In JavaScript, we will explain how to generate random numbers using the methods of the Math object and the library that generates random numbers.
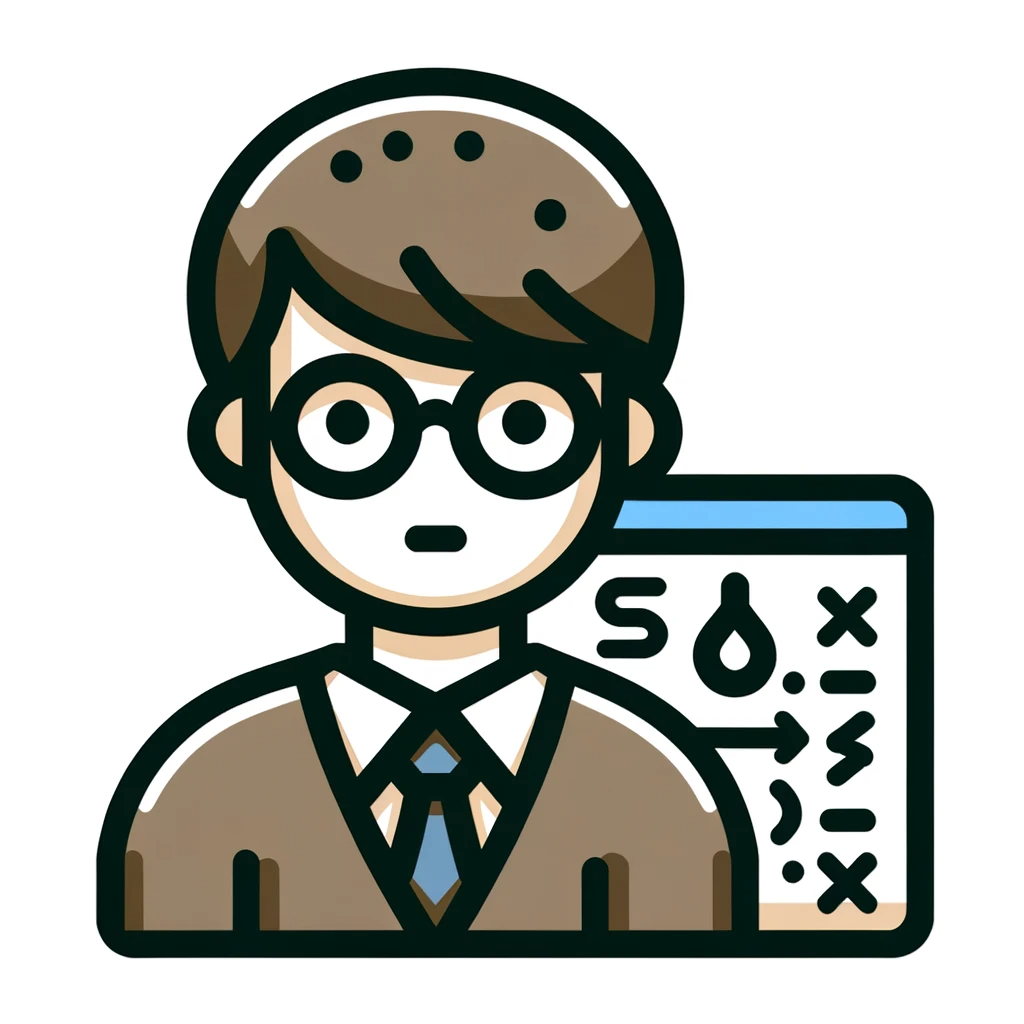
JavaScript has a method called Math.random() for generating random numbers.
“random” method to generate random numbers
To generate random numbers, use the Math.random method . This method randomly generates a decimal number between 0 and less than 1.
Math.random()
However, this value is generally used to generate random numbers within a certain range, so calculate it as follows.
(generated random number) * (range) + (minimum value of range)
// 乱数を生成し、1から10の範囲内の整数を取得する場合
var randomNum = Math.floor(Math.random() * 10) + 1;
console.log(randomNum);
In the above sample, the random number generated by Math.random() is converted to a range from 0 to 9, the decimal part is rounded down by Math.floor(), and 1 is added at the end to create an integer in the range from 1 to 10. I’m getting it.
Fix the random number generation pattern by specifying a seed value
By specifying a seed value, you can fix the random number generation pattern. JavaScript provides a standard method called Math.random(), but when you use this method, a different random number is generated each time.
To specify a seed value, you can use the method Math.seedrandom() .
Math.seedrandom()
// シード値を指定した乱数の生成
Math.seedrandom("seedvalue");
console.log(Math.random());
console.log(Math.random());
In the above program, the seed value “seedvalue” is specified using Math.seedrandom(“seedvalue”). This fixes the random number pattern generated by Math.random() and ensures that the same value is generated every time.
Specifying a seed value makes it easier to test and debug your program. It can also be used to recreate specific situations in games, applications, etc.
However, please note that compared to not specifying a seed value, the pattern of random number generation is fixed, which makes the numbers less likely to be random.
Introduction to libraries that generate random numbers
In JavaScript, when using Math.random() to generate random numbers, the generated random numbers have a uniform distribution. On the other hand, JavaScript has libraries and tools for generating random numbers that follow various probability distributions.
For example, there are libraries like the following.
- Chance.js:
- This is a library that can generate random numbers that follow various probability distributions. You can also generate random names, addresses, email addresses, etc.
- Distributions.js:
- This is a library that can generate random numbers that follow a probability distribution. Includes normal distribution, Poisson distribution, beta distribution, and more.
- random-js:
- A high-quality random number generator for JavaScript. Supports various random number distributions.
By using these libraries and tools, you can generate more accurate random numbers. However, each library or tool has different usage methods and the properties of the random numbers generated, so be sure to read the documentation before using them.
Summary of “How to generate random numbers”
The “method for generating random numbers” is summarized below.
- Random numbers can be generated using the method Math.random().
- The generated random number is a decimal number between 0 and less than 1.
- Generally, the generated random numbers are used to generate random numbers within a specific range.
- To change the range of random numbers, use (generated random number) * (range) + (minimum value of range).
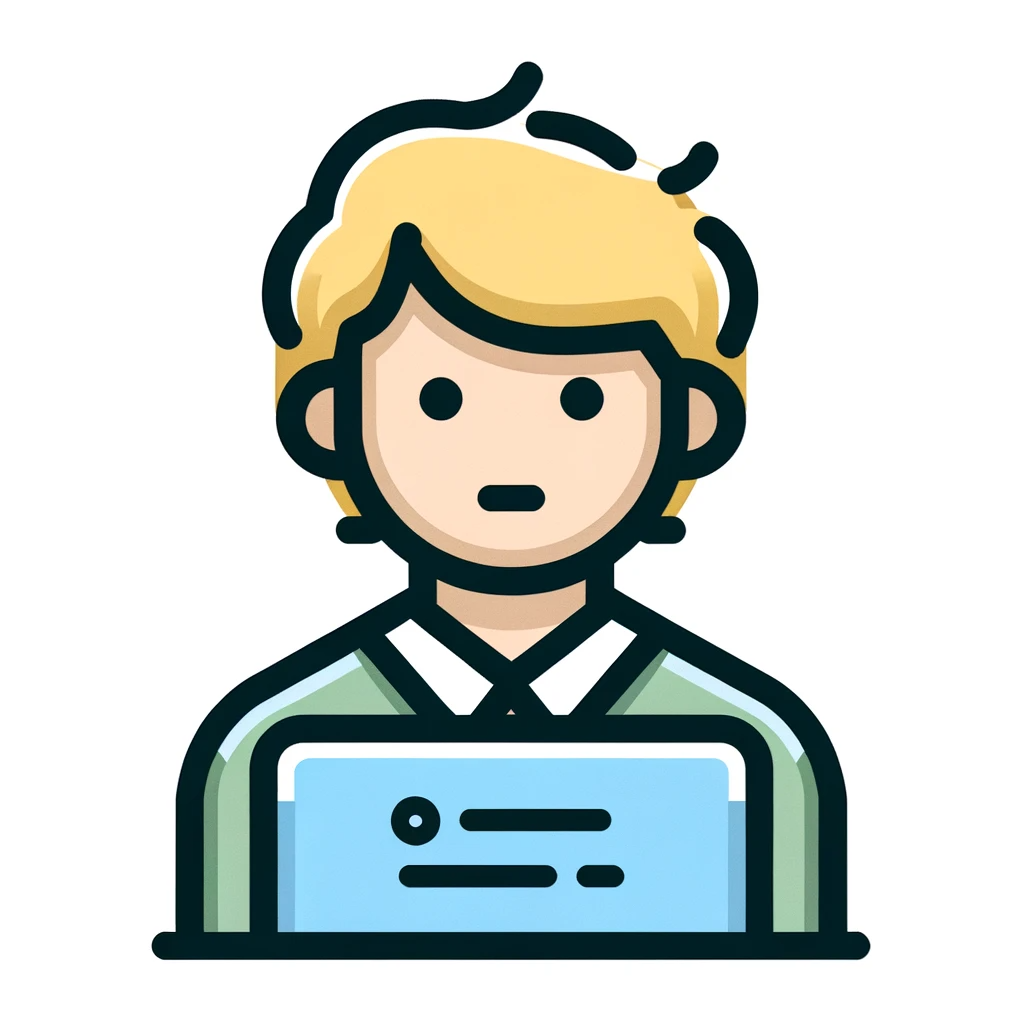
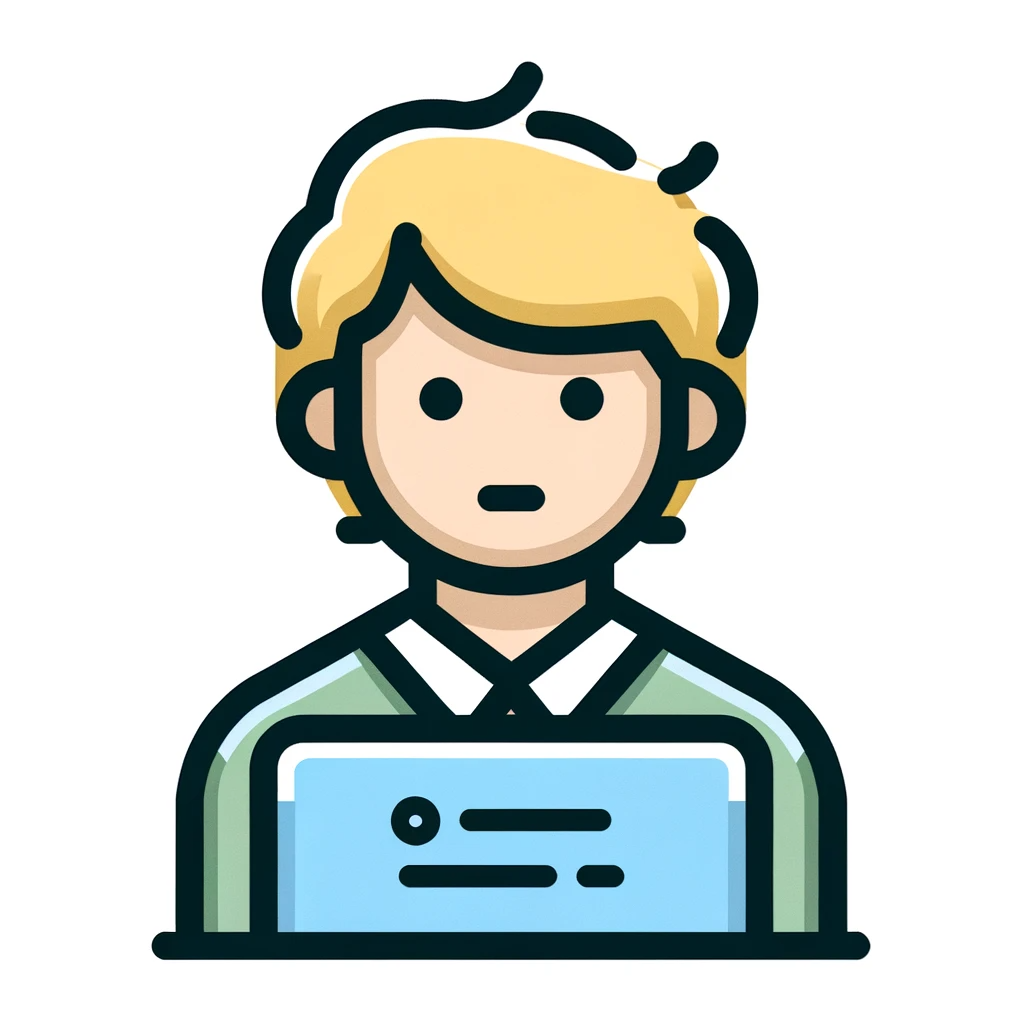
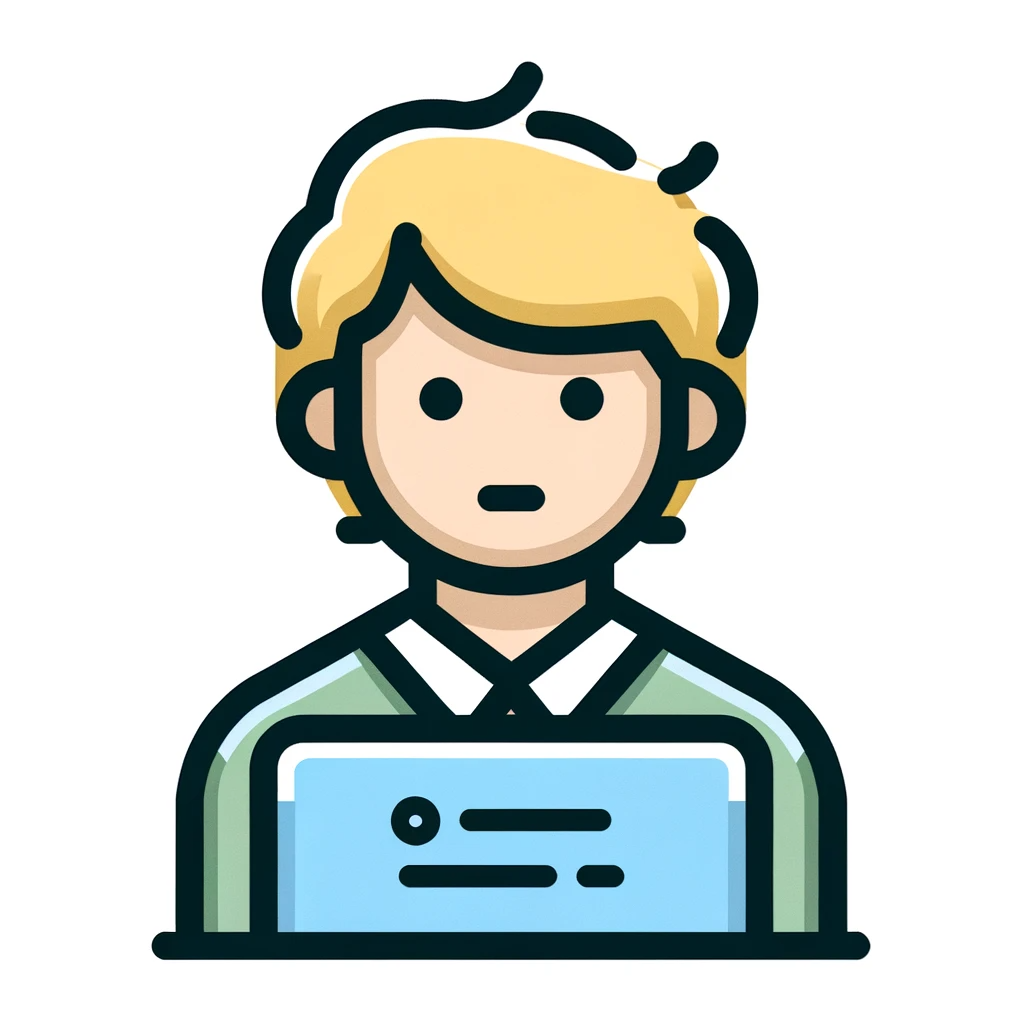
I found the method of fixing the random number pattern by specifying a seed value to be useful for testing and debugging programs!
Comments