This section explains how to use increment and decrement .
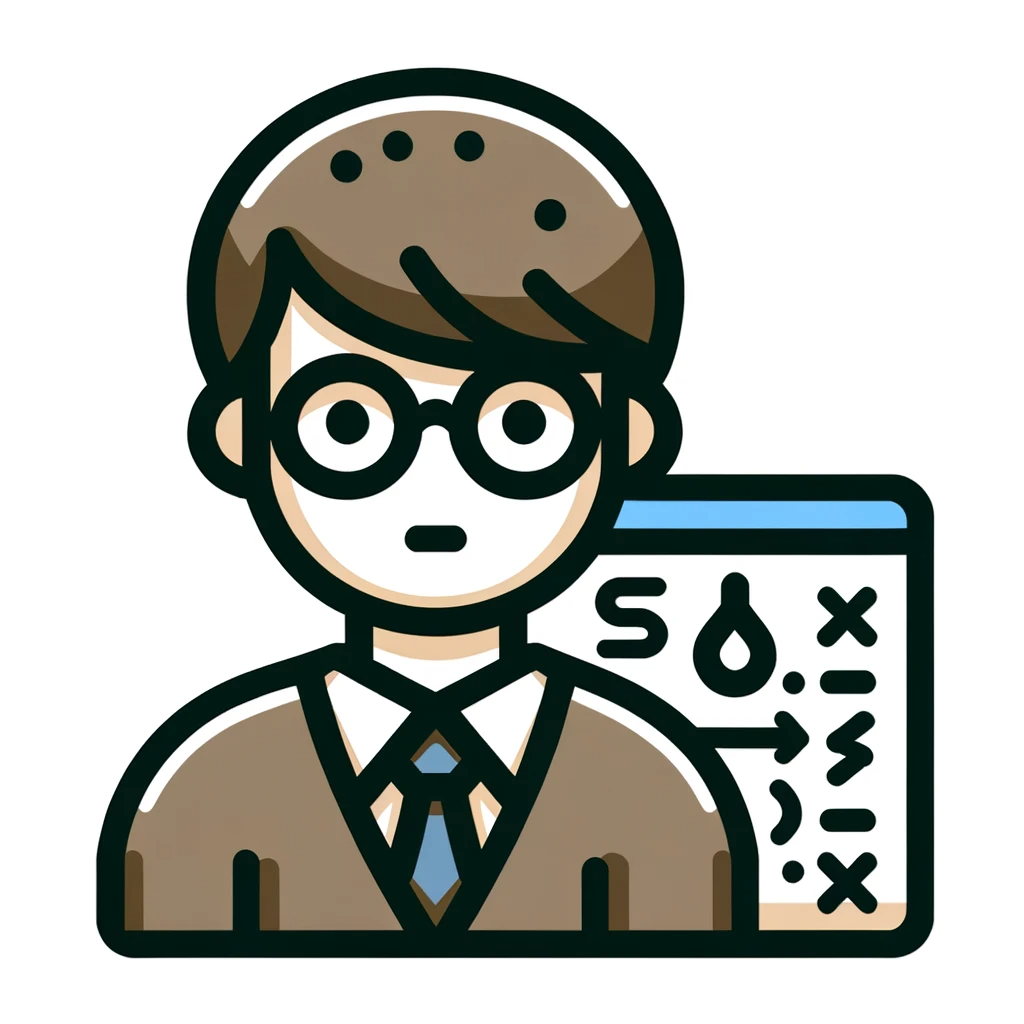
Increment (++) and decrement (–) are operators that allow you to increase (or decrease) the value of a variable by 1.
How to use the increment operator (++) and decrement operator (–)
You can increase (increment) or decrease (decrement) the value of a variable by using the increment operator (++) and decrement operator (–) .
x++;
x–;
For example, you can increase the value of variable x by 1 as shown below.
let x = 10;
x++;
console.log(x); // 11
You can also decrease the value of variable x by 1 as shown below.
let x = 10;
x--;
console.log(x); // 9
You can also decrease the value of variable x by 1 as shown below.
let x = 10;
x--;
console.log(x); // 9
In this way, the basic usage is that the increment operator increases the value of a variable by 1, and the decrement operator decreases the value of the variable by 1.
Difference between prefix and postfix operators
There are two ways to increment and decrement: prefix operators (++x, –x) and postfix operators (x++, x–).
- Prefix operators operate on variables before using the operator .
- Postfix operators operate on variables after using the operator .
For example, if you use the prefix operator as shown below,
let x = 10;
console.log(++x); // 11
If you use the postfix operator as below,
let x = 10;
console.log(x++); // 10
In this way, prefix and postfix operators differ in the timing at which variables are manipulated before and after the operator is used.
for loop with increment and decrement
A for loop with increment ++
and decrement operators --
can be a useful coding pattern under certain conditions.
++
In a for loop, you can use the increment and decrement operators to increase or decrease the value of a variable by 1 --
. By incorporating these into the conditional expression of a for loop, you can perform loop processing while controlling the values of variables.
Example of a for loop with increment
Below is ++
an example of a for loop using an increment operator.
for (let i = 0; i < 10; i++) {
console.log(i);
}
This code i
initializes starting at 0 and looping through increments of 1 i
as long as is less than 10 . i
As a result of the loop, the numbers from 0 to 9 are printed to the console in sequence.
Example of a for loop with decrement
Below is --
an example of a for loop using the decrement operator.
for (let i = 10; i > 0; i--) {
console.log(i);
}
This code i
initializes , starting at 10, and loops down by 1 i
as long as is greater than 0 . i
As a result of the loop, the numbers from 10 to 1 are printed to the console in sequence.
Application example of for loop using increment and decrement
The following is an example of an application that combines the increment operator ++
and the decrement operator --
.
for (let i = 0, j = 10; i < j; i++, j--) {
console.log(`i: ${i}, j: ${j}`);
}
In this code, variable i is initialized and starts at 0, and variable j is initialized and starts at 10. It then loops through increasing i by 1 and simultaneously decreasing j by 1 as long as i is less than j.
The result of the loop alternates between the values of variable i and variable j, as shown below. The following is the output result.
i: 0, j: 10
i: 1, j: 9
i: 2, j: 8
i: 3, j: 7
i: 4, j: 6
In this way, multiple variables can be specified in the conditional expression of a for loop, and loop processing can be performed while controlling them.
summary
Let’s summarize about increment and decrement.
- The increment operator (++) increases the value of a variable by 1
- The decrement operator (–) reduces the value of a variable by 1
- Prefix and postfix operators differ in the timing at which variables are manipulated before and after the operator is used.
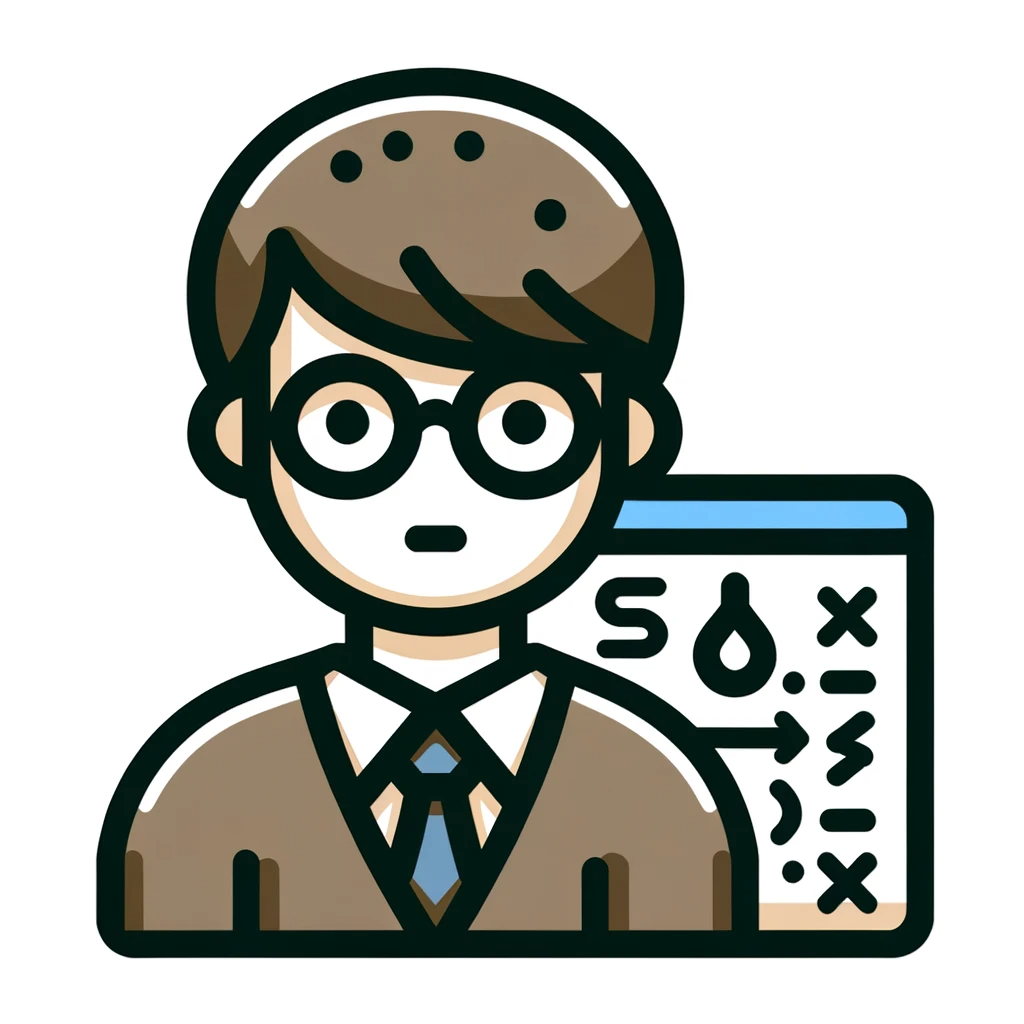
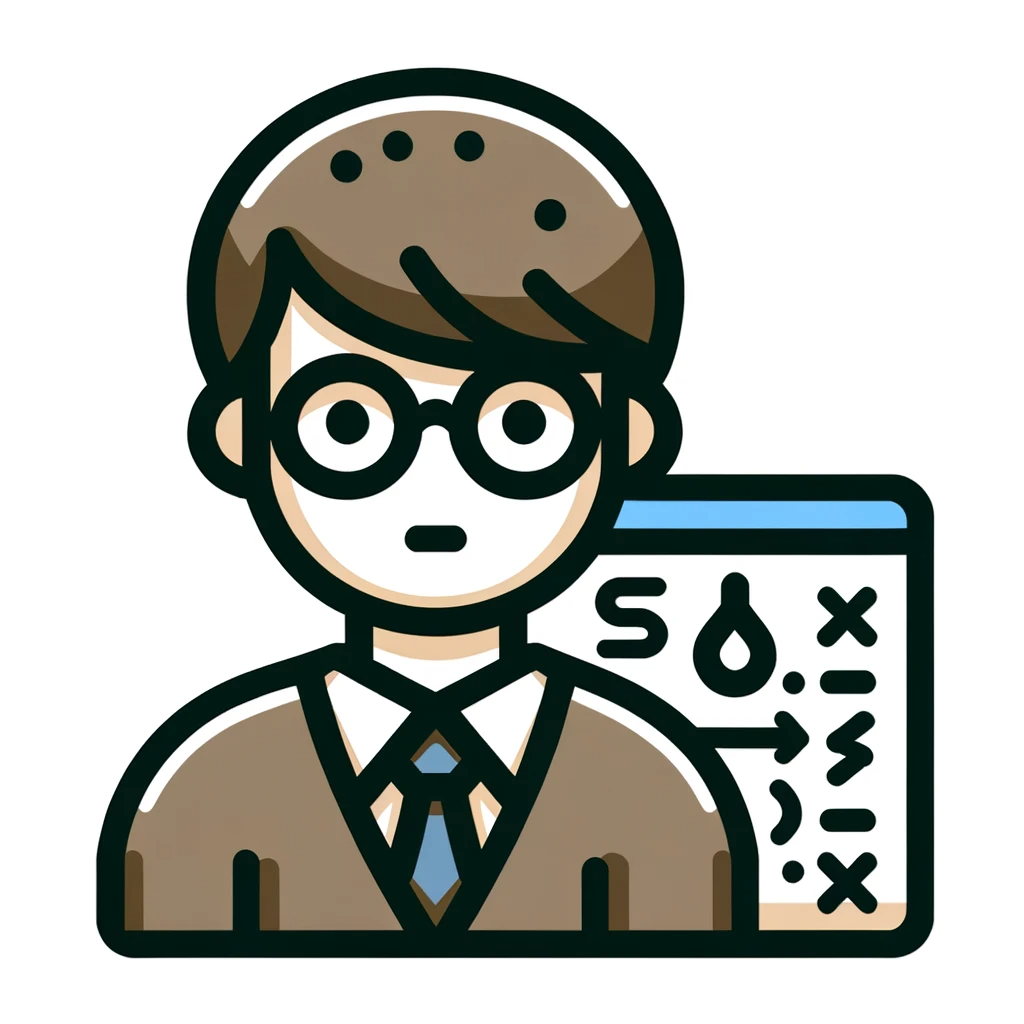
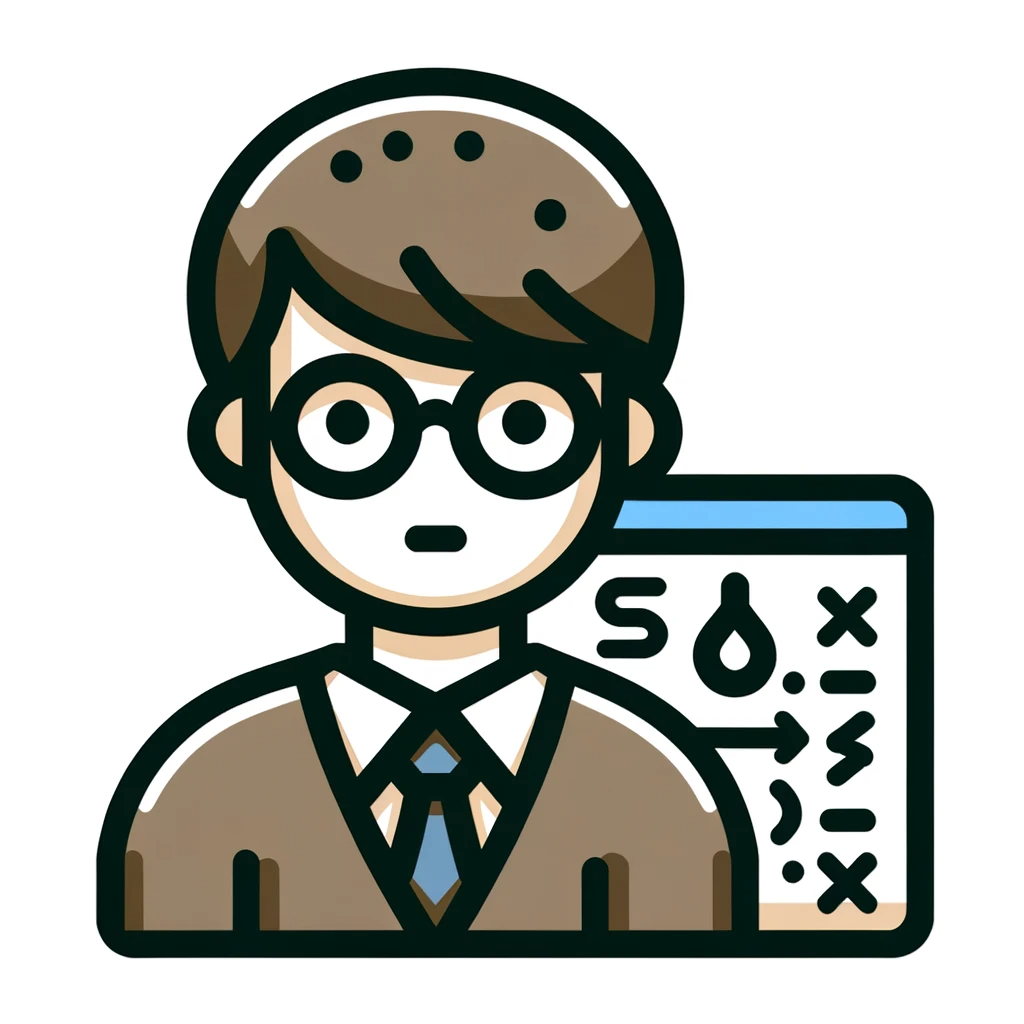
Incrementing and decrementing are very useful because they allow you to easily manipulate variables, such as during iteration.
Comments