This article explains how to use JavaScript’s hasAttribute method to determine whether a specified attribute exists in an HTML element.
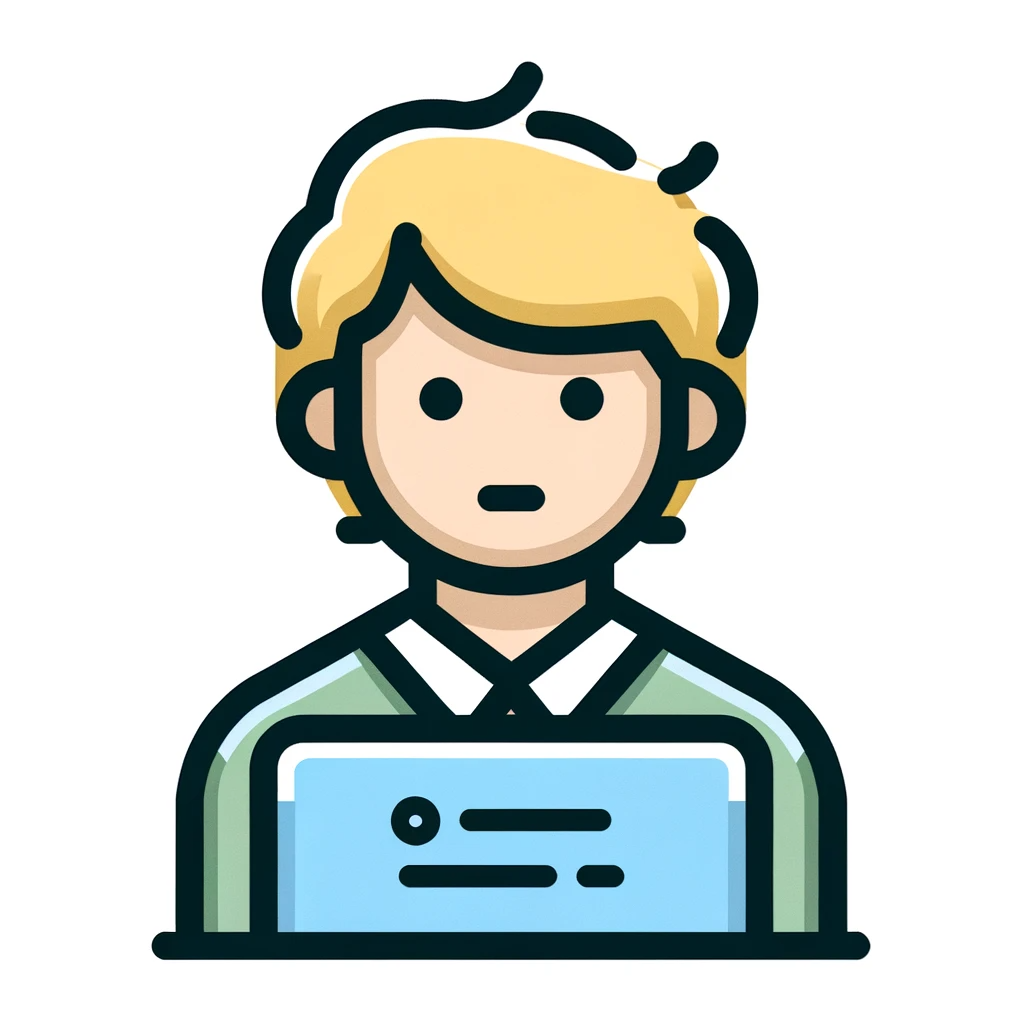
How can I determine if an attribute of an HTML element exists?
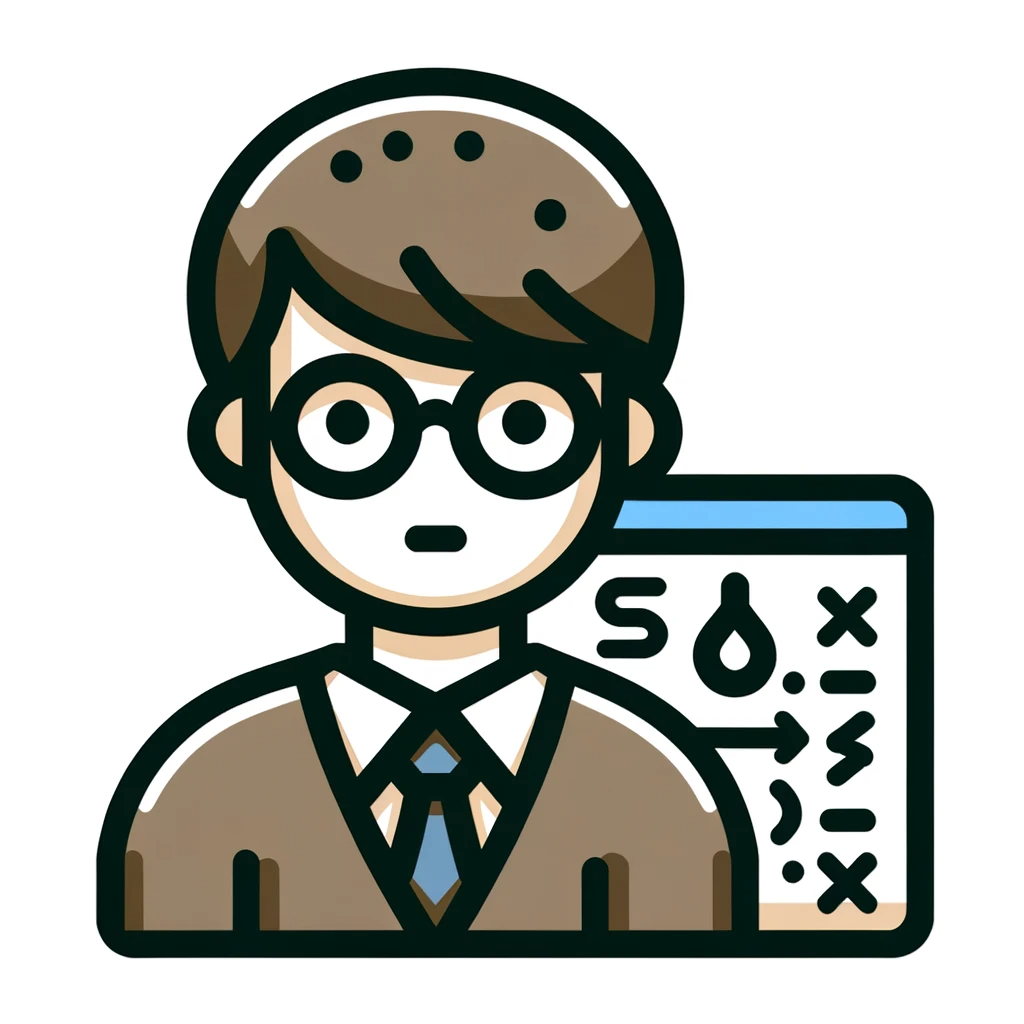
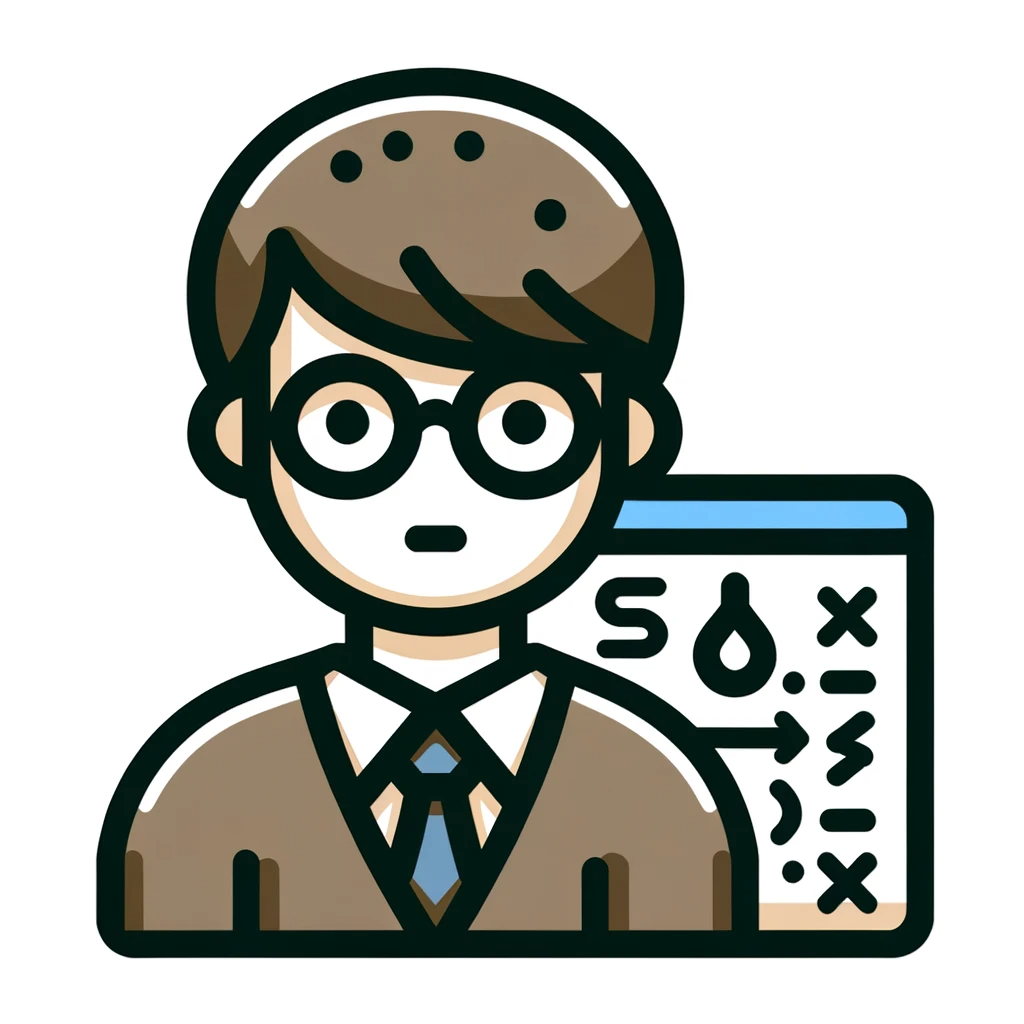
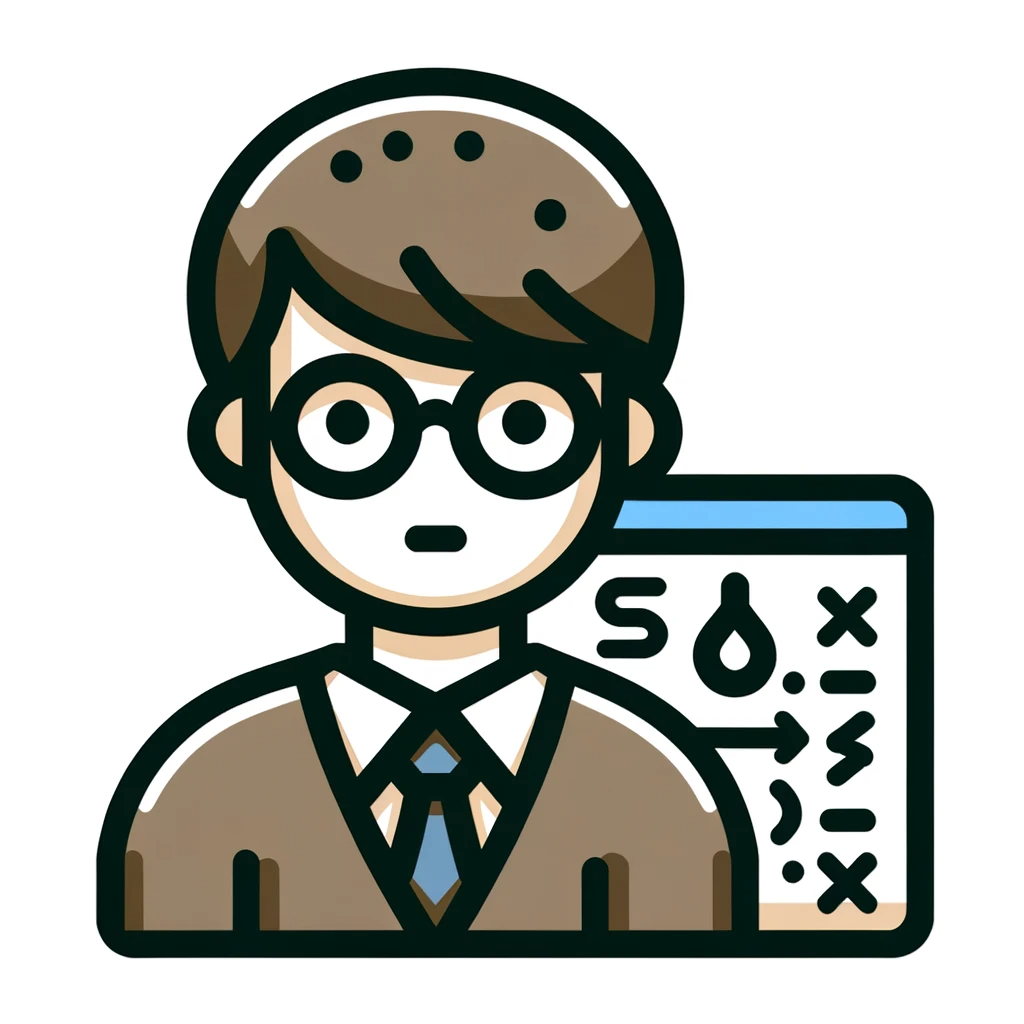
You can easily determine this using the hasAttribute method.
What is hasAttribute method?
The hasAttribute method is used to determine whether an HTML element has a specified attribute . Returns true if the specified attribute exists, false otherwise.
To use the hasAttribute method, write as follows.
element.hasAttribute(“attribute name”);
The following sample program is a script that determines whether the class attribute exists in an HTML element.
<!DOCTYPE html>
<html>
<head>
<script>
function checkAttribute() {
var element = document.getElementById("myDiv");
if (element.hasAttribute("class")) {
alert("The element has a class attribute.");
} else {
alert("The element does not have a class attribute.");
}
}
</script>
</head>
<body>
<div id="myDiv" class="example">This is a div element</div>
<button onclick="checkAttribute()">Check Attribute</button>
</body>
</html>
This example calls the hasAttribute method on the element with ID “myDiv” to determine whether the “class” attribute exists.
If the “class” attribute is present, an alert will display “The element has a class attribute”. If it doesn’t exist, “The element does not have a class attribute” is displayed.
Summary of this article
We explained how to use the hasAttribute method to determine whether a specified attribute exists in an HTML element.
- hasAttribute method is a method to determine whether a specified attribute of an HTML element exists.
- It can be used by writing element.hasAttribute(“attribute name” ).
- Returns true if the specified attribute exists, false otherwise.
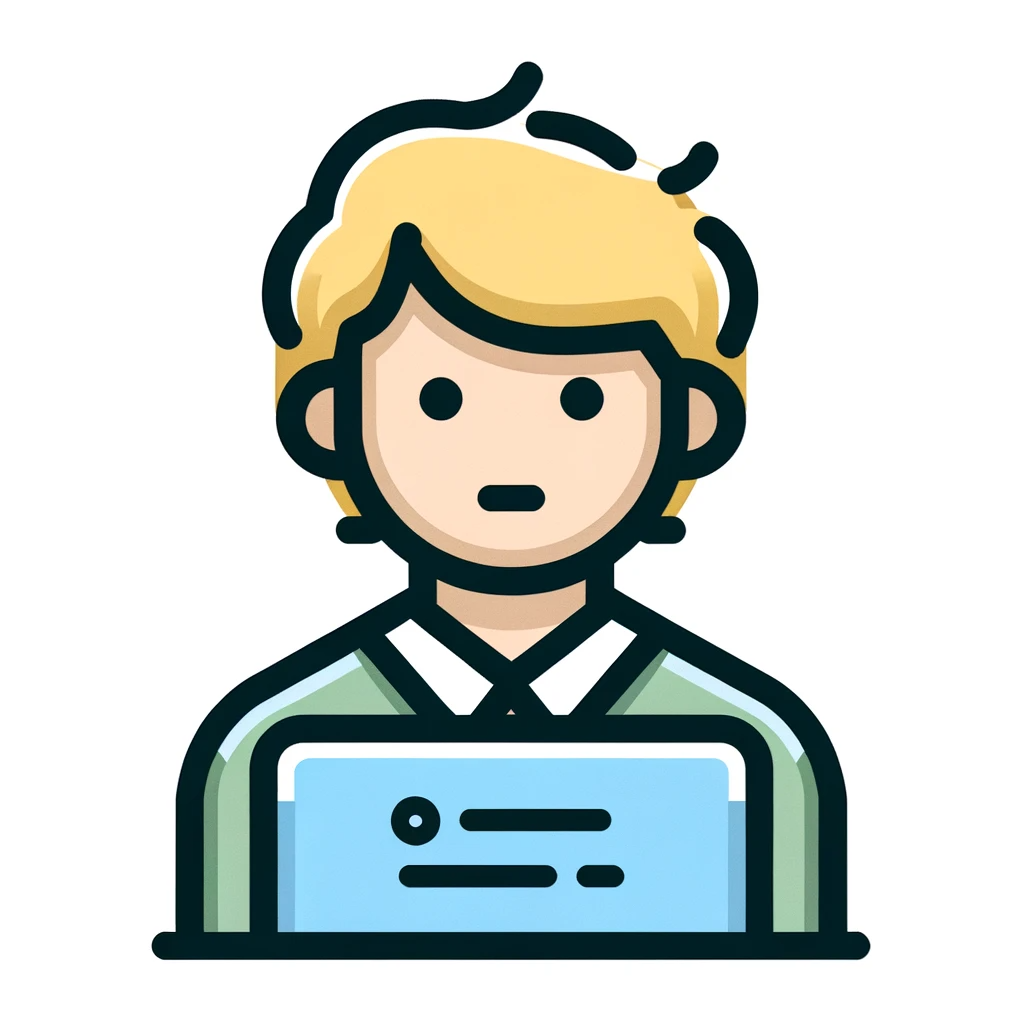
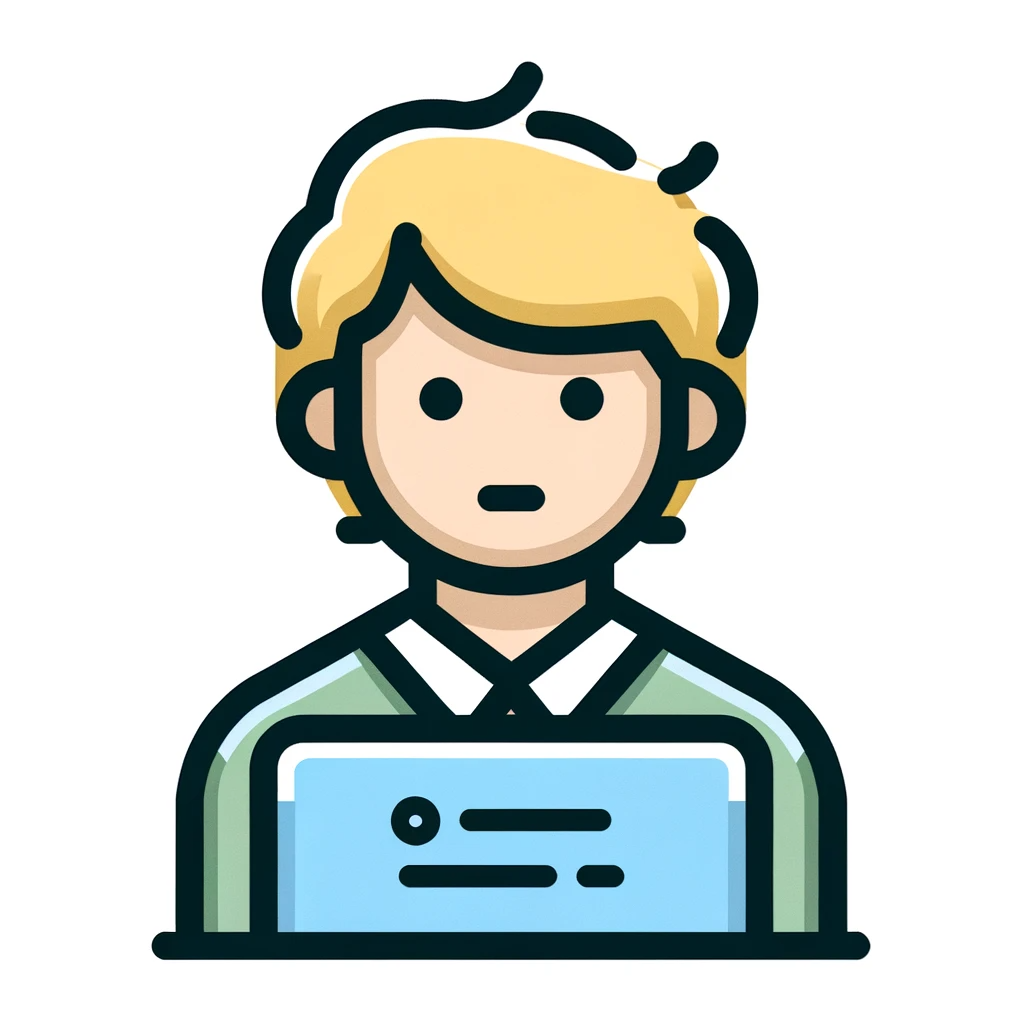
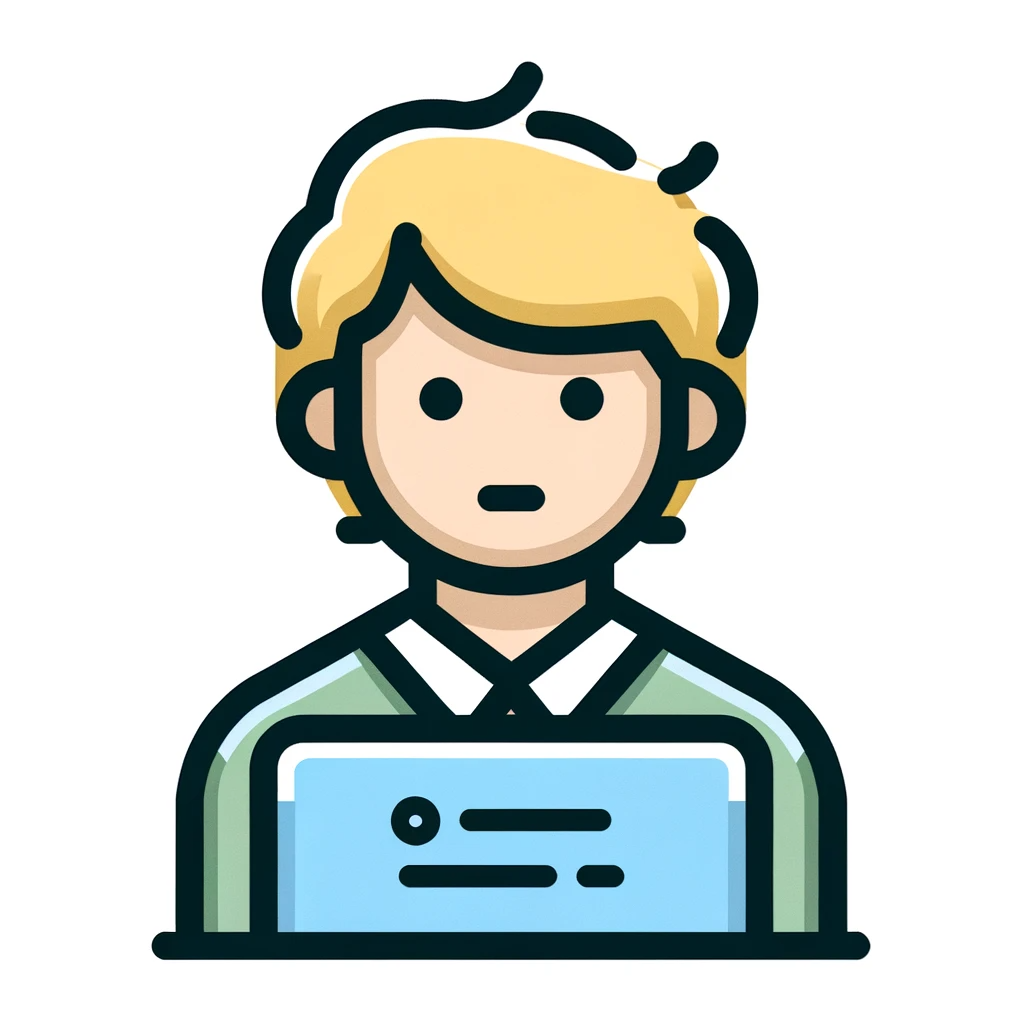
The hasAttribute method sounds useful!
I would like to write a script that uses this to determine the attributes of an element.
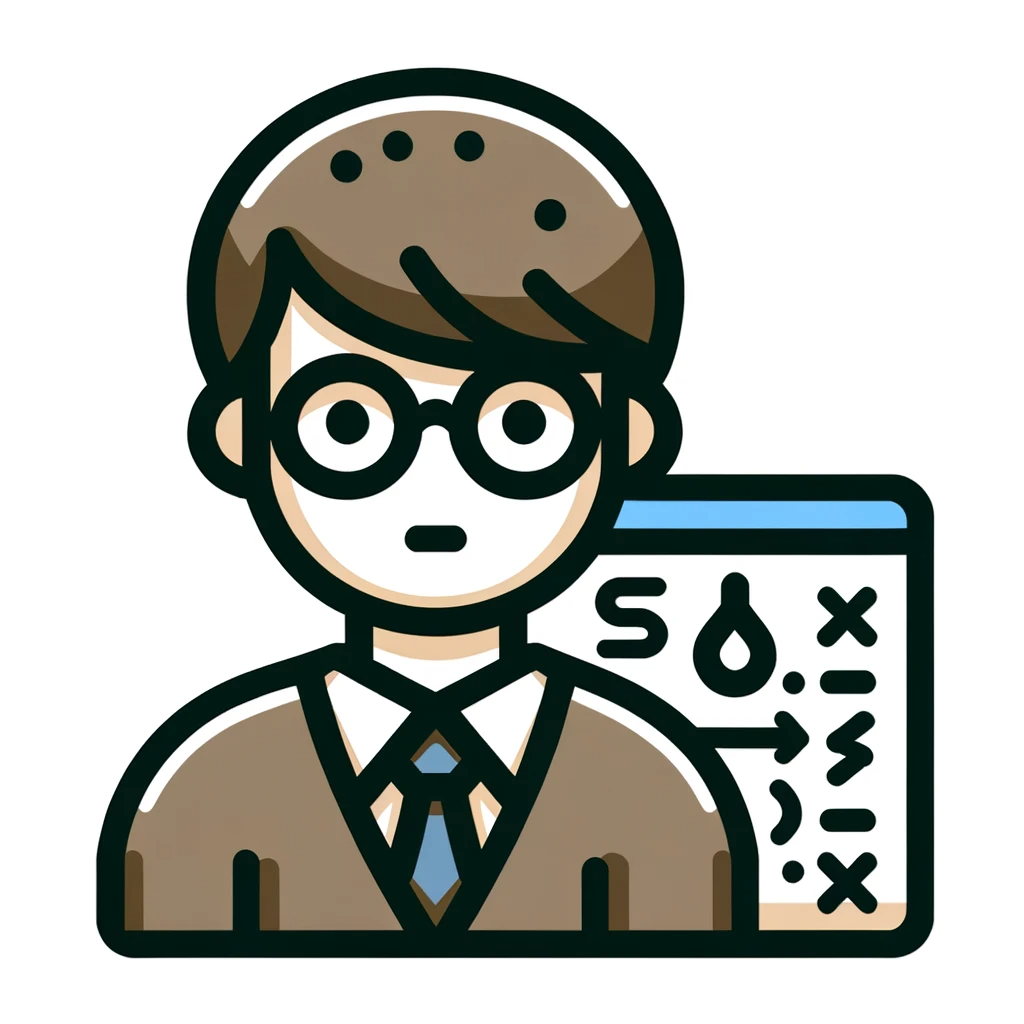
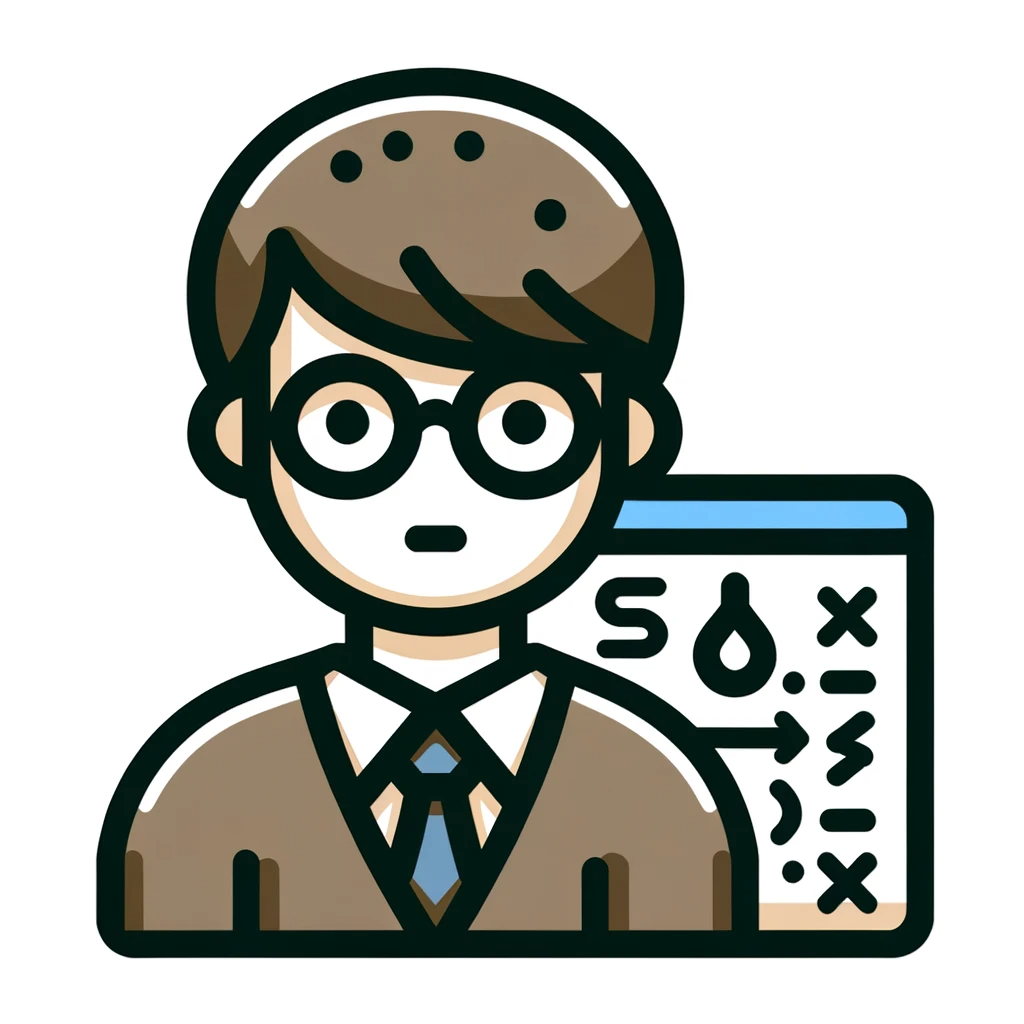
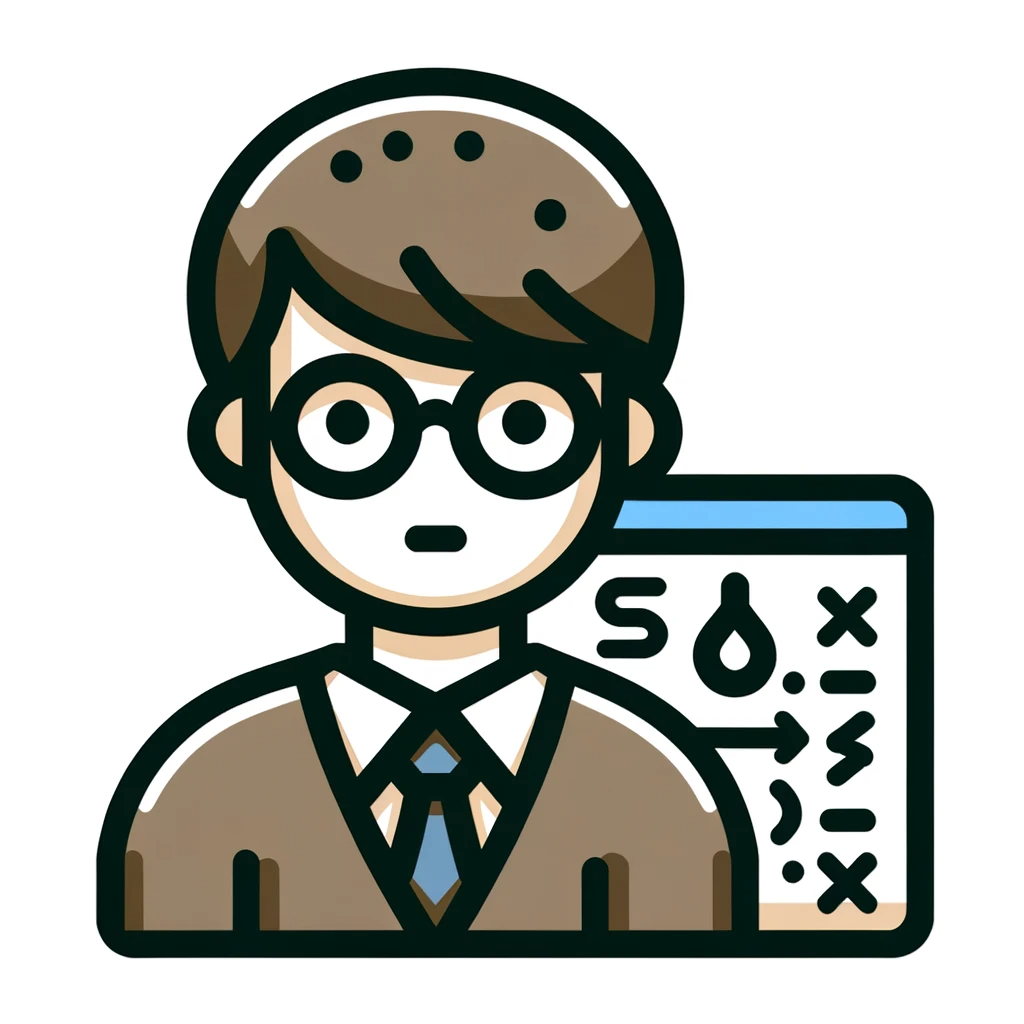
The hasAttribute method is a very useful method for manipulating HTML elements with JavaScript.
Please try using it when determining whether an attribute of an element exists.
Comments