This article explains how to search for the position of an array element in JavaScript.
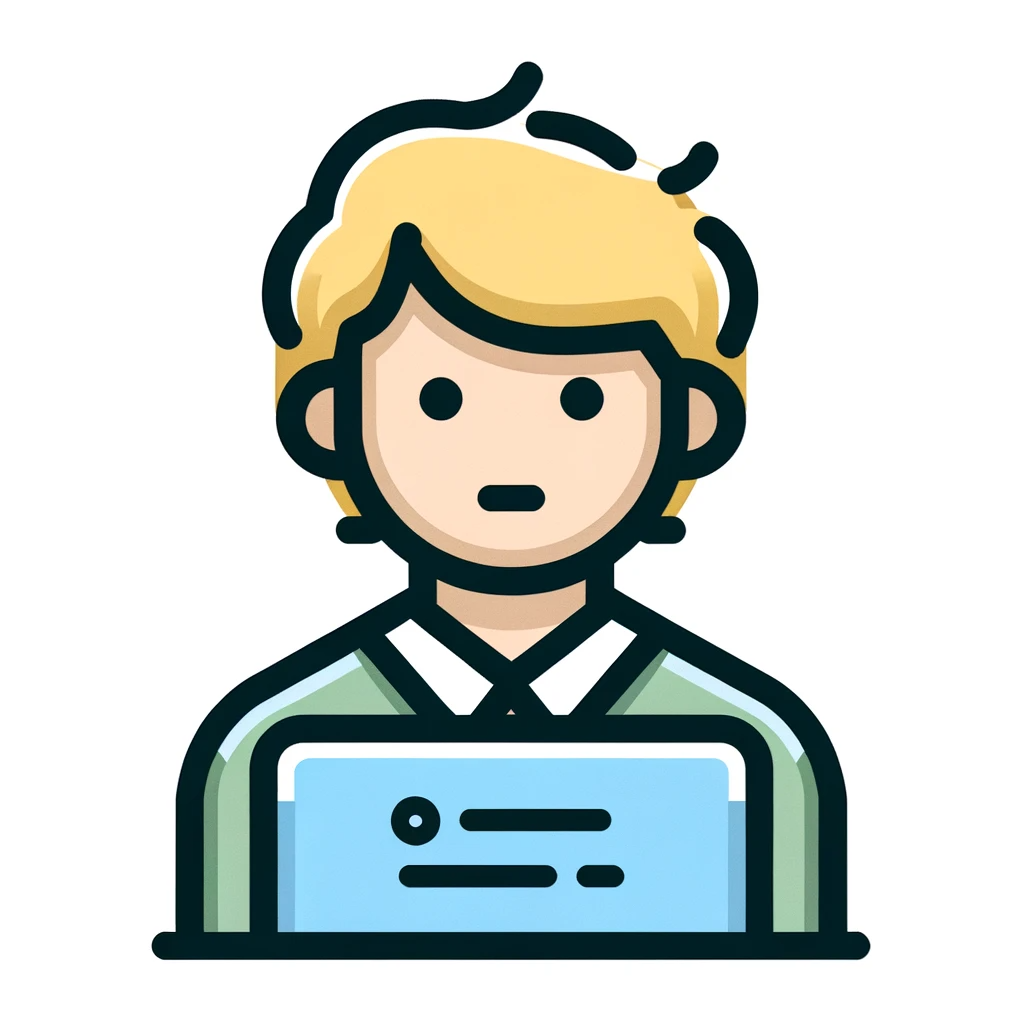
Is there a way to find the position of an element in an array?
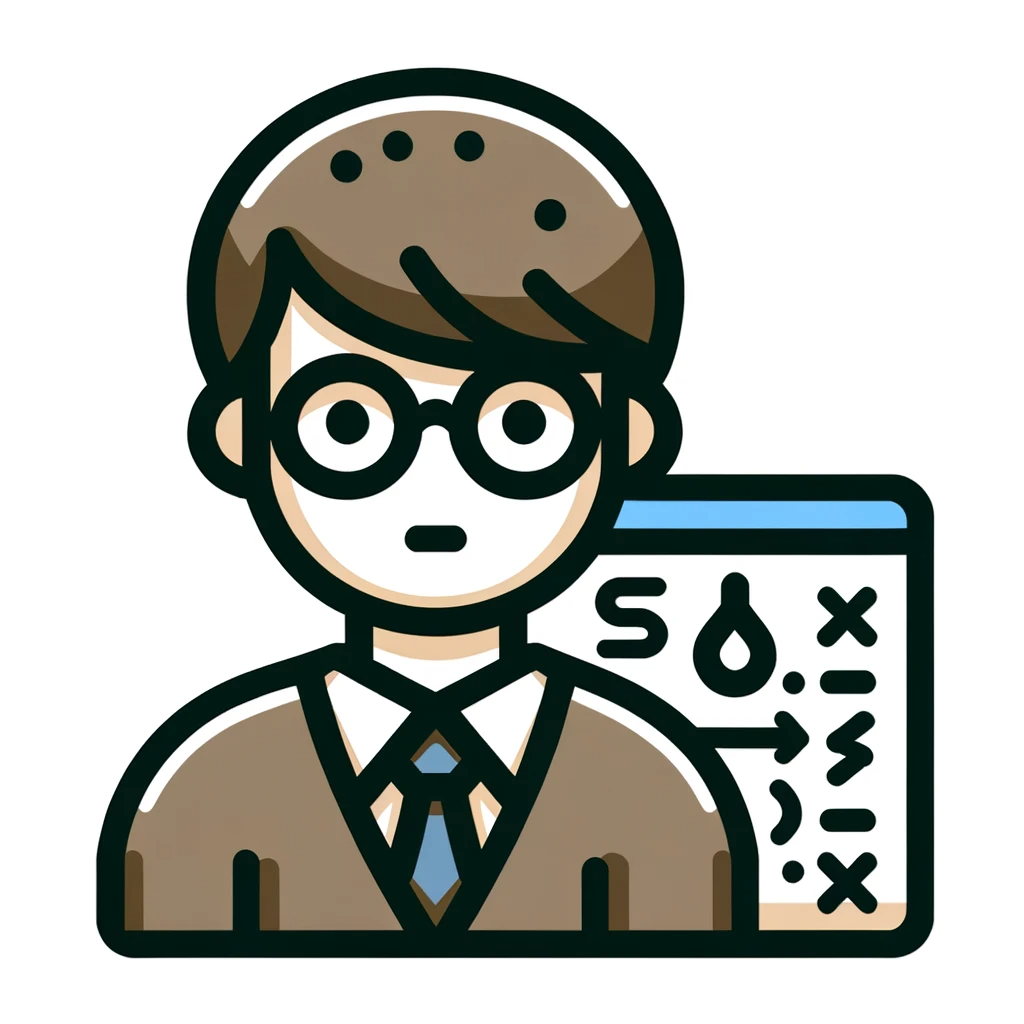
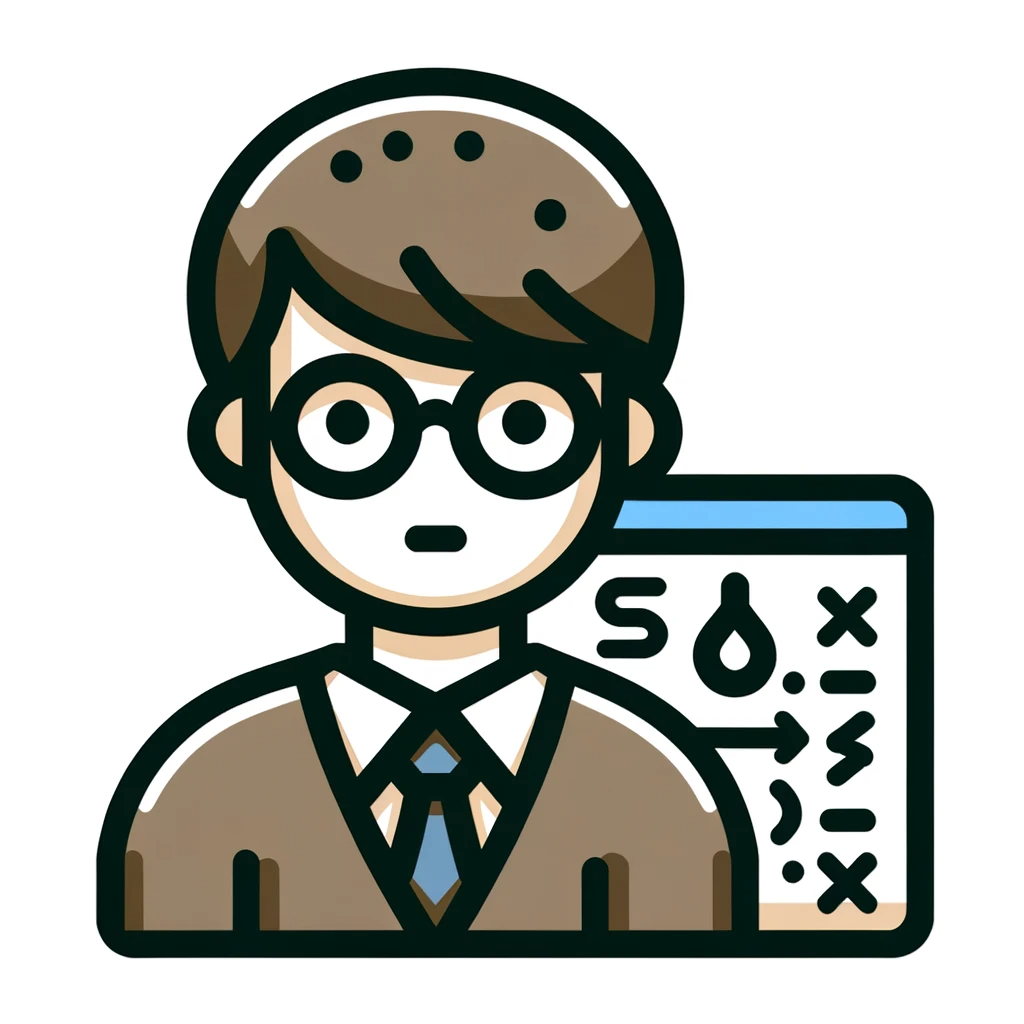
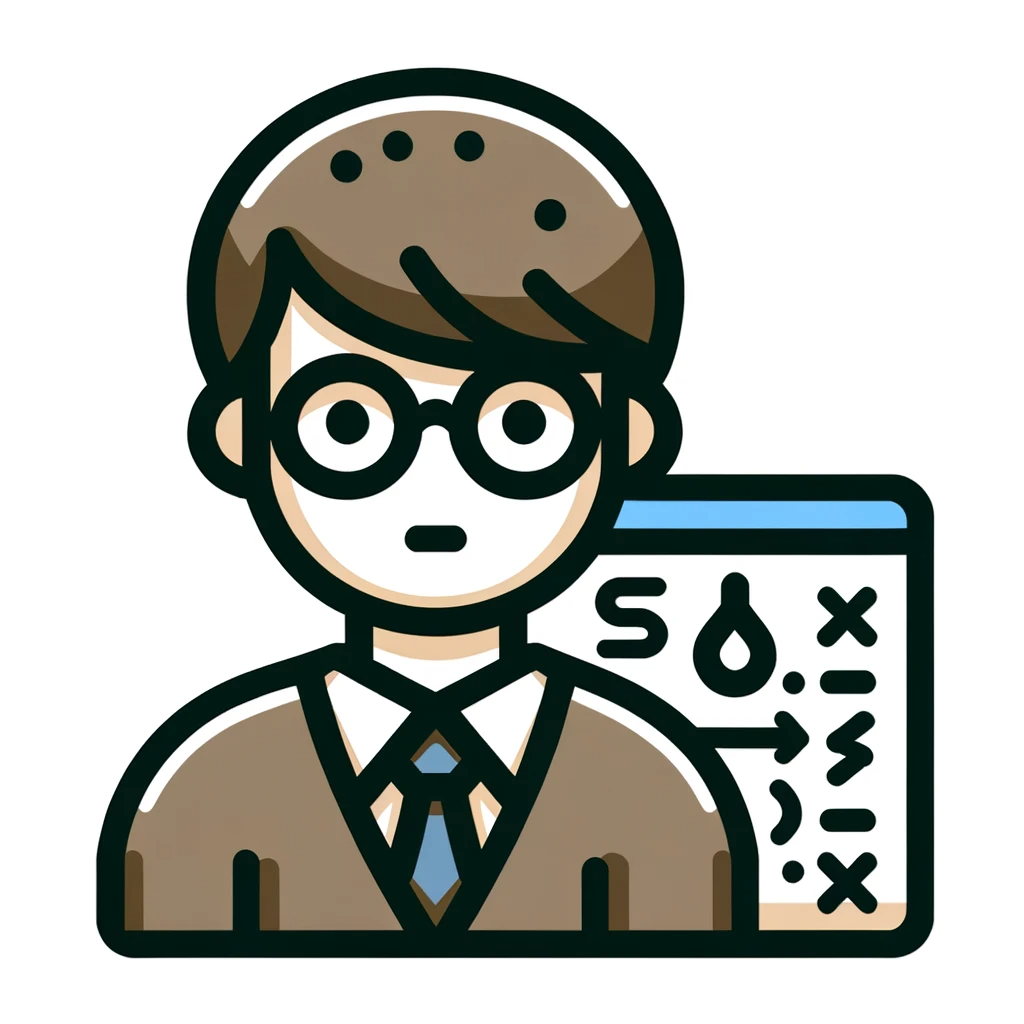
There are multiple ways to find the position of an array element.
I will show you how to use the indexOf method and findIndex method.
How to find the position of an array element using the indexOf method
The indexOf method returns the first index of the specified element from the array .
Searches from the beginning of the array and returns the index of the first element found. -1 is returned if the element specified in the argument does not exist in the array.
const fruits = ['apple', 'banana', 'orange'];
console.log(fruits.indexOf('banana')); // 1
How to find the position of an array element using the lastIndexOf method
The lastIndexOf method returns the last index of a specified element from an array .
Searches from the end of the array and returns the index of the last element found. -1 is returned if the element specified in the argument does not exist in the array.
const fruits = ['apple', 'banana', 'orange', 'banana'];
console.log(fruits.lastIndexOf('banana')); // 3
How to find the position of an array element using the findIndex method
The findIndex method returns the index of an element from an array that matches the specified criteria .
It takes a function as an argument and returns the index of the first element for which the function returns true. -1 is returned if there are no matching elements in the array.
const numbers = [1, 2, 3, 4, 5];
console.log(numbers.findIndex(num => num > 3)); // 3
How to create a loop and search by yourself
To get the index of all matching elements, you can create a loop and do the search yourself. Checks the array one by one and stores the index in the array if the elements match.
const fruits = ['apple', 'banana', 'orange', 'banana'];
// Get all matching indices
let indexes = [];
for (let i = 0; i < fruits.length; i++) {
if (fruits[i] === 'banana') {
indexes.push(i);
}
}
console.log(indexes); // [1, 3]
By creating a loop and searching like this, you can get all the indexes of multiple elements.
Summary
The following is a summary of how to search for the position of an array element.
- The indexOf method returns the first index of the specified element from the array.
- The lastIndexOf method returns the last index of the specified element from the array.
- The findIndex method returns the index of the element that matches the specified condition from the array.
- To get the index of all matching elements, create a loop and do the search yourself.
You can quickly locate an element by using the indexOf, findIndex, and lastIndexOf methods.
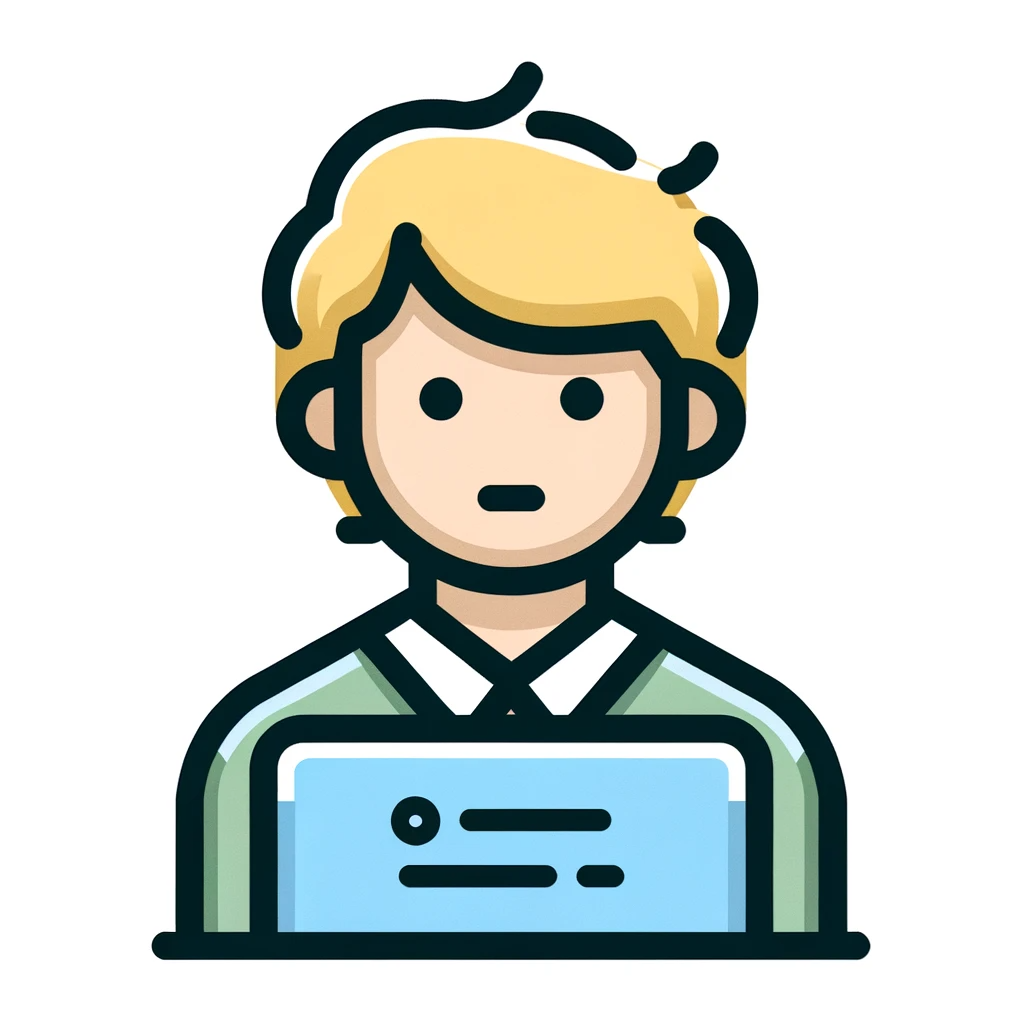
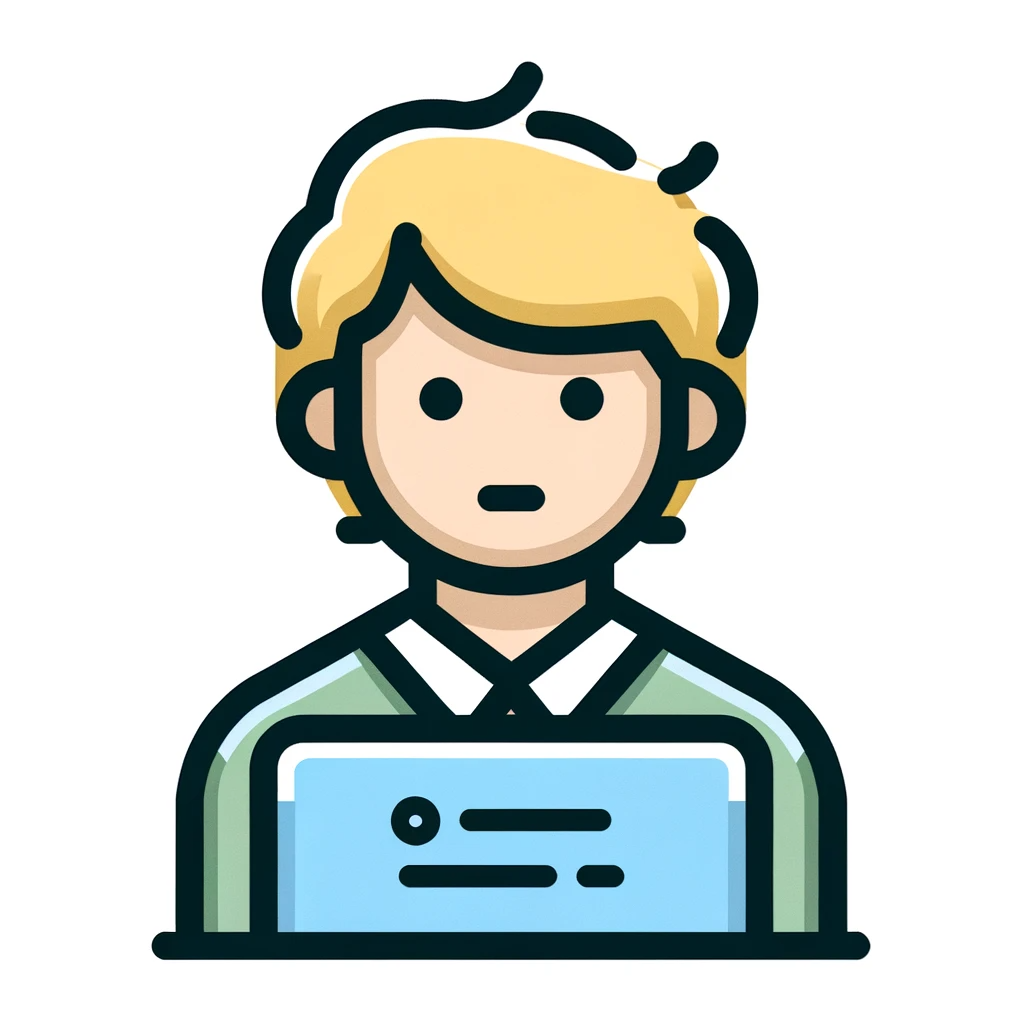
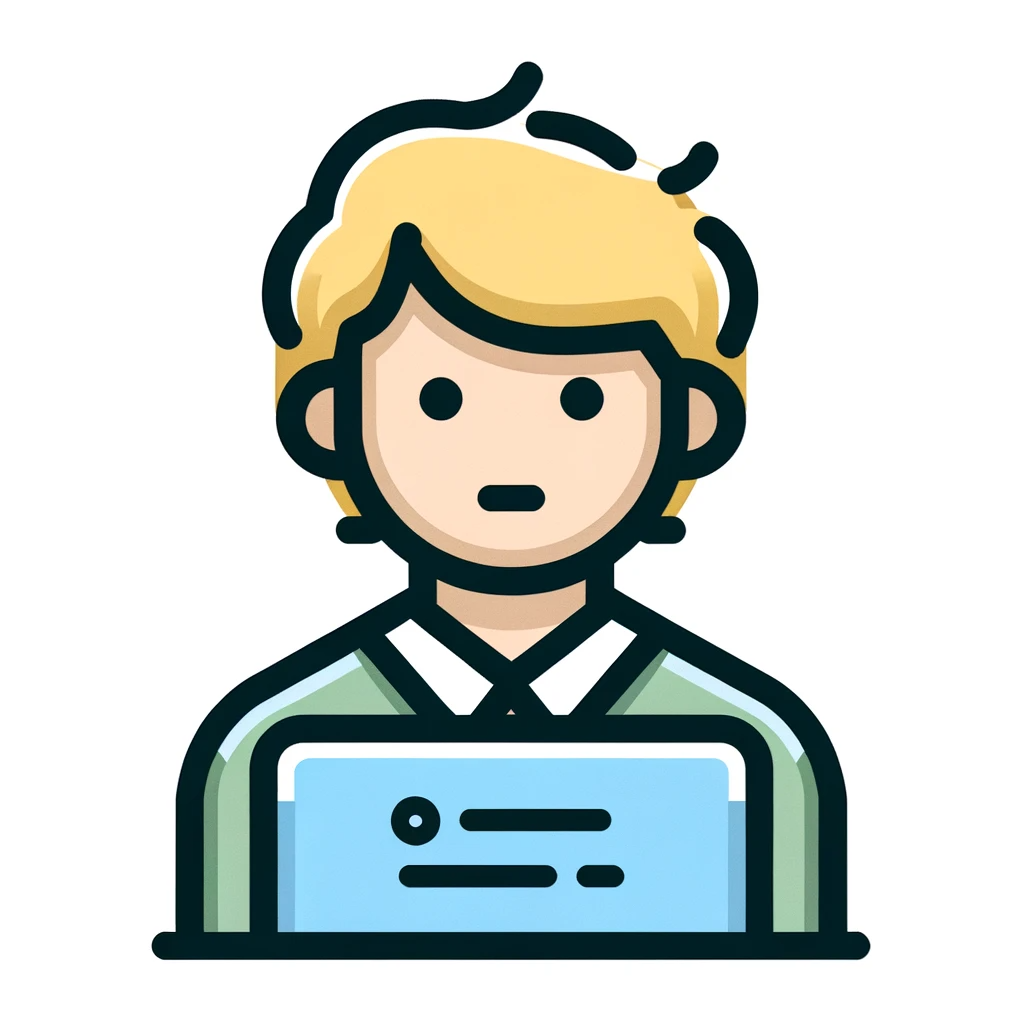
By using the indexOf and findInde) methods, we were able to easily locate the element!
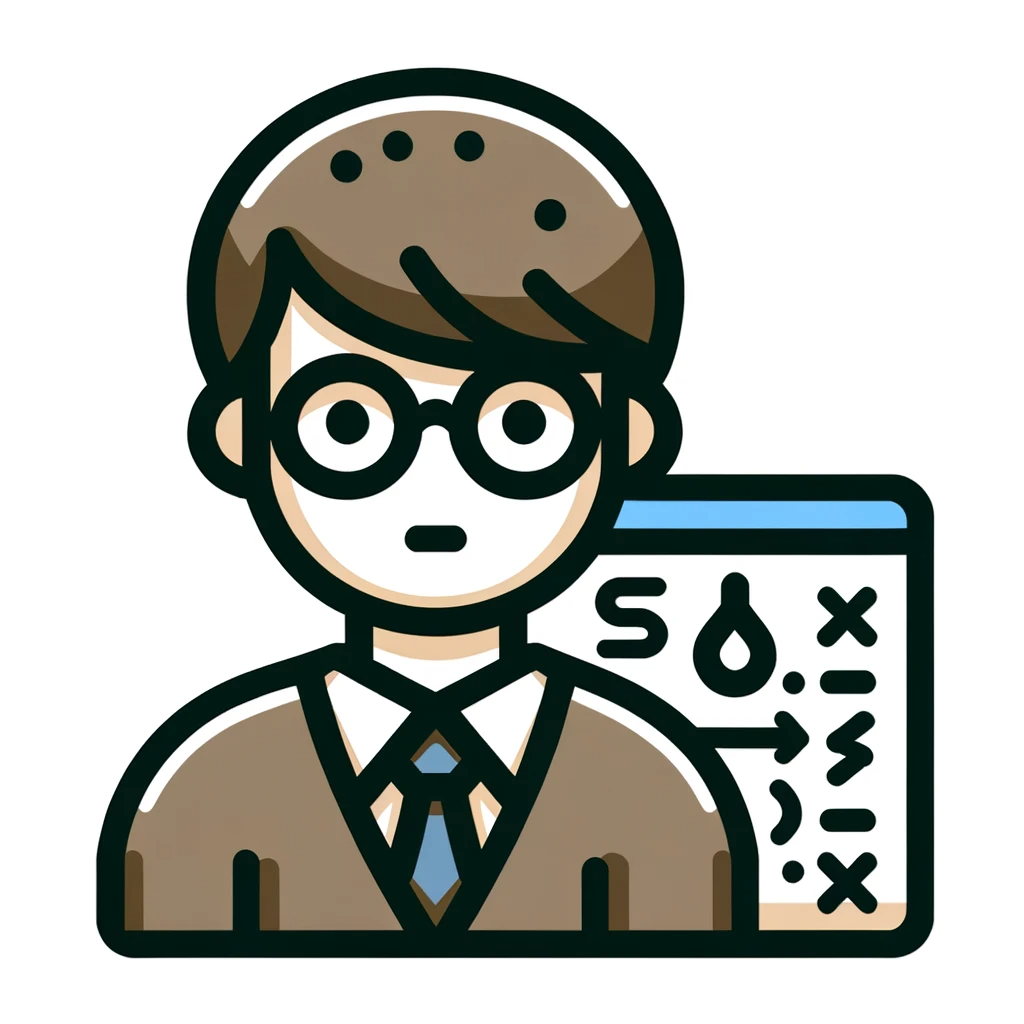
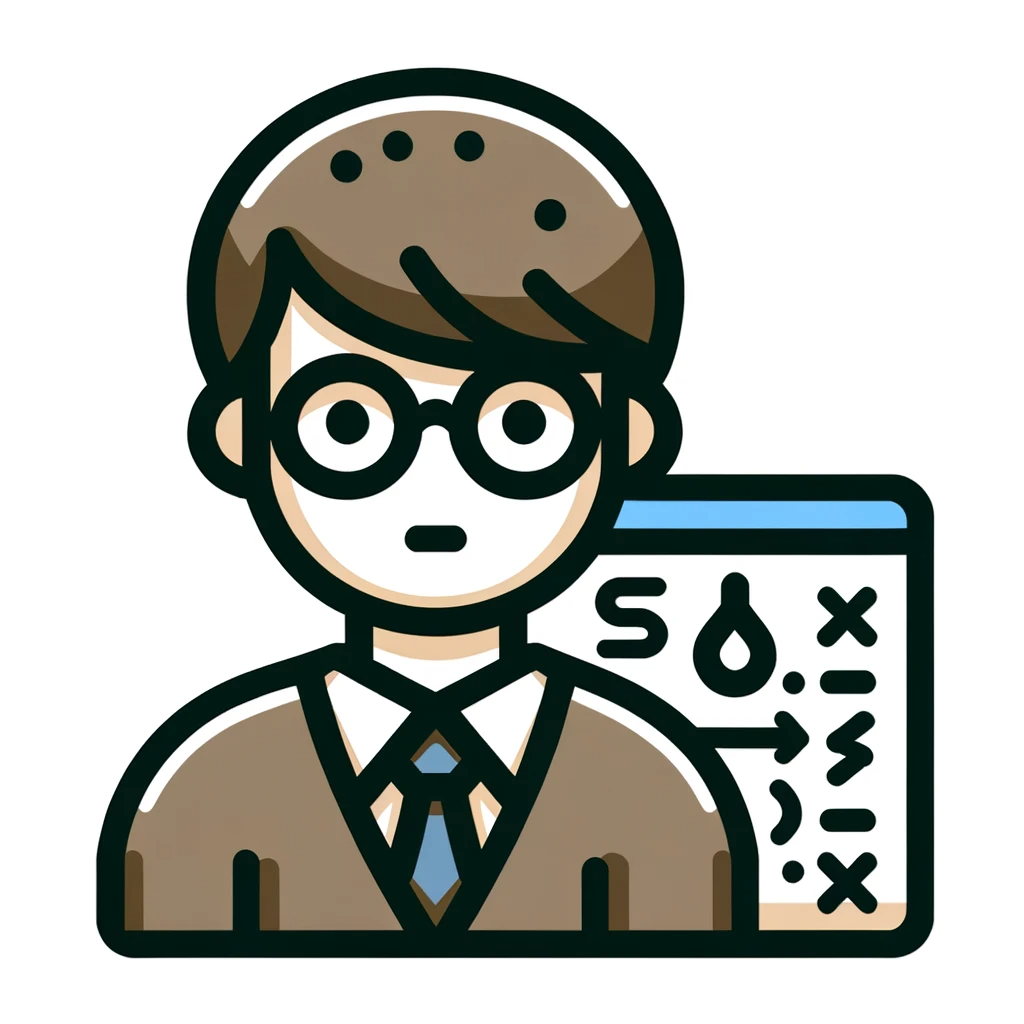
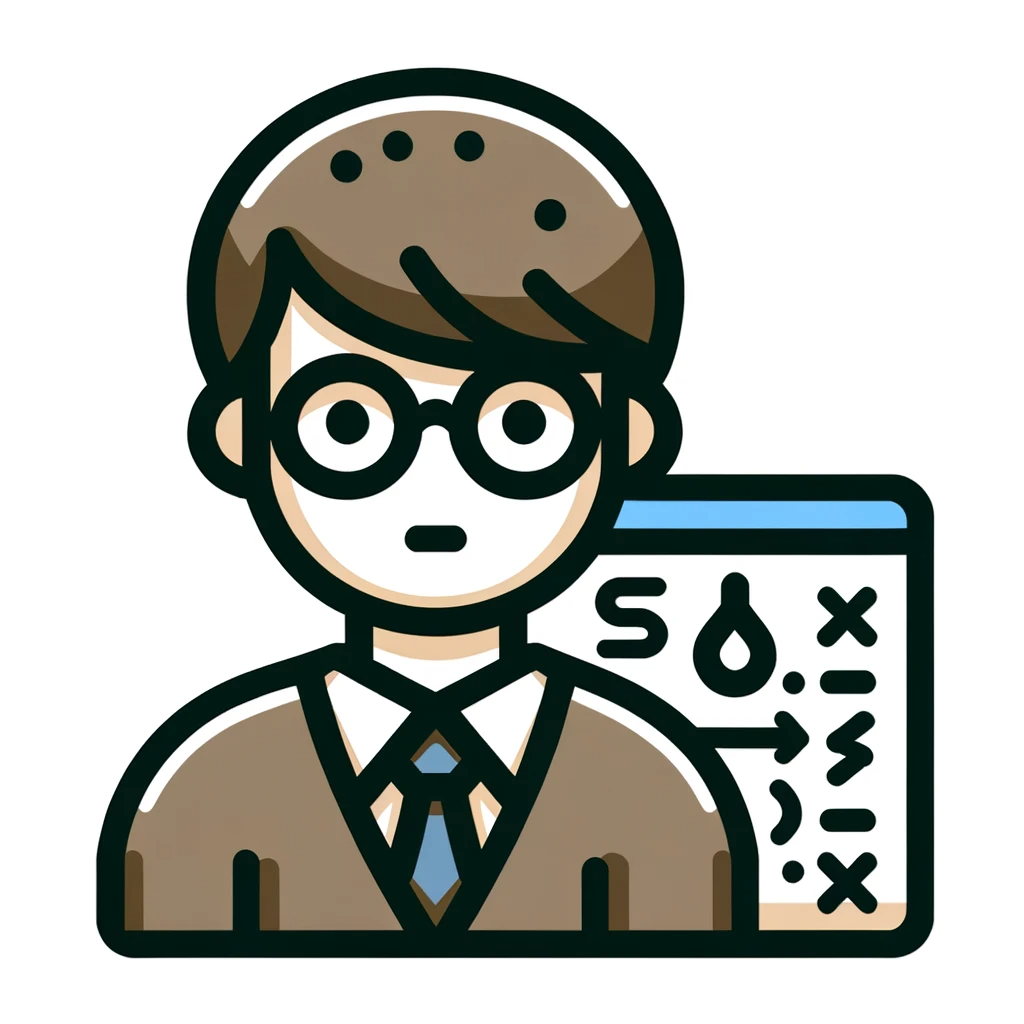
Knowing the position of elements is important when working with arrays, so try using these methods to take advantage of arrays.
Comments