This article explains how to use the removeChild method to delete an existing HTML element (child node) using JavaScript .
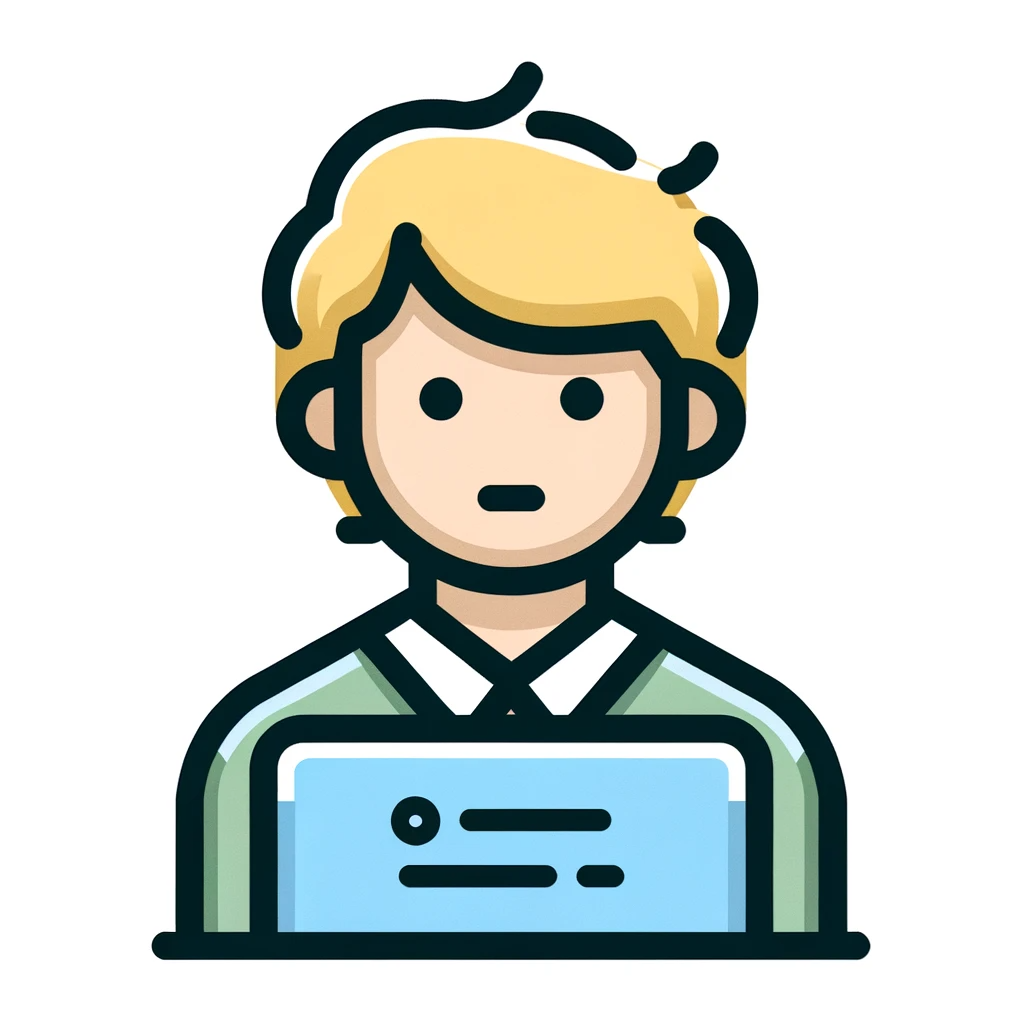
Is there a way to delete HTML elements?
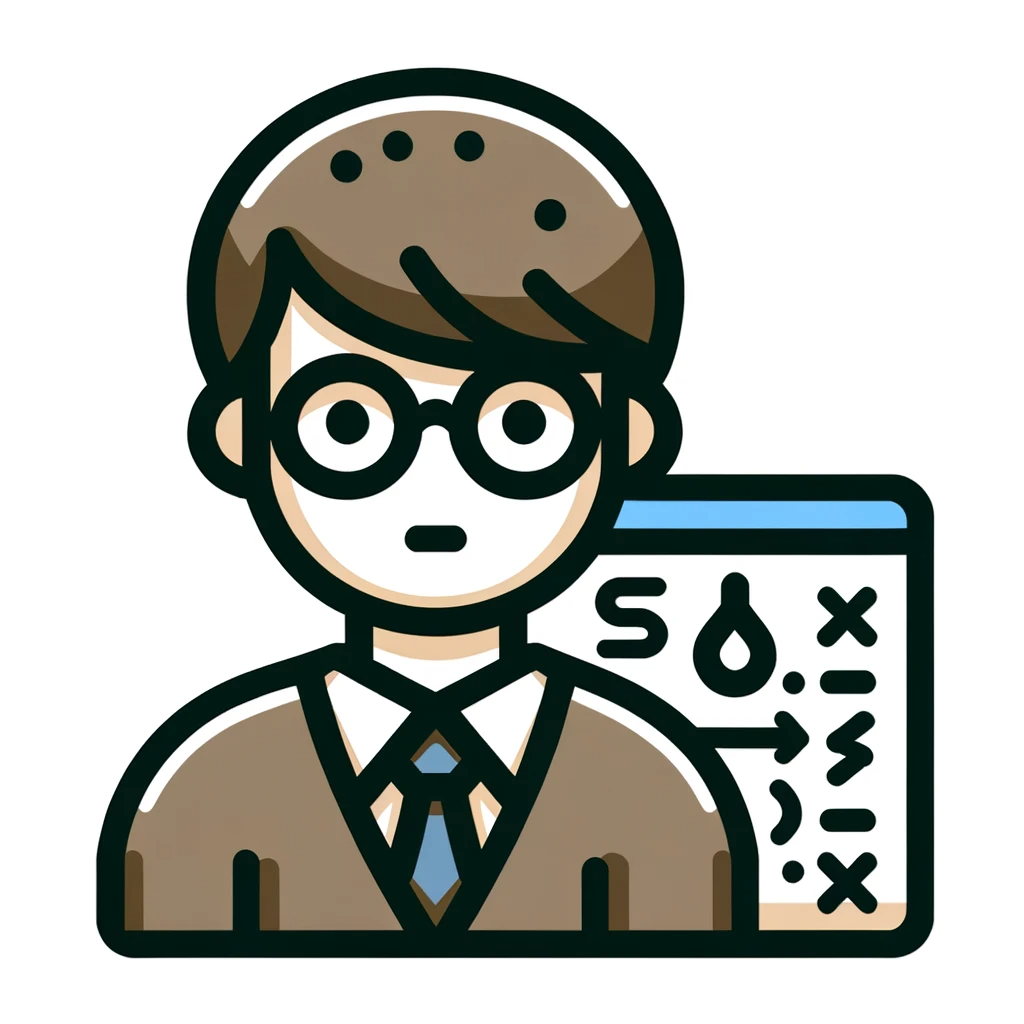
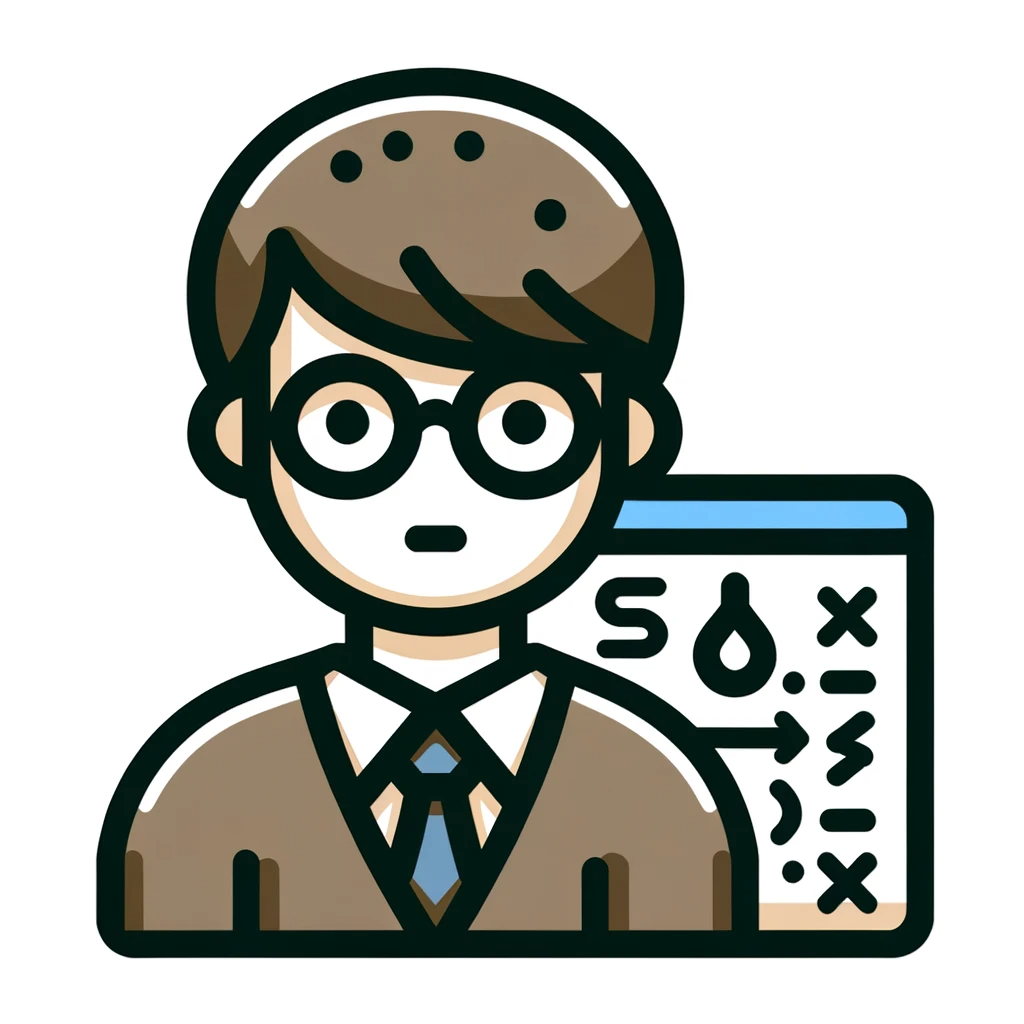
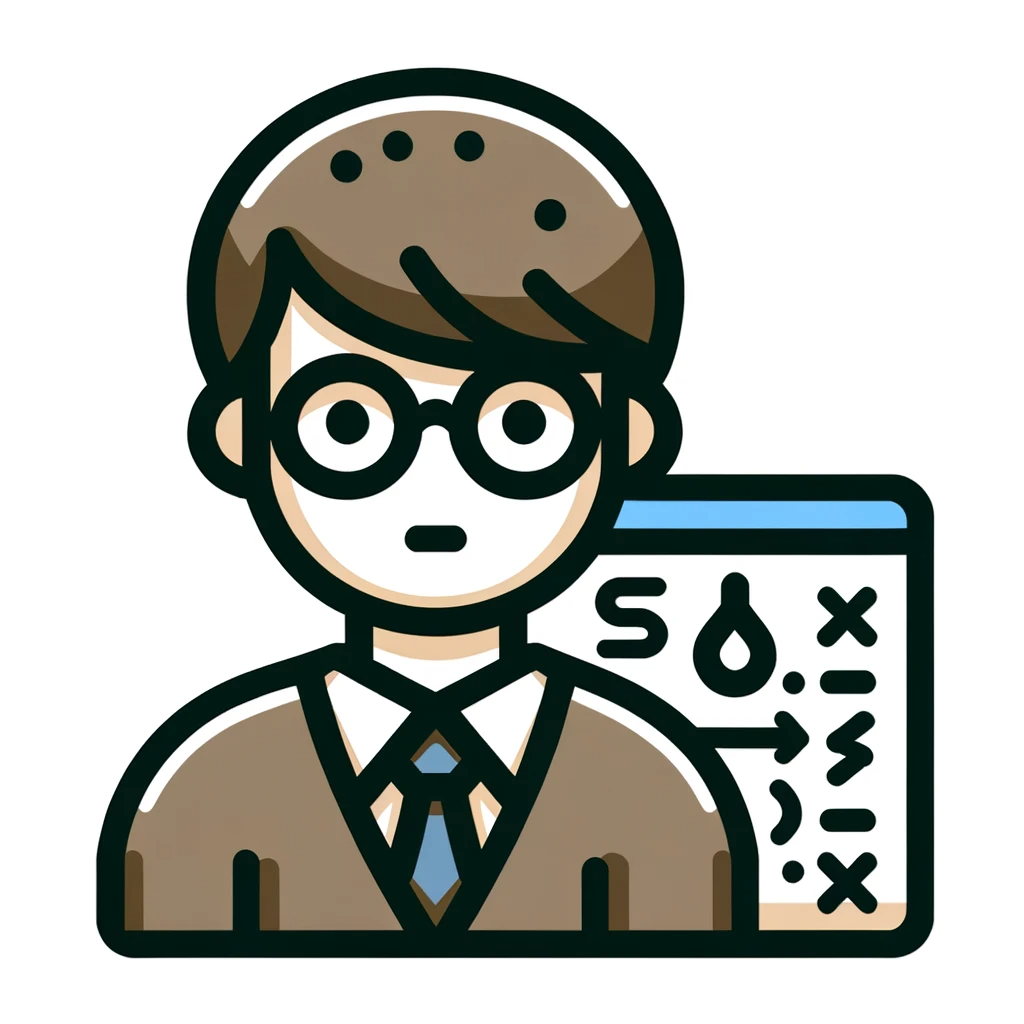
You can remove an existing element using the
removeChild method .
What is the removeChild method?
The removeChild method is a method for removing an HTML element (child element) .
This method can remove the specified child element.
Specifically, use the format parent element.removeChild(child element) . Here, parent element specifies the parent element that contains the child element you want to delete, and child element specifies the element you want to delete.
Parent element . removeChild ( child element );
Child element: Child node to delete
How to use removeChild method
Below is a sample code to remove an existing HTML element using removeChild method .
// Retrieving HTML Elements
const parent = document.getElementById('parent');
const child = document.getElementById('child');
// Use the removeChild method to remove a child element
parent.removeChild(child);
This code uses the getElementById method to get the parent element and child element , and uses the removeChild method to remove the child element in the format parentelement.removeChild(childelement) .
Deleted elements are completely erased from memory and cannot be reused later. If you need to reuse it, you should save it to another variable before deleting it.
Delete multiple child elements one by one in a loop
If there are multiple child elements, in order to remove them all, you must retrieve the child elements and use a loop to remove them one by one .
Below is a sample code of how to do that.
<div id="parent">
<div id="child1">child element1</div>
<div id="child2">child element2</div>
<div id="child3">child element3</div>
</div>
// Retrieving HTML Elements
const parent = document.getElementById('parent');
// Get all child elements
const children = parent.children;
// Delete child elements one by one
while (children.length > 0) {
parent.removeChild(children[0]);
}
This code uses getElementById method to get the parent element and parent.children to get the list of child elements. Then, we use a while loop to delete all child elements. Inside the while loop, we are removing child elements one by one using the removeChild method in the form parent.removeChild(children[0]) until there are no more child elements to remove.
Note that if you want to delete multiple child elements like this, you must use a loop to delete them one by one.
Remove all child elements using lastChild
I will also introduce a method using lastChild.
Below is a sample code that uses lastChild to remove all child elements .
// Retrieving HTML Elements
const parent = document.getElementById('parent');
// Retrieve and delete the last child element
while (parent.lastChild) {
parent.removeChild(parent.lastChild);
}
This code uses the getElementById method to get the parent element and a while loop to remove the last child element. The conditional expression in the while loop is such that the loop continues if parent.lastChild is not null or undefined.
parent.lastChild becomes null or undefined when there are no more child elements. Inside the loop, we remove the last child elements one by one using the format parent.removeChild(parent.lastChild).
The advantage of using this method is that it simplifies your code because you don’t have to remove child elements one by one. However, note that the loop continues until all child elements are removed, which can impact performance if there are a large number of child elements.
summary
We explained how to use the removeChild method to delete an existing HTML element (child node).
- You can use the removeChild method to remove an existing HTML element.
- Use the format parent element.removeChild(child element).
- Before deleting an element, you need to retrieve the element you want to delete.
- If there are multiple child elements, you must use a loop to remove them one by one.
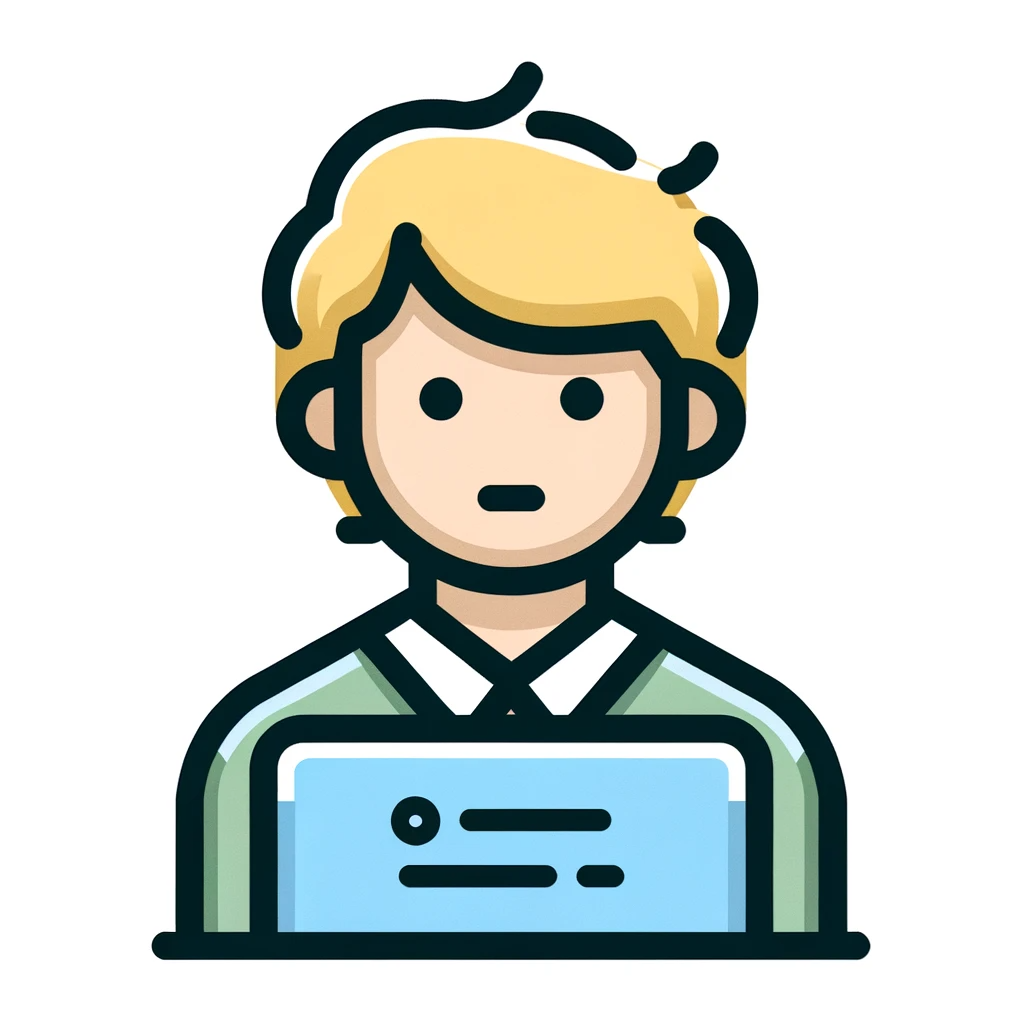
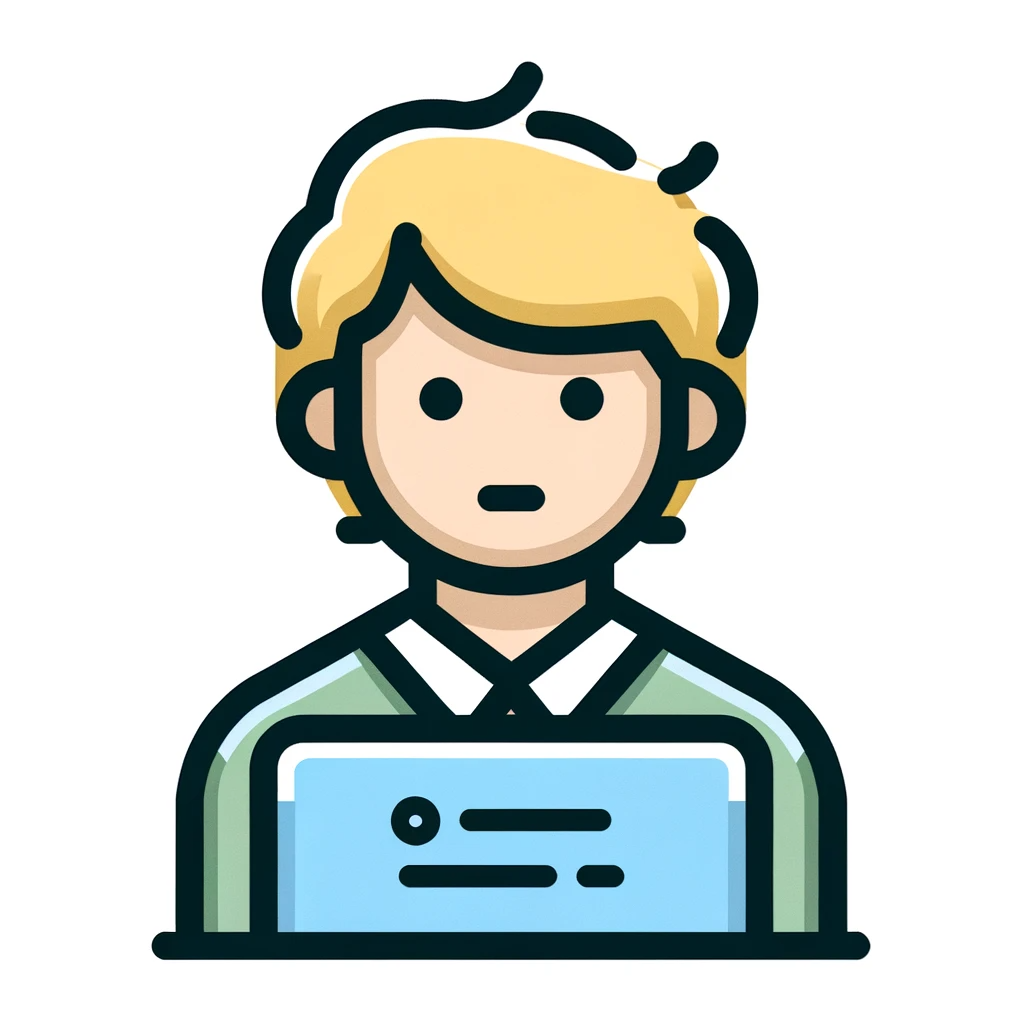
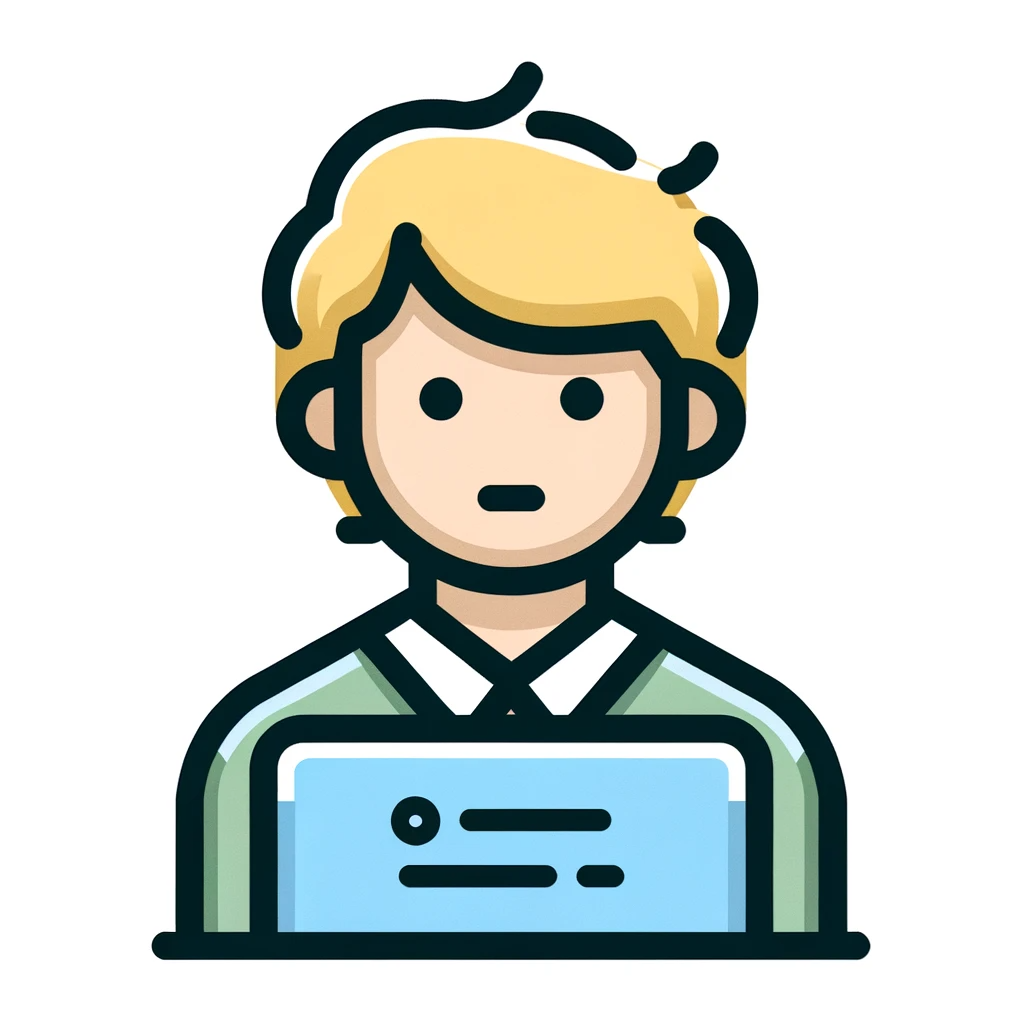
I see, you can easily remove HTML elements using the removeChild method.
Removing loop processing also seems useful.
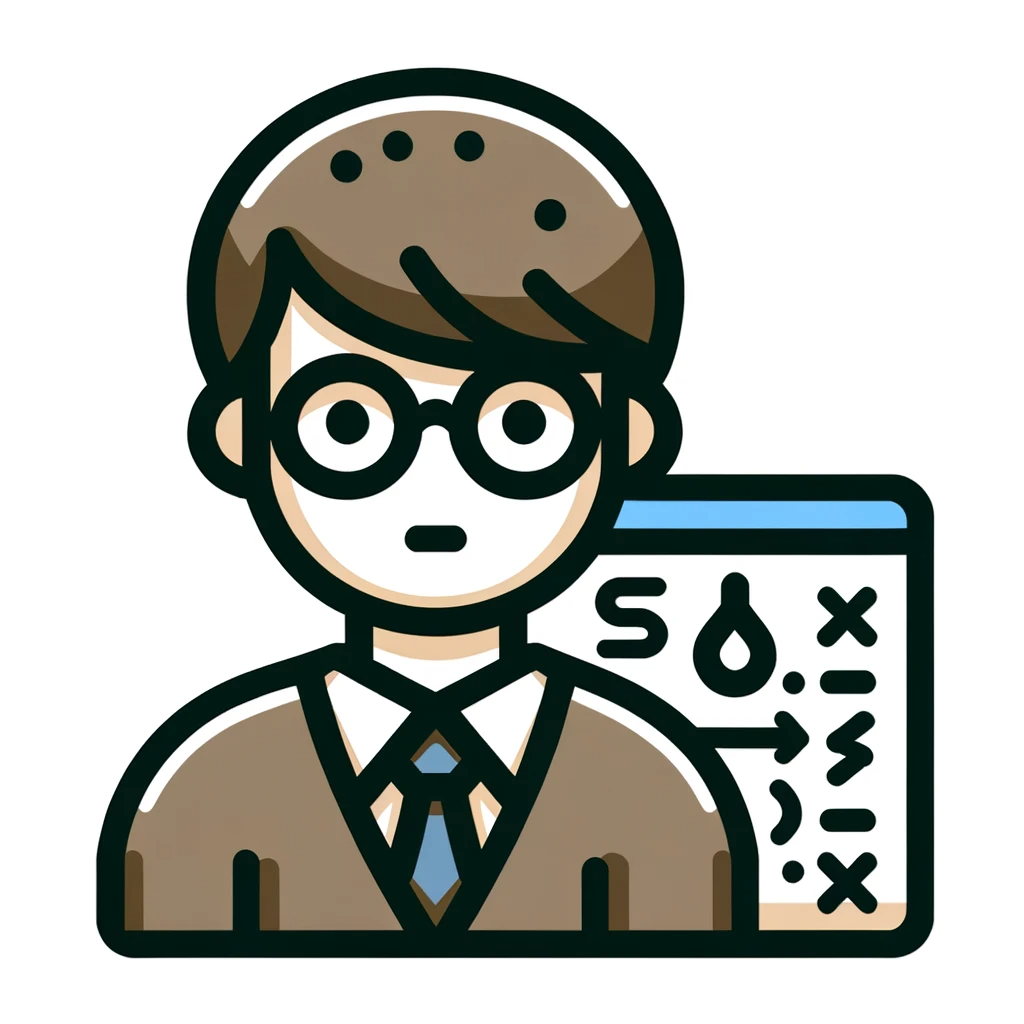
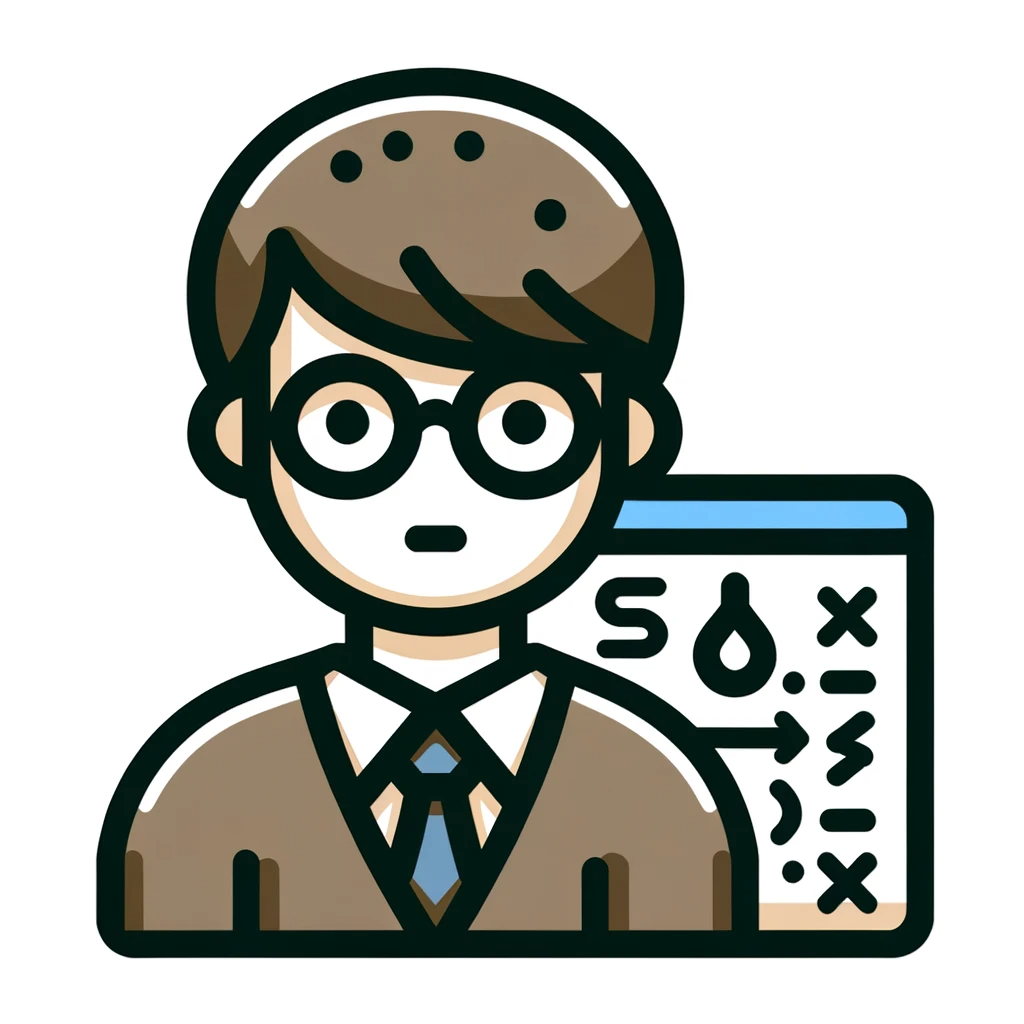
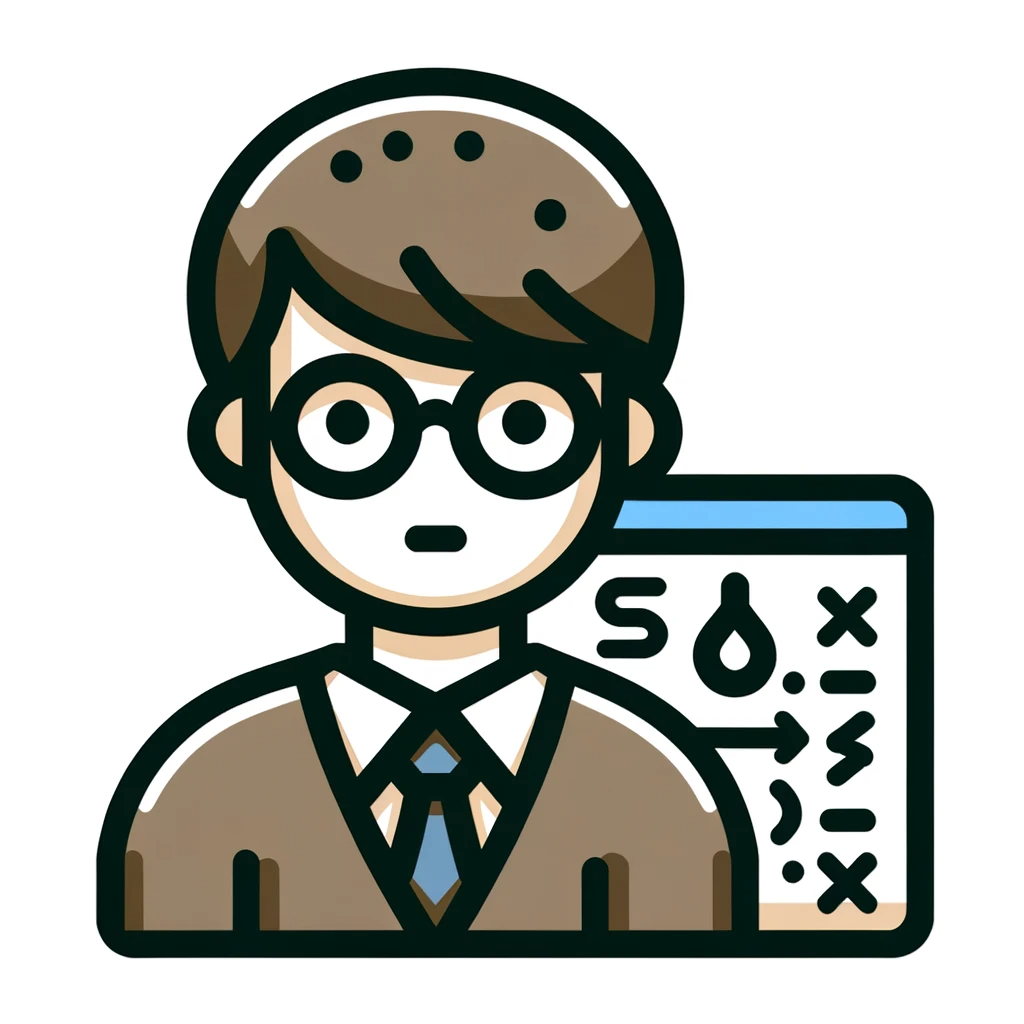
You can easily remove HTML elements using the removeChild method.
Before deleting, don’t forget to retrieve the element you want to delete.
Additionally,
if you want to reuse deleted elements later, we recommend that you save the deleted elements in a variable.
Comments