This article explains in detail how to override base class methods in JavaScript.
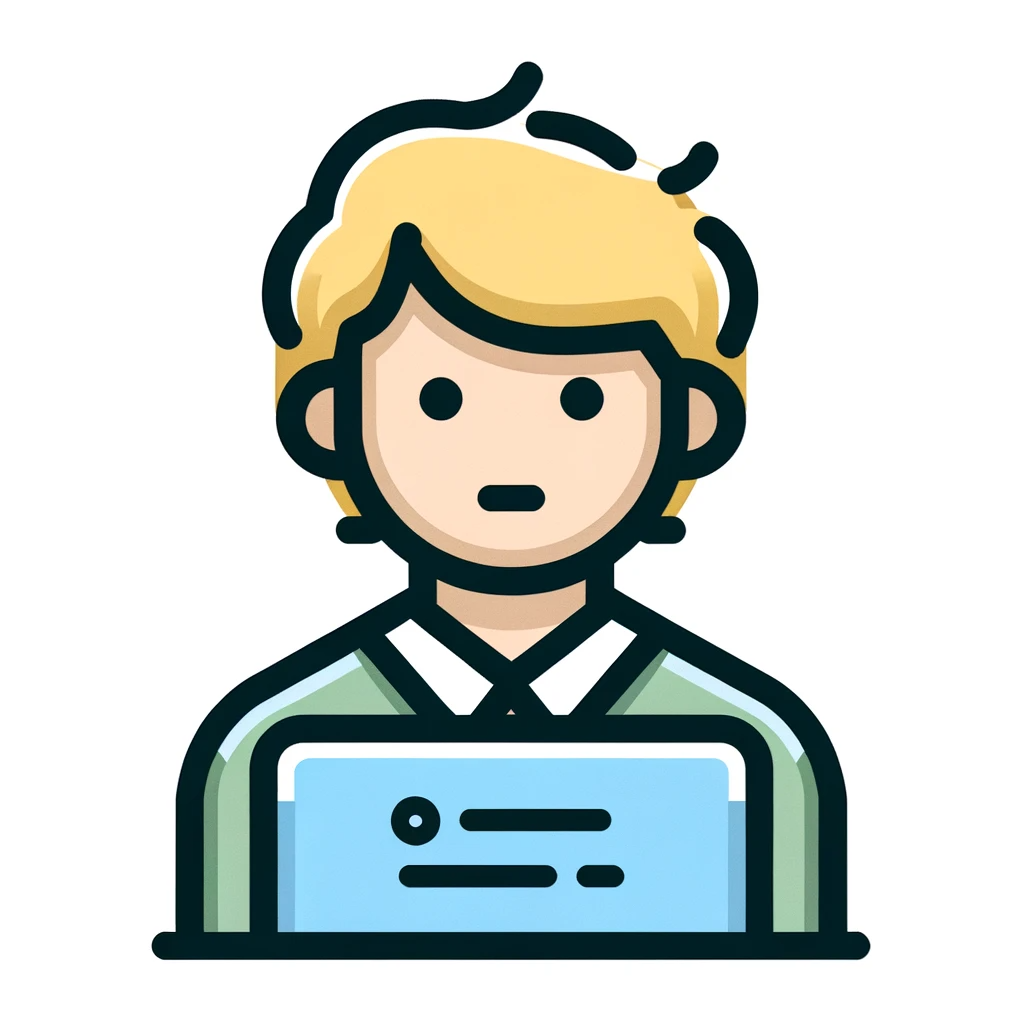
How can I override a base class method?
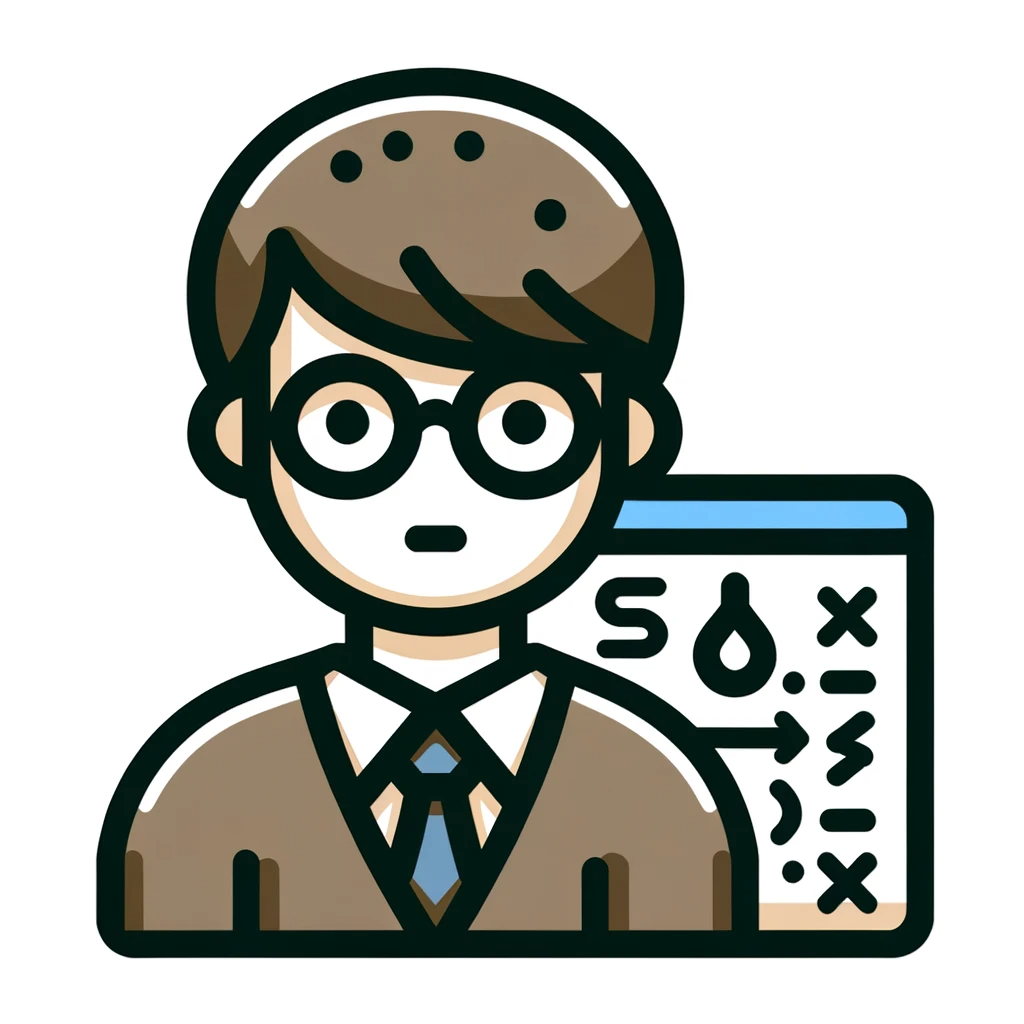
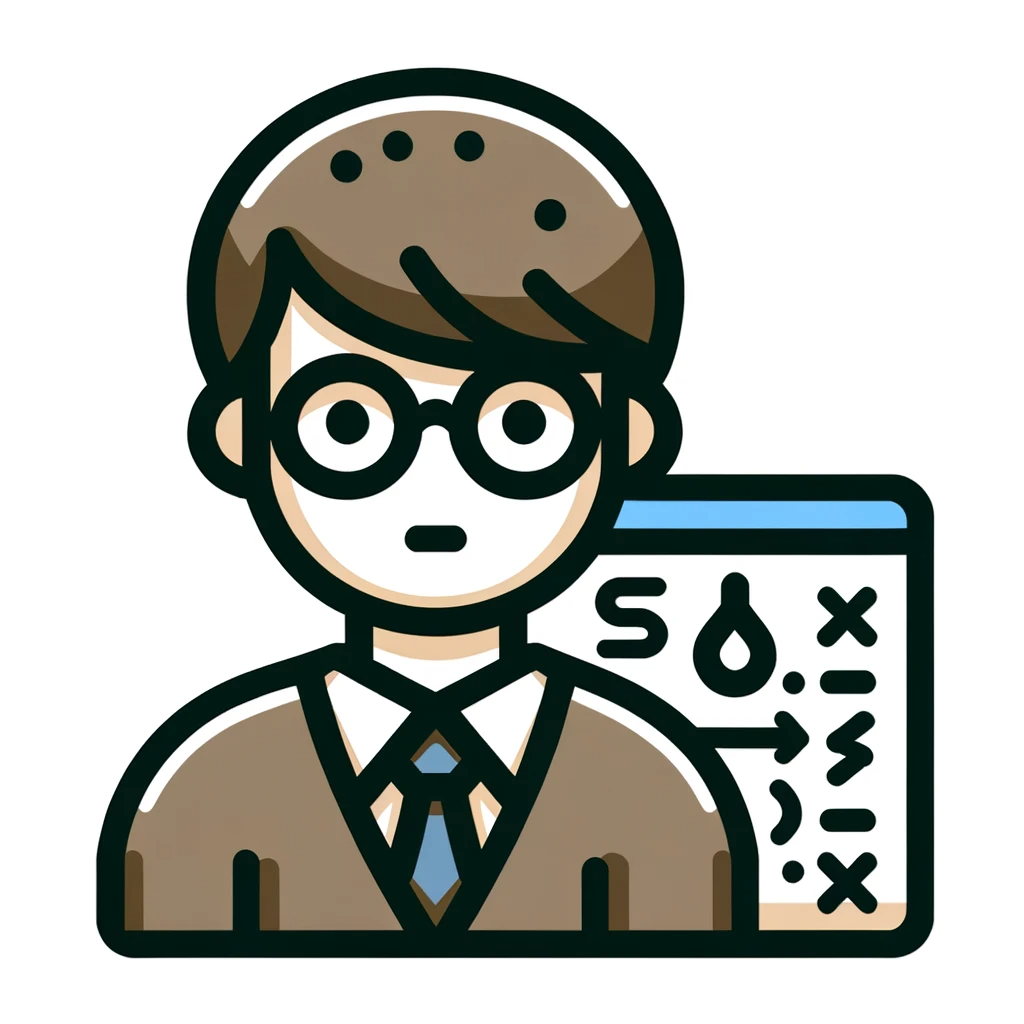
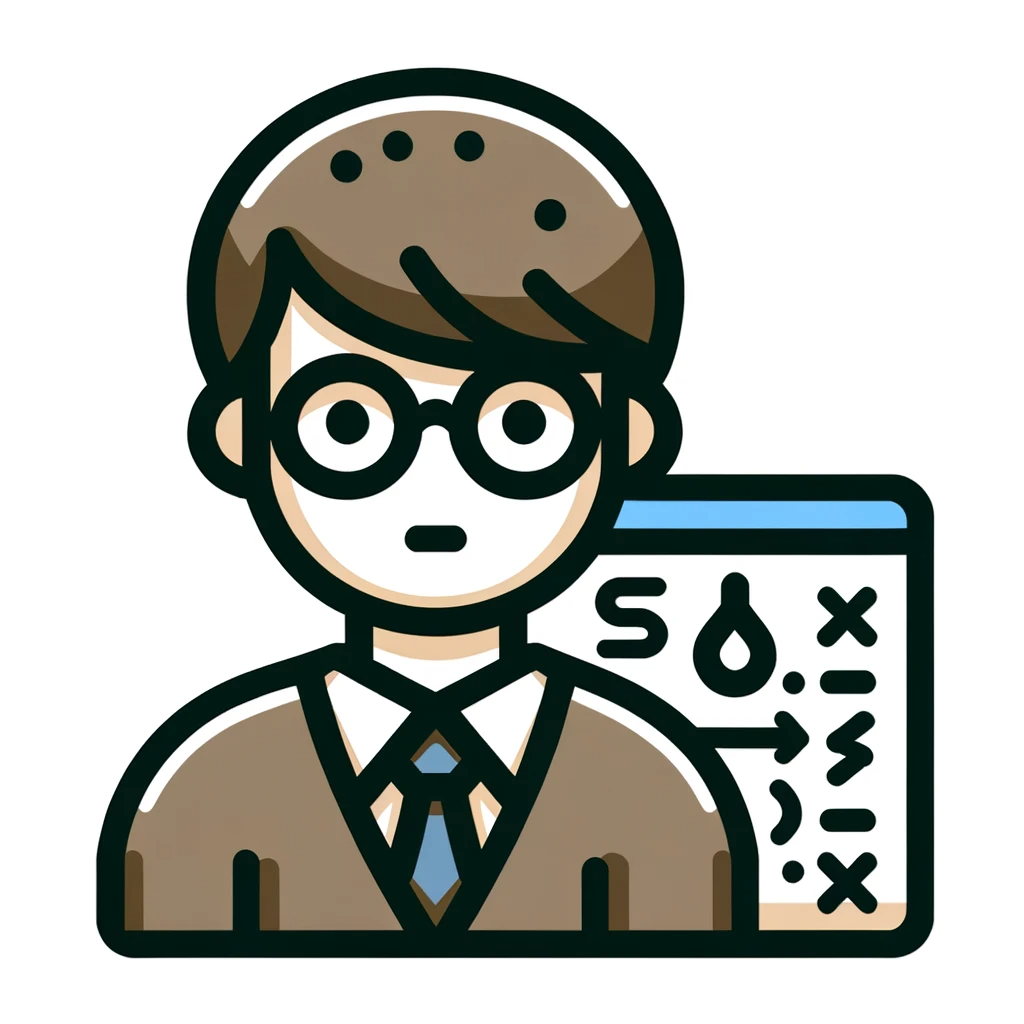
Use overrides to override base class methods.
How to call a superclass method from a subclass
super
Use keywords to call methods of a superclass from a subclass . This super
keyword is for calling methods of the parent class.
For example, I have a class like this:
class Animal {
move() {
console.log("Motion.");
}
}
class Dog extends Animal {
move() {
console.log("Running.");
}
}
Here, Dog
the class Animal
inherits from the class. Also, Dog
the class is move
overriding methods.
How to implement overrides
The function that allows a subclass to override a superclass method is called “override.”
Overriding is accomplished by defining a method with the same name in a subclass.
For example, I have a class like this:
class Animal {
move() {
console.log("Motion.");
}
}
Here, the Animal class has a move method defined. This move method can be overridden by subclasses such as the following.
class Dog extends Animal {
move() {
console.log("Running.");
}
}
Here, the Dog class inherits from the Animal class. The Dog class also defines a move method. This move method can define its own processing by overriding the move method of the Animal class.
In this case, to call the move method of the Animal class from the Dog class, do the followingmove
class Dog extends Animal {
move() {
super.move();
console.log("Running.");
}
}
Thus, by writing super.move(), the move method of the Animal class is called.
While overriding is called overriding, it is rare to completely rewrite the functionality of the base class, and it is common to use it in such a way that the functionality of the base class is diverted and the difference functionality is added, as shown above.
The above is an explanation of how to implement overrides in JavaScript.
About property overrides
In JavaScript, properties cannot be directly overridden.
Alternatively, a subclass can define a new property that overwrites a property with the same name , but it cannot overwrite the inherited property. You can also directly reference inherited properties.
Below is a sample code that overwrites the inherited property by defining a new property in the subclass.
class Animal {
constructor(name, type) {
this.name = name;
this.type = type;
}
}
class Dog extends Animal {
constructor(name) {
super(name, "dog");
}
}
const dog = new Dog("Pochi");
console.log(dog.name); // Pochi
console.log(dog.type); // dog
In this sample, two properties, name and type, are defined in the Animal class. Next, the Dog class inherits from the Animal class and newly defines the name property.
This also allows the type property to be referenced from instances of the Dog class.
Summary of this article
We explained how to override base class methods.
- The function that allows a subclass to override a superclass method is called “override.”
- Use the super keyword to invoke a method of a superclass from a subclass.
- Overriding is achieved by defining a method with the same name in a subclass.
- Properties cannot be overridden directly. Instead, you can override a property with the same name by defining a new property in your subclass.
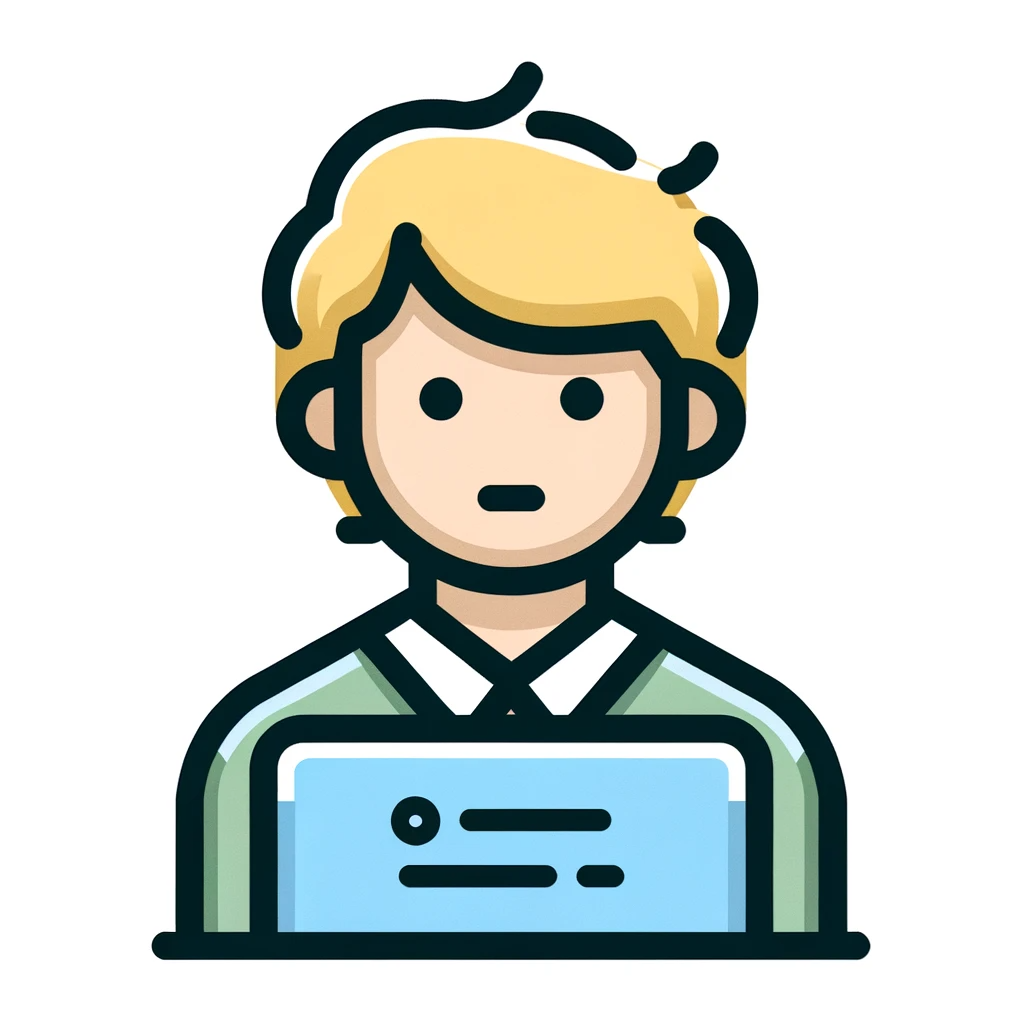
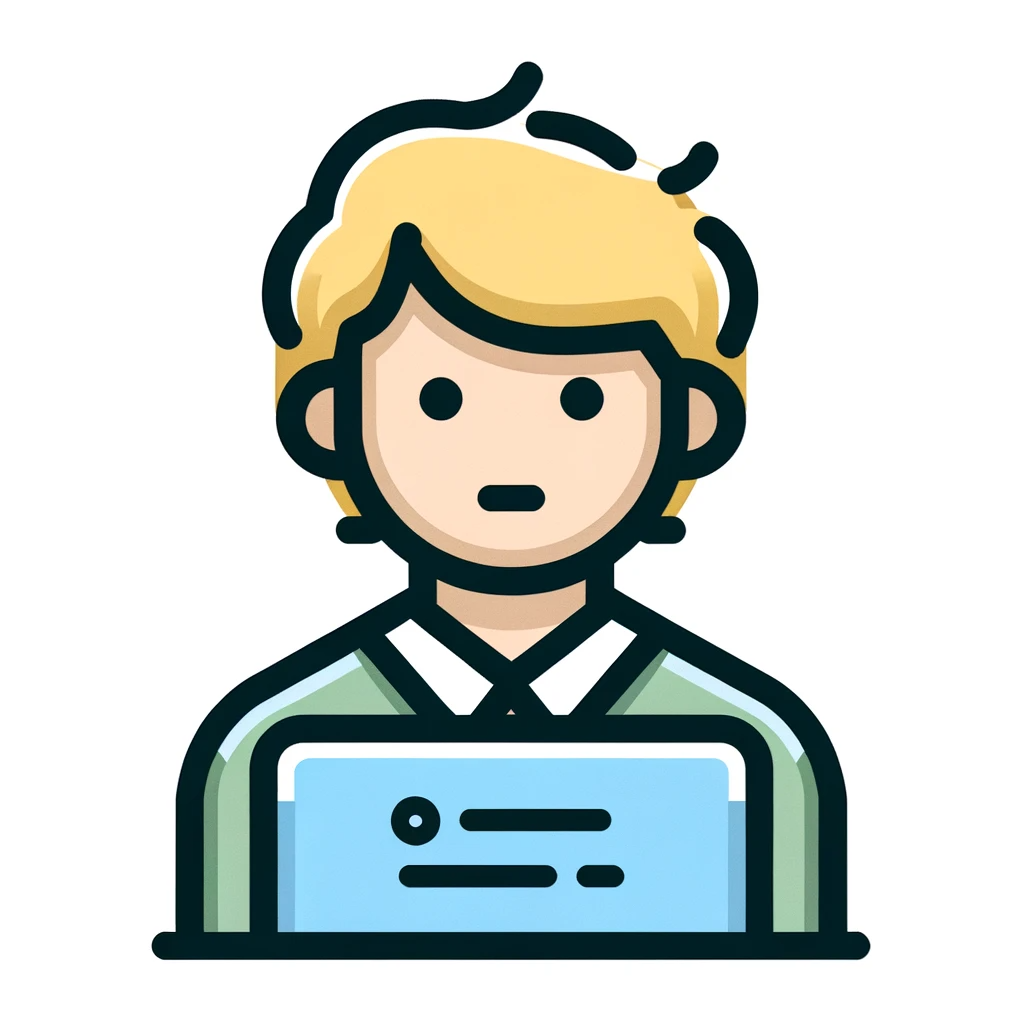
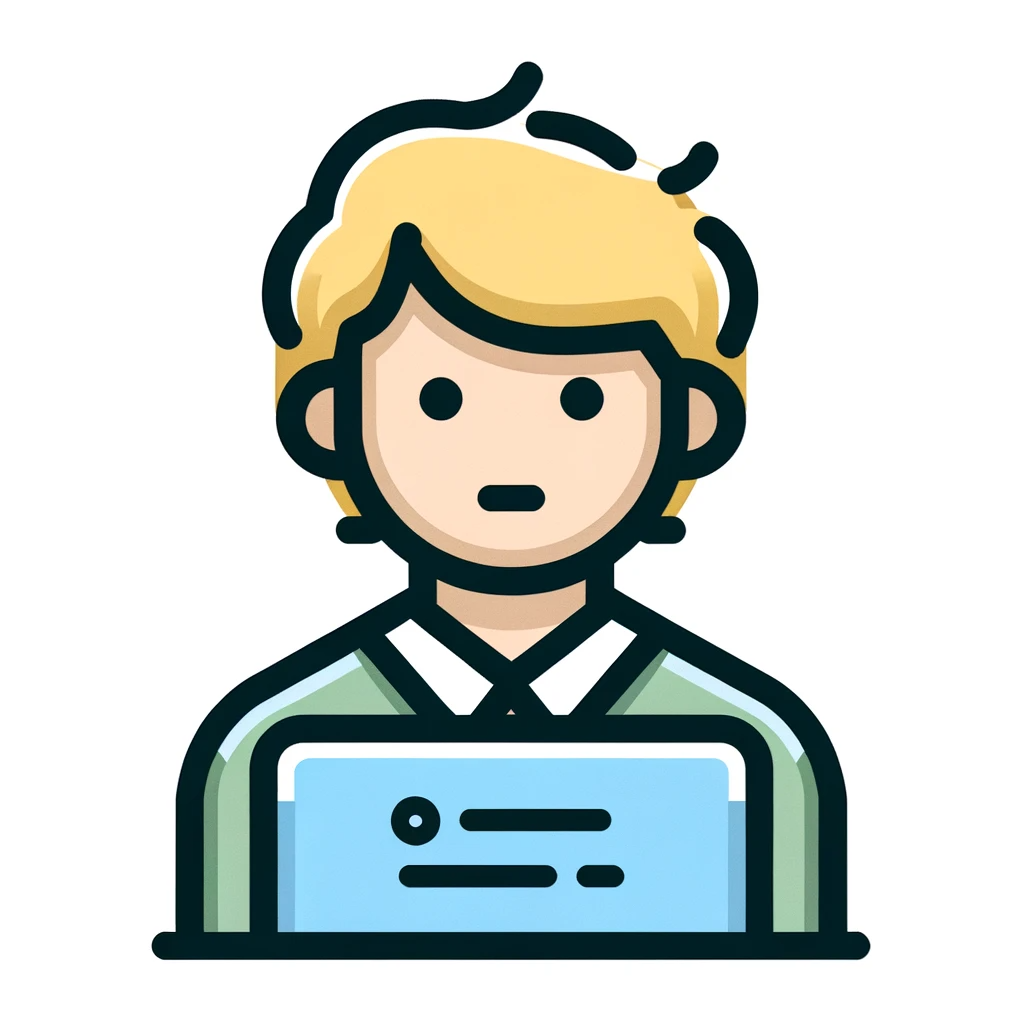
The override feature seems very useful for overriding inherited methods.
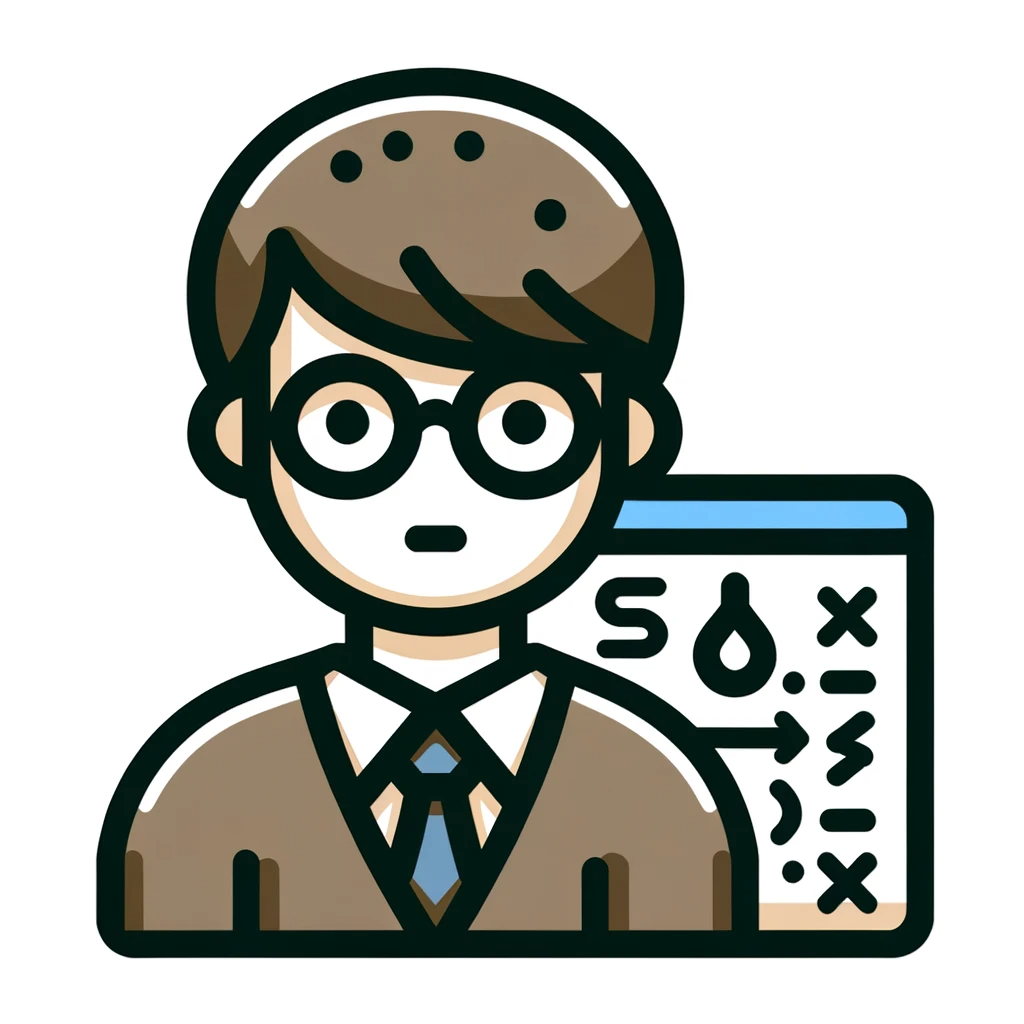
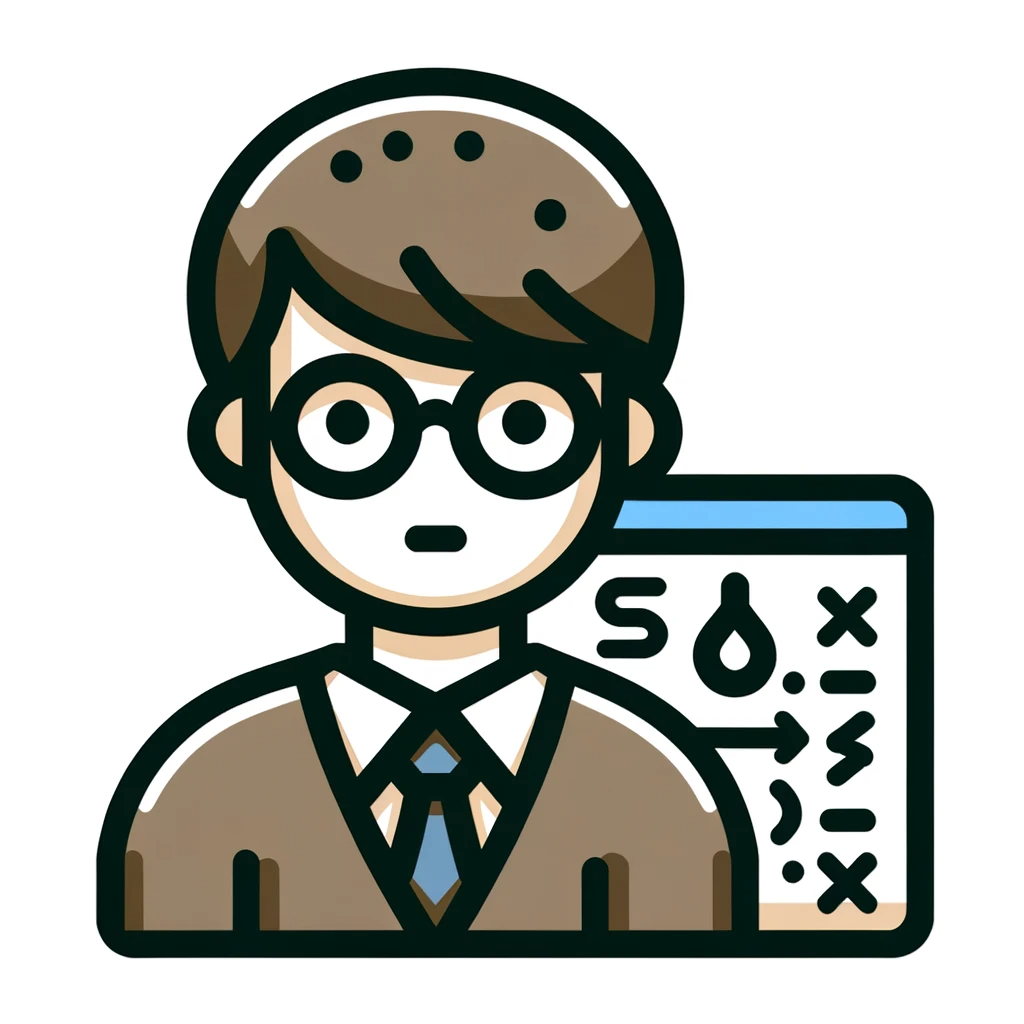
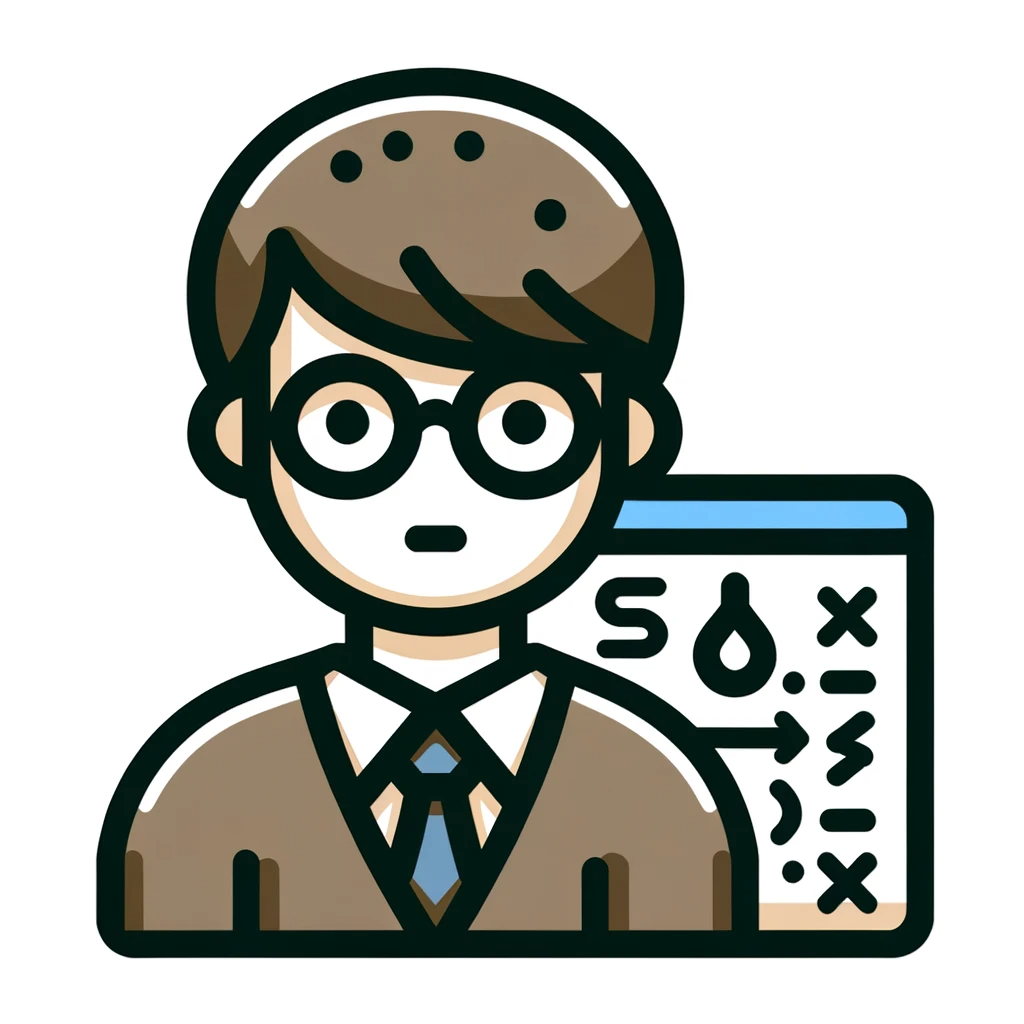
Overriding is a very important feature in JavaScript.
This feature allows you to override the inherited method.
You can also use overrides to achieve more complex processing.
Comments