We will explain the steps using AJAX to send POST data on a website using JavaScript.
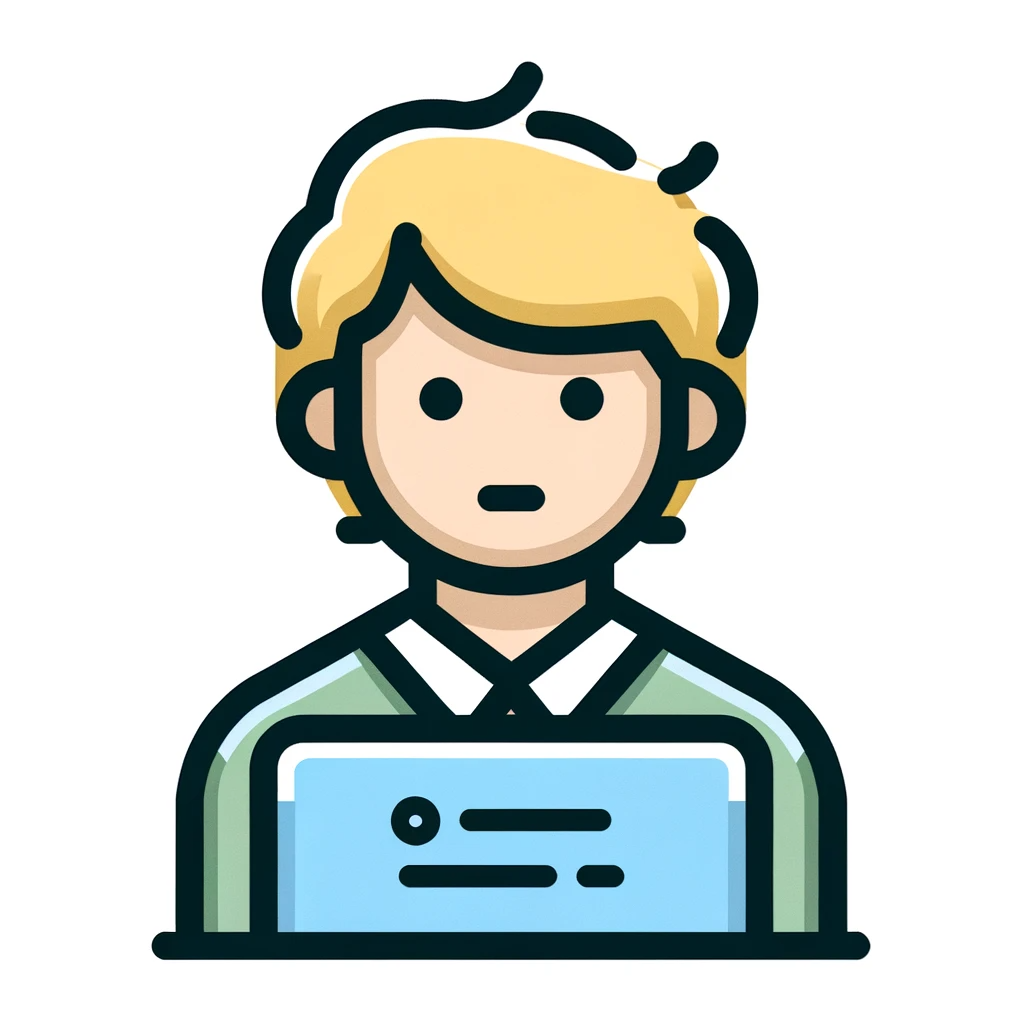
I don’t know how to send POST data with JavaScript, what should I do?
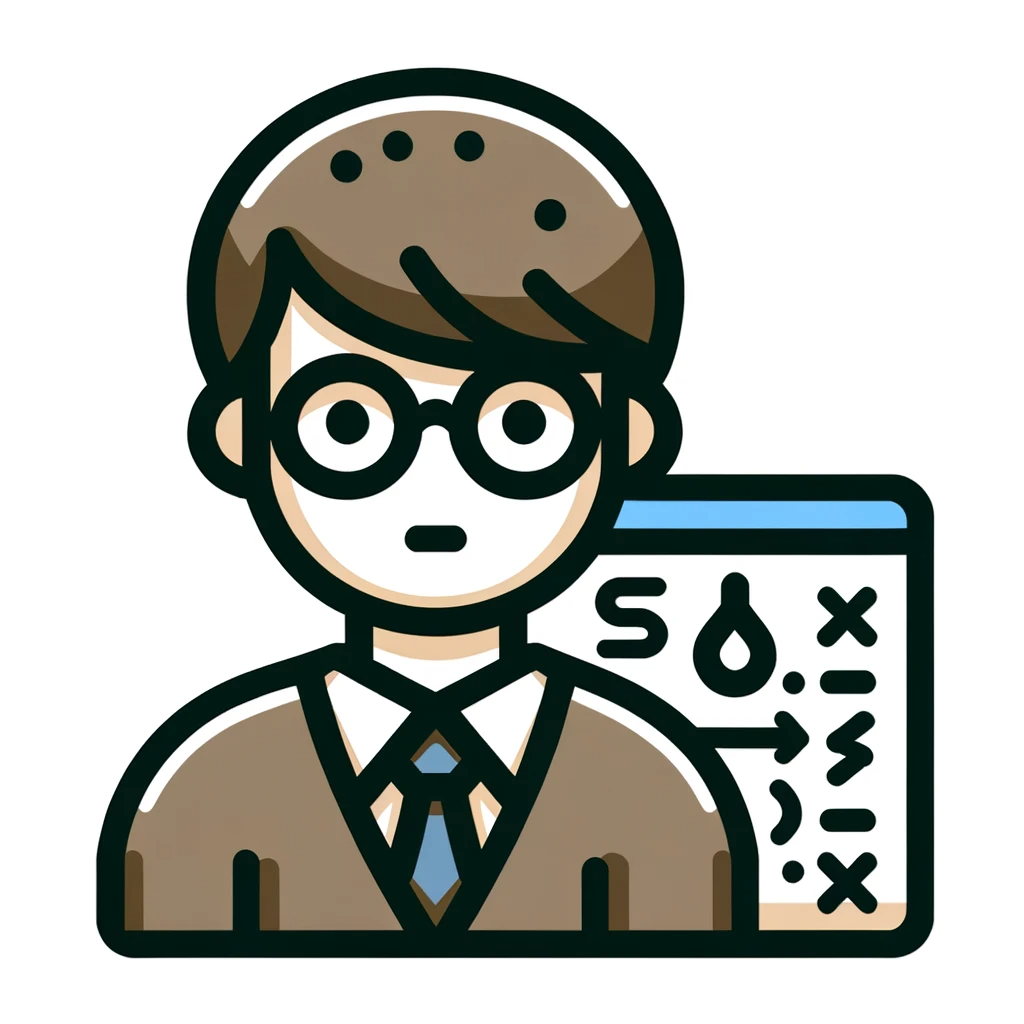
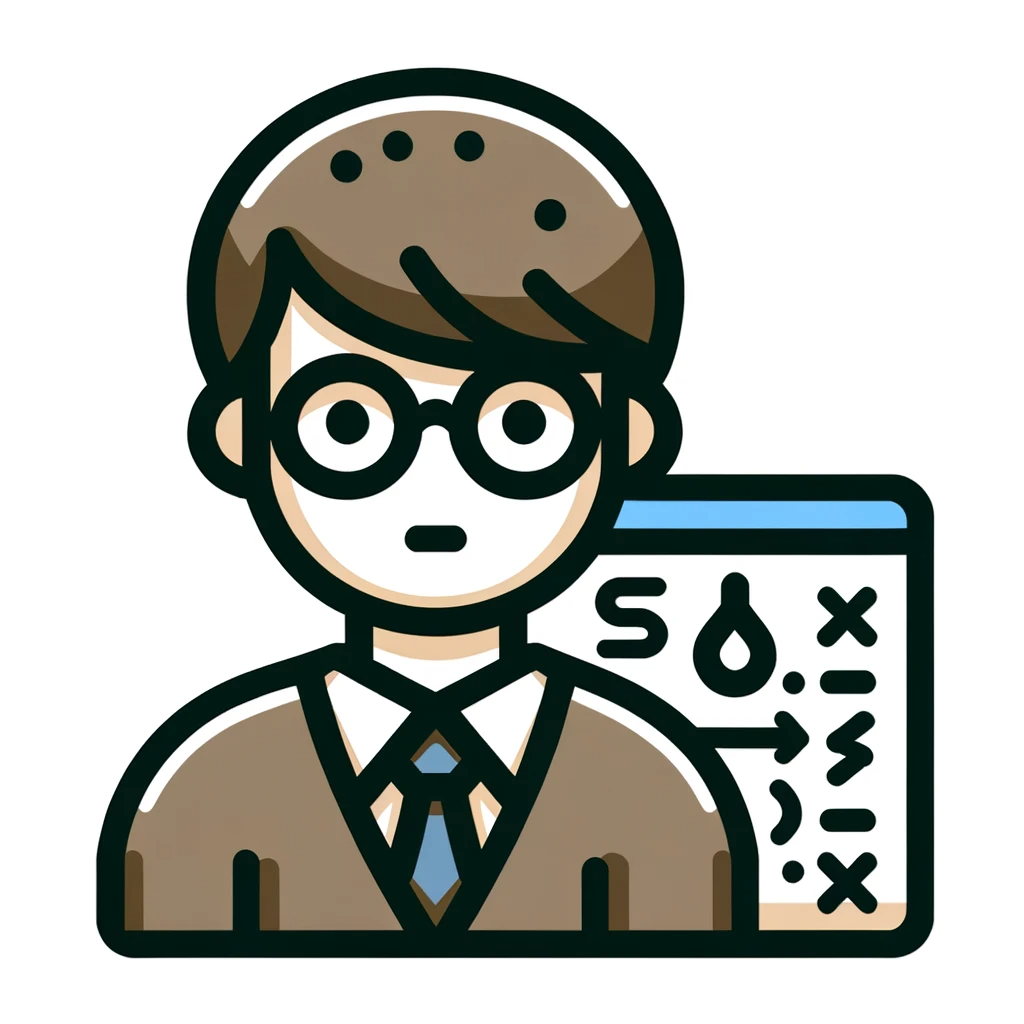
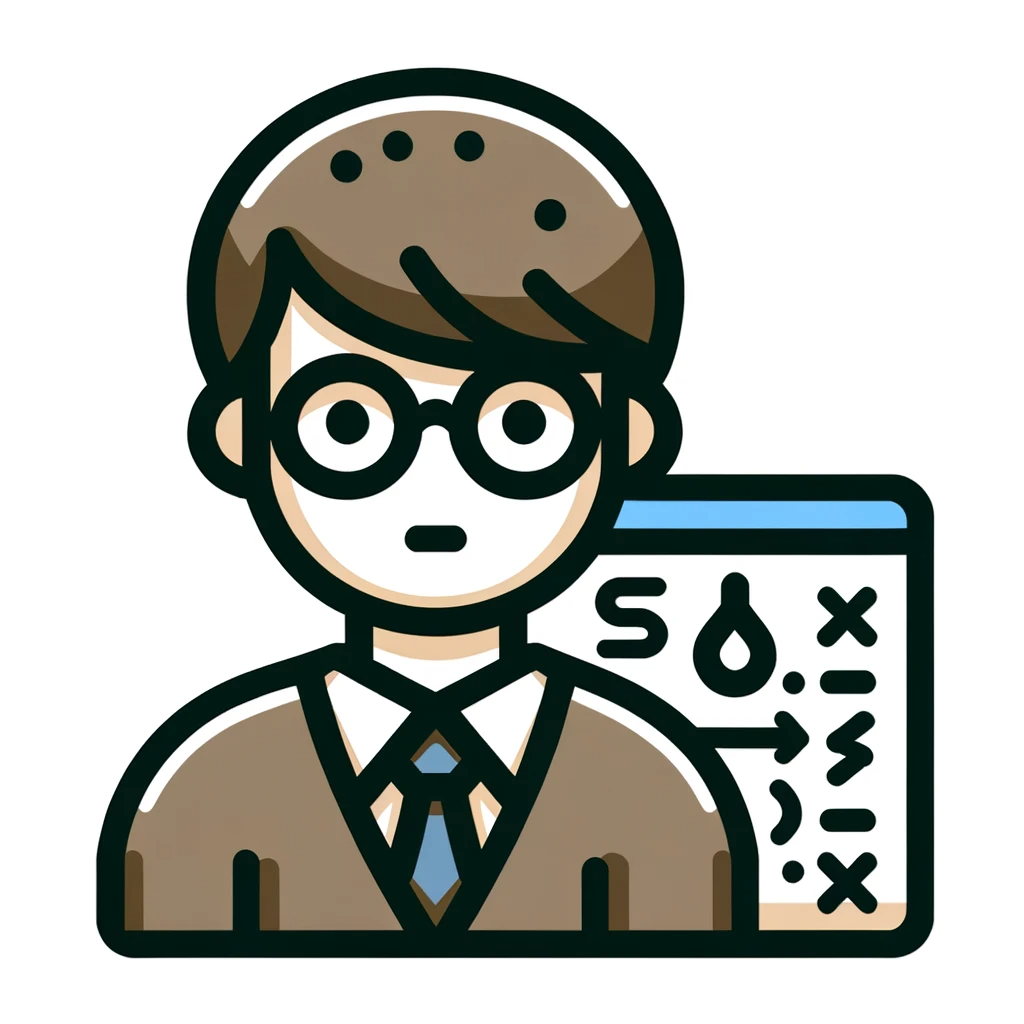
You can send POST data using AJAX.
Below, we will explain the steps in detail.
What is AJAX?
AJAX is a technology for asynchronous communication using JavaScript . Using this technology, you can communicate with the server and exchange data without reloading the web page. You can also receive data in JSON or XML format.
Procedure for sending POST data using AJAX
The steps to send POST data using AJAX are as follows.
- Create an XMLHttpRequest object
- Create data to send
- Start communication with the open() method
- Send data with send() method
- receive response
1. Create an XMLHttpRequest object
To perform AJAX communication, you need to create an XMLHttpRequest object .
Create an XMLHttpRequest object using the new operator as shown below.
const xhr = new XMLHttpRequest();
2. Create data to send
When sending data using the POST method, you need to create the data.
The following is an example of creating JSON format data.
const data = {
'key1': 'value1',
'key2': 'value2'
};
3. Start communication with open() method
After creating the XMLHttpRequest object, call the open() method to start communication .
The following is an example of starting communication using the open() method.
xhr.open('POST', 'Destination URL', true);
Specify the HTTP method in the first argument. In this case, we are specifying the POST method. Specify the destination URL in the second argument. The third argument specifies whether to use asynchronous communication. If true is specified, asynchronous communication will be performed.
Also, Content-Type must be set when sending data. This is to tell the server side the format of the data to send. Below is an example of setting Content-Type.
xhr.setRequestHeader('Content-Type', 'application/json');
In this example, Content-Type is set to application/json. If you want to send JSON format data, you need to set Content-Type like this. Additionally, when sending form data, you need to set the Content-Type such as application/x-www-form-urlencoded.
4. Send data using send() method
After creating the data and starting communication, call the send() method to send the data .
The following is an example of sending data using the send() method.
xhr.send(JSON.stringify(data));
The first argument specifies the data to send. In this case, we are specifying the JSON format data we created earlier that was converted to a string using the JSON.stringify() method.
5. Receive the response
To receive the response, monitor the onreadystatechange event of the XMLHttpRequest object .
The following is an example of receiving a response using the onreadystatechange event.
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
console.log(xhr.responseText);
}
}
Once the readyState property is 4 and the status property is 200, you can receive the response. The response data is stored in the responseText property. In the above example, the response data is displayed on the console.
Explanation using a sample program
Below is a sample code for sending POST data using AJAX. You can actually use this code to send POST data.
const xhr = new XMLHttpRequest();
const url = 'Destination URL';
const data = { 'key': 'value' };
xhr.open('POST', url, true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
console.log(xhr.responseText);
}
}
xhr.send(JSON.stringify(data));
First, we are creating an XMLHttpRequest object.
Next, set the destination URL and the data to send. In this example, URL specifies the actual destination URL. Also, the data to be sent is in JSON format, with a key name called key and a value called value.
const url = 'Destination URL';
const data = { 'key': 'value' };
Next, set up the XMLHttpRequest object.
To send using the POST method, specify POST as the first argument. Specify the destination URL in the second argument. The third argument specifies whether to perform asynchronous communication. If true is specified, asynchronous communication will be performed.
Next, set the Content-Type. In this example, Content-Type is set to application/json to send data in JSON format.
Next, configure settings to receive responses. Set the onreadystatechange event and receive the response data when readyState is 4 and status is 200. The received data is stored in the responseText property. This example outputs the response data to the console.
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
console.log(xhr.responseText);
}
Finally, send the data. Use the JSON.stringify() method to convert the data to a JSON format string, and send the data using the send() method.
summary
We explained the steps using AJAX to send POST data on a website using JavaScript.
- You can use AJAX to send POST data on a website using JavaScript.
- The sending procedure is to create an XMLHttpRequest object, create data, start communication, send data, and receive a response.
- When sending data, Content-Type must be set.
- For security reasons, the destination server must support CORS.
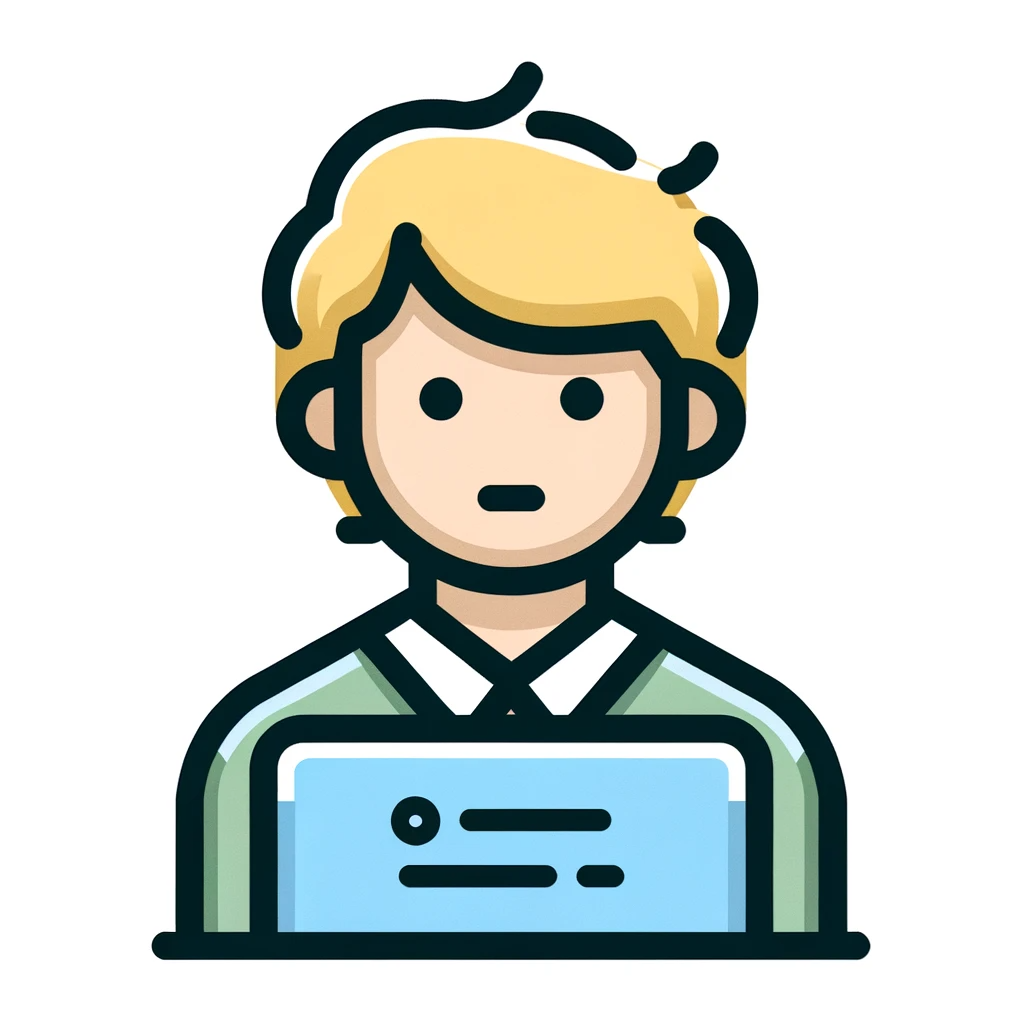
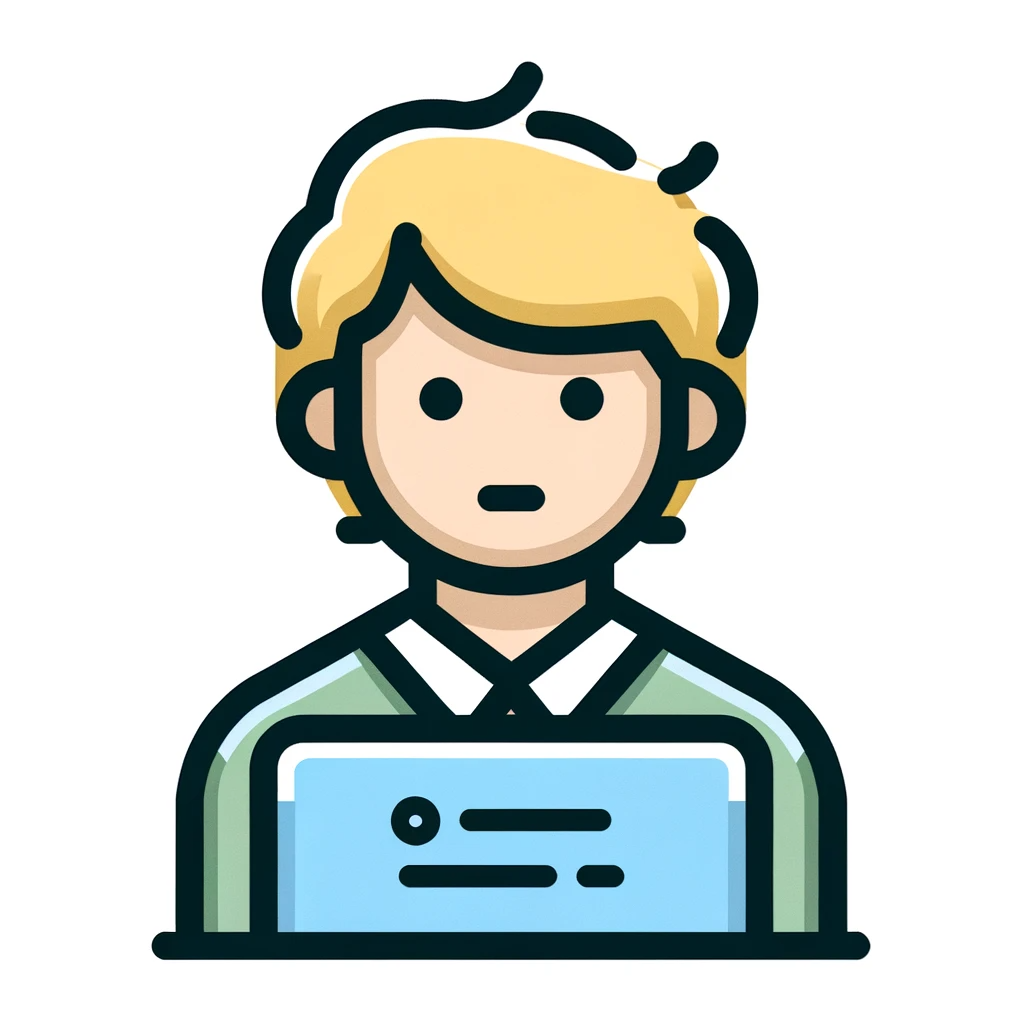
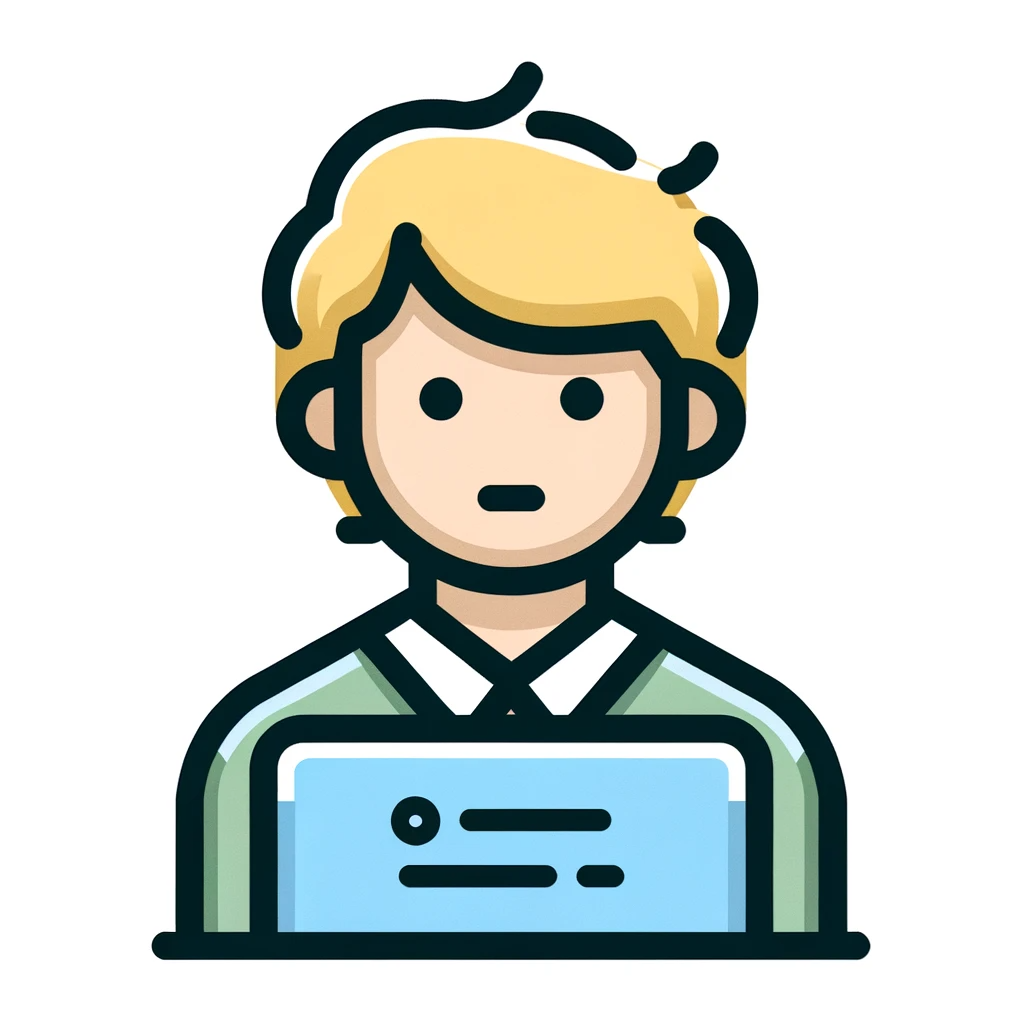
Now you know the steps to send POST data using AJAX.
I would like to actually use it.
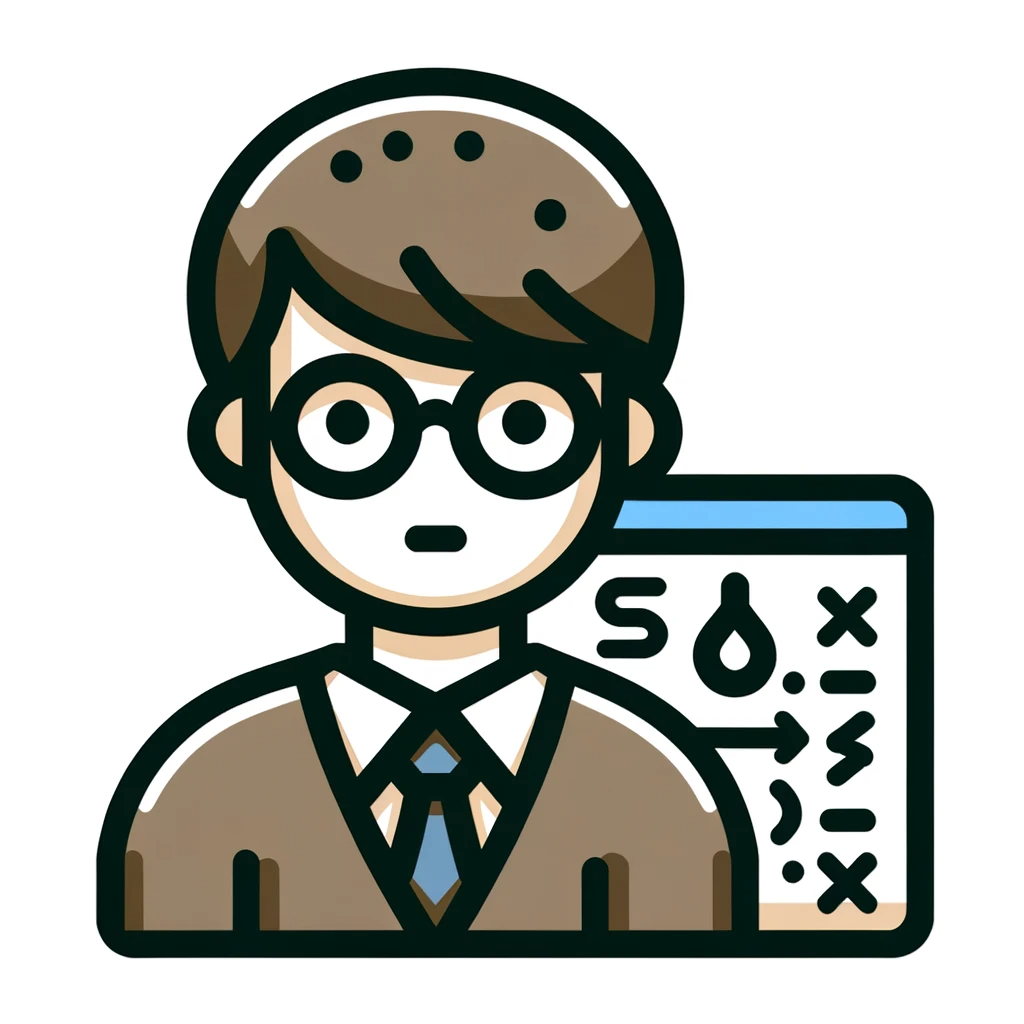
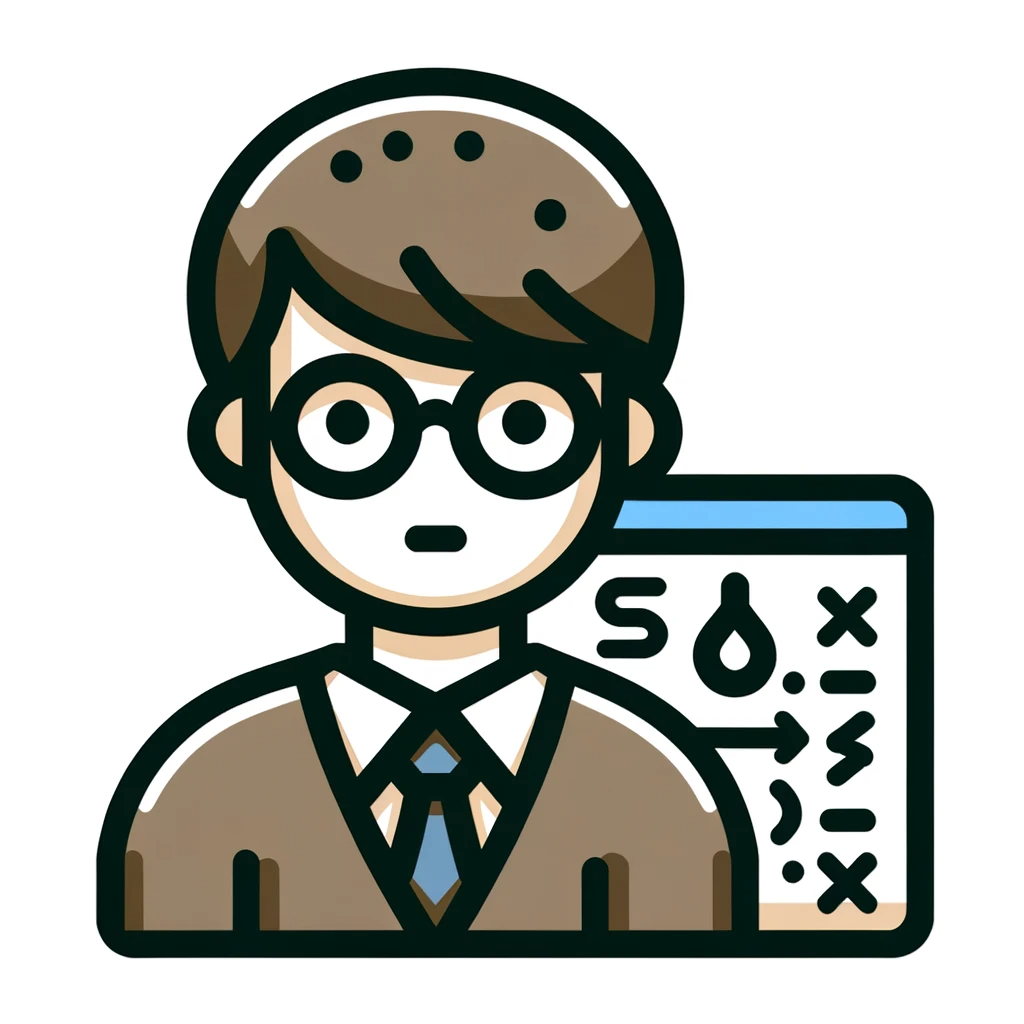
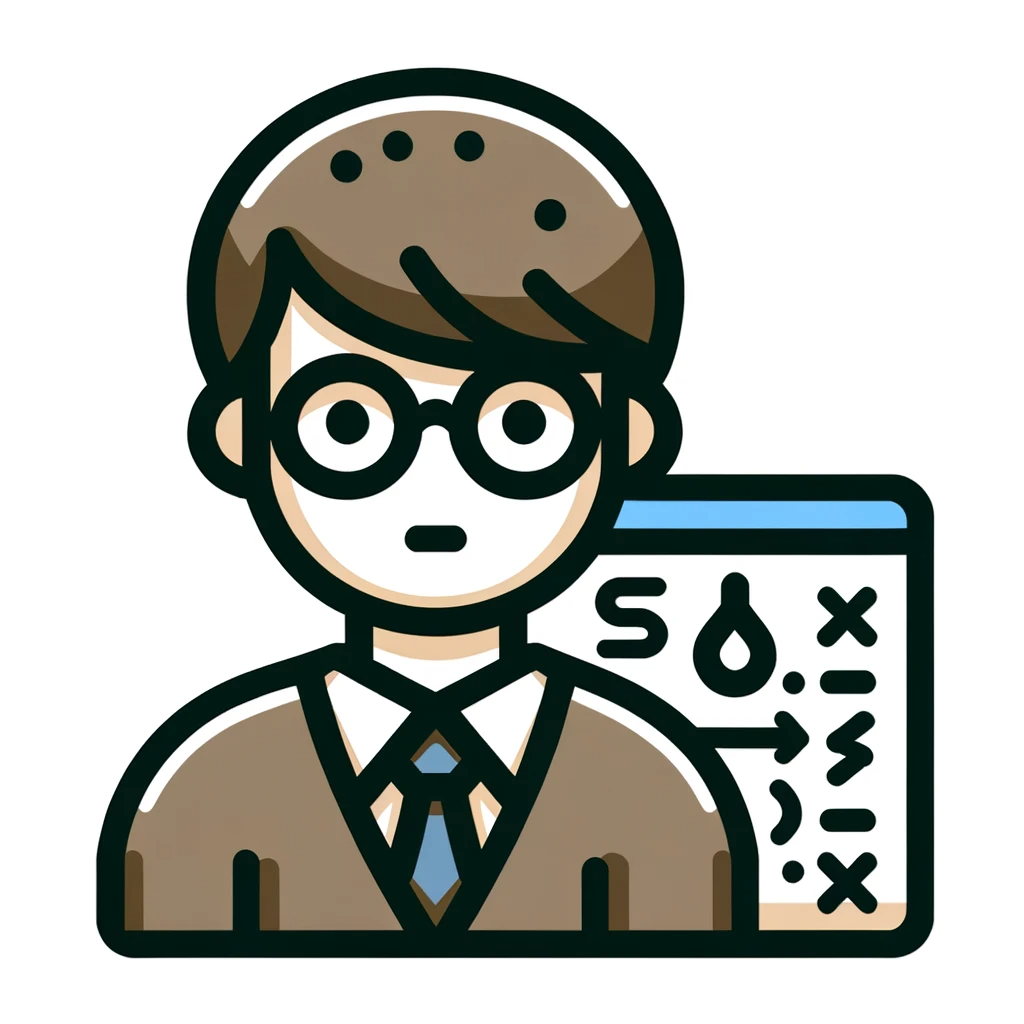
Sending POST data using AJAX is an important technique that allows you to send and receive data on a website using JavaScript.
Additionally, it can be easily implemented using frameworks and libraries.
Always incorporate the latest technology and keep security in mind as you work on web development.
Comments