This article explains how to embed variables in strings using JavaScript.
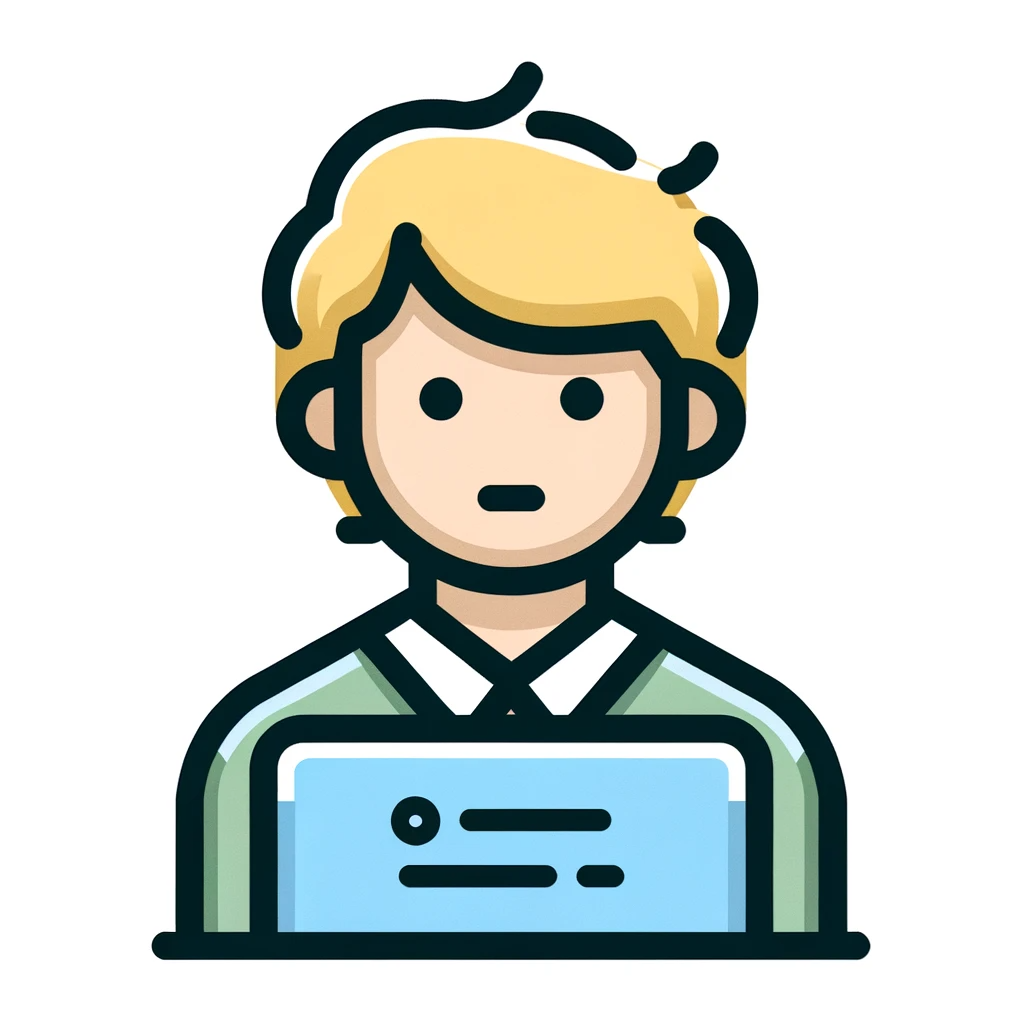
I want to embed a variable in a string with JavaScript and output it.
What should I do now?
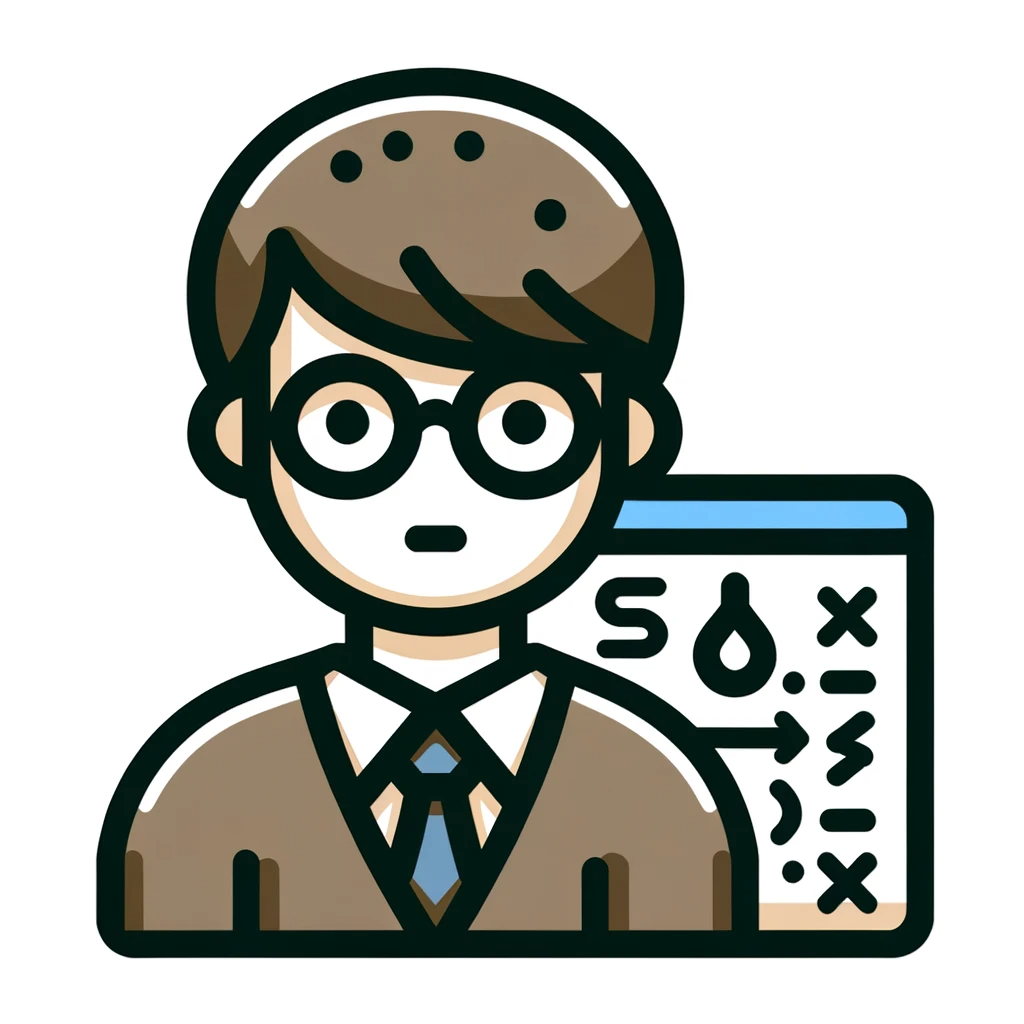
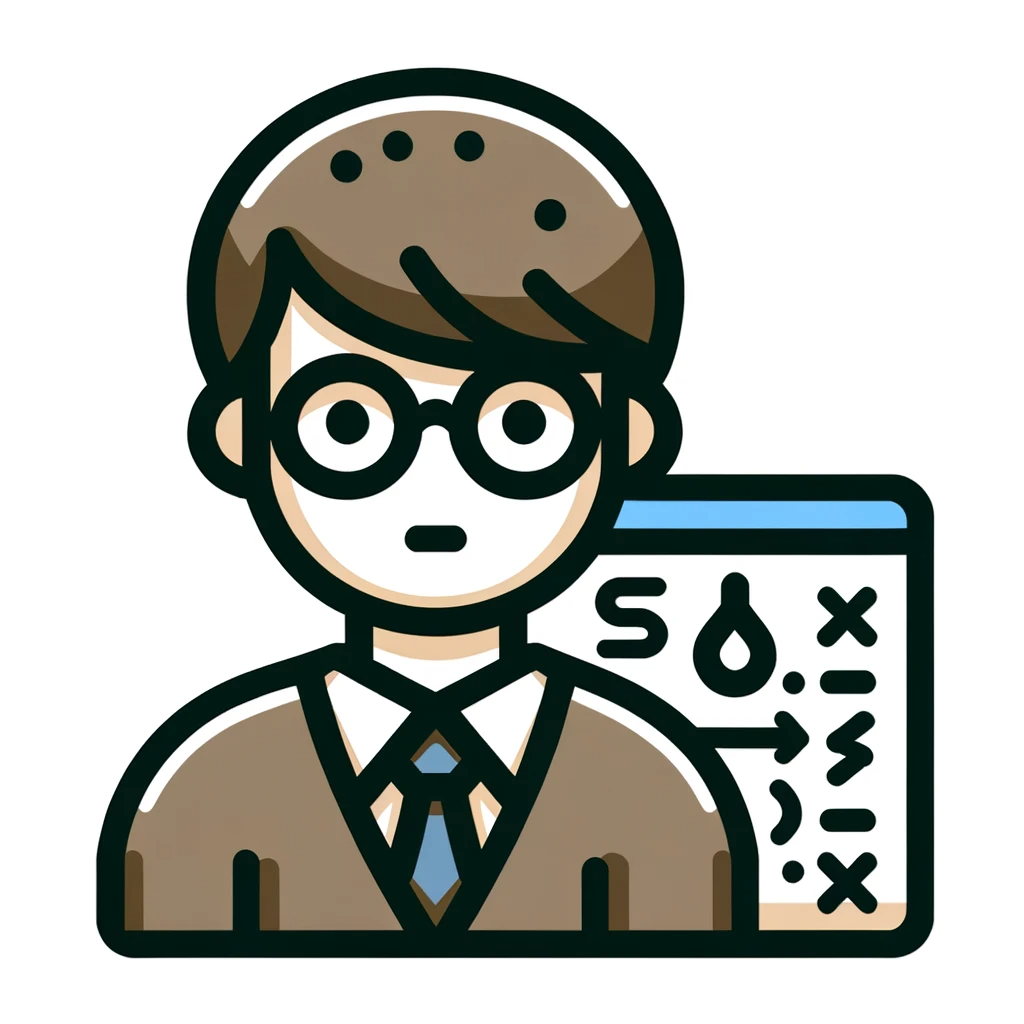
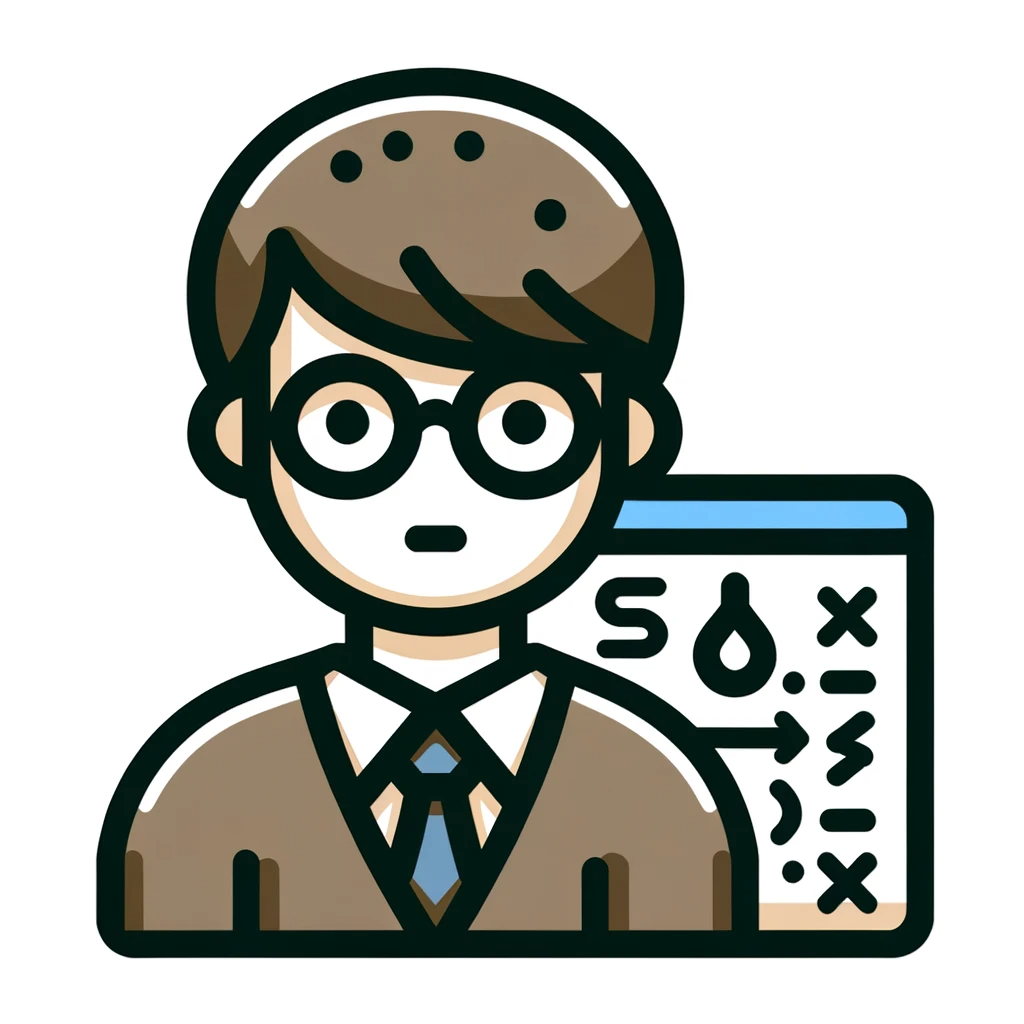
${variable}
You can embed variables in strings by writing something like this or +
by concatenating strings using operators.
Embedding variables in strings
There are two ways to embed variables in strings : use template literals , and combine ${variable}
strings with +
operators .
What is a template literal?
JavaScript template literals are a feature that makes it easier and more intuitive to assemble strings . This allows you to embed variables and expressions in strings.
Traditional methods of creating strings require concatenating multiple strings to create a string. However, template literals allow you to easily construct strings by embedding variables and expressions within them.
To create a template literal ${variable}
use backticks
How to use template literal ${variable name} (ES2015~)
The method of using template literals works with browsers that support ES2015.
${variable}
To write a template ${variable}
literal , write it as follows.
// Define Variables
var name = "John";
// Embed variables in strings
var str = `Hello, ${name}!`;
// Output Variables
console.log(str); // "Hello, John!"
Also, if you want to output ${variable} as a string, \
you can output it as a character by adding a backslash.
console.log(`Hello, \${name}!`);
//Execution Result
"Hello, \${name}!"
How to combine strings with the + operator
+
You can also use the plus sign to concatenate strings . It also works with older browsers such as IE11.
// Define Variables
var name = "John";
// Embed variables in strings
var str = "Hello, " + name + "!";
// Output Variables
console.log(str); // "Hello, John!"
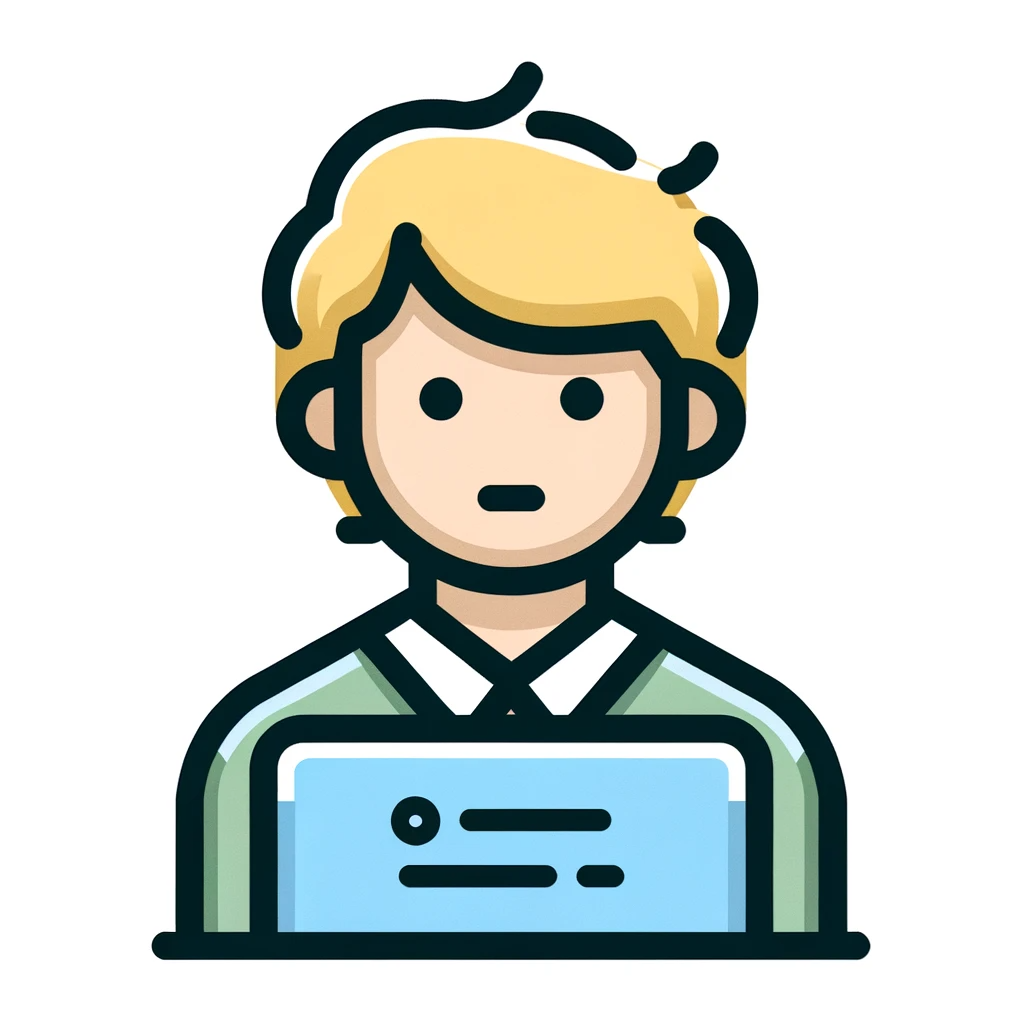
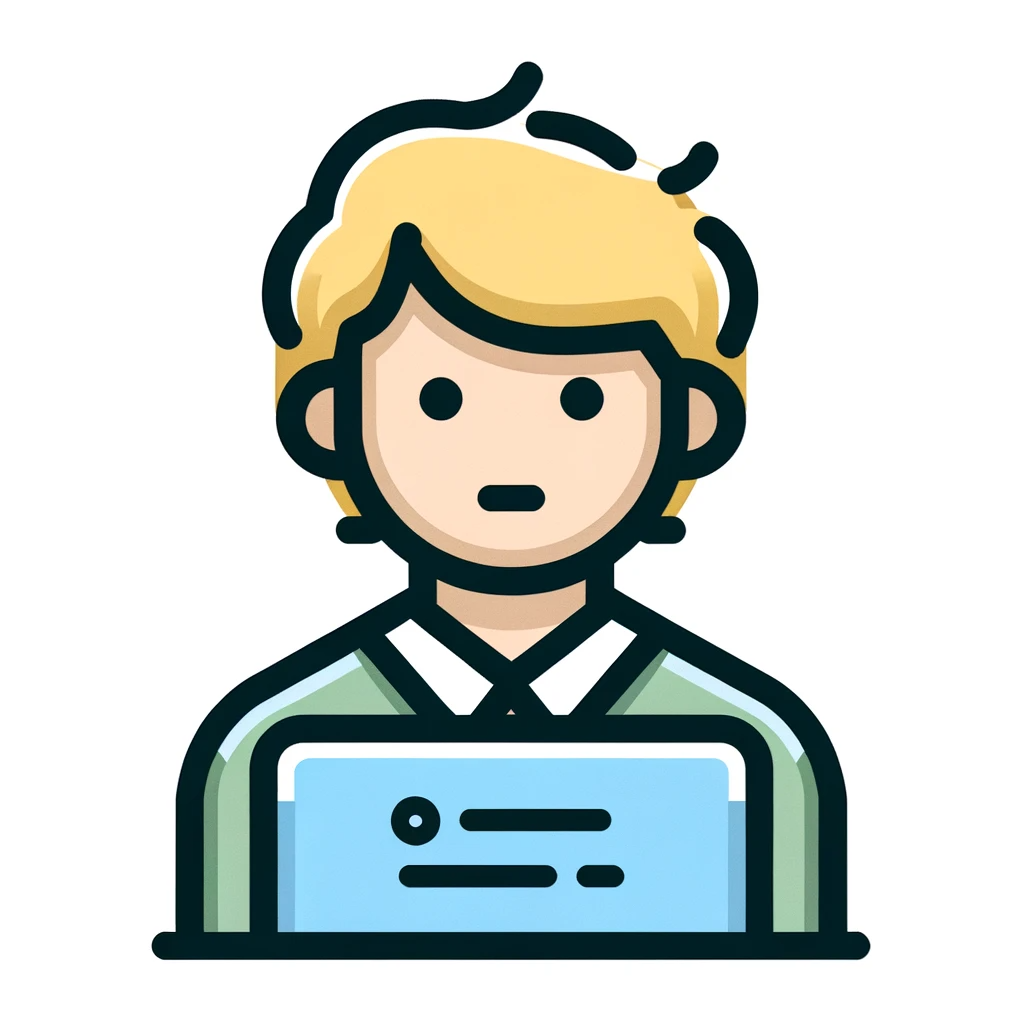
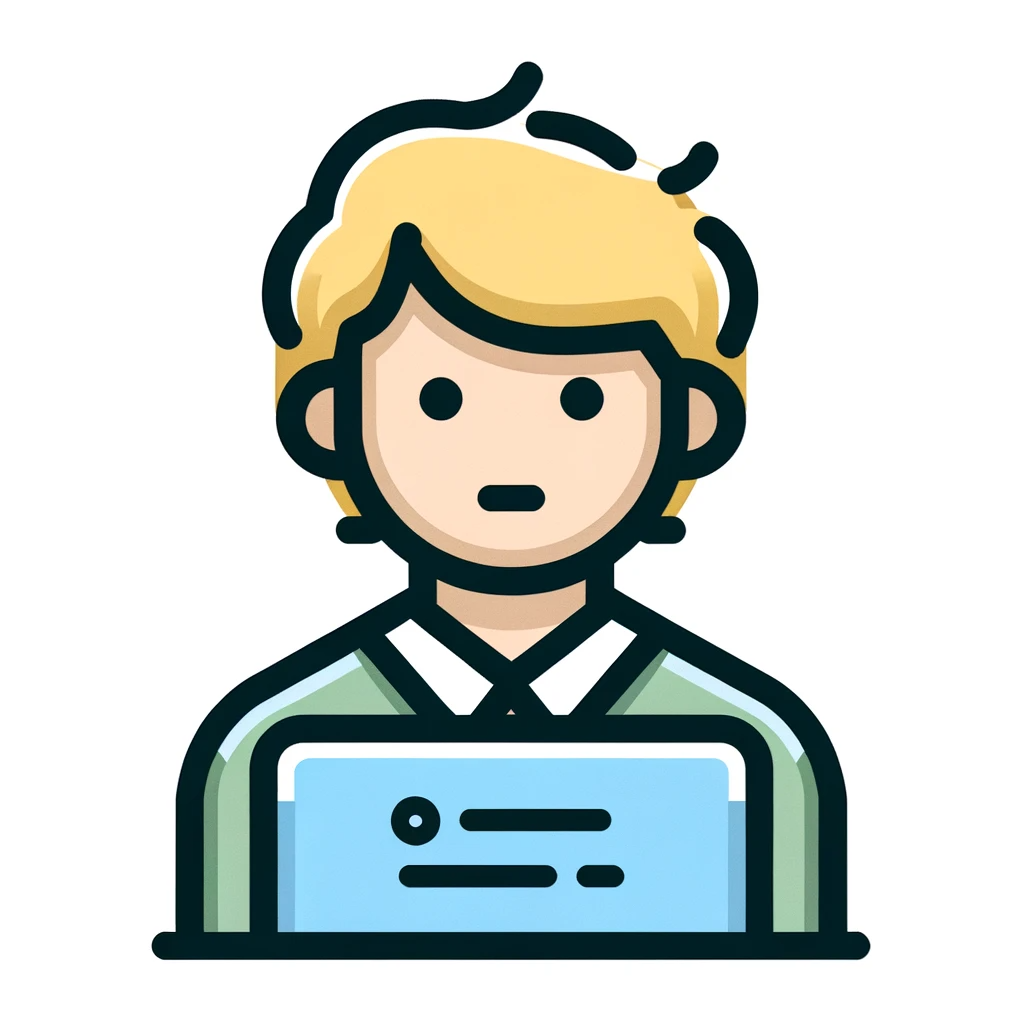
It is important to consider the readability of the code and decide which method is better depending on the situation.
Put a variable in a string and reflect it in HTML
To embed a variable in a string with JavaScript and have it reflected in HTML, do the following:
<!DOCTYPE html>
<html>
<body>
<h1>How to embed variables in strings in JavaScript</h1>
<p id="demo">The string is output here.</p>
<script>
// Define Variables
var name = "John";
// Embed variables in strings
var str = `Hello, ${name}!`;
// Output variables in HTML elements
document.getElementById("demo").innerHTML = str;
</script>
</body>
</html>
In the above example, document.getElementById("demo").innerHTML
we are using to output a string inside an HTML element. We also use template literals to embed variables within the string.
This allows you to reflect strings created with JavaScript in HTML documents.
summary
The following is a summary of how to embed variables in strings.
- To embed variables in strings in JavaScript, use the plus sign (+) or use template literals.
- If you use the plus sign (+), embed variables by concatenating strings.
- When using a template literal,
${variable}
embed the variable by writing:
You can embed variables in strings by using the plus sign (+) or by using template literals. Furthermore, by outputting a string in an HTML element, you can display a string created with JavaScript within an HTML document.
Comments