This article explains how to get and set the values of a multi-selection list box using JavaScript.
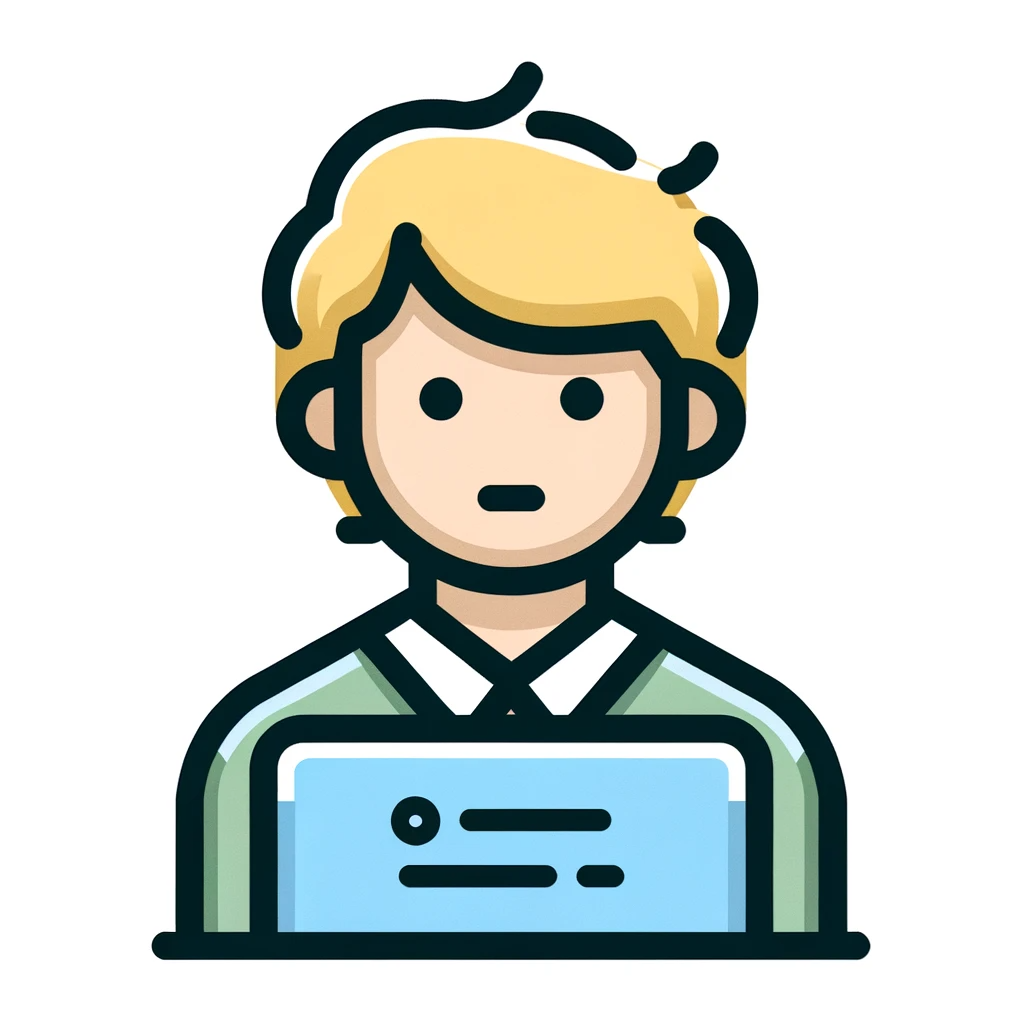
Can anyone tell me how to work with a multiple selection listbox?
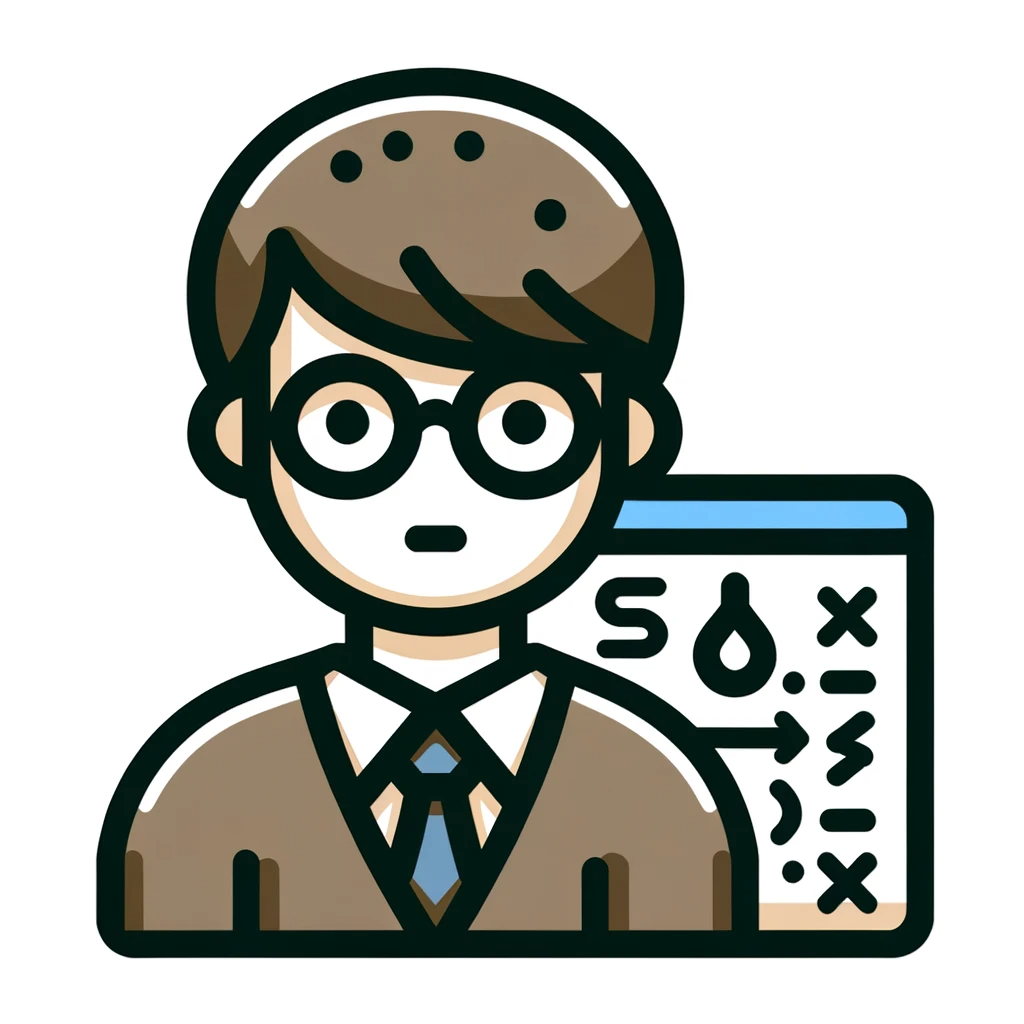
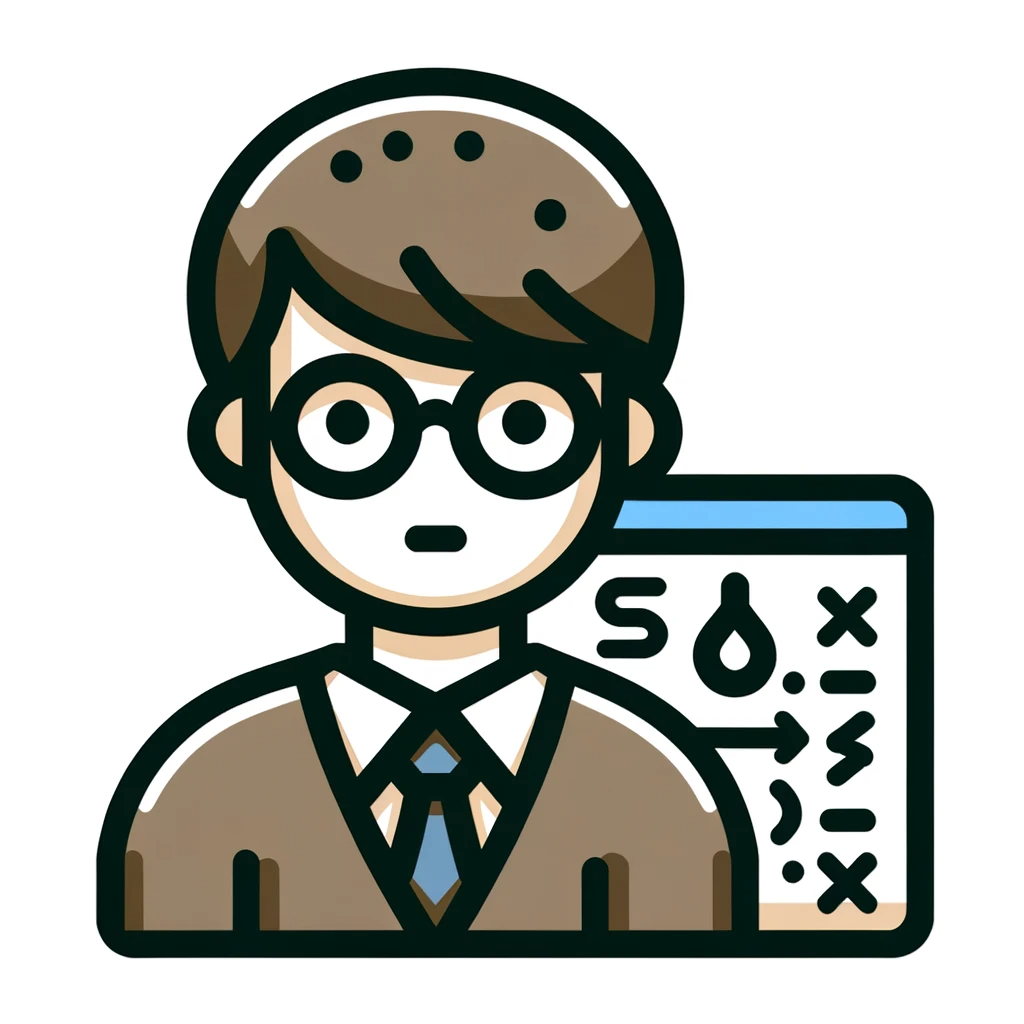
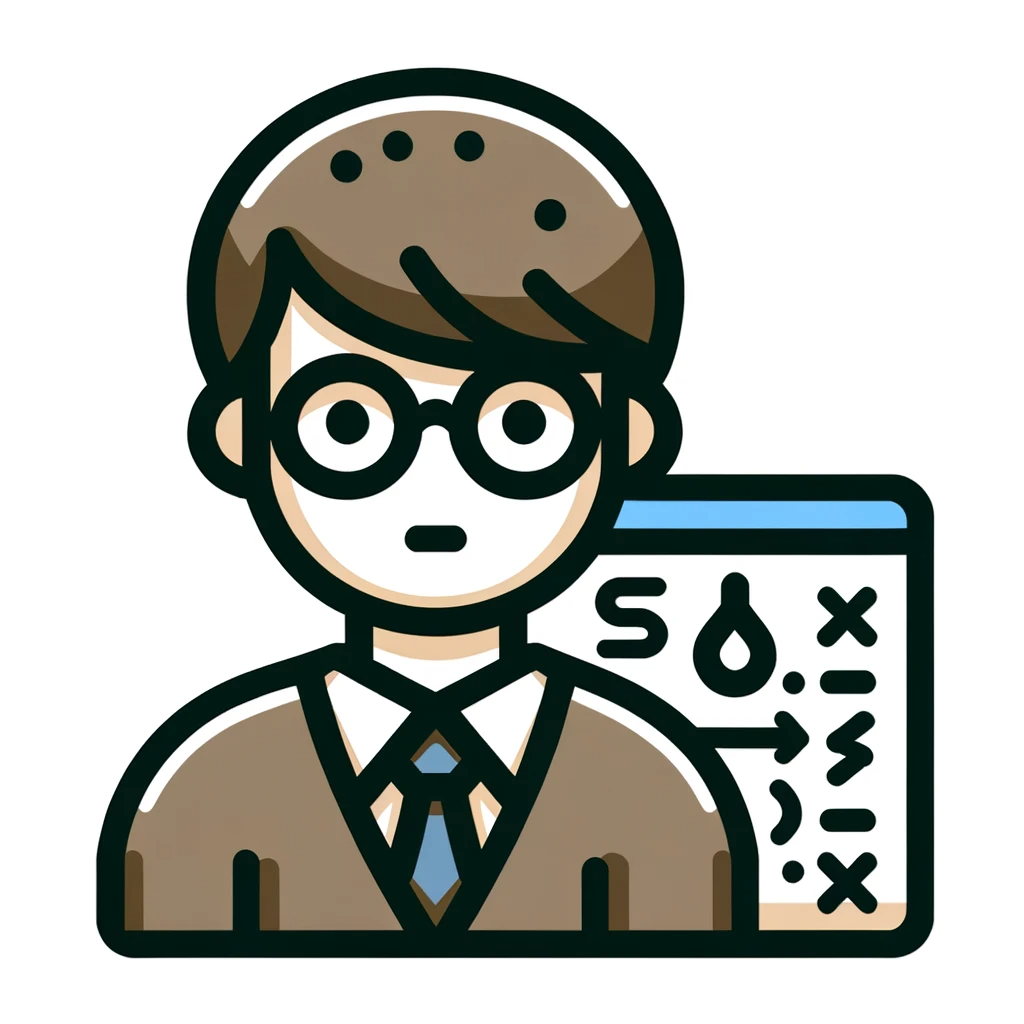
This section explains how to get and set values from a multiple selection list box.
How to get the value of a multi-select listbox
There are several ways to get values from a multi-selection list box:
How to get selected items one by one
A for loop is commonly used to retrieve selected items one by one from a multi-selection list box.
Within a for loop, you can retrieve the selected item by retrieving the value property of the item whose selected property is true .
Below is a sample program that shows how to retrieve selected items one by one from a multi-selection list box.
<select multiple id="mySelect">
<option value="value1">item1</option>
<option value="value2">item2</option>
<option value="value3">item3</option>
<option value="value4">item4</option>
</select>
<script>
const select = document.querySelector("#mySelect");
const options = select.options;
for (let i = 0; i < options.length; i++) {
if (options[i].selected) {
console.log(options[i].value);
}
}
</script>
How to get all selected items
To get all selected items from a multi-select list box, you can use the querySelectorAll method.
You can retrieve all selected items by specifying the “:checked” selector in the querySelectorAll method .
Below is a sample program of how to get all selected items from a multi-select listbox.
<select multiple id="mySelect">
<option value="value1">item1</option>
<option value="value2">item2</option>
<option value="value3">item3</option>
<option value="value4">item4</option>
</select>
<script>
const selectedOptions = document.querySelectorAll("#mySelect option:checked");
for (const option of selectedOptions) {
console.log(option.value);
}
</script>
How to set the value of a multi-selection list box
There are two ways to set the value of a multi-selection list box: using the HTML DOM’s “selected” property, and using JavaScript’s “for” statement.
To use the “selected” property, write as follows.
document.getElementById("List box ID").options[インデックス番号].selected = true;
In this method, it becomes selected by setting the “selected” property through the “options” object .
The method using JavaScript’s “for” statement is written as follows.
for (var i = 0; i < Number of list box options; i++) {
document.getElementById("List box ID").options[i].selected = (i == index number);
}
In this method, the elements of the “options” object are retrieved one by one using a “for” statement and set to the “selected” property , thereby making them selected.
The sample program looks like this:
<!DOCTYPE html>
<html>
<head>
<script>
function setSelection() {
var selectBox = document.getElementById("mySelect");
for (var i = 0; i < selectBox.options.length; i++) {
selectBox.options[i].selected = (i == 1 || i == 3);
}
}
</script>
</head>
<body>
<select id="mySelect" multiple>
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="cherry">Cherry</option>
<option value="orange">Orange</option>
<option value="peach">Peach</option>
</select>
<br><br>
<button onclick="setSelection()">Set Selection</button>
</body>
</html>
This sample uses the HTML DOM’s “getElementById” method to obtain a list box with the ID “mySelect”. Next, we use the “for” statement to extract the elements of the “options” object one by one and set the “selected” property. Finally, press the button “Set Selection” and “Banana” and “Orange” will be selected.
In this way, “How to set the value of a multi-selection list box” can be easily achieved by using the “for” statement in JavaScript.
summary
We explained how to get and set the values of a multi-selection list box.
- You can retrieve values from a multi-selection list box by using an array and the options property.
- You can set values in a multi-selection list box by using the options and selected properties.
- When retrieving values from a multi-selection list box, you can use a for loop or forEach method to access them one by one.
- When setting values in a multi-selection list box, you can select a specific item by setting its selected property to true.
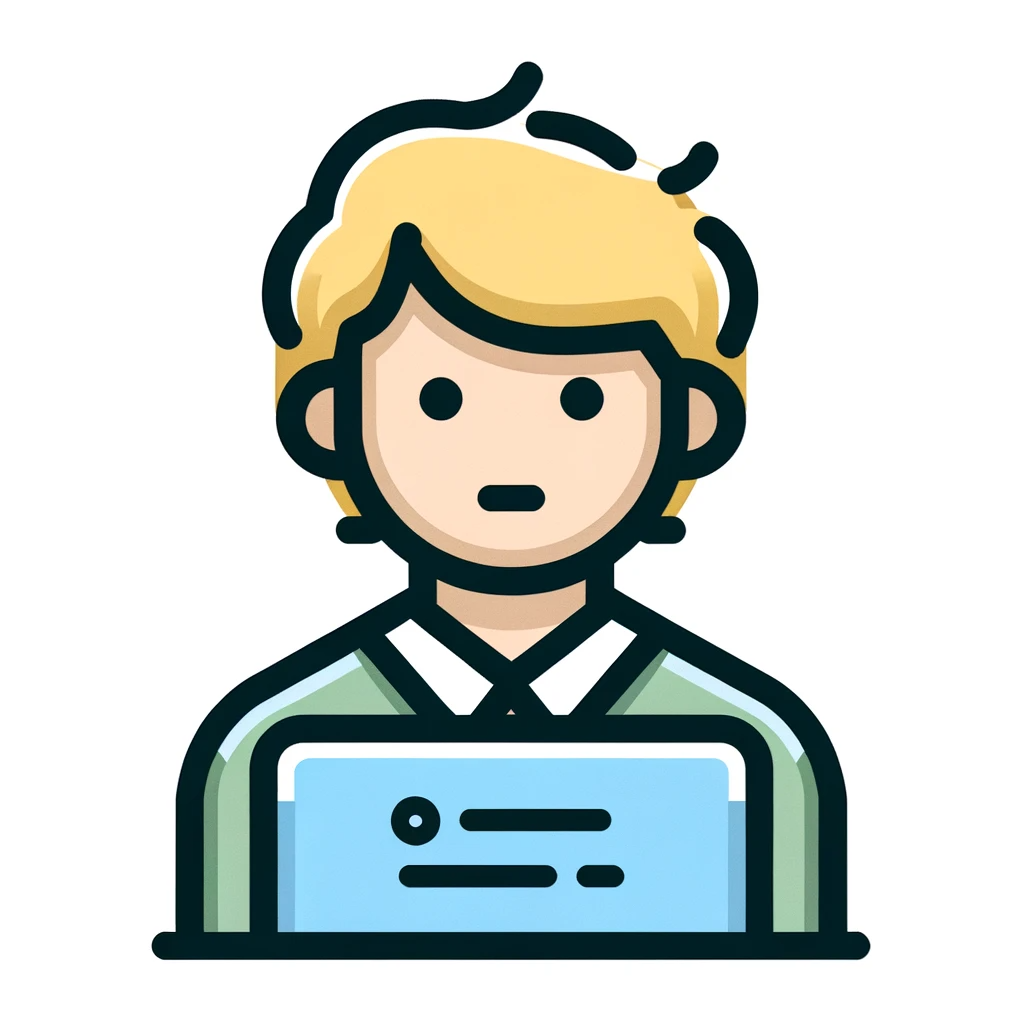
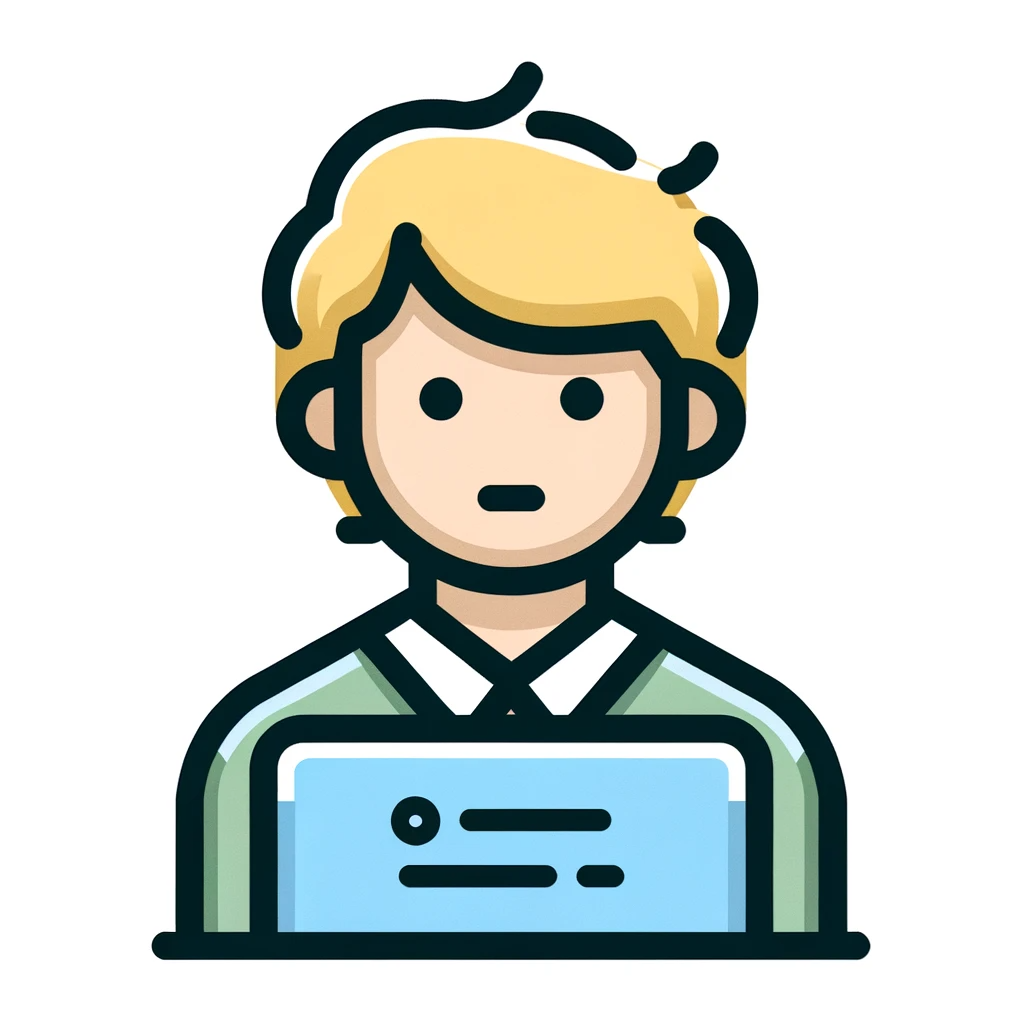
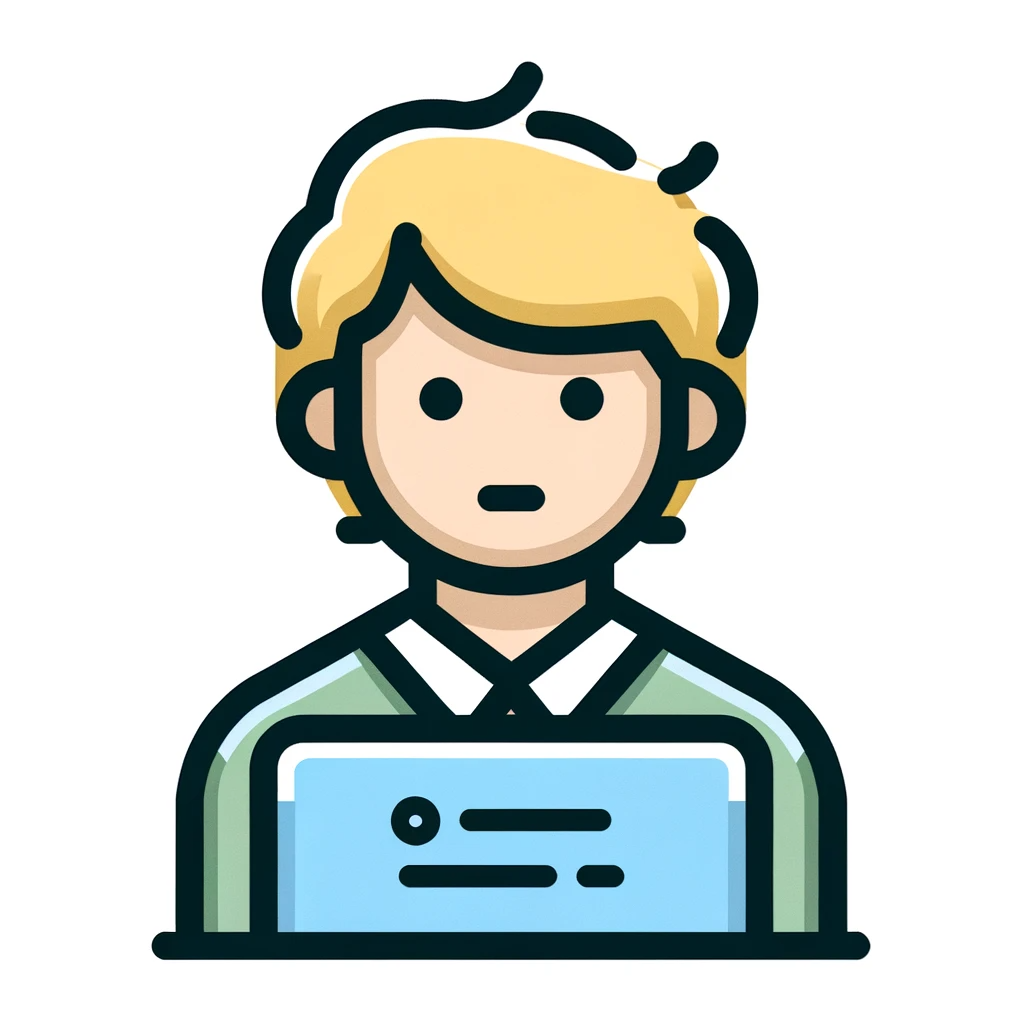
I now know how to get values from a multi-select listbox.
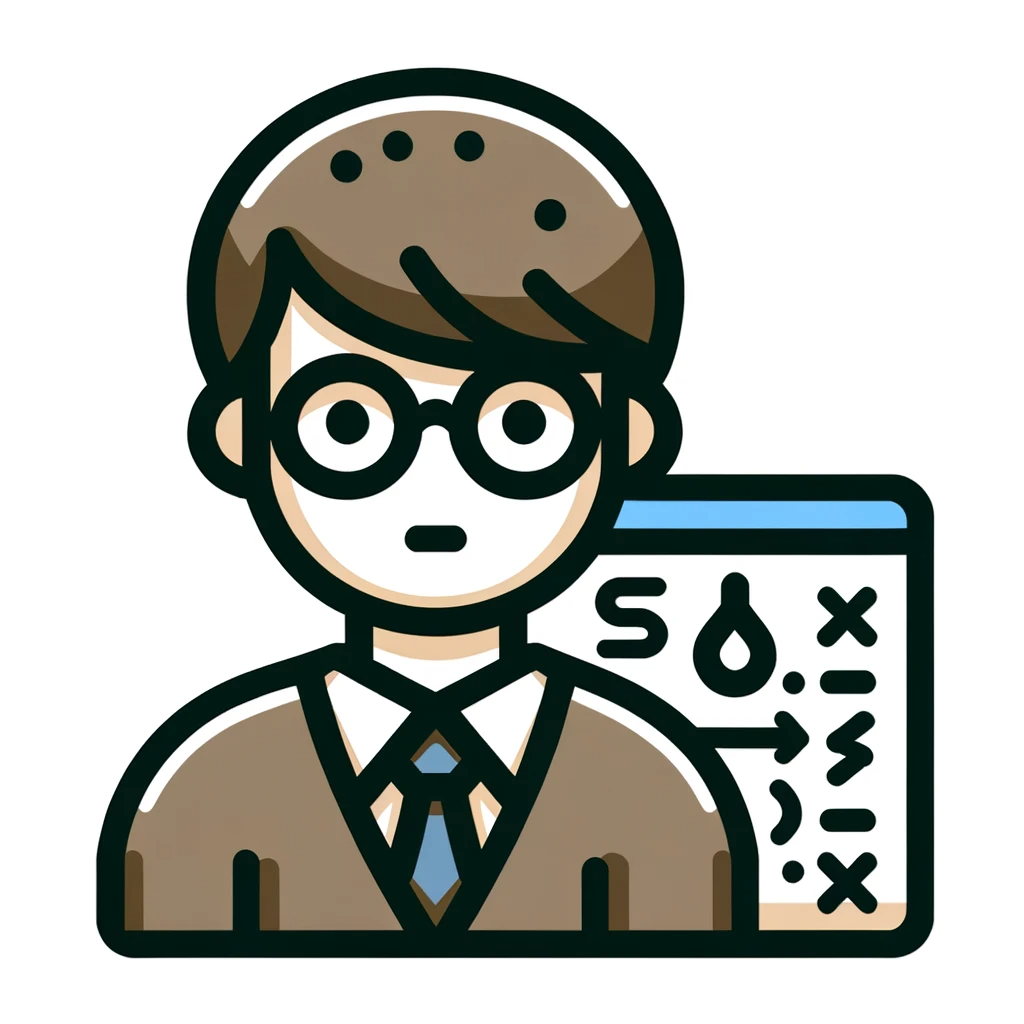
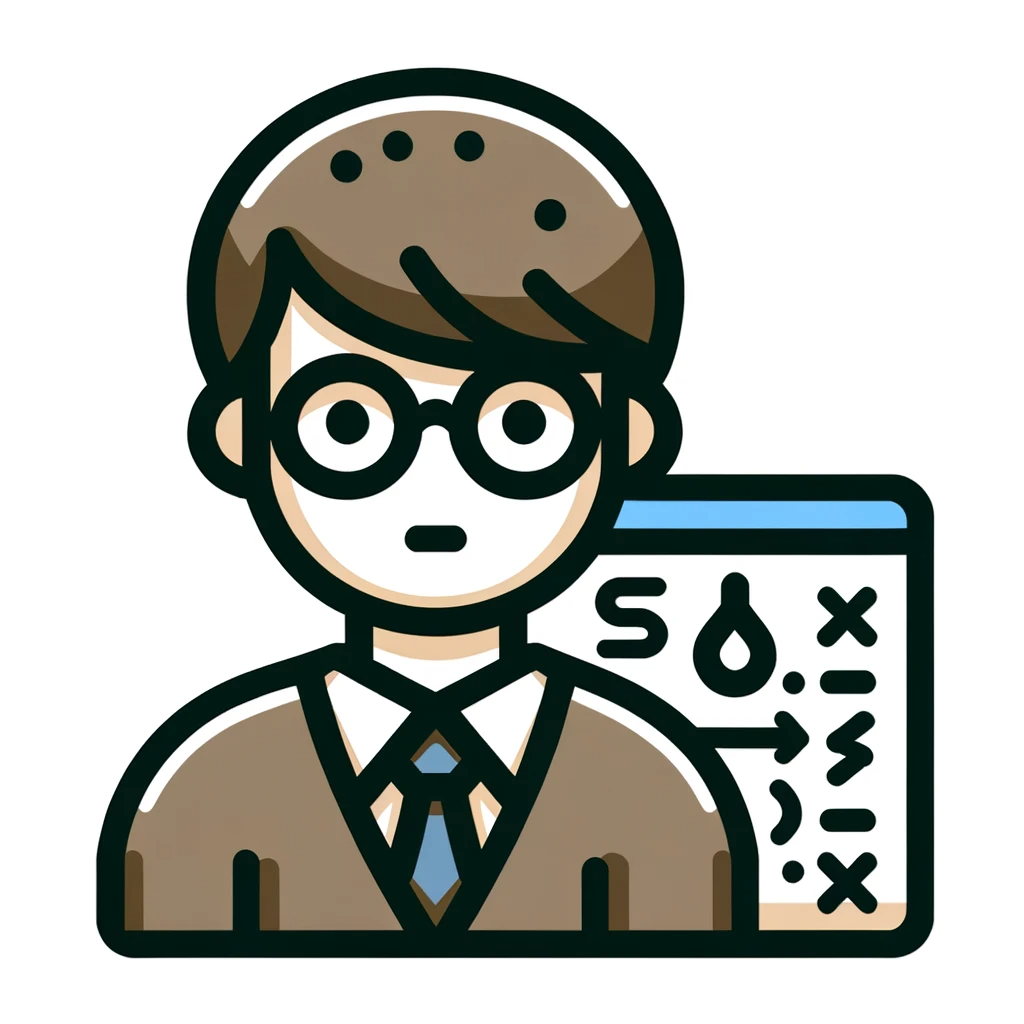
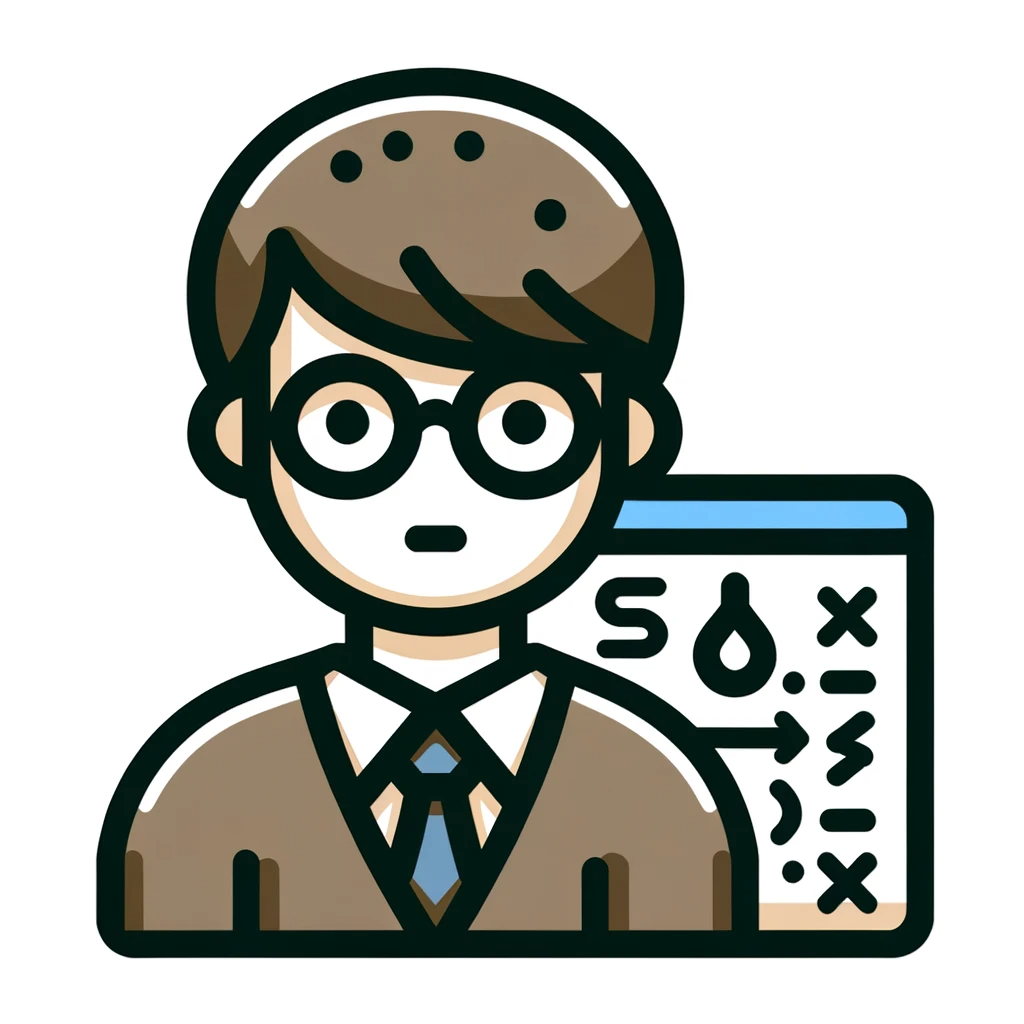
The “:checked” selector we talked about this time is also often used when retrieving form values in JavaScript, so it’s a good idea to remember it.
Comments