We will explain in detail how to delete event listeners in JavaScript.
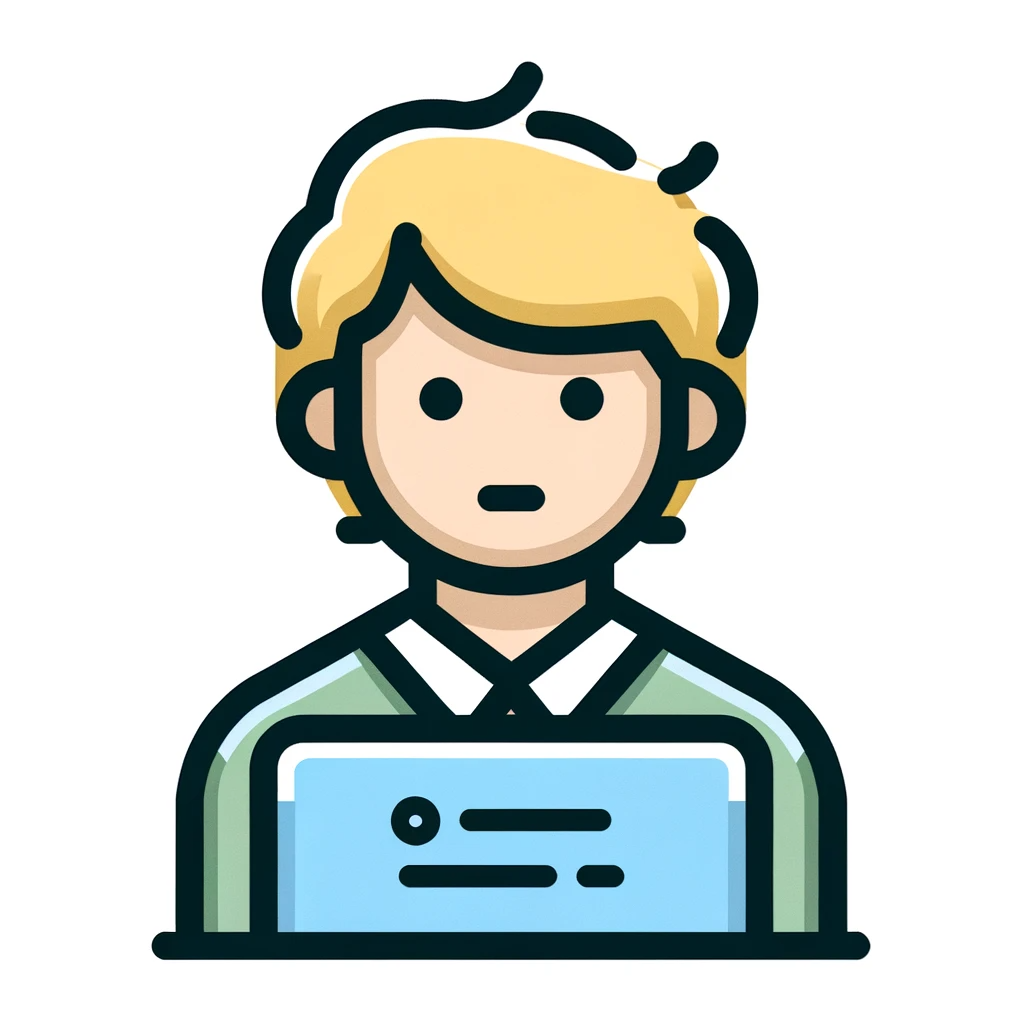
How do I delete an event listener?
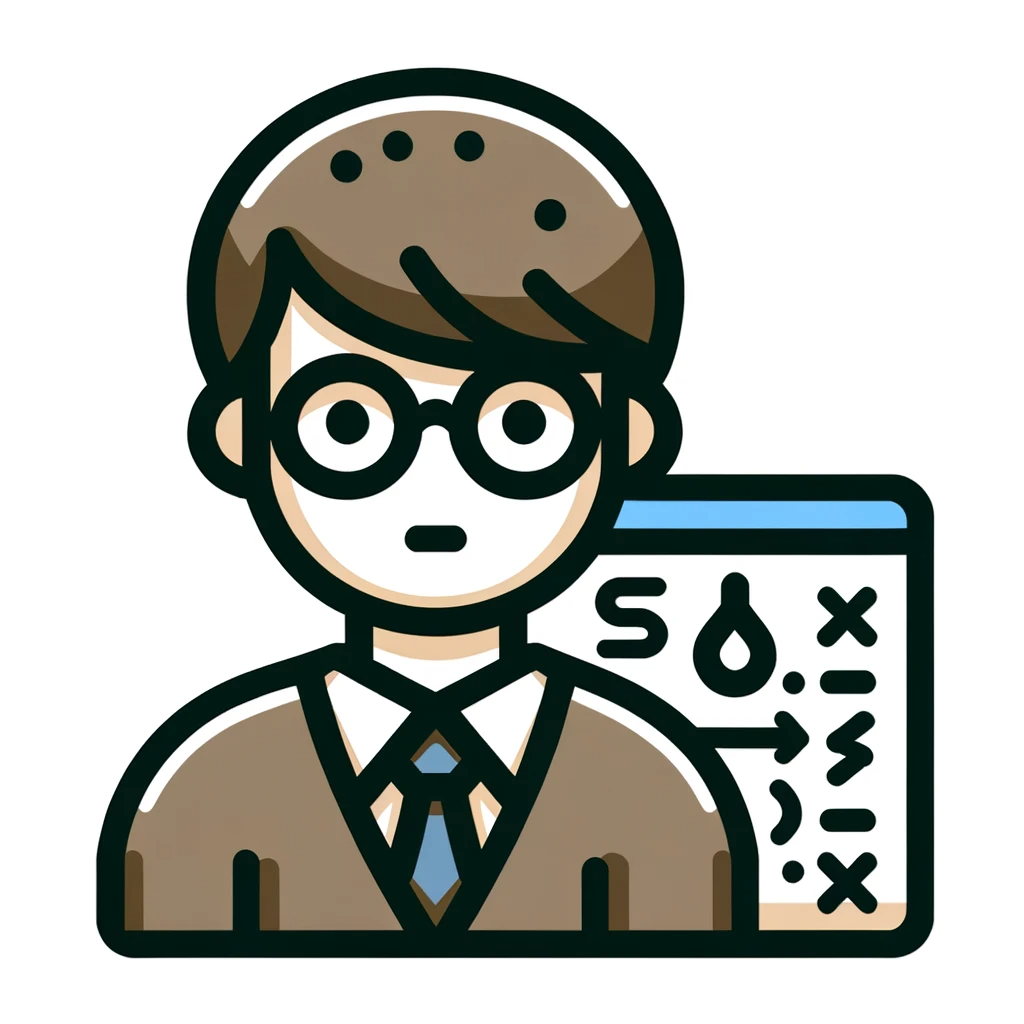
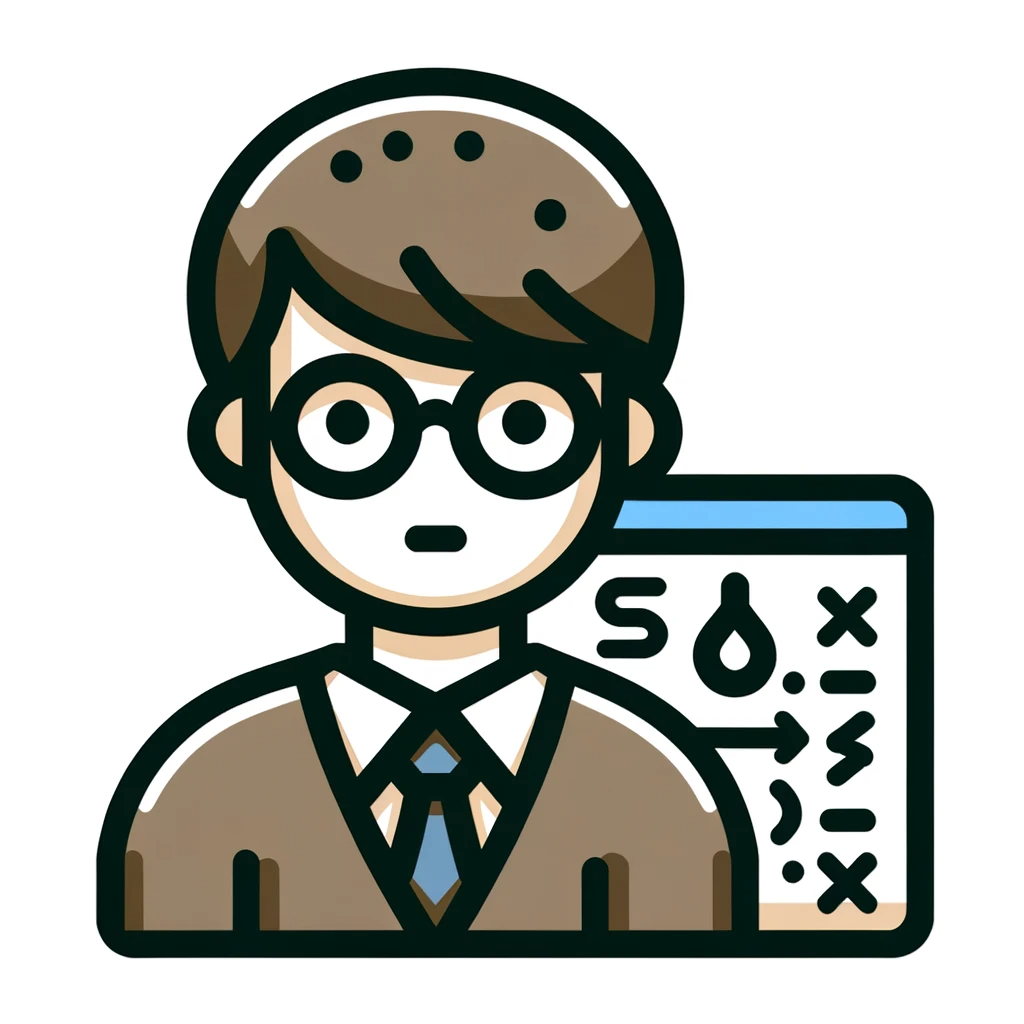
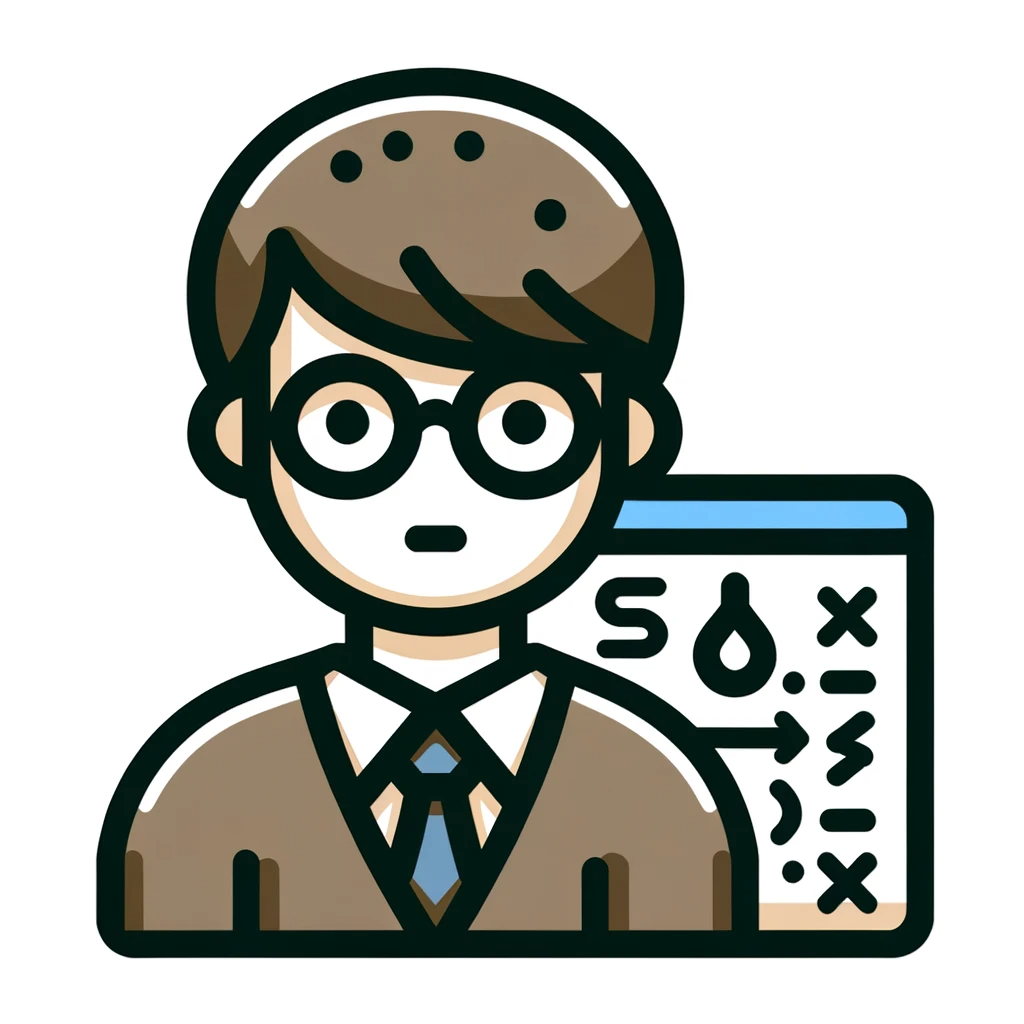
To remove an event listener, specify the event handler and use the removeEventListener() method.
How to remove event listeners
To remove an event listener, get the element to which the event listener to be removed has been added and call the removeEventListener() method. removeEventListener() method takes the following three arguments.
- Event type: Specify the same event type as the listener you want to delete. For example, if you want to remove the click event listener, specify ‘click’.
- Event handler function: Specify the same function as the listener you want to remove. If the event listener you want to remove is defined as an anonymous function, you must redefine the anonymous function.
- Optional: This is optional. Provides information to specify the listener to remove.
The following code retrieves the element with ID myElement and click
adds a listener for the event.
const myElement = document.getElementById("my-element");
function myFunction() {
console.log("Clicked.");
}
myElement.addEventListener("click", myFunction);
To remove an event listener, get the same element and removeEventListener()
call the method. The code below click
removes the event listener.
myElement.removeEventListener("click", myFunction);
The event listener has now been successfully removed.
When deleting event listeners, it is important to delete them in the reverse order that they were added. Also, be careful not to cause memory leaks by removing event listeners.
summary
We explained how to delete event listeners.
- To remove an event listener, use the removeEventListener() method.
- The removeEventListener() method requires an event type, an event handler function, and options.
- By specifying the event handler function, you can identify and delete event listeners.
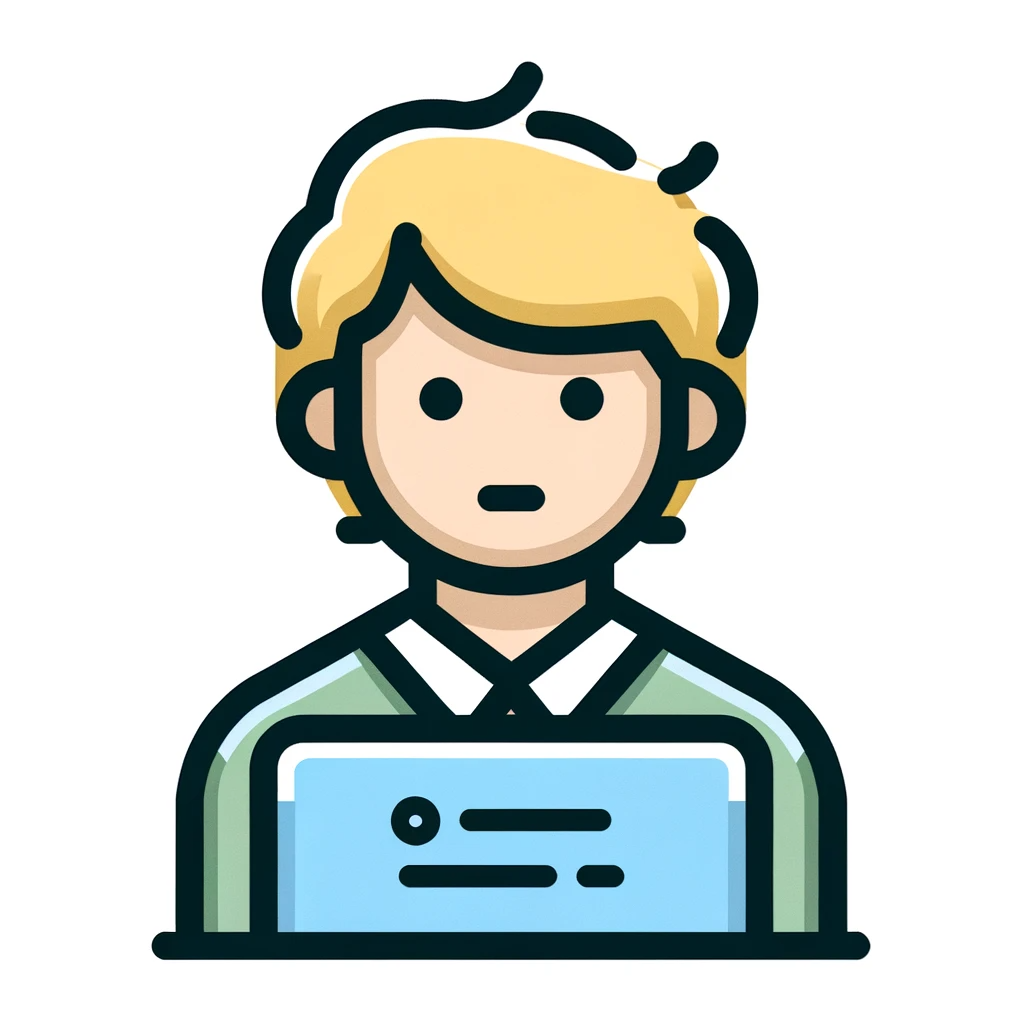
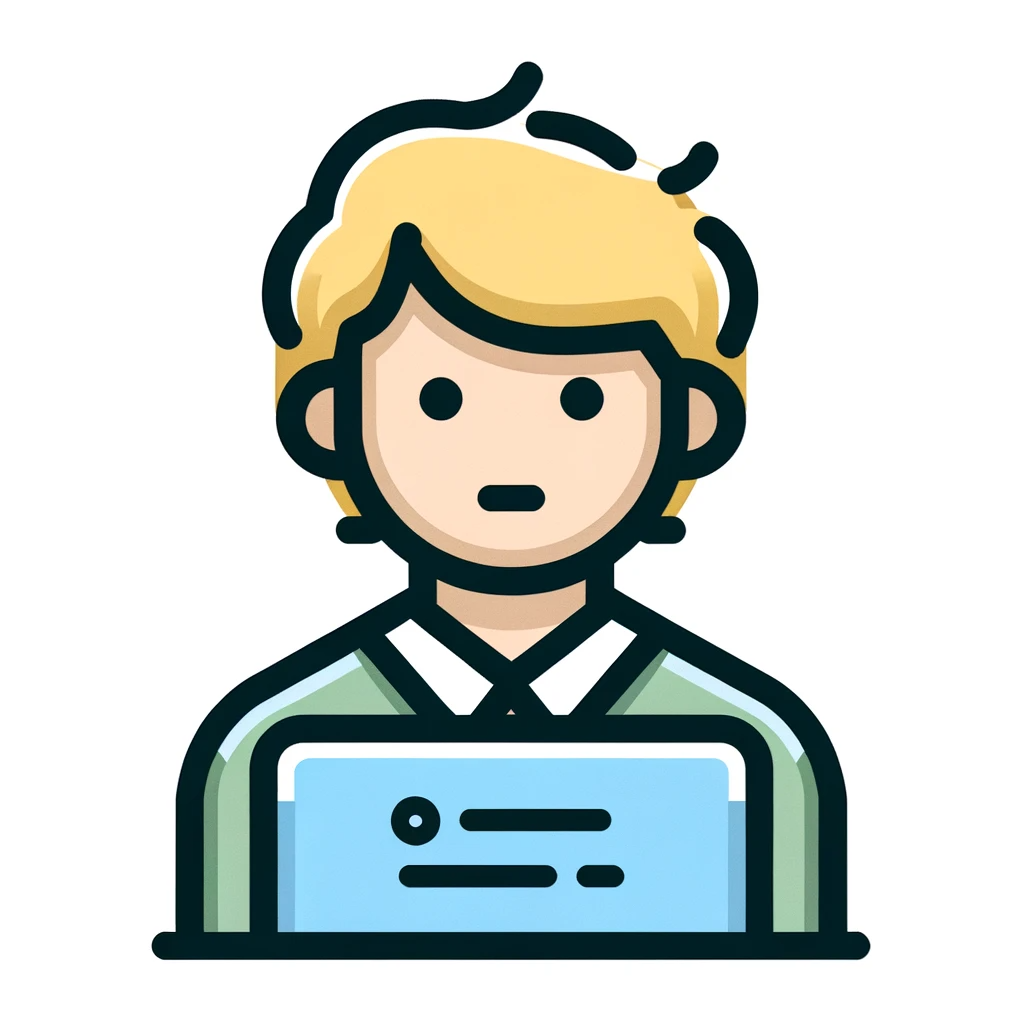
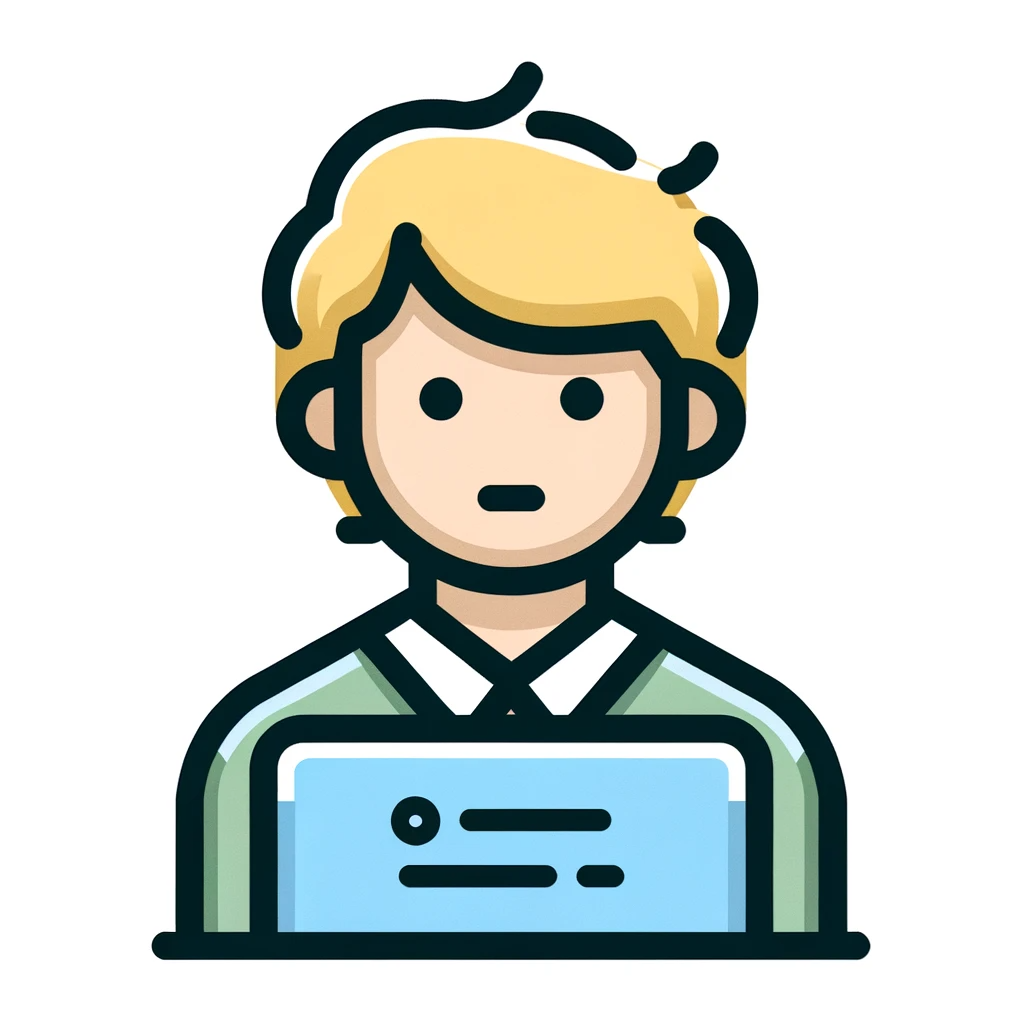
Now you know how to remove event listeners.
thank you!
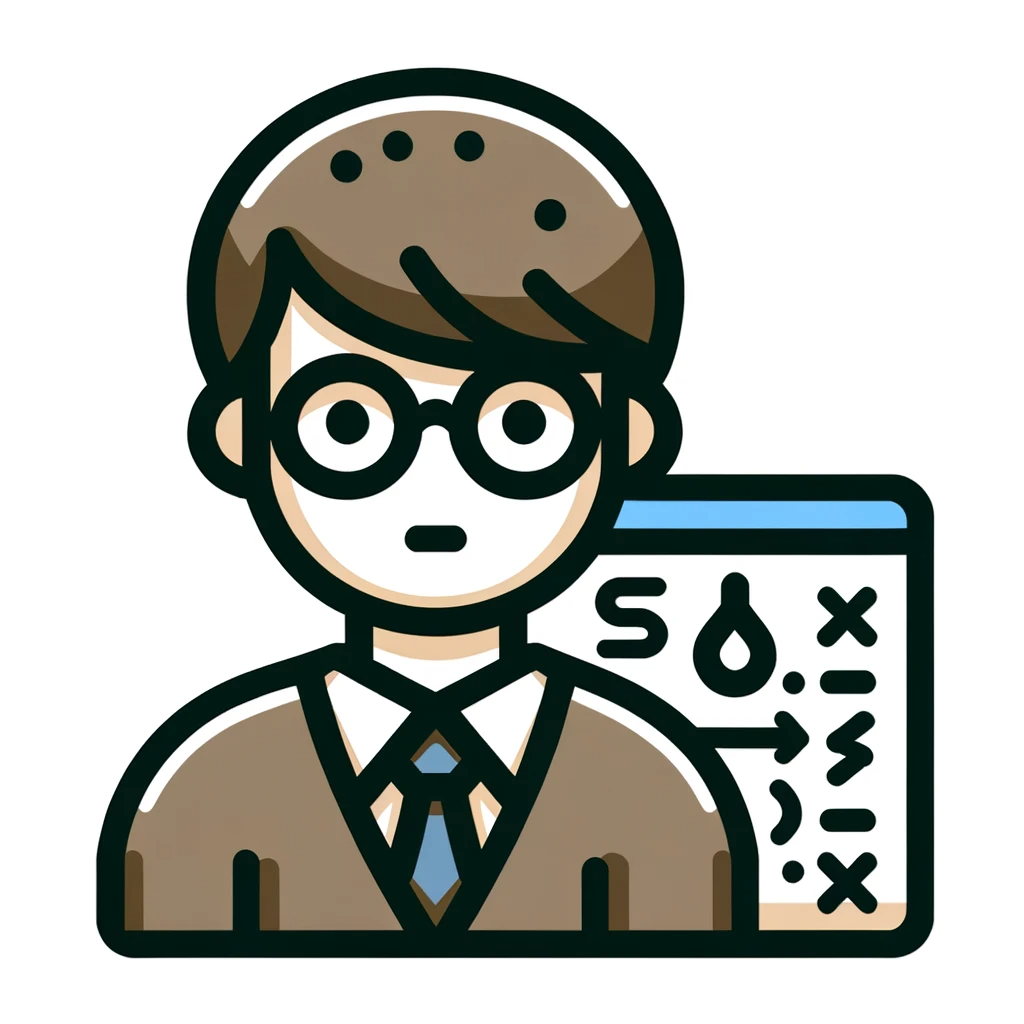
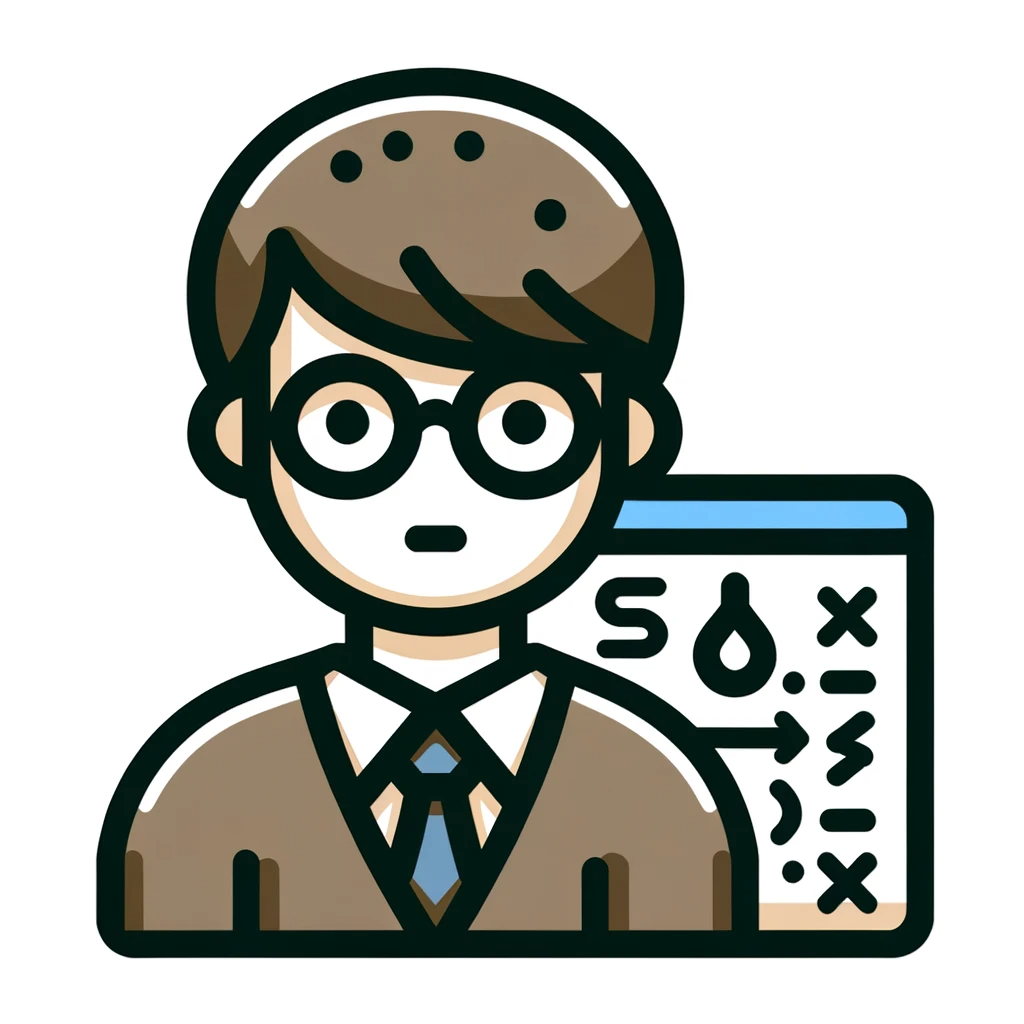
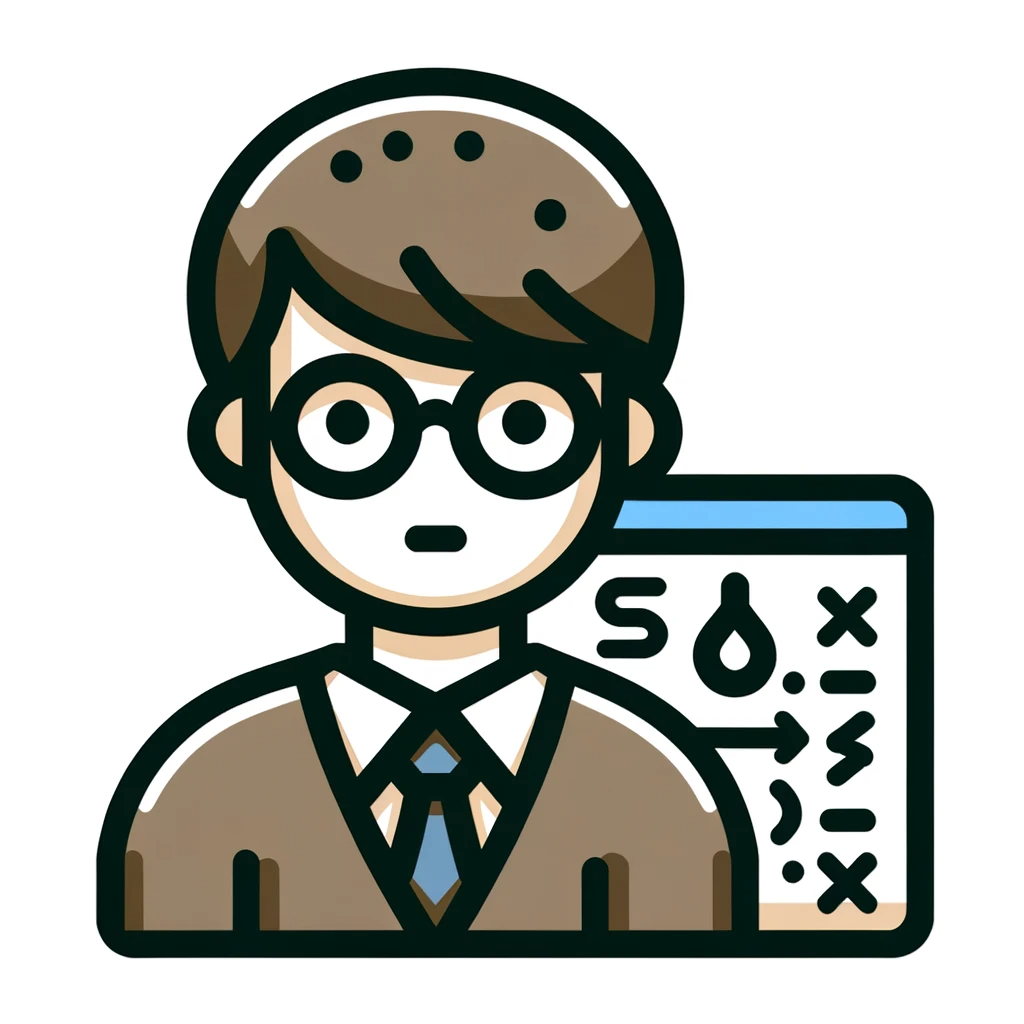
Adding and deleting event listeners is one of the most important functions when creating web pages with JavaScript.
By correctly adding and removing event listeners, you can efficiently manage the behavior of your web pages.
However, if you leave unnecessary listeners behind, memory leaks will occur, so be sure to periodically delete unnecessary listeners.
Also, if you delete the listener in the wrong order or the target element, it may cause unintended behavior, so please use caution.
Comments