This article explains how to convert JSON using JavaScript.
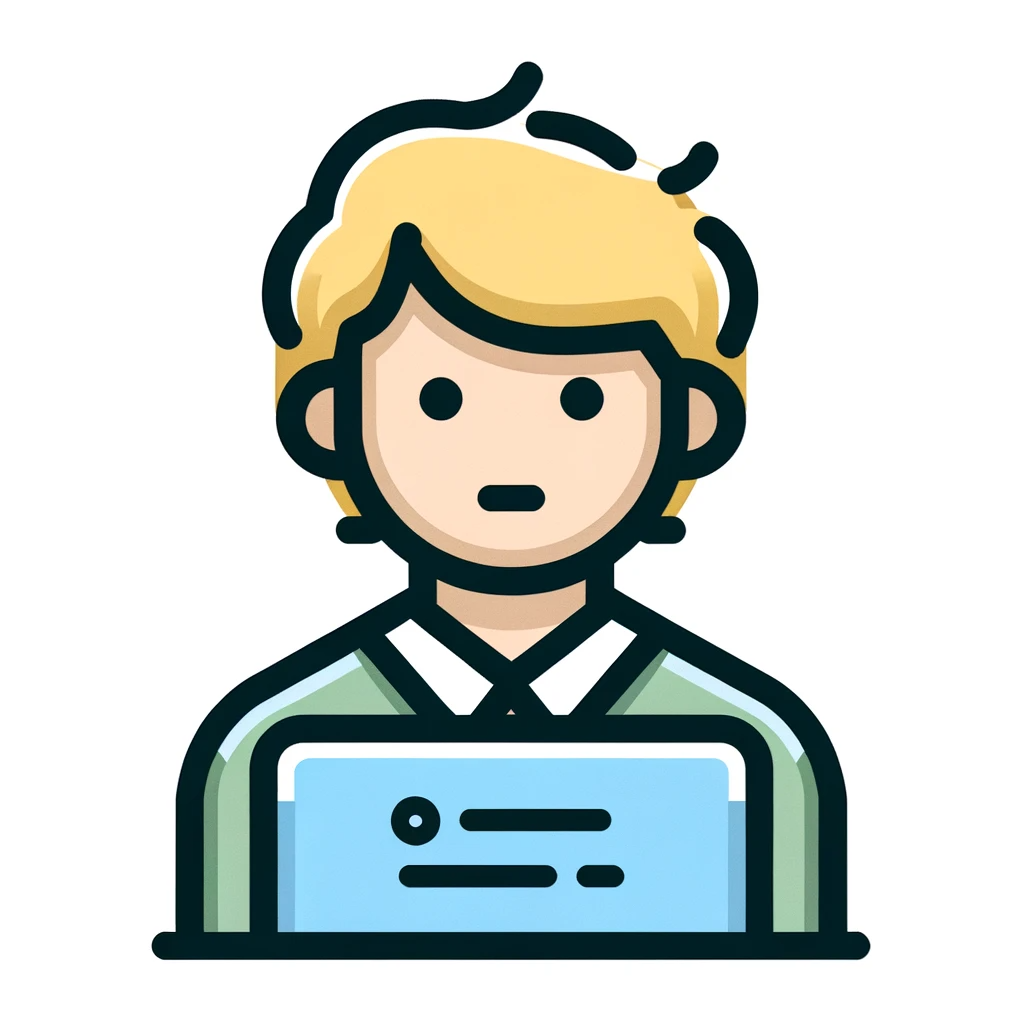
How can I convert JSON?
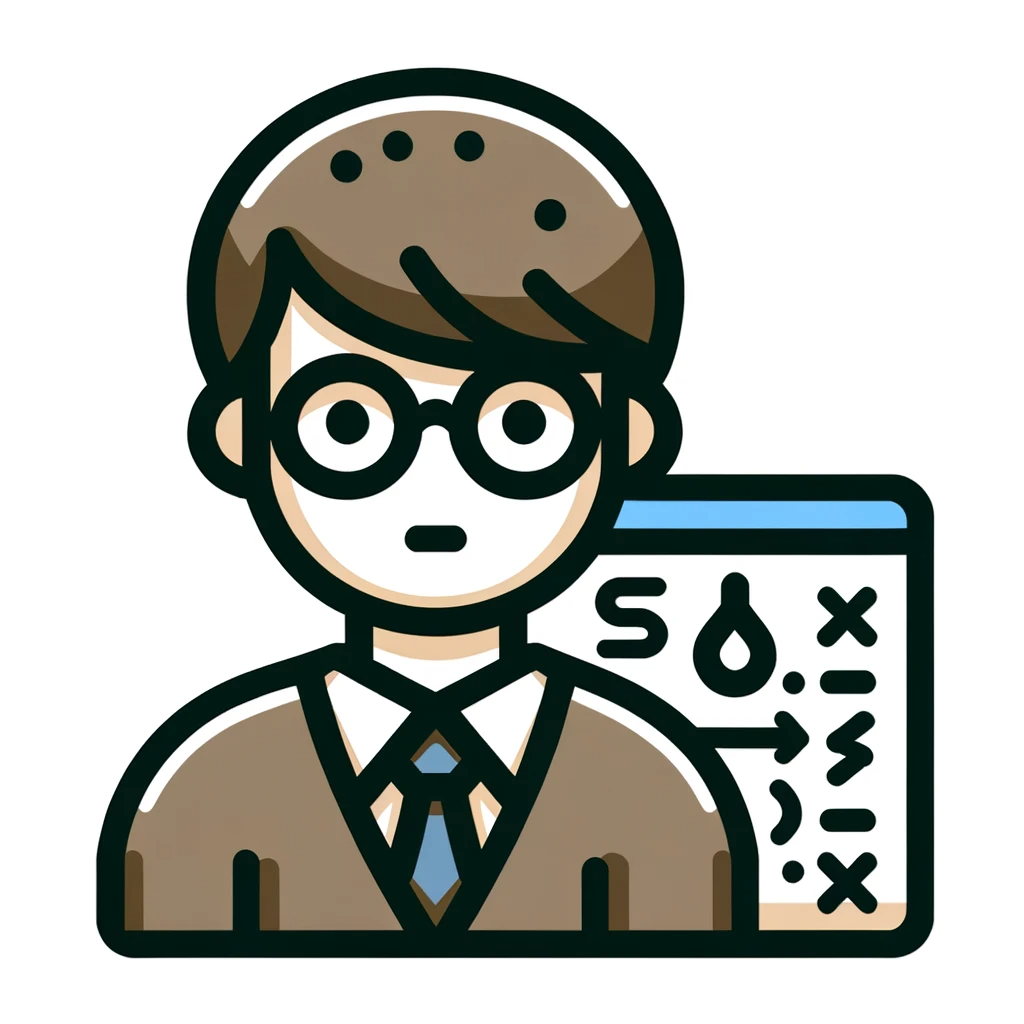
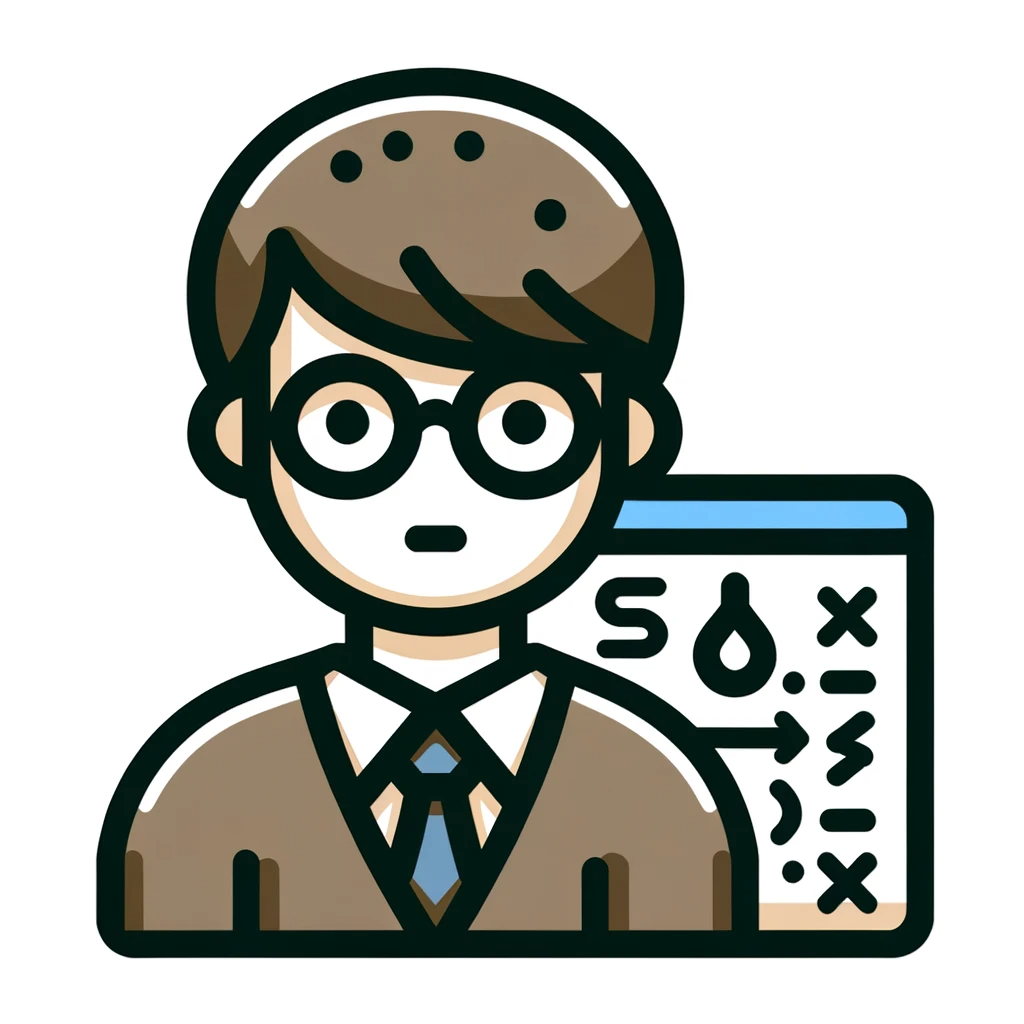
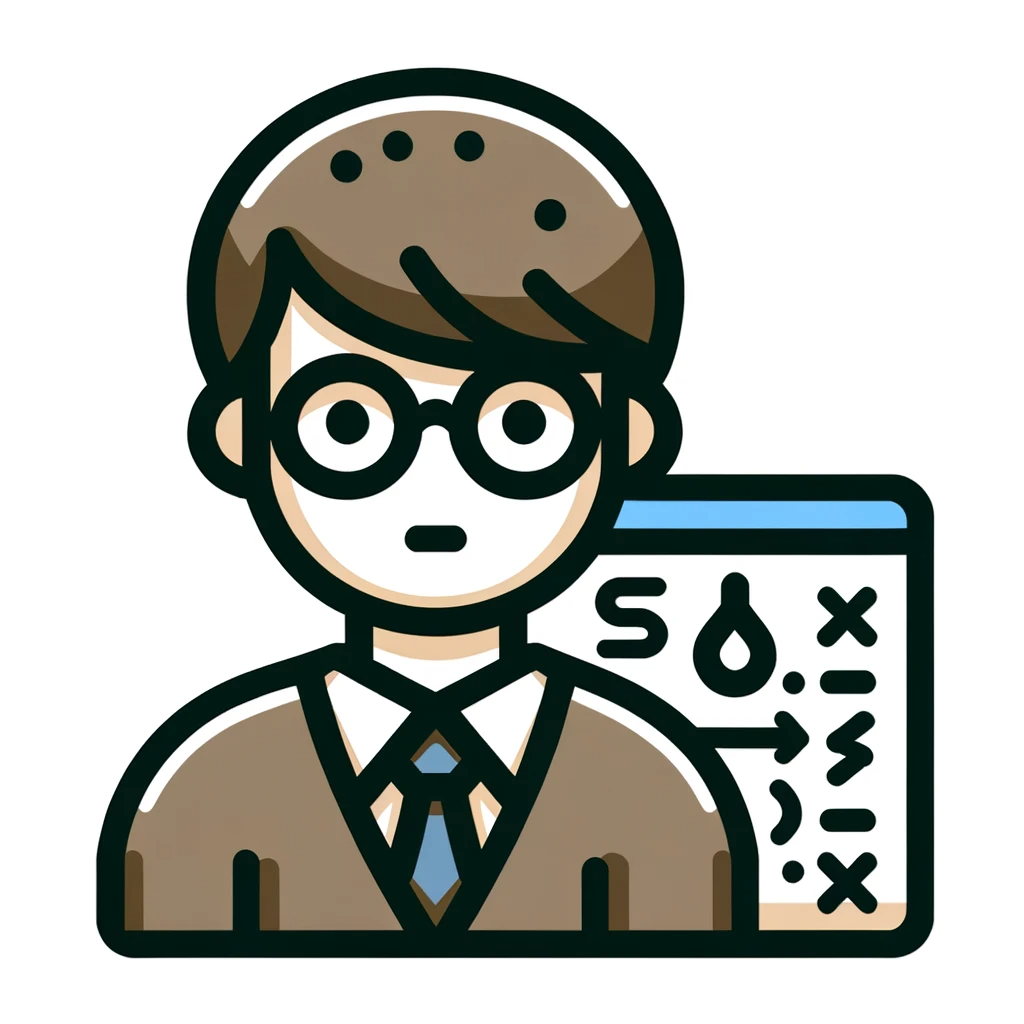
JSON conversion can be easily done using JSON.stringify() and JSON.parse().
JSON explanation
JSON (JavaScript Object Notation) is a lightweight data exchange format, and like XML, it is used for data exchange on the Web. JSON is very popular in web services and API development because it has a concise syntax and is a format that is easy to read for both humans and machines.
JSON is very easy to use in JavaScript because it is based on JavaScript’s object literal syntax. JSON can represent property name and value pairs, arrays, nested objects, etc. For example, the following JSON string represents a person named John with a name and age in a human- and machine-readable format.
{
"name": "John",
"age": 30
}
JSON is very useful when developing web applications with JavaScript because it can be converted to and from JavaScript objects.
Converting objects to JSON using JSON.stringify
JSON.stringify() method is used to convert an object to a JSON string.
This method takes a JavaScript object as one argument and returns a JSON string.
JSON.stringify(object)
For example, use JSON.stringify() like this:
const obj = { name: "John", age: 30 };
const json = JSON.stringify(obj);
console.log(json);
This will output a JSON string like this:
{“name”:”John”,”age”:30}
How to use JSON.parse to convert a JSON string to a JavaScript object
JSON.parse method is used to convert JSON string to JavaScript object. This method takes a JSON string as one argument and returns a JavaScript object.
JSON.parse(JSON string)
For example, use JSON.parse like this:
const json = '{"name":"John","age":30}';
const obj = JSON.parse(json);
console.log(obj);
This will output a JavaScript object like this:
{ name: “John”, age: 30 }
summary
The following is a summary of how to convert objects to JSON and JSON to objects.
- JSON is a data format commonly used in web application development.
- You can use JSON.stringify to convert JavaScript objects to JSON strings.
- You can use JSON.parse to convert a JSON string to a JavaScript object.
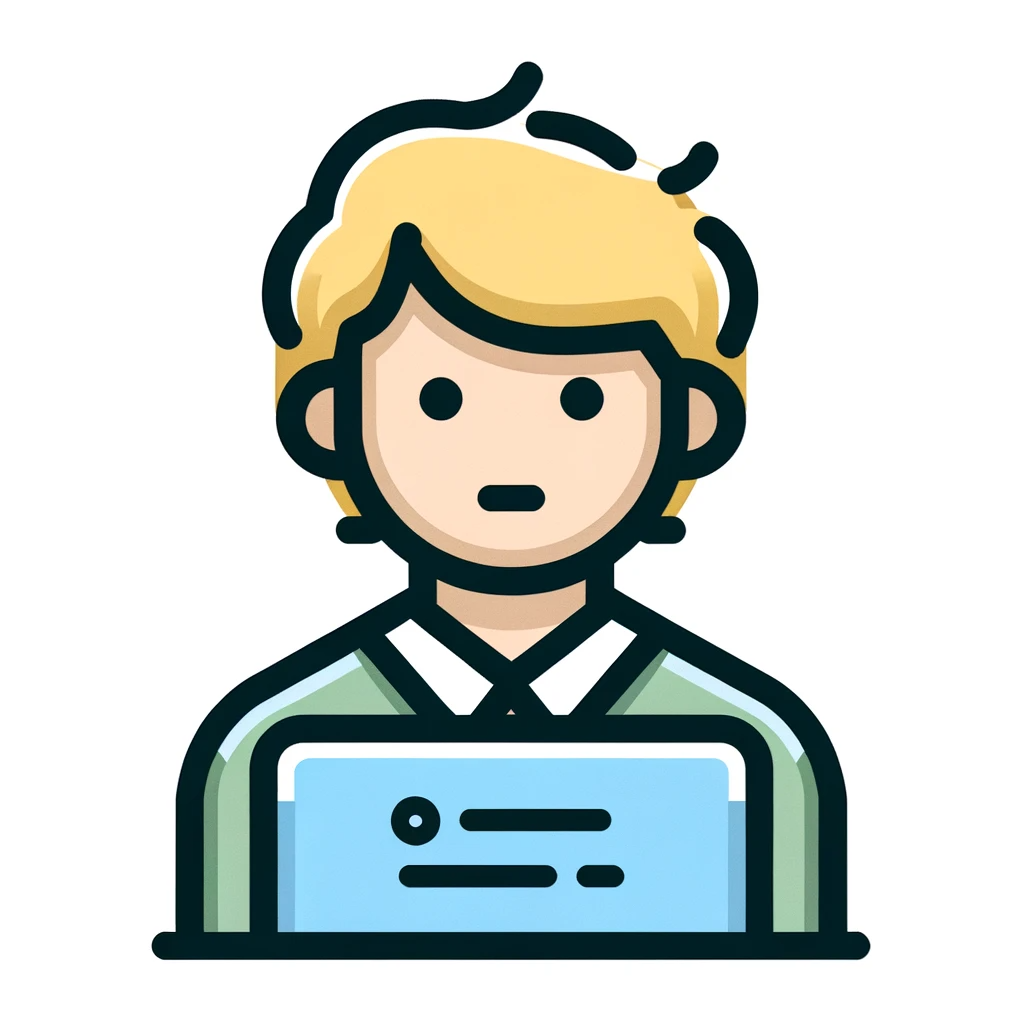
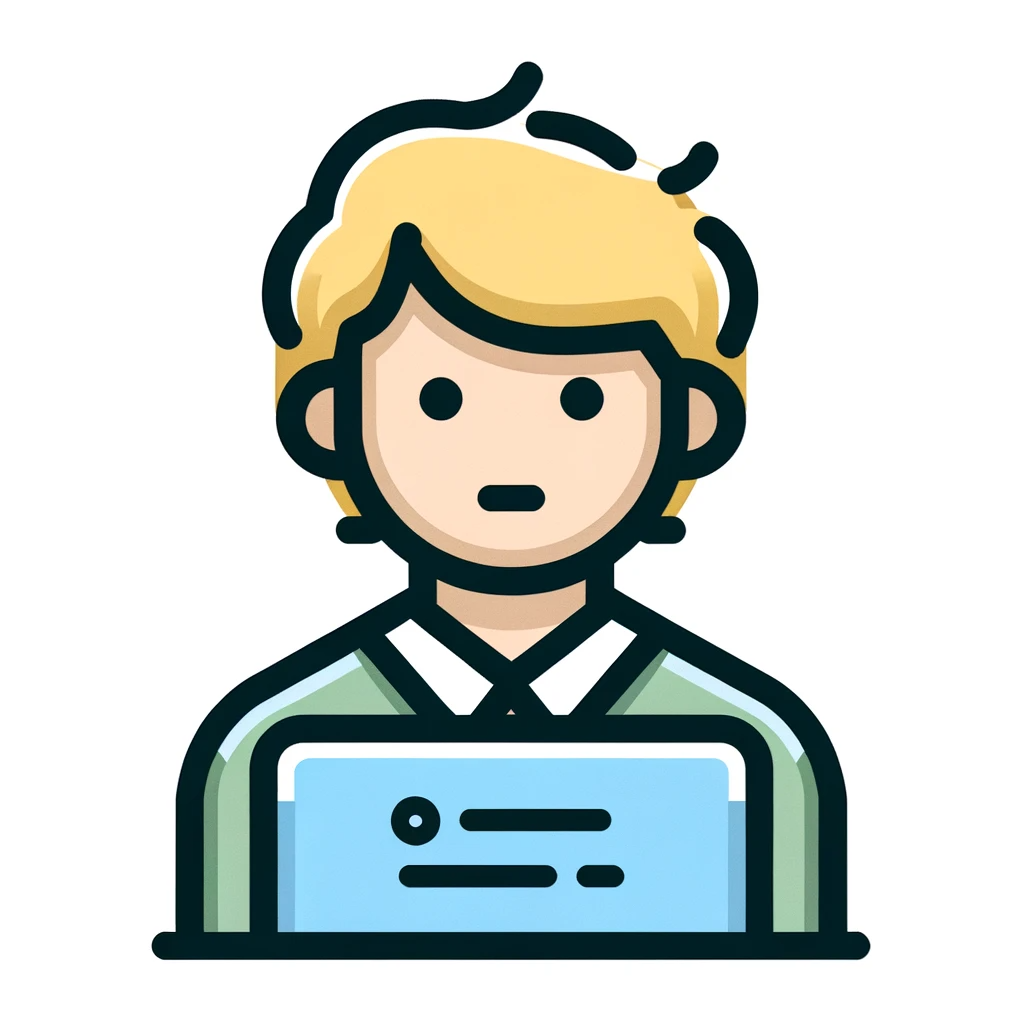
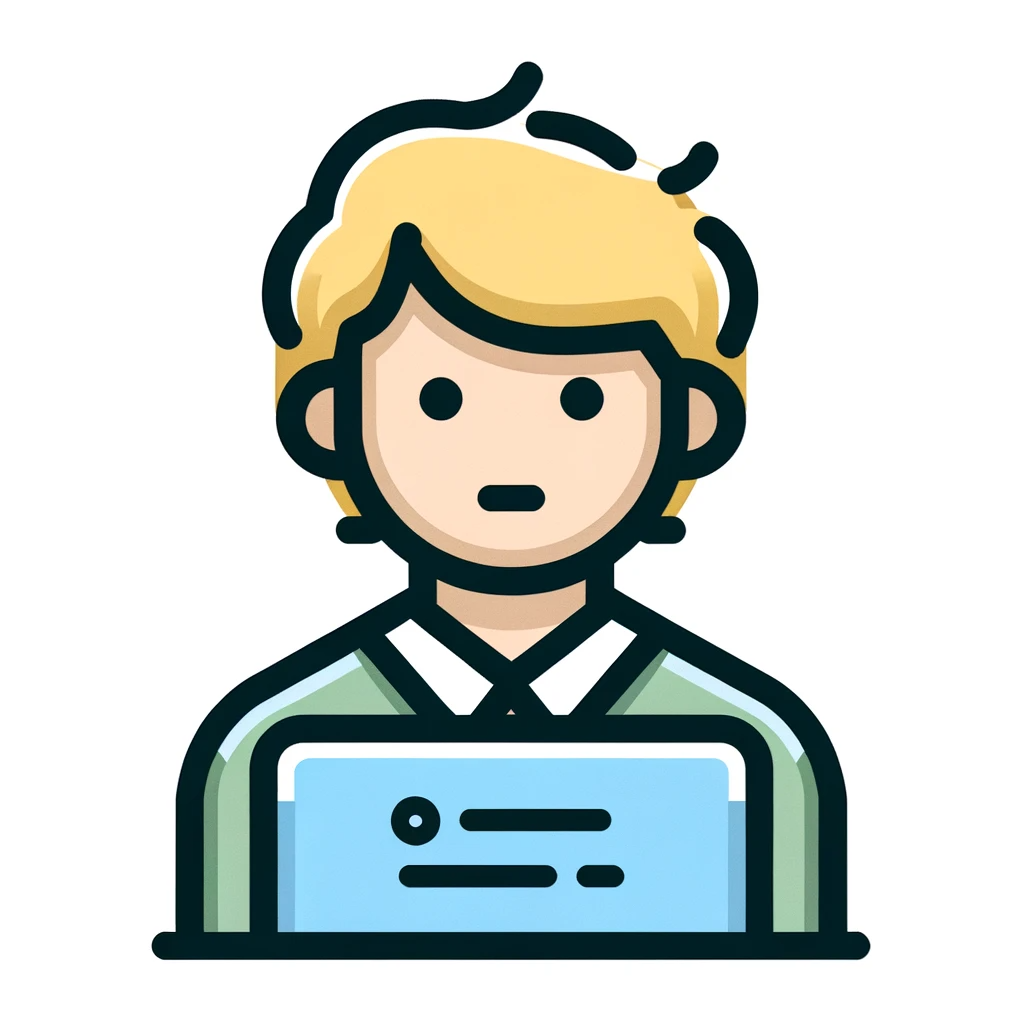
I now have a good understanding of how to convert JSON!
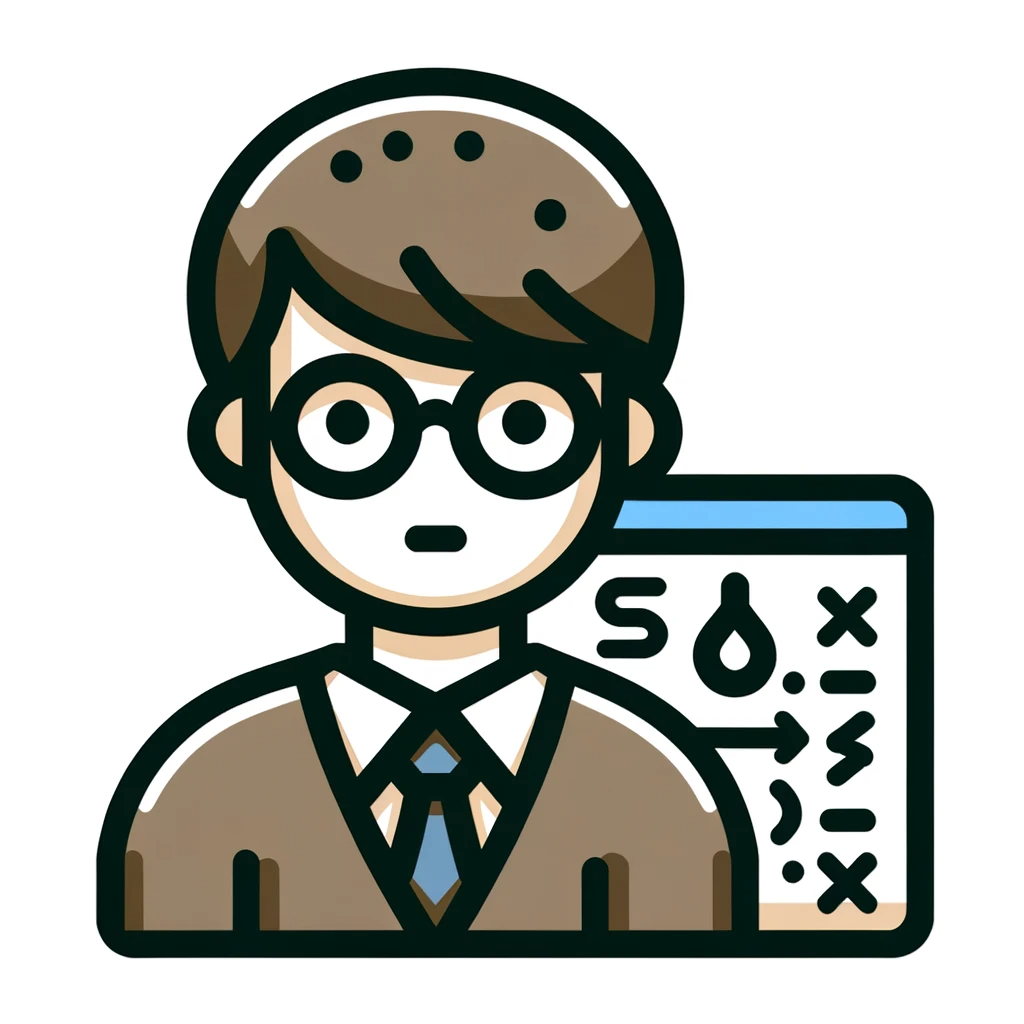
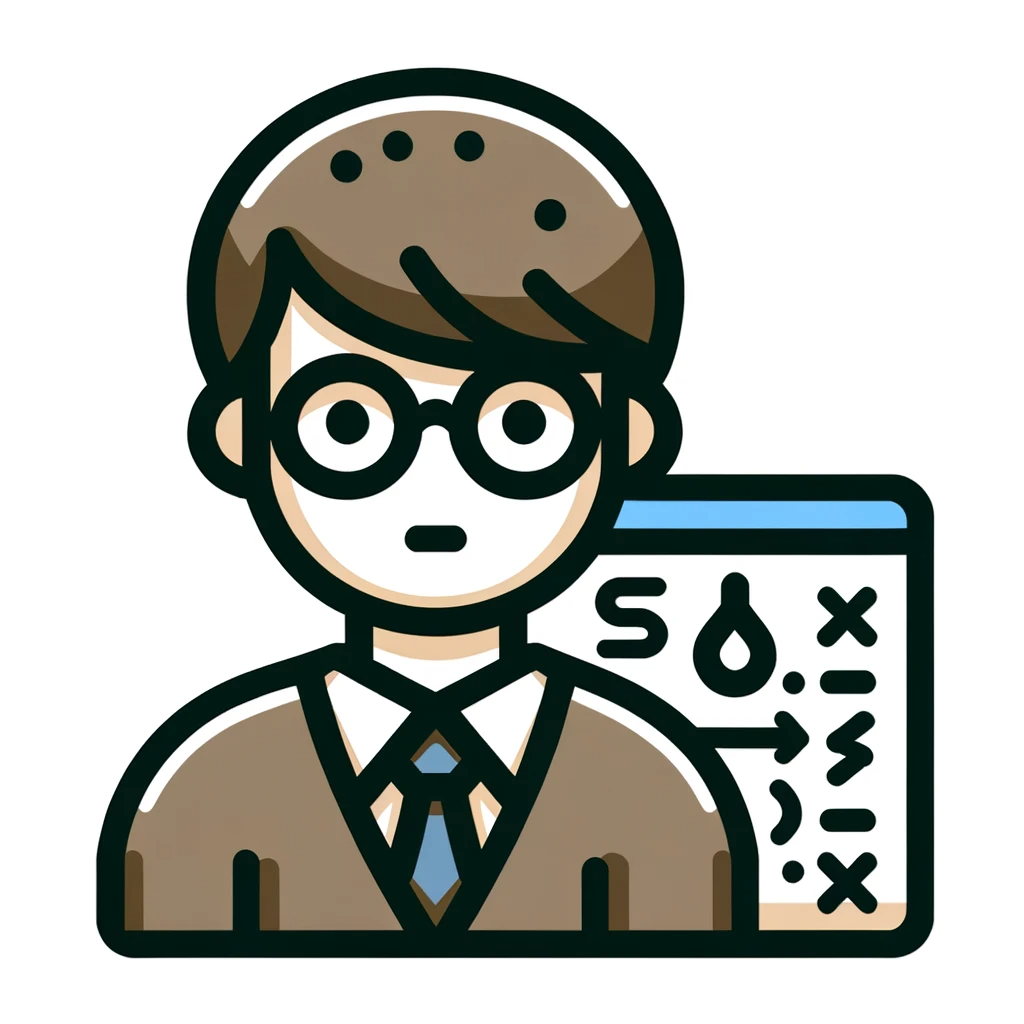
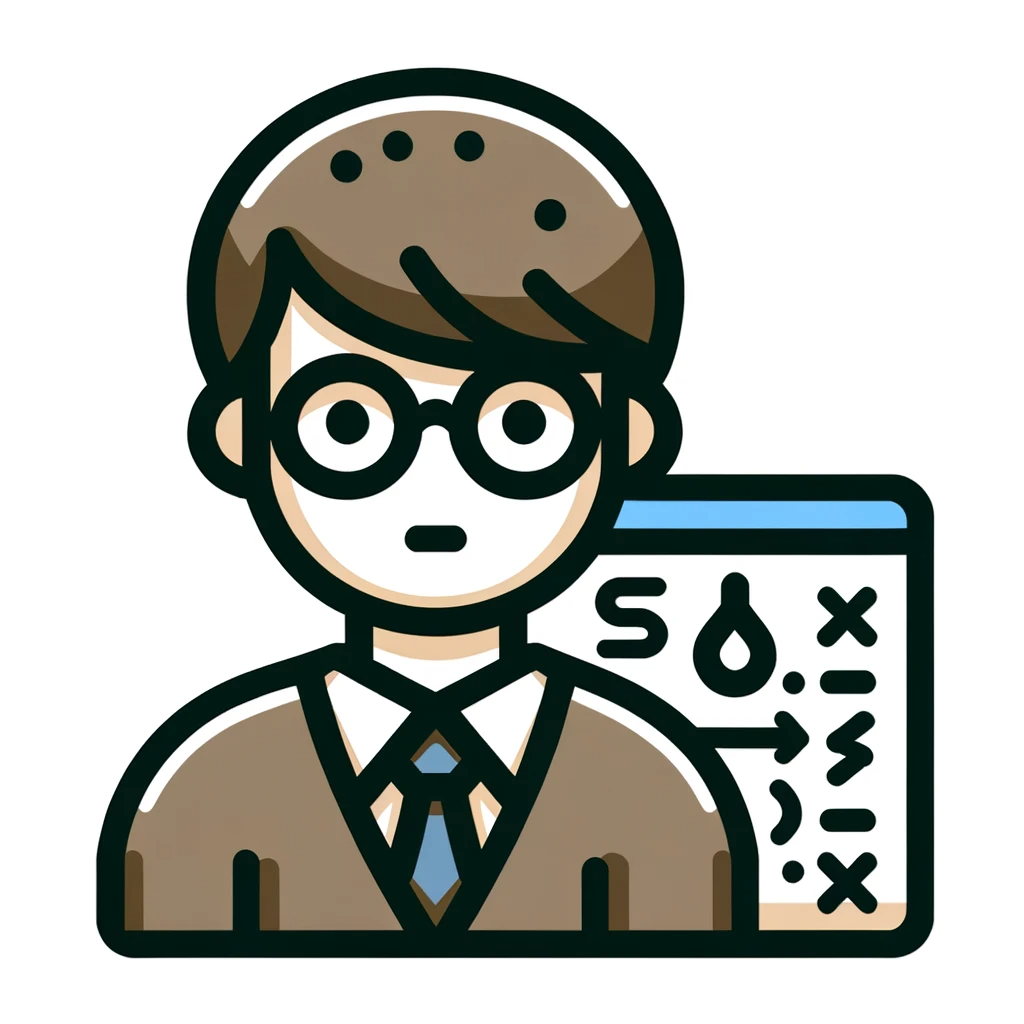
In addition to learning how to use JSON.stringify and JSON.parse, learn about JSON syntax and use it when actually working with data.
JSON is an important data format in web application development, and learning how to convert JSON with JavaScript is essential for web development skills. Learn how to convert JSON and try using it in web application development.
Comments