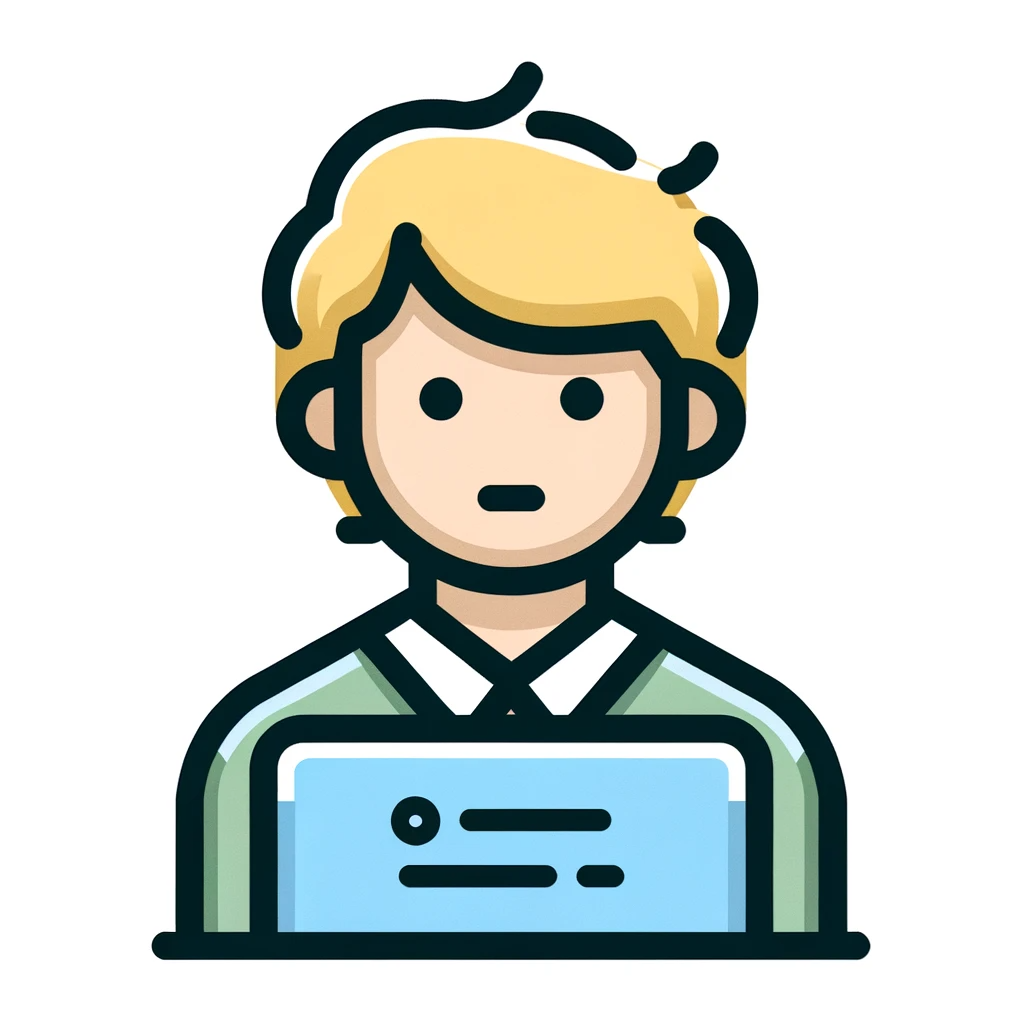
What are associative arrays and Maps in JavaScript?
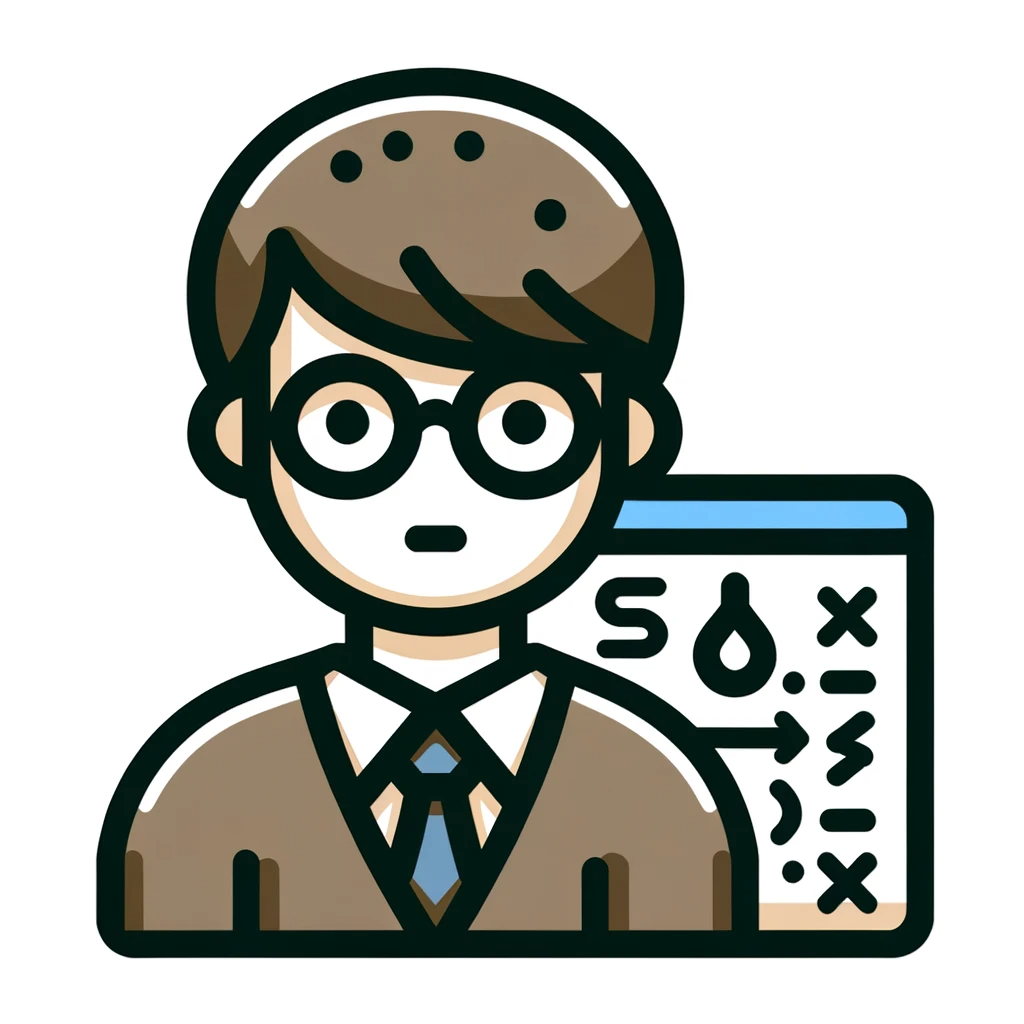
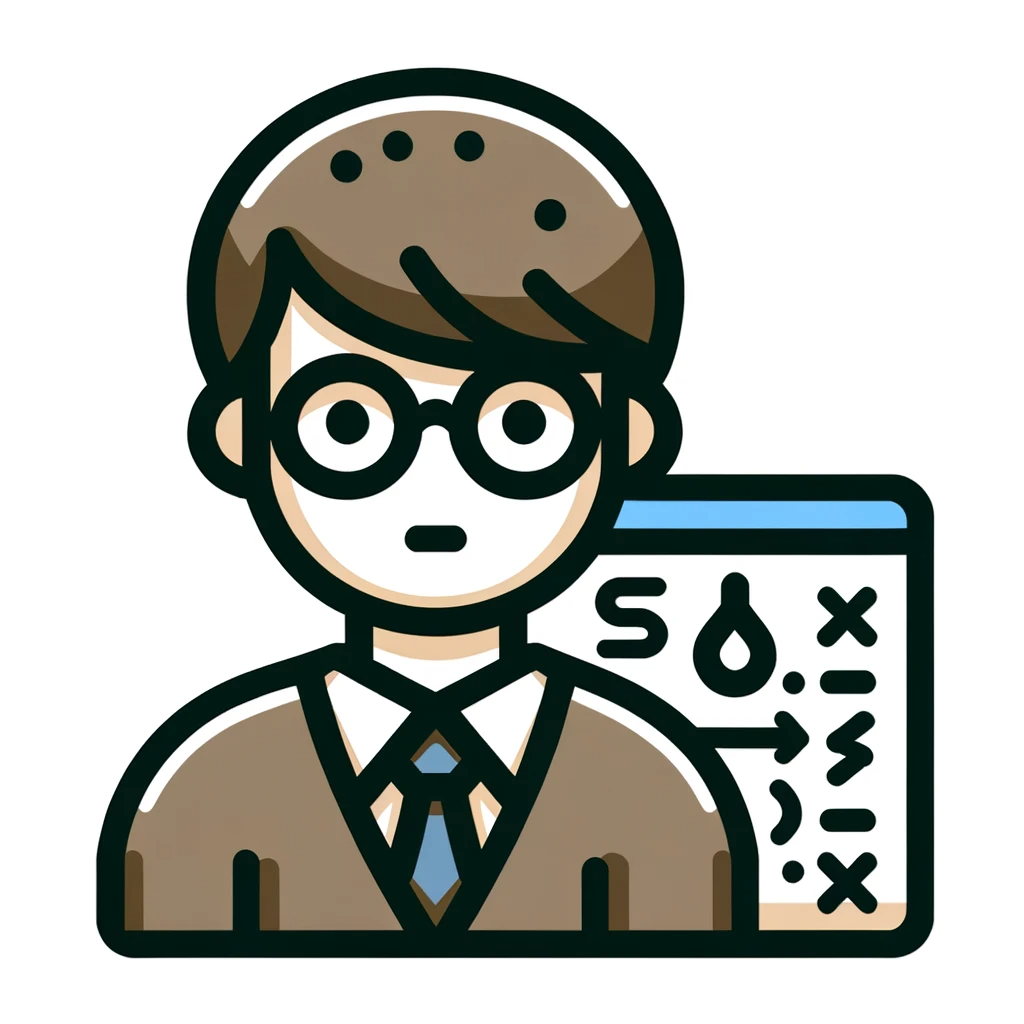
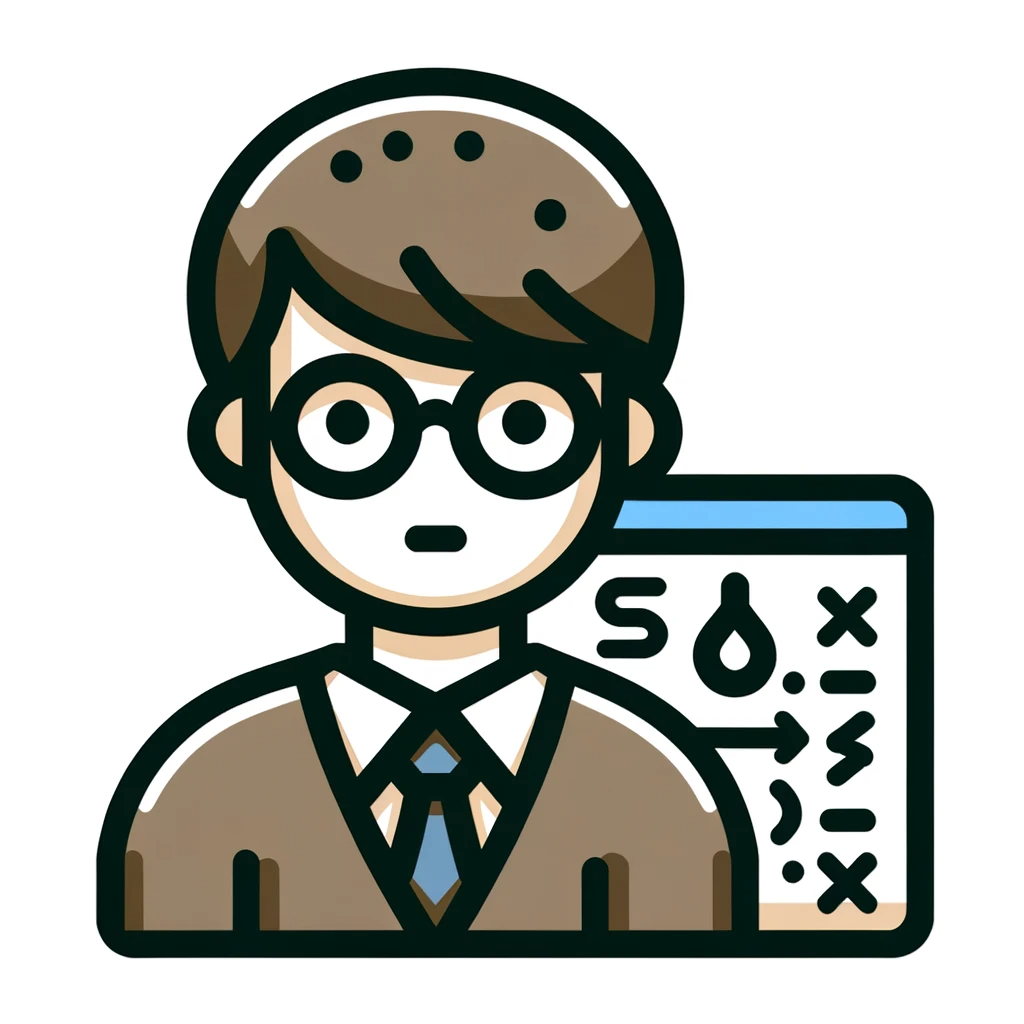
Now, let’s learn about associative arrays and Maps in JavaScript.
An associative array is an array that can be accessed using names as keys.
Maps can store data as key-value pairs.
What is an associative array?
An “associative array” is an object that has key-value pairs, and is a type of JavaScript object.
Regular arrays use numbers as indexes to store values, whereas associative arrays use arbitrary values, such as strings or numbers, as keys to store their corresponding values.
How to use associative arrays
Associative arrays are implemented as JavaScript objects, so to use an associative array you need to create an object and define key-value pairs within it .
Object keys can be strings, numbers, symbols, etc.
Below, we use an associative array to store data and access it by name.
const object = {
key1: 'value1',
key2: 'value2',
key3: 'value3',
};
console.log(object.key1); // 'value1'
For example, you can create an associative array with key-value pairs by defining an object as shown below.
const fruits = {
apple: 100,
banana: 200,
orange: 300
};
In the above example, we define an object called fruits, which defines the keys apple, banana, and orange, and the corresponding values. In this case, a string is used as the key.
Add property
You can also add properties as below.
fruits.grape = 400;
In the above example, grape is added as a new key to the fruits object. grape’s value is set to 400.
access keys
To retrieve the value of an associative array, specify the key and access it as shown below.
console.log(fruits.apple); // 100
console.log(fruits['banana']); // 200
In the above example, the values corresponding to the keys “apple” and “banana” are retrieved from the fruits object. fruits.apple and fruits[‘banana’] can be used to specify keys, such as . or [] can be used to specify keys.
Update value by specifying key
You can also update the value by specifying the key as shown below.
fruits.apple = 150;
In the above example, the value corresponding to apple in the fruits object is updated to 150.
Thus, objects can be used to easily create and manipulate associative arrays with key/value pairs.
What is a Map object?
A Map object is a data structure added in ES6, and is one of the data structures for realizing an associative array with key-value pairs . Like associative arrays, they can hold key-value pairs, but unlike objects, keys can be of different types.
Below, Map
we use to store data and retrieve the value by specifying the key.
const map = new Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
map.set('key3', 'value3');
console.log(map.get('key1')); // 'value1'
How to use Map objects
For example, you can define a Map object like this:
const fruits = new Map([
['apple', 100],
['banana', 200],
['orange', 300]
]);
In the above example, a Map object is created using new Map() and key/value pairs are defined as an array. The first element of the array is the key and the second element is the value.
Add key-value pairs with set
In a Map object, you can also add key/value pairs using the set() method. For example, the set() method can be used to add a new key/value pair as follows.
fruits.set('grape', 400);
In the above example, the set() method is used to add the key ‘grape’ and value pair 400.
Get the value corresponding to the key with get
The Map object get()
allows you to use methods to retrieve the value corresponding to a key. For example, you can use the following get()
method to get the value corresponding to a key.
console.log(fruits.get('apple')); // 100
console.log(fruits.get('banana')); // 200
In the above example, the get() method is used to retrieve the value corresponding to the key ‘apple’ or ‘banana’.
Like an associative array, a Map object can hold key/value pairs, but it can use keys of various types, allowing for flexibility of use. The size property can also be used to obtain the number of key/value pairs contained in a Map object.
Map can use any type as a key, not just strings. Object literals can use property names as keys, but only strings can be used as keys. However, Map objects allow you to use any type as a key. For example, NaN and undefined can also be used as keys.
Difference between associative array and Map
The main difference between associative arrays and Map objects is how keys are handled. Associative arrays can use strings as keys, but Map objects can use various types as keys.
Specifically, associative arrays can use strings as keys. On the other hand, Map objects can use types other than strings as keys.
Also, Map objects size
can use properties to get the number of key-value pairs, but with associative arrays there is no way to get the number of key-value pairs.
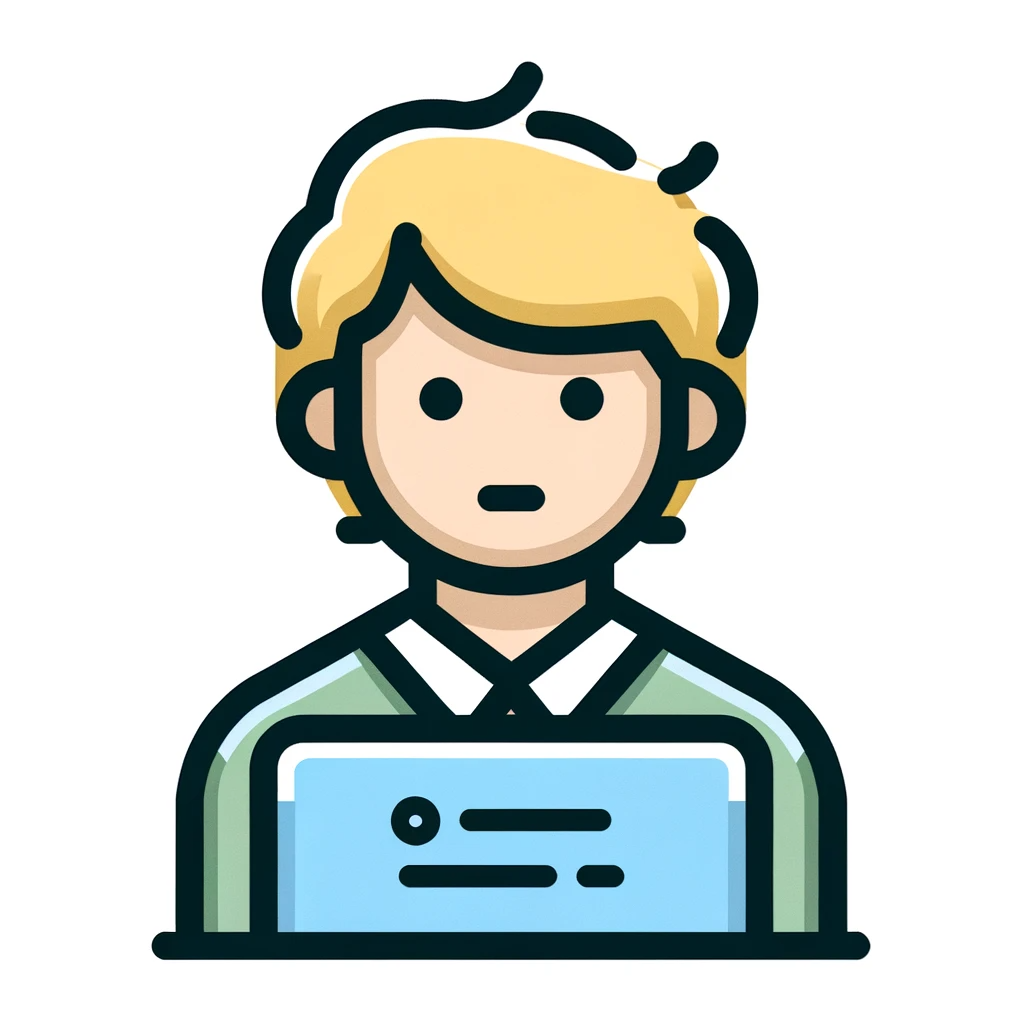
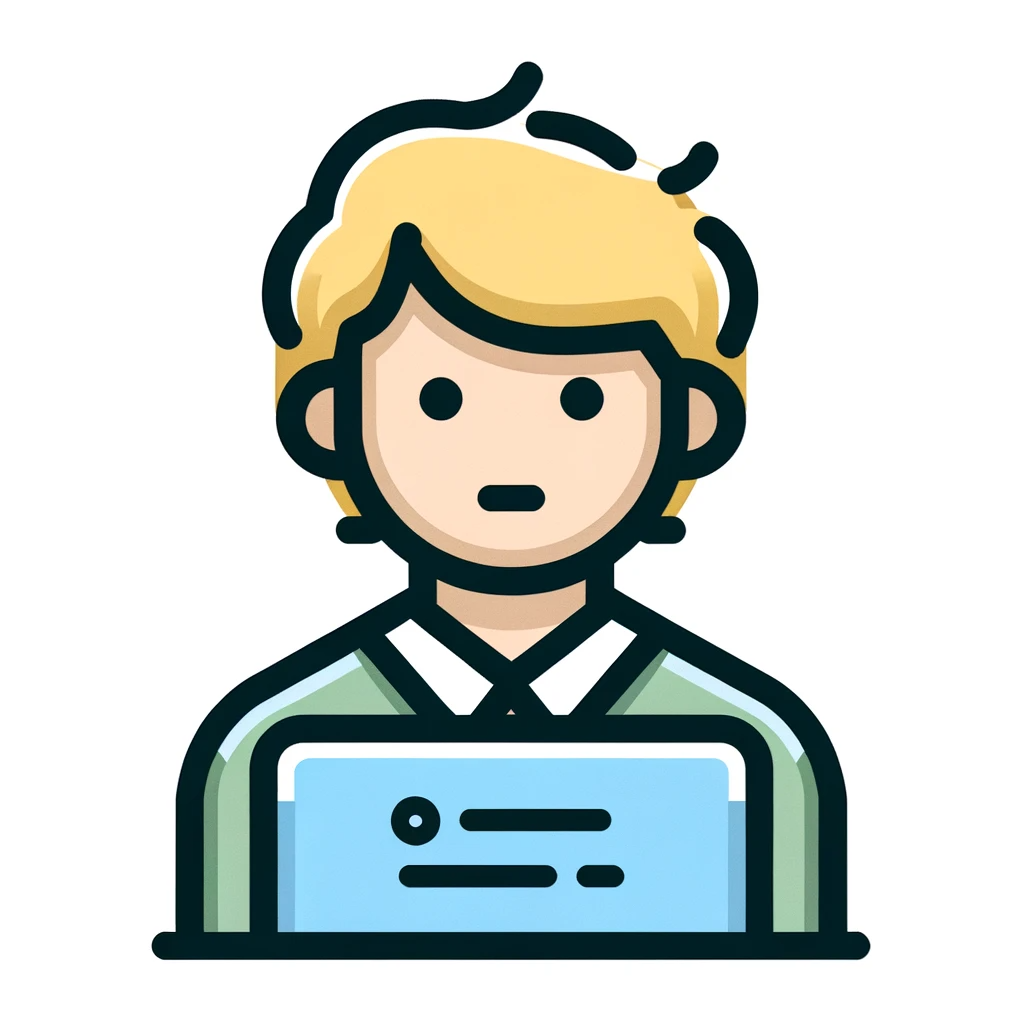
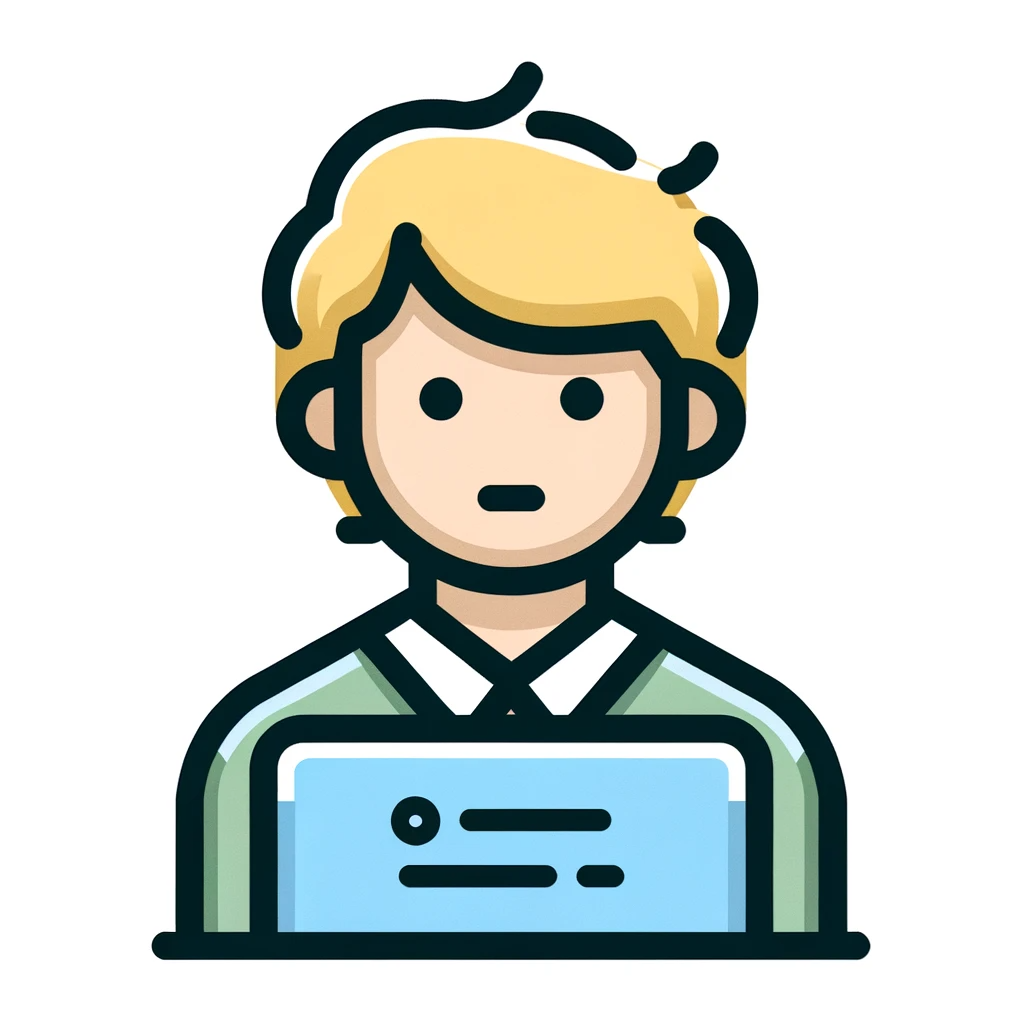
Associative array and Map are very similar, but which one should I use?
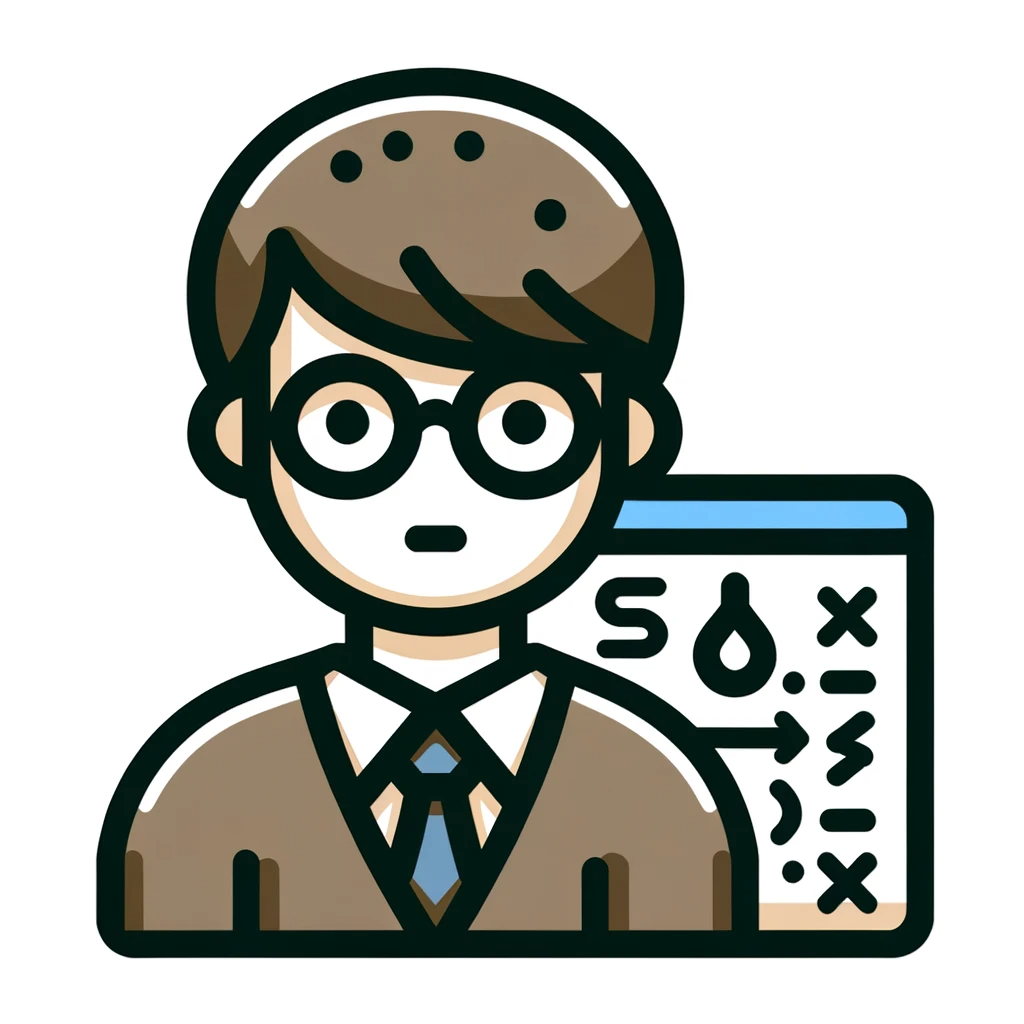
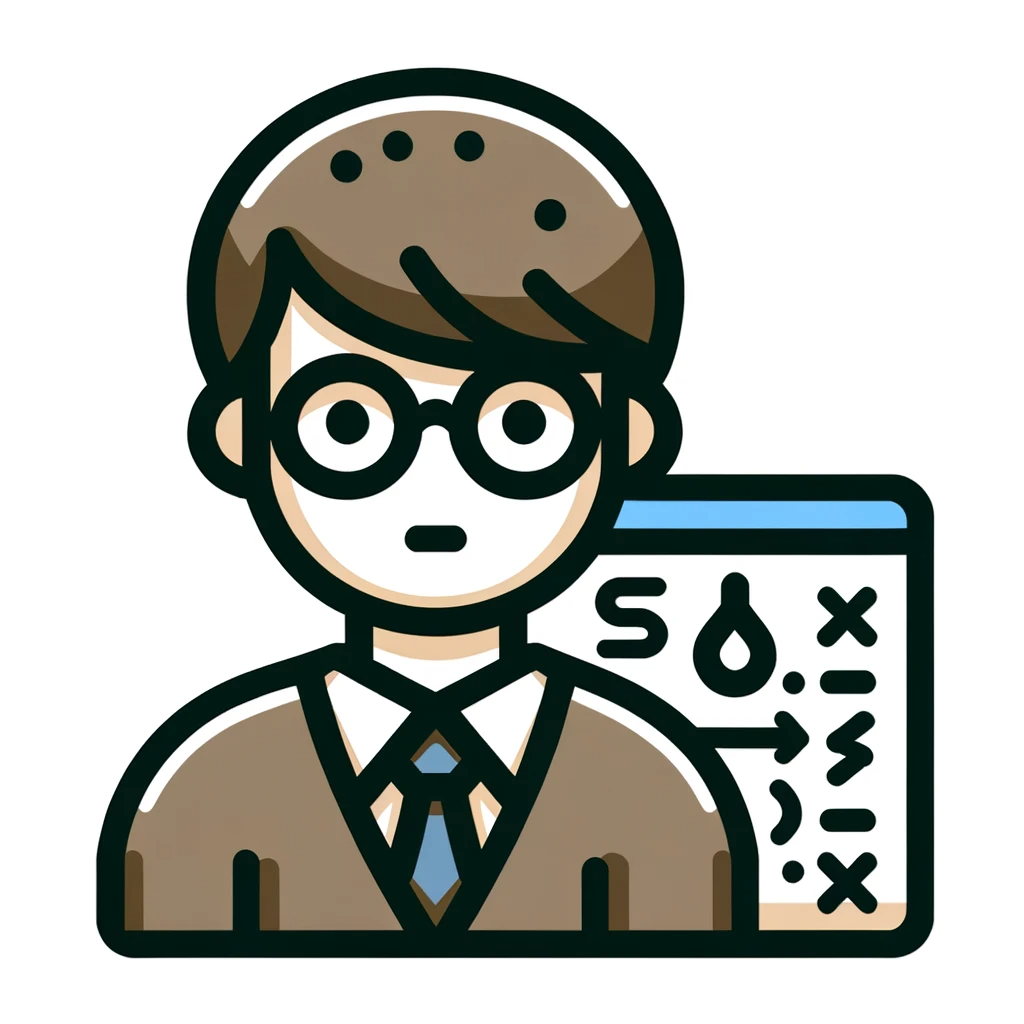
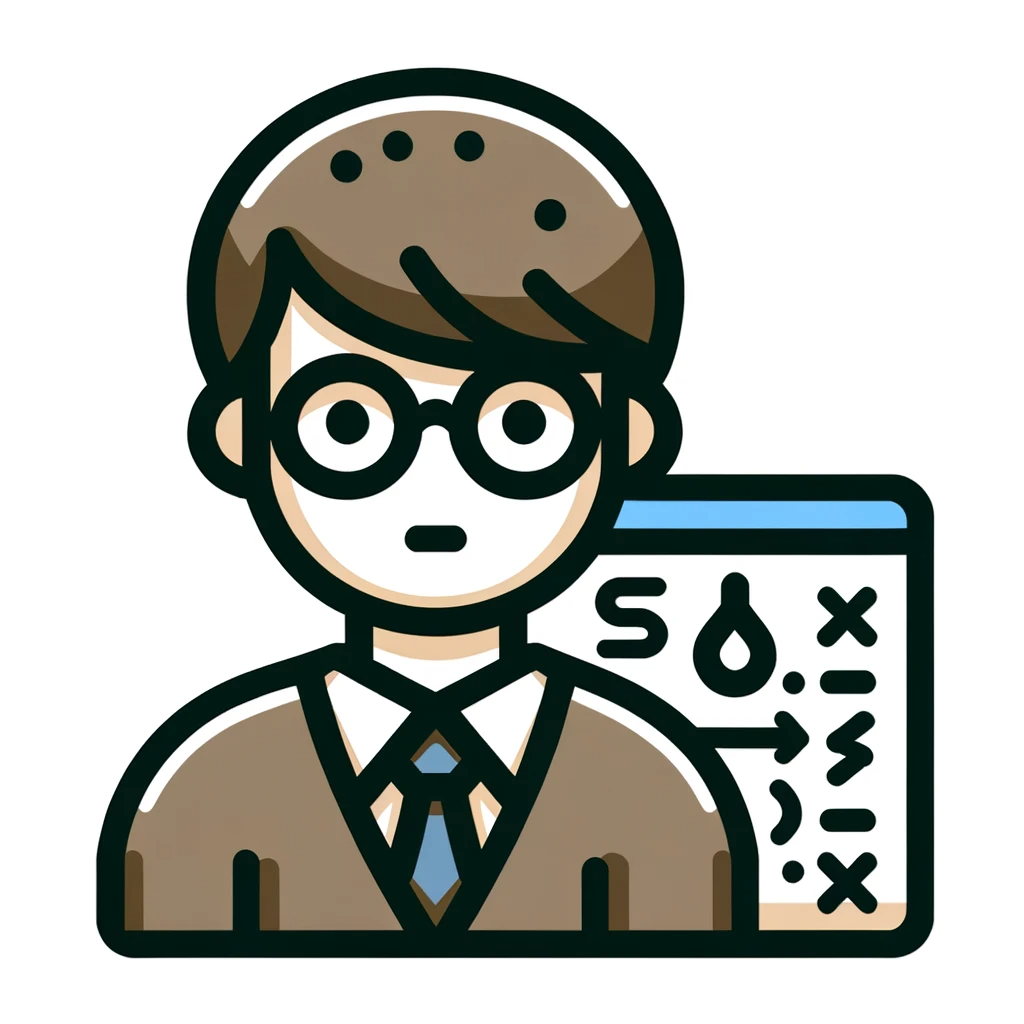
The new code encourages the use of Maps whenever possible; they are superior to associative arrays because they are more flexible and can maintain order.
summary
This is a summary of “Learning how to use associative arrays and Maps”.
- Associative arrays can store data as key-value pairs.
- Associative arrays allow you to access data by name.
- Map is a data structure that can use different data types as keys.
- A Map is similar to an associative array, but you can use any type as a key, not just strings.
- Map allows you to maintain the order in which data is added.
- Maps are better than associative arrays because they are more flexible and can maintain order.
Associative arrays are useful for organizing data because they allow you to access data by name.
On the other hand, Maps are more flexible than associative arrays because you can use different data types as keys. Also, using Map allows you to maintain the order in which data was added, which is useful when you want to preserve the order.
Which one you should use depends on the situation and purpose, but it is recommended that new code use Map whenever possible.
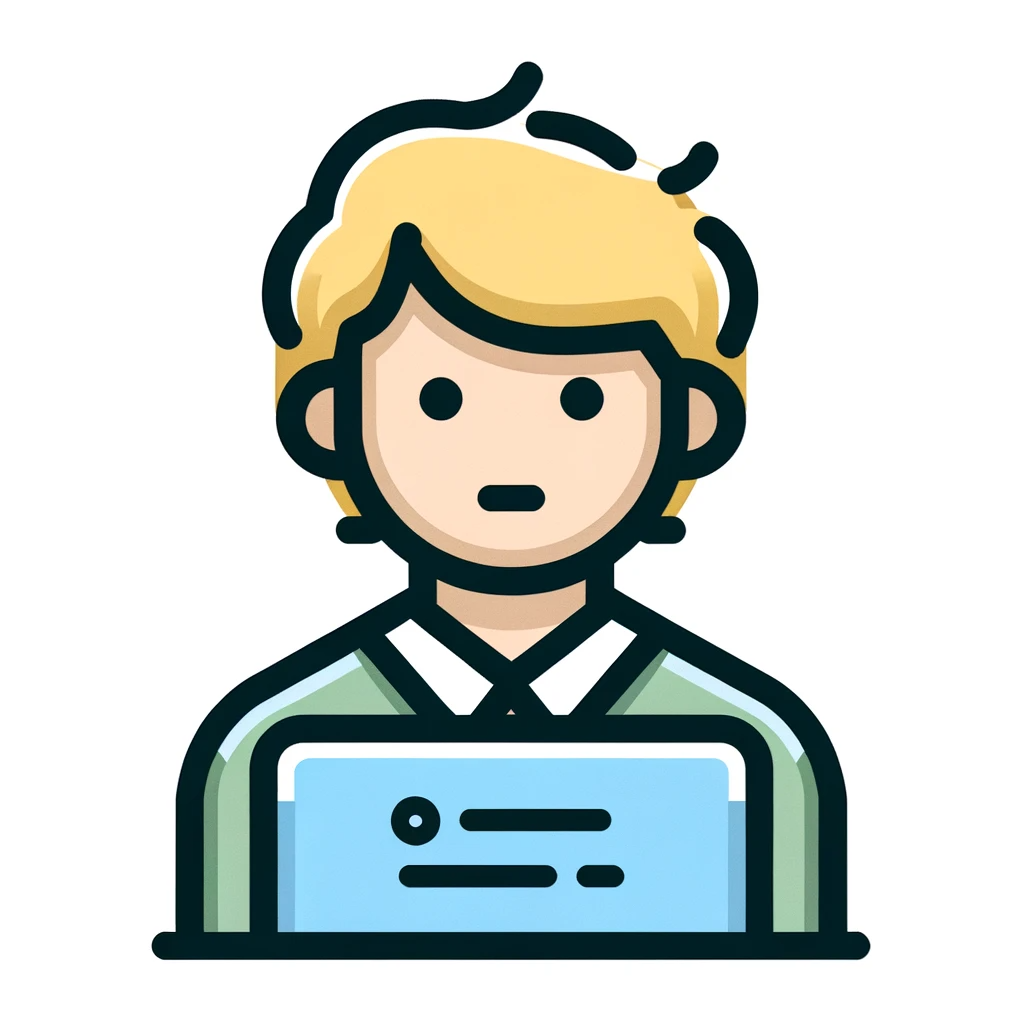
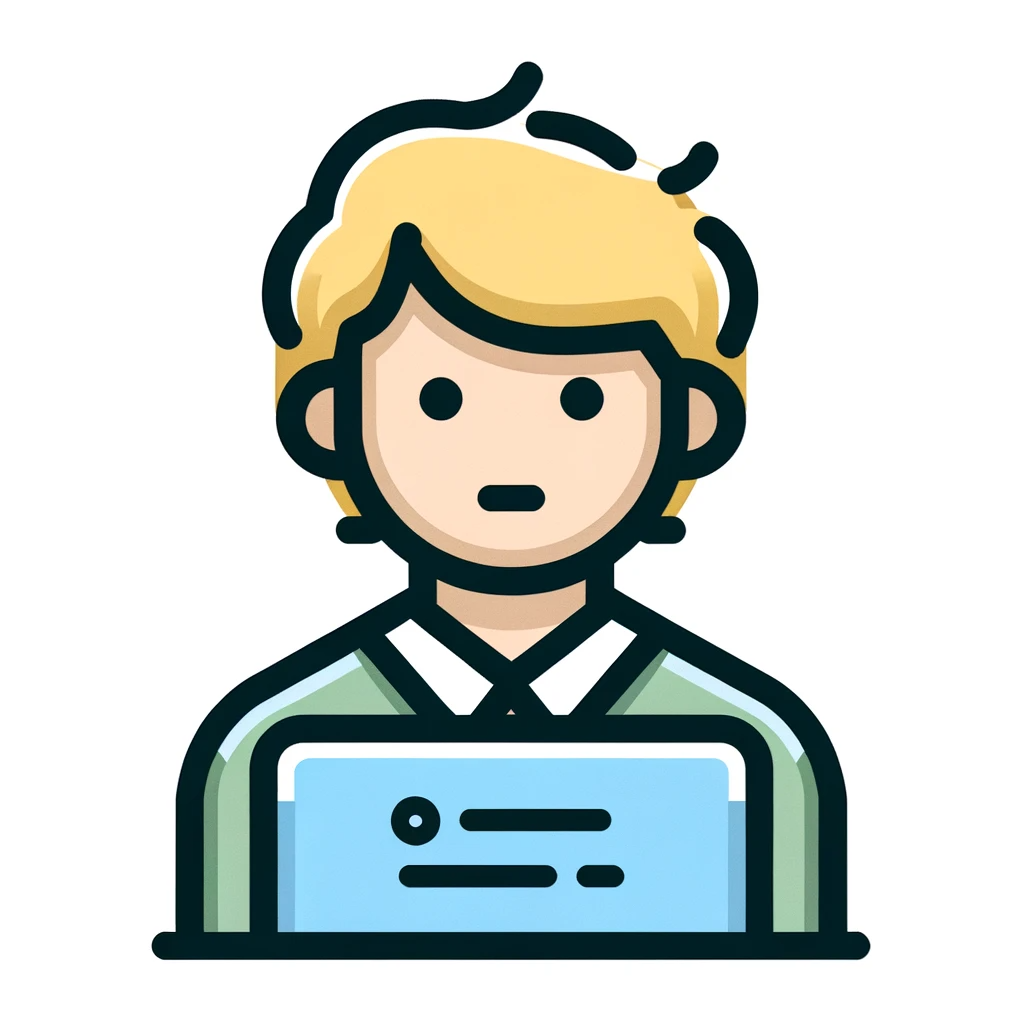
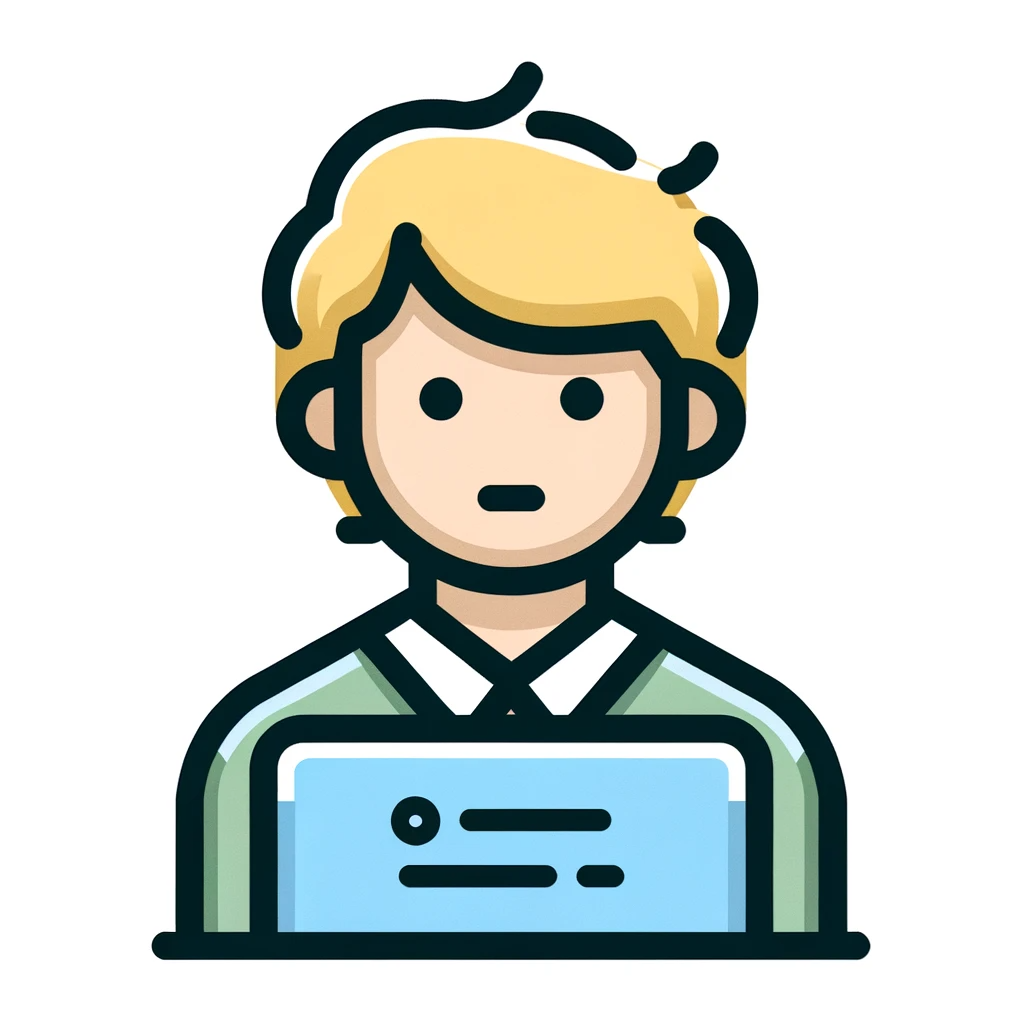
It was very helpful as it explained the differences between associative arrays and Maps and the basic usage in an easy-to-understand way!
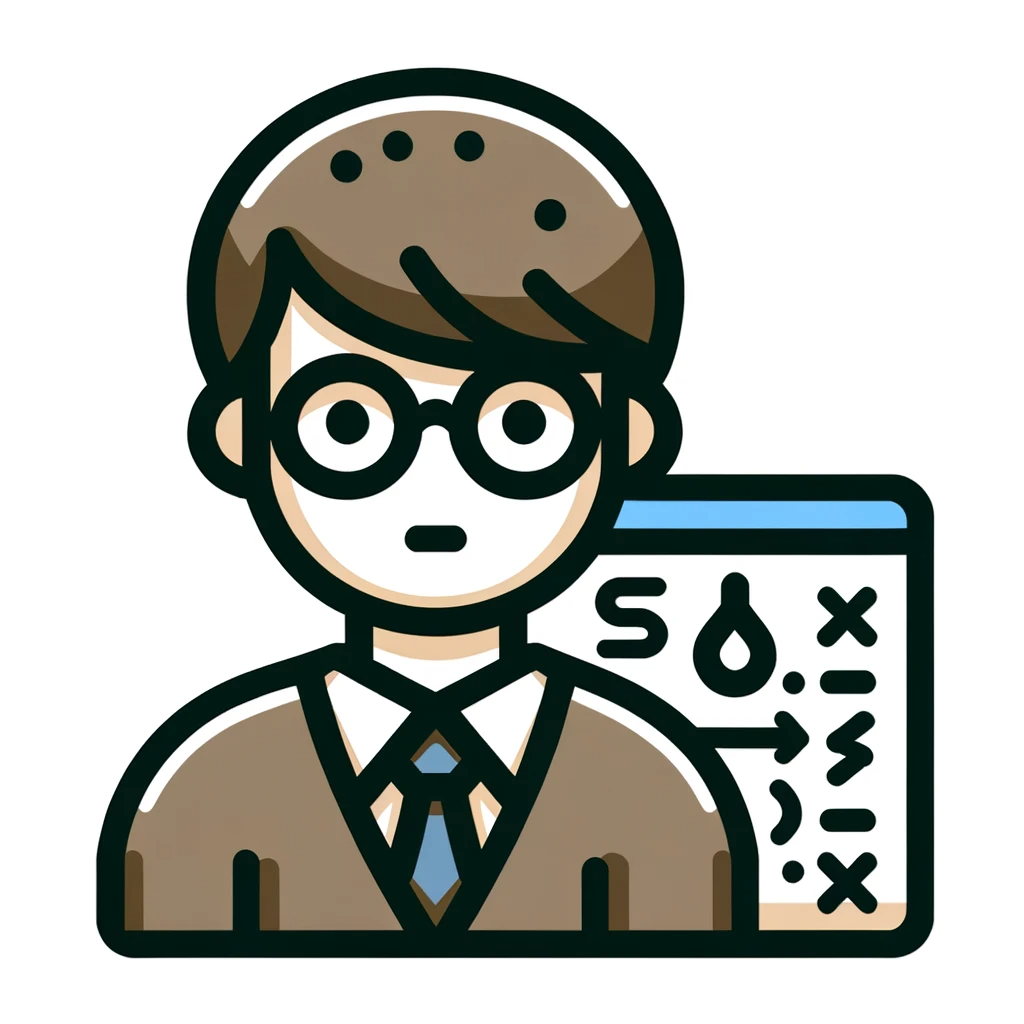
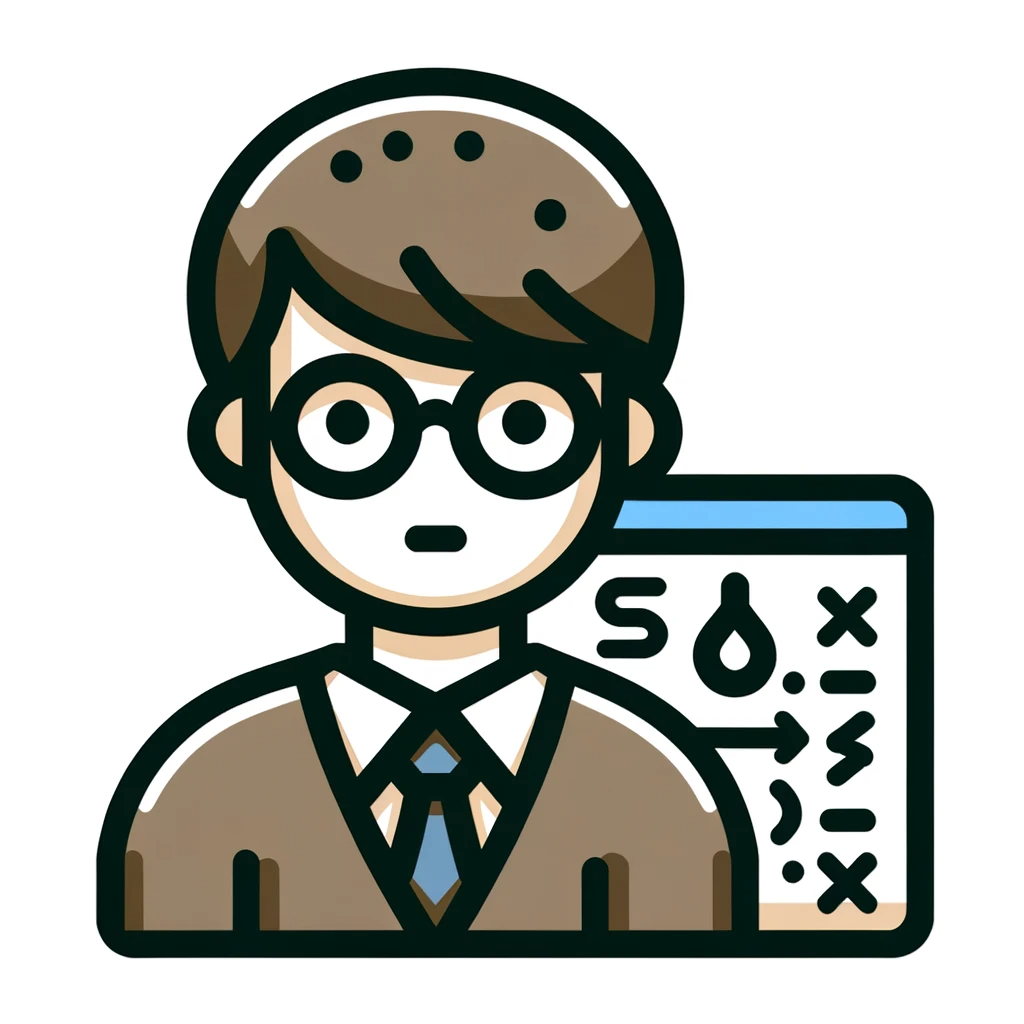
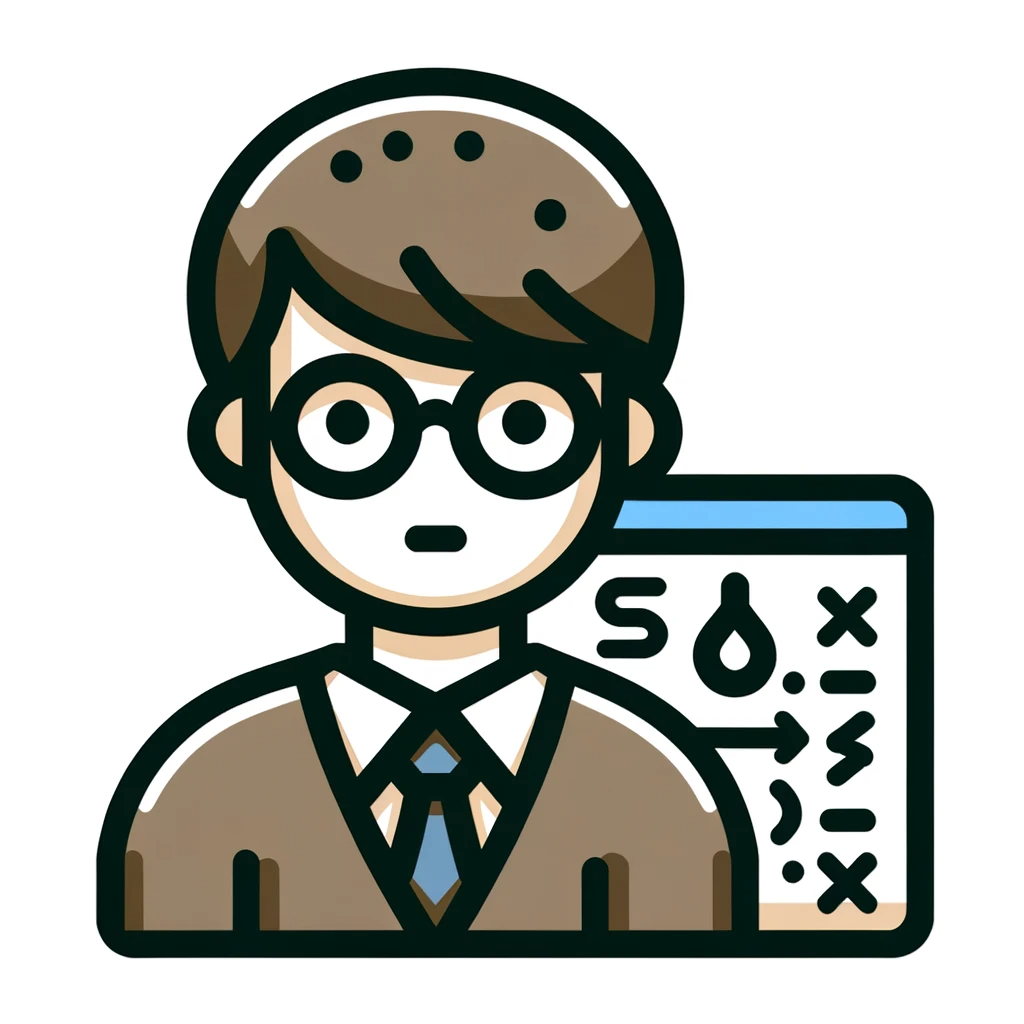
You can use both associative arrays and Map objects, but the decision between which to use depends on your project and task.
It is common to use an associative array if you need to treat strings as keys, and a Map object otherwise.
Also, since ES6, the Map object has more functionality and is often used commonly.
However, older browsers may not support Map objects, so if you need to accommodate them, you might consider using an associative array.
Comments