We will explain in detail how to use ” querySelector ” and ” querySelectorAll ” , which are used when retrieving elements from an HTML document in JavaScript .
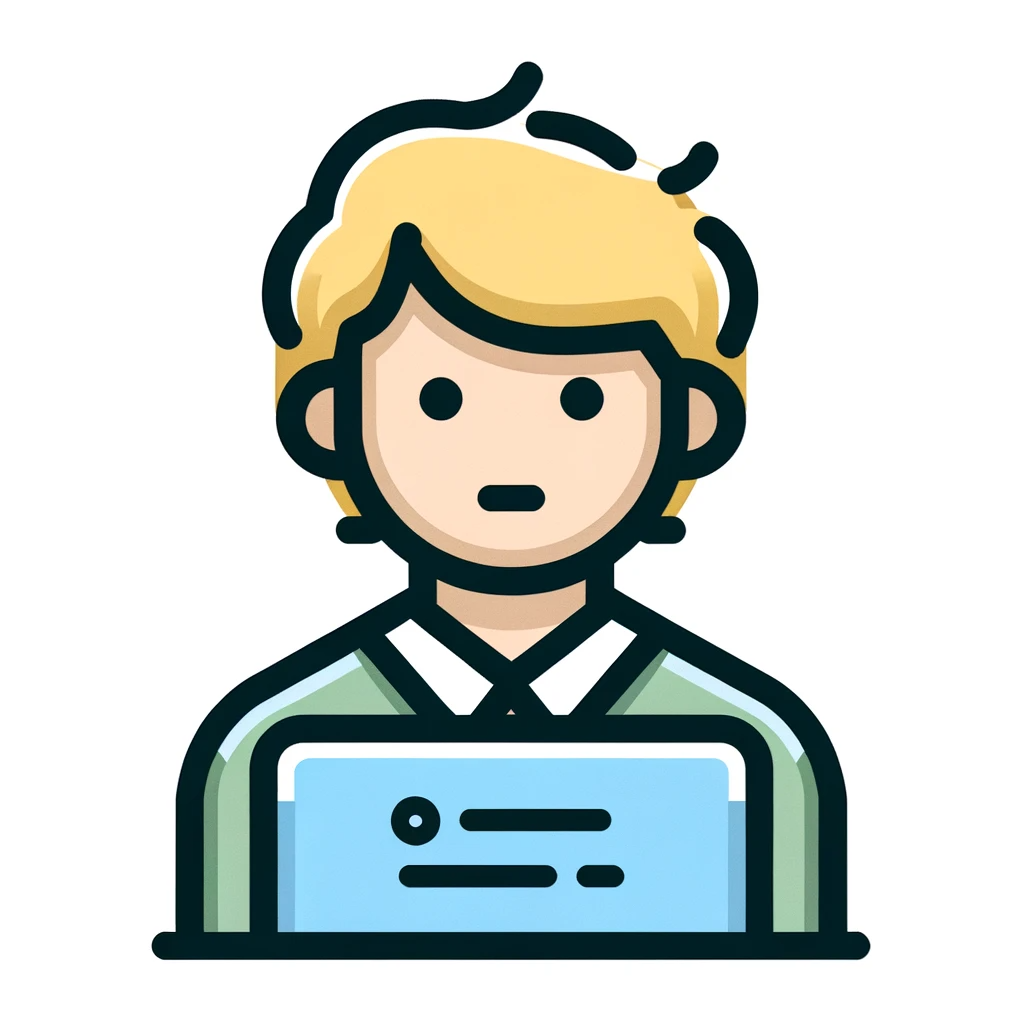
Do you have any recommendations on how to retrieve elements from an HTML document?
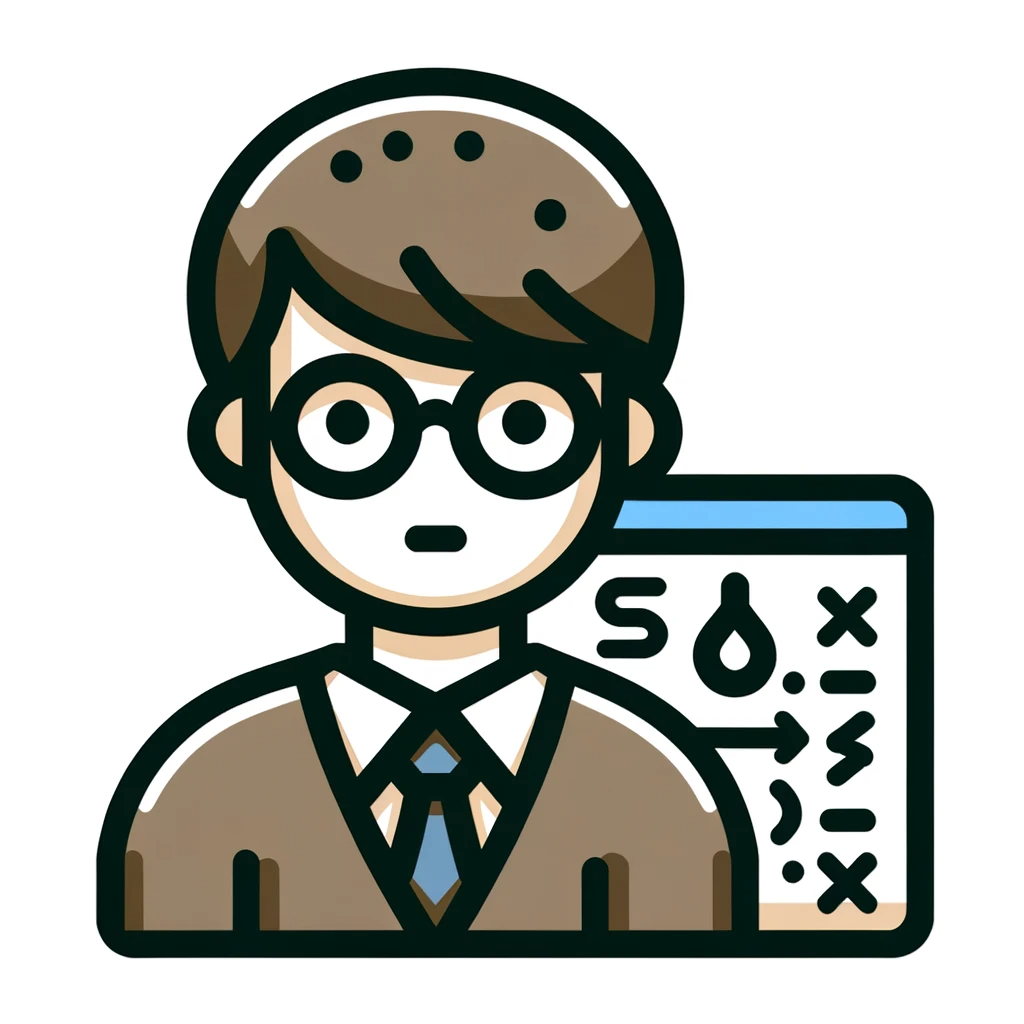
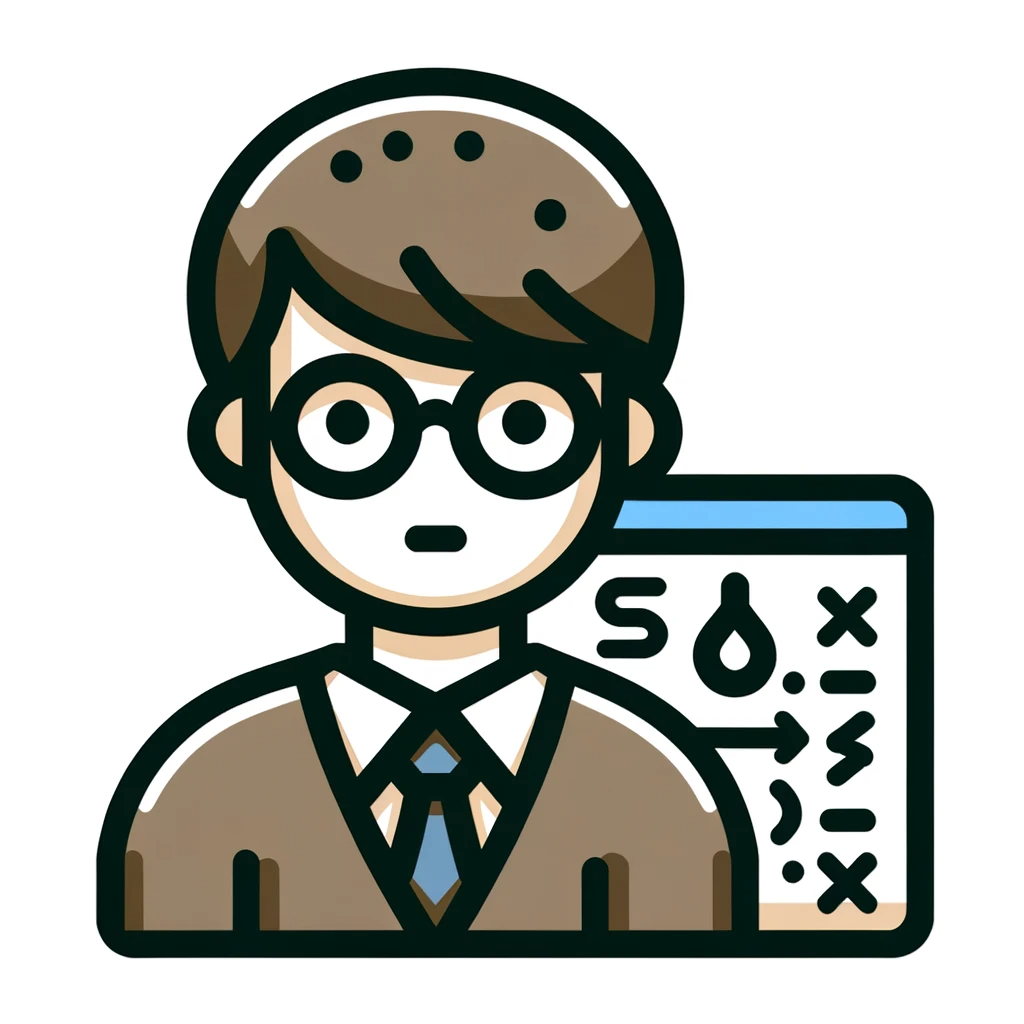
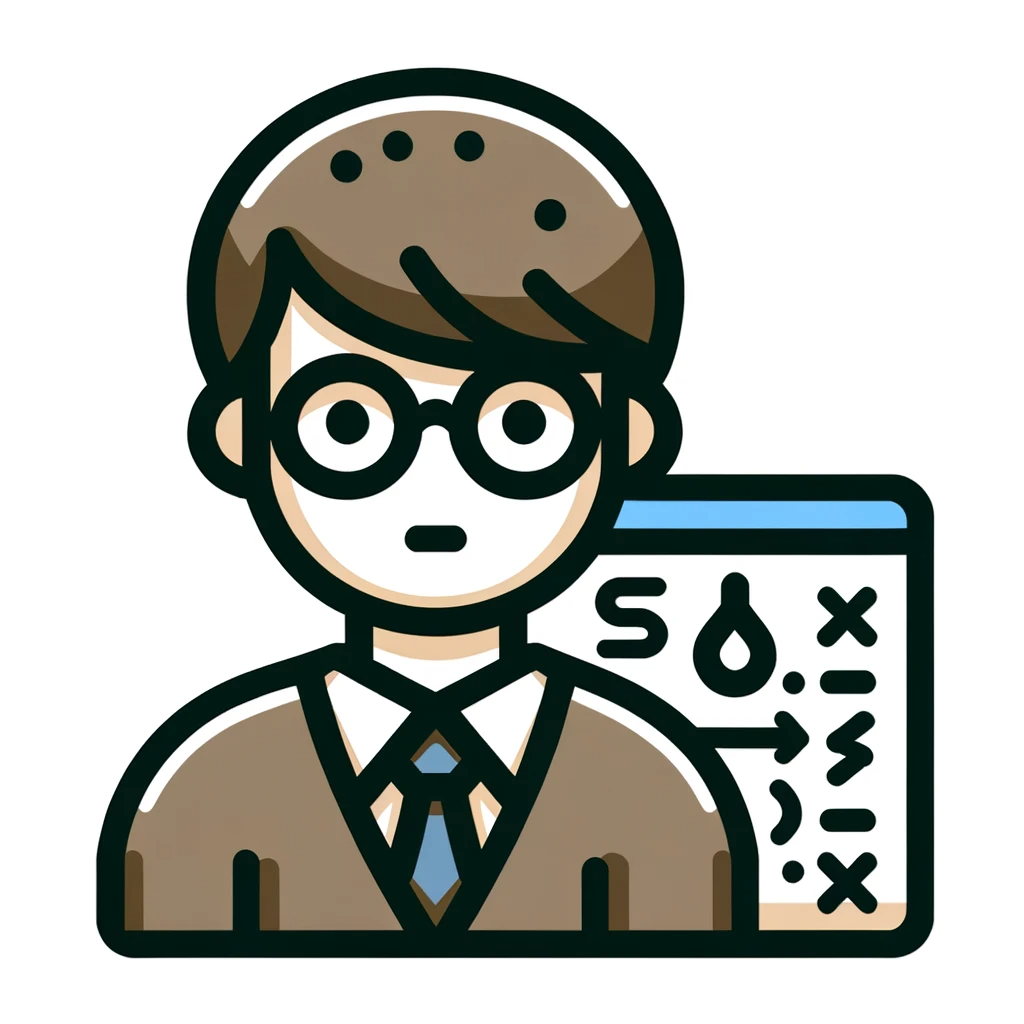
Then why not try using “querySelector” and “querySelectorAll”?
You can easily retrieve HTML elements using selector expressions.
What is querySelector/querySelectorAll? How to use it
The querySelector and querySelectorAll methods are methods for retrieving elements from an HTML document.
The querySelector method retrieves the first element found .
Also, the querySelectorAll method retrieves all found elements as an array .
You can easily retrieve HTML elements by specifying a selector expression.
To retrieve the element with “id=”myId” using the querySelector method, write as follows.
let element = document.querySelector("#myId");
console.log(element);
Also, if you want to retrieve the elements of “.myClass” using the querySelectorAll method, write as follows.
let elements = document.querySelectorAll(".myClass");
console.log(elements);
Explanation using sample programs of querySelector and querySelectorAll
The sample below uses the querySelector method and querySelectorAll method in two files: index.html and test.js.
<!DOCTYPE html>
<html>
<head>
<title>querySelector/querySelectorAll Example</title>
</head>
<body>
<header id="header">
<h1>Header Title</h1>
</header>
<nav class="navbar">
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
<main>
<p class="lead">Welcome to my website!</p>
</main>
<footer id="footer">
<p>Copyright © 2023</p>
</footer>
<script src="test.js"></script>
</body>
</html>
// querySelector
const header = document.querySelector('#header');
console.log(header);
// querySelectorAll
const links = document.querySelectorAll('.navbar a');
console.log(links);
In the above sample, in the index.html file, the id attribute “header” is set for the header element, the class attribute “navbar” is set for the nav element, and the class attribute “lead” is set for the p element.
In the test.js file, we use the querySelector method to retrieve the header element. The selector expression “#header” specifies the id attribute “header”. The retrieved element is assigned to the variable header and displayed using the console.log method.
Also, the querySelectorAll method is used to retrieve the a element in the class attribute “navbar”. The selector expression “.navbar a” specifies the a element within the class attribute “navbar”. The retrieved elements are assigned to the variable links and displayed using the console.log method.
By implementing in this format, you can easily retrieve HTML elements using “querySelector” and “querySelectorAll”.
Commonly used syntax for selector arguments
There are multiple classifications of selector expressions.
Below is a table listing CSS syntax, categorized into basic, hierarchy, filter (basic), filter (attribute), filter (child element), and filter (form state), so please use it as a reference.
syntax | overview | example |
---|---|---|
tagname | Get element by tag name | document.querySelectorAll('p') |
.classname | Get element by specifying class attribute | document.querySelectorAll('.lead') |
#idname | Get element by specifying id attribute | document.querySelector('#header') |
syntax | overview | example |
---|---|---|
element element | Get element by descendant | document.querySelectorAll('header h1') |
element > element | Get element by direct child | document.querySelectorAll('.navbar > ul > li') |
element ~ element | Get element by specifying siblings | document.querySelectorAll('.lead ~ footer') |
syntax | overview | example |
---|---|---|
:first-child | select first child element | p:first-child |
:last-child | select last child element | p:last-child |
:nth-child(n) | select nth child element | p:nth-child(2) |
:nth-last-child(n) | Select the nth child element counting from the back | p:nth-last-child(2) |
:only-child | select only child element | p:only-child |
syntax | overview | example |
---|---|---|
[attribute] | Select elements with attributes | input[type='text'] |
[attribute=value] | Select elements with matching attributes and values | input[type='text'] |
[attribute~=value] | Select elements whose attribute value contains value | input[type~='text'] |
[attribute|=value] | Select elements whose attribute value starts with value or value- | input[type|='text'] |
[attribute^=value] | Select elements whose attribute value starts with value | input[type^='text'] |
[attribute$=value] | Select elements whose attribute value ends with value | input[type$='text'] |
[attribute*=value] | Select elements whose attribute value contains value | input[type*='text'] |
syntax | overview | example |
---|---|---|
:enabled | Select valid form elements | <input>:enabled |
:disabled | Select invalid form element | <input>:disabled |
:checked | Select selected checkbox/radio button | <input type="checkbox">:checked |
Summary of this article
I explained how to use ” querySelector ” and ” querySelectorAll “.
- querySelector and querySelectorAll are methods for retrieving HTML elements.
- querySelector gets the first element found, querySelectorAll gets all elements.
- You can easily retrieve HTML elements using selector expressions.
- There are several ways to use querySelector and querySelectorAll by specifying id, class, tag name, etc.
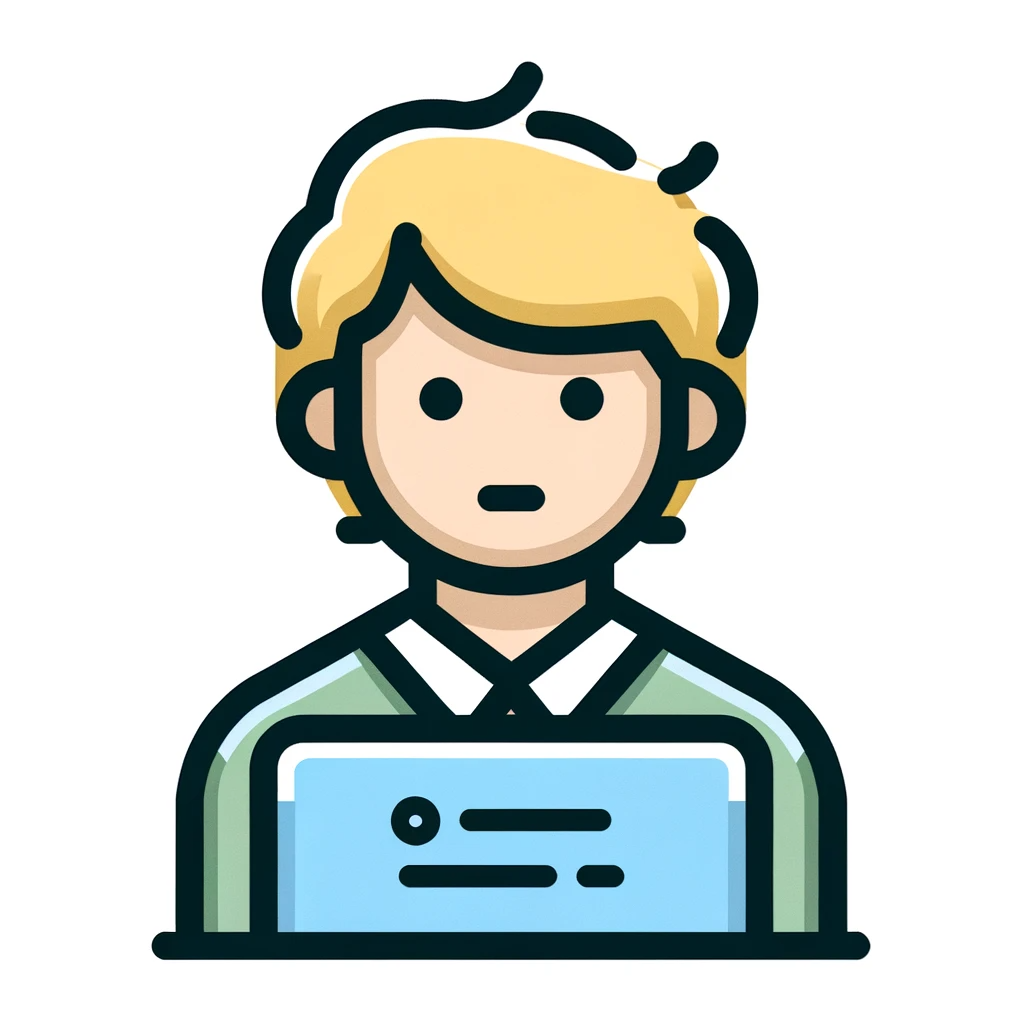
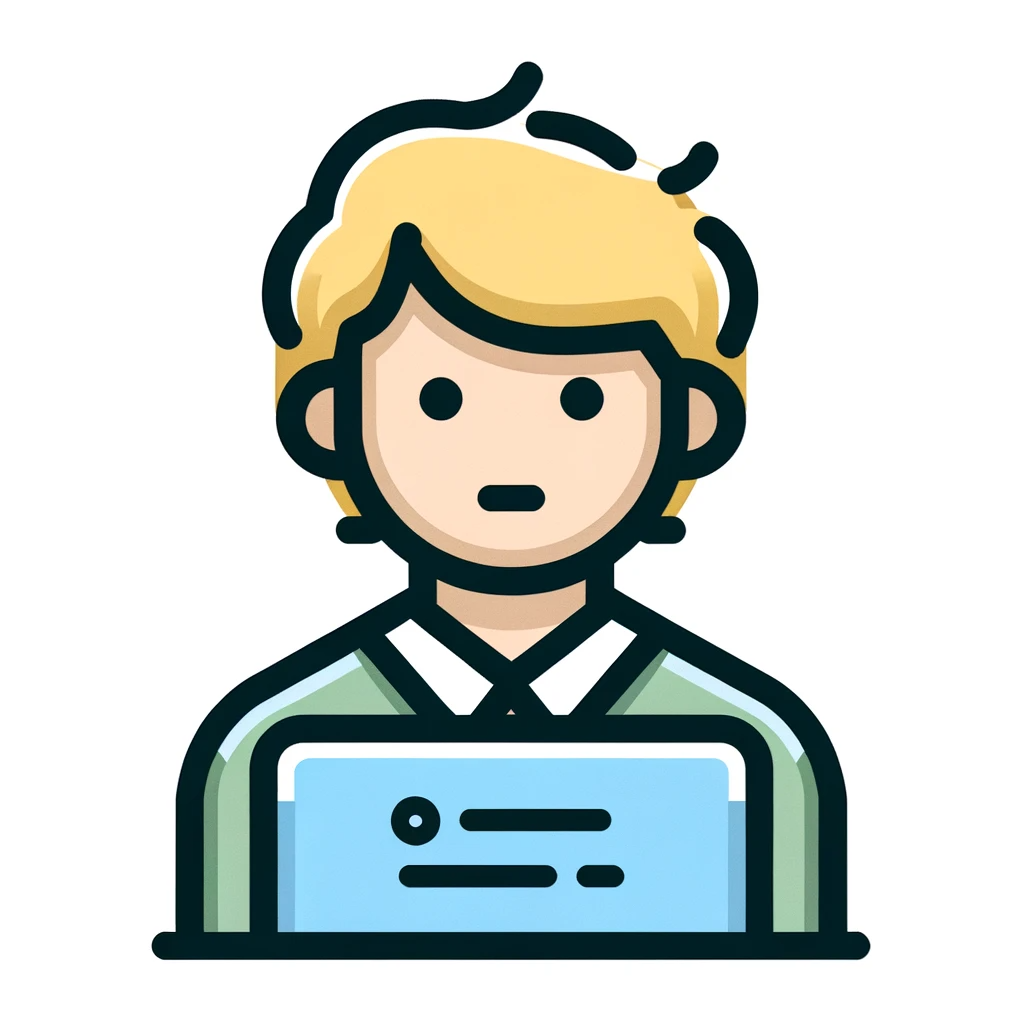
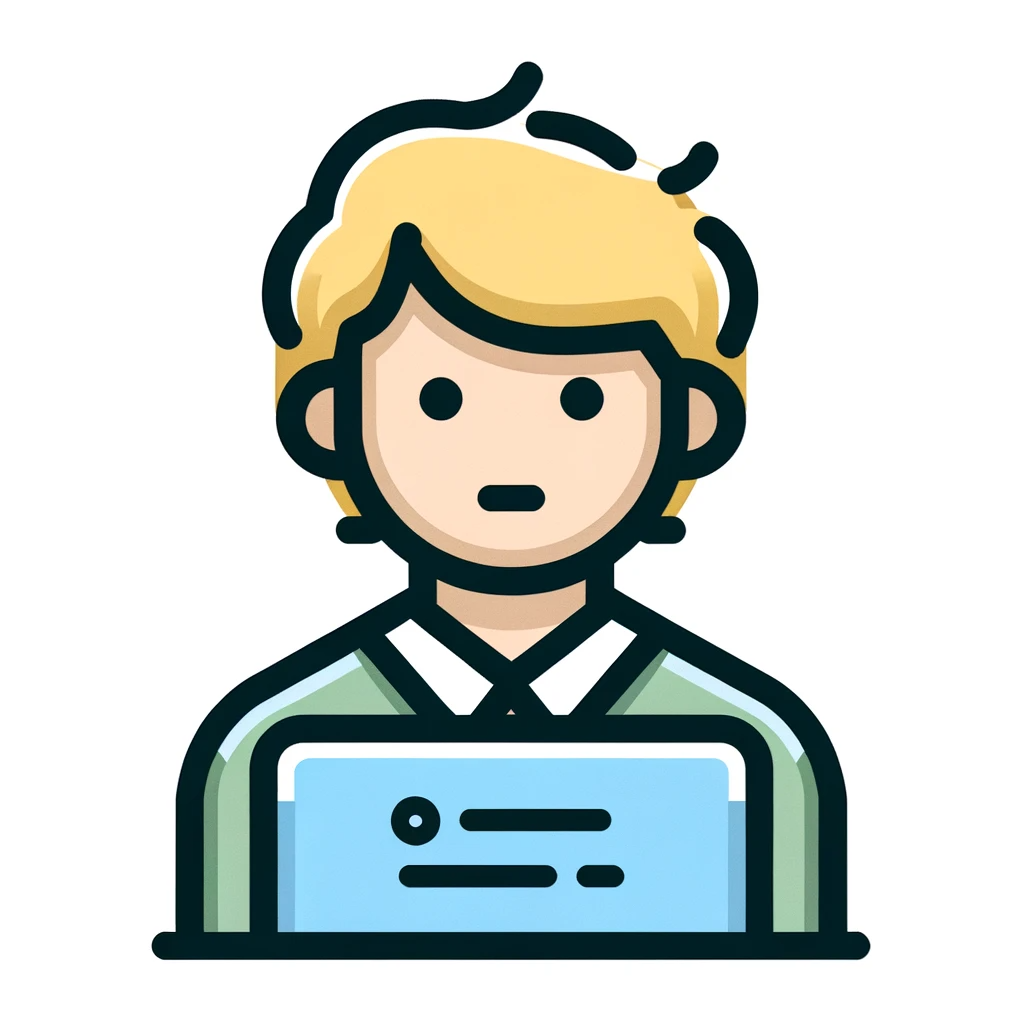
The querySelector and querySelectorAll methods seem easy to use.
I would like to try it right away!
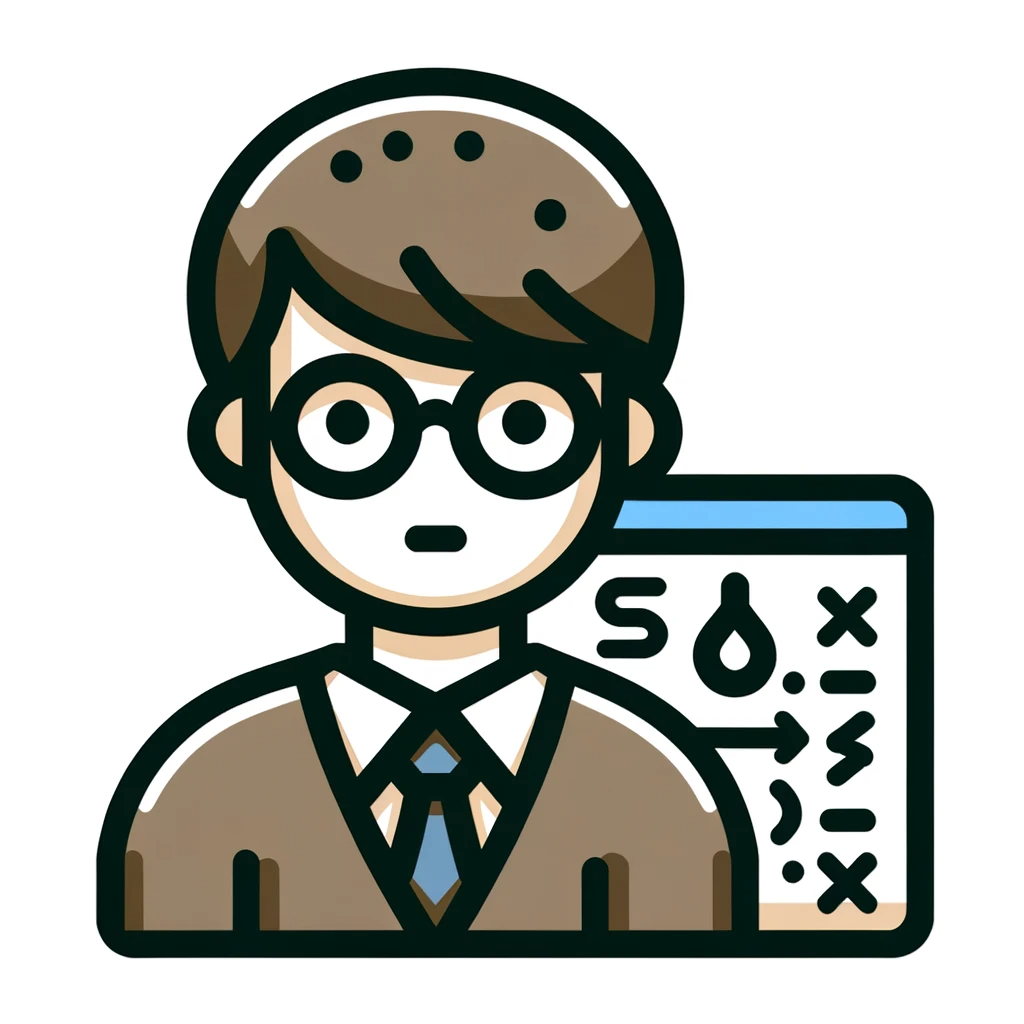
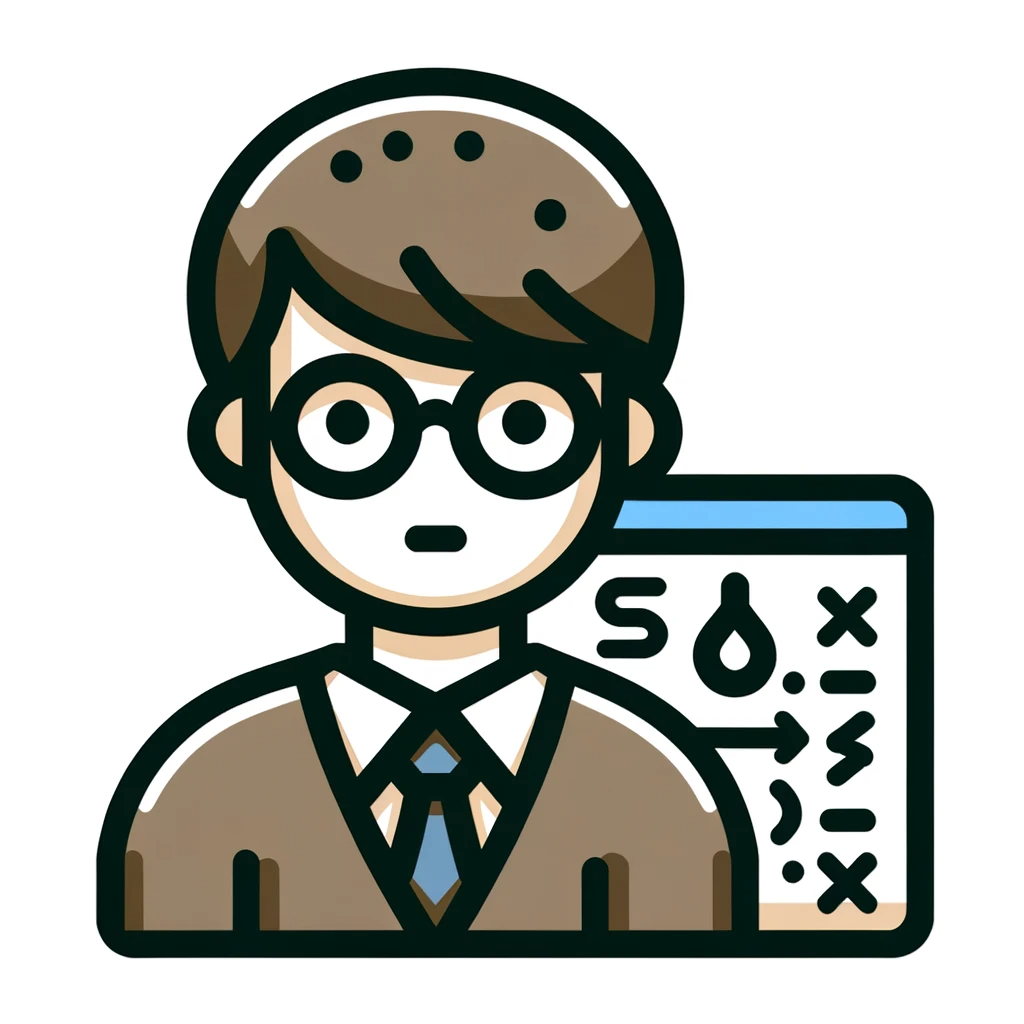
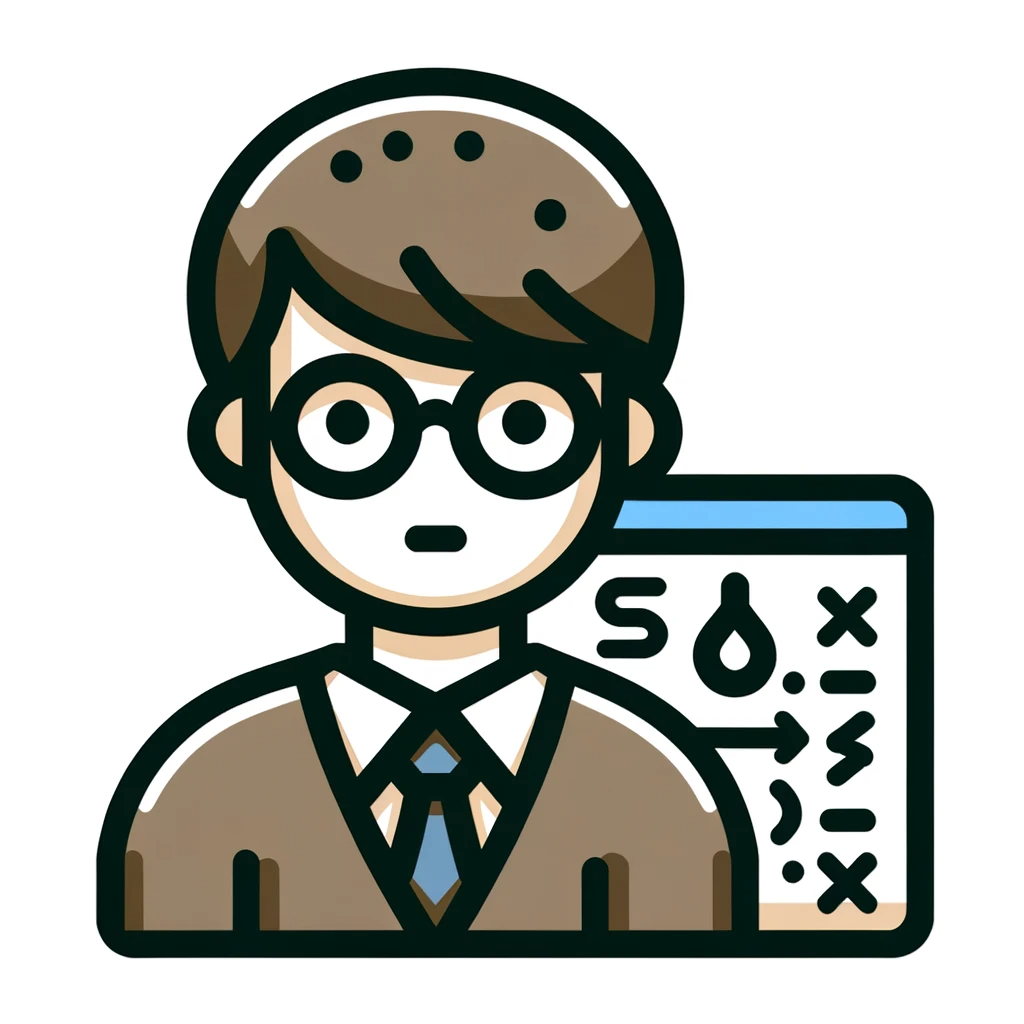
Remembering how to specify selector expressions will be very useful when retrieving HTML elements in the future.
Comments