This article explains ” How to execute multiple asynchronous processes in parallel ” using JavaScript.
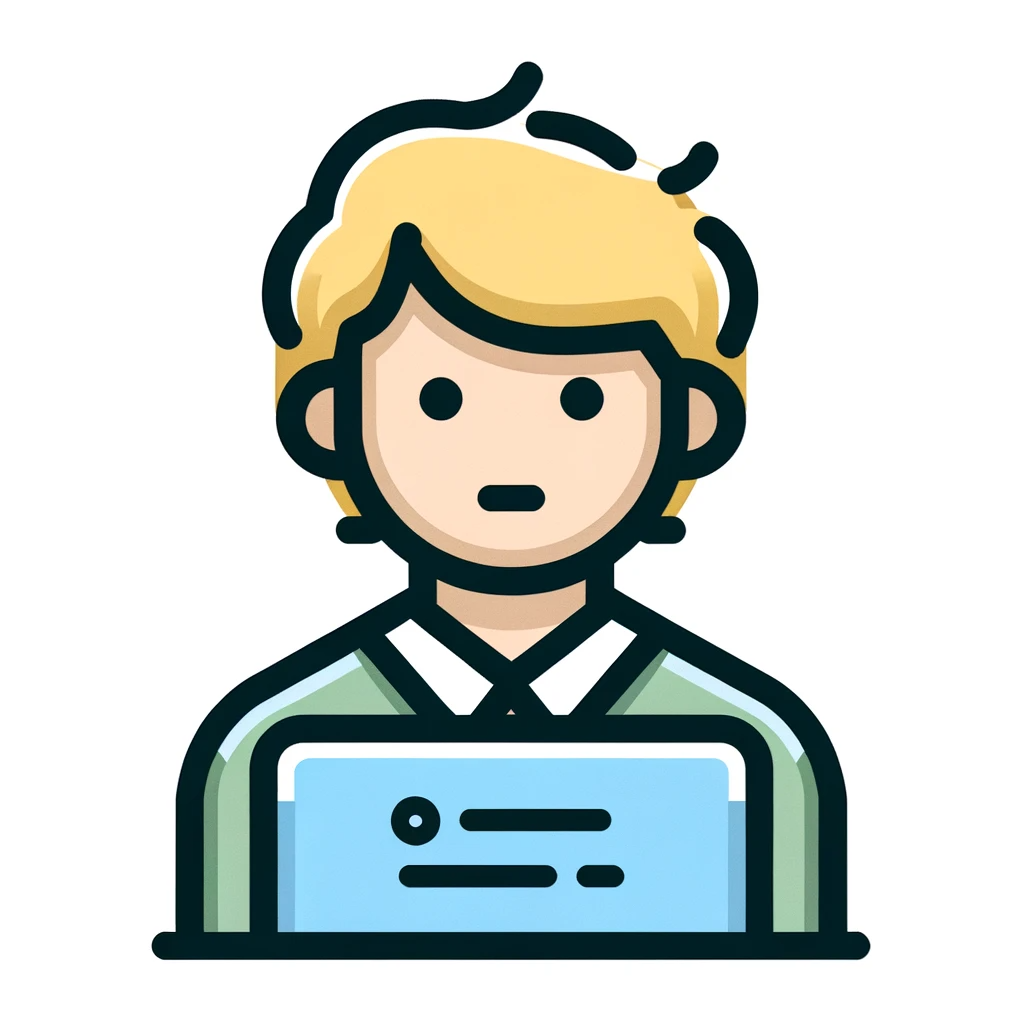
How can I run multiple asynchronous operations in parallel?
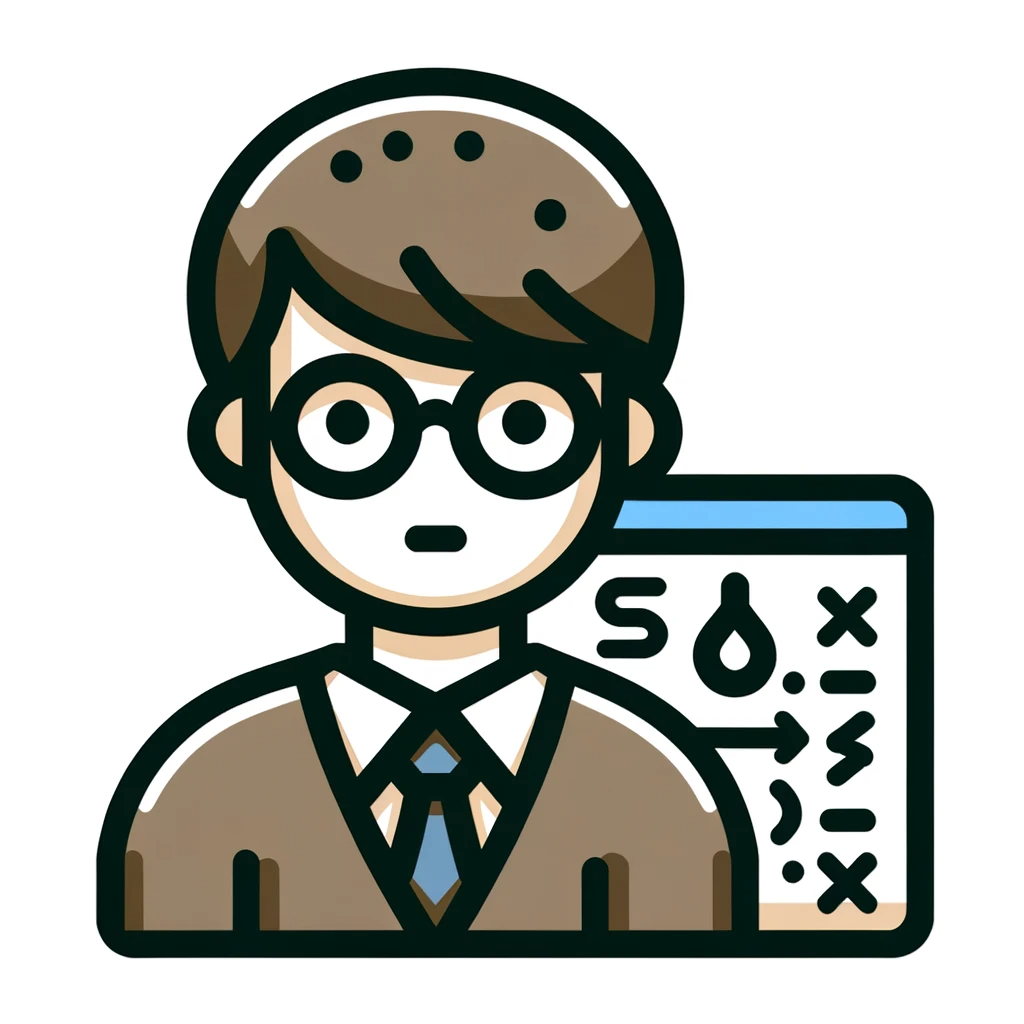
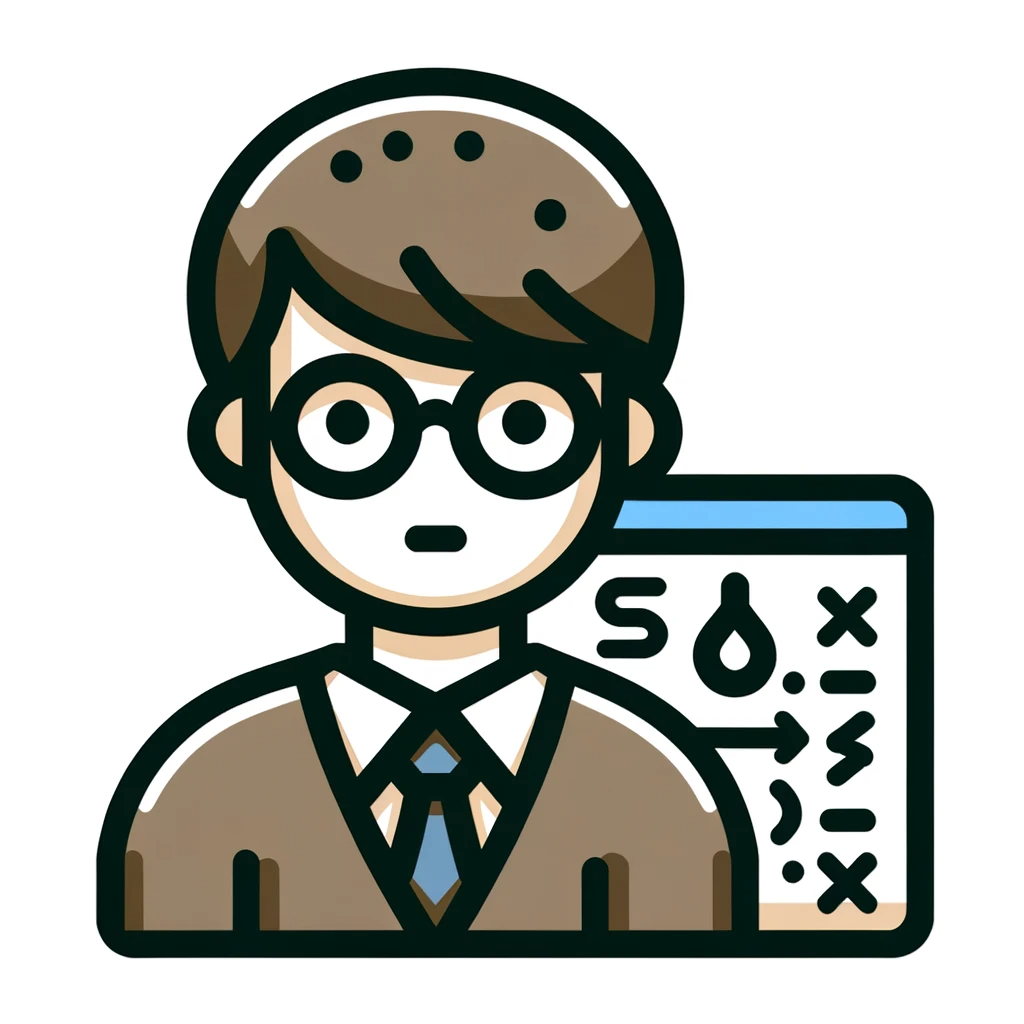
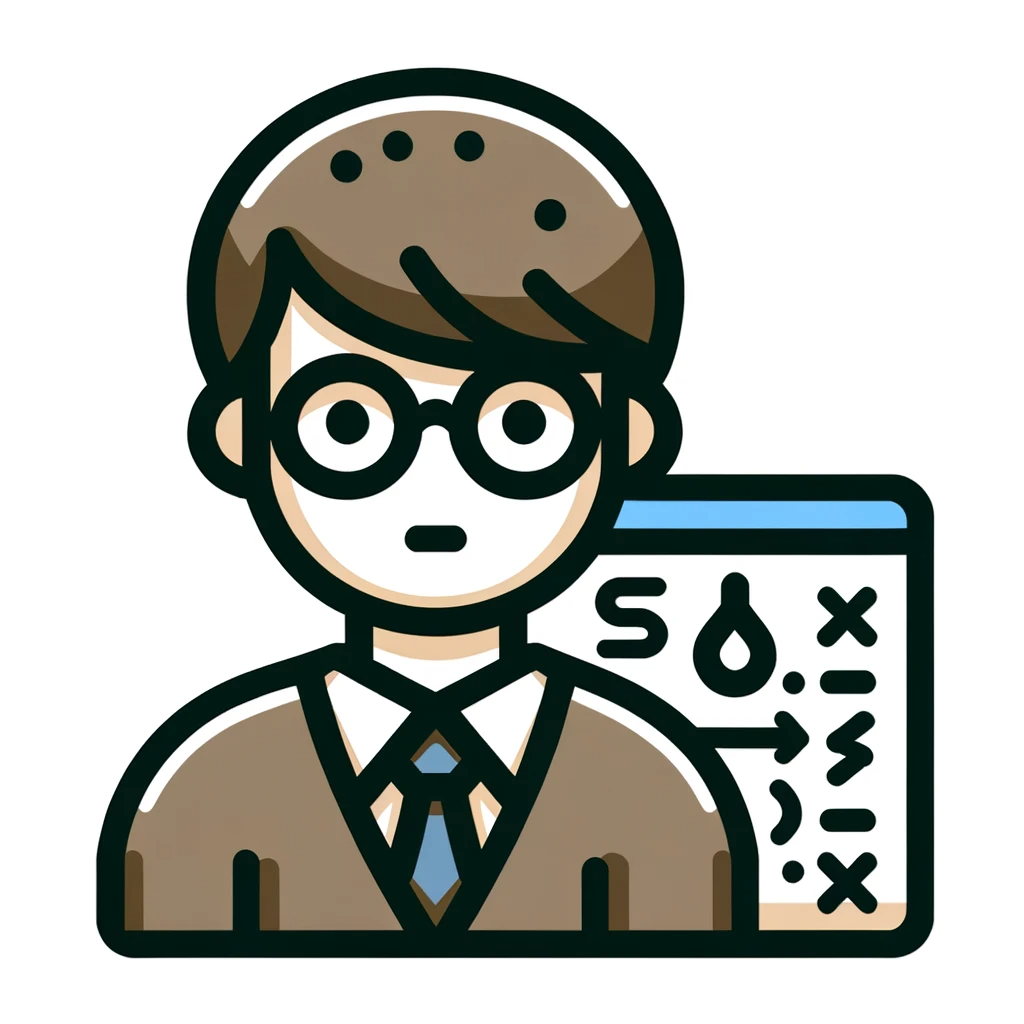
Promise.all allows you to execute multiple asynchronous operations in parallel.
“Promise.all” performs multiple asynchronous processes in parallel
The Promise.all method is a method for executing multiple asynchronous processes in parallel using Promise objects.
Pass an array containing Promise objects to perform asynchronous processing to Promise.all.
Promise.all (array of Promise objects to monitor)
Promise.all returns a Promise object that returns the result once all Promise objects stored in the array have been processed.
const promise1 = Promise.resolve('promise 1');
const promise2 = 10;
const promise3 = new Promise((resolve, reject) => {
setTimeout(resolve, 100, 'promise 3');
});
Promise.all([promise1, promise2, promise3]).then((values) => {
console.log(values);
});
In the above example, we are passing promise1, promise2, promise3 to Promise.all.
promise1 is resolved immediately, but promise3 is resolved after 100ms. Promise.all waits until all promises are resolved and returns the results in an array.
Summary of “How to execute multiple asynchronous processes in parallel”
“How to execute multiple asynchronous processes in parallel” is summarized below.
- Promise.all is a method for executing multiple asynchronous processes in parallel using Promise objects.
- Pass an array containing Promise objects that perform asynchronous processing.
- Promise.all returns a Promise object that returns the result once all Promise objects stored in the array have been processed.
Promise.all allows you to execute multiple asynchronous operations in parallel. You can improve application performance by running asynchronous operations in parallel.
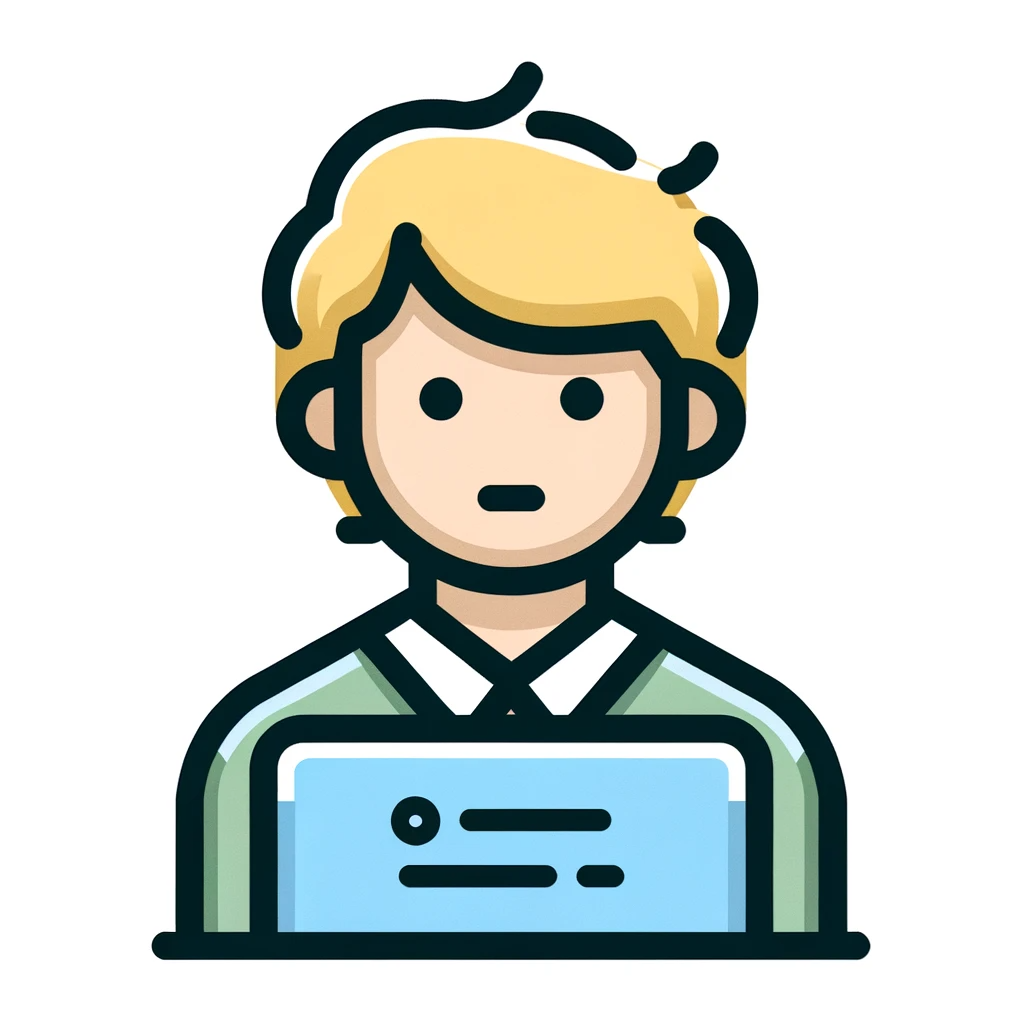
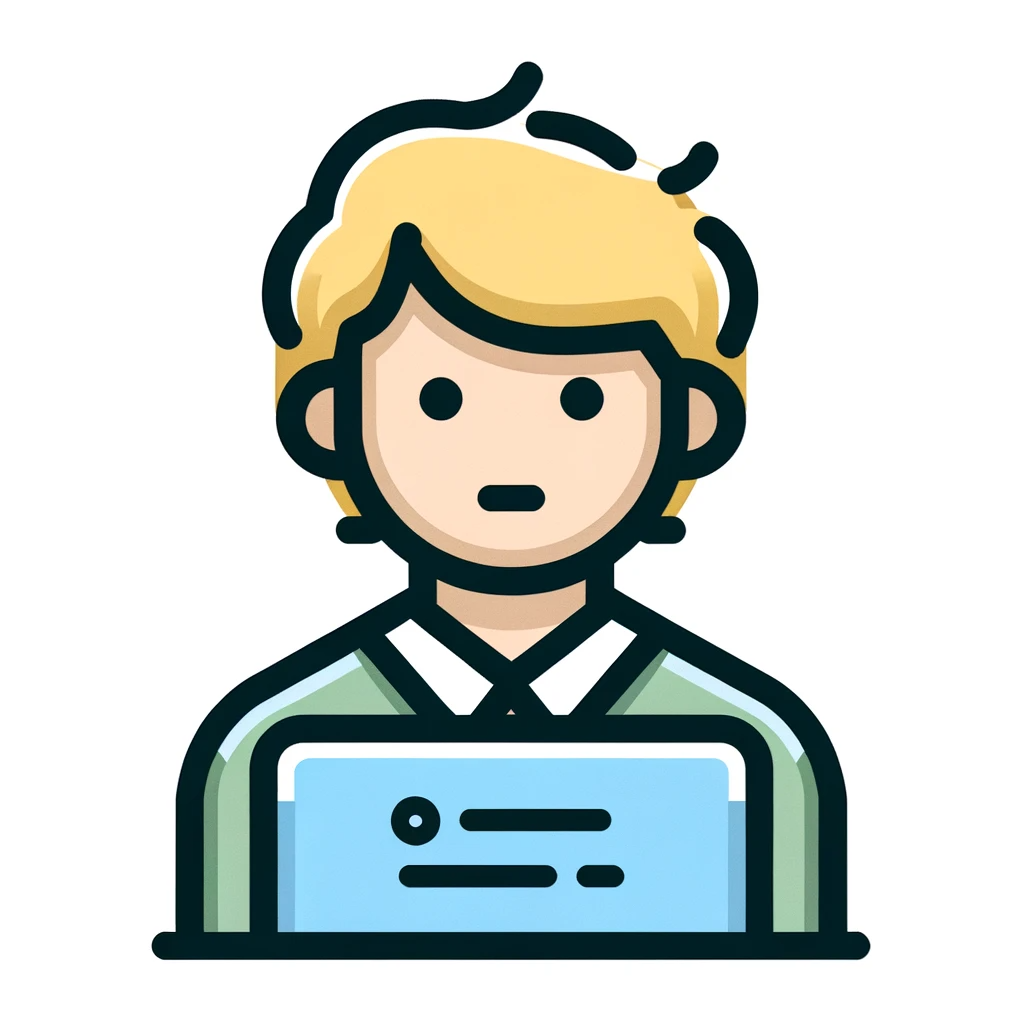
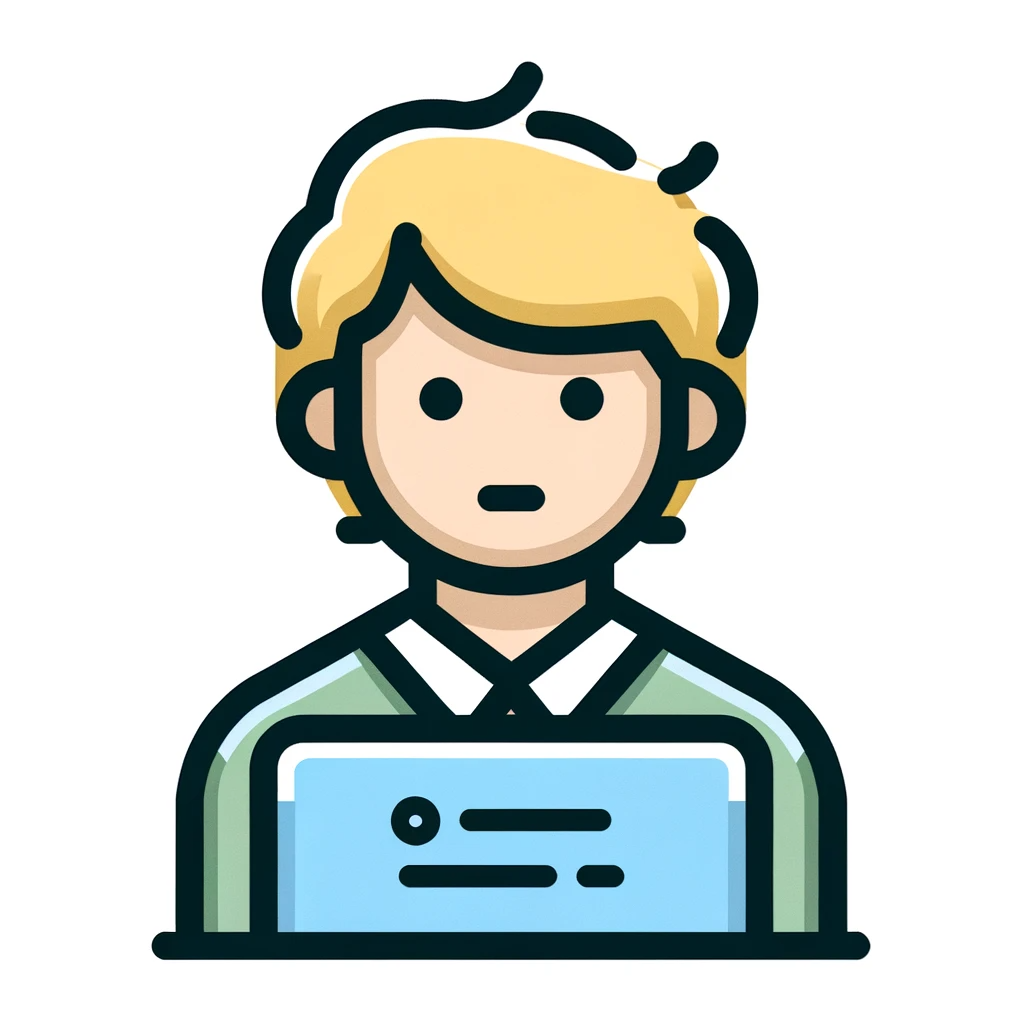
I found Promise.all to be very useful because it allows you to easily execute multiple asynchronous processes in parallel.
When using Promise.all, be careful as the results may change depending on the order in which asynchronous processing is resolved.
Comments