This article explains how to use JavaScript’s getElementsByClassName method to retrieve HTML elements using class attributes as keys . We will also explain the querySelectorAll method, which allows similar acquisition.
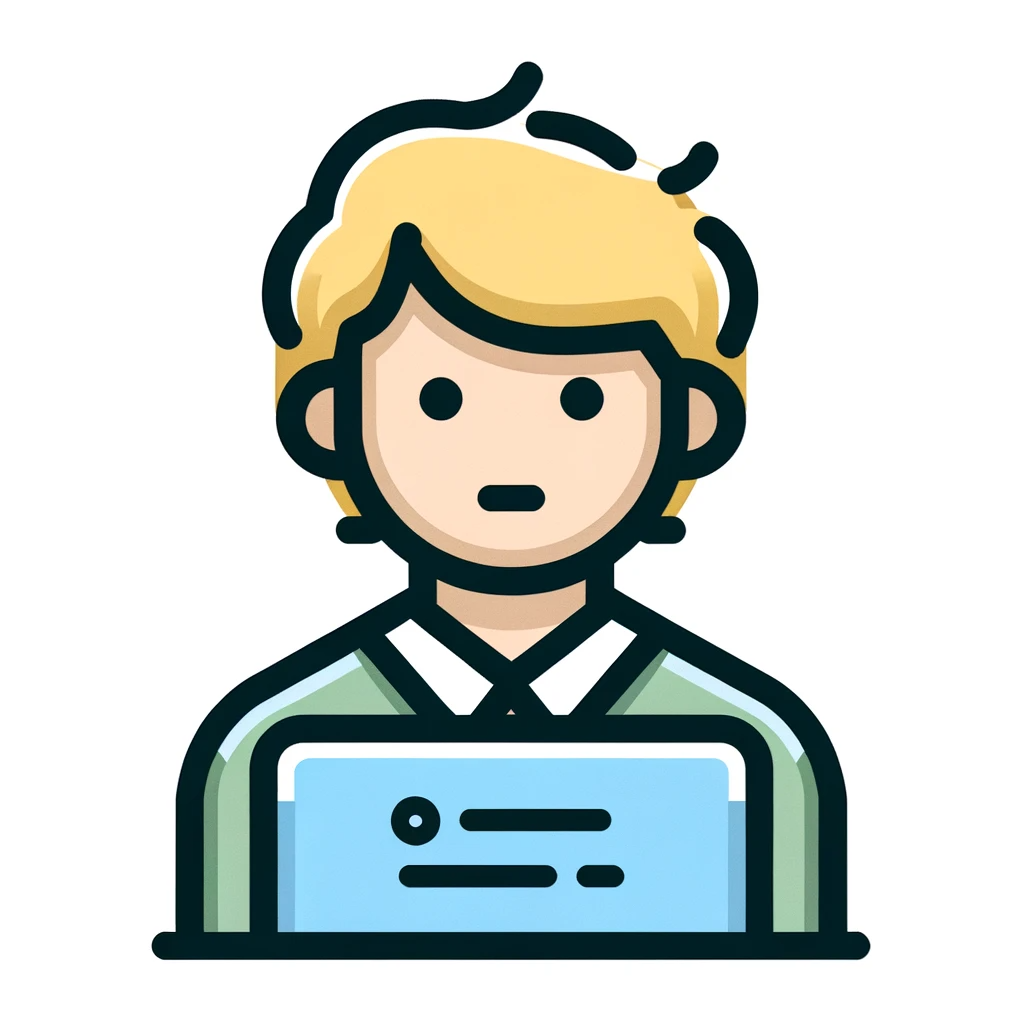
I would like to retrieve HTML elements using class attributes as keys. Is there a good way?
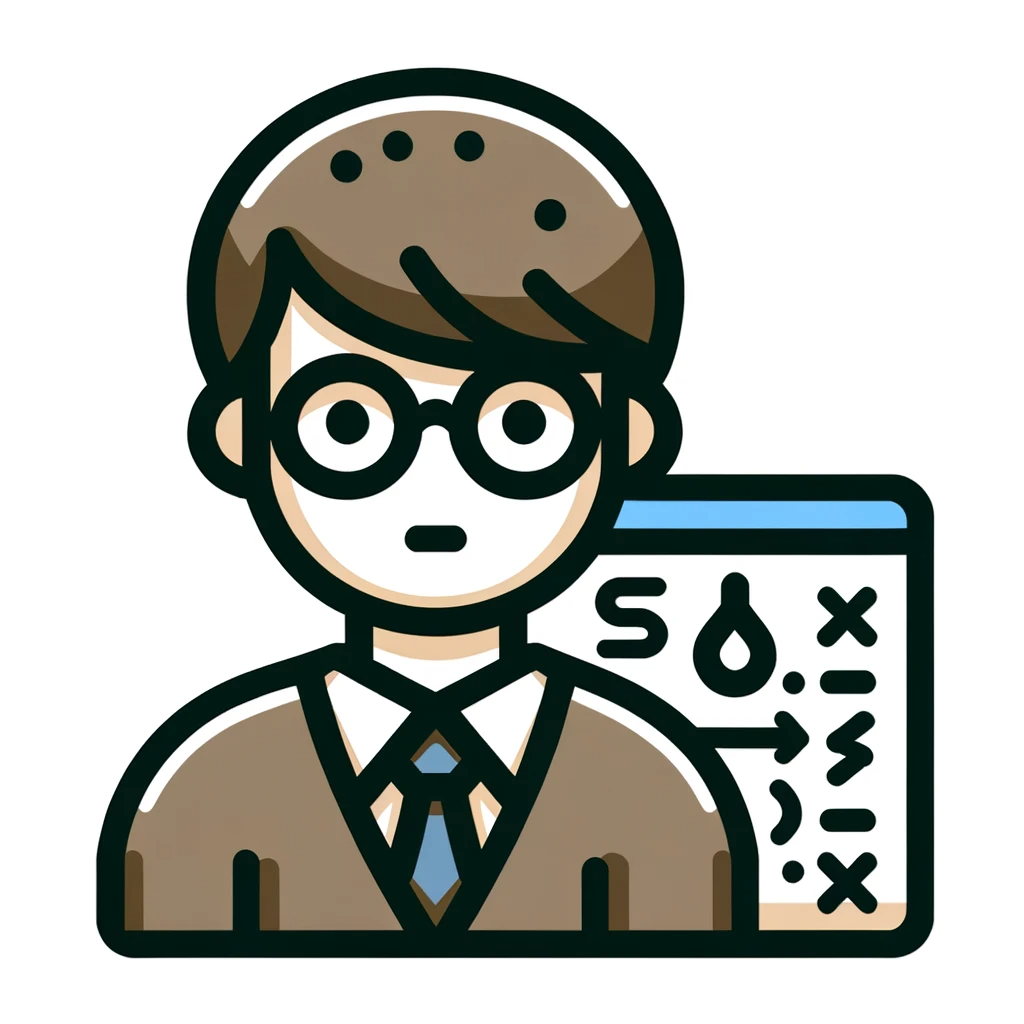
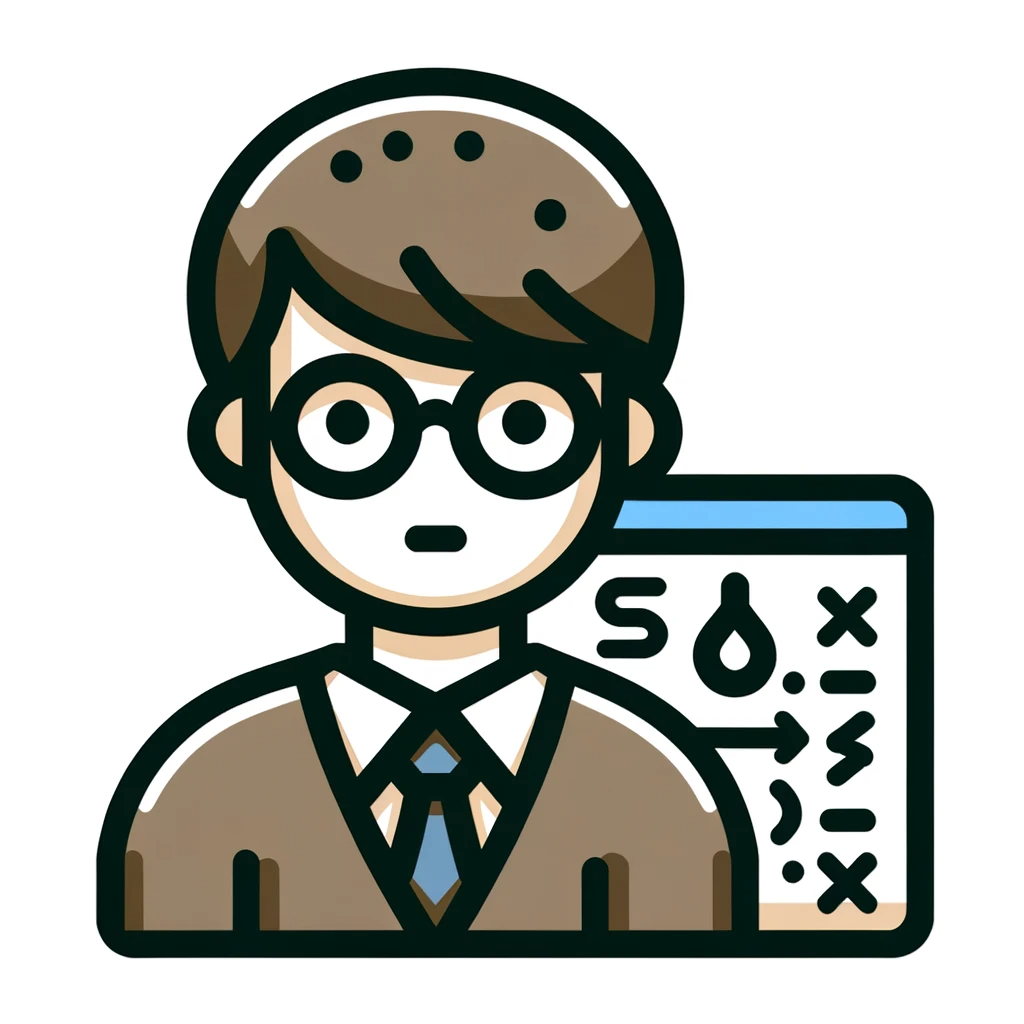
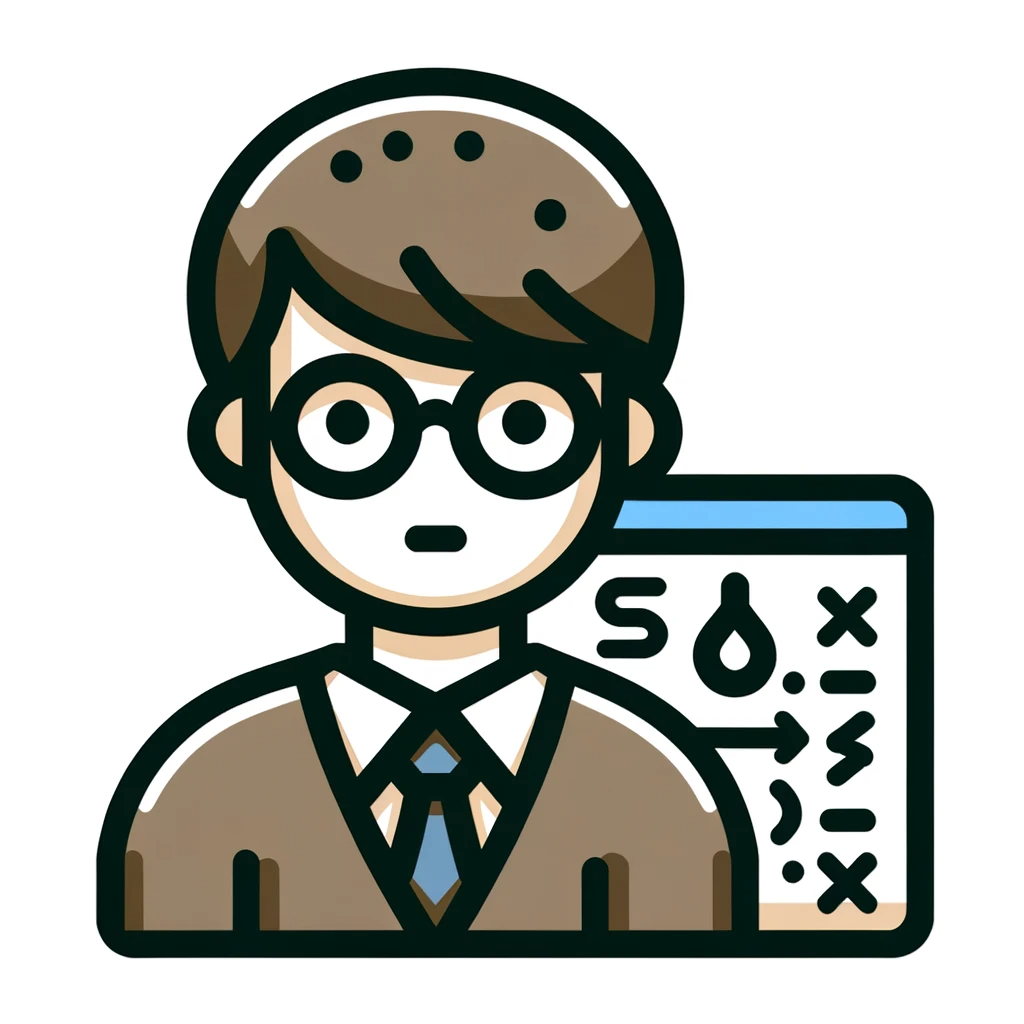
There are two ways to get class attributes as keys: using the “getElementsByClassName method” and using “querySelectorAll”.
Overview and usage of getElementsByClassName method
The getElementsByClassName method returns an HTMLCollection object containing all elements with the specified class name in the HTML document .
By using this method, you can perform operations on elements with the specified class name all at once . “HTMLCollection” is like an array and you can access individual elements.
document.getElementsByClassName(class name);
The sample program below retrieves all elements with the class name “test-class” in an HTML file and outputs the text content of each to the console.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Get Elements By Class Name</title>
</head>
<body>
<div class="test-class">element1</div>
<div class="test-class">element2</div>
<div class="test-class">element3</div>
<script src="test.js"></script>
</body>
</html>
var elements = document.getElementsByClassName("test-class");
for (var i = 0; i < elements.length; i++) {
console.log(elements[i].textContent);
}
Overview and usage of querySelectorAll method
The querySelectorAll method is a method that allows you to select elements in an HTML document using CSS selectors .
document.querySelectorAll(CSS selector);
This method returns a “NodeList” object, which is like an array and allows you to access individual elements.
The sample program below uses the CSS selector “.test-class” to retrieve all elements with the class name “test-class” in an HTML document and outputs the text content of each to the console.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Get Elements By Class Name</title>
</head>
<body>
<div class="test-class">要素1</div>
<div class="test-class">要素2</div>
<div class="test-class">要素3</div>
<script src="test.js"></script>
</body>
</html>
var elements = document.querySelectorAll(".test-class");
for (var i = 0; i < elements.length; i++) {
console.log(elements[i].textContent);
}
Difference between querySelectorAll method and getElementsByClassName method
The querySelectorAll and getElementsByClassName methods are JavaScript methods for selecting elements in an HTML document, but there are differences.
- Differences in method:
- The getElementsByClassName method selects elements using the class name as a key, and the querySelectorAll method selects elements using CSS selectors. For this reason, the querySelectorAll method is more flexible and allows complex selections.
- Differences in returned objects:
- The getElementsByClassName method returns an “HTMLCollection” object, while the querySelectorAll method returns a “NodeList” object. “HTMLCollection” is an old specification, and “NodeList” is said to be faster.
- Differences in updated objects:
- The “HTMLCollection” obtained with the getElementsByClassName method is updated dynamically, but the “NodeList” obtained with the querySelectorAll method is not updated. For this reason, the querySelectorAll method is faster and allows you to reuse elements once retrieved.
In this way, we can say that querySelectorAll method is better than getElementsByClassName method.
Summary of this article
I will explain the etElementsByClassName method and querySelectorAll method.
- Using the getElementsByClassName method, you can retrieve elements using the class attribute as a key.
- The getElementsByClassName method is for retrieving multiple elements with a class attribute, returned as an HTMLCollection object.
- The querySelectorAll method allows you to select elements in an HTML document using CSS selectors and returns a NodeList object.
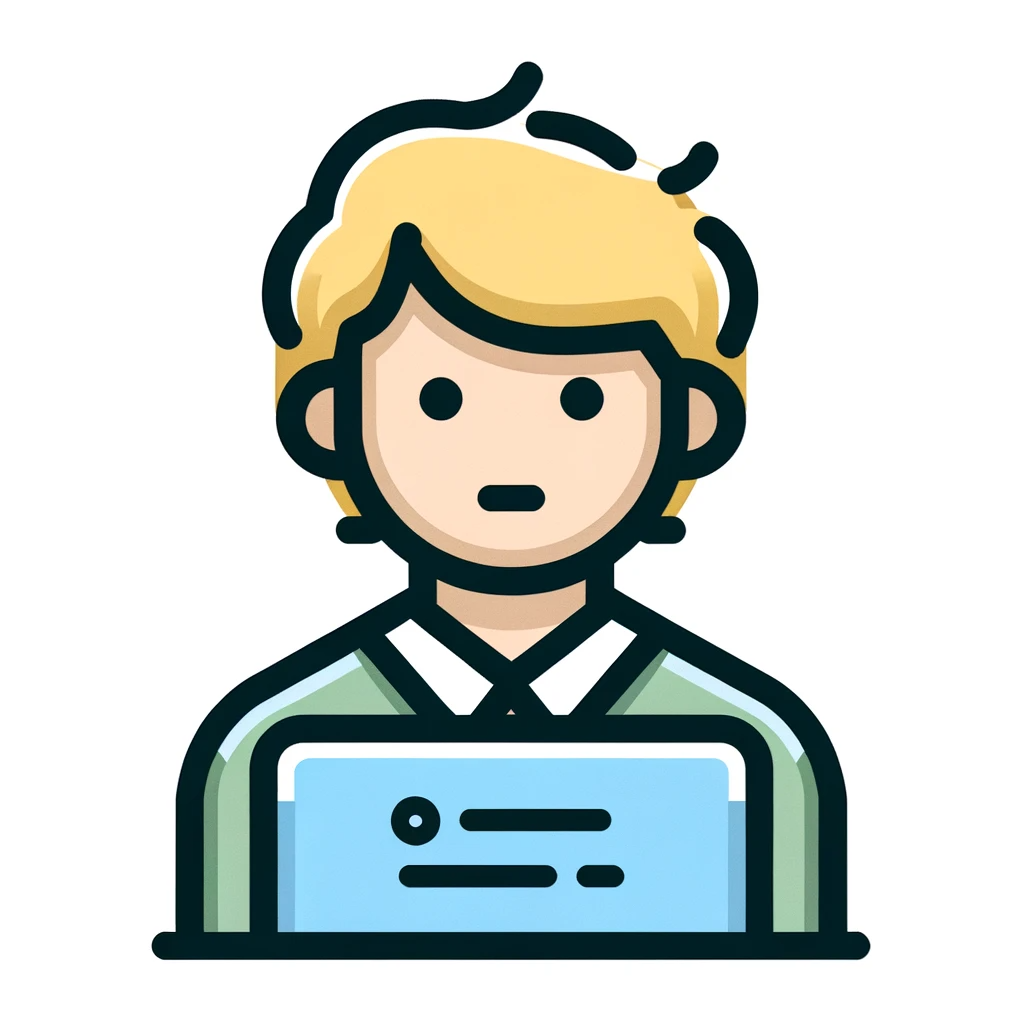
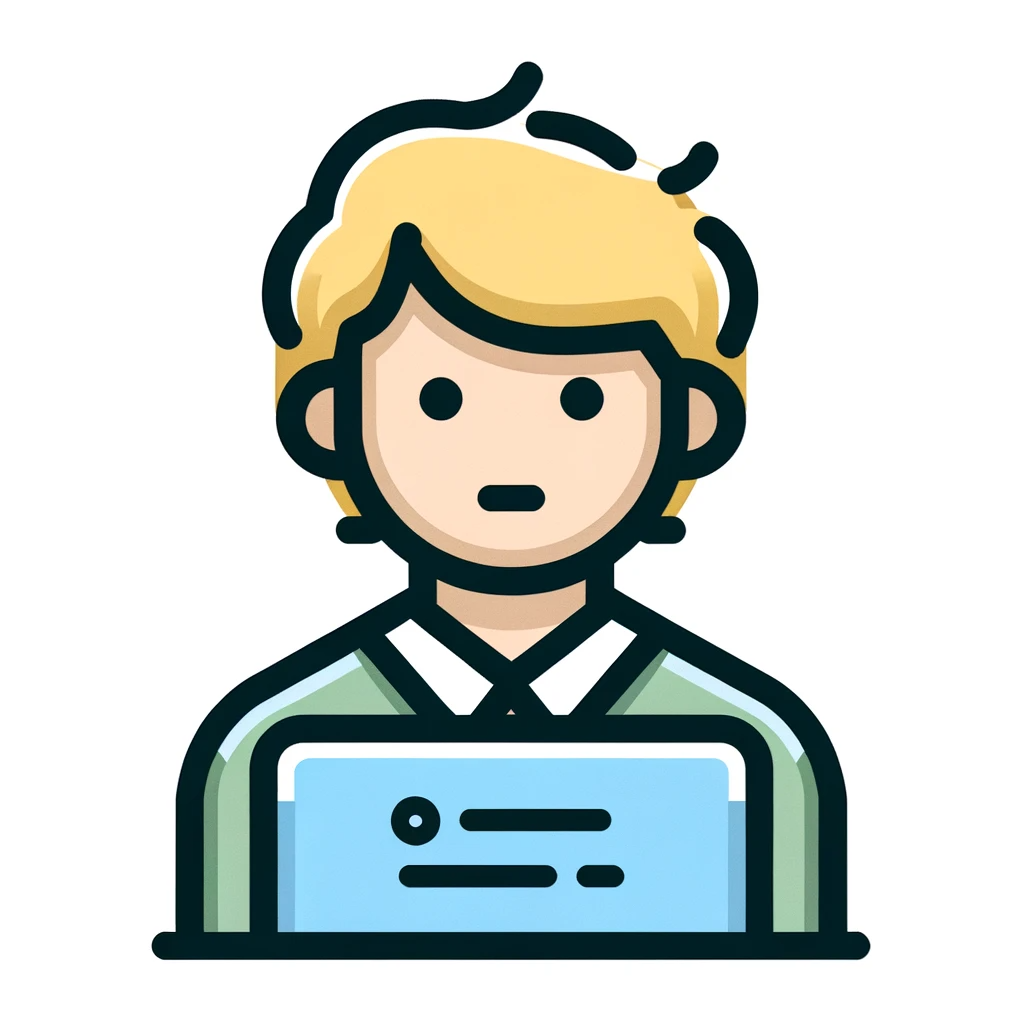
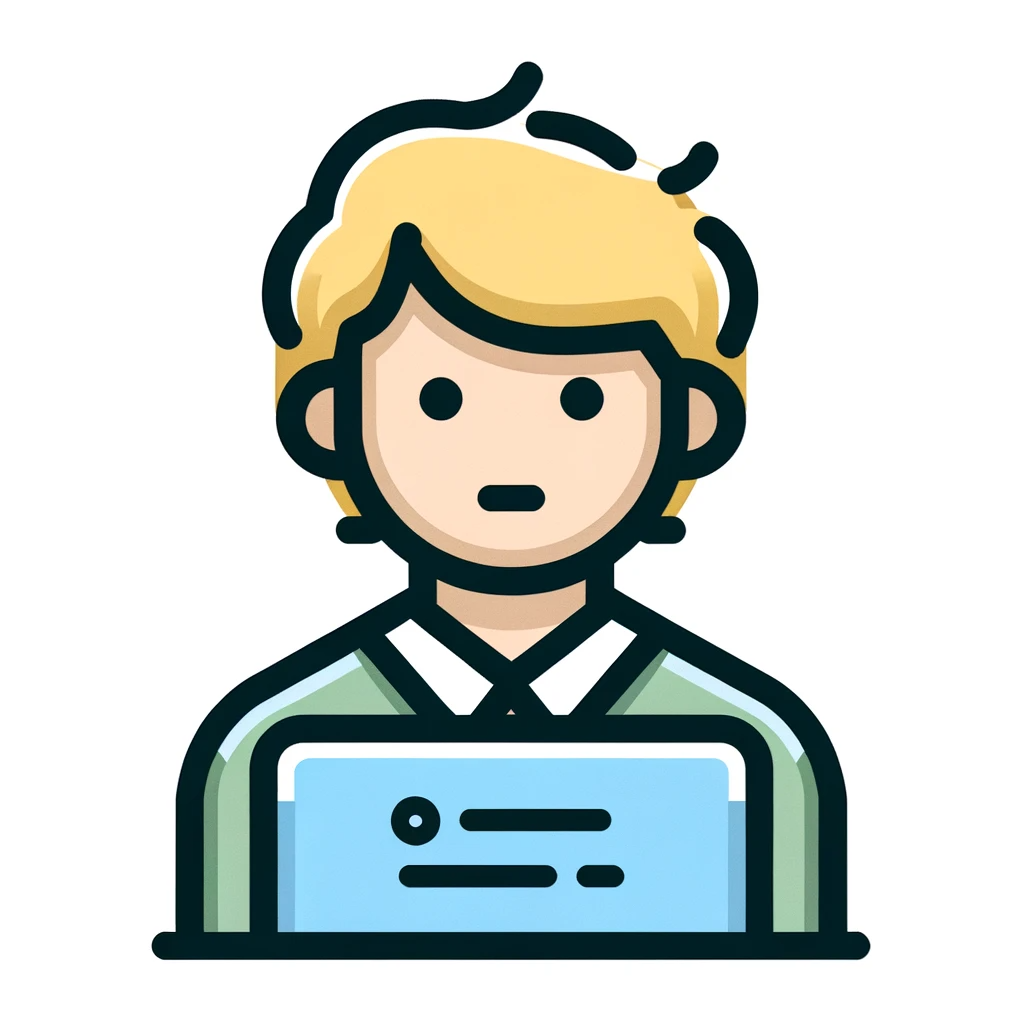
The two methods were easy to use and I was able to easily retrieve HTML elements.
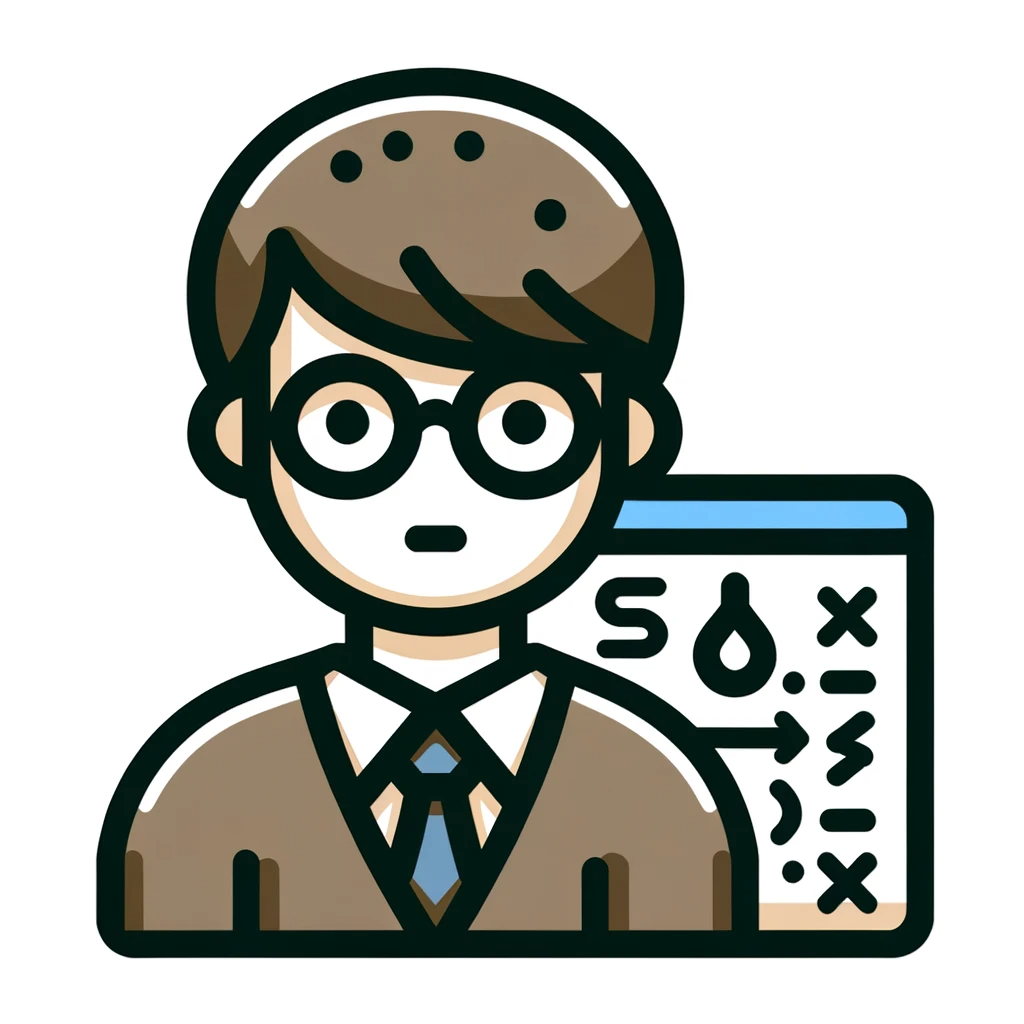
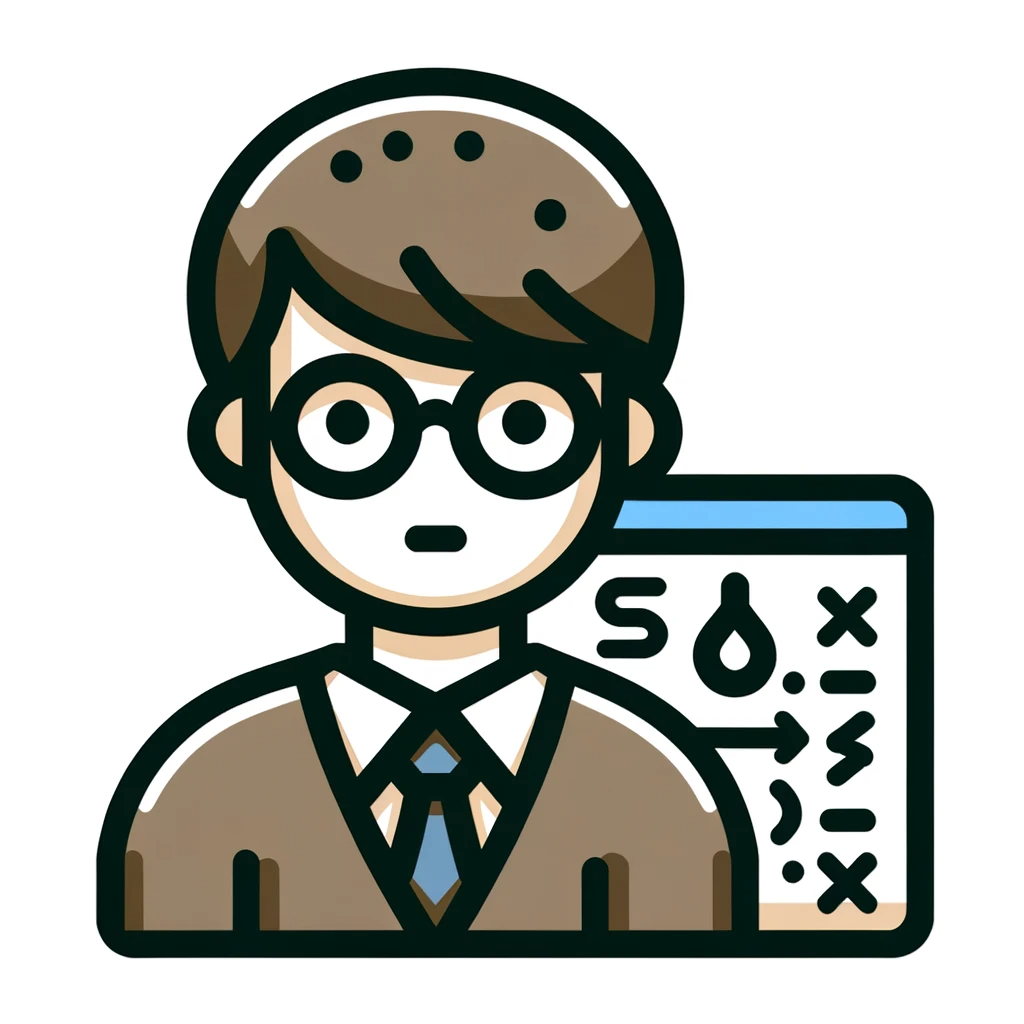
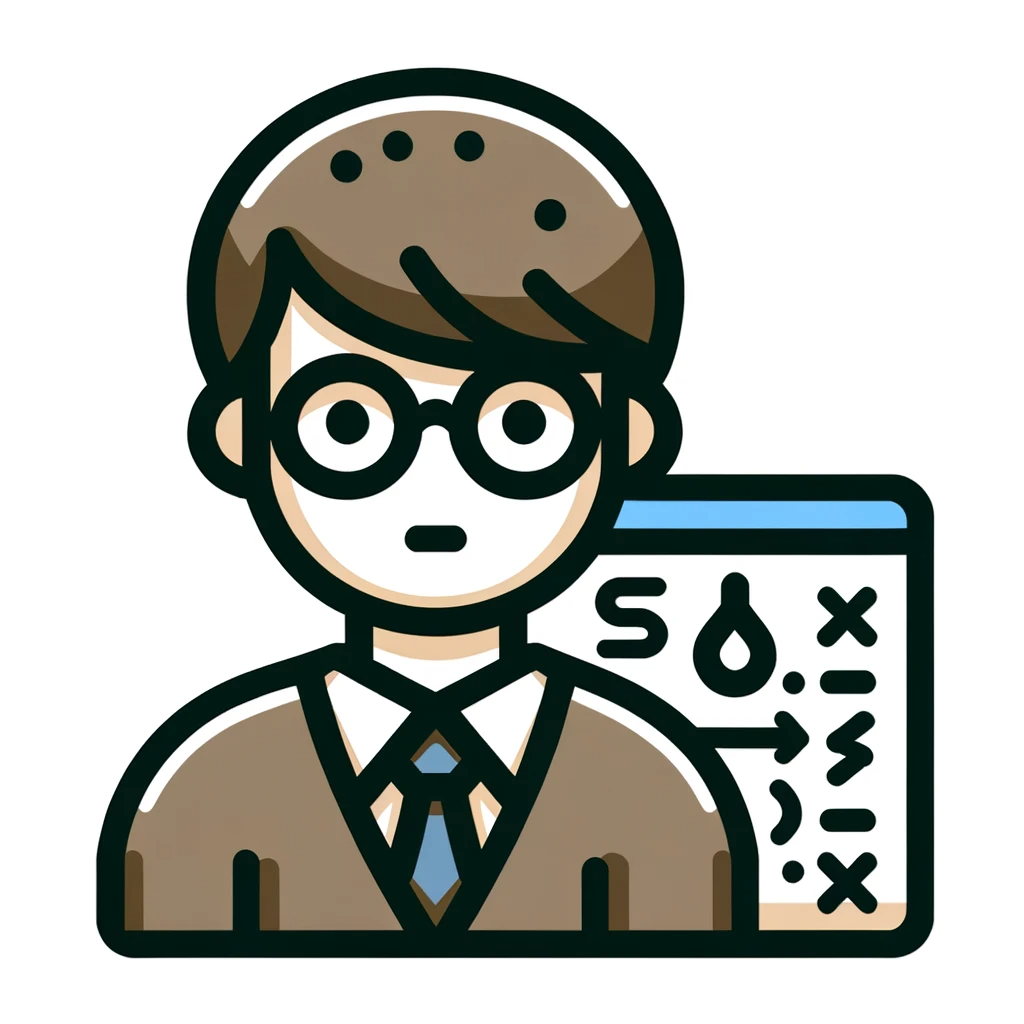
Similar to the “getElementsByClassName” method, the “querySelectorAll” method is also very useful.
Choose which method to use depending on your project and purpose.
Comments