This article explains how to use the getElementsByName method to retrieve elements using the name attribute as a key in JavaScript.
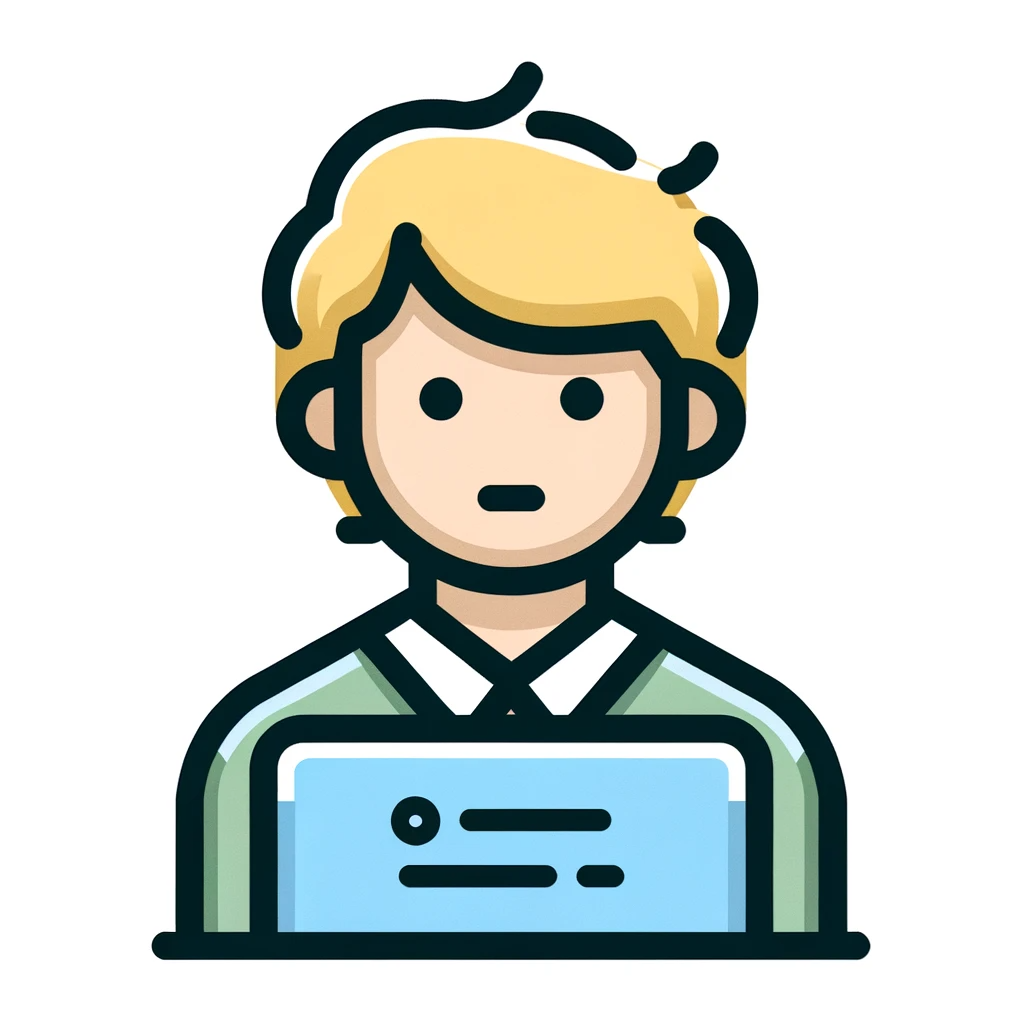
Please tell me how to retrieve an element using the name attribute as a key!
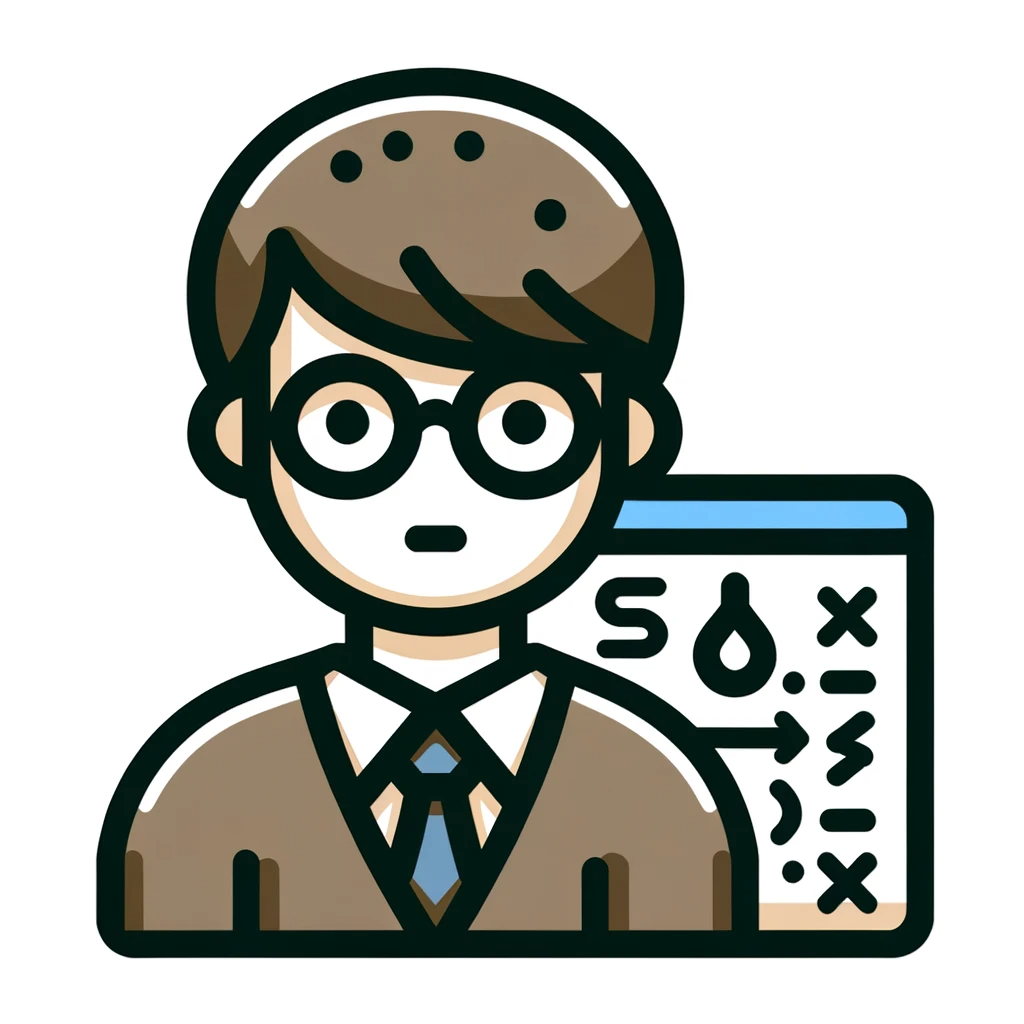
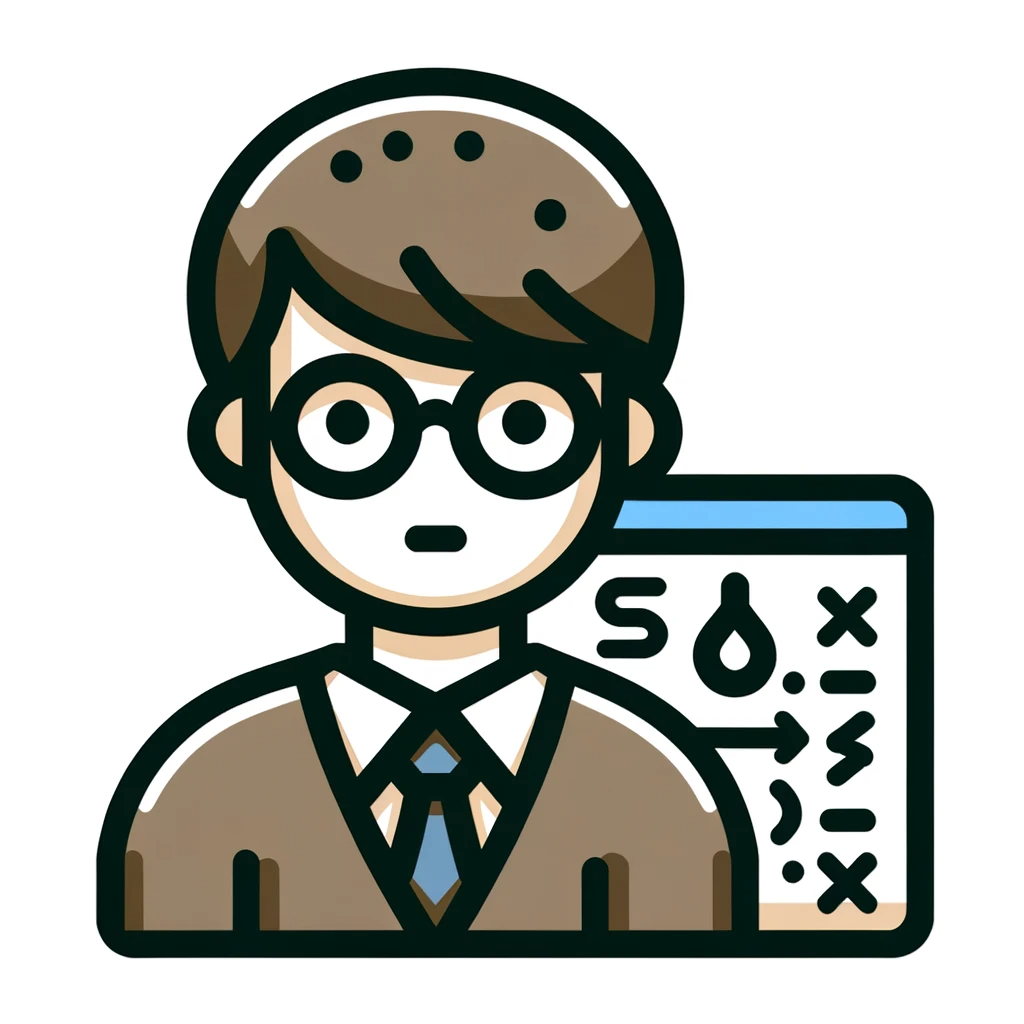
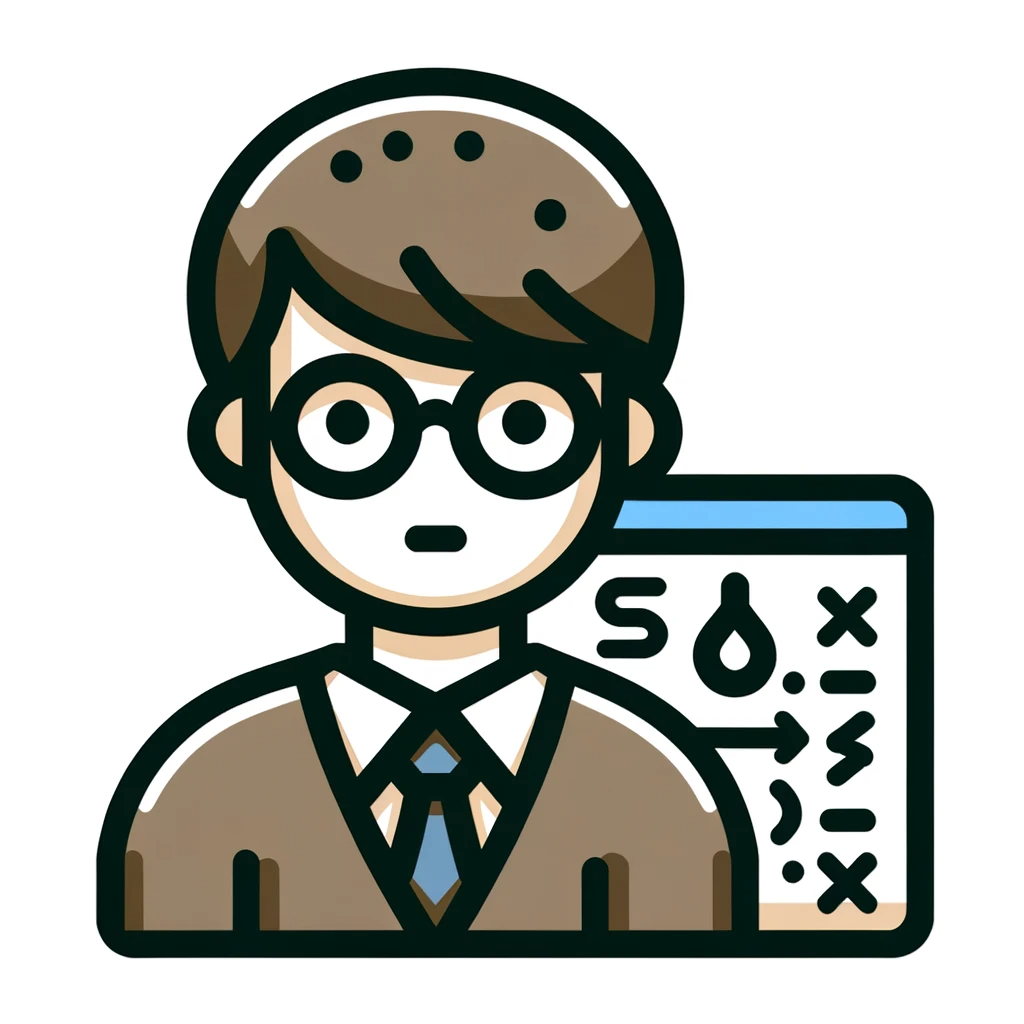
By using the getElementsByName method, you can retrieve elements using the name attribute as a key.
What is getElementsByName method?
The getElementsByName method can retrieve elements using the HTML name attribute value as a key. This method can retrieve multiple elements, so you can operate on multiple elements at once.
How to use getElementsByName method
The getElementsByName method can be called from the document object.
Pass the name attribute value of the element you want to retrieve as an argument to the getElementsByName method. Also, the retrieved elements are array-like objects, and the array index is used to access each element.
By writing something like the following, you can retrieve the element whose HTML name attribute value is “sample”.
var elements = document.getElementsByName("sample");
The retrieved elements are array-like objects. Use the array index to access each element. You can access the first retrieved element by writing something like the following:
elements[0].value = "Hello World";
In this way, by using the getElementsByName method, you can retrieve elements using the HTML name attribute as a key. You can dynamically change HTML elements by manipulating the retrieved elements.
Explanation using a sample program
The sample program below shows an example of getting an input element with the name attribute value “sample” and changing the value to “Hello World”.
<input type="text" name="sample" value="">
<input type="text" name="sample" value="">
<input type="text" name="sample" value="">
<script>
var elements = document.getElementsByName("sample");
for (var i = 0; i < elements.length; i++) {
elements[i].value = "Hello World";
}
</script>
In this sample, there are three text boxes in the HTML.
In JavaScript, use the getElementsByName method to get the text box whose name attribute is “sample”. We use a for statement to access each retrieved text box and set the value to “Hello World.”
In this way, you can use the getElementsByName method to retrieve elements using the HTML name attribute as a key. You can dynamically change HTML elements by manipulating the retrieved elements.
Summary of this article
We explained how to use the getElementsByName method to retrieve elements using the name attribute as a key.
- getElementsByName method can retrieve multiple elements.
- Get the HTML elements by using the getElementsByName method of the document object.
- Pass the name attribute value of the element to be retrieved as an argument.
- The retrieved elements are array-like objects, and the array index is used to access each element.
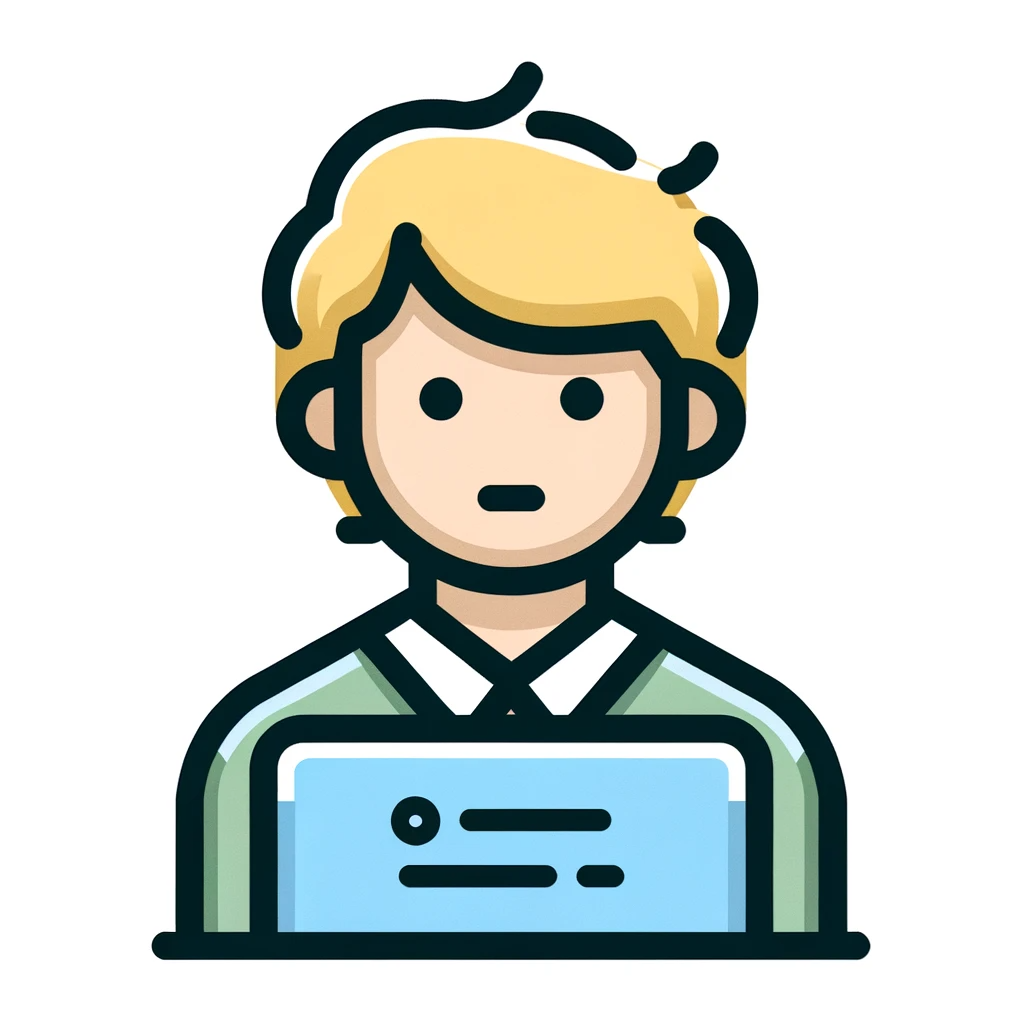
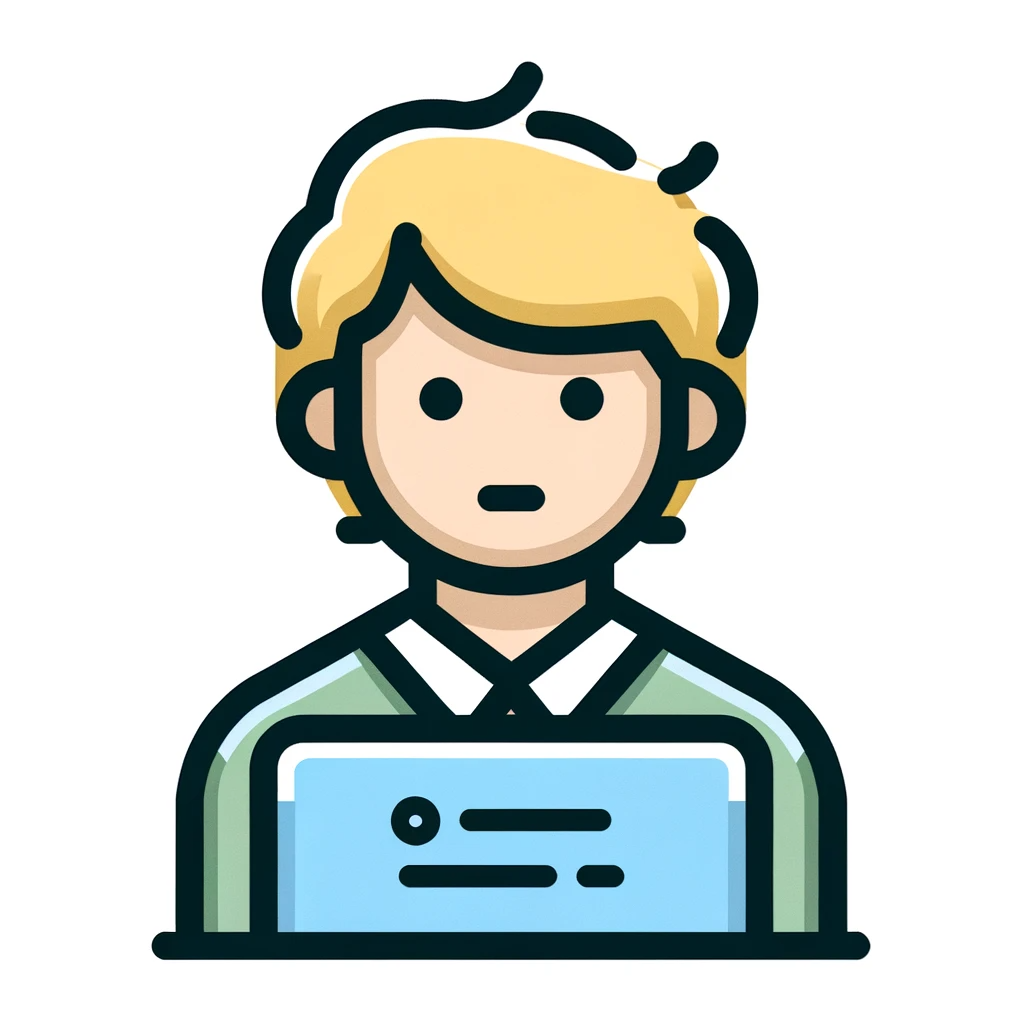
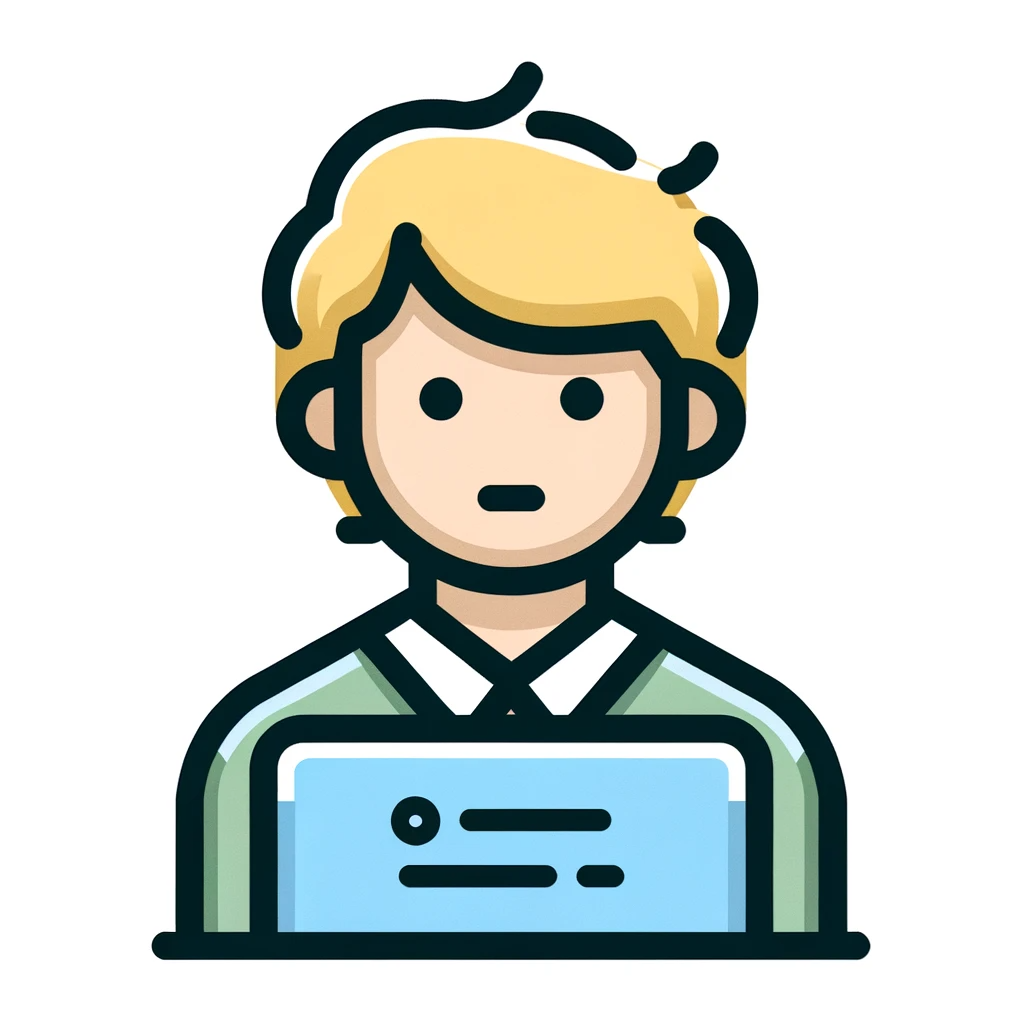
I was able to retrieve elements from the name attribute using the getElementsByName method!
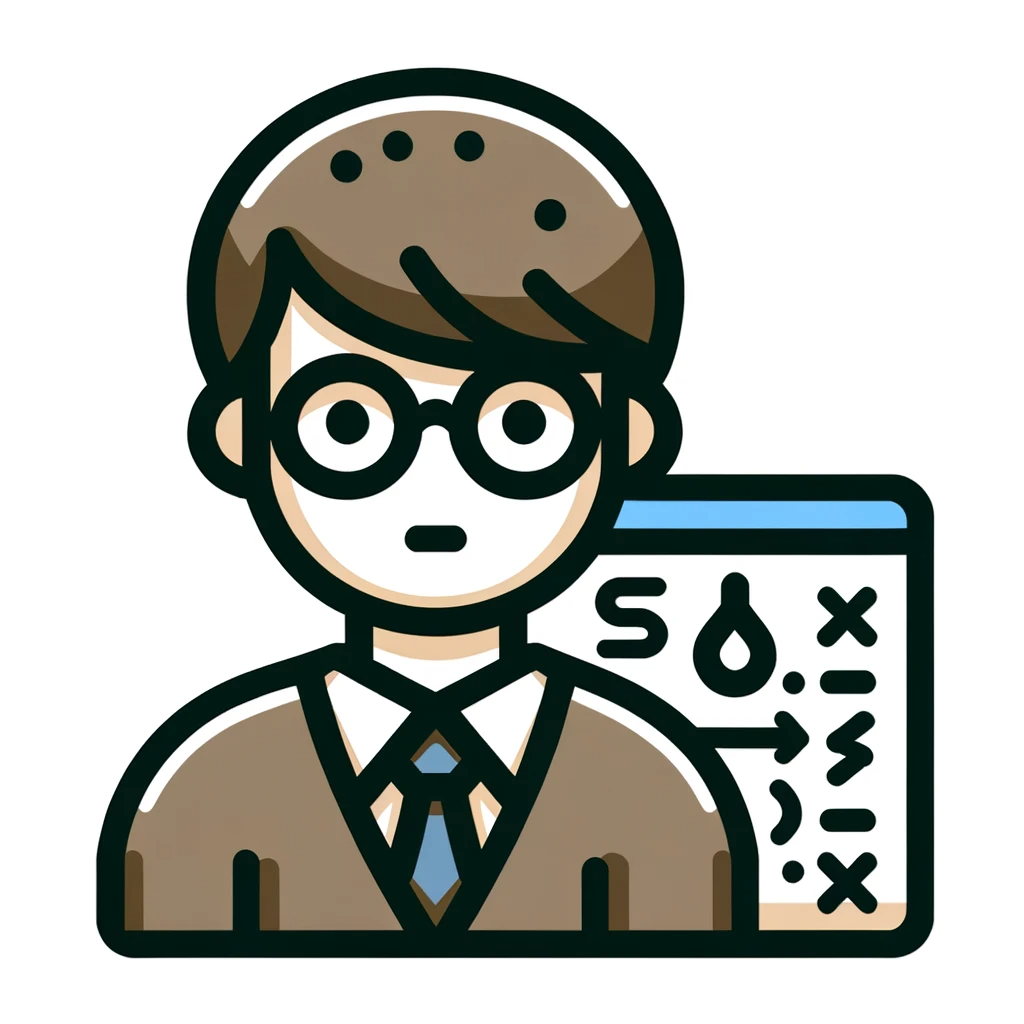
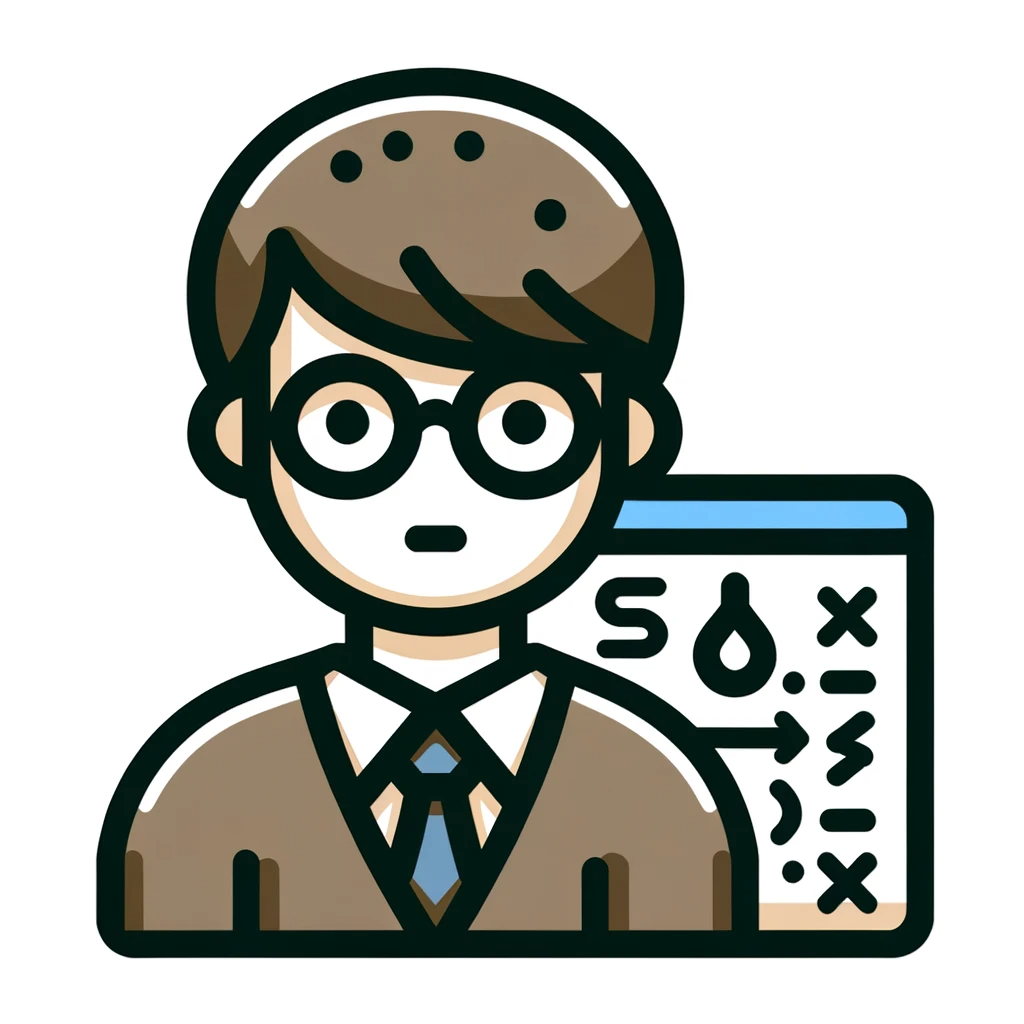
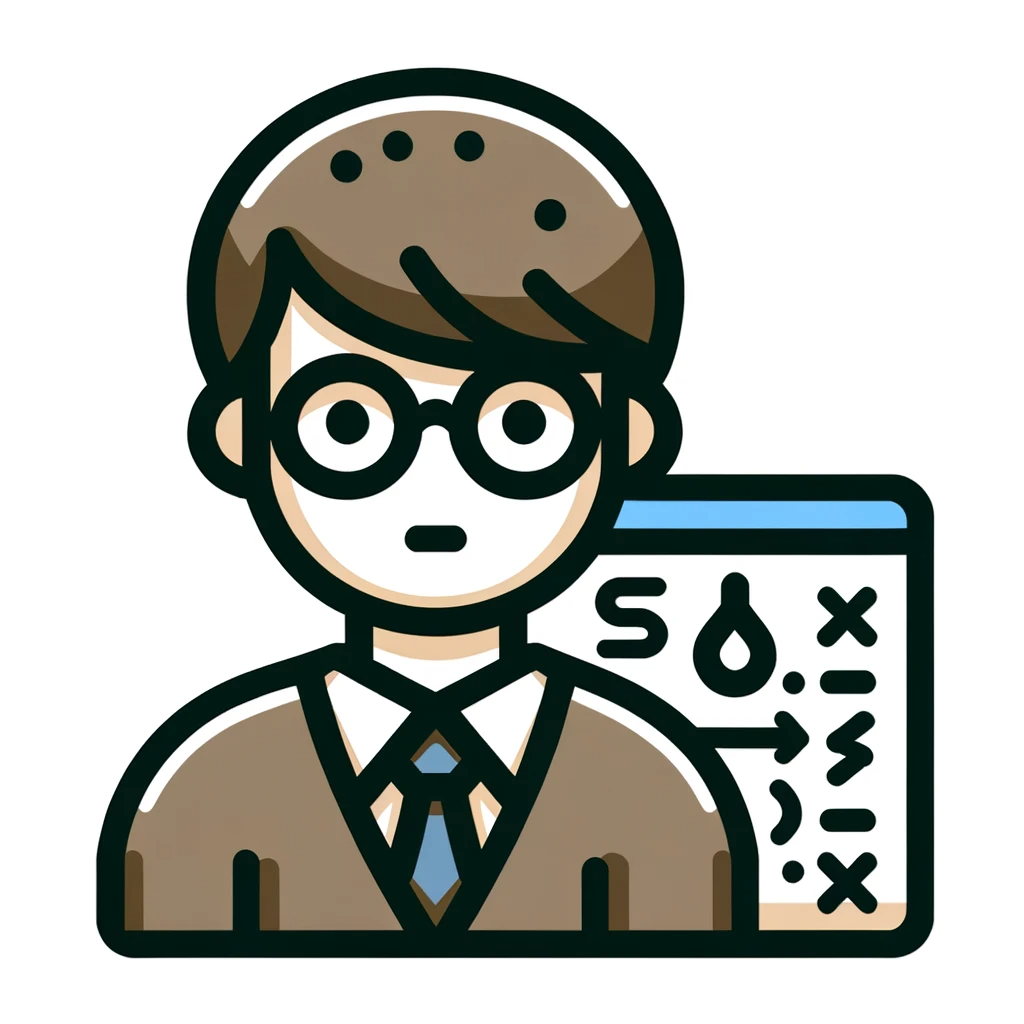
There are other ways to obtain HTML elements, so you can improve your skills by learning various methods.
Comments