This section explains in detail how to obtain the size of an array in JavaScript.
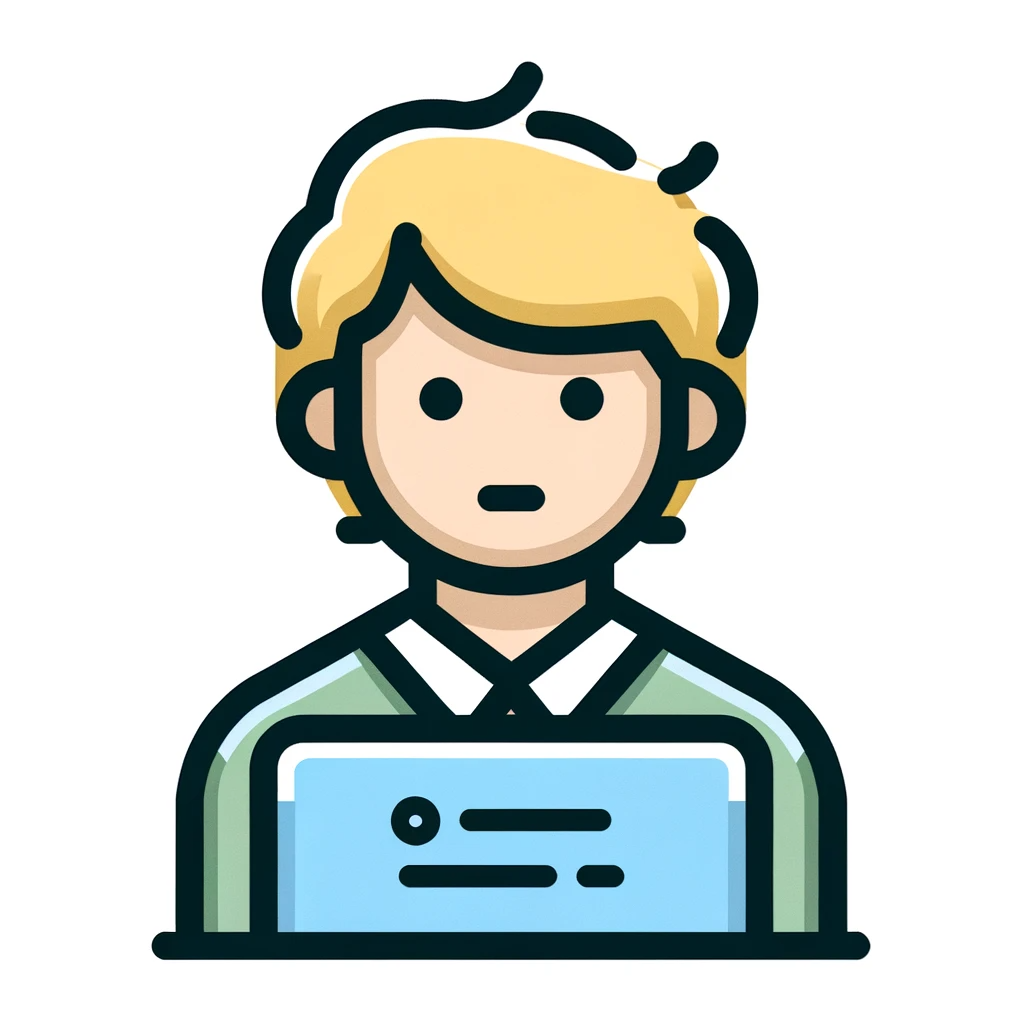
How can I get the size of an array in JavaScript?
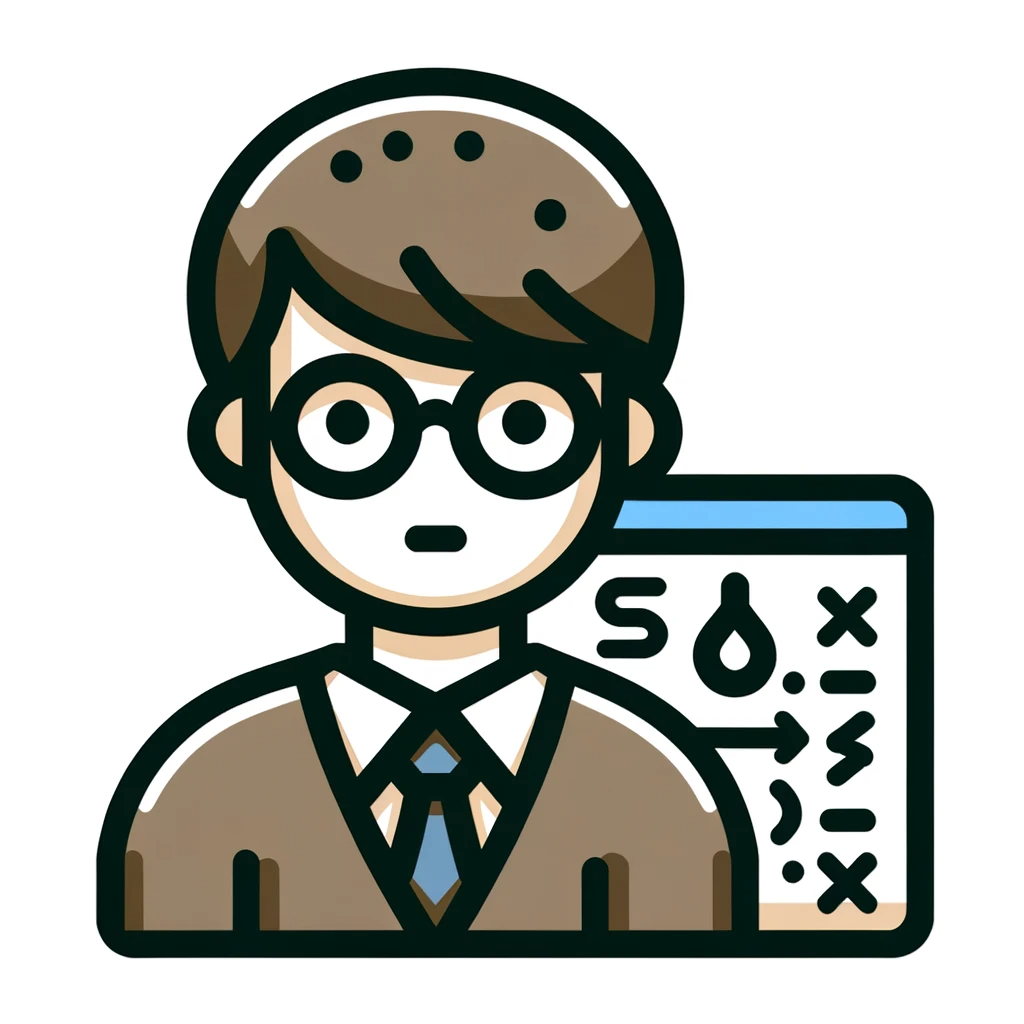
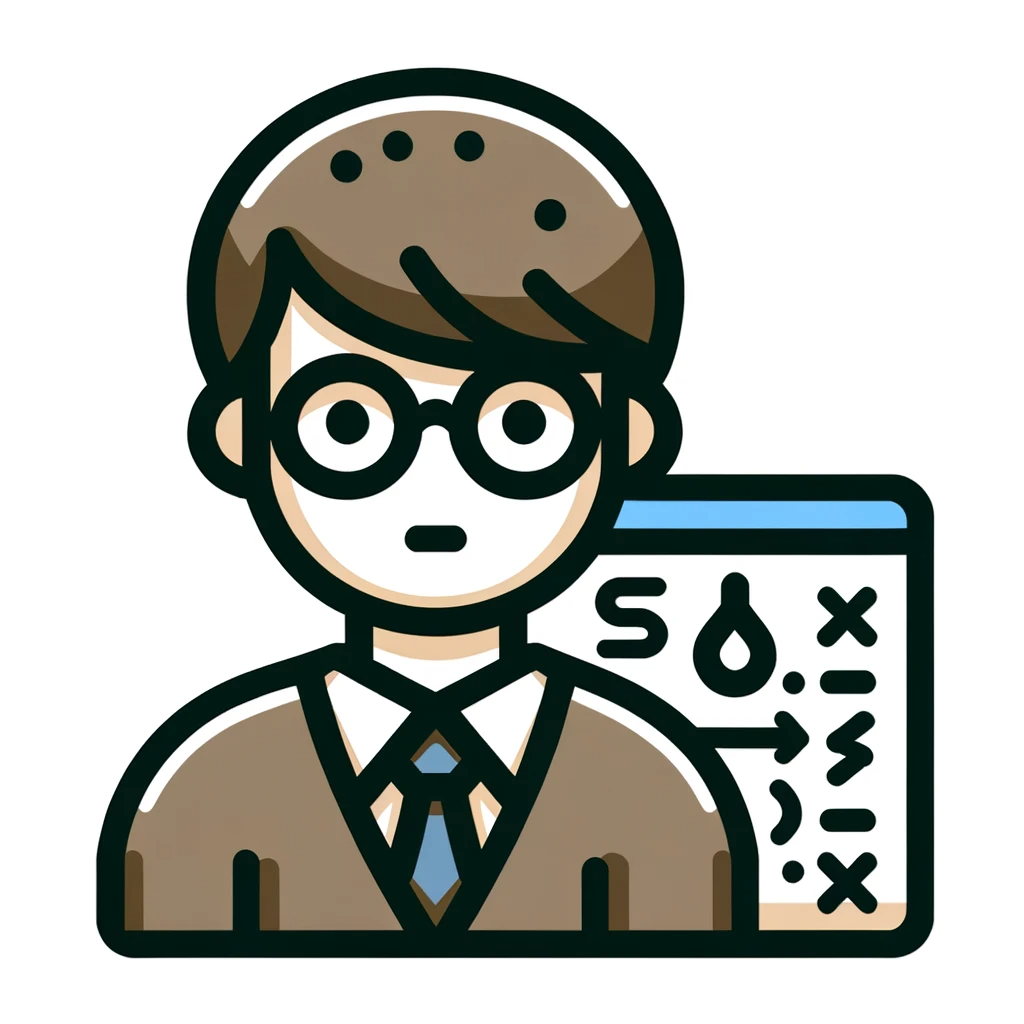
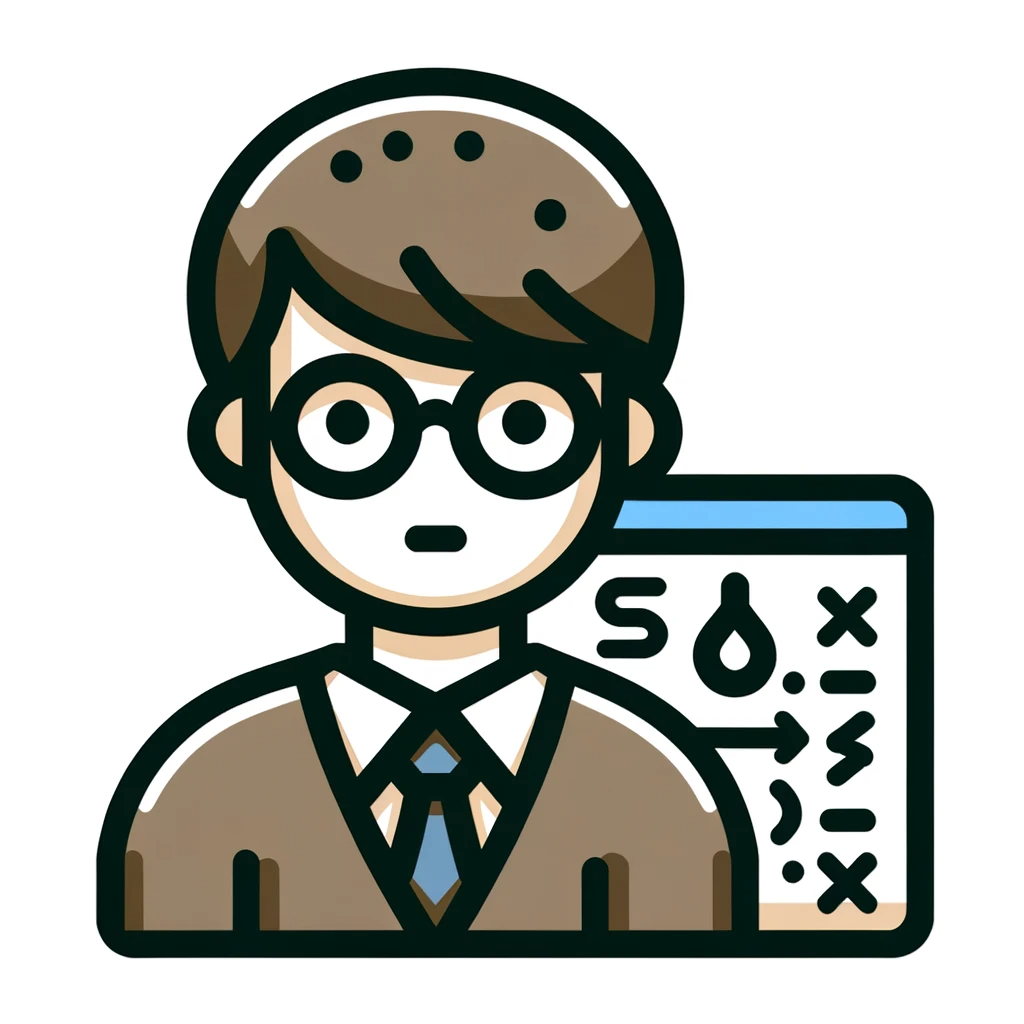
How can I get the size of an array in JavaScript?
Get the size of an array using the length property
To get the length of an array, use the length property .
The length property returns the number of elements stored in the array.
array.length
The length property is automatically updated when you add a new element to the end of the array or remove an element from the array.
// Create array
var fruits = ["Apple", "Banana", "Cherry"];
// Get array length
console.log(fruits.length); // 3
As an example of using the size obtained using the length property, it is often used in a for loop, but if you want to extract an element, you can also use “for…of”.
How to get the elements of an array in order “for…of”
A “ for…of ” loop can be used to retrieve the elements of an array or object.
let fruits = ["Apple", "Banana", "Cherry"];
for (let fruit of fruits) {
console.log(fruit);
}
In the above example, the elements of the fruits array are retrieved in order and displayed using console.log().
The for…of loop can be used not only for arrays, but also for iterable objects that have objects, Maps, Sets, etc.
summary
The following is a summary of how to obtain the size of an array.
- Use the length property to get the size of the array.
- The length property returns the number of elements stored in the array.
- The length property is also automatically updated when you add new elements to the end of the array or remove elements from the array.
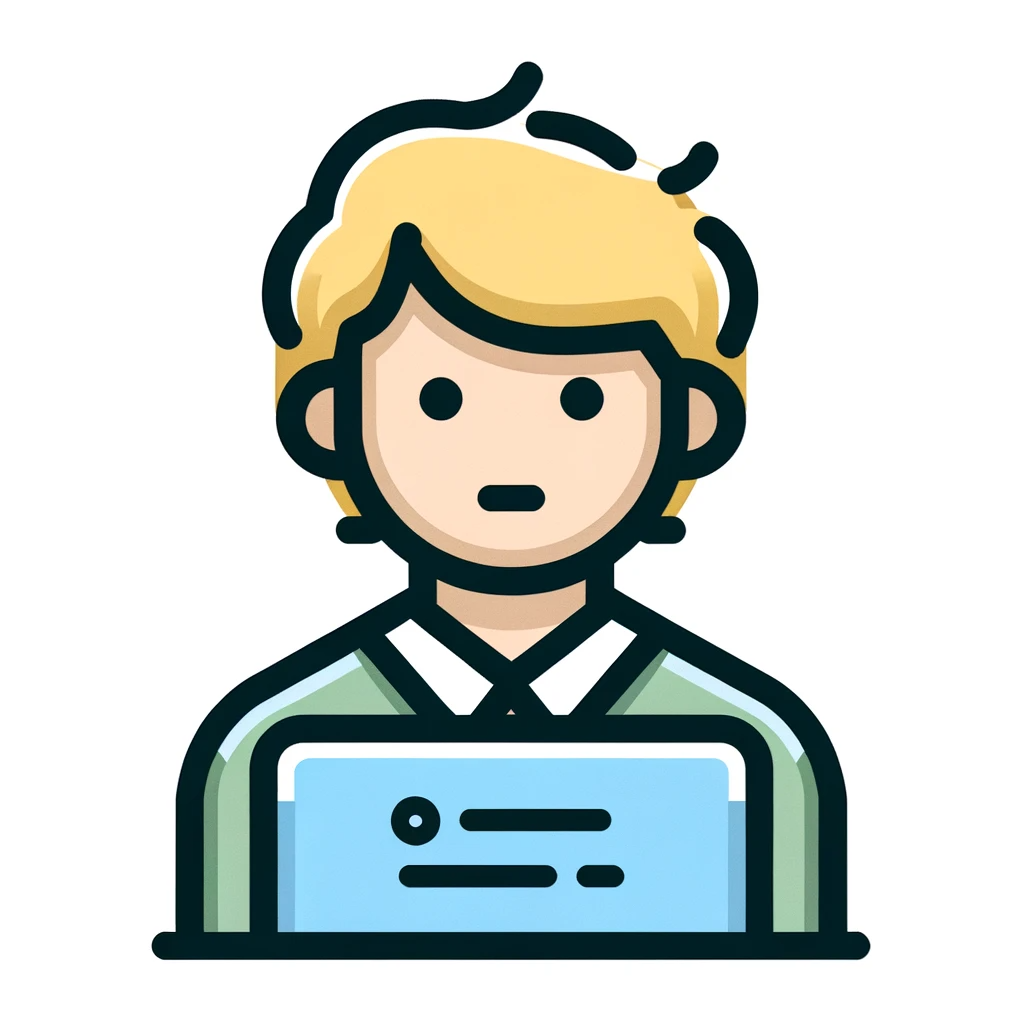
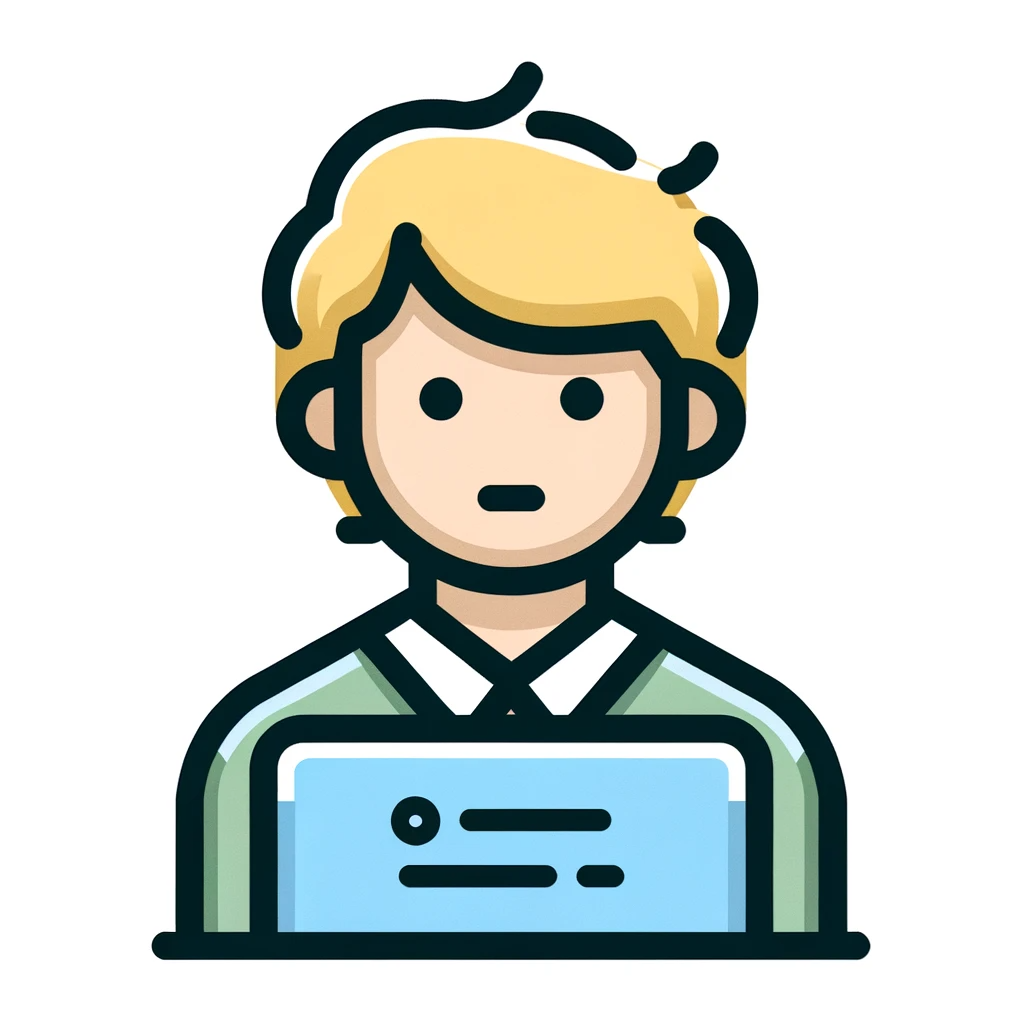
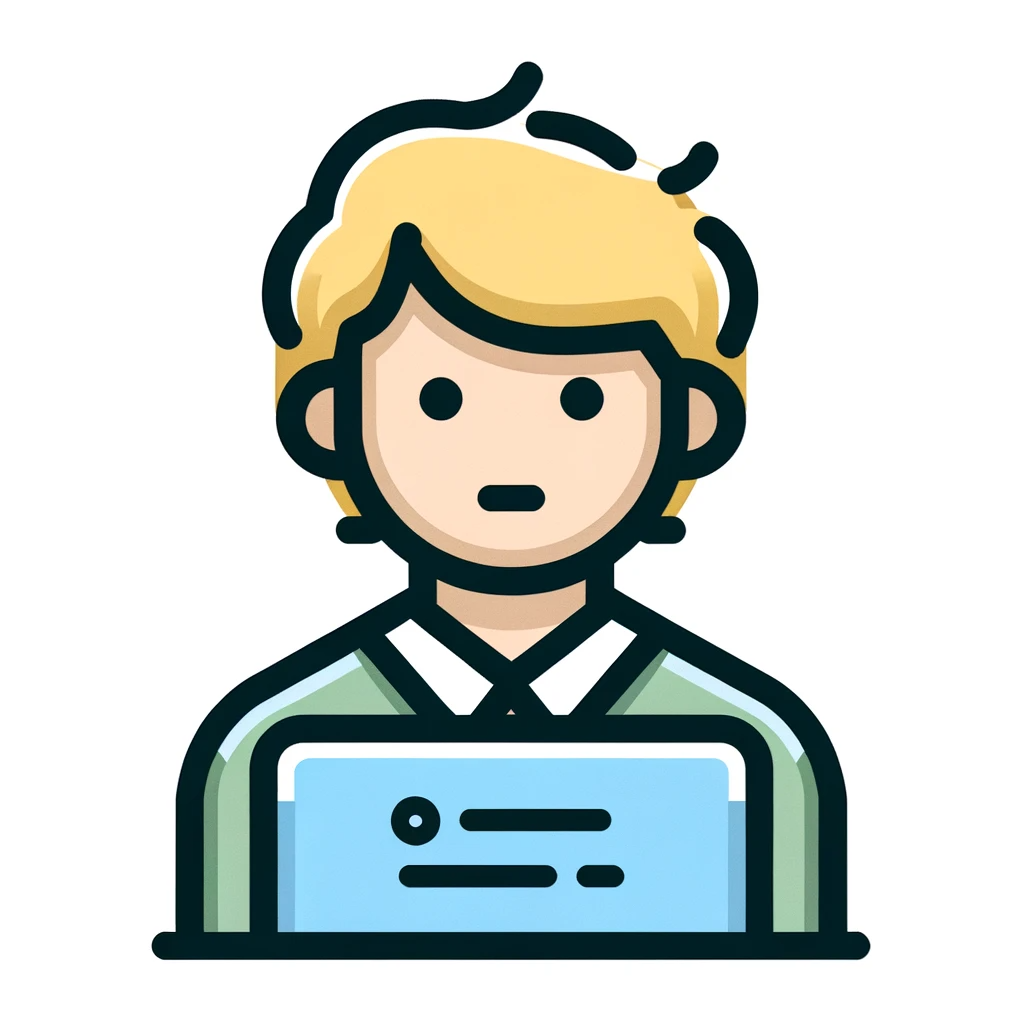
Now you know how to get the size of an array!
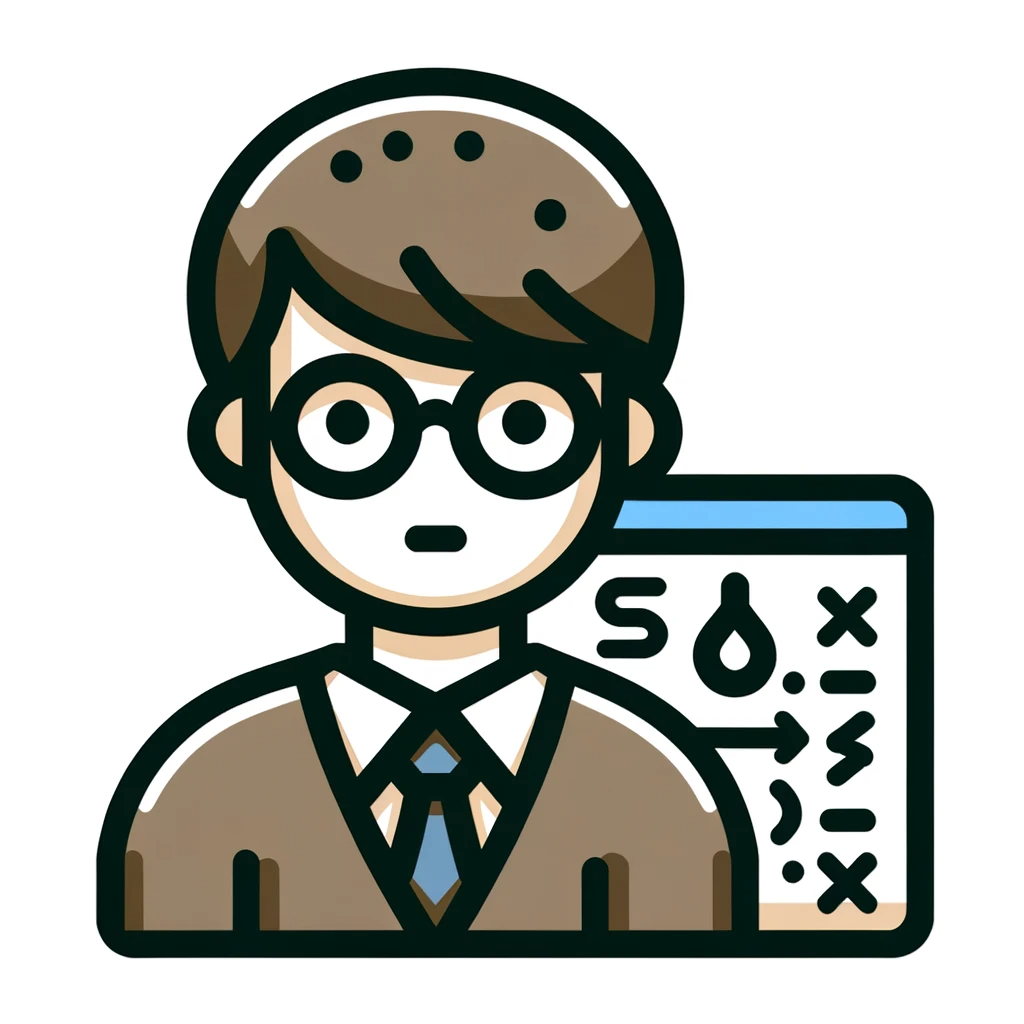
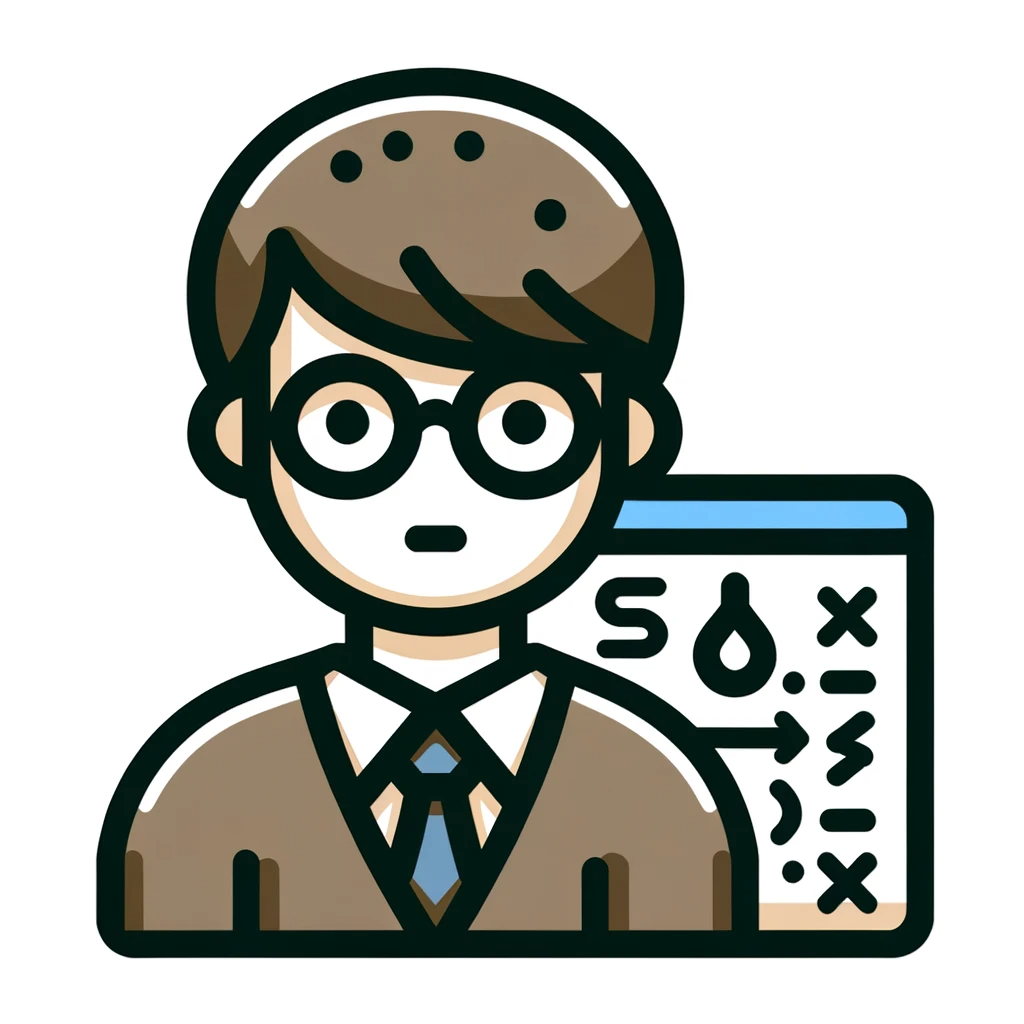
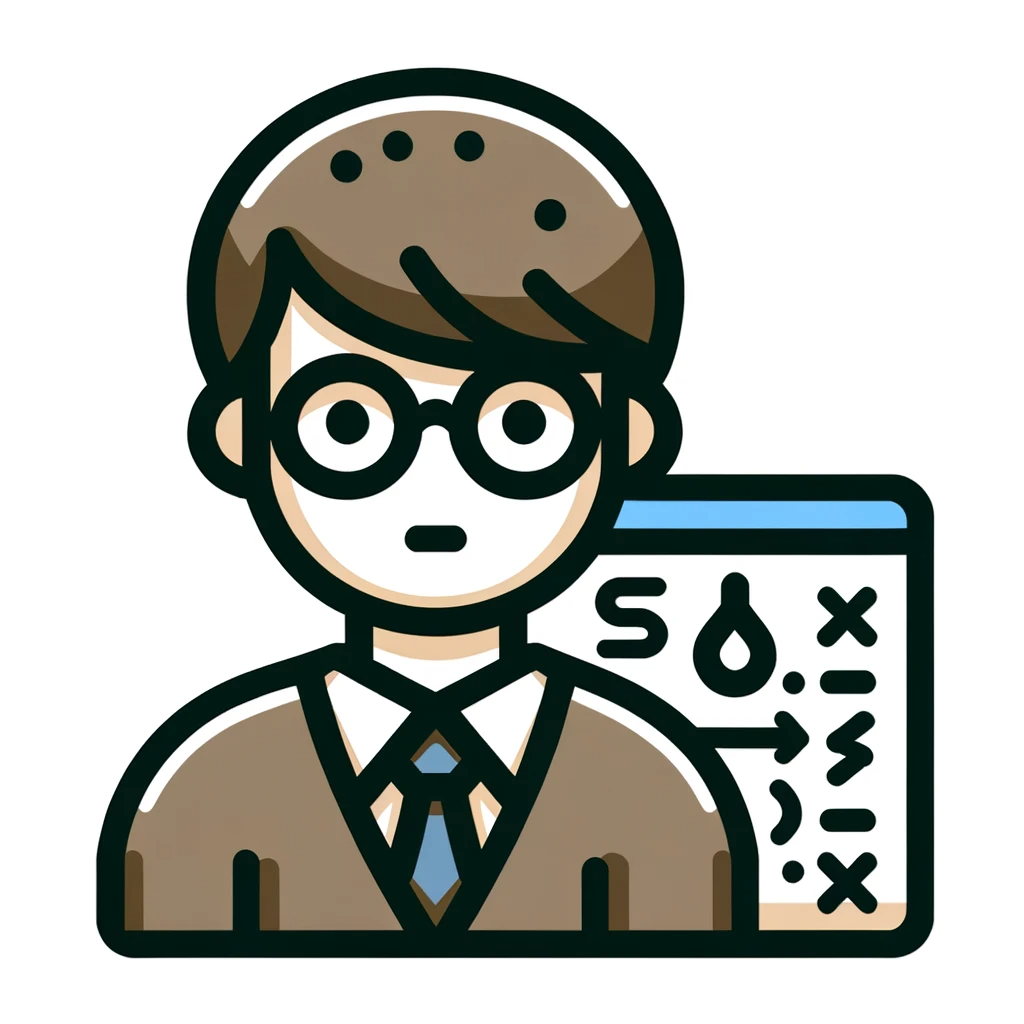
Obtaining the length of an array is important when manipulating and processing arrays, so it is useful to remember.
Comments