We will explain how to perform four arithmetic operations using arithmetic operators in JavaScript.
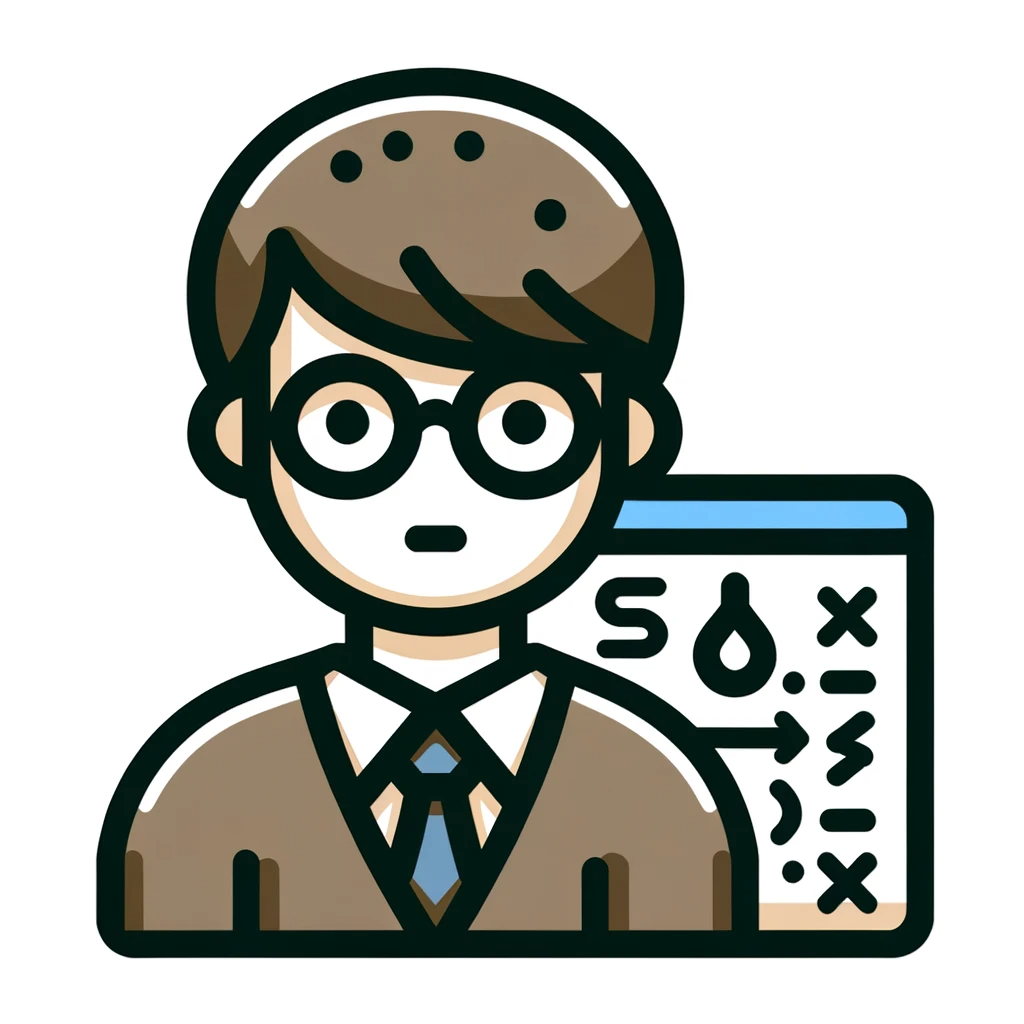
Use arithmetic operators to perform four arithmetic operations.
Arithmetic operators include addition and subtraction.
Types of numbers and arithmetic operators
The types of numbers that can be handled in JavaScript include integers, floating point numbers, and NaN (Not a Number).
Arithmetic operators include addition (+), subtraction (-), multiplication (*), division (/), and finding the remainder (%).
The main arithmetic operators are summarized in a table below.
operator | overview | example |
---|---|---|
+ | addition | 1 + 2 = 3 |
– | subtraction | 3 – 2 = 1 |
* | multiplication | 2 * 3 = 6 |
/ | division | 6/3=2 |
% | surplus | 7% 3 = 1 |
** | power | 2**3=8 |
NaN (Not a Number) is a value that cannot be interpreted as a number . For example, dividing 0 by 0 or attempting to interpret a string as a number will return NaN. It is prone to errors and should be handled with care.
Four arithmetic operations methods
You can use numbers and arithmetic operators to add, subtract, multiply, divide, and find remainders.
For example, you can create a formula using numbers and arithmetic operators, such as 2 + 3, and display the results with console.log().
The sample code below uses arithmetic operators to perform four arithmetic operations.
// Assign 10 to variable x and 5 to variable y.
let x = 10;
let y = 5;
// Output the result of x + y
console.log(x + y); // 15
// Output the x - y result
console.log(x - y); // 5
// Output the result of x * y
console.log(x * y); // 50
// Output x / y results
console.log(x / y); // 2
// Output the result of x % y
console.log(x % y); // 0
// Output the result of x ** y
console.log(x ** y); // 100000
How to perform complex operations
JavaScript provides several functions for performing complex operations.
- Use parentheses to specify the priority of operations
- By using parentheses to specify the priority of operations, complex operations can be expressed easily.
- For example, “3 + 4 * 5” can be multiplied first by writing “3 + (4 * 5)”.
- Combine multiple operators
- Complex operations can be performed by combining multiple operators.
- For example, “(3 + 4) * 5” can be calculated by first calculating “3 + 4” and then multiplying by “5”.
- Perform advanced operations using Math objects
- The Math object provides functions for performing mathematical operations.
- For example, “Math.pow(2, 3)” can calculate “2 to the third power.”
As mentioned above, JavaScript provides functions for performing complex operations. By using them in appropriate combinations, you can easily perform complex operations.
Operations using assignment operators
Assignment operators are used to assign values to variables. The assignment operator specifies the variable on the left side and the value on the right side. Additionally, some assignment operators can perform four arithmetic operations at the same time .
There are the following types of assignment operators:
- “=” operator
- This is an assignment operator for assigning a value to a variable.
- For example, if you write “x = 5”, 5 will be assigned to the variable x.
- “+=” operator
- This operator adds and assigns the value on the right side to the variable on the left side.
- For example, if you write “x += 5”, the value obtained by adding 5 to the variable x will be assigned to x again.
- “-=” operator
- This operator subtracts and assigns the value on the right side from the variable on the left side.
- For example, if you write “x -= 5”, the value obtained by subtracting 5 from the variable x will be assigned to x again.
- “*=” operator
- This operator multiplies and assigns the variable on the left side by the value on the right side.
- For example, if you write “x *= 5”, the value obtained by multiplying the variable x by 5 will be assigned to x again.
- “/=” operator
- This operator divides and assigns the variable on the left side by the value on the right side.
- For example, if you write “x /= 5”, the value obtained by dividing the variable x by 5 will be assigned to x again.
By using assignment operators, you can write calculation results for variables concisely. You can also combine multiple assignment operators. For example, addition and assignment can be performed at the same time, such as “x += 5”.
Below is a sample code for the assignment operator.
let x = 5; // Assign 5 to variable x
x += 3; // Add 3 to x and substitute (the value of x will be 8)
console.log(x);
x -= 2; // Subtract 2 from x and substitute (x value becomes 6)
console.log(x);
x *= 4; // Multiply x by 4 and substitute (the value of x will be 24)
console.log(x);
x /= 3; // Divide x by 3 and substitute (x value will be 8)
console.log(x);
In this code, we first assign 5 to the variable x. We then use the “+=” operator, then the “-=” operator, then the “*=” operator, and finally the “/=” operator on x. By using each operator, the value of variable x is changed concisely. Finally, you can see that the value of variable x is 8.
summary
This time, we learned about the four arithmetic operations used in JavaScript, remainders, and powers. These operators allow you to manipulate numbers.
- JavaScript allows you to perform four arithmetic operations using numbers and arithmetic operators.
- Numeric values include integers, floating point numbers, and NaN.
- Arithmetic operators include addition (+), subtraction (-), multiplication (*), division (/), and finding the remainder (%).
- To perform complex operations, you can use parentheses to specify operator precedence.
- Complex operations can be performed by combining multiple operators.
- The Math object provides functions for performing mathematical operations.
- Some assignment operators can perform four arithmetic operations simultaneously.
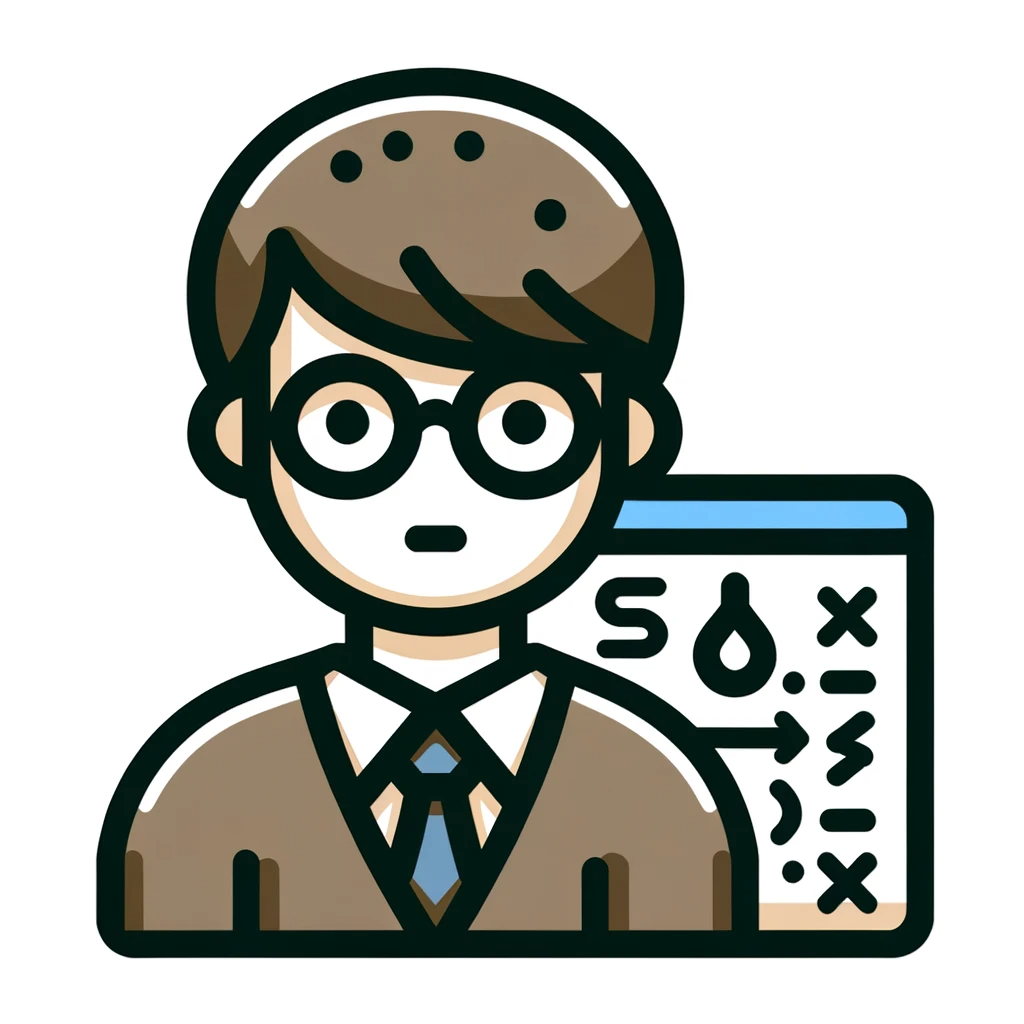
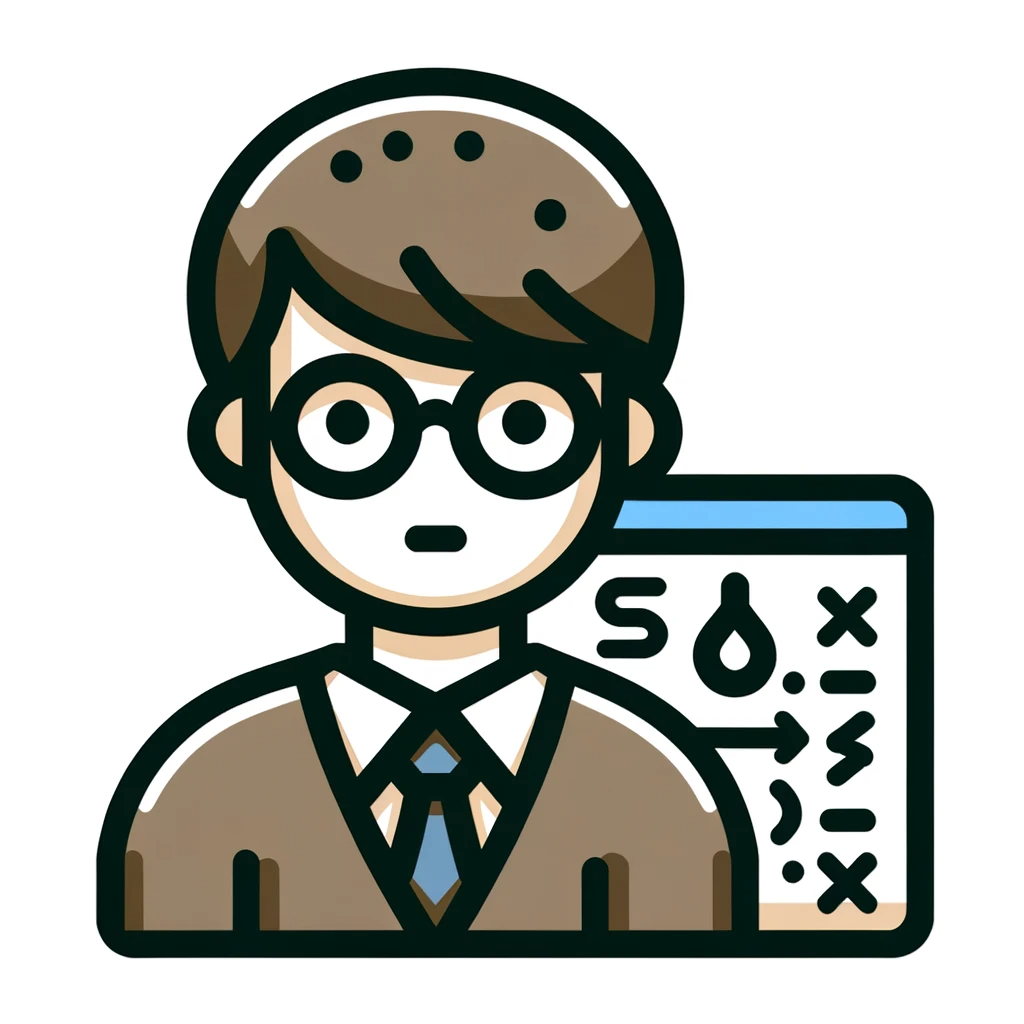
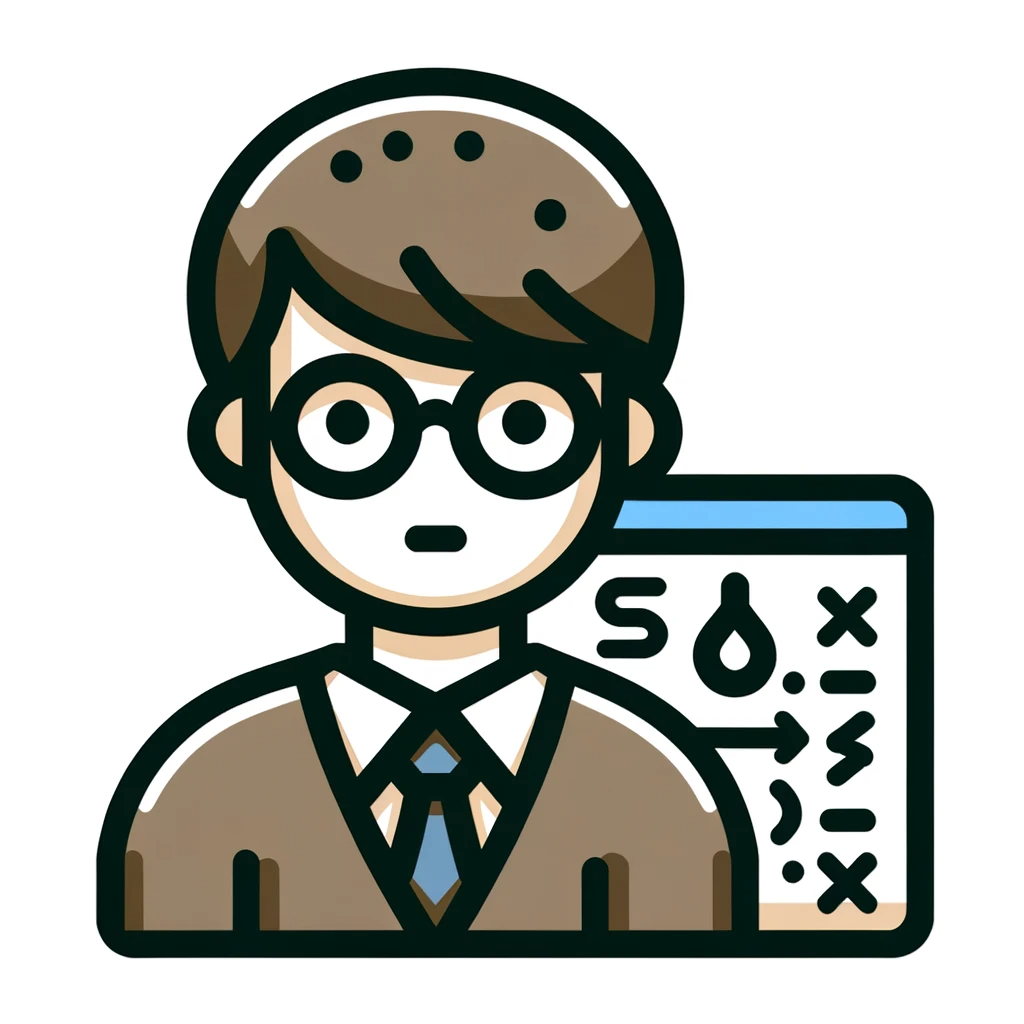
By using arithmetic operators, you can easily perform four arithmetic operations on numbers.
By using assignment operators, you can save yourself the trouble of updating values.
Use this article as a reference to master how to use these operators and use them for efficient programming.
Also, when calculating numbers, it is important to pay attention to the value range and the number of digits after the decimal point.
Comments