We will explain in detail how to determine the type of an object in JavaScript .
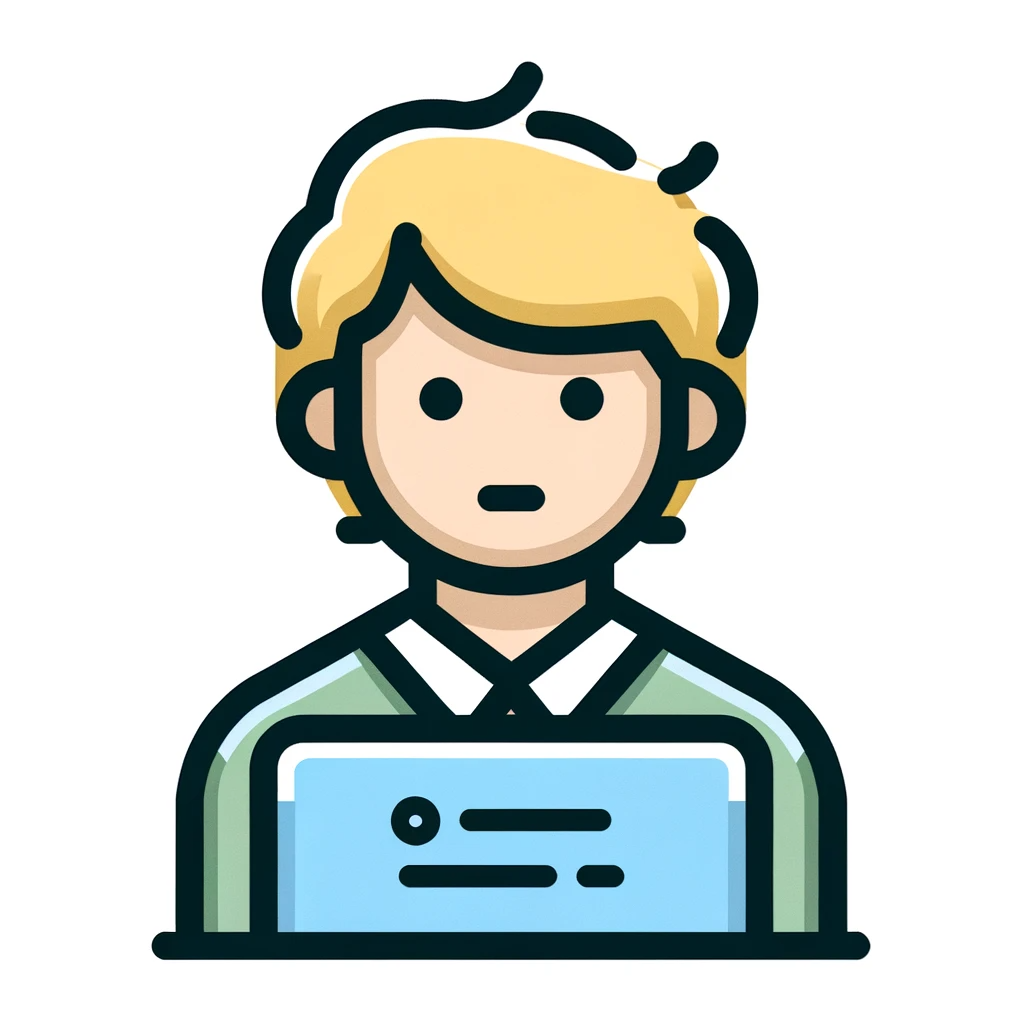
I would like to know how to determine the type of an object, but what should I do?
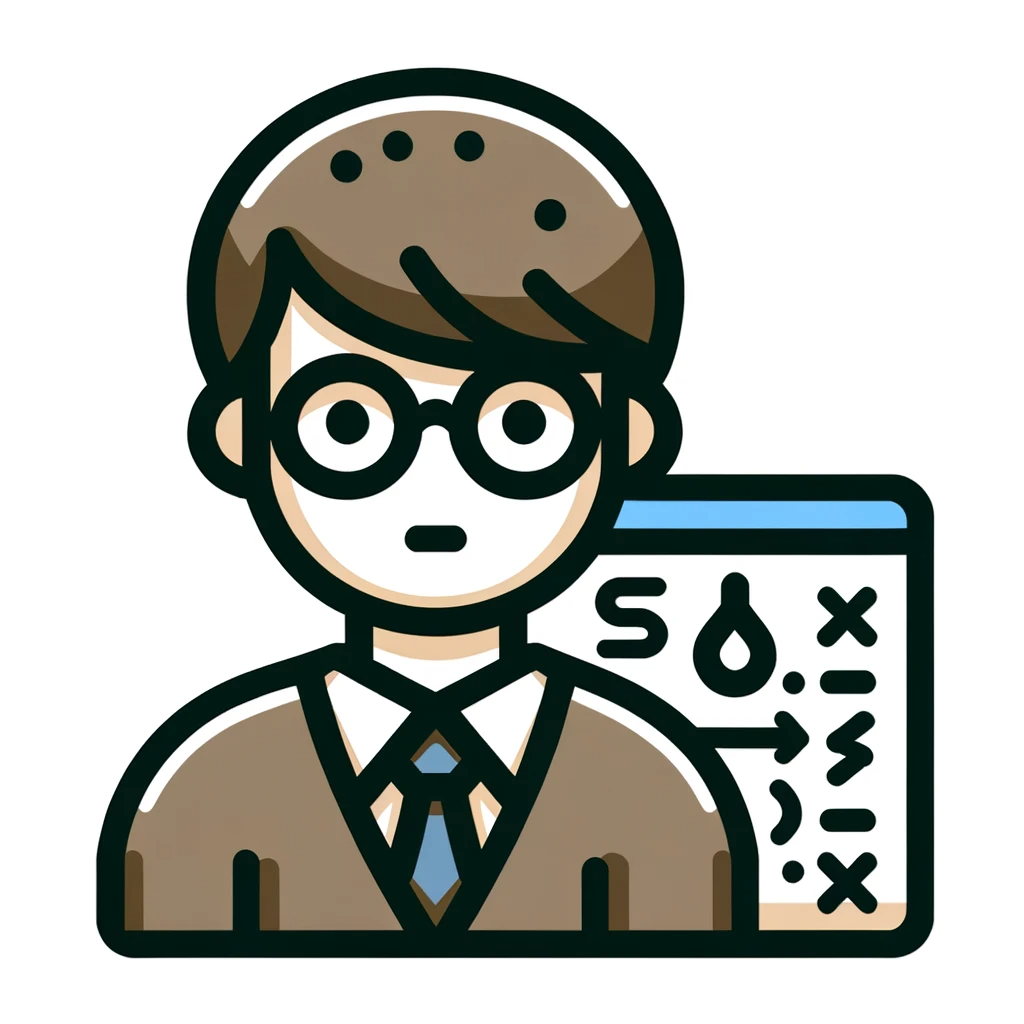
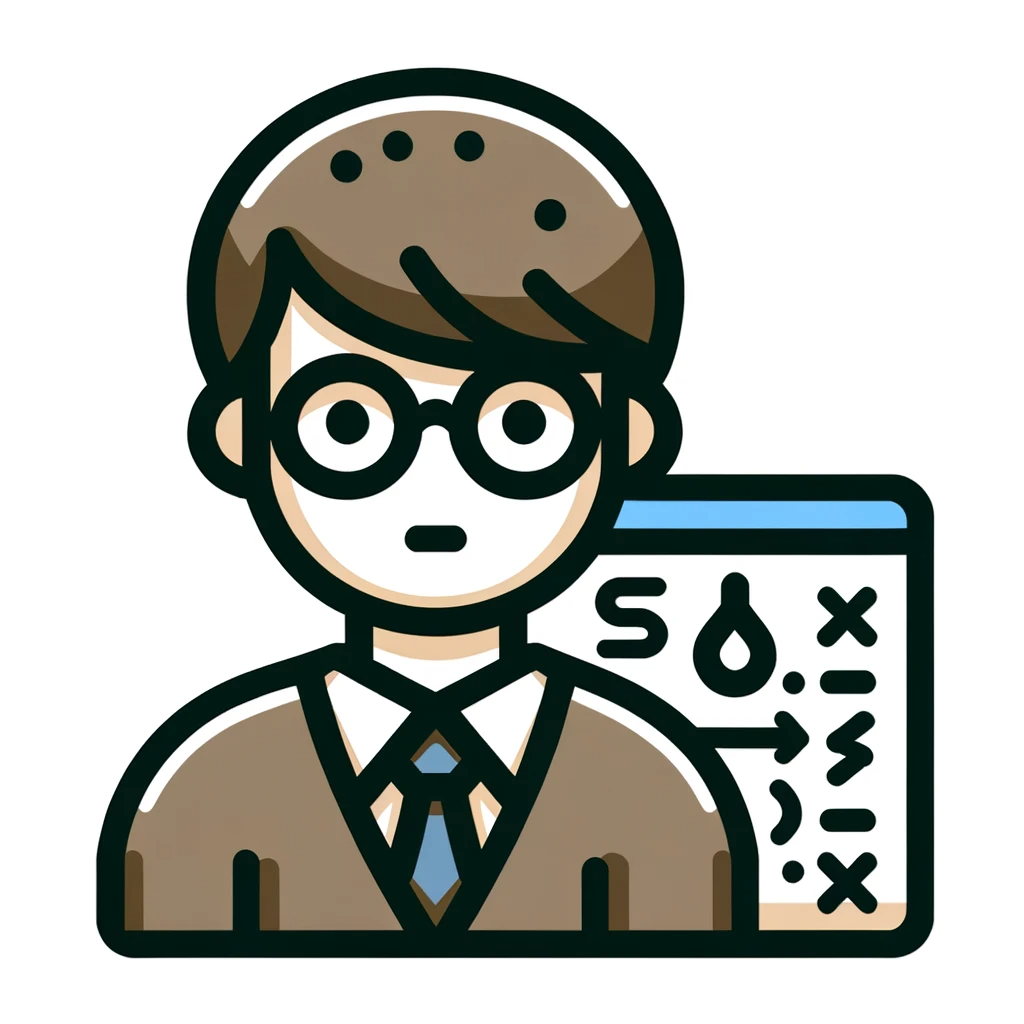
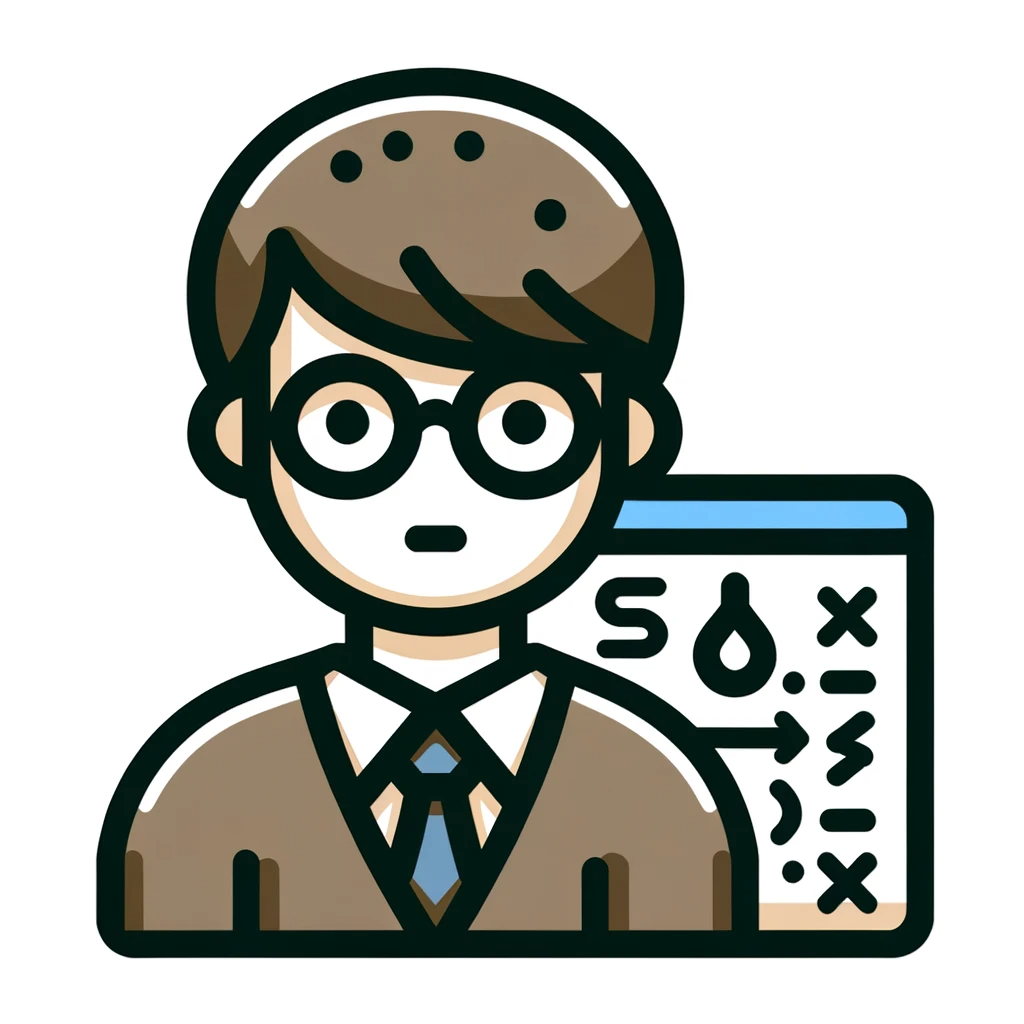
There are ways to determine the type of an object, such as using the instanceof operator, in operator, isPrototypeOf method, and constructor property.
Determining the type of an object using the instanceof operator
The instanceof operator is used to determine whether an object is an instance of a particular constructor. The operator passes the object on the left side and the constructor on the right side.
object instanceof constructor
The instanceof operator checks whether the specified constructor is included in the object’s prototype chain. If it does, the operator returns true. Otherwise, returns false.
Below is a sample program using the instanceof operator.
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
let myCar = new Car('Toyota', 'Camry', 2019);
console.log(myCar instanceof Car); // true
console.log(myCar instanceof Object); // true
console.log(myCar instanceof Array); // false
This sample uses the Car constructor to create a new Car object.
Next, we use the instanceof operator to determine that this object is an instance of the Car constructor. The instanceof operator returns true because the object is an instance of the Car constructor.
It then determines that this object is an instance of the Object constructor, which also returns true. Finally, it determines that this object is an instance of the Array constructor, but returns false.
Determining the type of an object using the in operator
The in operator is used to determine whether an object has a particular property. The operator passes the property name on the left side and the object on the right side.
propertyName in object
The in operator checks whether the specified property is included in the object or its prototype chain. If it does, the operator returns true. Otherwise, returns false.
The following is a sample program using the in operator.
let myCar = {
make: 'Toyota',
model: 'Camry',
year: 2019
};
console.log('make' in myCar); // true
console.log('model' in myCar); // true
console.log('color' in myCar); // false
This sample uses object literals to create new objects.
Next, we use the in operator to determine that this object has a ‘make’ property. The in operator returns true because the object has a ‘make’ property.
Next, we determine that this object has a ‘model’ property, which also returns true. Finally, we determine that this object has a ‘color’ property, which returns false.
Determining the type of an object using the isPrototypeOf method
A method similar to the instanceof operator is the isPrototypeOf method.
The isPrototypeOf method is used to determine whether an object is included in the prototype chain of another object.
prototype.isPrototypeOf(object)
The isPrototypeOf method returns true if the object on the left is included in the prototype chain of the object on the right. Otherwise, returns false.
The following is a sample program using the isPrototypeOf method.
let car = {
make: 'Toyota',
model: 'Camry'
};
let myCar = Object.create(car);
myCar.year = 2019;
console.log(car.isPrototypeOf(myCar)); // true
In this sample, we first create a car object. Next, create a new object using the Object.create method. This new object has the car object as its prototype.
Finally, we use the isPrototypeOf method to determine whether the car object is included in the myCar object’s prototype chain. The isPrototypeOf method returns true because the car object is included in the myCar object’s prototype chain.
Determining the type of an object using the constructor property
The constructor property refers to the constructor function in which the object was created.
object.constructor
You can use the constructor property to determine which constructor function an object was created with.
The following is a sample program that uses the constructor property.
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
let myCar = new Car('Toyota', 'Camry', 2019);
console.log(myCar.constructor === Car); // true
In this sample, we first define a constructor function called Car. Next, use the new operator to create a new object called myCar.
Finally, determine whether the myCar object’s constructor property and the Car function are equal. The myCar object was created by the Car function, so the constructor property references the Car function. Therefore, myCar.constructor === Car is true.
When determining a derived class using the constructor property
The following is an example of determining derived classes. Here, we will create an SUV class that inherits from the Car class.
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
function SUV(make, model, year, seatingCapacity) {
Car.call(this, make, model, year);
this.seatingCapacity = seatingCapacity;
}
SUV.prototype = Object.create(Car.prototype);
SUV.prototype.constructor = SUV;
let mySUV = new SUV('Toyota', 'Highlander', 2019, 7);
console.log(mySUV.constructor === SUV); // true
console.log(mySUV.constructor === Car); // false
In this sample, we first define a constructor function called Car. Next, define a derived class called SUV. The SUV class inherits from the Car class. Finally, use the new operator to create a new object called mySUV.
Next, determine whether the mySUV object’s constructor property references the SUV function or the Car function.
The mySUV object was created by the SUV function, so the constructor property references the SUV function. Therefore, mySUV.constructor === SUV will be true and mySUV.constructor === Car will be false.
Summary of this article
We explained how to determine the type of an object .
- Type determination using instanceof operator
- Used to determine whether an object is an instance of a particular constructor.
- Type determination using the in operator
- Used to determine whether an object has a particular property.
- Type determination using isPrototypeOf method
- Used to determine whether an object is included in another object’s prototype chain.
- Type determination using constructor property
- You can determine which constructor function an object was created with.
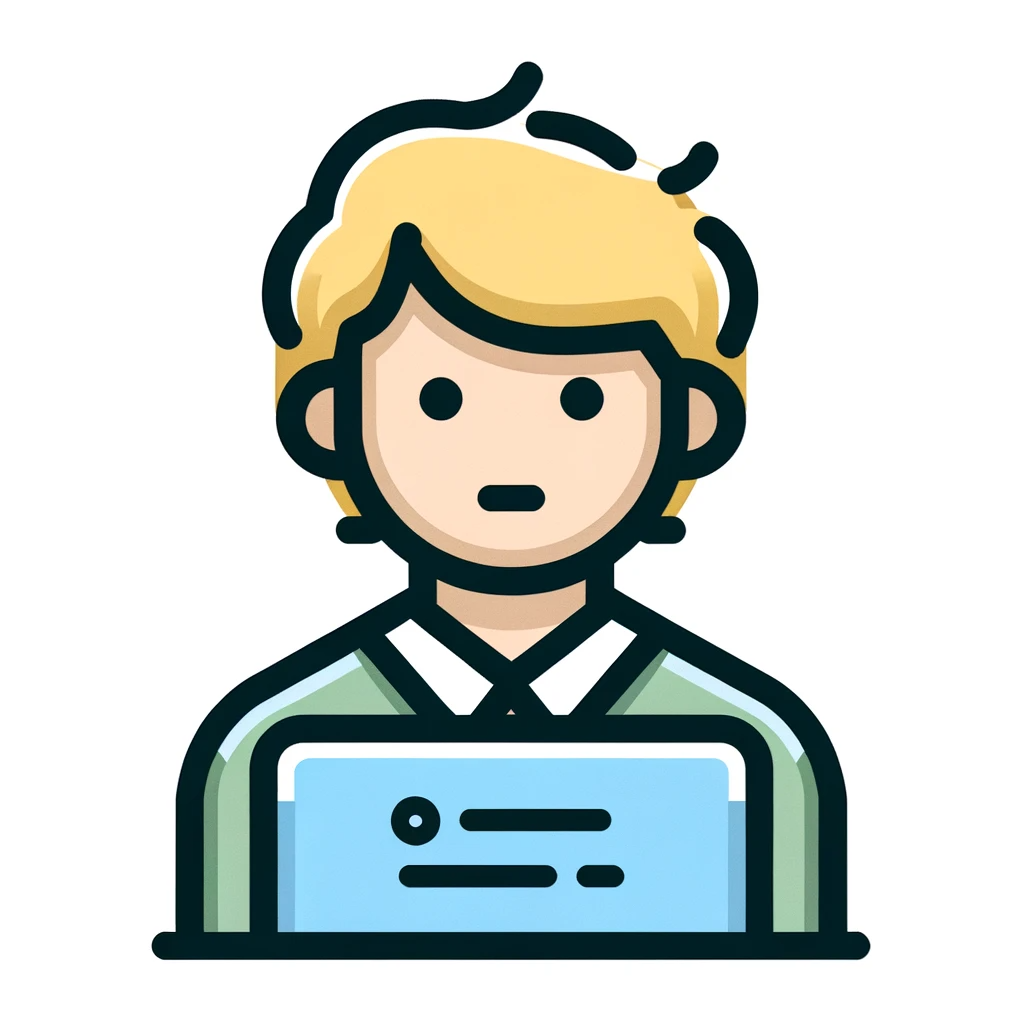
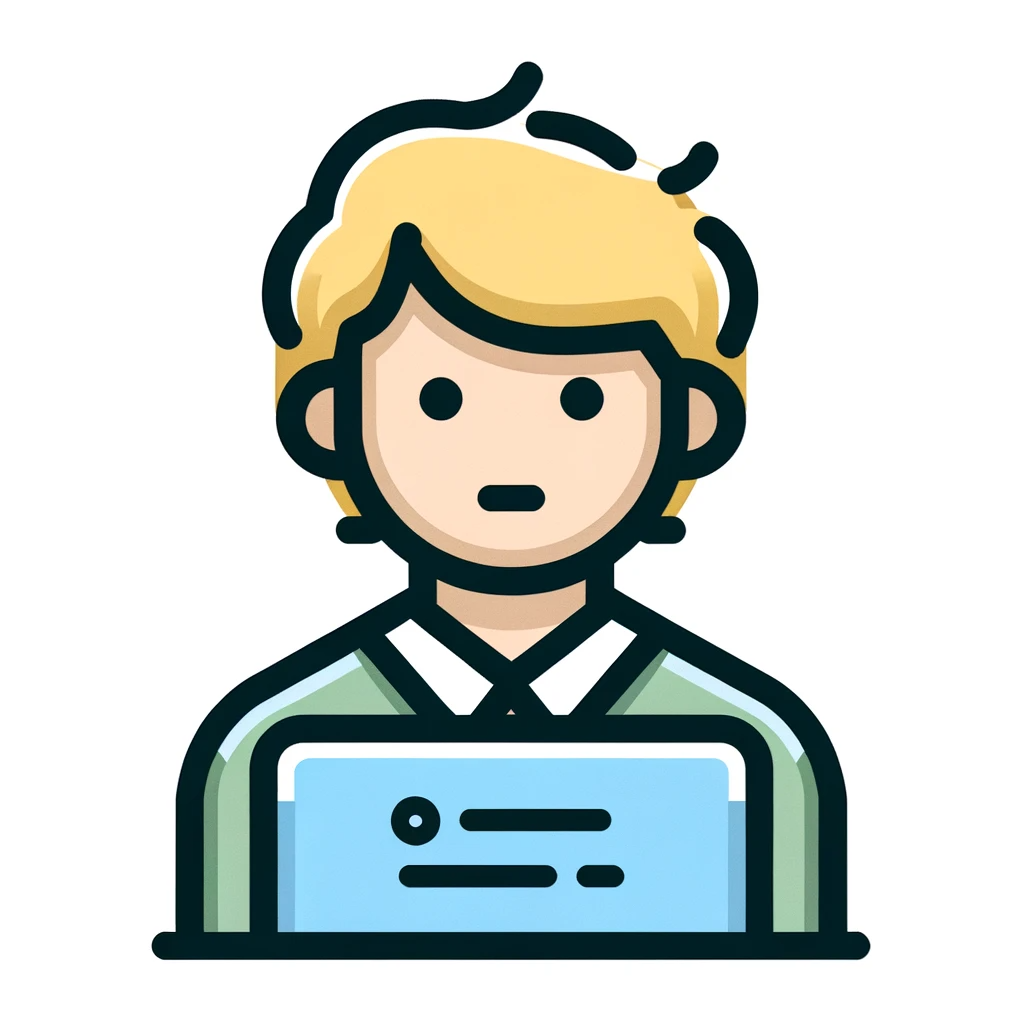
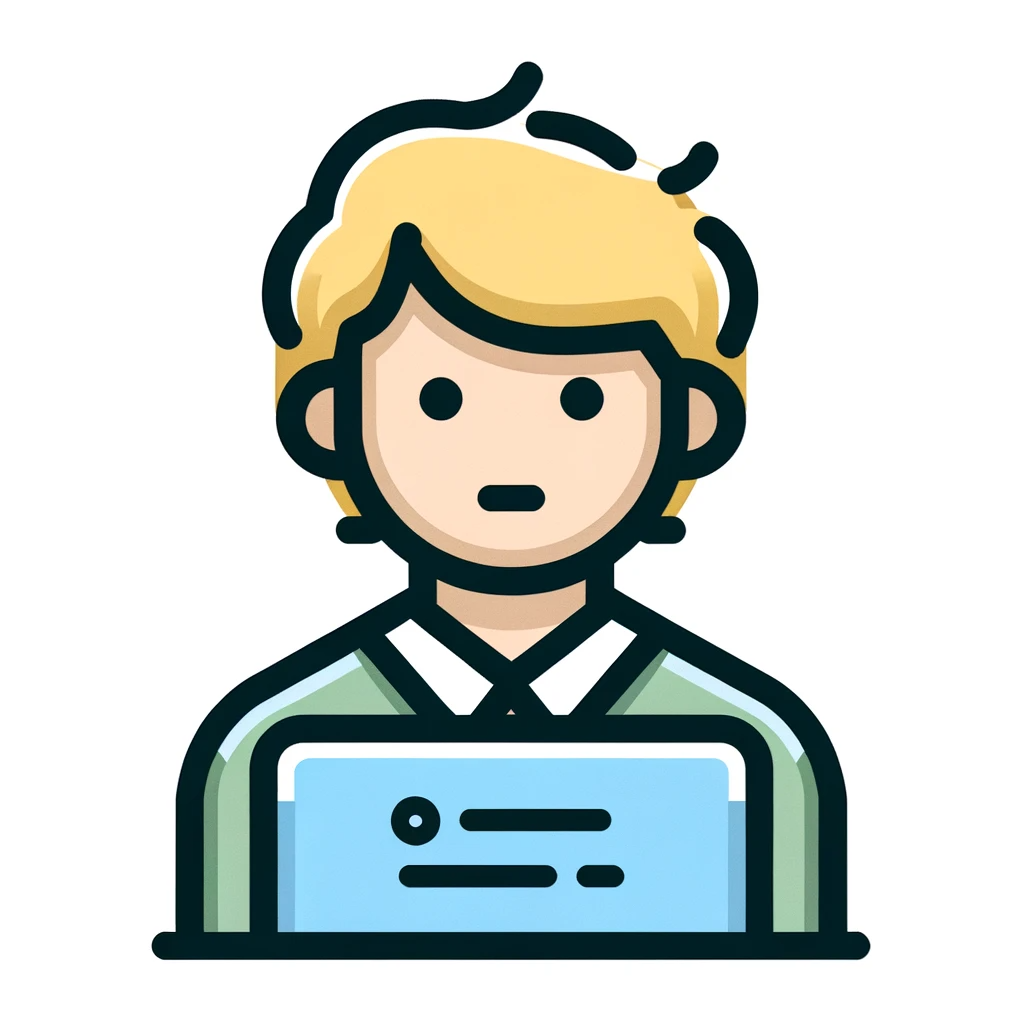
There are multiple ways to determine the type of an object, so it is important to choose the appropriate method depending on the purpose and conditions!
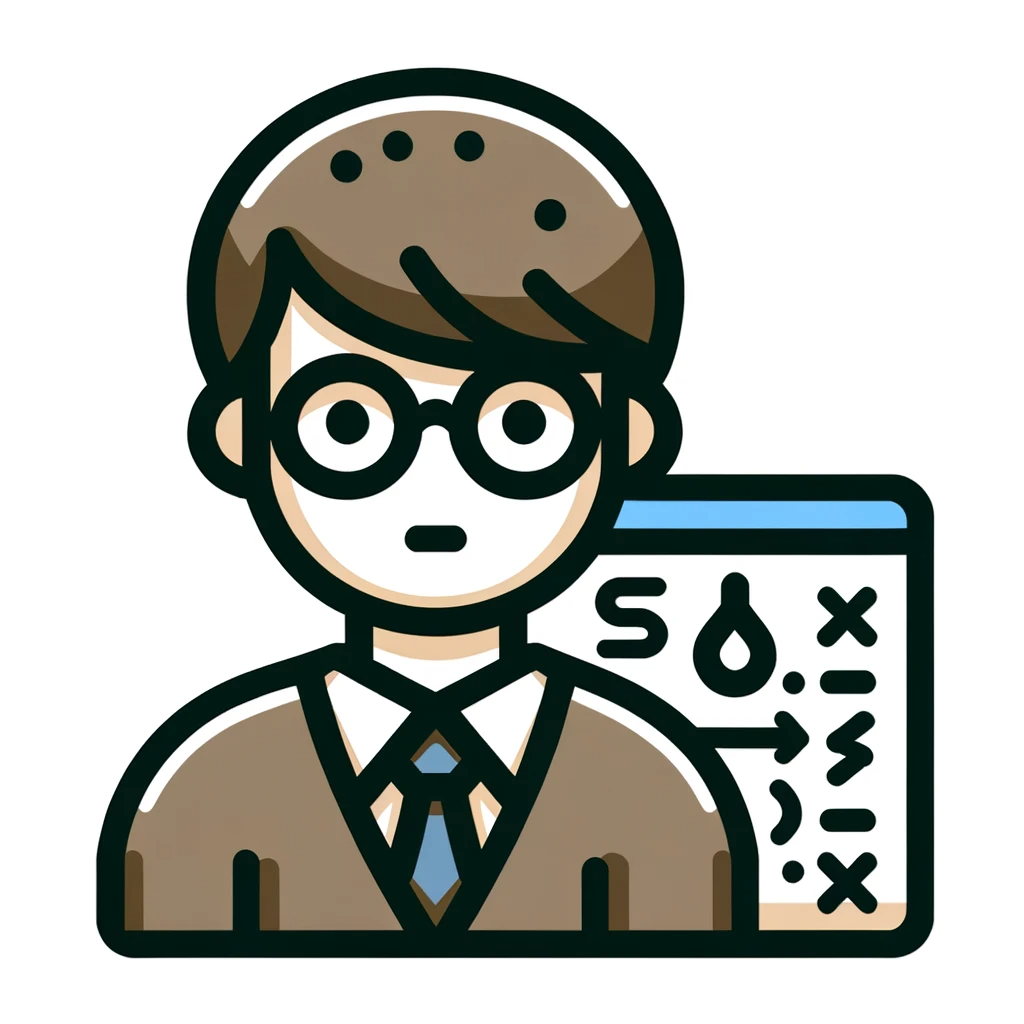
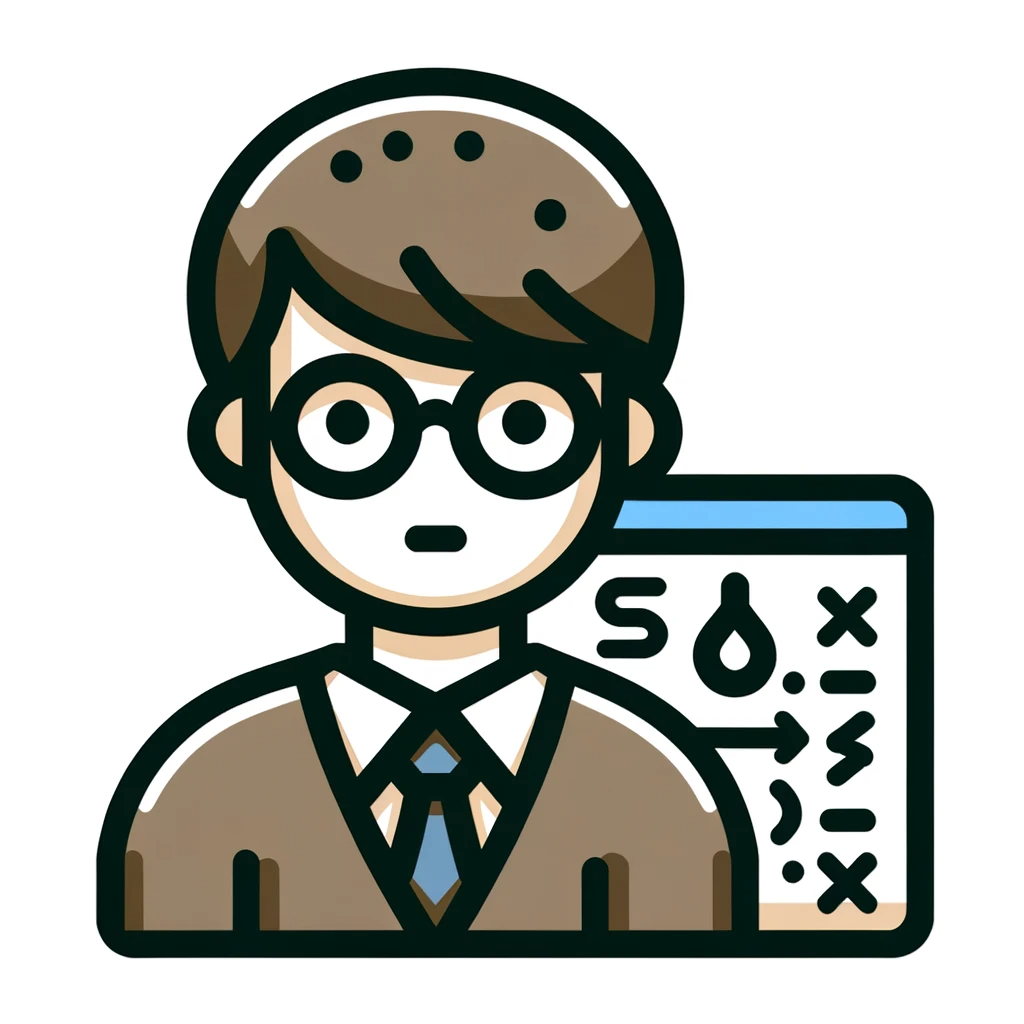
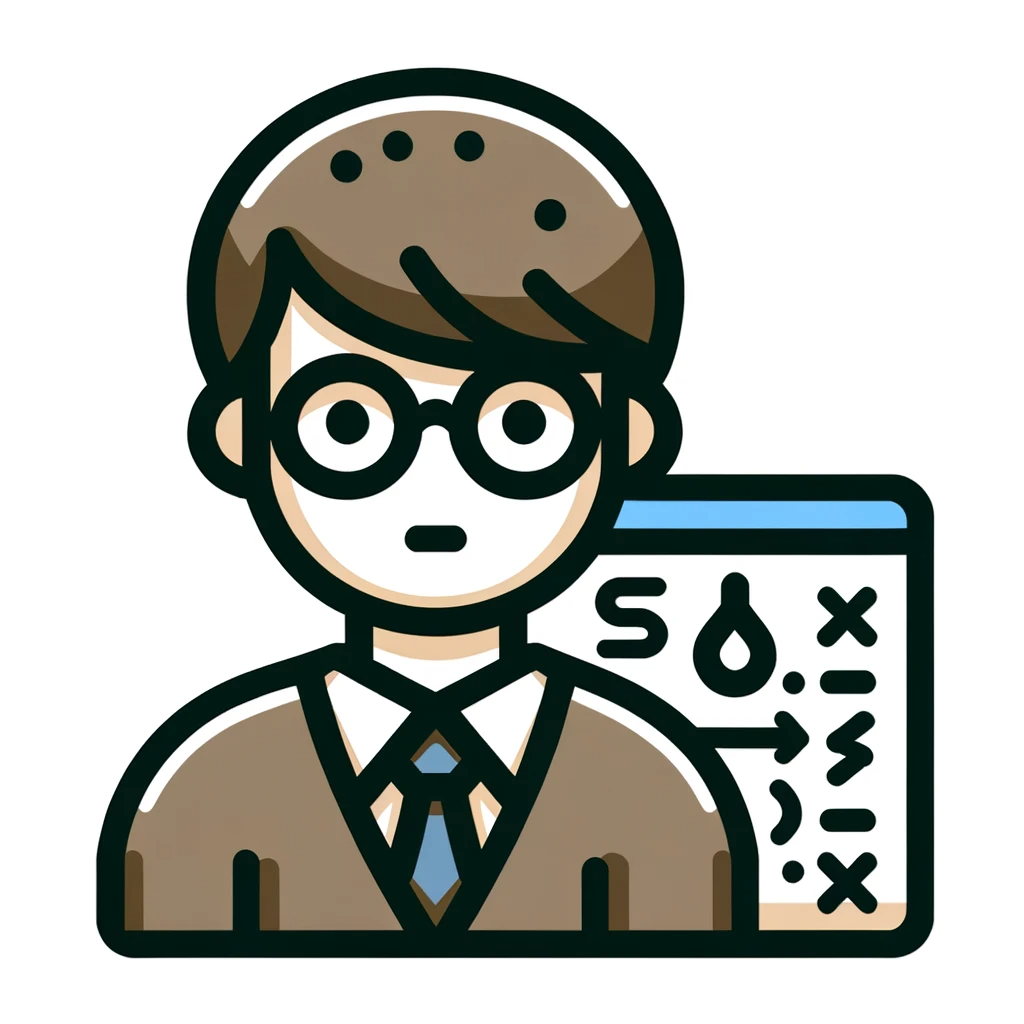
There are many ways to determine the type of an object, but they are not always accurate.
It is important to understand the use of each determination method and select the appropriate method.
Comments