This article explains how to use JavaScript to send data through query information.
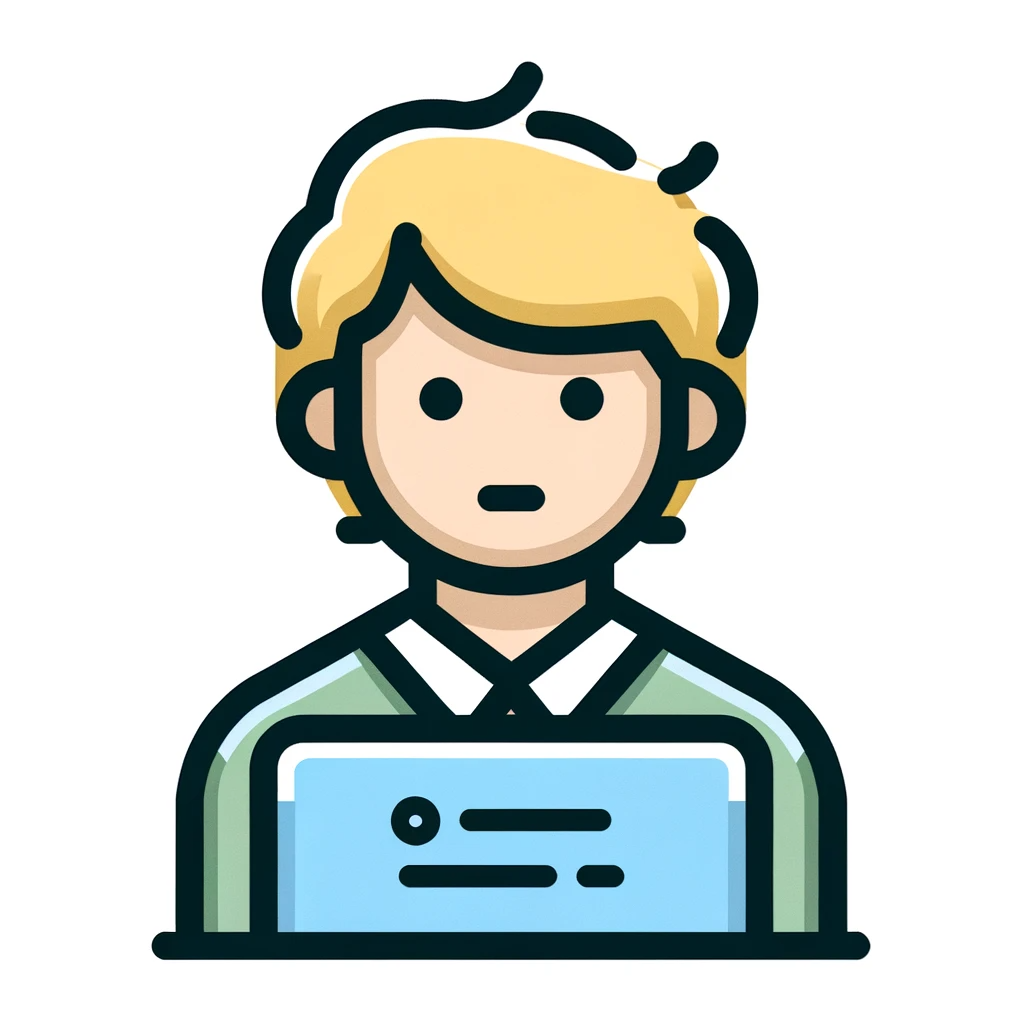
What are the ways to send data via query information?
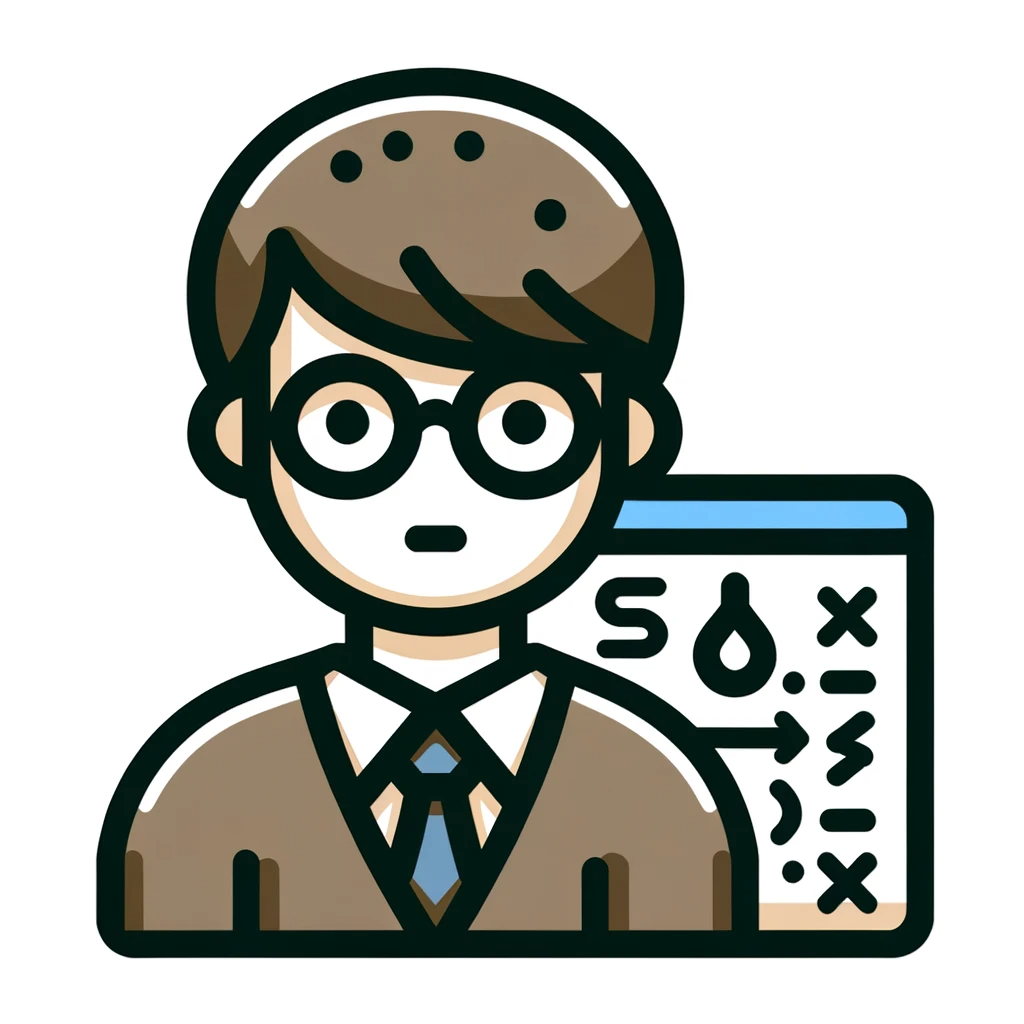
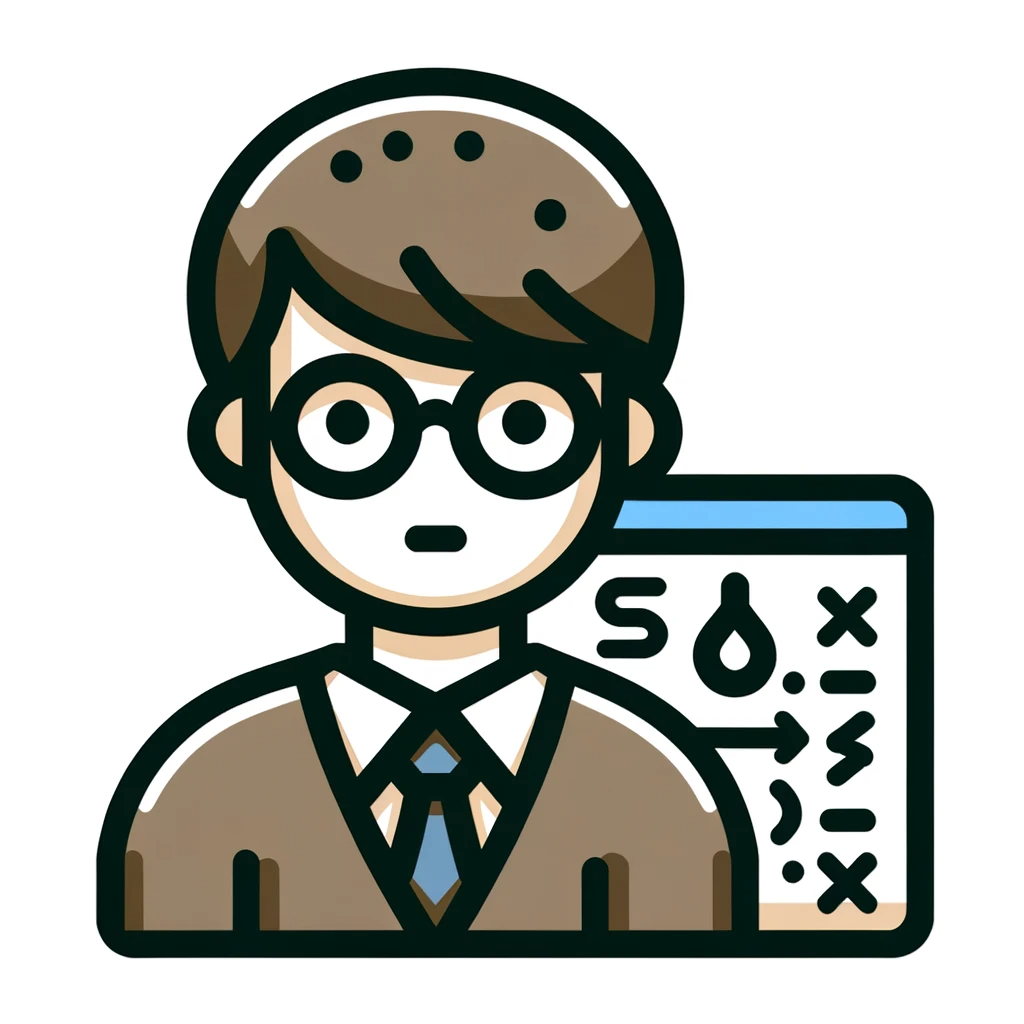
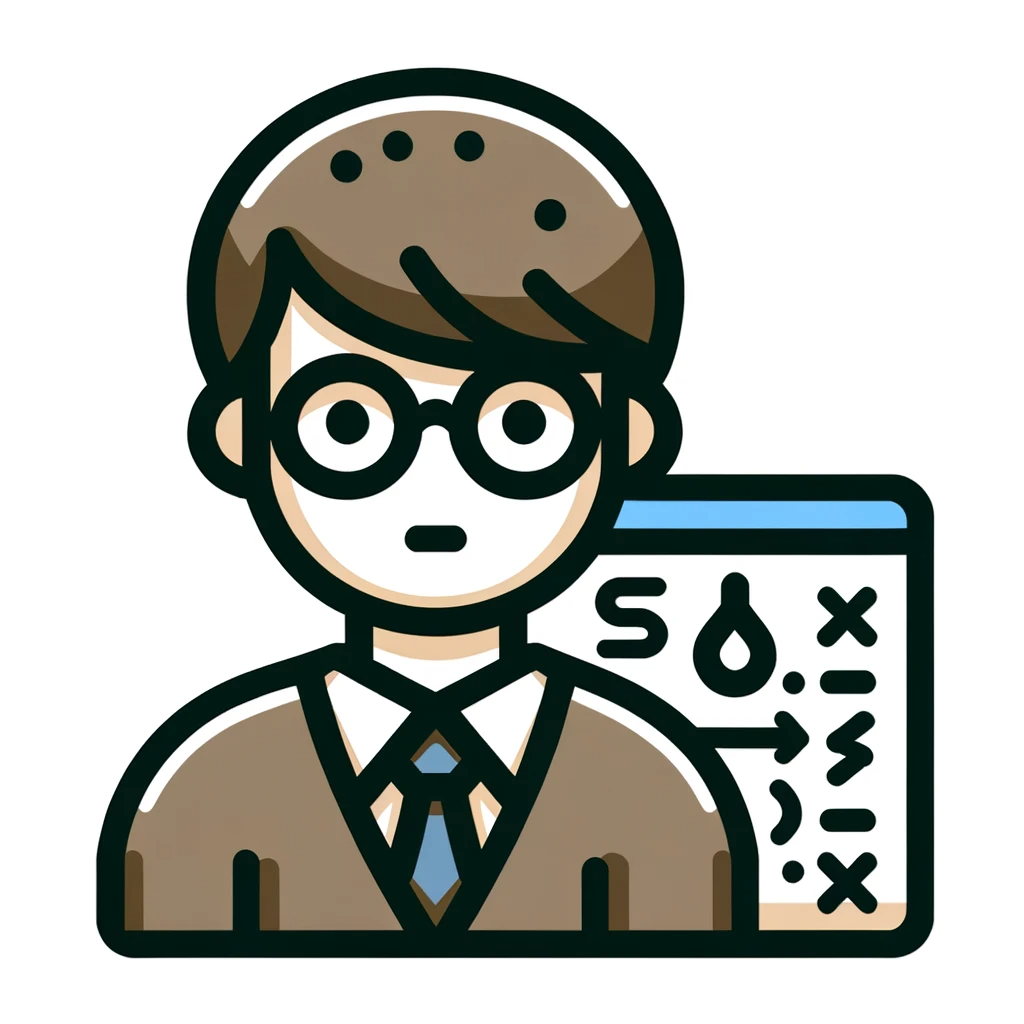
Data can be sent by acquiring data with JavaScript and adding it to the URL as query information.
What is query information?
Query information is a string of key-value pairs that appears at the end of a URL. Query information can be used for purposes such as sharing or searching and filtering data by adding various information about web pages and applications to URLs.
Query information is written after the “?” in the URL, and multiple keys and values are separated by “&”. For example, the URL below contains the key-value pair “q=JavaScript”.
https://example.com/search?q=JavaScript
Query information is a string of key-value pairs that appears at the end of a URL. Query information can be used for purposes such as sharing or searching and filtering data by adding various information about web pages and applications to URLs.
Query information is written after the “?” in the URL, and multiple keys and values are separated by “&”. For example, the URL below contains the key-value pair “q=JavaScript”.javascriptCopy codehttps://example.com/search?q=JavaScript
To obtain query information, use the search property of the JavaScript location object. The search property returns the query information contained at the end of the current page’s URL as a string. Also, by using the URLSearchParams object, you can easily handle query information.
How to send data using query information
There are several ways to send data through query information using JavaScript:
- Data can be sent by canceling the form’s submit event, acquiring the data using JavaScript, and adding it to the URL as query information.
- You can also send a request to a URL containing query information using XMLHttpRequest or fetch API.
How to get query information
To obtain query information for the current page in JavaScript, use the search property of the location object. The search property returns the query information contained at the end of the current page’s URL as a string. Specifically, it can be obtained with the following code.
const queryString = window.location.search;
The retrieved query information can be easily handled using the URLSearchParams object. The URLSearchParams object is an object that parses query information and breaks it down into key-value pairs for easy handling.
The following is an example of using the URLSearchParams object to handle retrieved query information.
const queryString = window.location.search;
const searchParams = new URLSearchParams(queryString);
// Obtain values from query information
const q = searchParams.get('q'); // 'JavaScript'
// Convert query information to objects
const paramsObj = Object.fromEntries(searchParams.entries());
// { q: 'JavaScript' }
The URLSearchParams object can use the get method to retrieve the value corresponding to the specified key from the query information. You can also use the entries method to convert query information to an array, and then use the Object.fromEntries method to convert it to an object.
Sample program for sending data using query information
The sample program below shows how to append data entered in a form to a URL as query information.
<form id="my-form">
<input type="text" name="name" placeholder="Name">
<input type="email" name="email" placeholder="Email Address">
<button type="submit">send</button>
</form>
const form = document.getElementById('my-form');
form.addEventListener('submit', function(event) {
event.preventDefault(); // Cancel the default behavior of the form
const formData = new FormData(form); // Retrieve form data
const searchParams = new URLSearchParams(formData); // Converting a FormData object to a URLSearchParams object
const queryString = searchParams.toString(); // Convert URLSearchParams object to string
const url = window.location.href + '?' + queryString; // Append query information to the URL
window.location.href = url; // Reload the page
});
This program cancels the form’s submit event, retrieves the data entered in the form, and converts it into a FormData object. FormData object is an object that allows you to easily retrieve data entered in a form.
Next, convert the FormData object to a URLSearchParams object. The URLSearchParams object is an object for easily handling query information. You can easily create query information by converting FormData objects to URLSearchParams objects.
Finally, send the data by converting the URLSearchParams object to a string, appending it to the end of the URL, and reloading the page.
summary
We explained how to use JavaScript to send data through query information.
- Query information is a string consisting of key-value pairs written at the end of the URL.
- There are two ways to send data through query information using JavaScript: appending it to a URL using a form, and using XMLHttpRequest or the fetch API.
- You can use the search property of the location object to get the query information for the current page.
- By using the URLSearchParams object, you can easily handle query information.
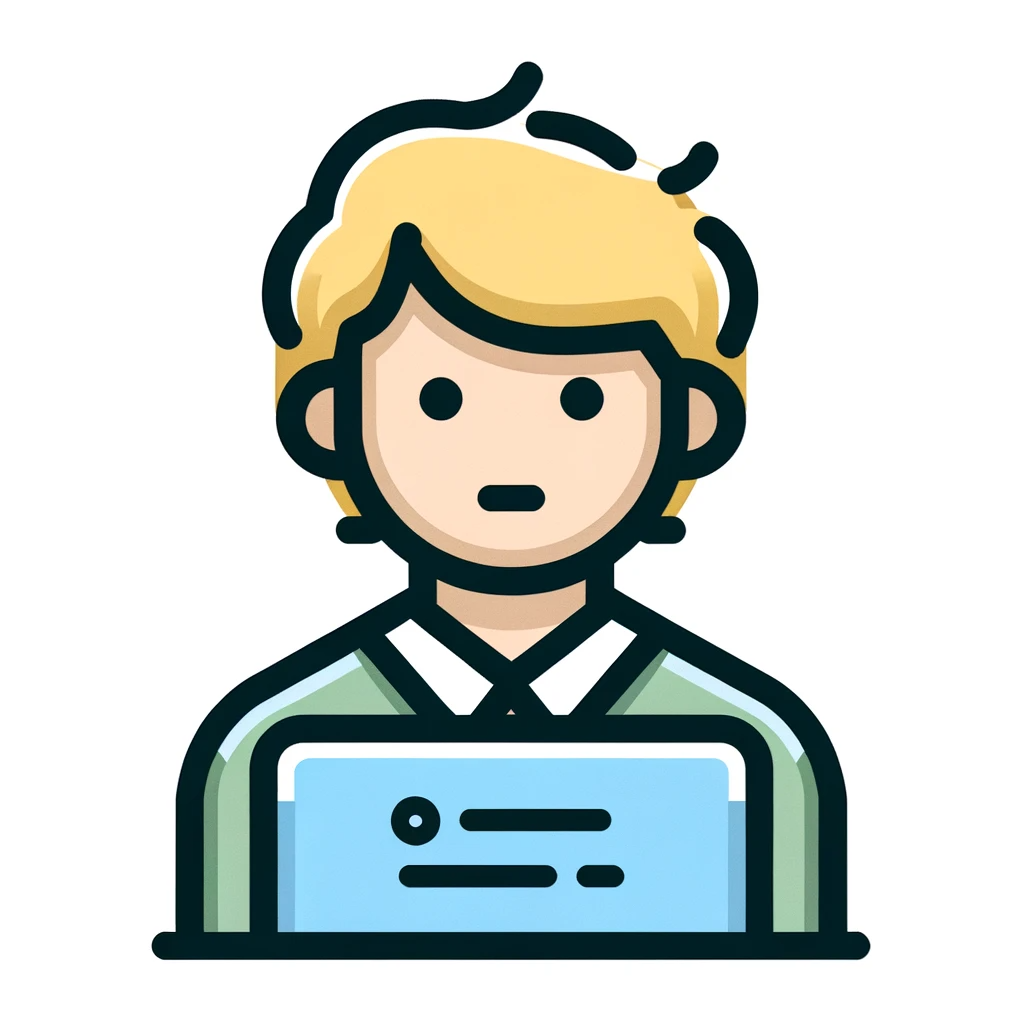
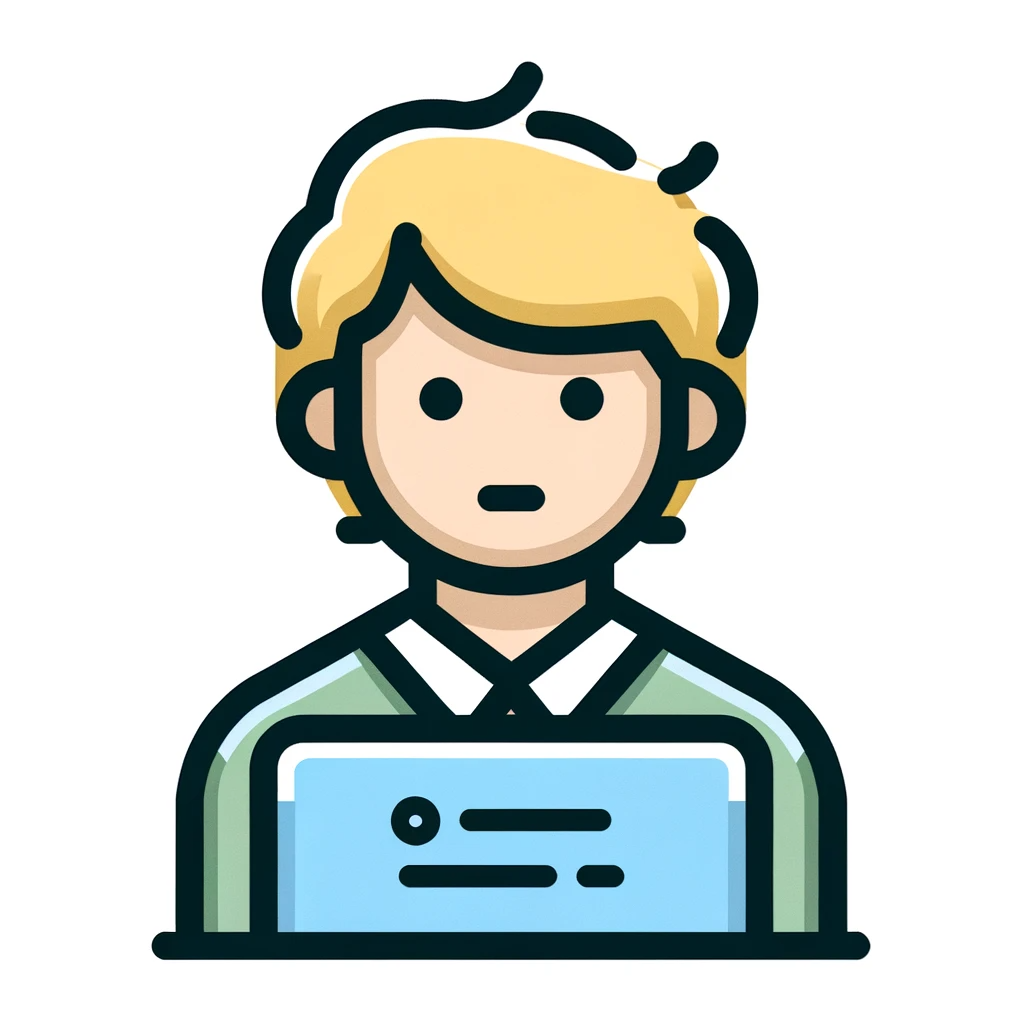
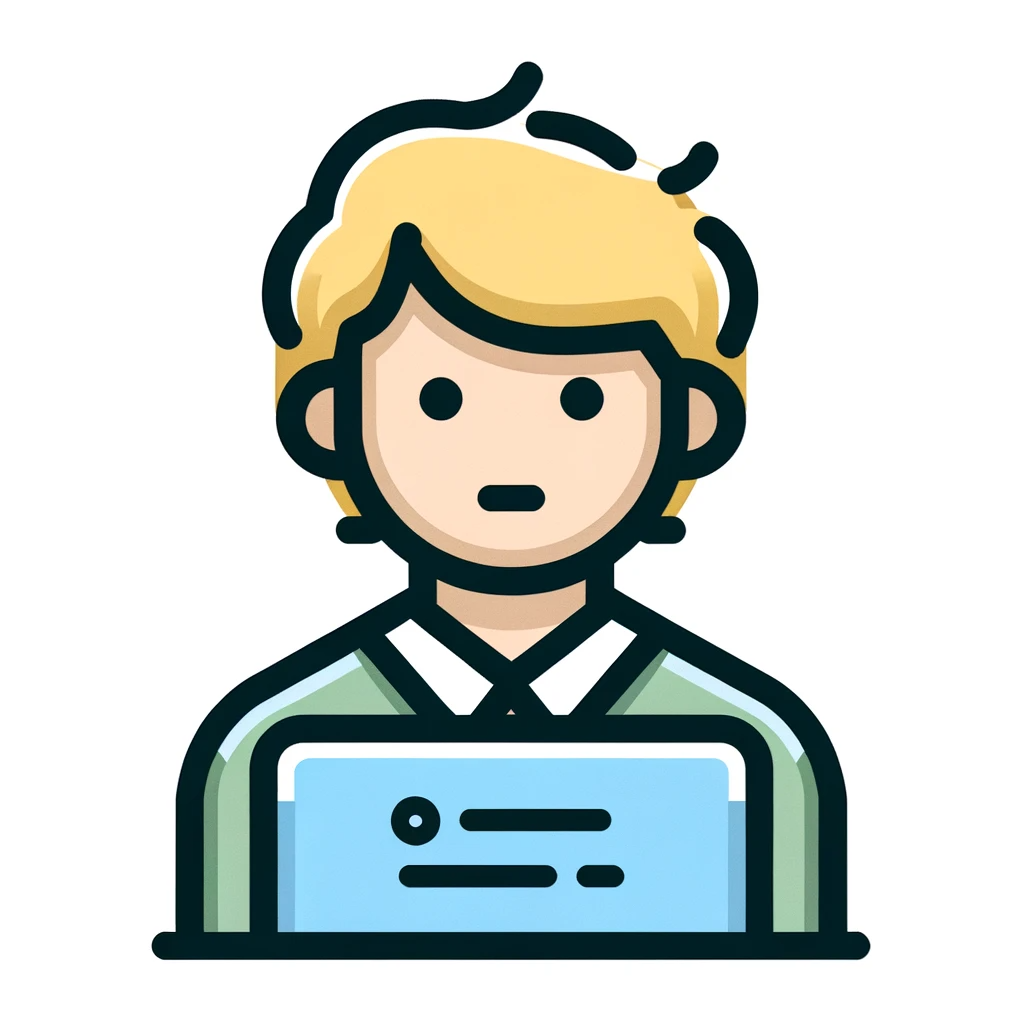
Using JavaScript to send data through query information is a simple yet extremely useful method!
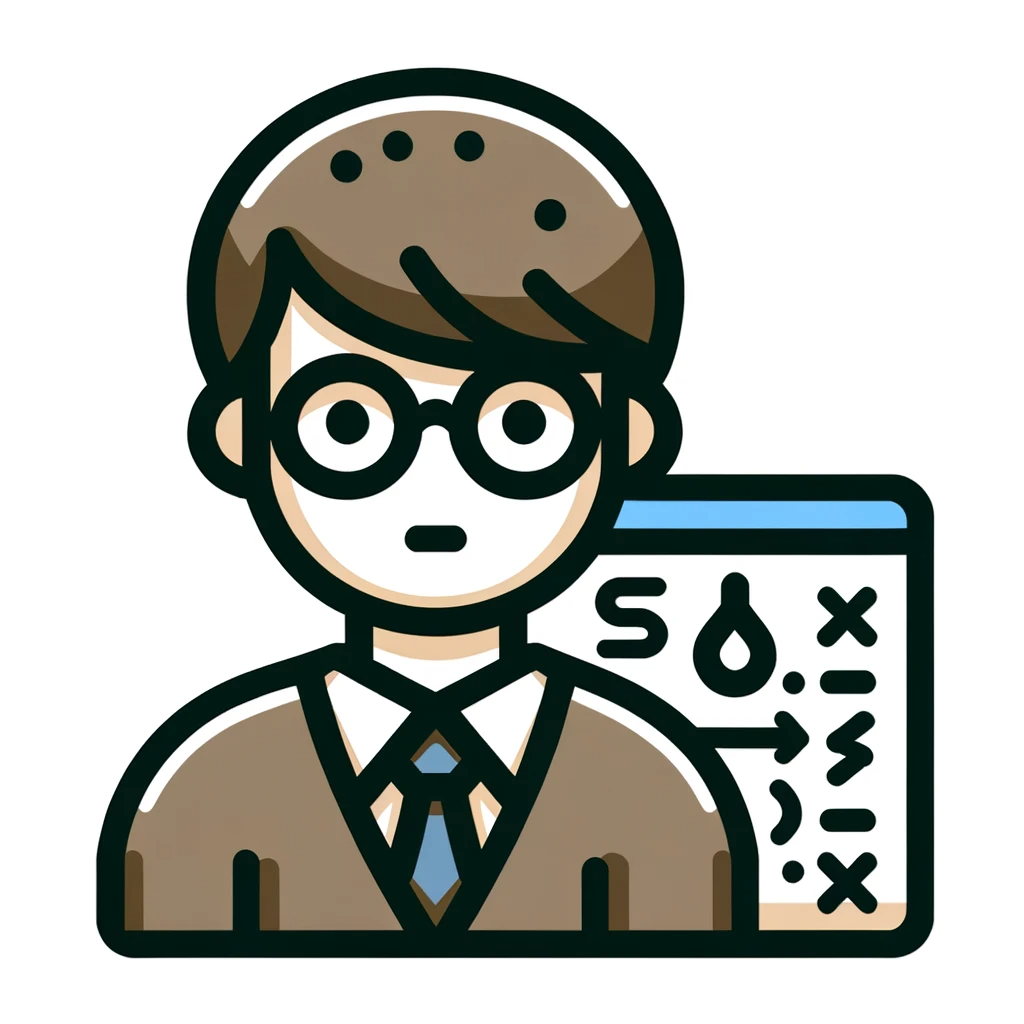
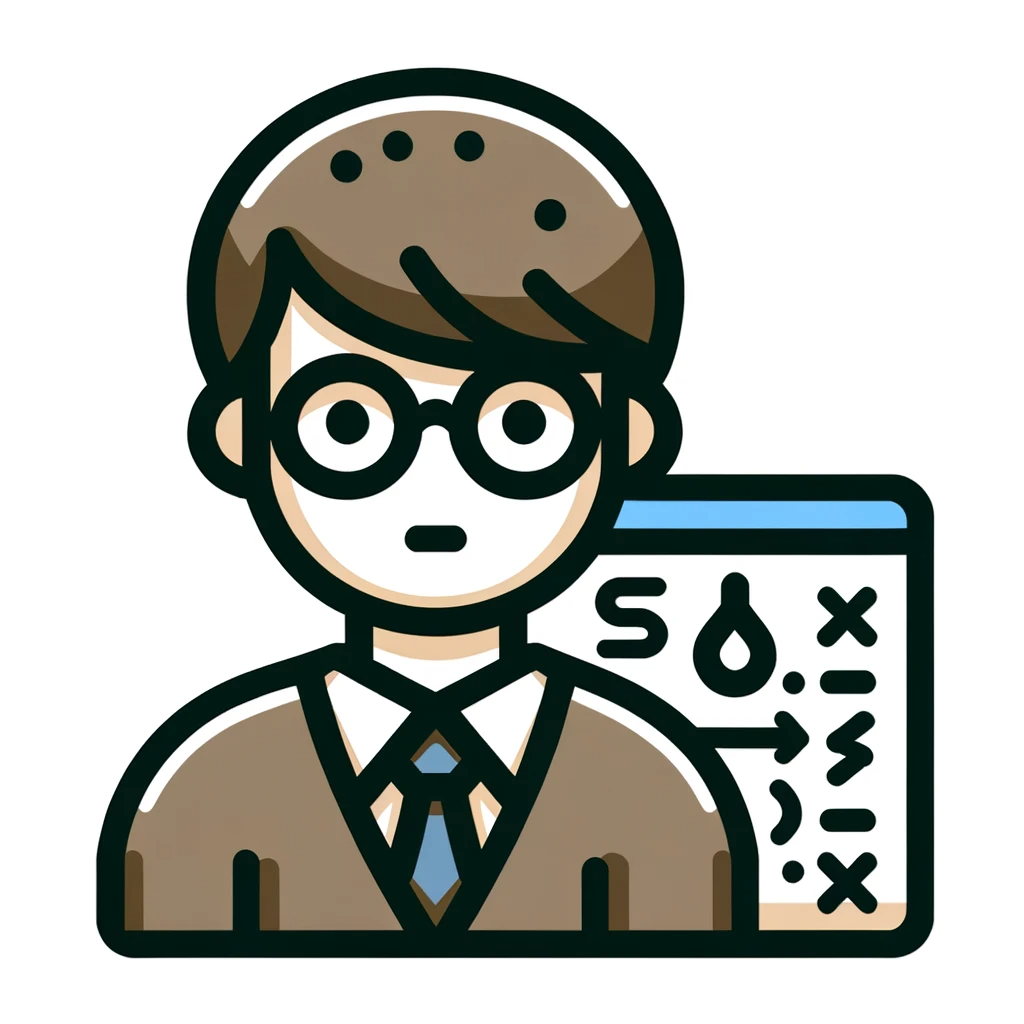
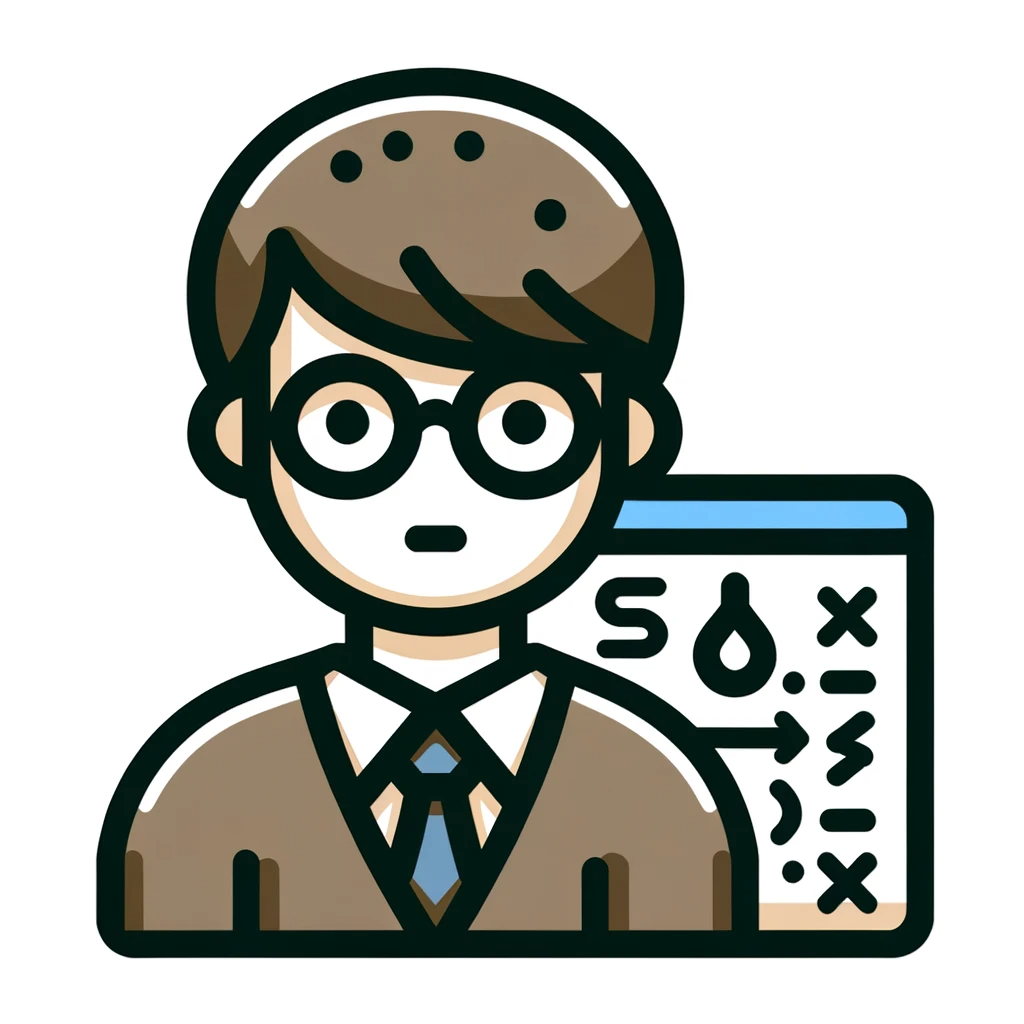
Sending data using query information is one of the most important techniques in web development.
However, query information has a limit on the length of the URL, so it is not suitable for sending large amounts of data.
You also need to be careful about security.
Avoid including sensitive information such as passwords in your query information.
Comments