This article explains how to combine array elements using commas, spaces, etc. in JavaScript.
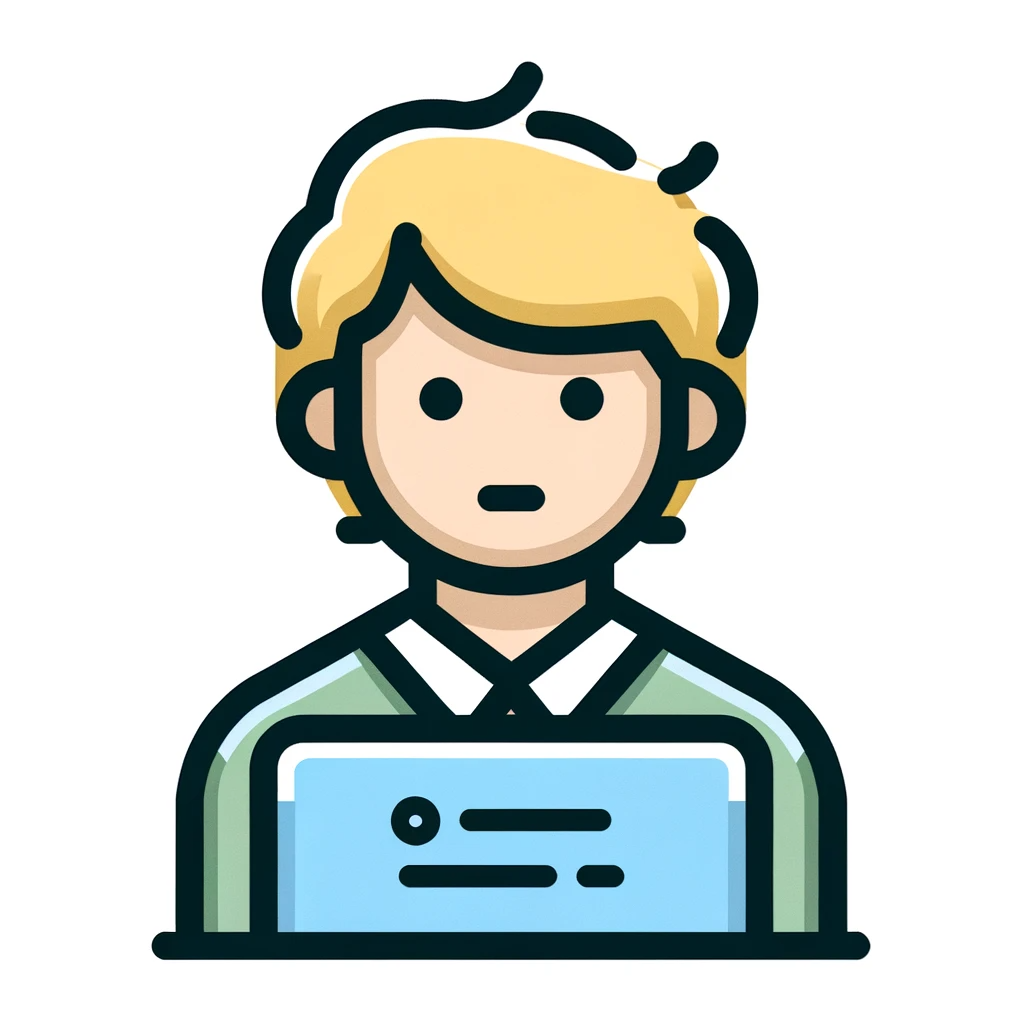
Is there a way to combine array elements by separating them with commas or spaces?
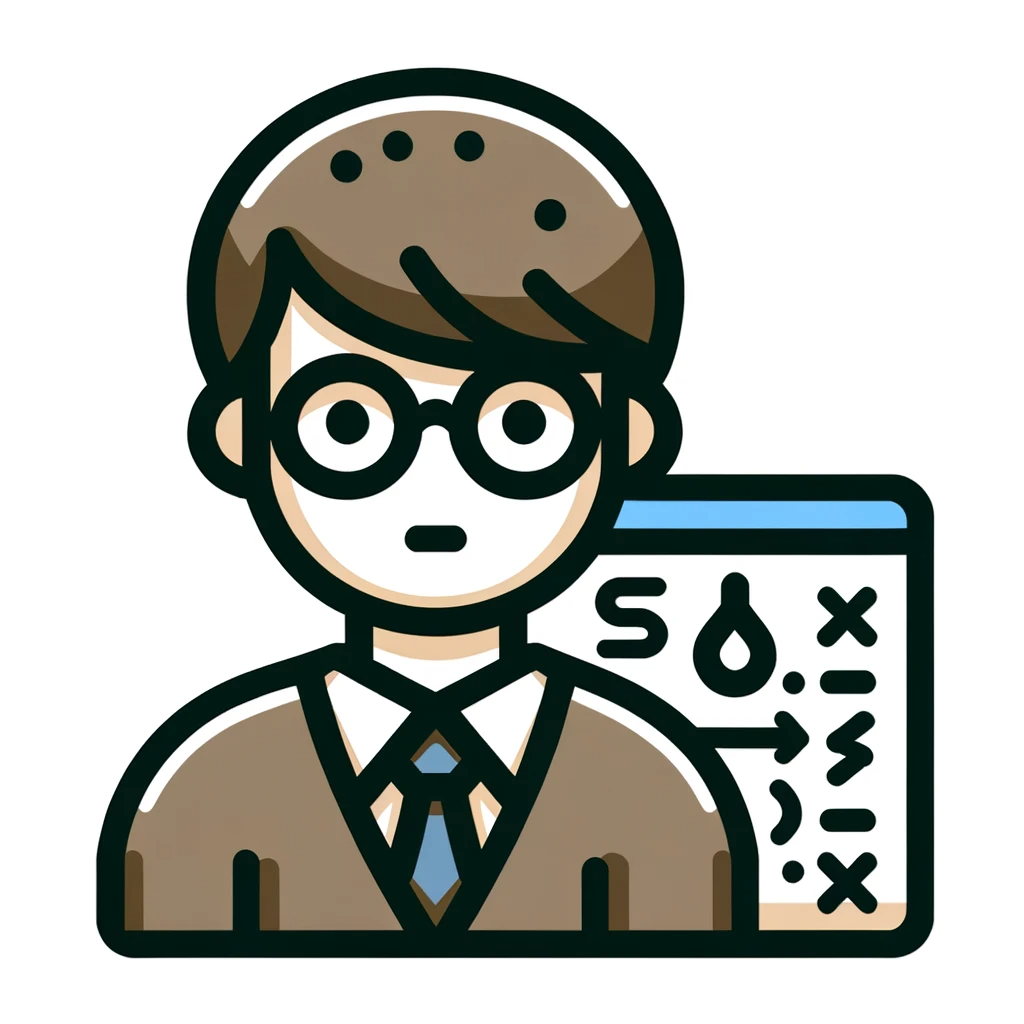
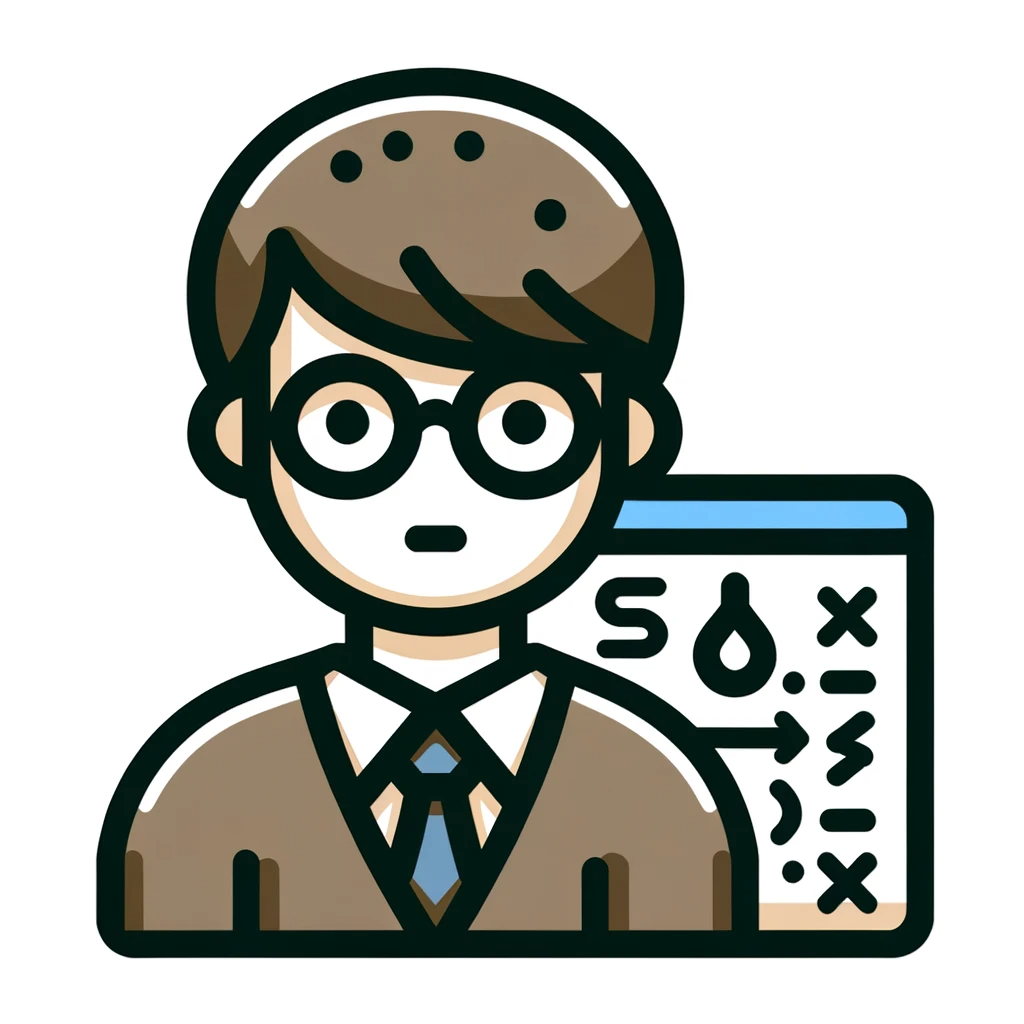
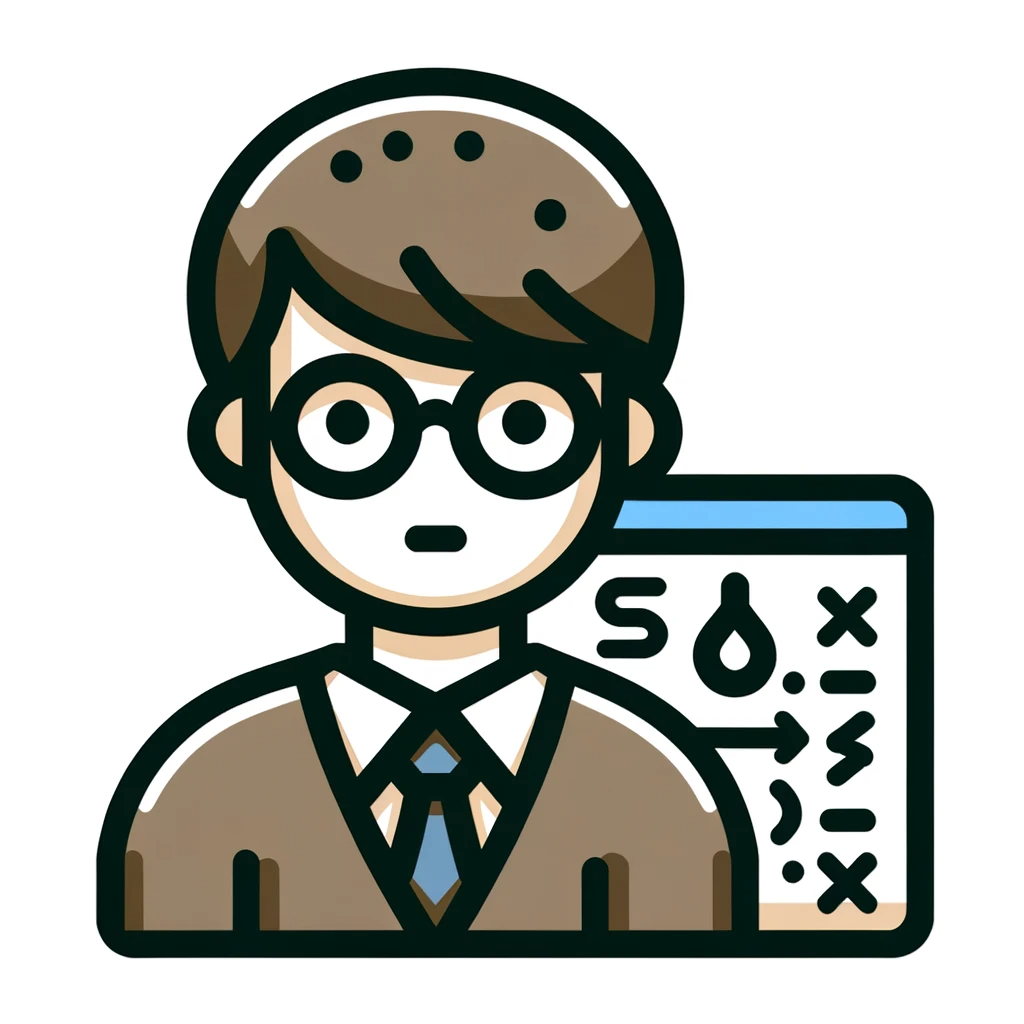
Use the join method to join array elements by separating them with commas or spaces.
How to join arrays using join method
JavaScript arrays have a join method that allows you to join array elements by separating them with commas or spaces .
array.join([delimiter]);
The join method joins the elements of an array by specifying the delimiter as the first argument. If the argument is omitted, a comma is the default delimiter.
let fruits = ["apple", "banana", "orange"];
let fruitsString = fruits.join(", ");
console.log(fruitsString); // "apple, banana, orange"
In the above sample, the elements of the fruits array are concatenated with commas and spaces and stored in fruitsString.
If you want to join with blank spaces, write as follows.
const array = ["apple", "orange", "banana"];
const joined = array.join(" "); // "apple orange banana"
Note that the join() method can be used to join array elements with a specified string. If the argument is omitted, the elements are joined by comma by default. Also, the original array is not changed, but a new string is created.
How to combine elements of other arrays
In addition to the join() method, there are several other ways to join array elements. Some examples are shown below.
How to use concat() method
const array1 = [1, 2];
const array2 = [3, 4];
const joined = array1.concat(array2).join(","); // "1,2,3,4"
The concat() method can be used to combine an array passed as an argument with the original array. In the above example, array1 and array2 are combined to create a new array and then,
How to use reduce() method
The reduce() method can be used to traverse each element of an array one by one and return the final single value. You can use this to create comma-separated strings.
The following example shows how to use the reduce() method to convert a given array to a comma-separated string.
const arr = [1, 2, 3, 4, 5];
const result = arr.reduce((prev, current) => {
if (prev === '') {
return current.toString();
} else {
return prev + ',' + current.toString();
}
}, '');
console.log(result); // "1,2,3,4,5"
In this example, the reduce() method has two arguments. The first argument is the return value of the previous call, and the first call passes an empty string as the initial value. The second argument is a callback function, which is used to process each element of the array.
The callback function receives the return value (or initial value) of the previous call and the current element. For the first element, the previous return value is the initial value, an empty string. Then use a conditional branch to convert the current element to a string and return it if the previous return value was empty. If the previous return value is not empty, appends a comma-separated string of the current elements to the previous return value and returns it.
Ultimately, the reduce() method processes each element and returns a comma-separated string after all elements are processed.
How to combine multiple arrays
To concatenate multiple arrays, you can use the concat method or the spread operator .
summary
The following is a summary of how to combine array elements using commas and spaces.
- The join method can join elements of an array separated by commas or spaces.
- Combine the elements of the array by specifying the delimiter as the first argument.
- If omitted, comma is the default delimiter.
- You can also combine using delimiters with the concat() and reduce() methods.
JavaScript arrays have a join method that allows you to join array elements by separating them with commas or spaces. The join method joins the elements of an array by specifying the delimiter as the first argument.
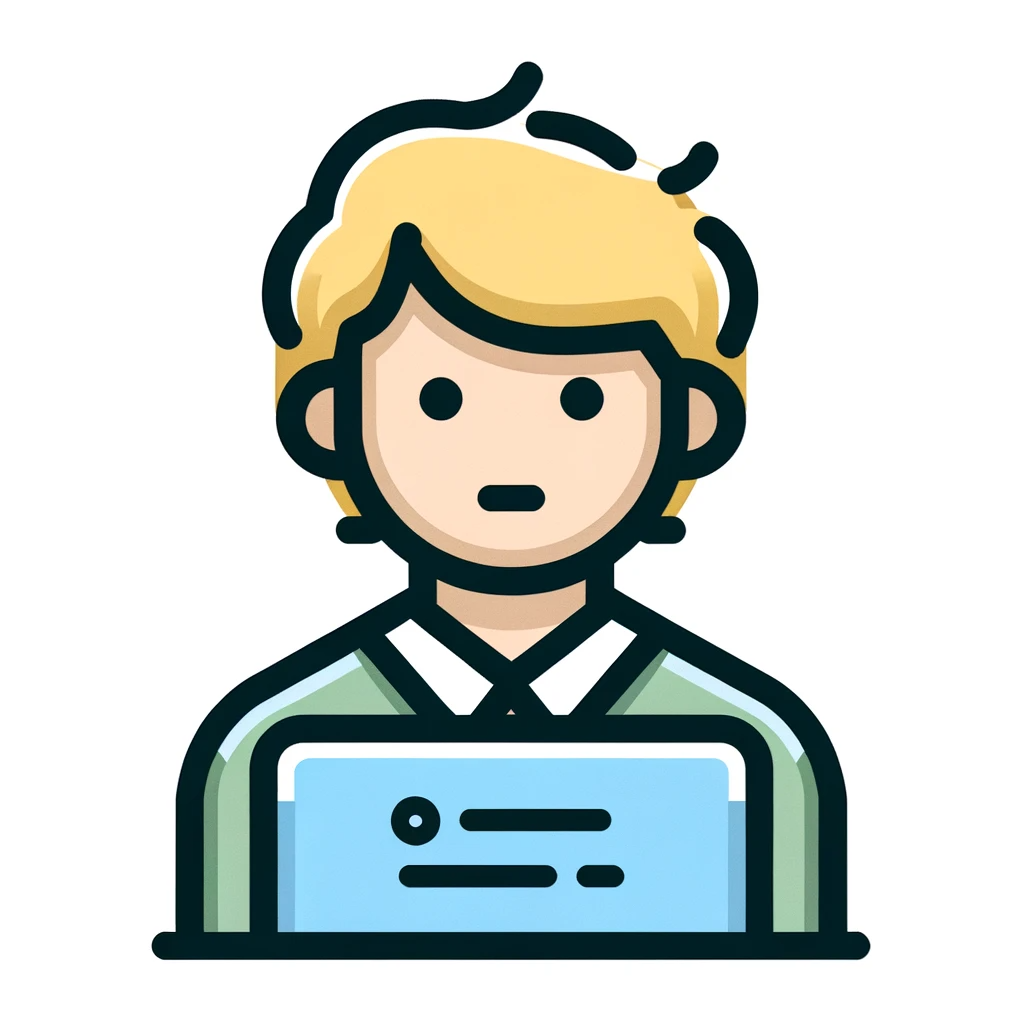
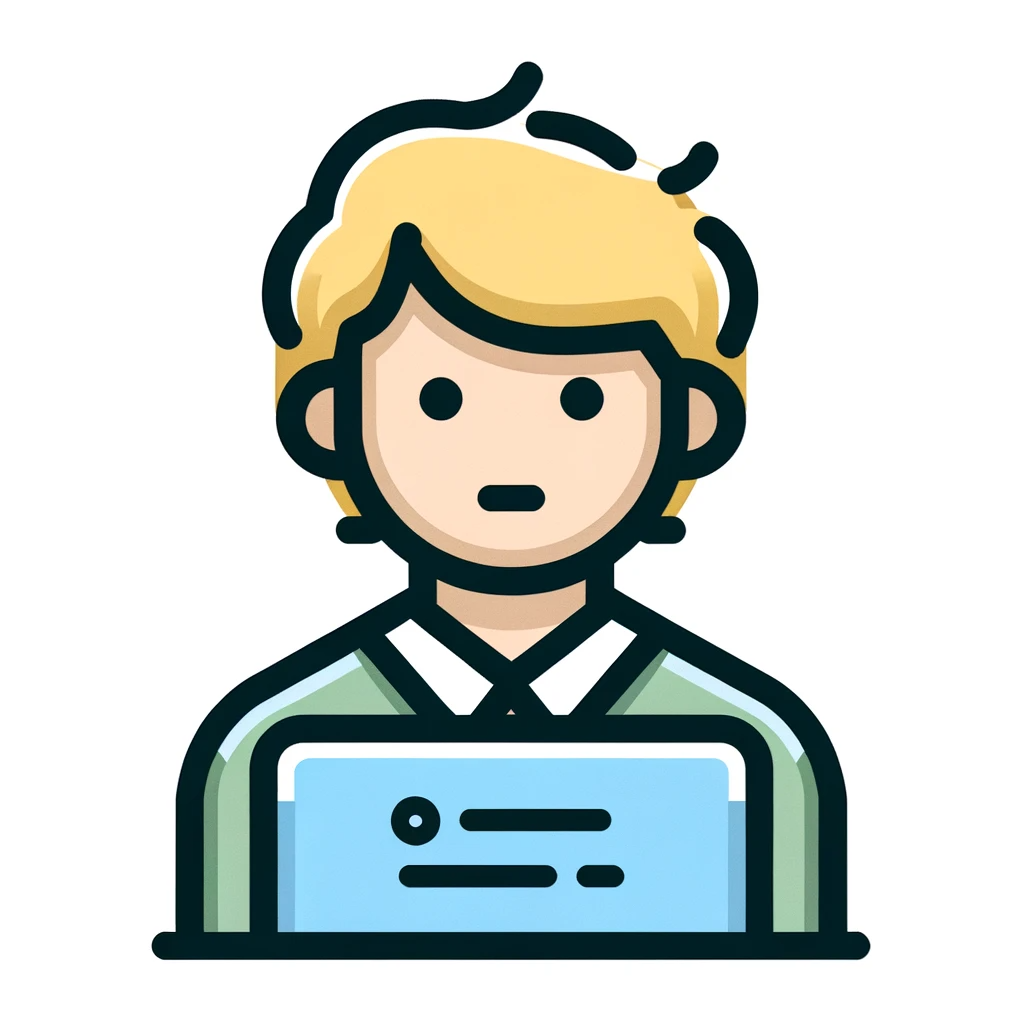
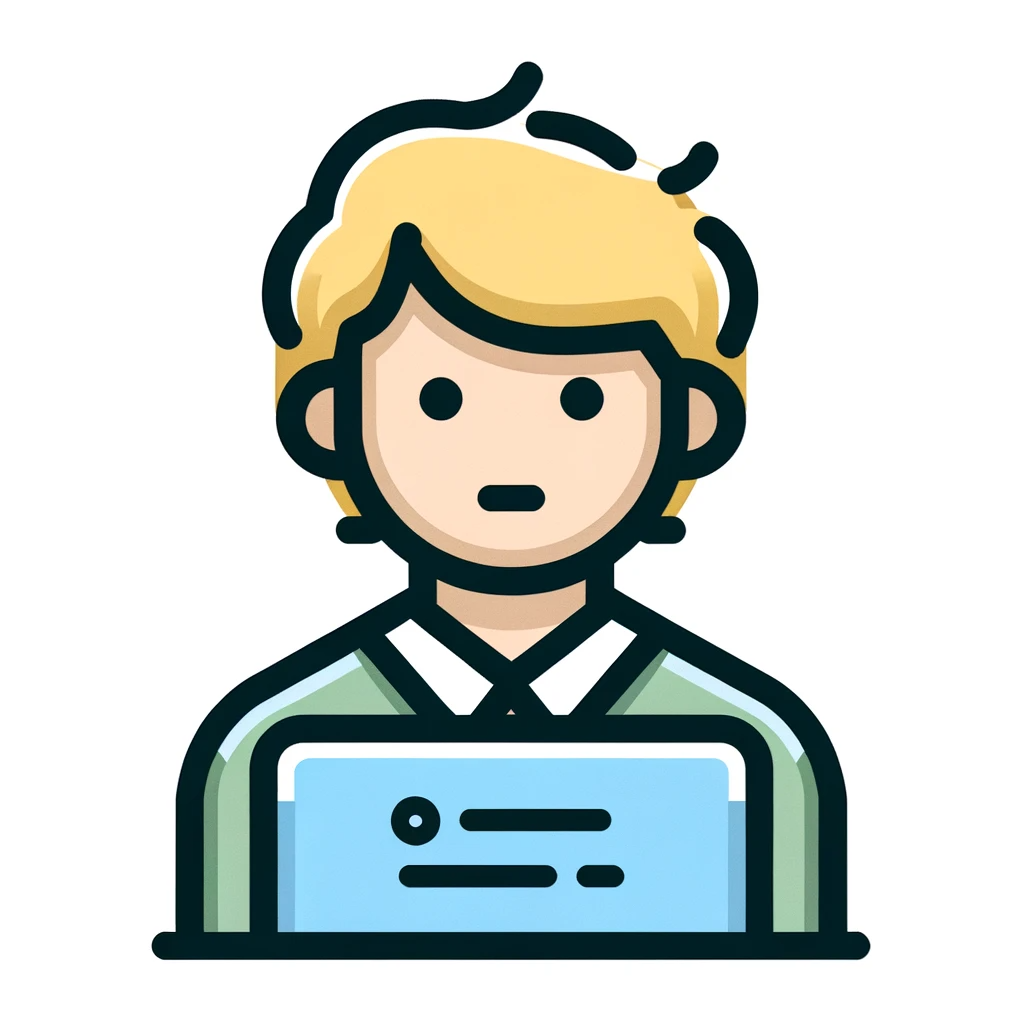
I didn’t know about the join method, but it seems very useful.
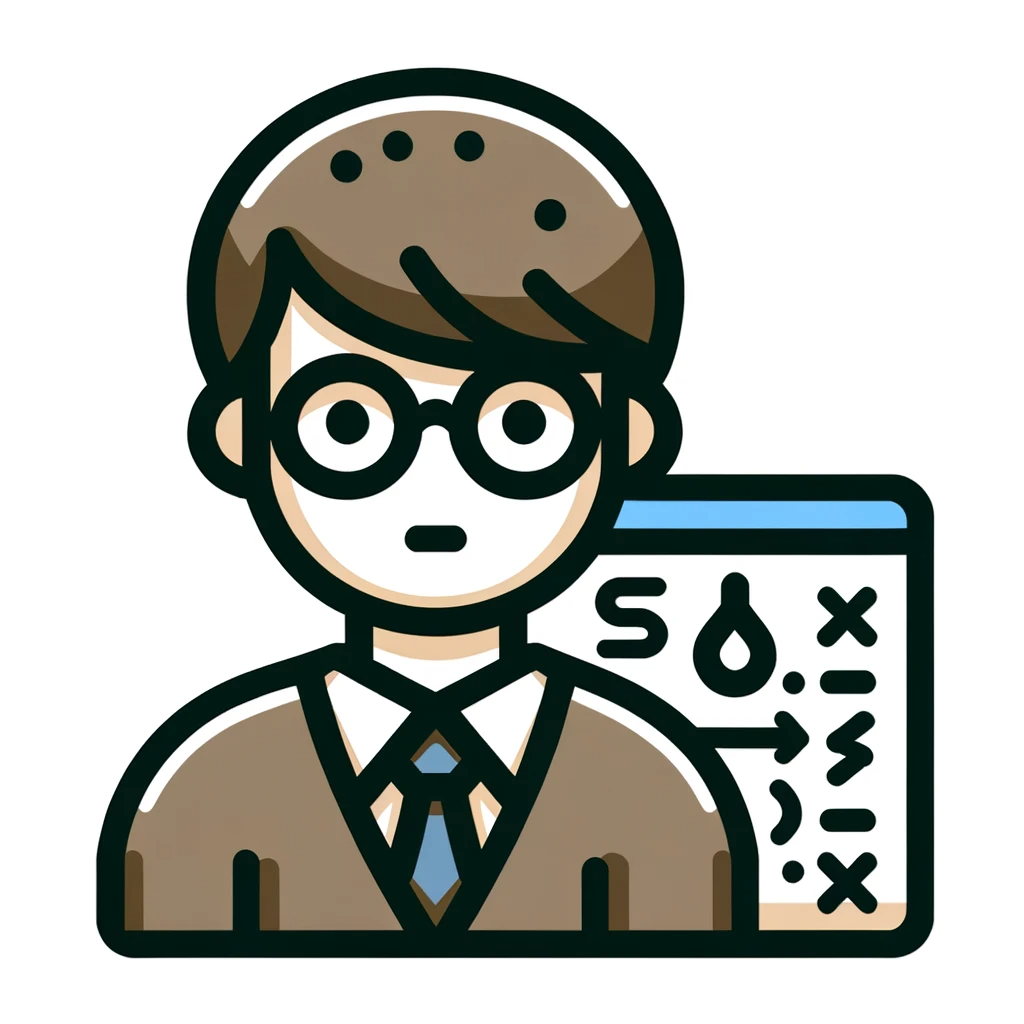
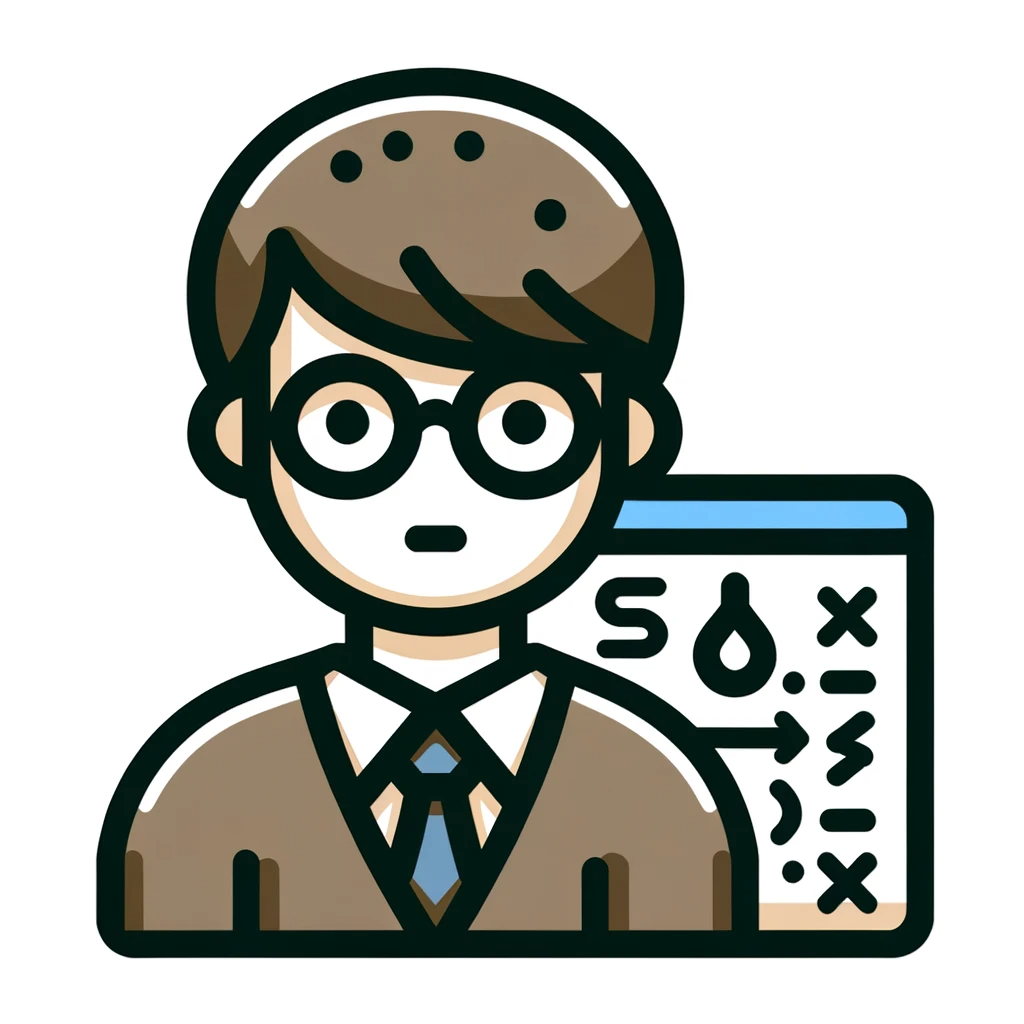
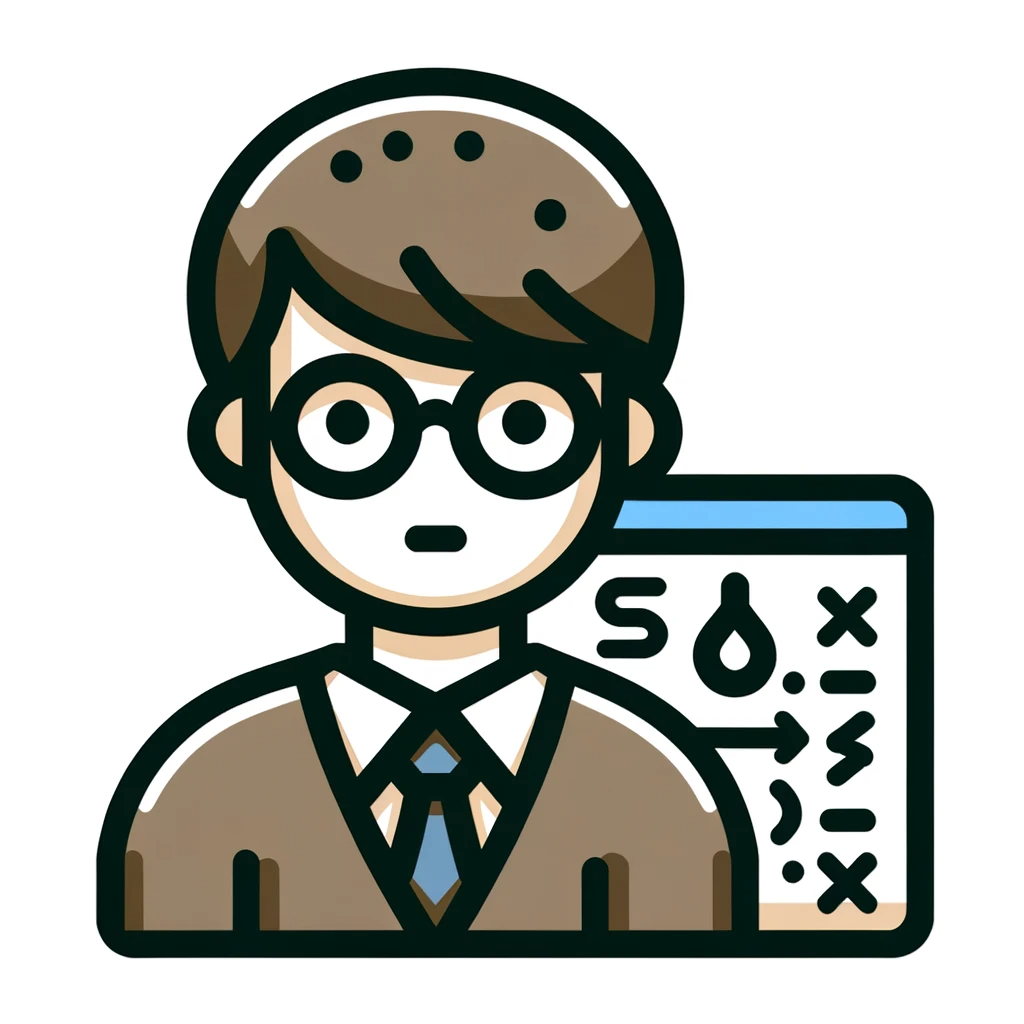
By combining array elements using the join method, you can treat the array elements as strings, which can also be used for string processing.
Comments