We will explain how to use the reduce method to process JavaScript array elements and return the results.
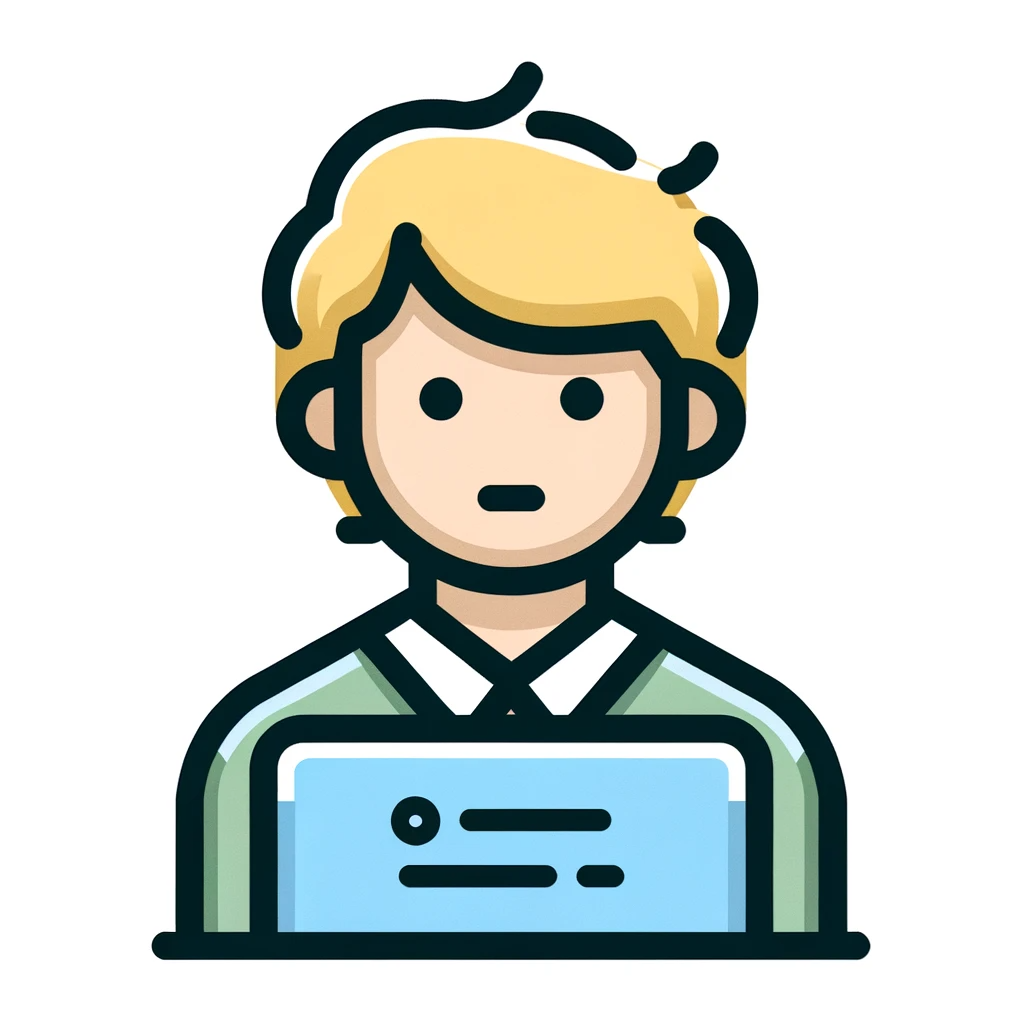
How can I process the elements of an array and return the result?
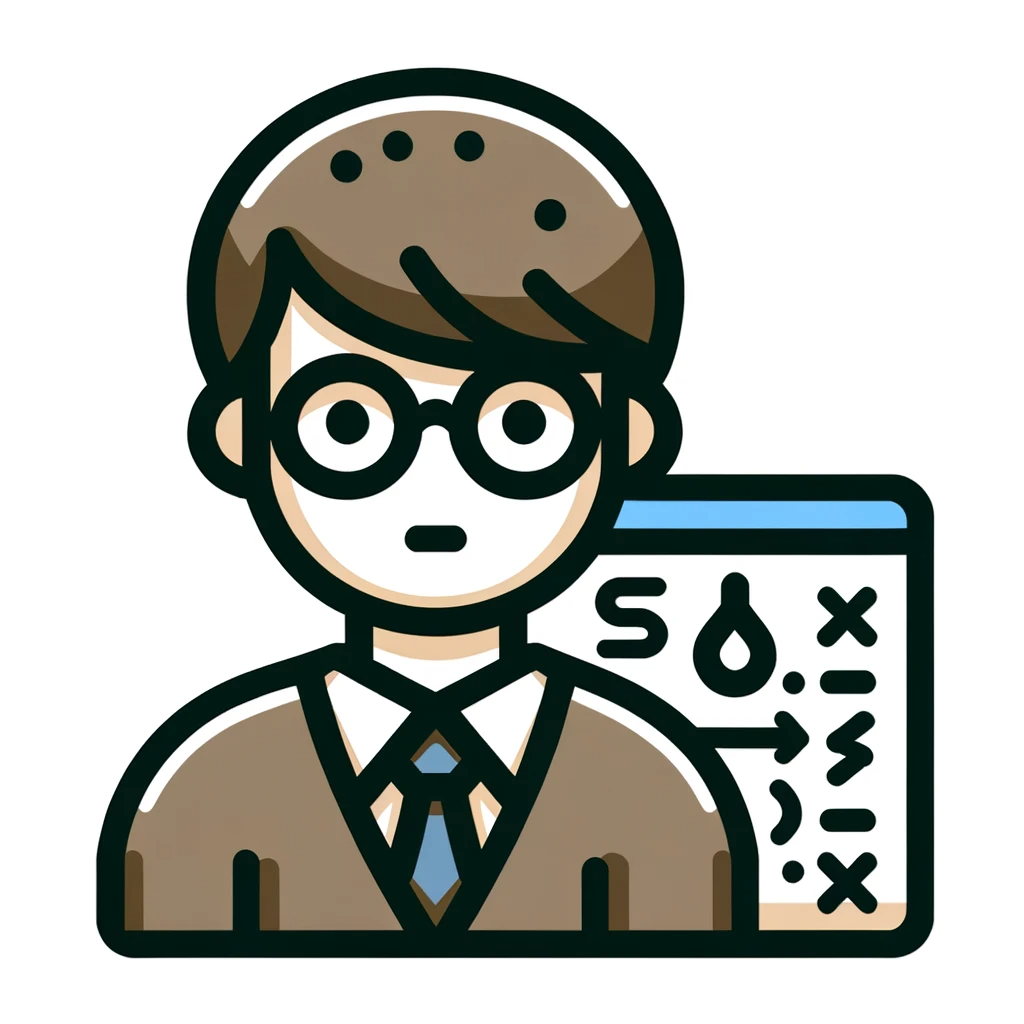
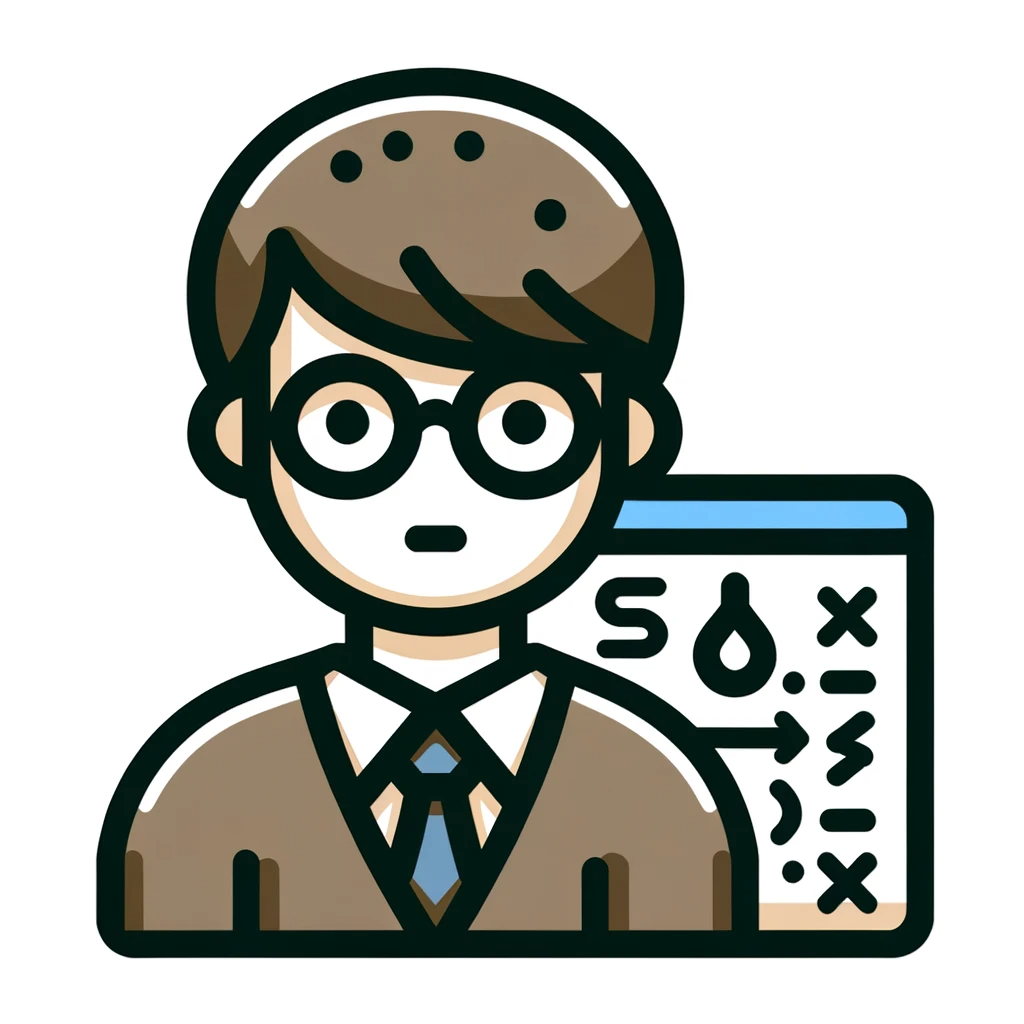
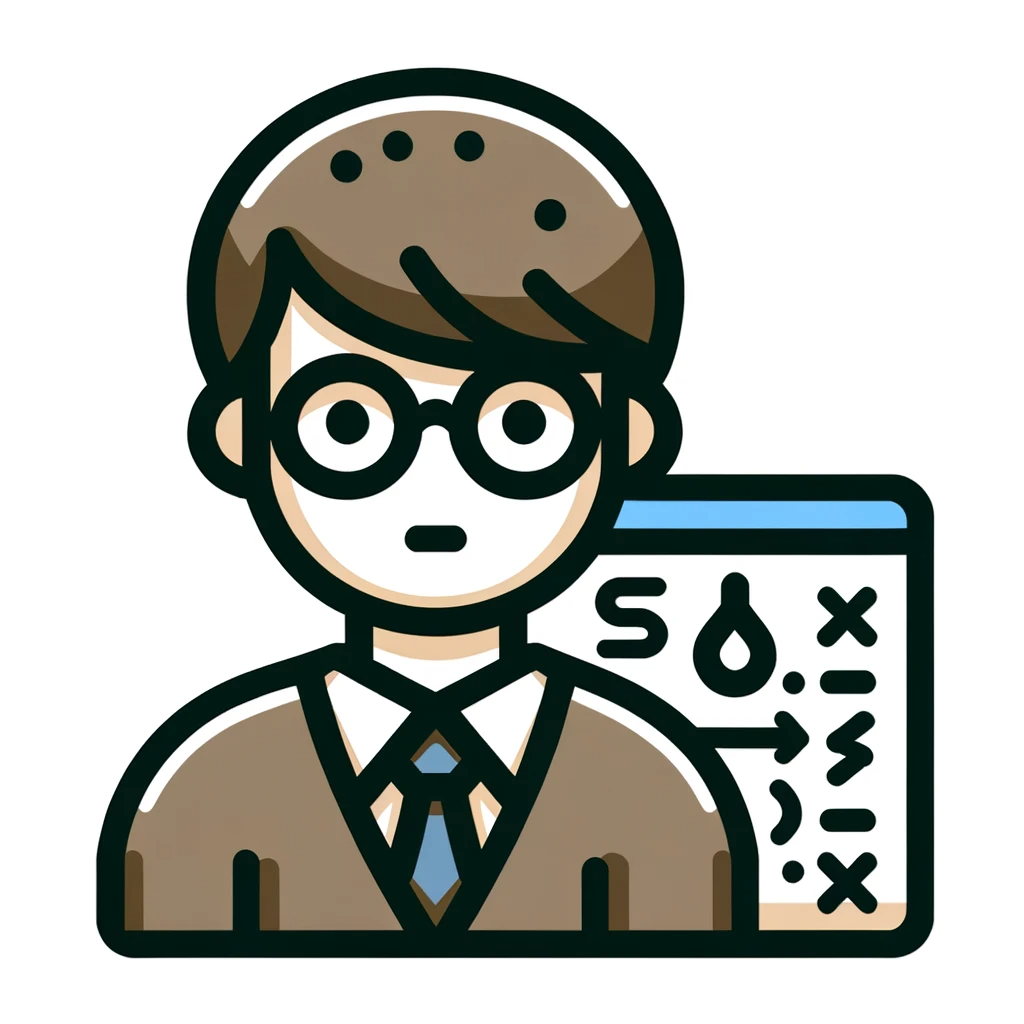
It is convenient to use the reduce method to process the elements of an array and return the results.
How to use reduce method
The reduce method processes each element of an array one by one and returns the result.
array.reduce(function(result value, element value, index value, original array){ …processing…}, initial value)
Executes the function in the first argument for each element and accumulates the results in the value in the second argument. Finally, it returns the accumulated results.
For example, if you want to add all the arrays [1, 2, 3, 4, 5], use the reduce method as shown below.
let array = [1, 2, 3, 4, 5];
let sum = array.reduce(function(a, b) {
return a + b;
}, 0);
console.log(sum); // 15
The function in the first argument adds the previous cumulative value and the current value. The value of the second argument is the first cumulative value.
The reduce method operates on the elements in the array from left to right, but by using the reduceRight method , it is also possible to operate from right to left . This makes it possible to perform processing with the contents of the array reversed, allowing for a richer range of processing.
Summary of this article
We explained how to use the reduce method to process array elements and return the results.
- The reduce method processes each element of an array one by one and returns the result.
- Executes the function in the first argument for each element and accumulates the results in the value in the second argument.
- Finally, it returns the accumulated results.
We introduced an example using the reduce method to process the elements of an array and return the results. By using the reduce method, we can easily process the elements of an array and return the results.
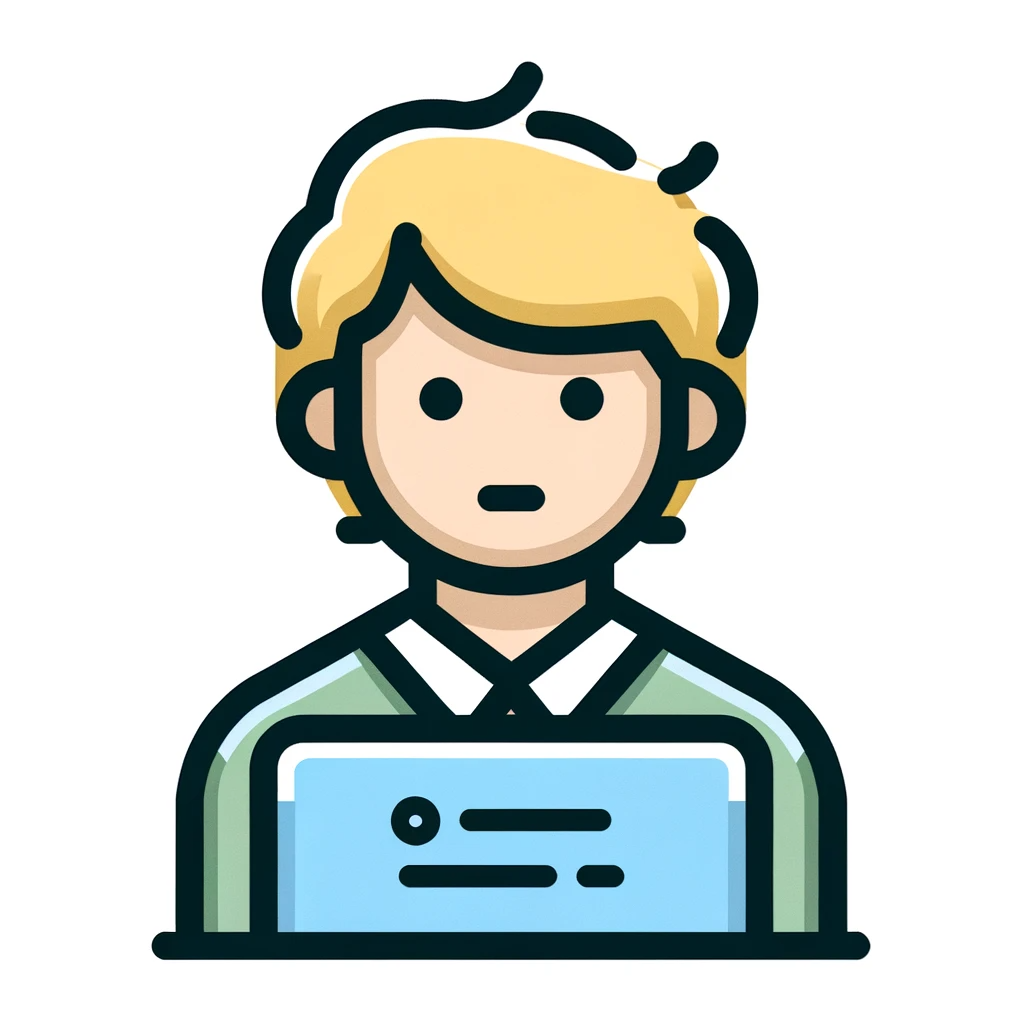
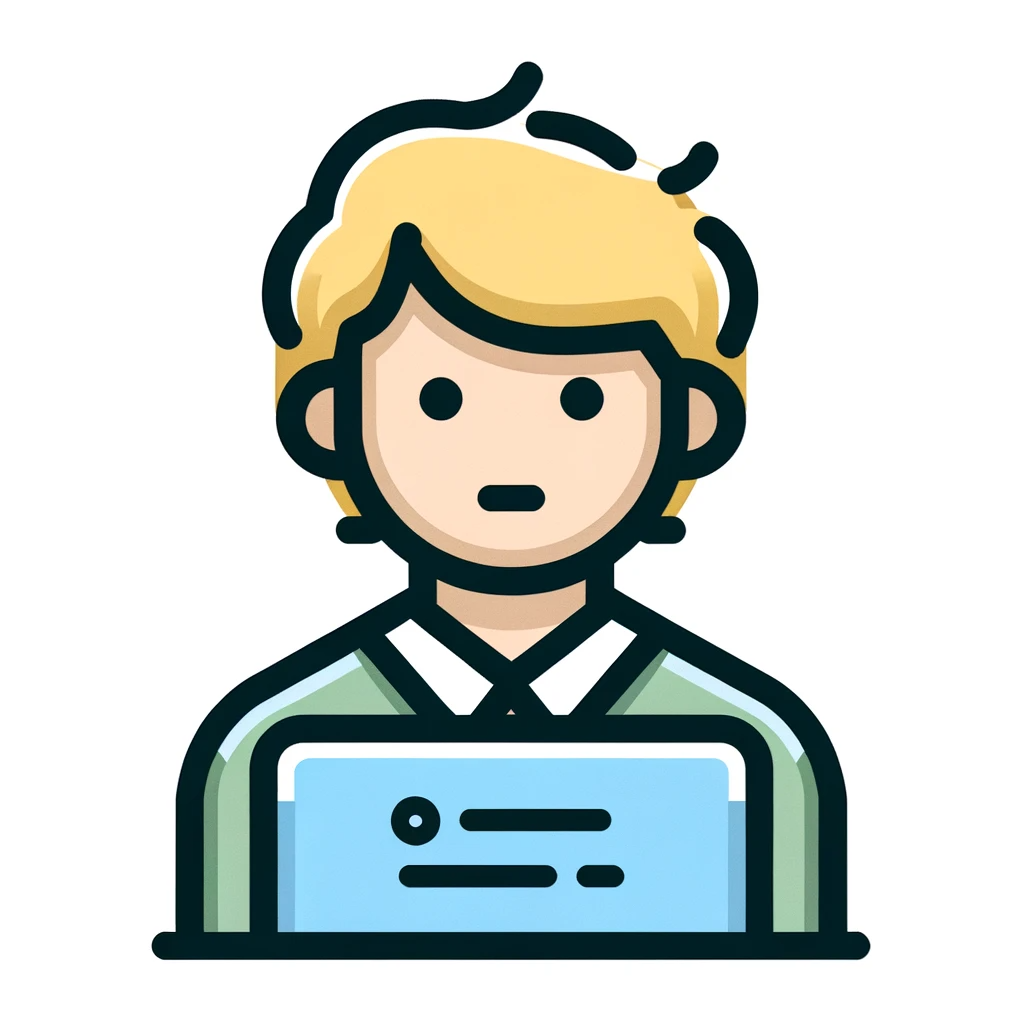
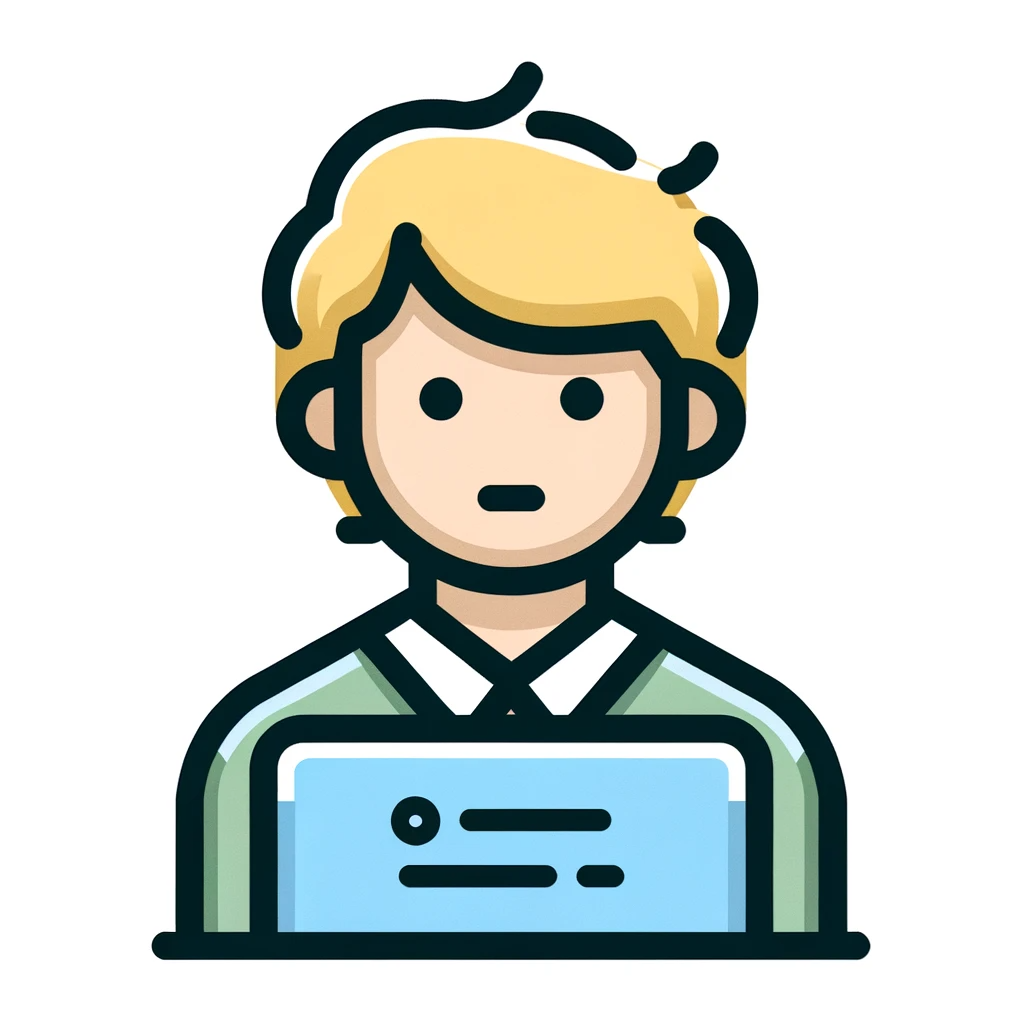
The reduce method seems useful when performing cumulative processing.
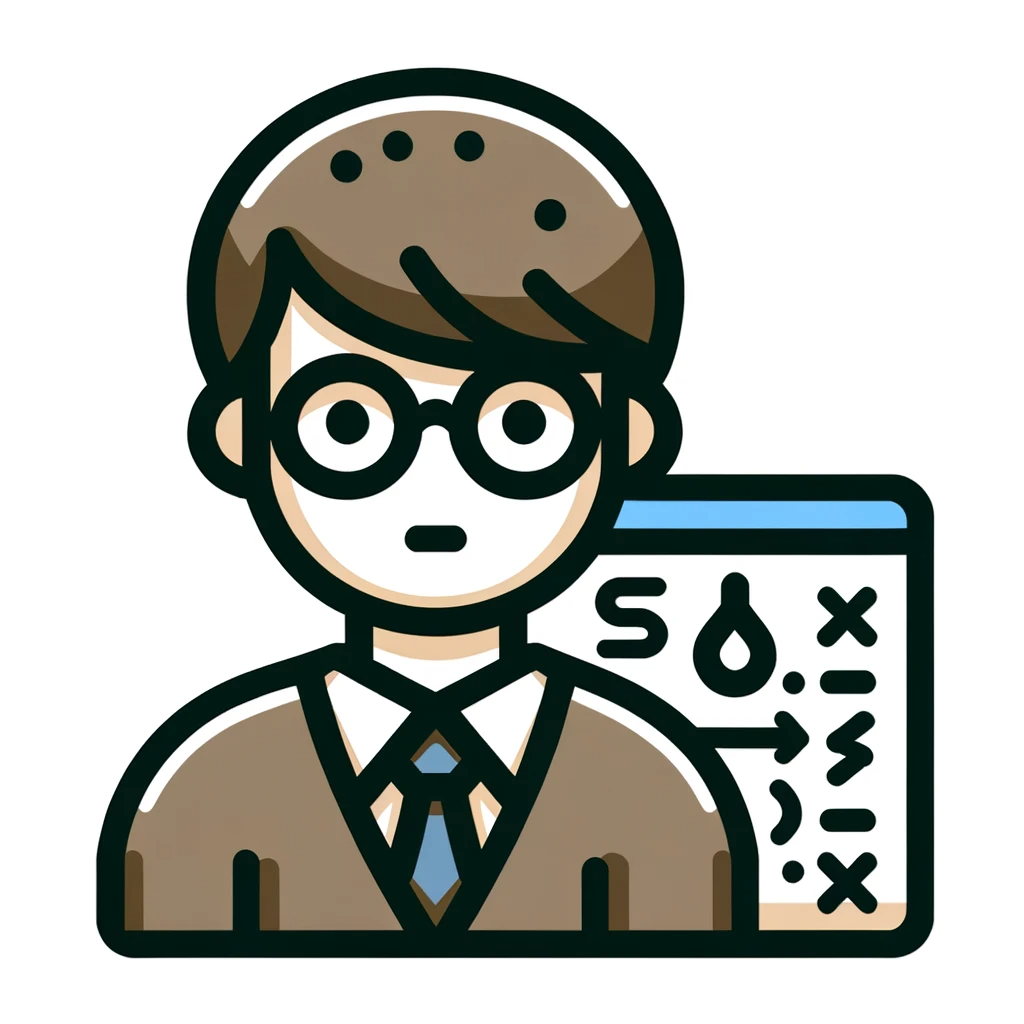
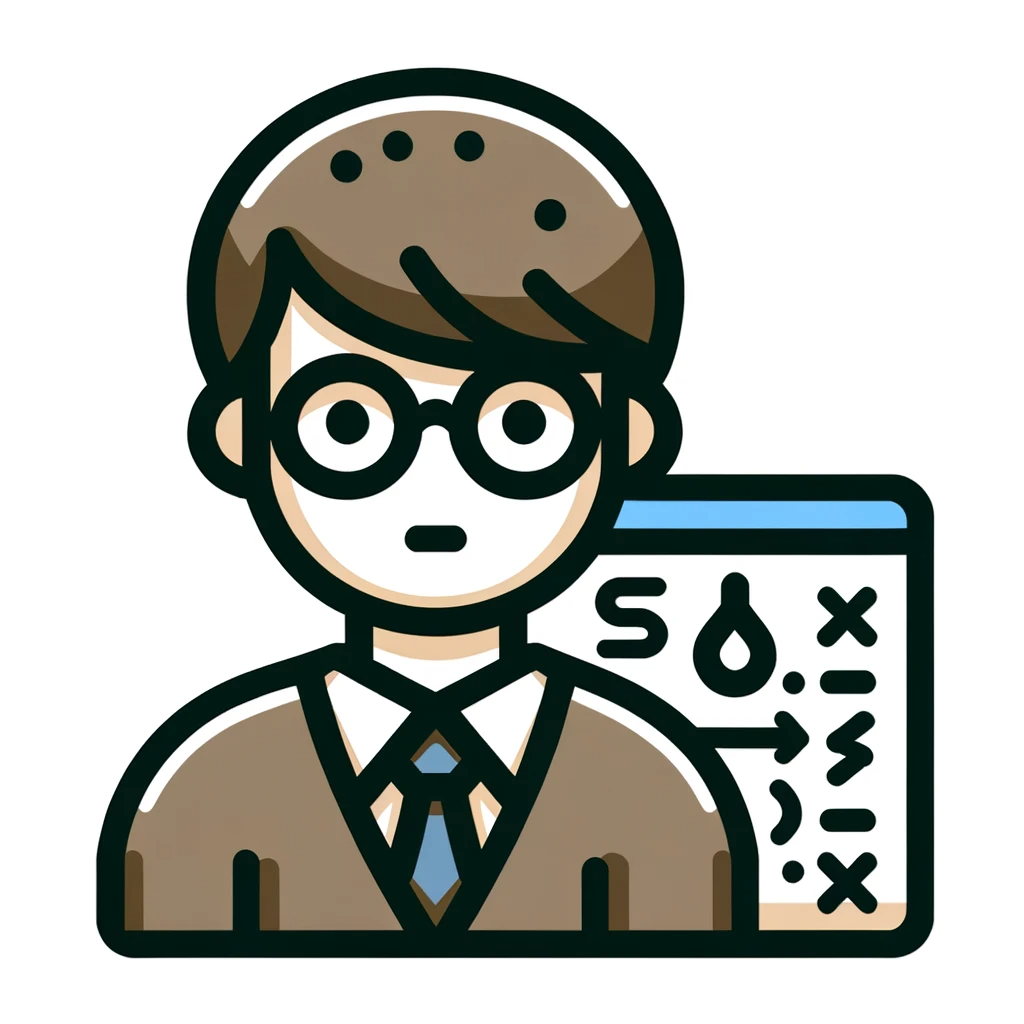
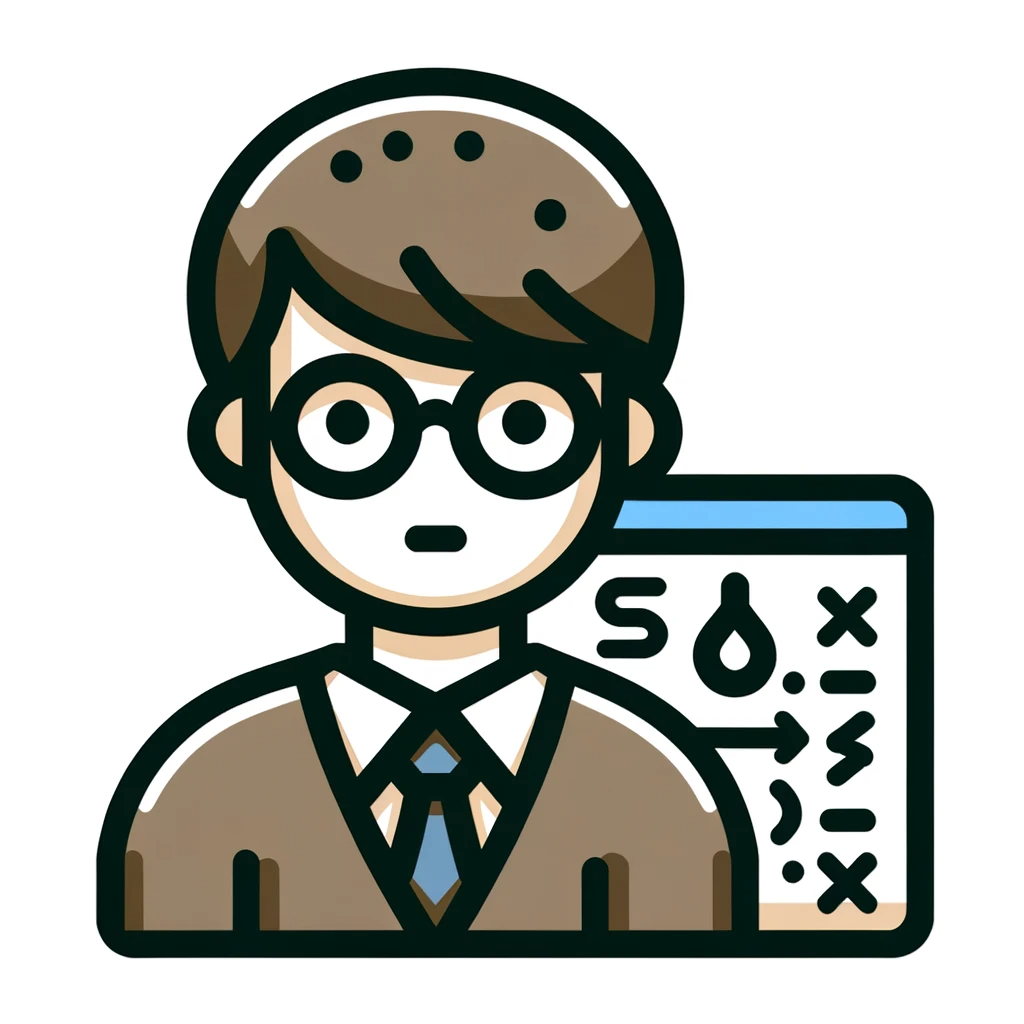
The reduce method is a general-purpose method that can be used not only for arrays but also for various types of objects, expanding the range of programming possibilities.
Comments