This article explains how to execute a process when one of multiple asynchronous processes is completed in JavaScript .
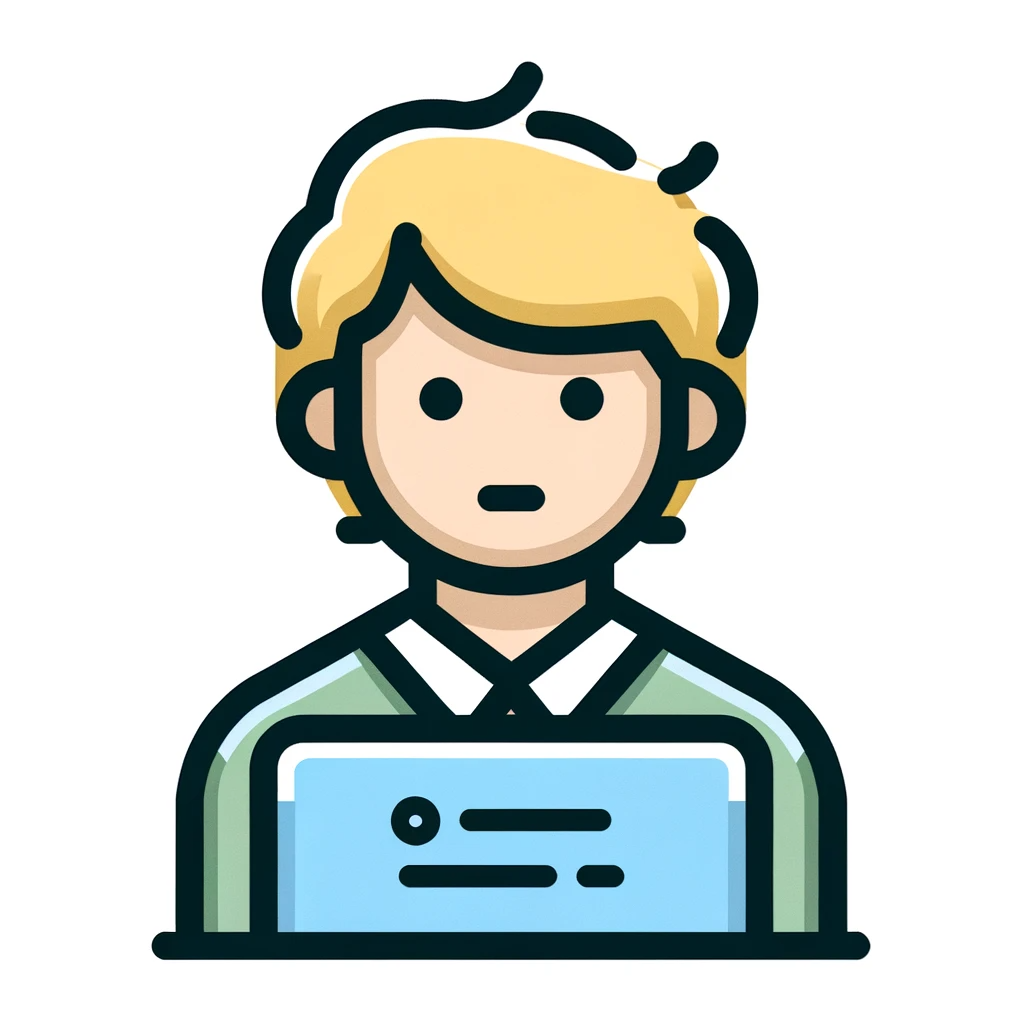
How can I run multiple asynchronous operations at the same time and continue processing when one completes?
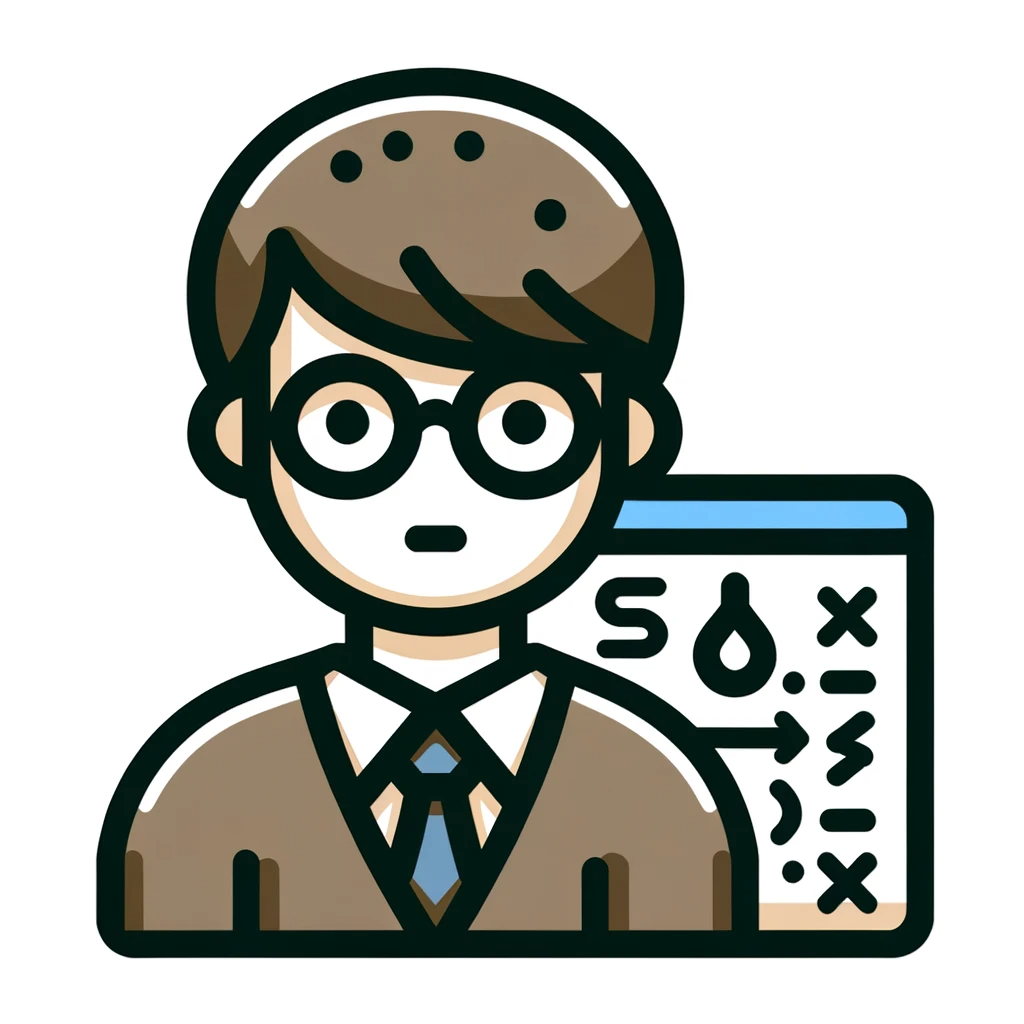
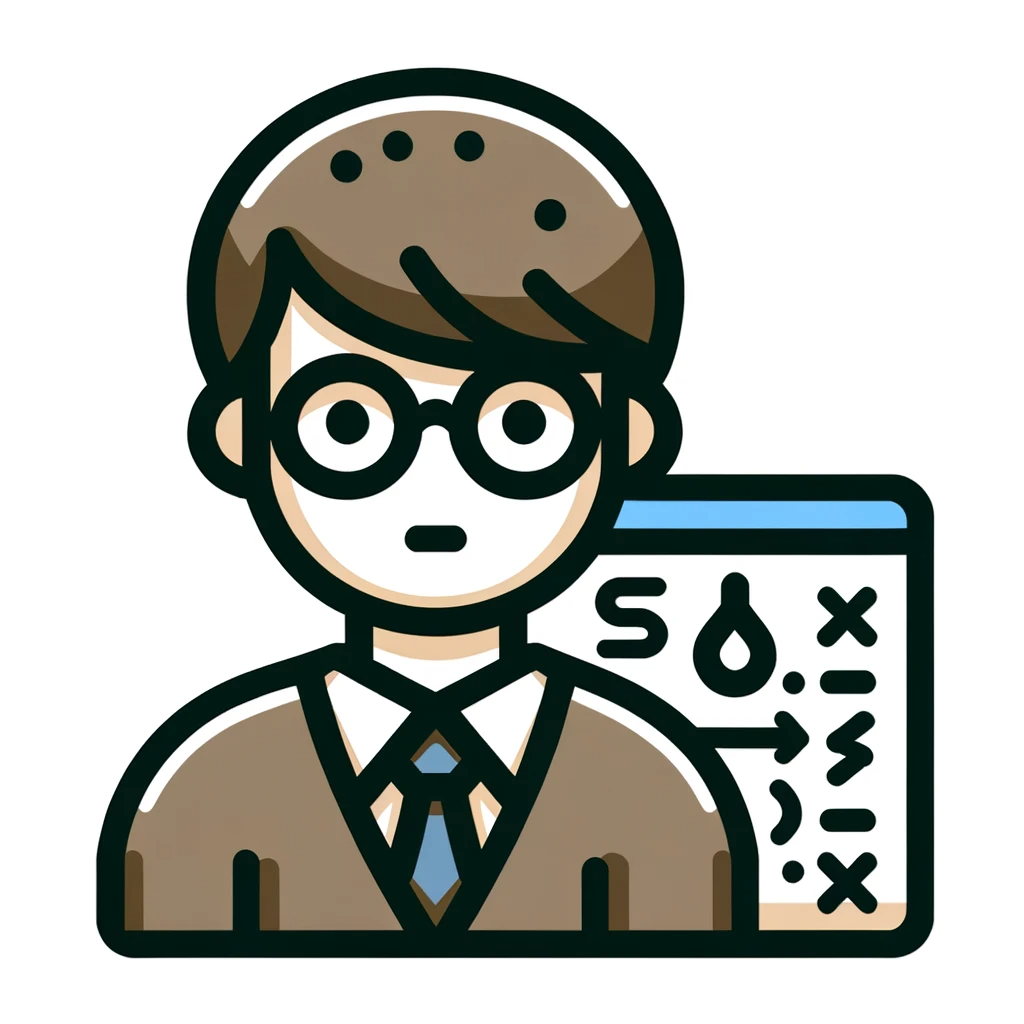
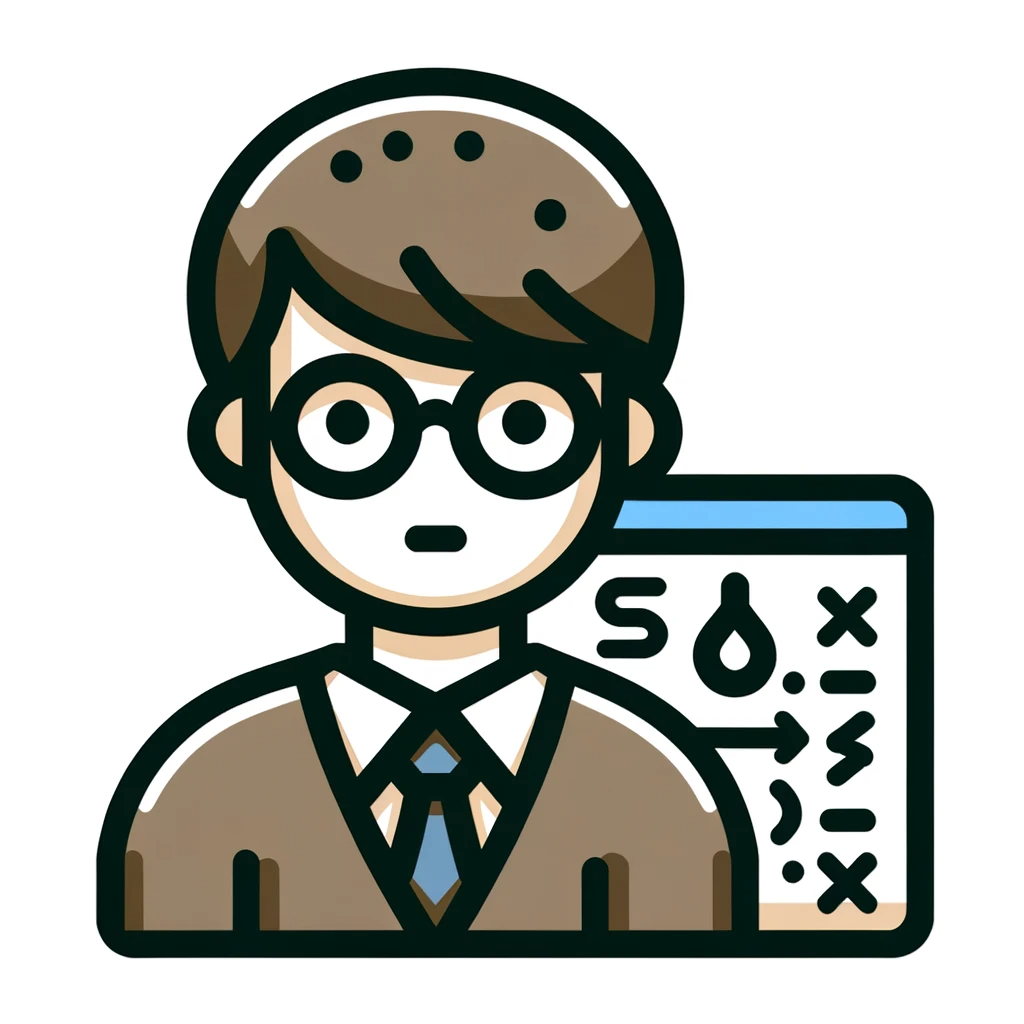
By using Promise.race, you can select the first completed asynchronous process to continue processing.
How to manage multiple asynchronous operations using Promise.race
Use Promise objects to perform asynchronous processing.
Promise.race accepts multiple Promise objects and selects the first completed Promise object.
This allows you to retrieve the first completed asynchronous process and continue processing.
Promise.race (array of Promise objects to monitor)
To use Promise.race, create Promise objects and pass them to Promise.race.
Promise.race accepts multiple Promise objects as an array. The first completed Promise object can be retrieved by the then method.
For example, you can call multiple APIs and get the response of the first completed API as shown below.
Promise.race([
fetch('/api1'),
fetch('/api2'),
fetch('/api3')
]).then(response => {
// Process the first completed API response
});
In the above sample, three APIs are called asynchronously using the fetch function . We use Promise.race to retrieve the response of the first completed API and continue processing.
Promise.race selects the first Promise object that completes, so Promise objects that don’t complete are ignored. Promise.race also picks up the first error that occurs.
Promise.race() is useful when asynchronous processing is in a race condition. This is useful, for example, when calling multiple APIs and using the data returned first.
It can also be used to implement timeout handling.
Fetch is an API for asynchronous communication that is standardly used in JavaScript. To use the Fetch API, call the fetch() function.
The Fetch function passes the URL you want to request as the first argument. Pass the options required for the request in the second argument.
Summary
The following is a summary of how to execute a process when one of multiple asynchronous processes completes.
- Use Promise objects to manage asynchronous processing in JavaScript.
- By using Promise.race, you can select the first completed asynchronous process among multiple asynchronous processes and continue processing.
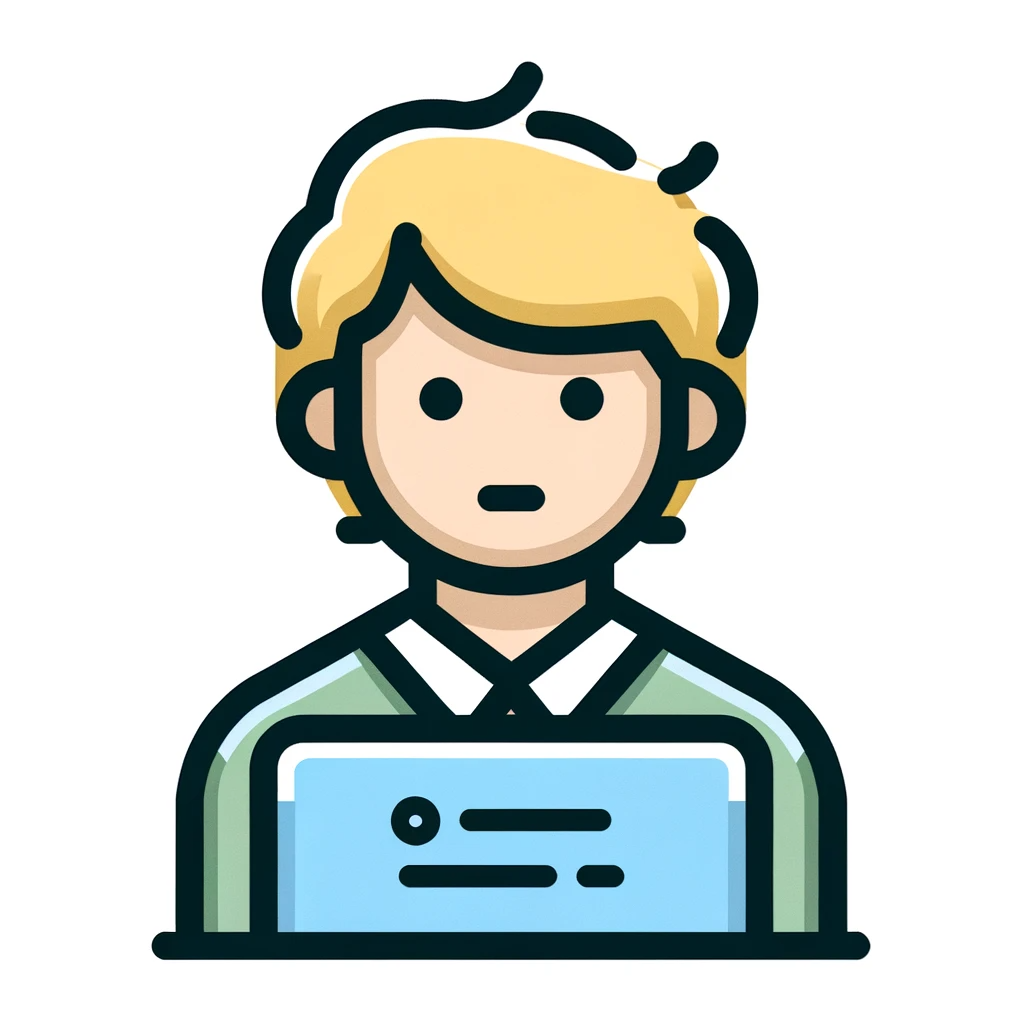
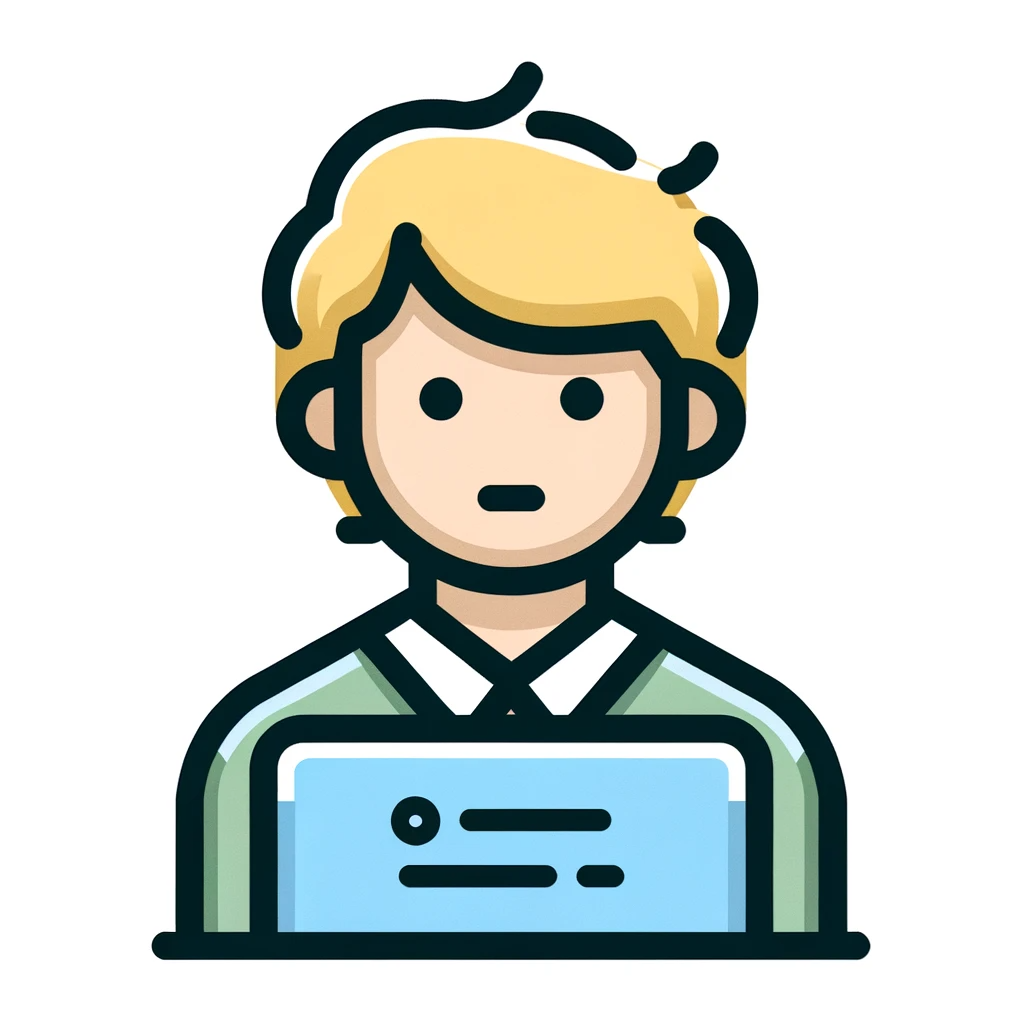
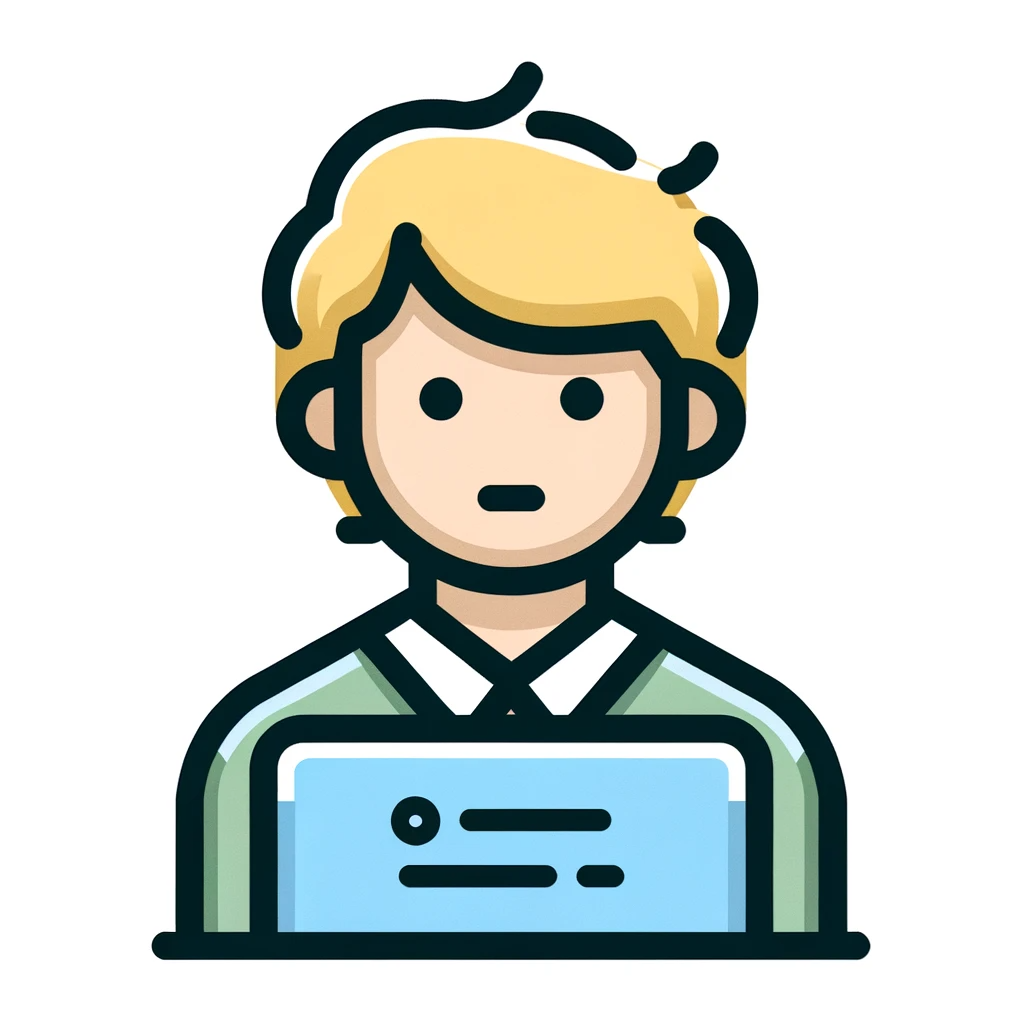
I found it very convenient to use Promise.race to run multiple asynchronous operations at the same time and choose the one that completed first!
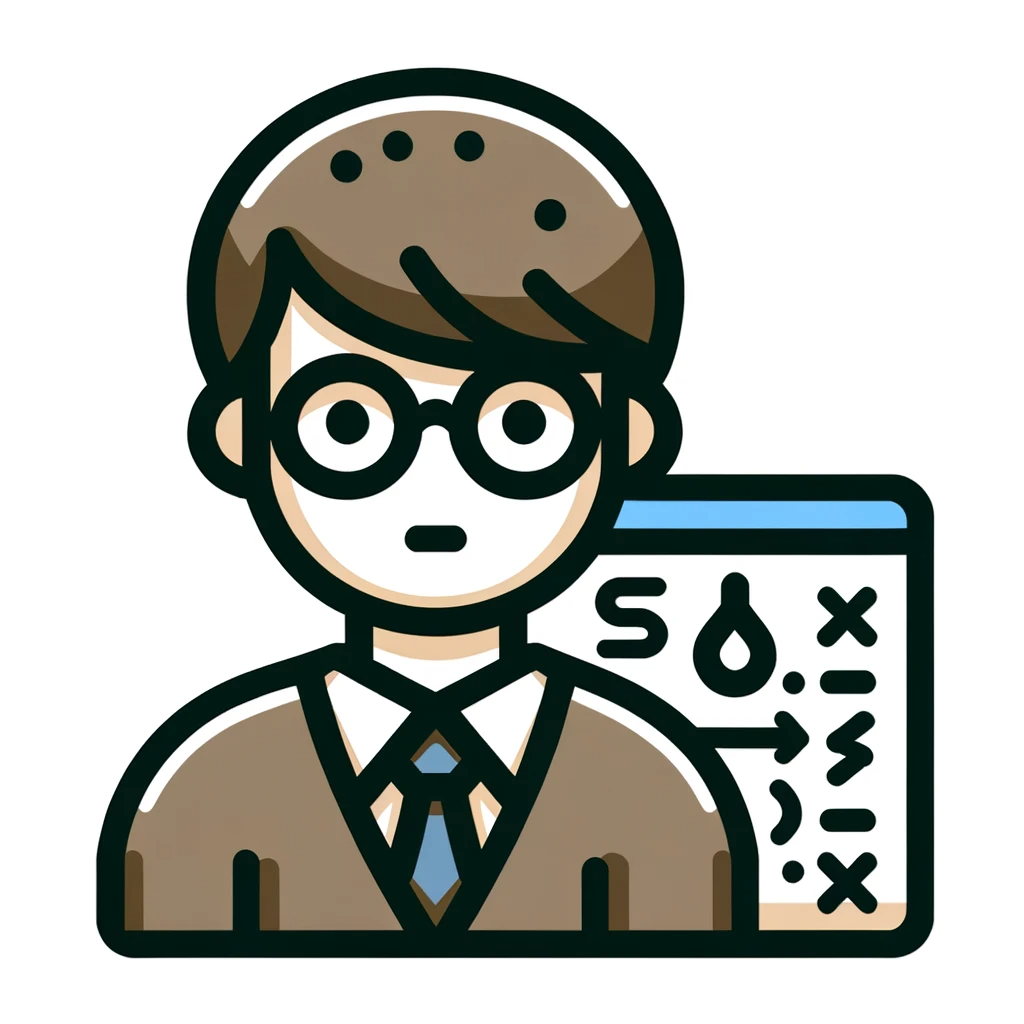
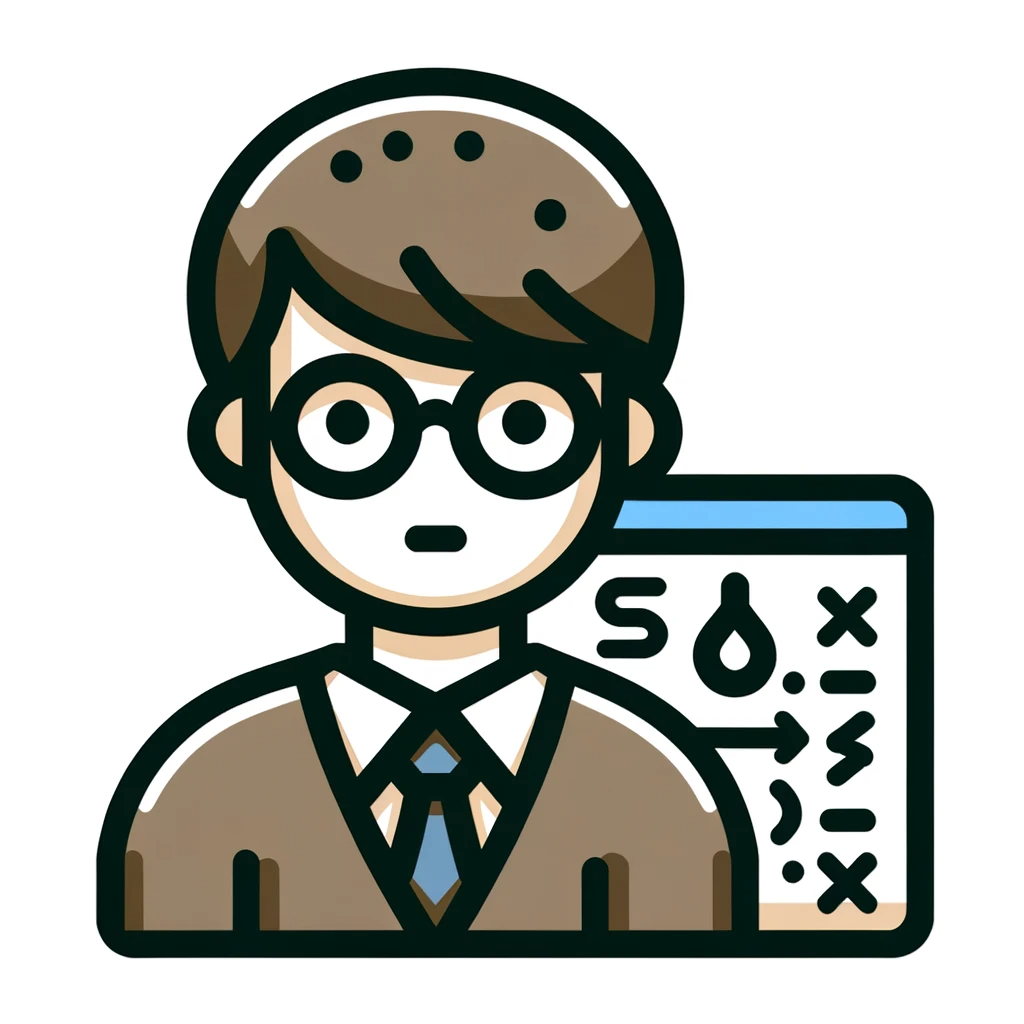
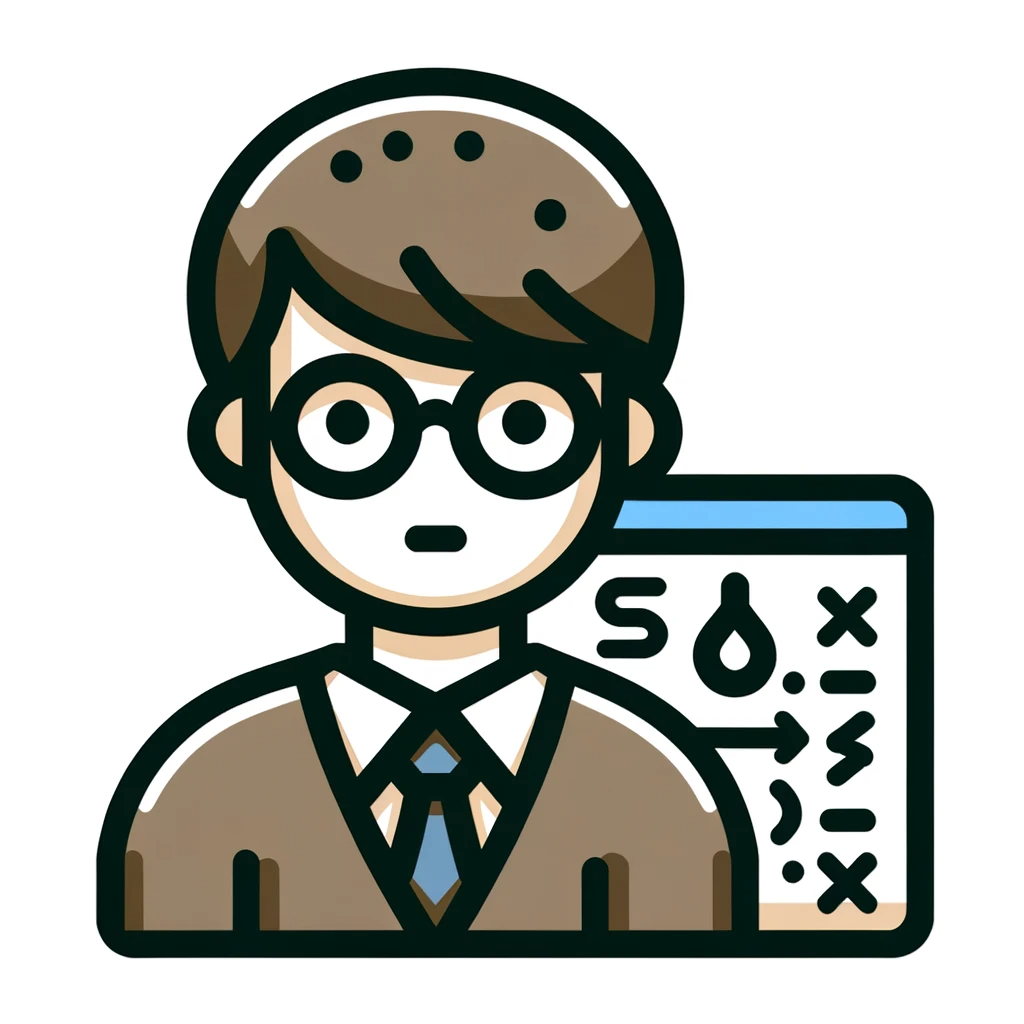
Asynchronous processing is an important part of JavaScript development.
Let’s learn how to use Promise.race!
Comments