This article explains how to retain the current page state in the web page history by using JavaScript’s pushState method.
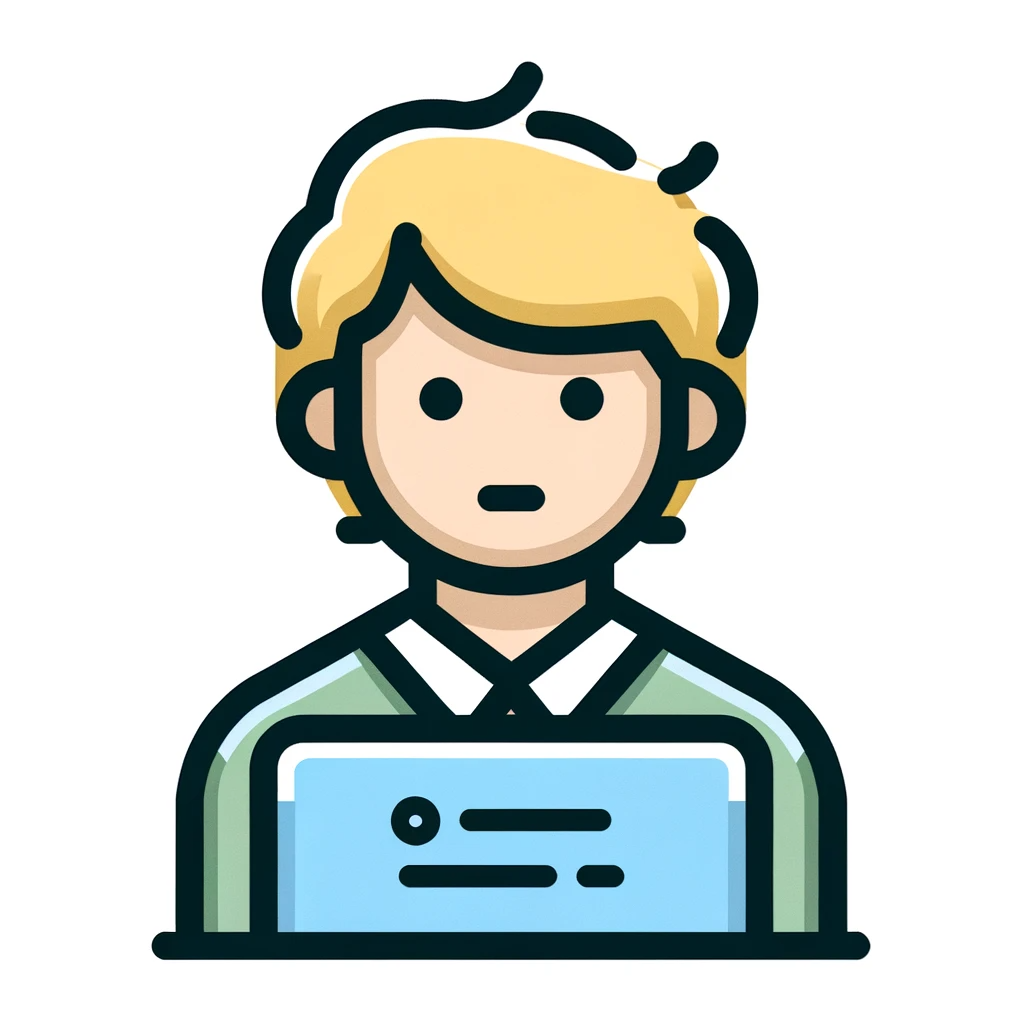
How can I manage my history?
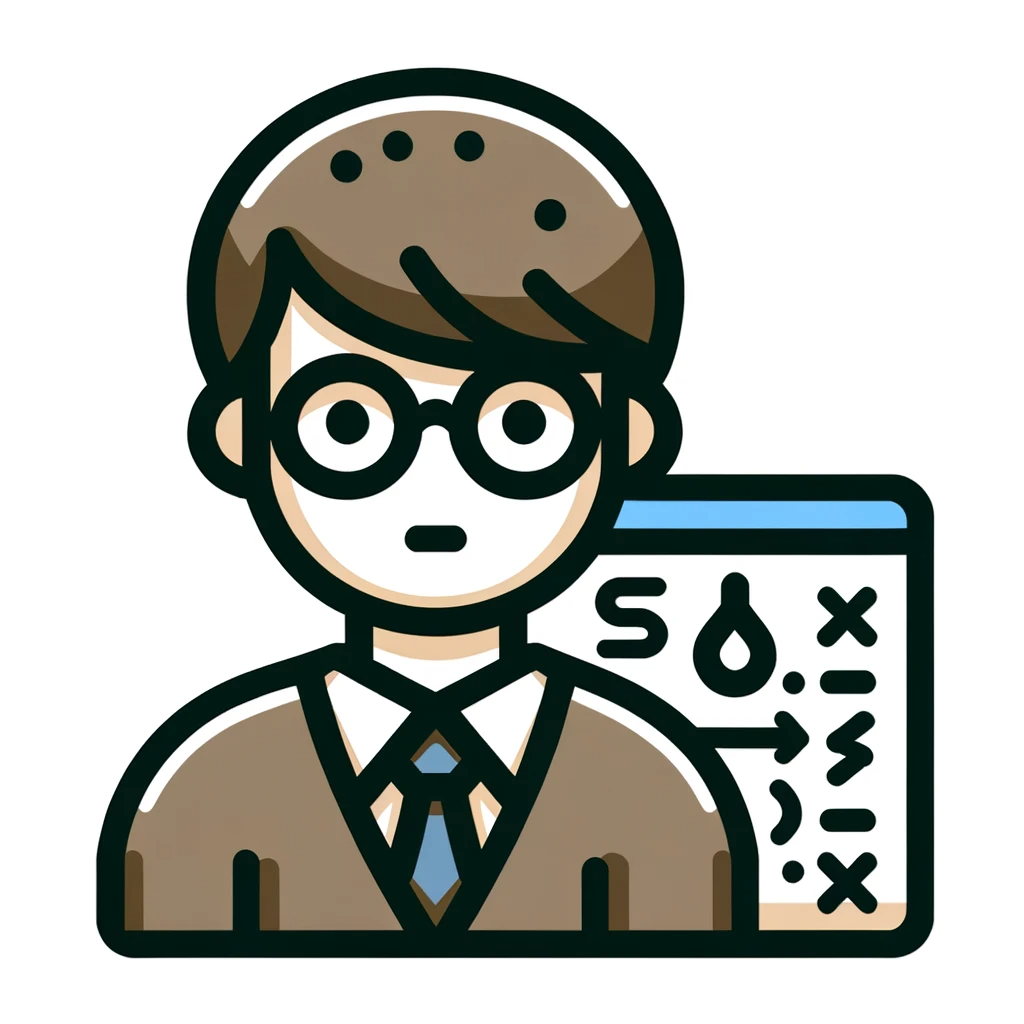
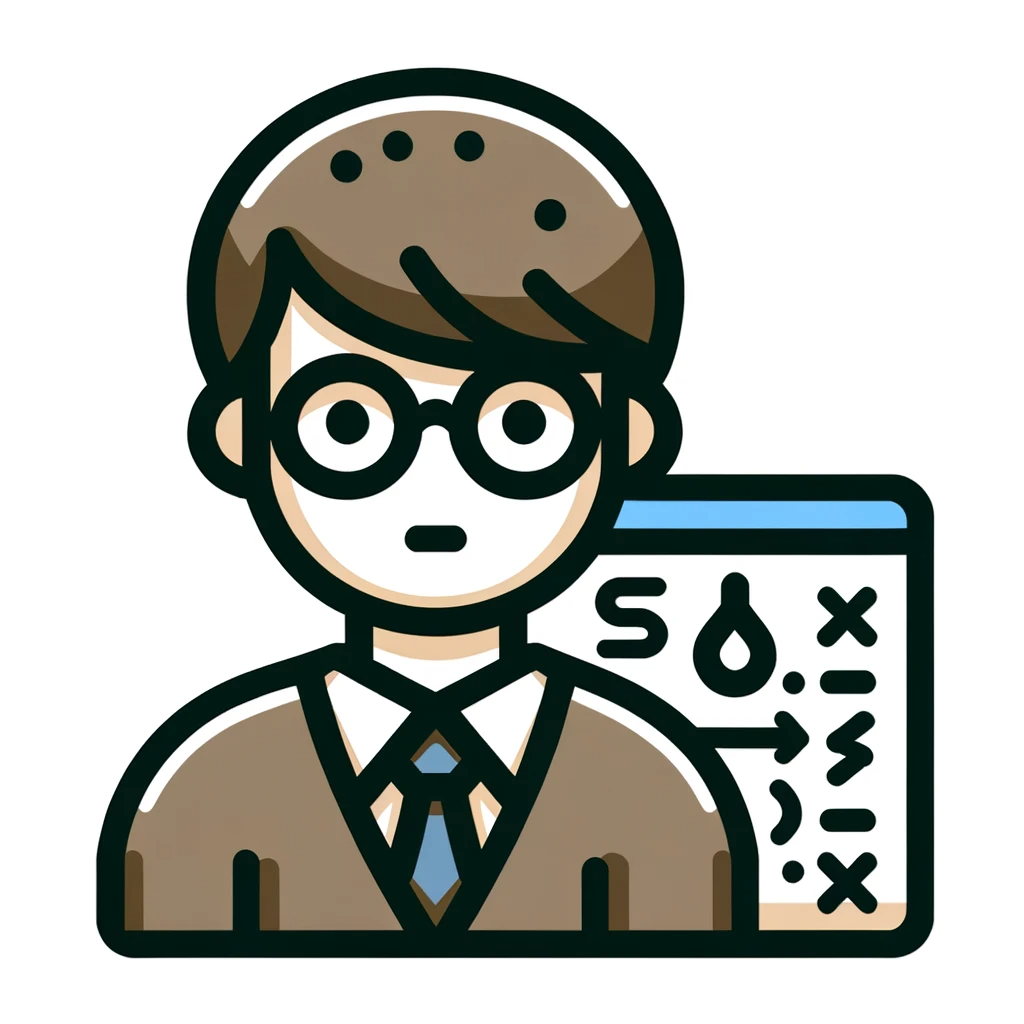
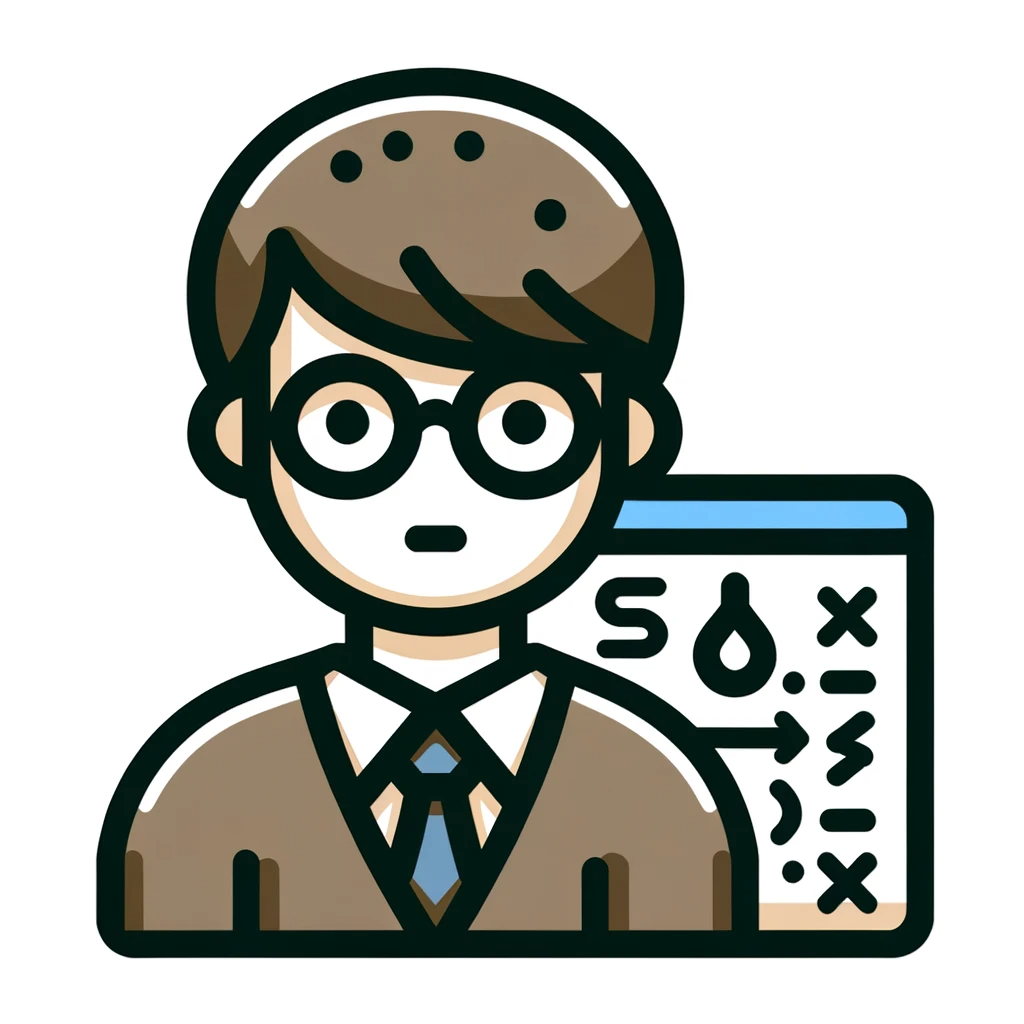
Use the pushState method to manage history.
The pushState method is a history management method that allows you to maintain the current page state in the web page history.
What is pushState method?
The pushState method is a history management method introduced in HTML5.
When viewing a web page, you may use your browser’s “back” or “forward” buttons to return to a page you have viewed in the past or a page you would like to view again. When you perform page transitions like this , the browser manages the history.
You can use JavaScript to add the current page state to the history by using the pushState method . For example, if part of your website is loaded using Ajax, you may not be seeing a new page, but new content being displayed using JavaScript. In this case, you can use the pushState method to add the correct page state to the history.
The pushState method also ensures that the correct page state is maintained as the user moves back and forth through the page. This prevents users from encountering problems when transitioning between pages.
The pushState method has the following syntax:
window.history.pushState( state, title, url );
state
: State object in object format. Information representing the state can be saved.title
: Title. A string that is displayed in a browser tab.url
:URL. Specify the URL to add to history.
You can add new entries to the browser’s history by using the pushState method. This ensures that the correct page state is maintained as you navigate back and forth through the page.
Explanation using a sample program
The sample code below adds the query parameter “?page=2” to the current URL and adds a new entry to the history by clicking a button.
<button onclick="addPageTwo()">Go to Page 2</button>
<script>
function addPageTwo() {
var stateObj = { page: 2 };
var title = "Page 2";
var newUrl = "?page=2";
history.pushState(stateObj, title, newUrl);
}
</script>
Executing this sample code will change the URL to https://example.com/?page=2 and add a new entry to the browser history.
The pushState method adds the new entry to the history stack for the current page and changes the history state. It passes stateObj, title, and newUrl as arguments. These arguments are used to identify the new history state.
By executing this program, you can dynamically change the state within a page and still be able to return to the previous state using the browser’s “back” button.
Thus, the pushState method makes it easy to manage the history of a Web page.
summary
We explained how to retain the current page state in the web page history by using the pushState method.
- The pushState method is a history management method introduced in HTML5.
- You can use JavaScript to add the current page state to the history by using the pushState method.
- Using the pushState method ensures that the correct page state is maintained as the user moves back and forth through the page.
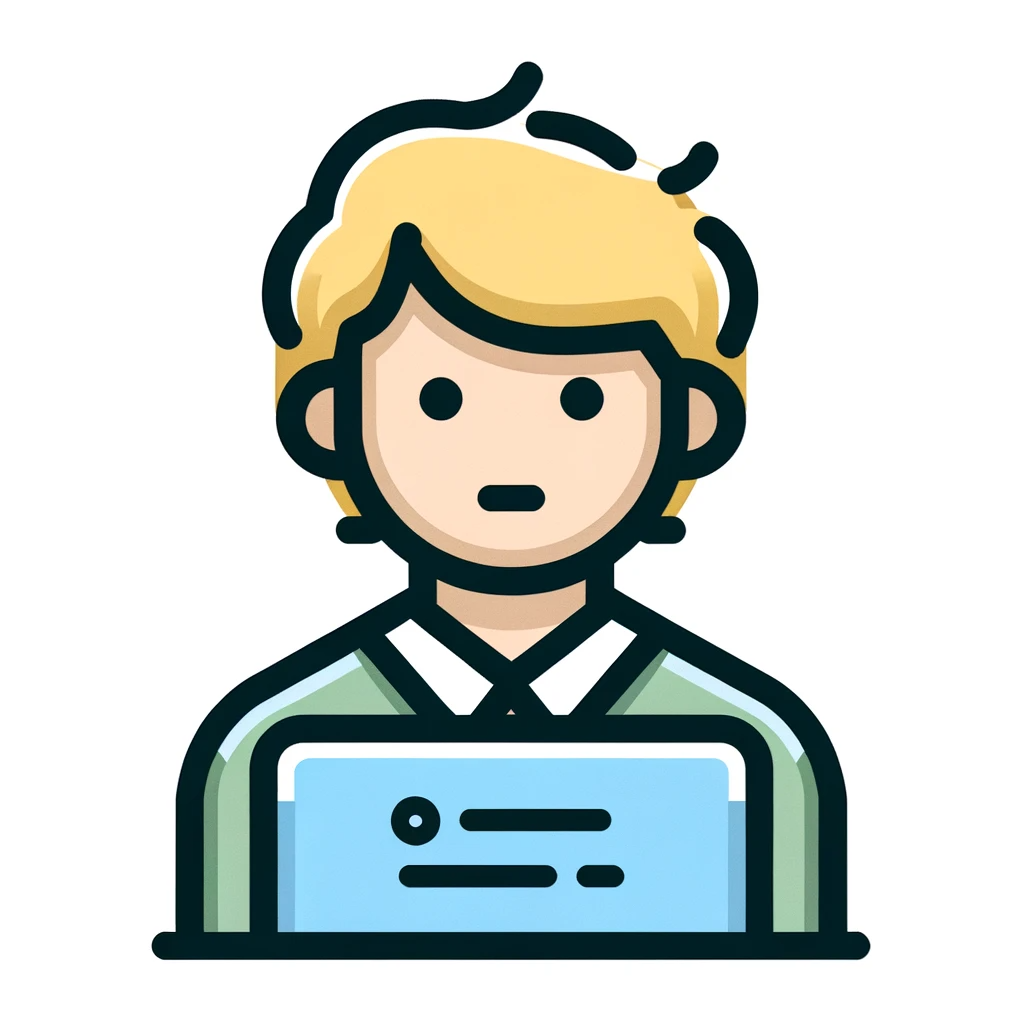
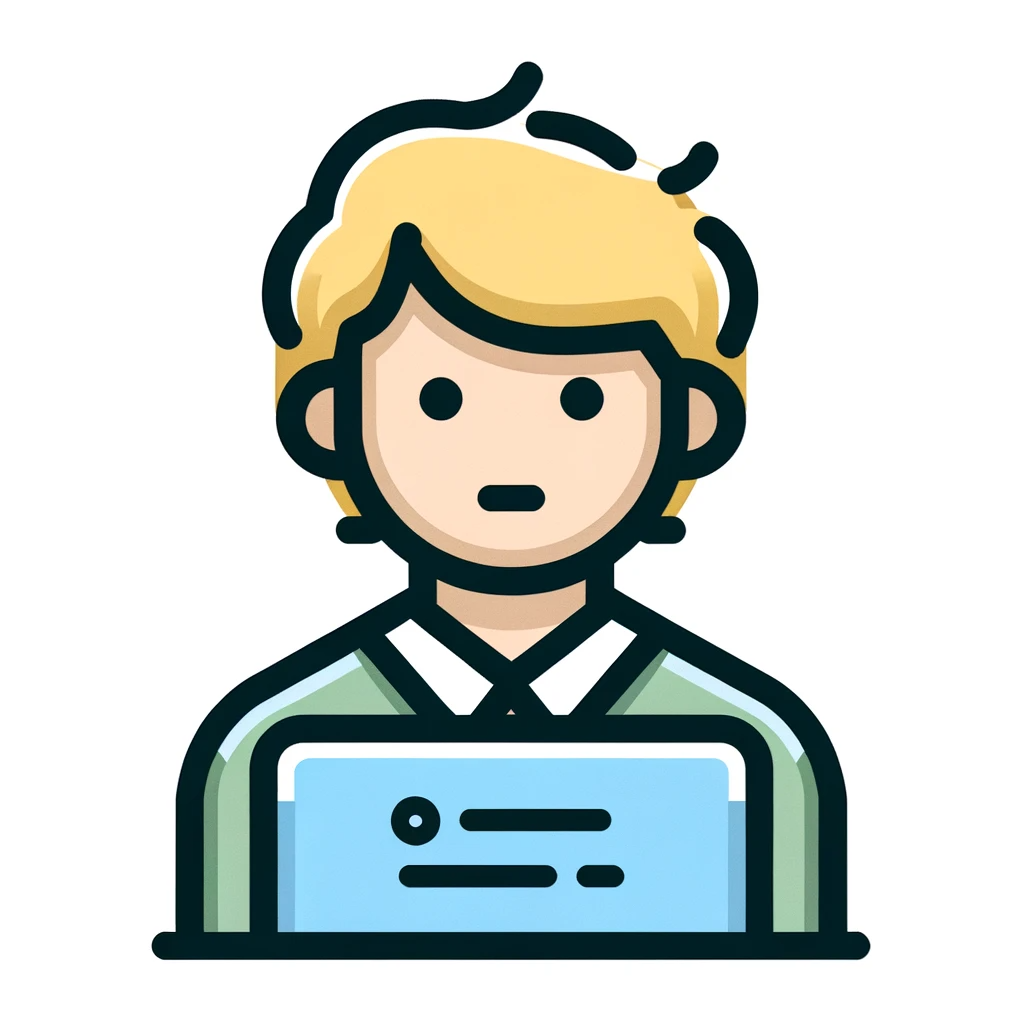
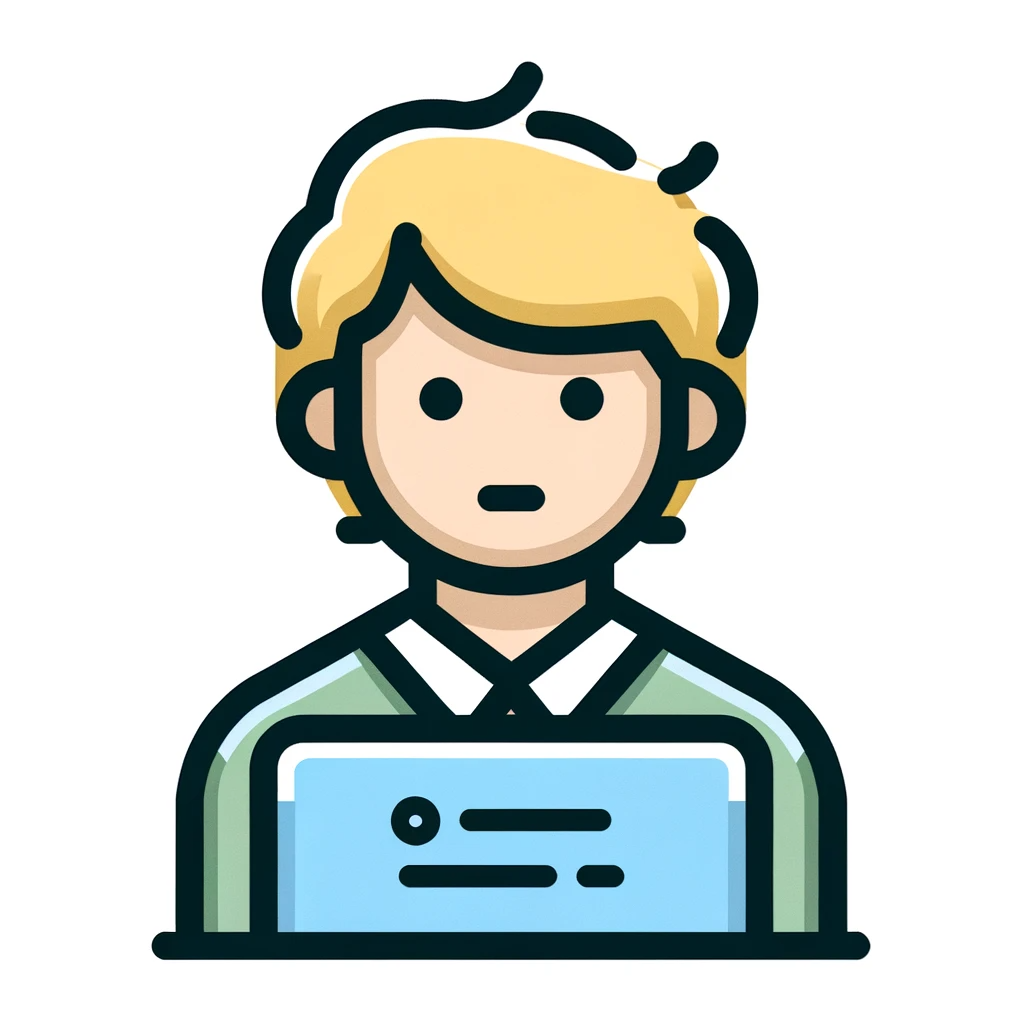
I would like to use the pushState method to effectively manage the history of web pages.
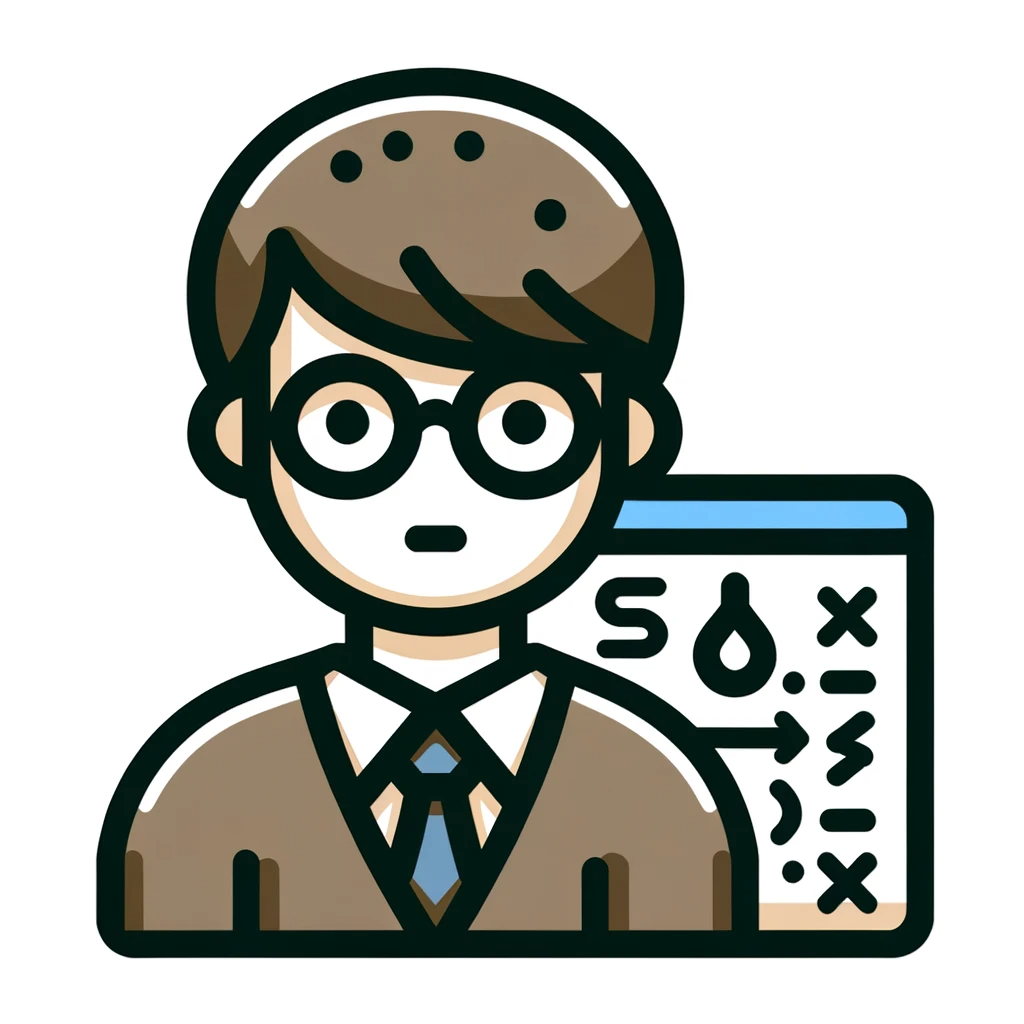
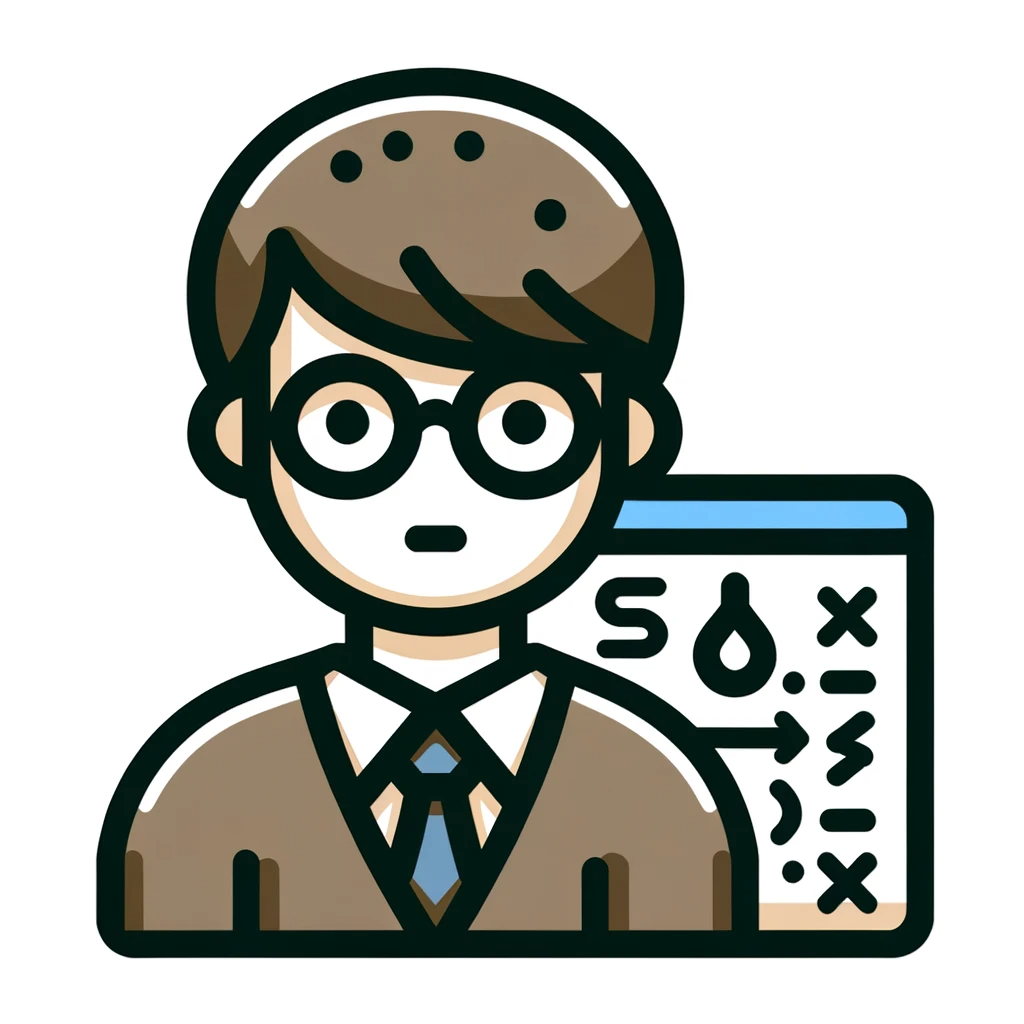
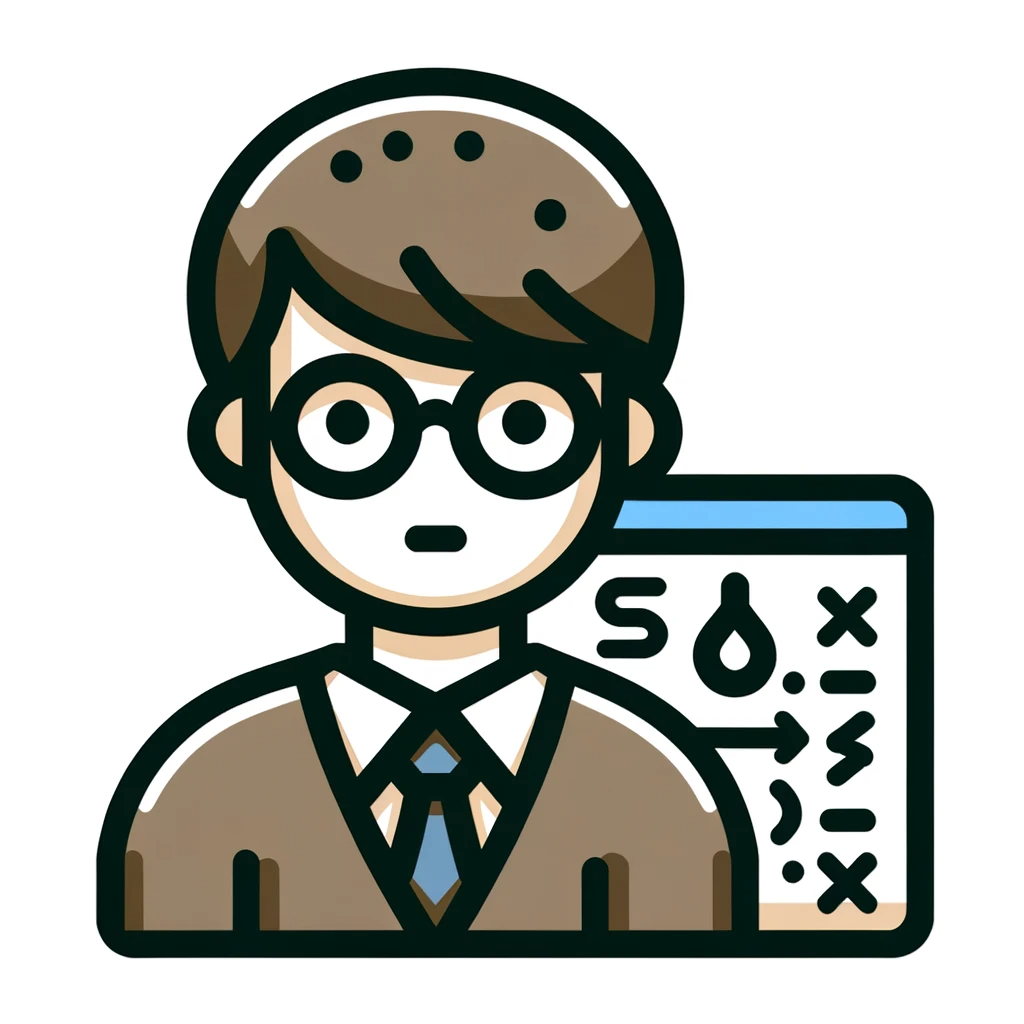
Effective use of web page history management can help improve the user experience.
Please try using the pushState method to make your web pages easier to use.
Comments