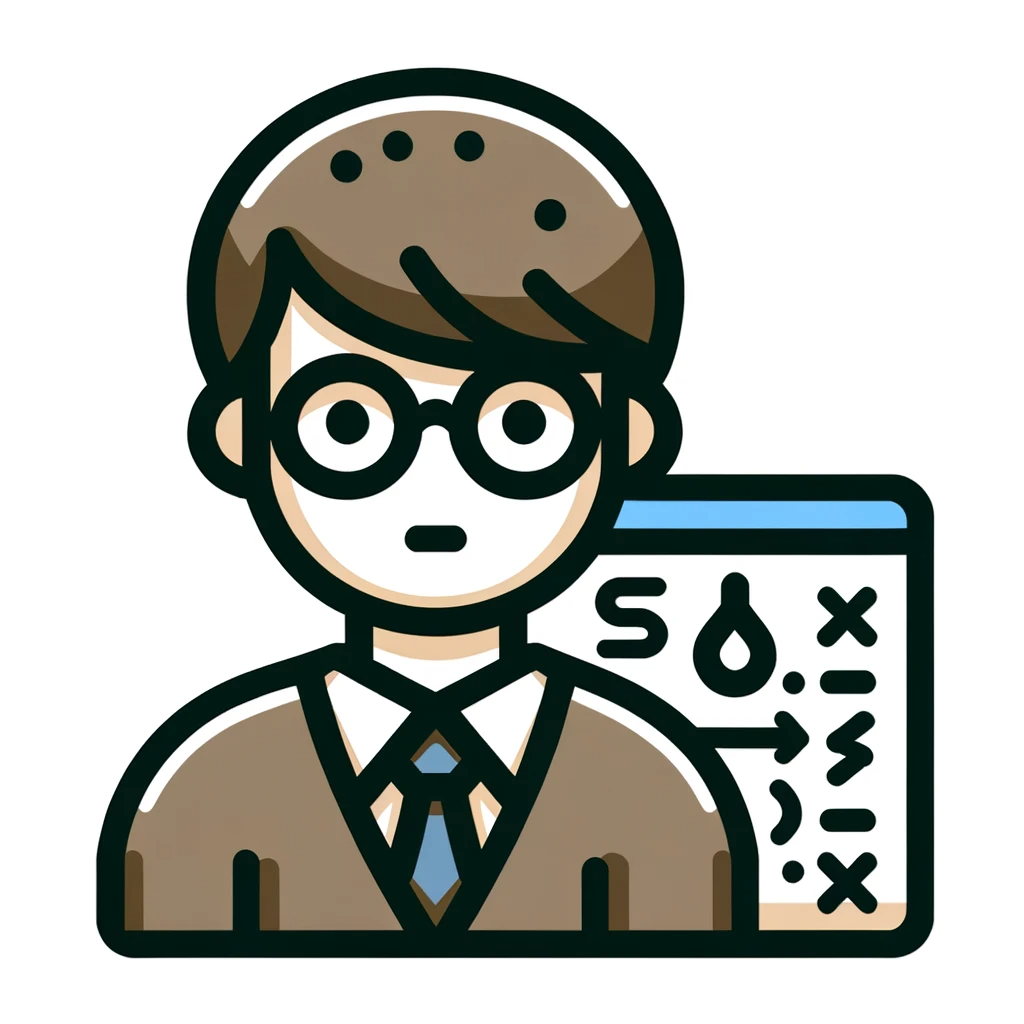
Let’s learn about JavaScript variables and constants.
What is a variable?
A variable is a virtual location for storing data used in a program . Variables allow you to reference and update values in your program.
How to write variables
use variables in JavaScript, let
declare them using keywords.
let variable name;
=
Assign values to variables using symbols. You can also declare a variable and assign a value to it at the same time.
let x;
x = 5;
let y = 10;
Variable names consist of letters, numbers, underscores, or dollars. Variable names must not start with a number .
JavaScript does not require you to explicitly declare the type of a variable . The type of value referenced by a variable is determined at runtime.
let x = 'hello';
x = 5;
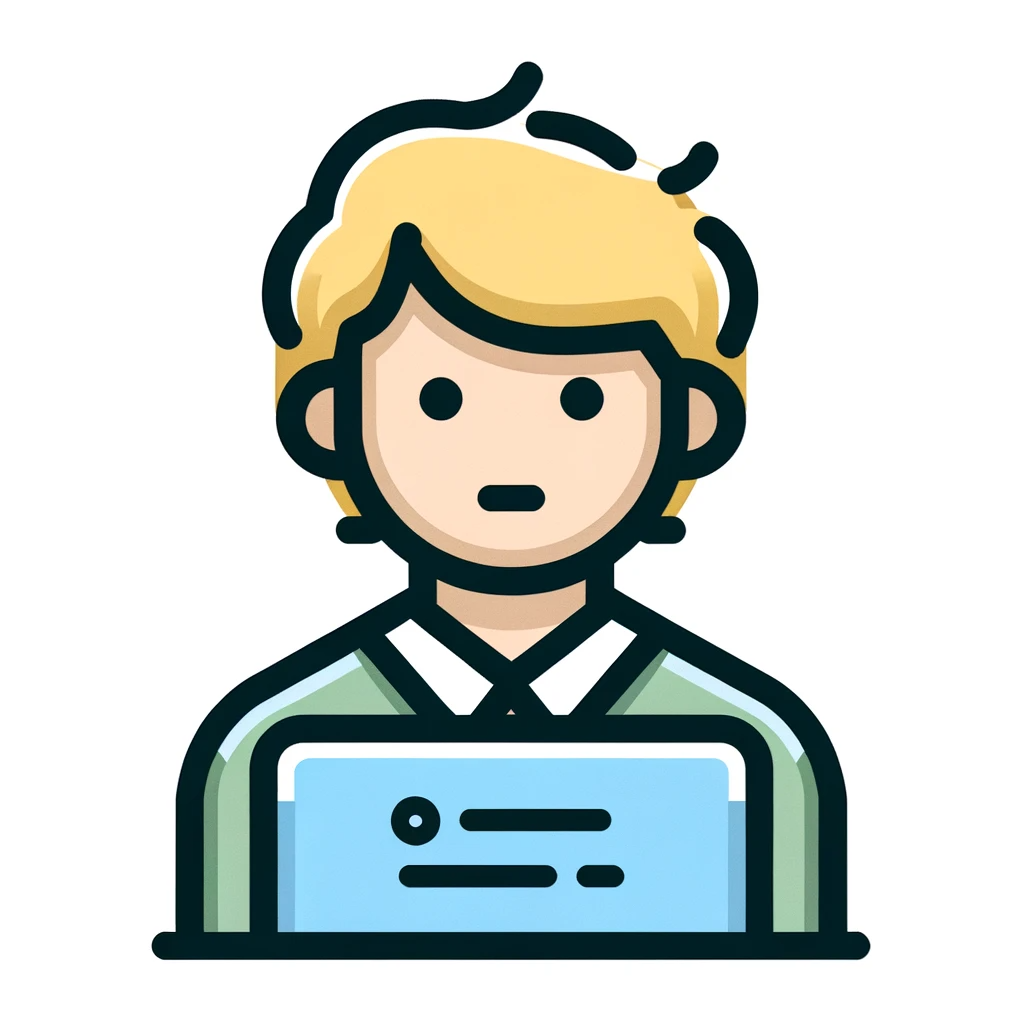
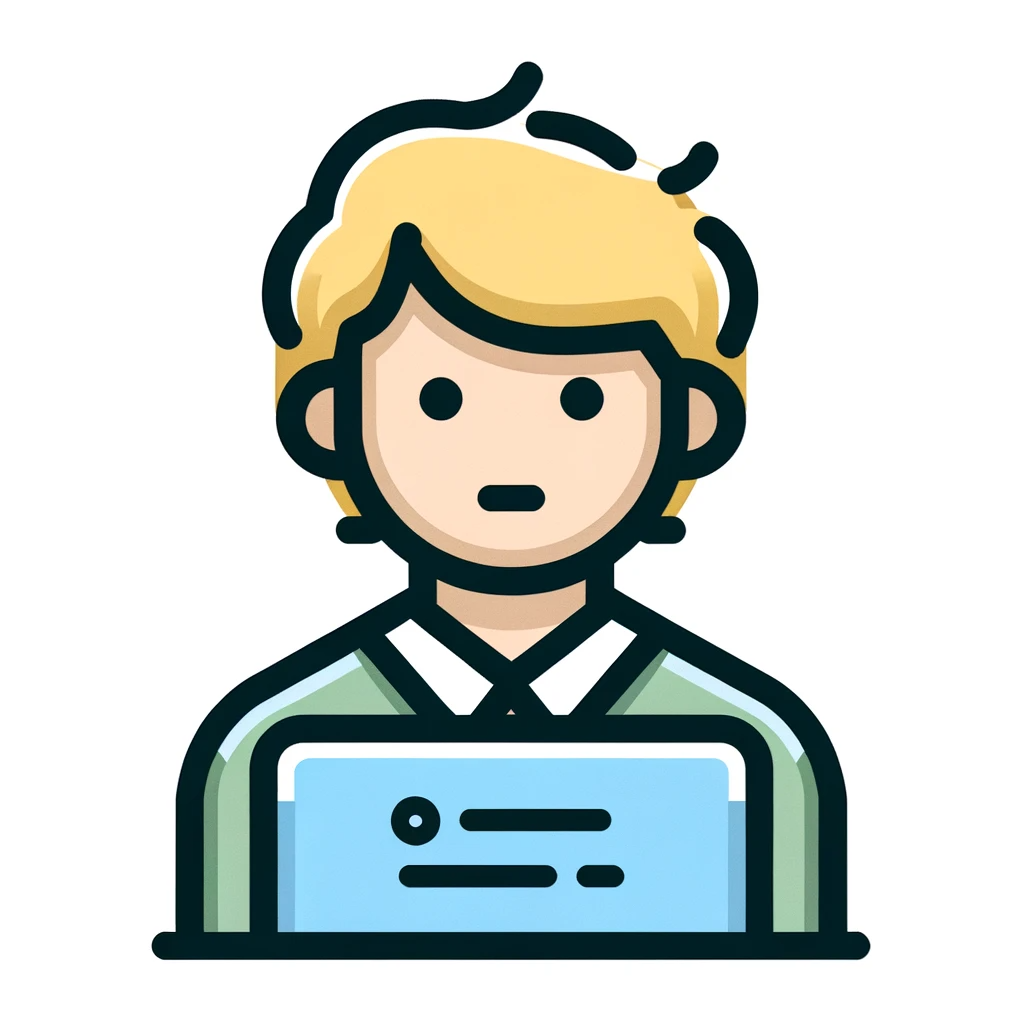
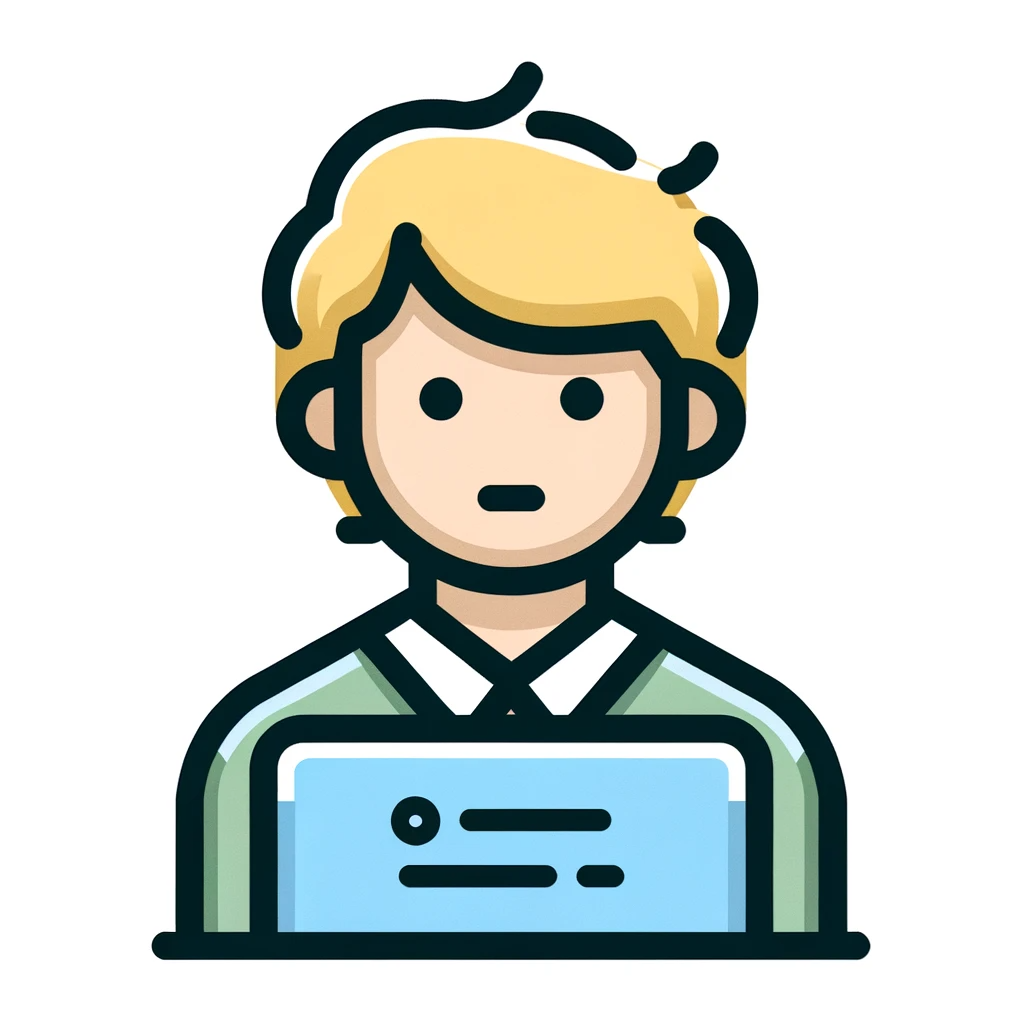
In JavaScript, variable types are not declared.
About variable naming rules
JavaScript variables have the following rules:
- The first character of a variable name must be an alphabet, an underscore
_
, or a dollar sign .$
- Subsequent characters can be any character available for the first character (alphabet, underscore, dollar sign, or number).
- Uppercase and lowercase letters are case sensitive.
- Variable names must not be reserved words that have meaning in JavaScript (such as const or if).
In addition to let, there is another way to declare variables in JavaScript using the var keyword.
In the case of var, the behavior is different and has properties such as “allowing variables with the same name” and “not recognizing block scope,” so the let keyword is a higher quality declaration method.
Unless there is a special reason, use let to make declarations.
How to write constants
In JavaScript, const
constants (variables that cannot be changed) can be declared using keywords. const
Constants declared using keywords are valid only within the scope in which they are declared.
Also, a constant cannot be assigned a value again after it is declared .
const constant name = assigned value;
Constants are valid only within the scope in which they are declared.
if (true) {
const PI = 3.14;
console.log(PI);
}
console.log(PI);
const PI = 3.14;
PI = 3.14159;
In the above example, {}
PI is defined as const within the if scope. In this case, this const is only valid within the scope. After that, I try to print the output, but this results in an error because it is out of scope.
Next, since it is out of scope, it is possible to define PI with const separately, but since const cannot be assigned after declaration, an error occurs when trying to assign it.
Summary of variables and constants
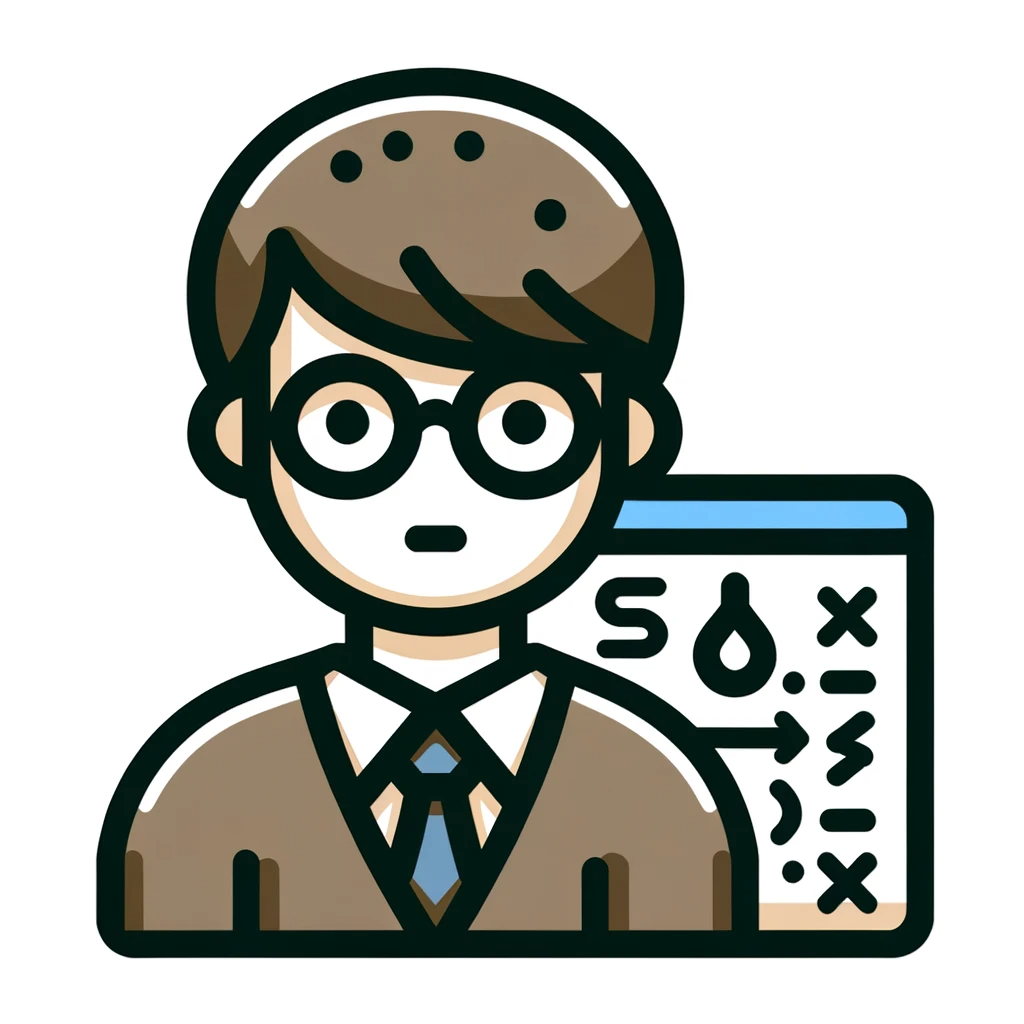
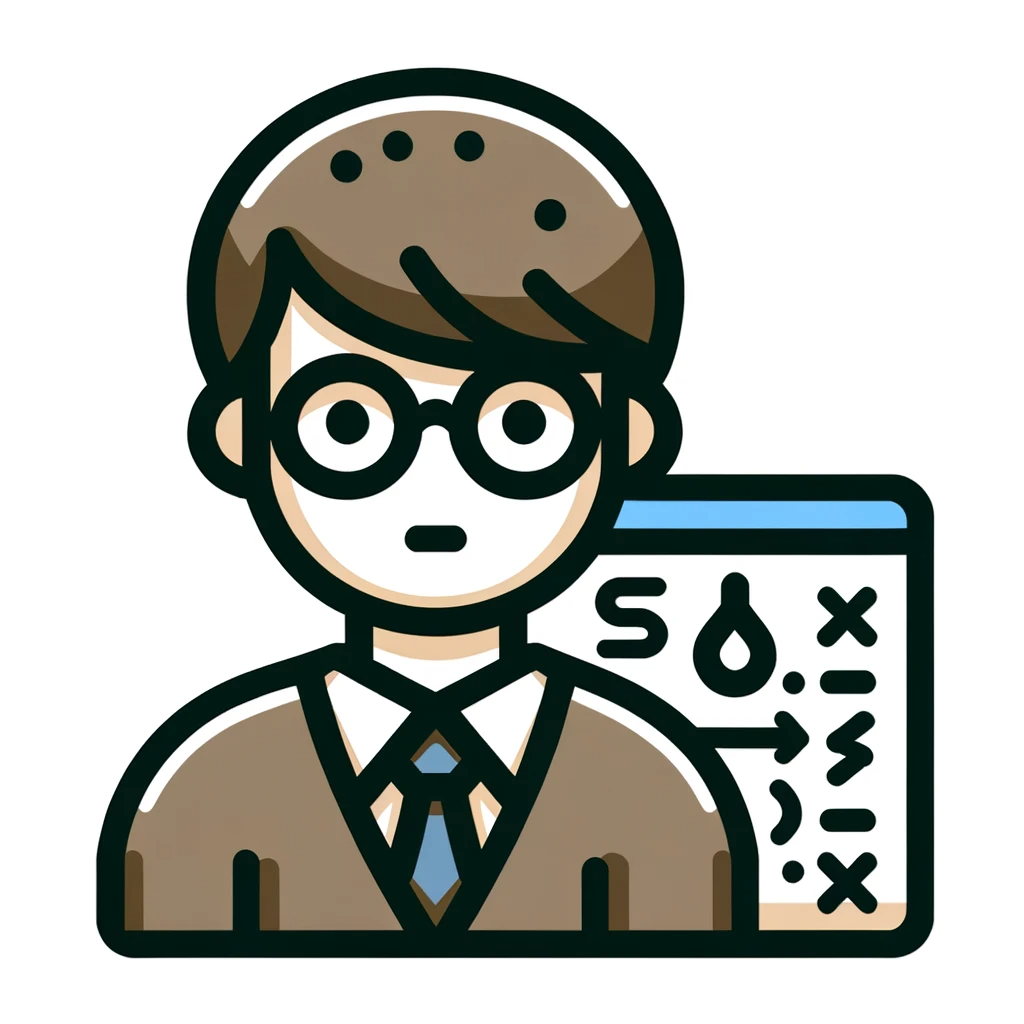
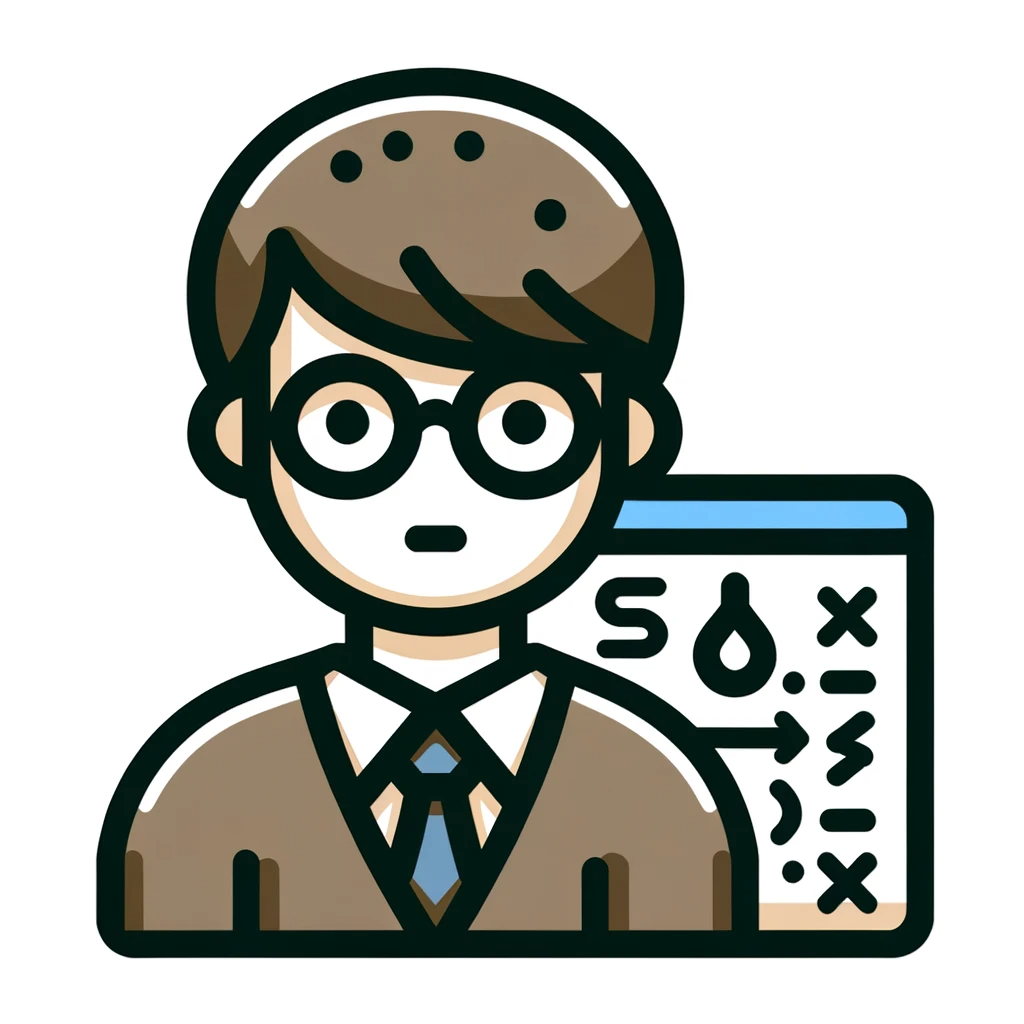
Let’s summarize the variables and constants we learned.
- Variables allow you to store, reference, and update values in your program.
- To use variables,
let
declare them using keywords and=
assign values using symbols. - Constants allow you to store and reference values in your program, but you cannot update them.
Comments