element. The first argument of the replaceChild method specifies the new element, and the second argument specifies the element to be replaced. I am creating a new element using the createElement method and setting the element’s text using the textContent property.
The replaceChild method can also replace multiple elements at once. Below is an example of replacing multiple elements.
const parentElement = document.querySelector('#parent');
const oldElements = document.querySelectorAll('.old');
const newElement = document.createElement('div');
newElement.textContent = 'New Element';
oldElements.forEach(oldElement => {
parentElement.replaceChild(newElement, oldElement);
});
This example replaces all elements with class “old” with new div elements. I am using the querySelectorAll method to get all the elements of the “old” class and using the forEach method to replace each element.
The replaceChild method replaces an element by specifying the element to be replaced and the element to replace from among the child elements under the specified parent element, so it is necessary to accurately understand the hierarchical structure of the elements before using it. there is.
Also, when using the replaceChild method, care must be taken to ensure that the element being replaced is a more exact match than the element being replaced. If the replacement destination element does not exactly match the replacement element, an unintended element may be replaced, so be careful.
What is replaceWith method?
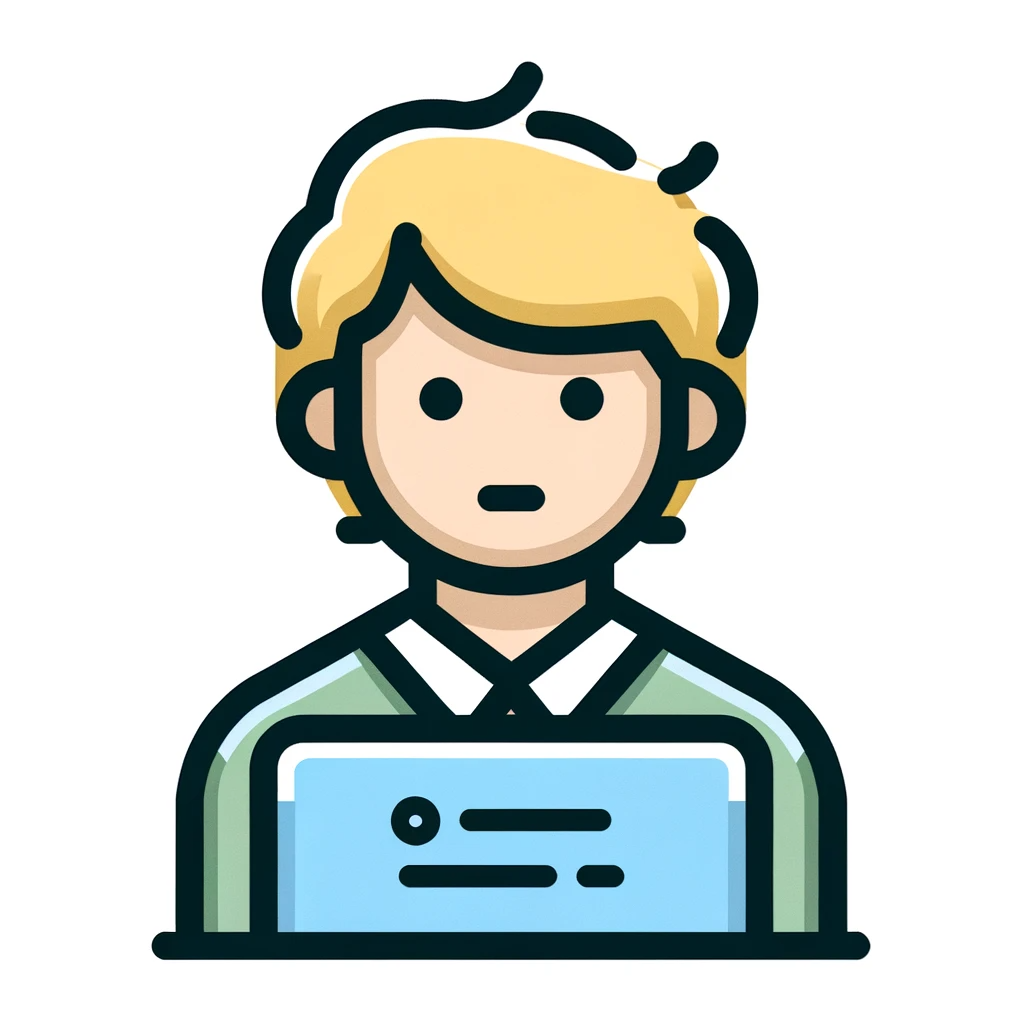
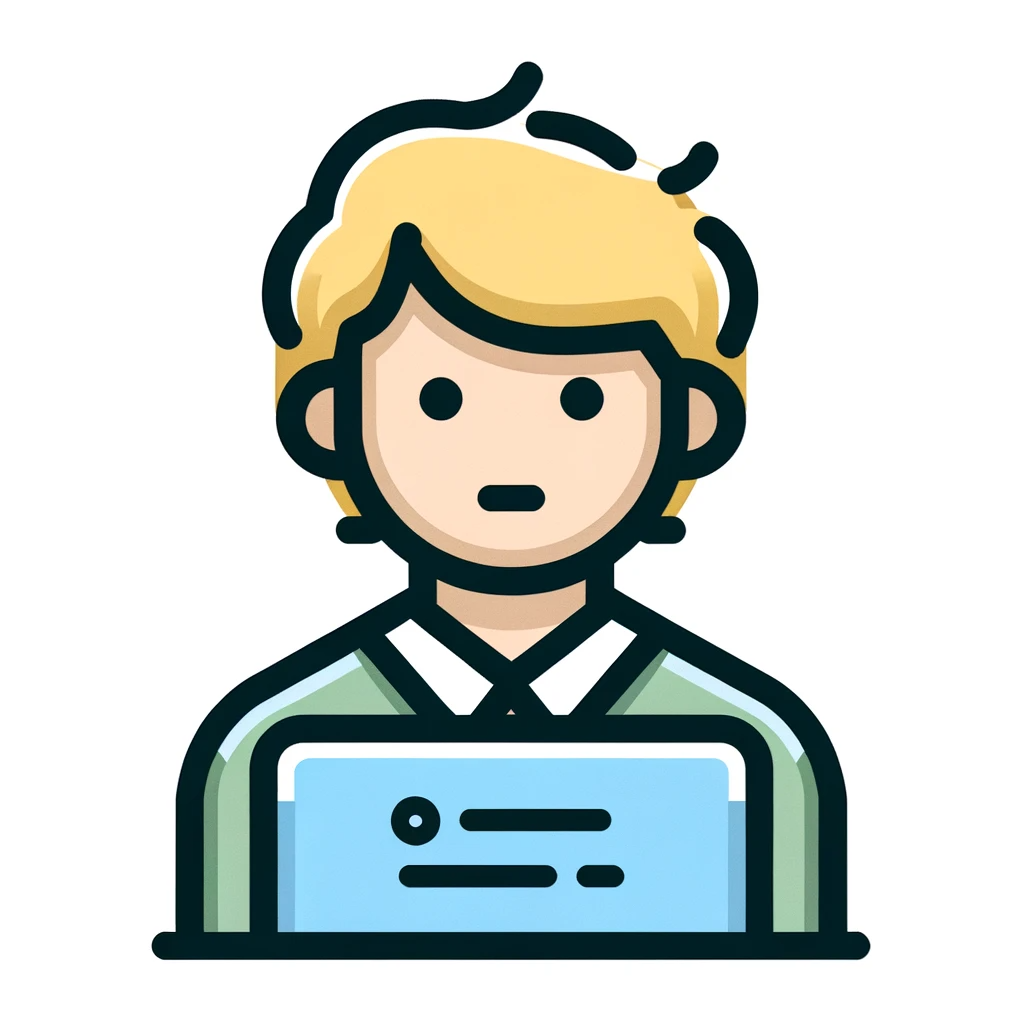
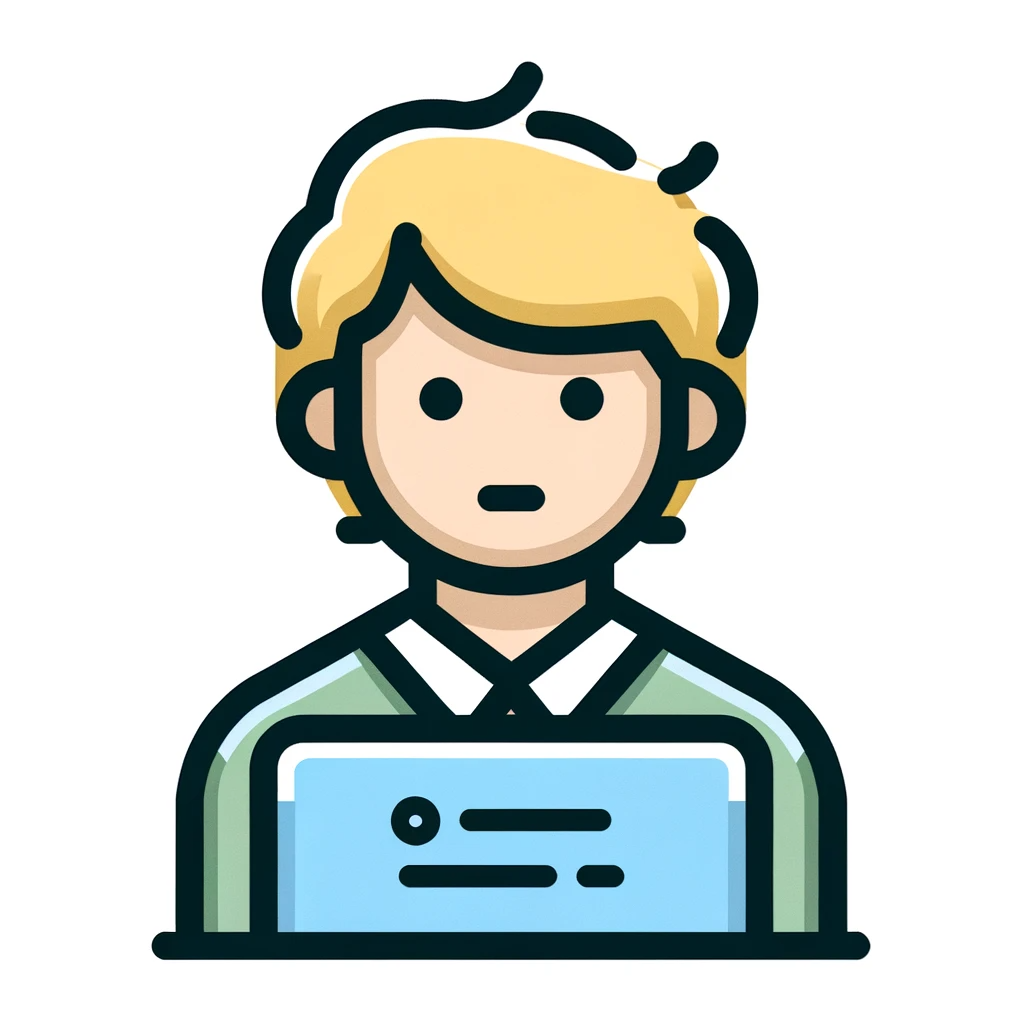
In addition to the replaceChild method, there seems to be a replaceWith method, but what is the difference?
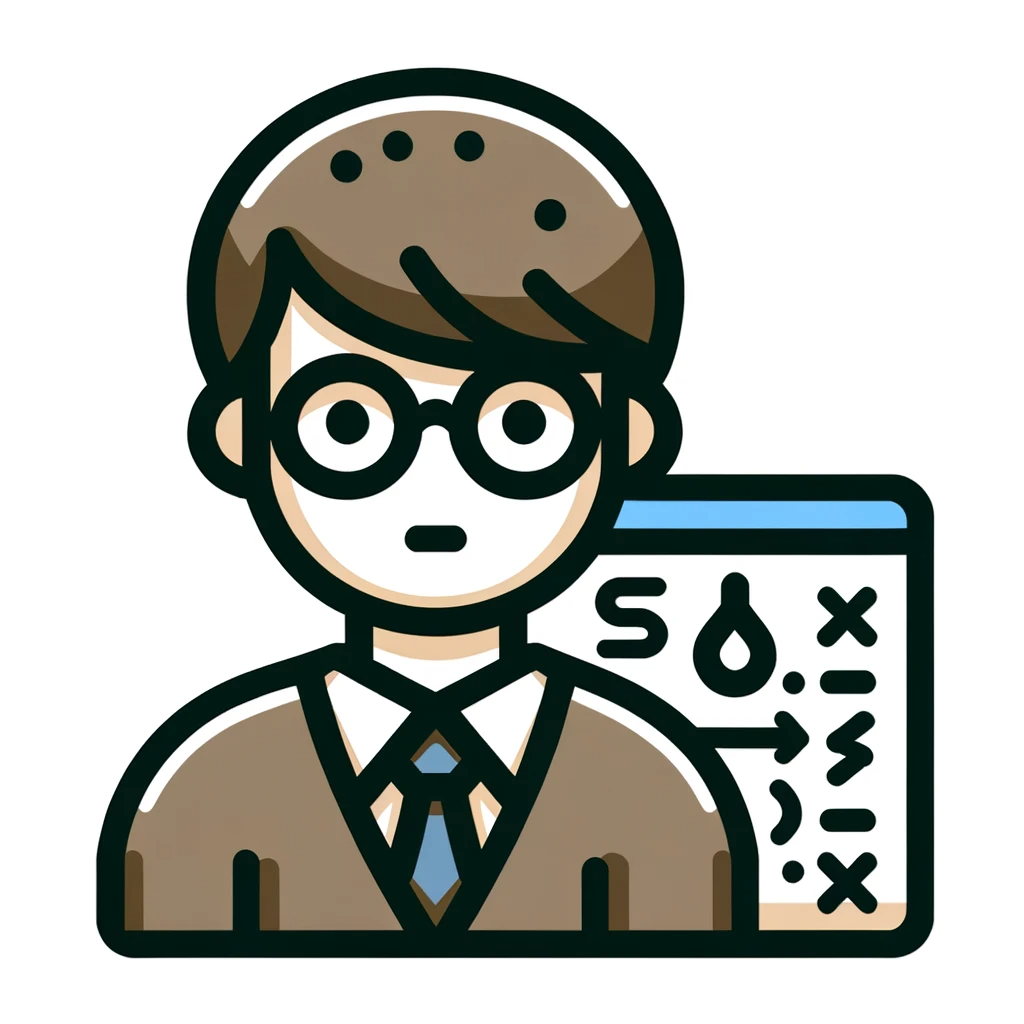
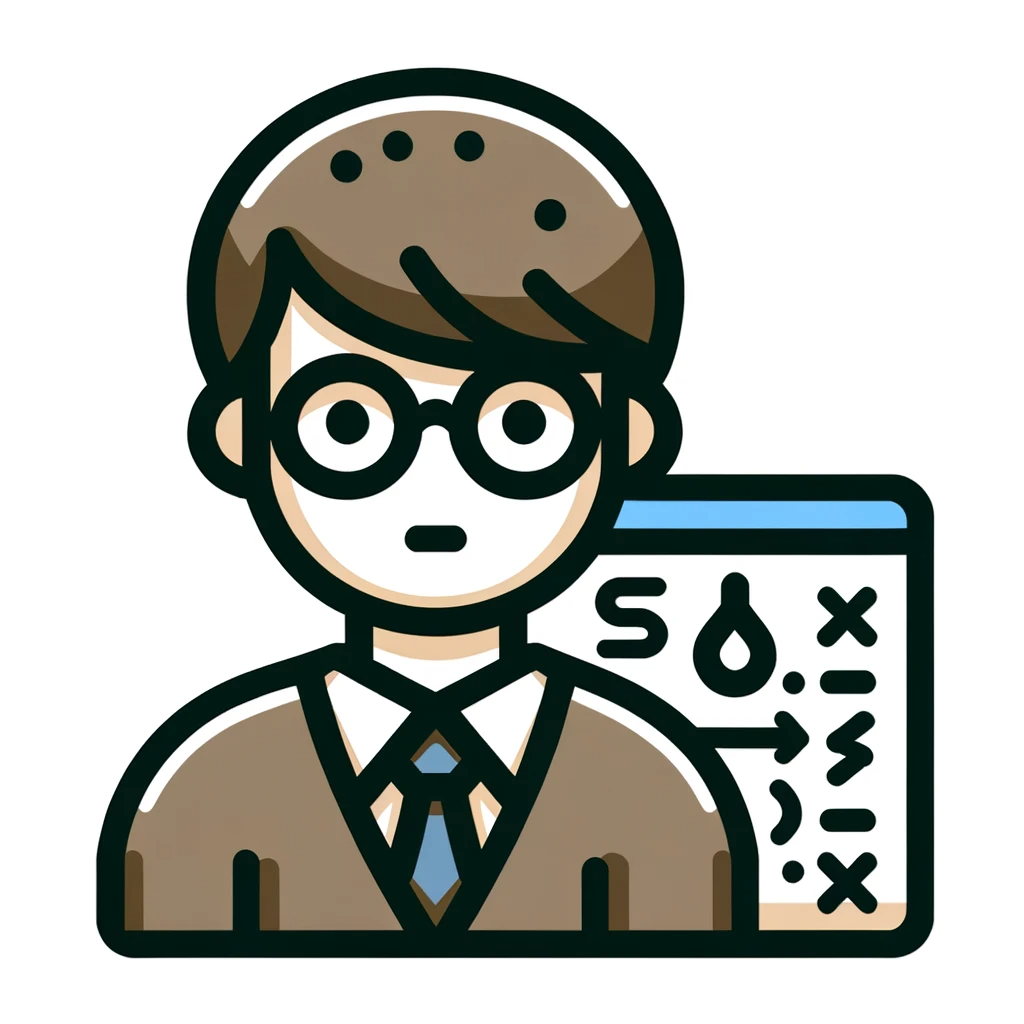
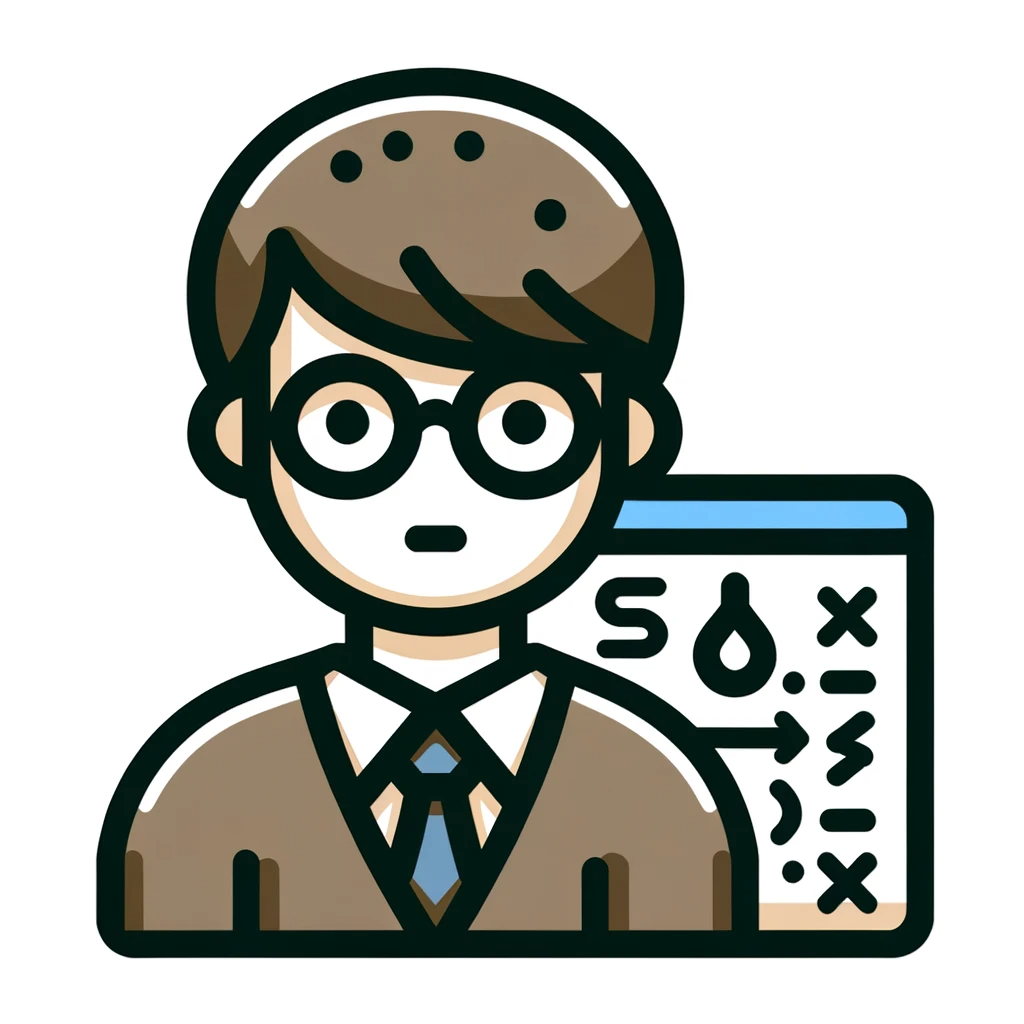
The replaceWith method, like the replaceChild method, is used to replace an element, but you don’t have to specify the source element, just the element you want to replace.
The replaceWith method replaces the specified element with another element, text, etc. This method is considered easier to use than the replaceChild method because you only need to specify the element to be replaced as an argument, and there is no need to specify the source element.
However, the replaceWith method is a relatively new JavaScript feature and older browsers may not support it, so you should be aware of browser support when using it.
How to use replaceWith method
You can use the replaceWith method by simply specifying the element to be replaced as an argument .
element before replacement.replaceWith(element after replacement);
The following is an example of basic usage of the replaceWith method.
const oldElement = document.querySelector('#old');
const newElement = document.createElement('div');
newElement.textContent = 'New Element';
oldElement.replaceWith(newElement);
In this example, the element with id “old” is replaced with a new div element. You can specify the element to be replaced in the replaceWith method argument. In this example, the createElement method creates a new element and the textContent property sets the element’s text.
The replaceWith method can also replace multiple elements at once. Below is an example of replacing multiple elements.
const oldElements = document.querySelectorAll('.old');
const newElement = document.createElement('div');
newElement.textContent = 'New Element';
oldElements.forEach(oldElement => {
oldElement.replaceWith(newElement);
});
This example replaces all elements with class “old” with new div elements. I am using the querySelectorAll method to get all the elements of the “old” class and using the forEach method to replace each element.
The replaceWith method is a relatively new JavaScript feature and may not be supported by older browsers, so you should be aware of browser support when using it.
Also, while the replaceWith method is a useful method for dynamically changing elements, you should be aware that using it more often than necessary can impair the performance of your web page.
summary
We explained how to replace different elements using the “replaceChild method” and “replaceWith method” and the differences between them.
Summary of replaceChild method
- You can replace a child element under a specified parent element with another element.
- Delete the element before replacement and add the element after replacement.
- It is necessary to accurately understand the hierarchical structure before using it.
- Care must be taken to ensure that the replaced element matches more precisely than the replaced element.
Summary of replaceWith method
- You can replace the specified element with another element.
- Delete the element before replacement and add the element after replacement.
- It is also possible to replace multiple elements at once.
- Some browsers may not support it, so you need to check the support status of your browser before using it.
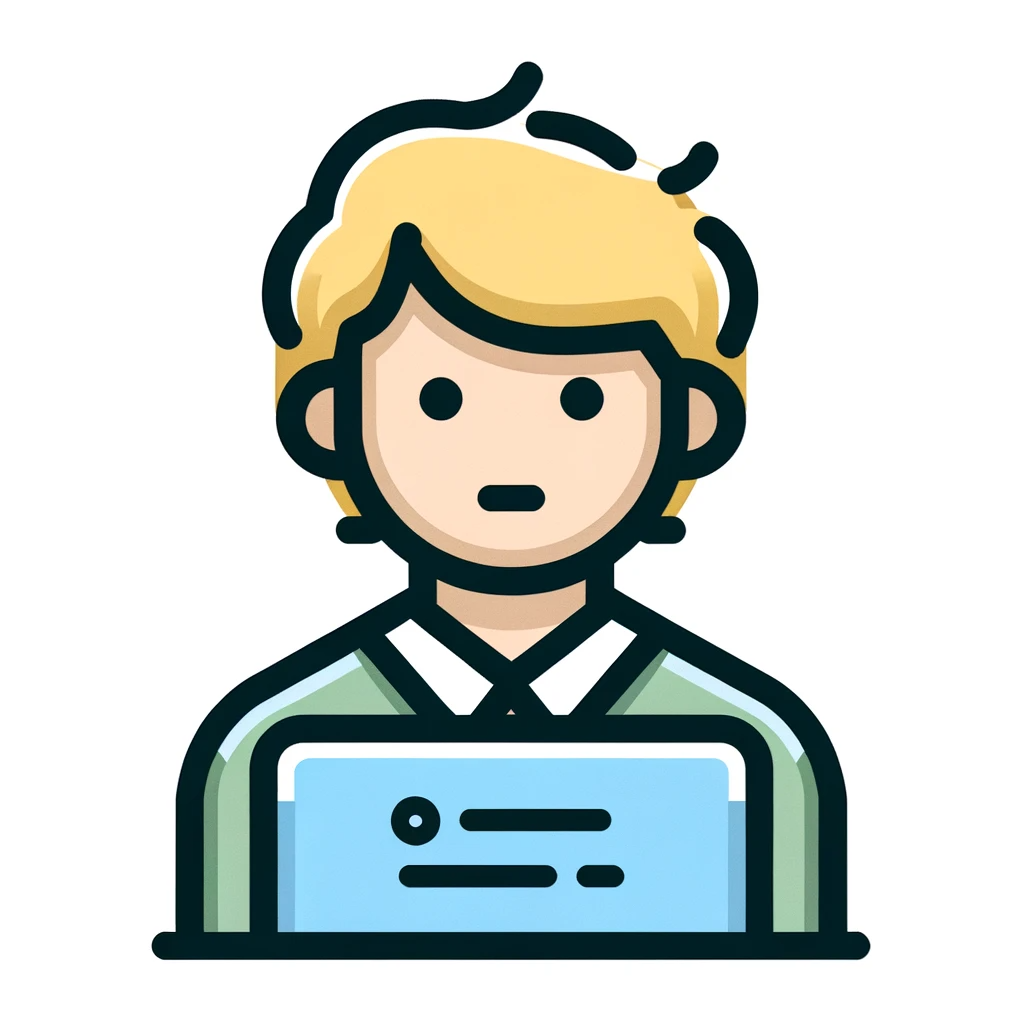
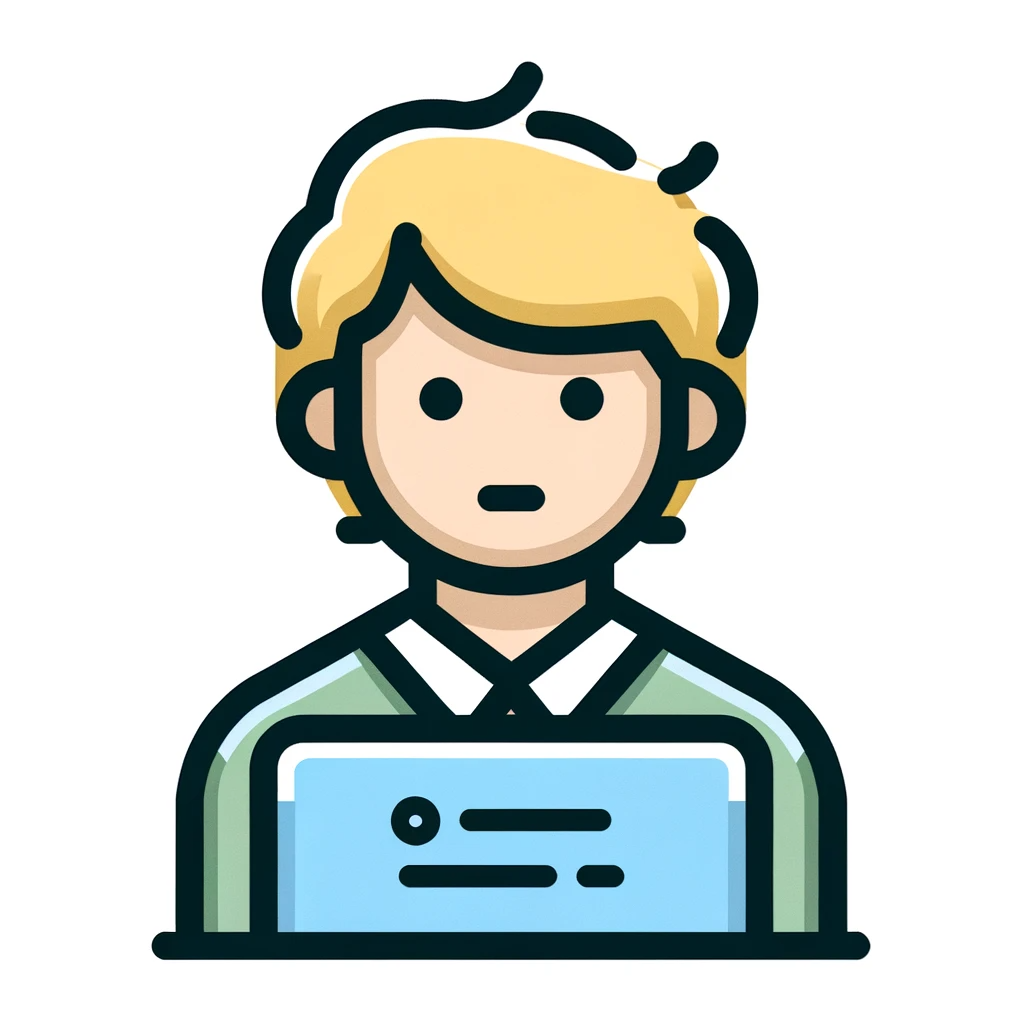
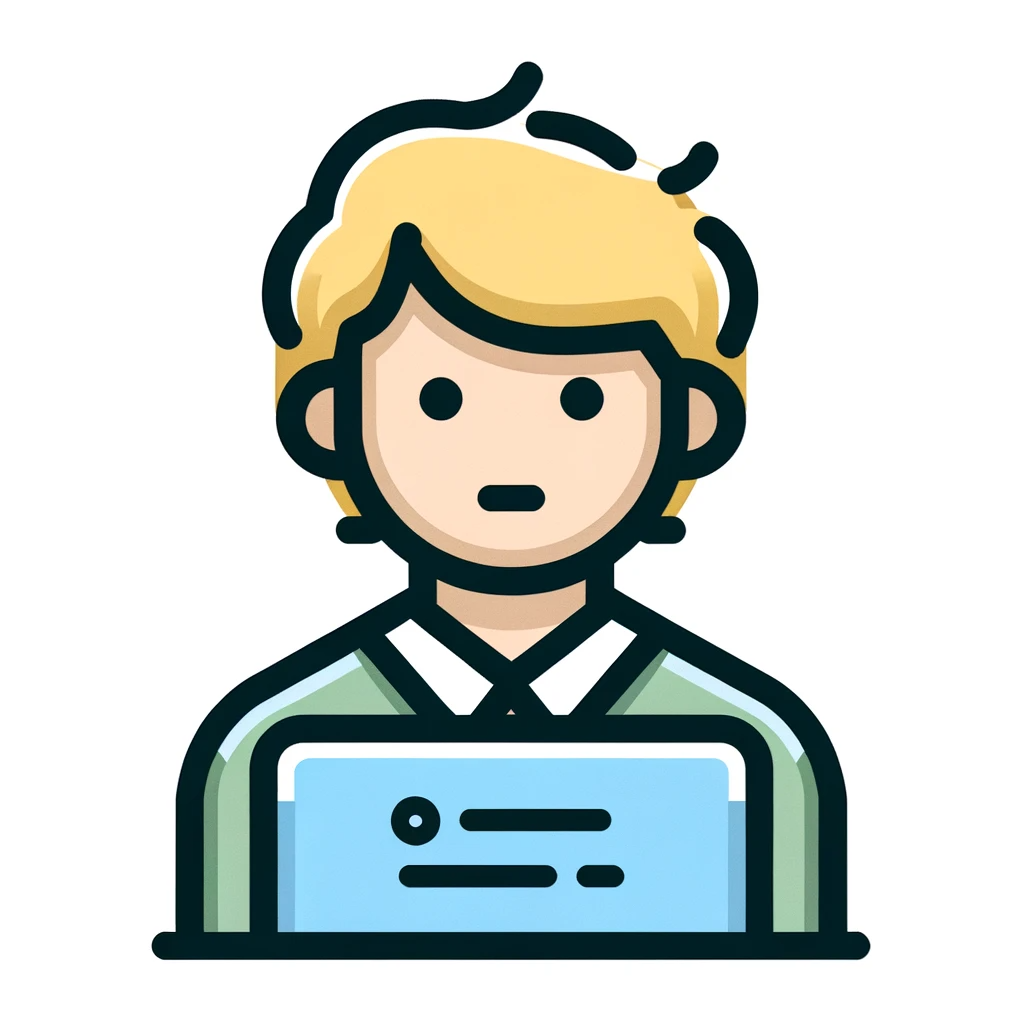
You can replace existing elements with new elements.
Being able to dynamically change web page elements is convenient!
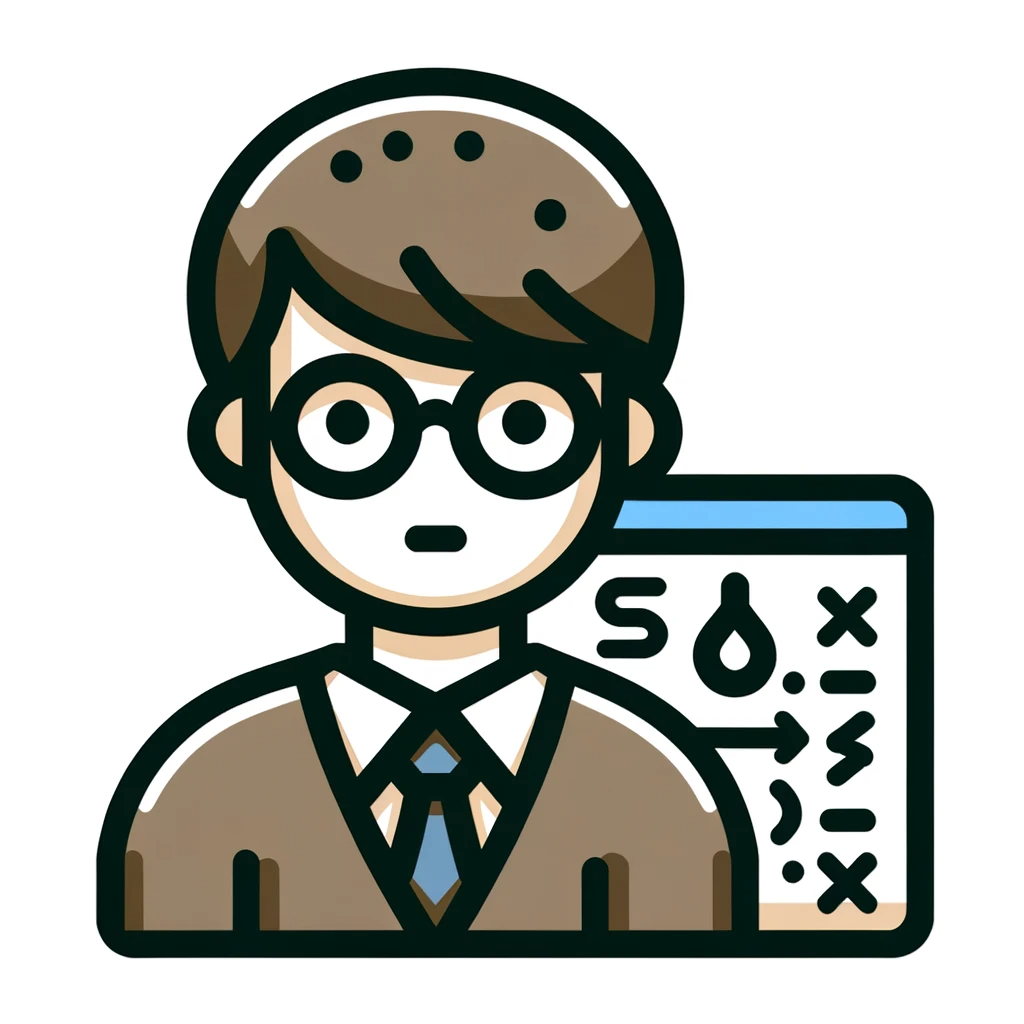
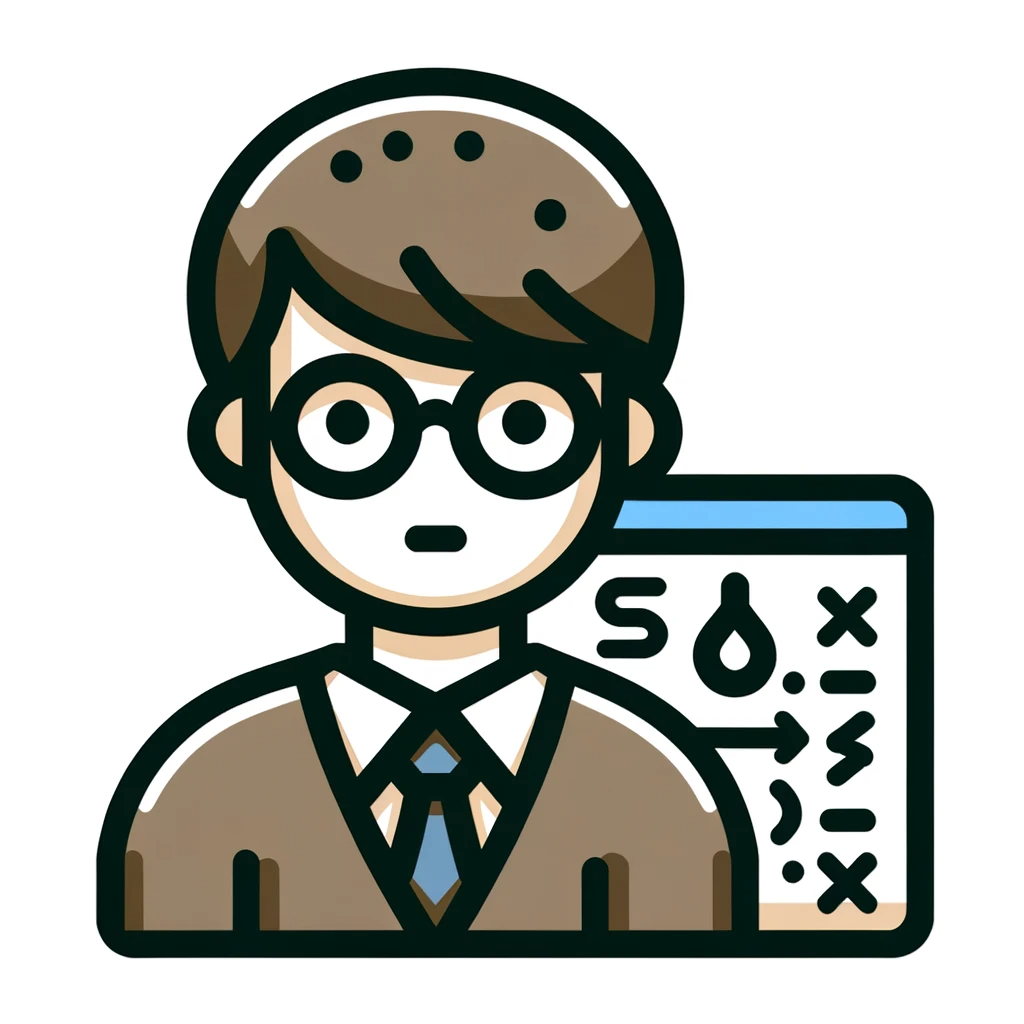
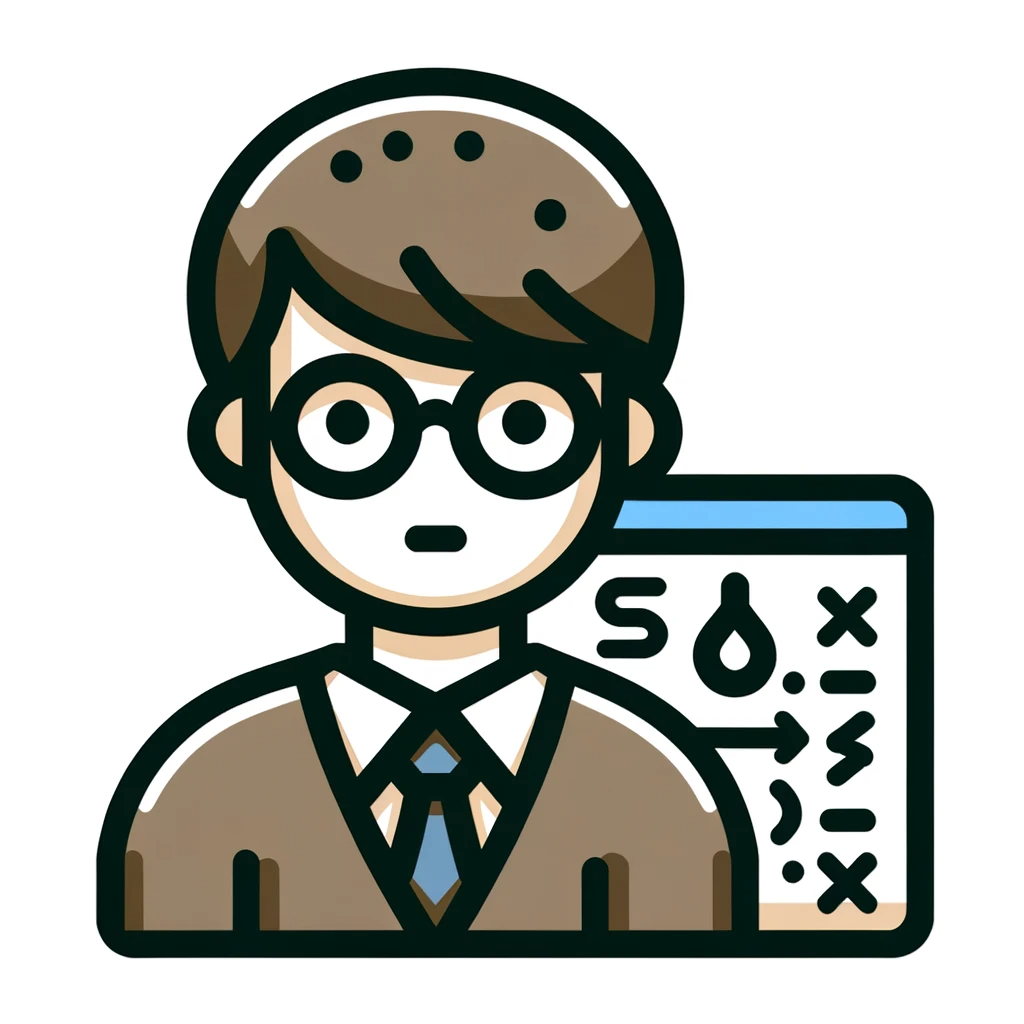
When using these methods, be careful to ensure that the element you’re replacing matches more precisely than the element you’re replacing, and check your browser’s support before using them.
Also, if you want to perform more advanced DOM manipulation, consider using libraries such as jQuery or React.
Comments