Introducing “How to get the length of a string ” in JavaScript .
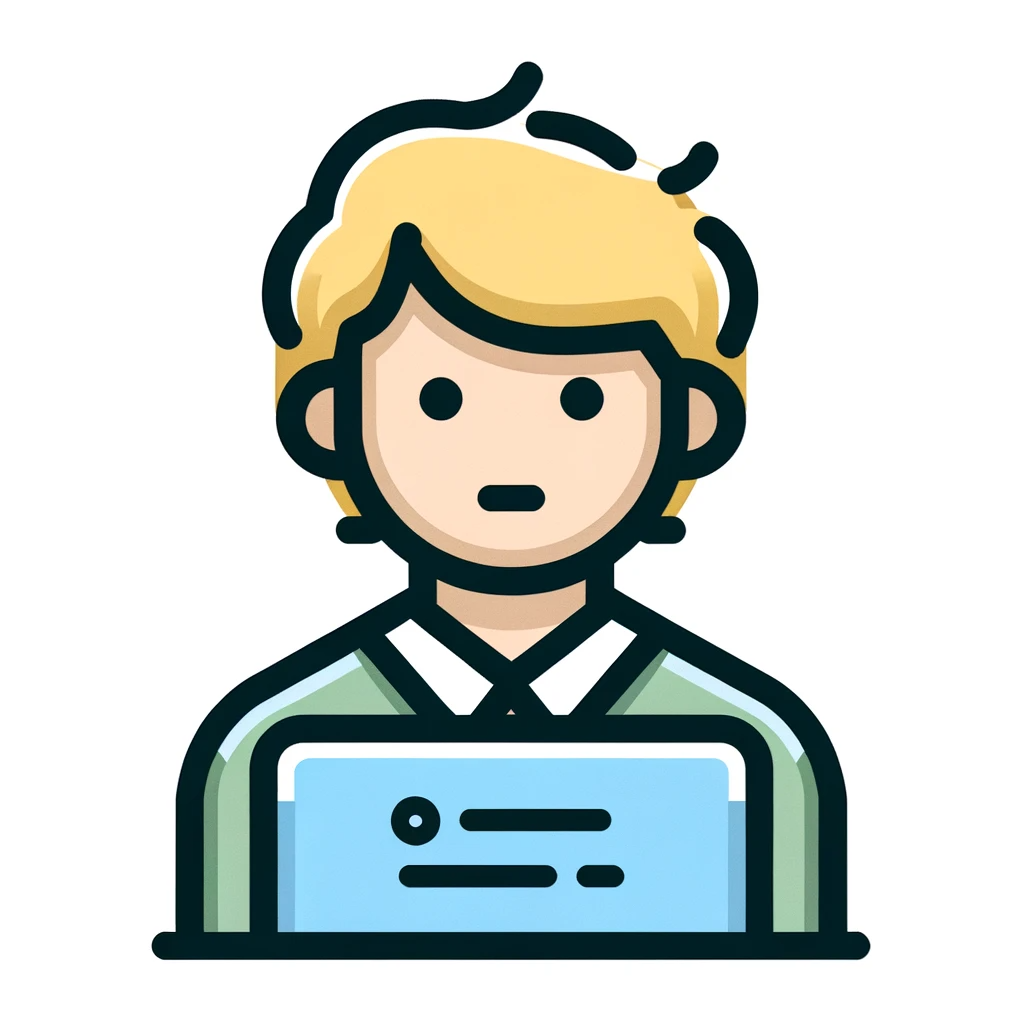
How can I get the length of a string?
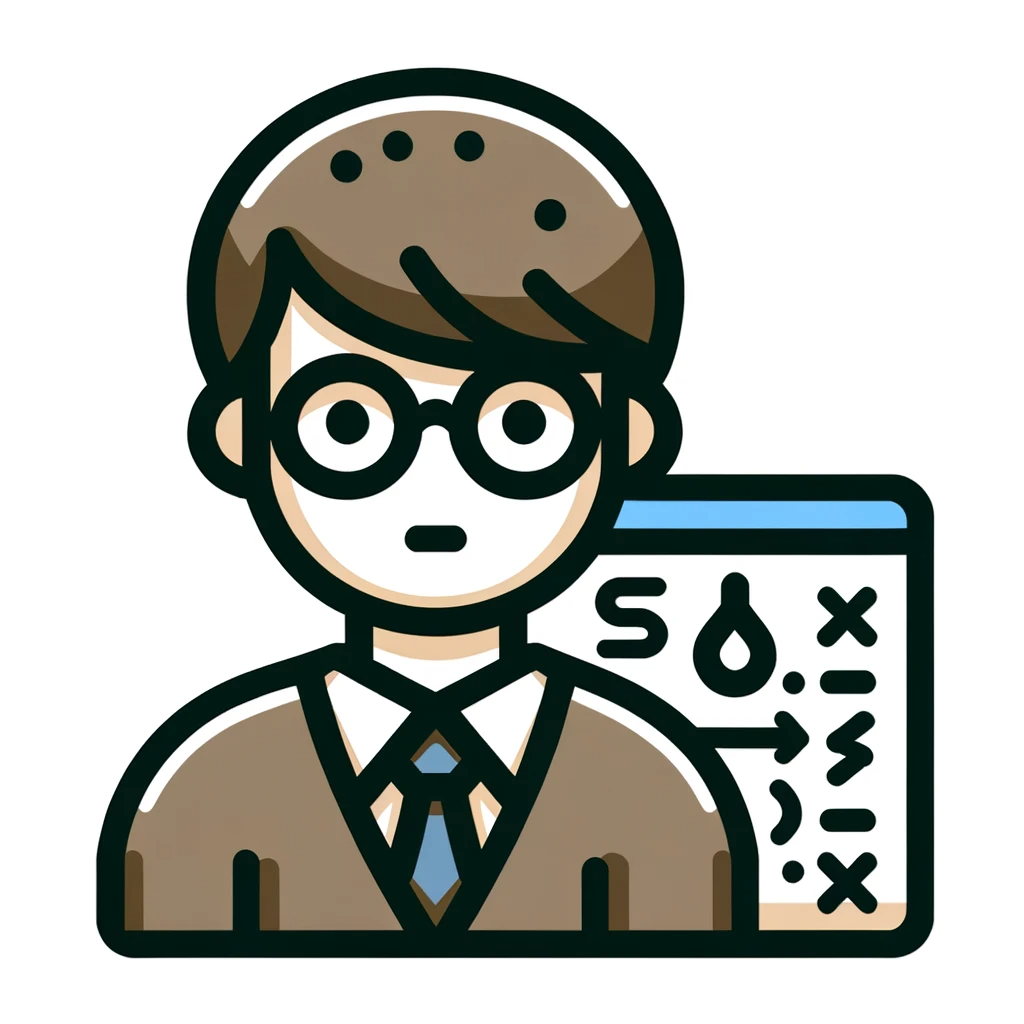
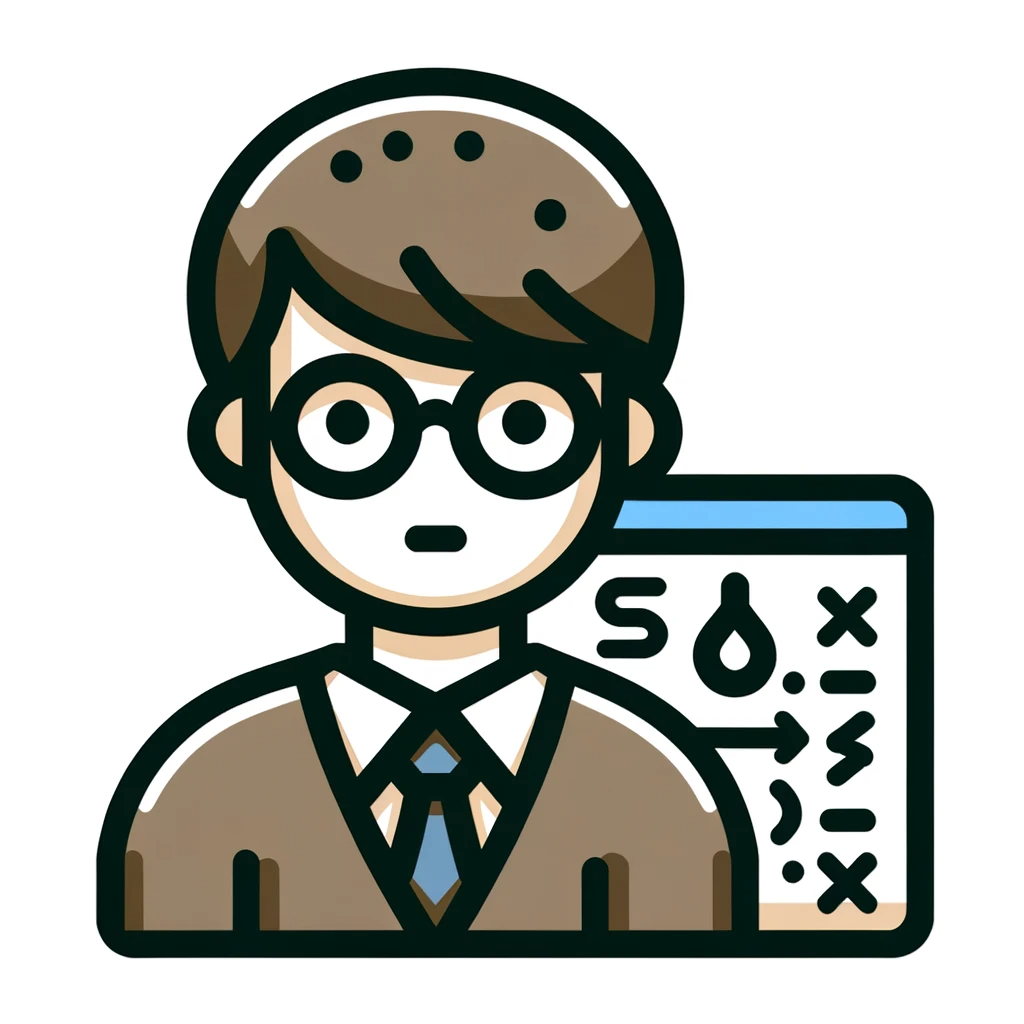
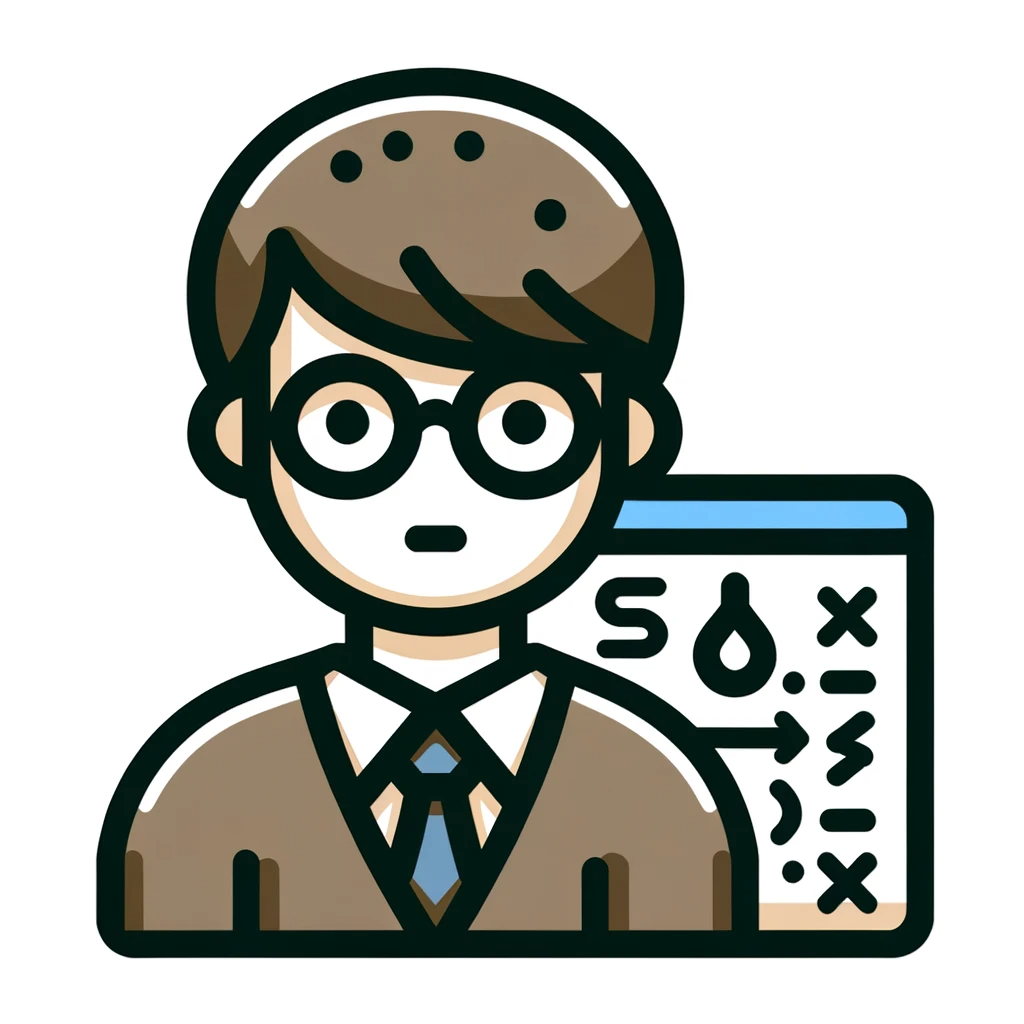
To get the length of a string, use the string.length property.
Get the length of a string with Length
To get the length of a string, add .length to the end of the string. This is one of the properties that strings have and returns the number of characters stored in the string.
string.length
For example, to get the length of the string “javascript”, write as follows.
let str = "javascript";
console.log(str.length); //10
If the string contains a surrogate pair (a combination of two characters used to represent some characters in Unicode), the .length property counts the surrogate pair as two characters by exception. . Therefore, the value returned may differ from the actual number of characters.
Spread operator that correctly counts surrogate pairs
The spread operator (…) is an operator for expanding arrays and objects in JavaScript. By using this, even characters in surrogate pairs can be counted as one character.
let str = "javascript🚀";
console.log([...str].length); // 11
In the case of an array, expand the contents of the array and extract each value.
let arr1 = [1, 2, 3];
let arr2 = [4, 5, 6];
console.log([...arr1, ...arr2]); // [1, 2, 3, 4, 5, 6]
For objects, expand the contents of the object to retrieve each key and value.
let obj1 = {a:1, b:2};
let obj2 = {c:3, d:4};
console.log({...obj1, ...obj2}); // {a:1, b:2, c:3, d:4}
Summary of “How to get the length of a string”
“How to get the length of a string” is summarized below.
- To get the length of a string in JavaScript, use string.length.
- .length is one of the properties of a string and returns the number of characters stored in the string.
- Surrogate pairs are counted as 2 characters, so if you want to count as 1 character, use the spread operator.
To get the length of a string, you can easily use .length. However, be careful if your string contains surrogate pairs.
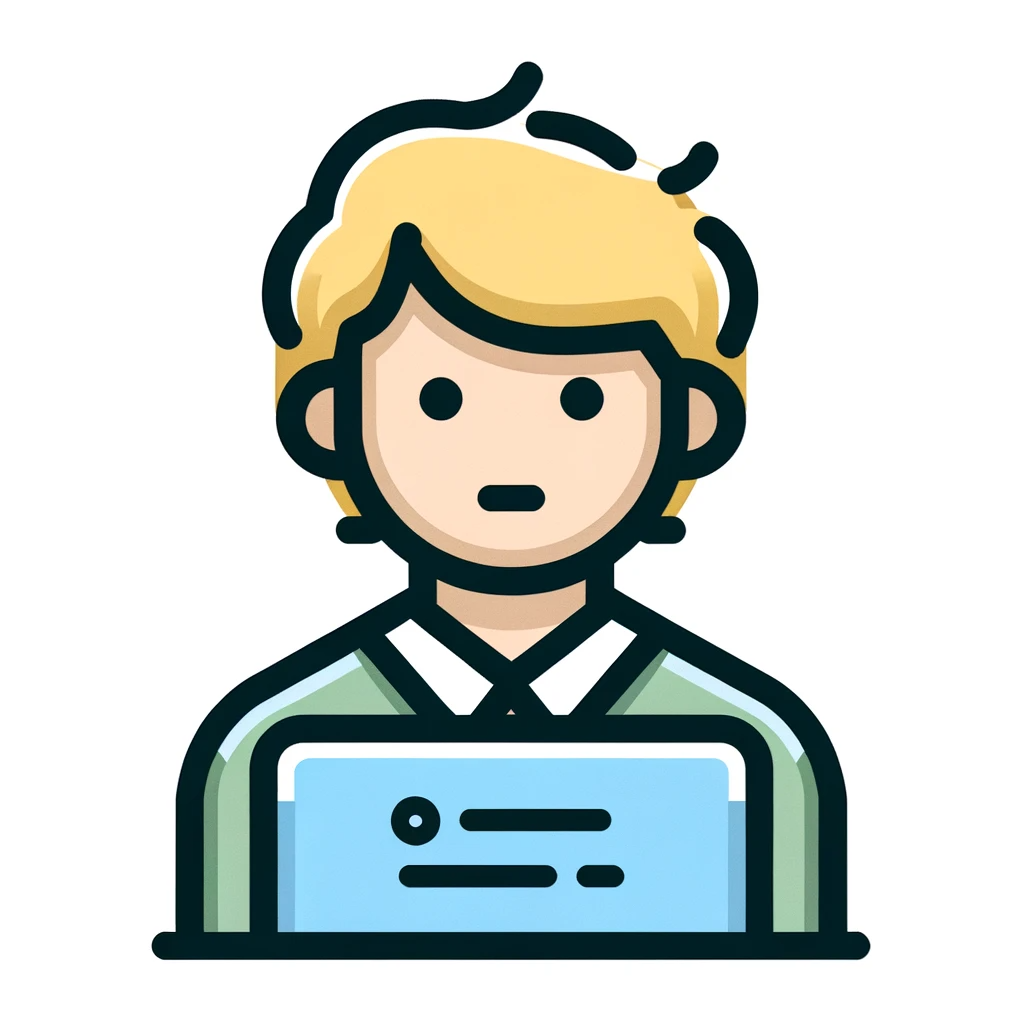
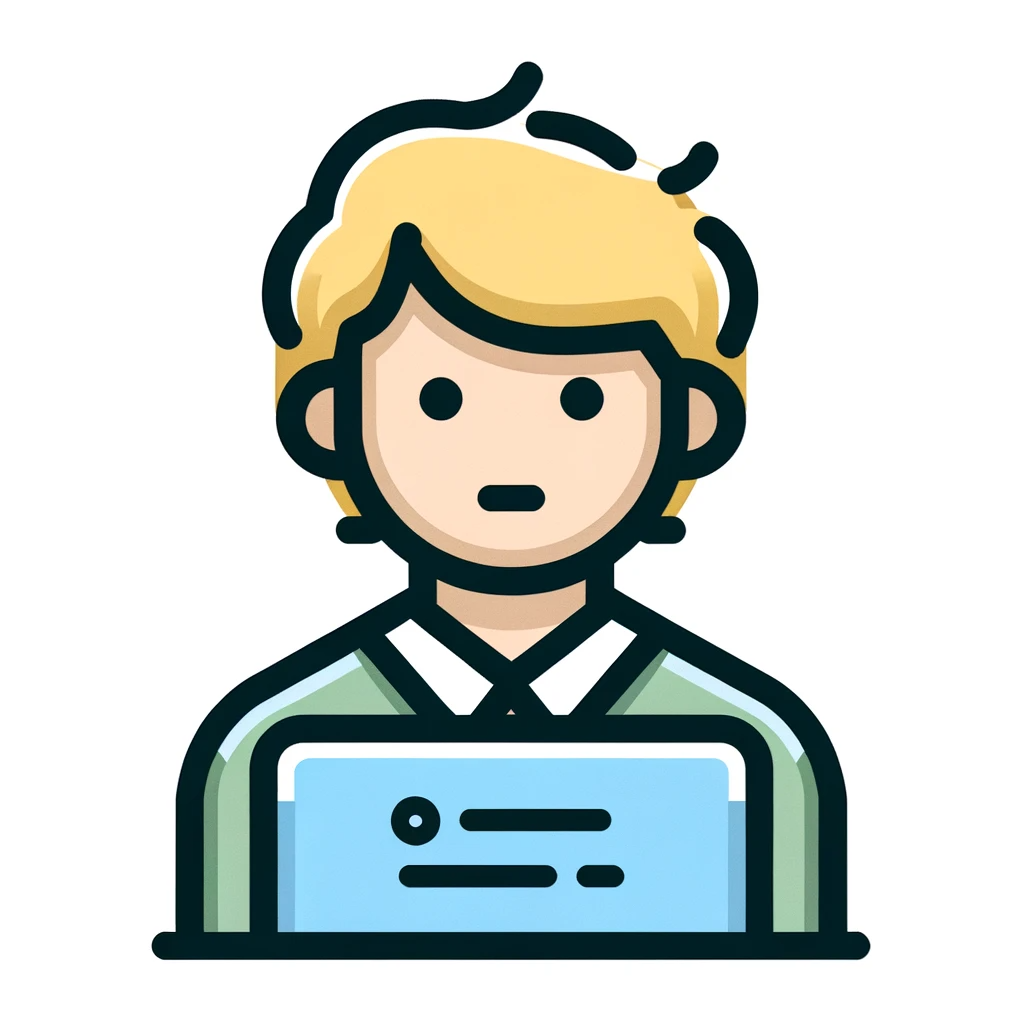
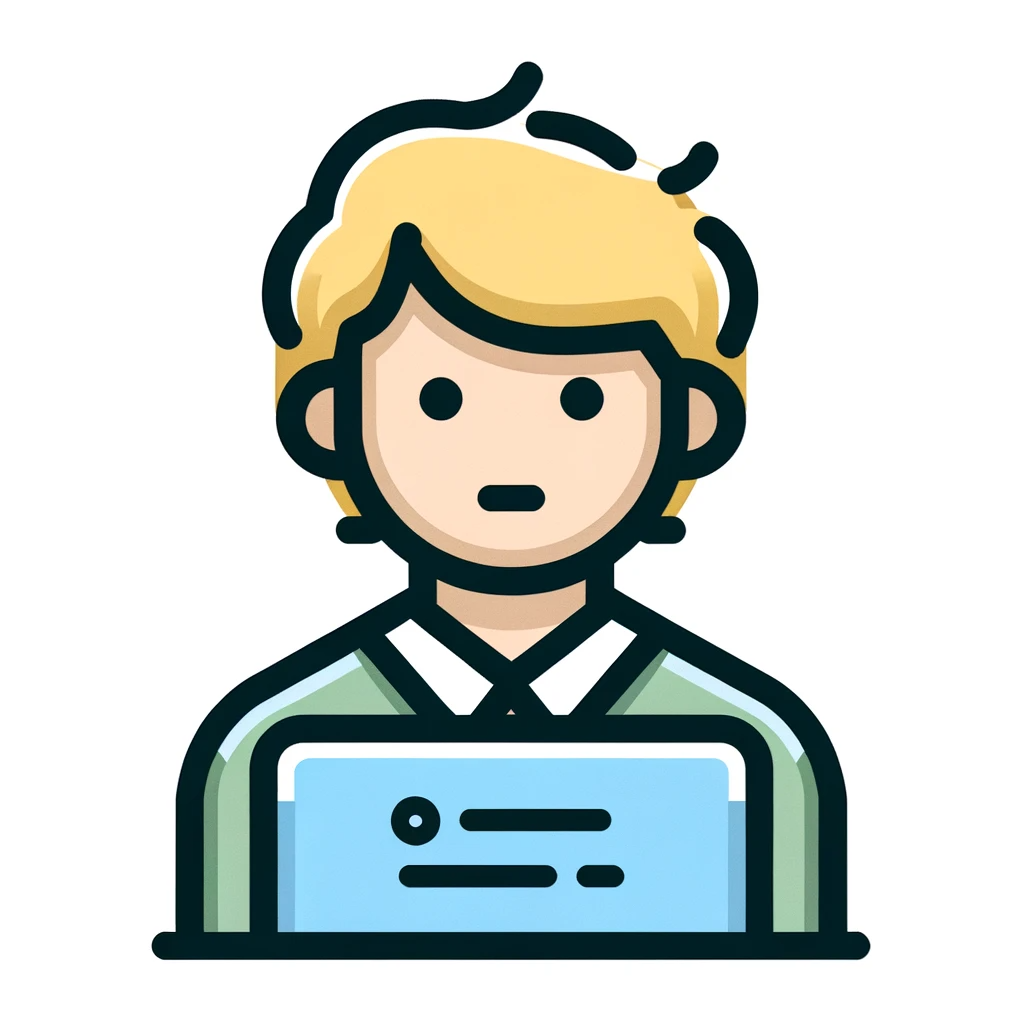
You can easily get the number of characters using the length property!
Comments