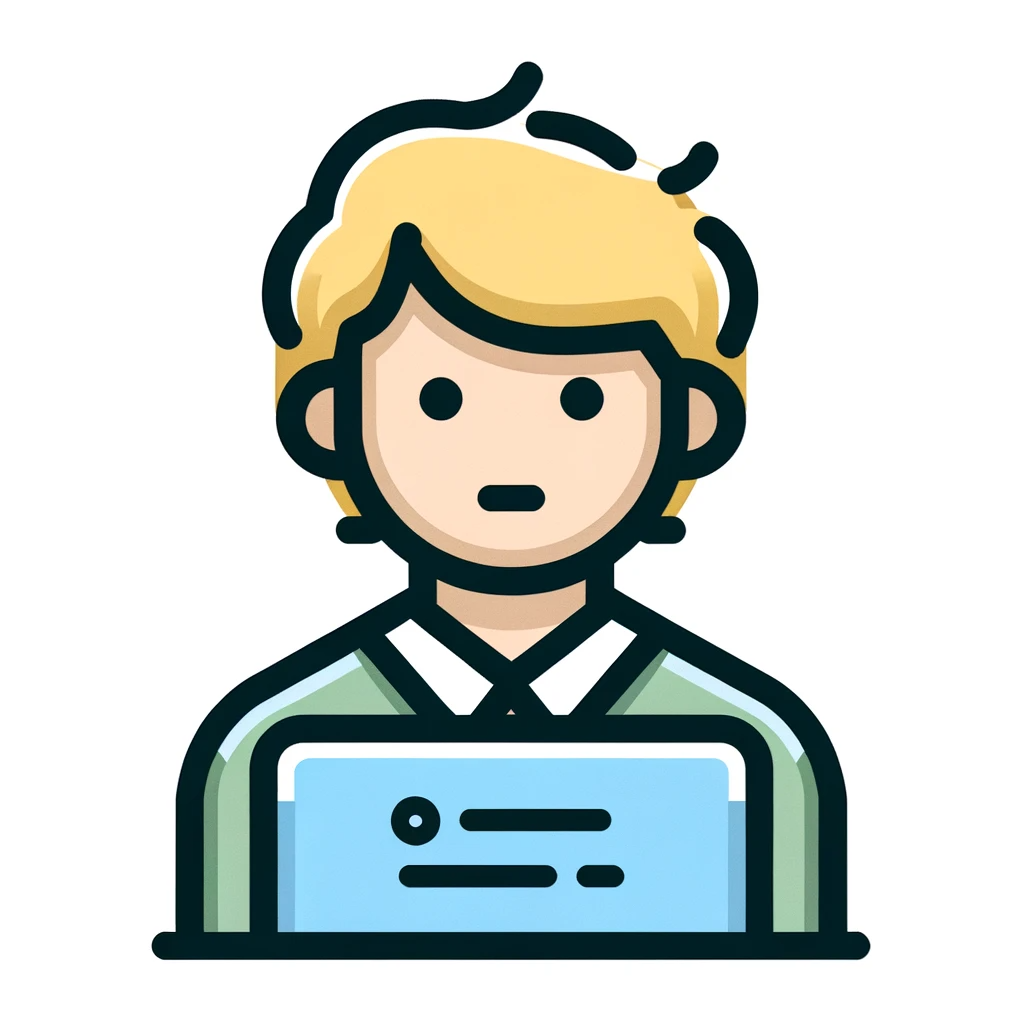
How can I convert a string to a number?
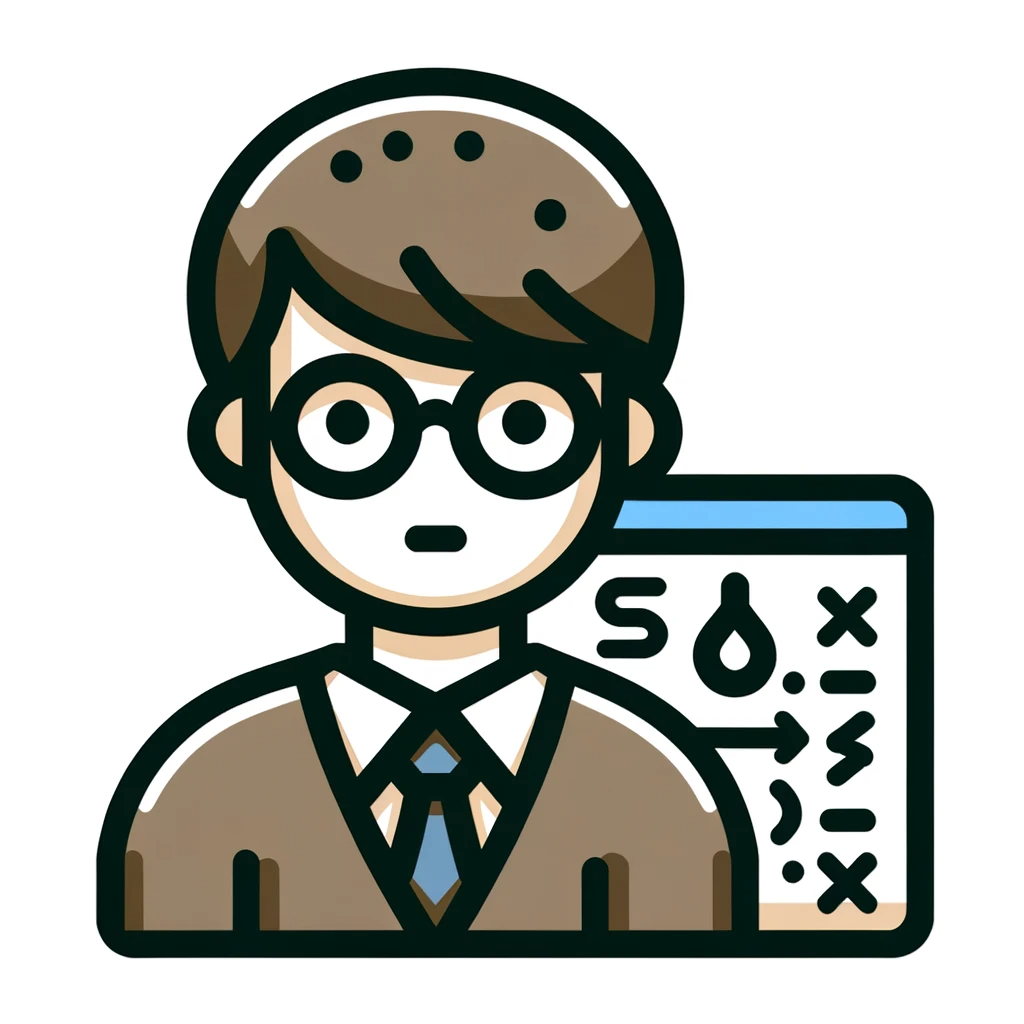
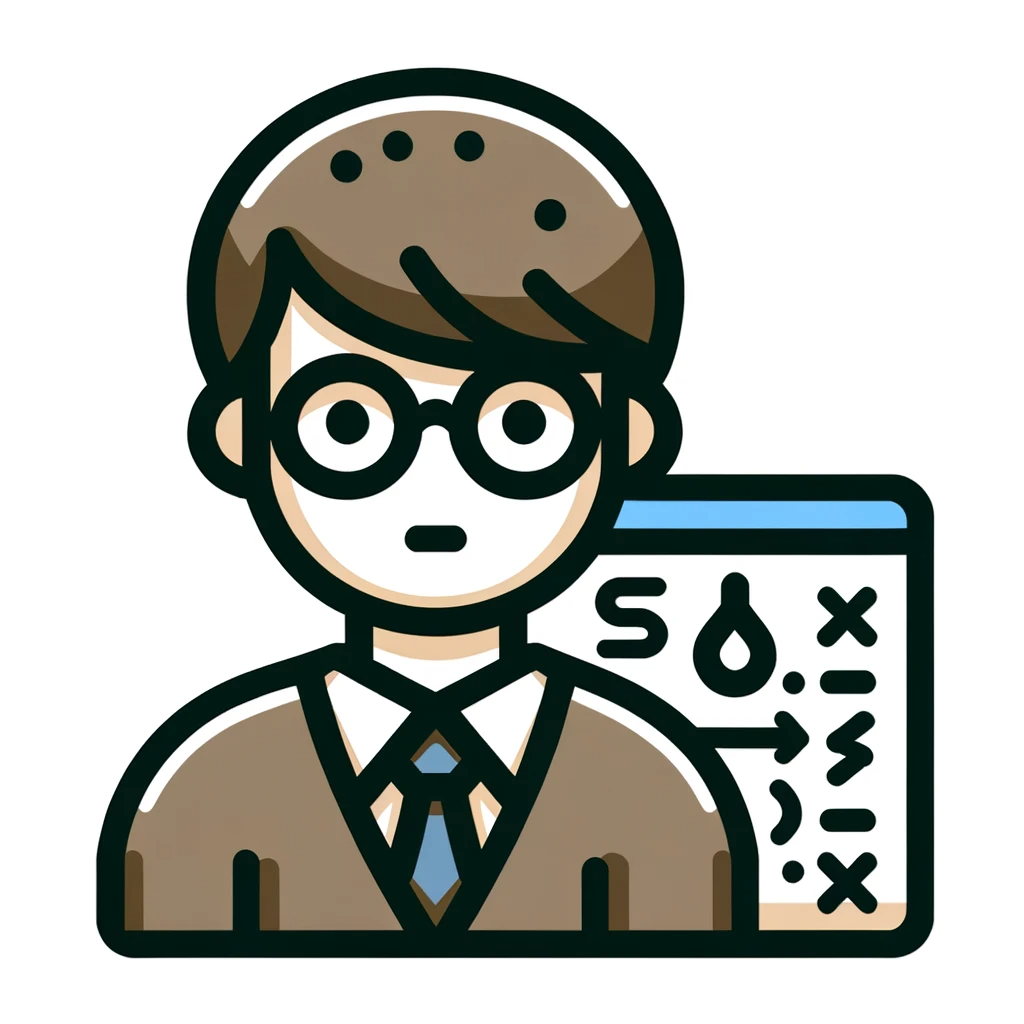
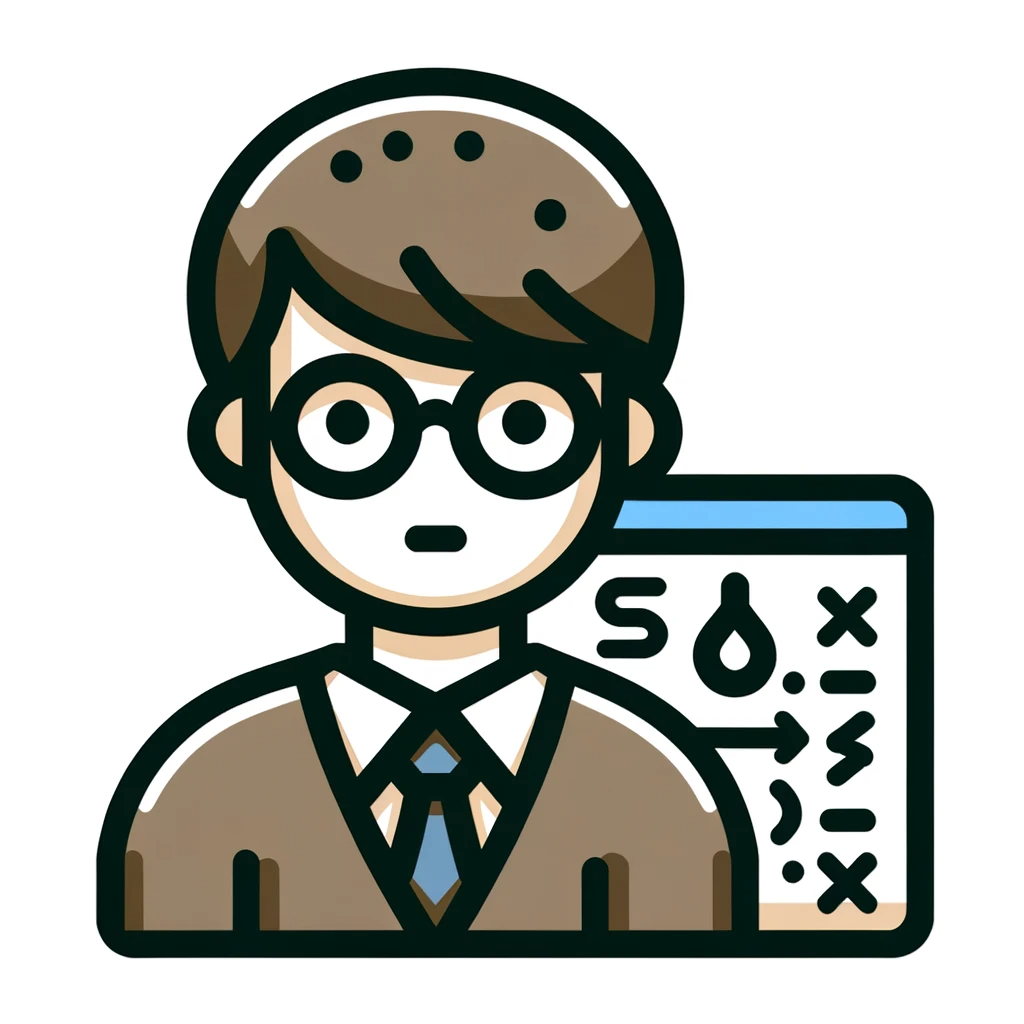
parseInt
, parseFloat
and Number
the constructor.
How to convert a string to a number using “parseInt” and “parseFloat”
The Number functions parseInt and parseFloat can be used to convert strings to numbers.
These parse strings and convert them to numbers. The difference is that each can handle integers and floating point numbers.
How to use parseInt
To convert a string to an integer number, parseInt
you can use a function.
How to use it is as follows.
const str = '123';
const num = parseInt(str);
console.log(typeof num); // -> 'number'
parseInt
The second argument of the function allows you to specify the radix to use when parsing the string. For example, to parse a binary string, specify 2 as the second argument.
const str = '1101';
const num = parseInt(str, 2);
console.log(num); // -> 13
How to use parseFloat
To convert a string to a number with a decimal point, parseFloat
you can use a function.
How to use it is as follows.
const str = '123.45';
const num = parseFloat(str);
console.log(typeof num); // -> 'number'
The parseFloat function can also convert an integer to a number with a decimal point.
const str = '123';
const num = parseFloat(str);
console.log(typeof num); // -> 'number'
If there is a leading number, the leading number is converted as a number.
The behavior is also slightly different when converting hexadecimal or octal numbers.
If you try to convert a string that is not a number, NaN (Not a Number) is returned.
Using the “Number” function
To convert a string to a number, you can also use the Number function.
The usage is as follows.
const str = '123';
const num = Number(str);
console.log(num); // -> 123
const str2 = '123abc';
const num2 = Number(str2);
console.log(num2); // -> NaN
Number conversion returns NaN (Not a Number) in case of confusion of strings, etc.
summary
The following is a summary of how to convert a string to a number .
parseInt
Functions are available to convert integers .parseFloat
Function to convert numbers with decimal pointNumber
Another way is to use functions.
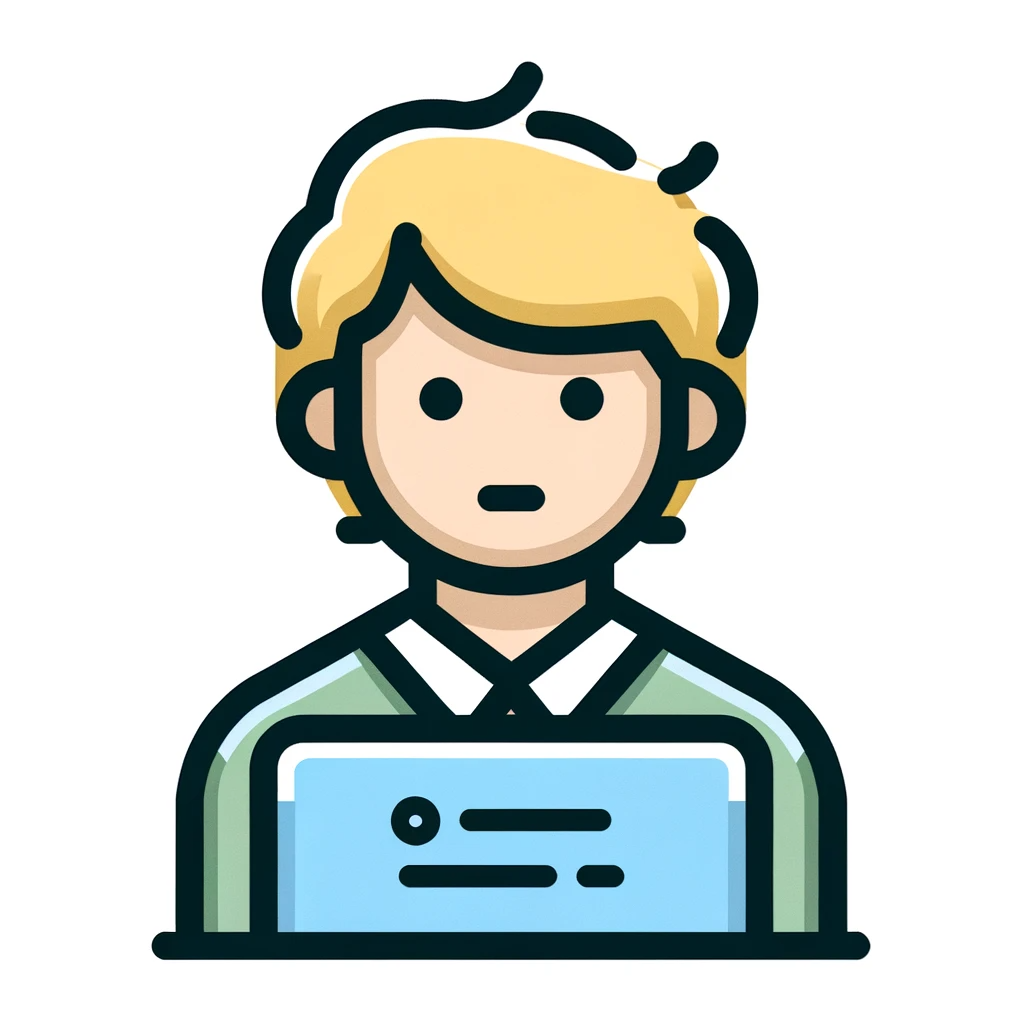
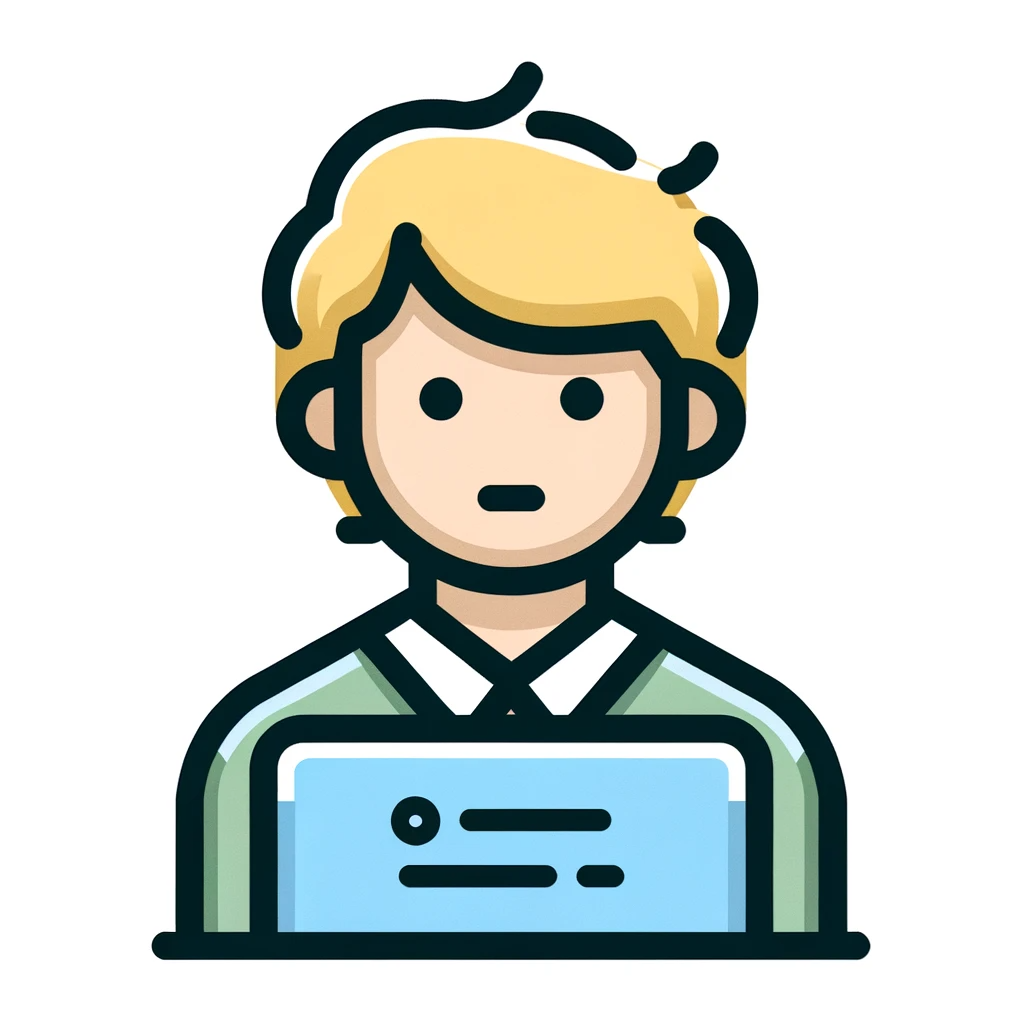
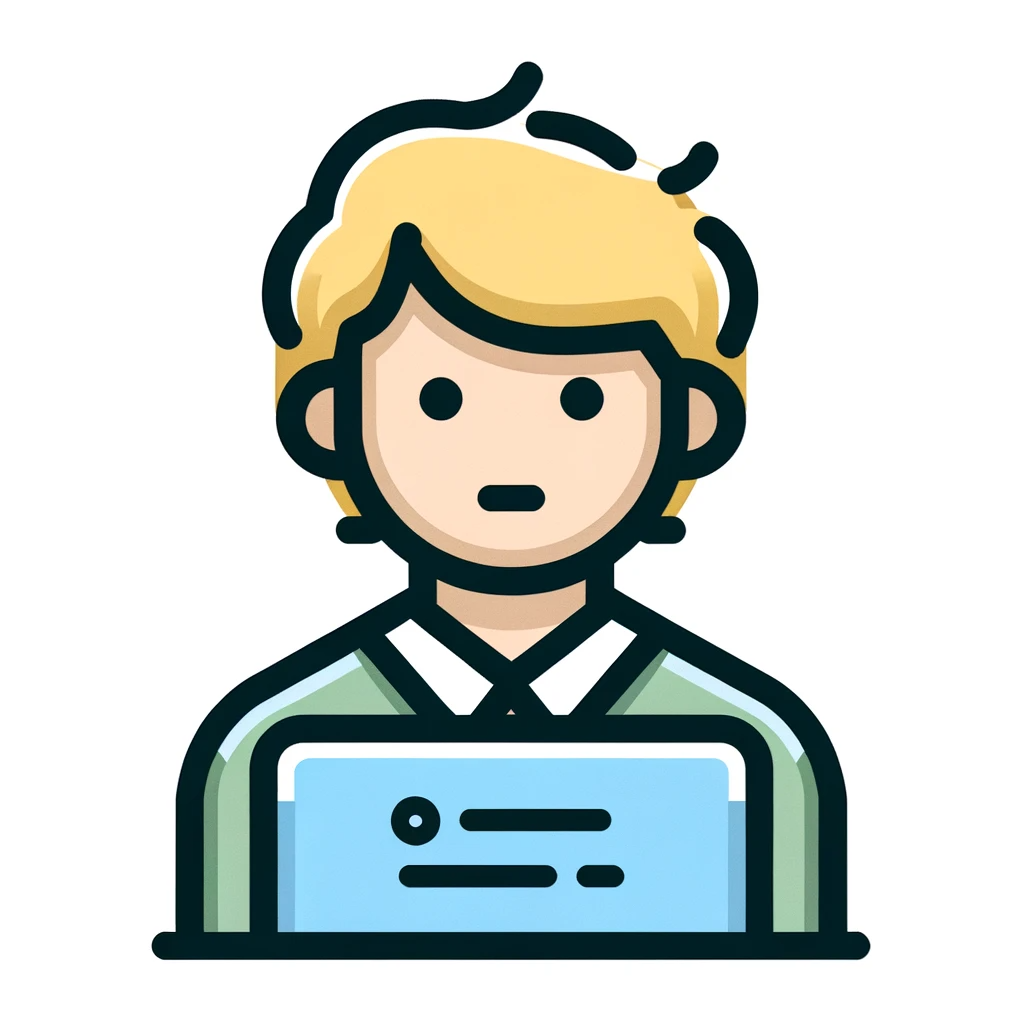
It is important to choose which method to use depending on the purpose and string used!
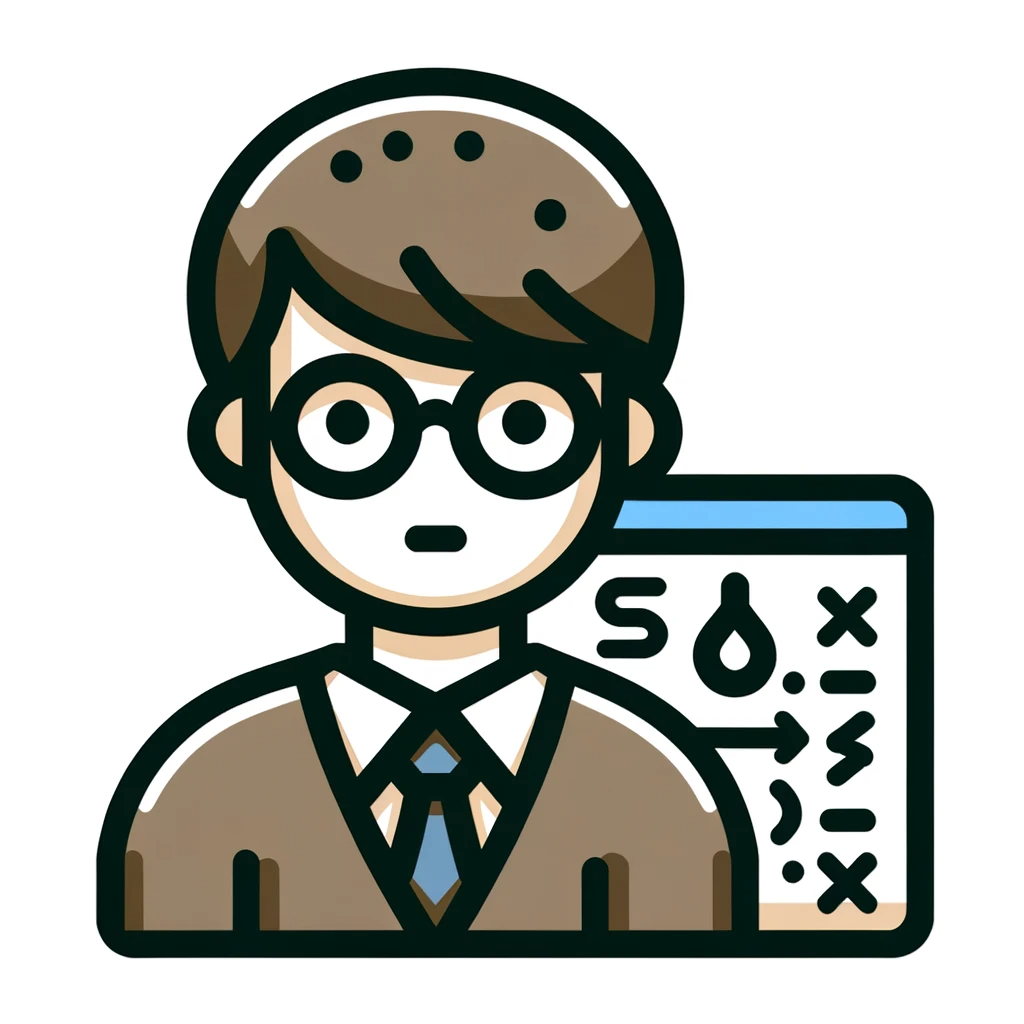
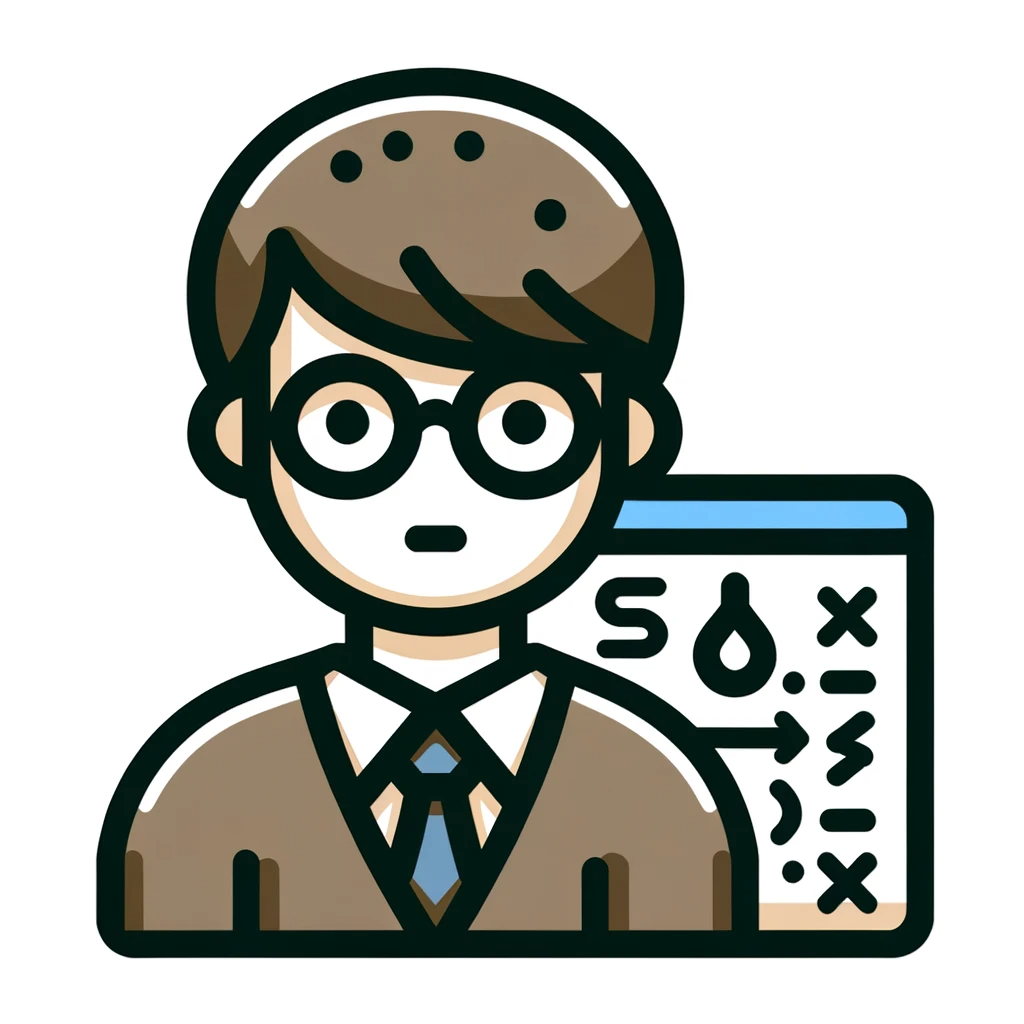
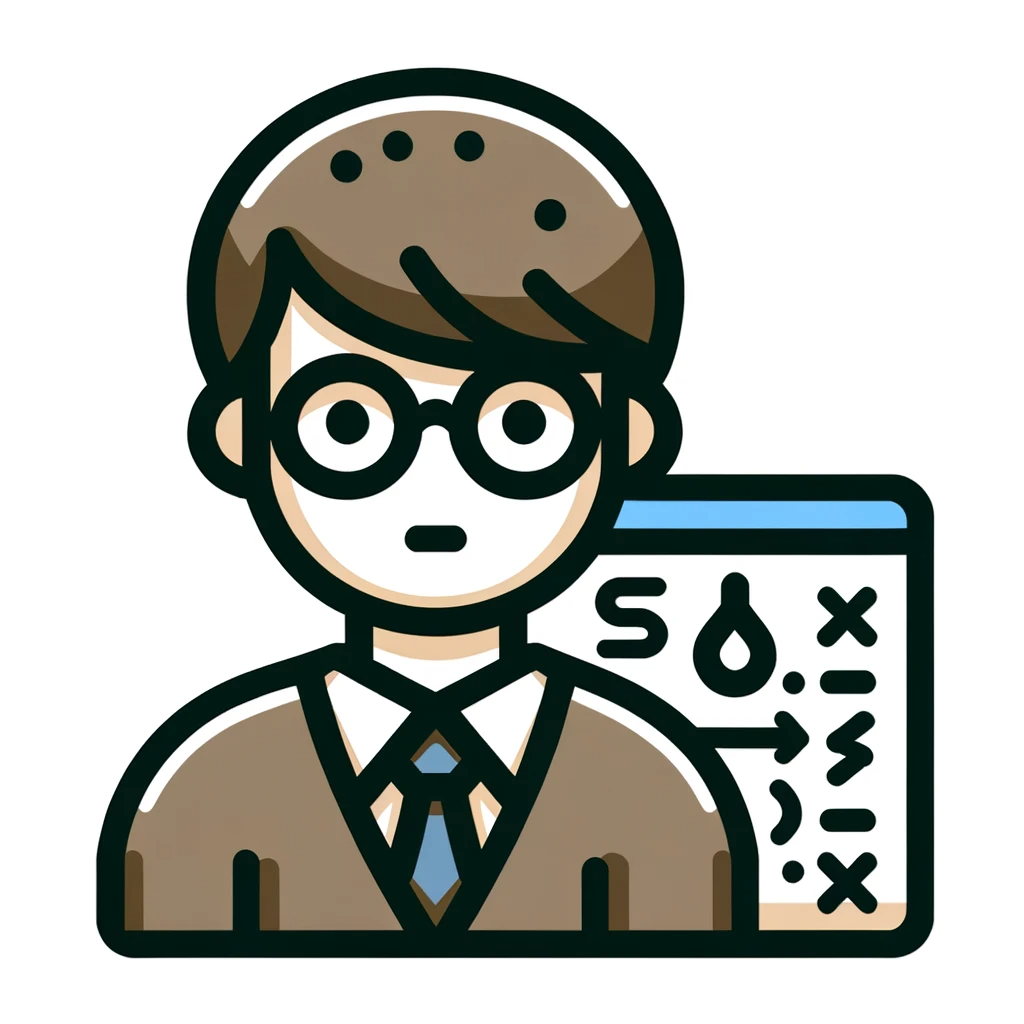
It is also important to note that attempting to convert a non-numeric string NaN
will return .
Comments