This article explains how to process an array sequentially using forEach in JavaScript.
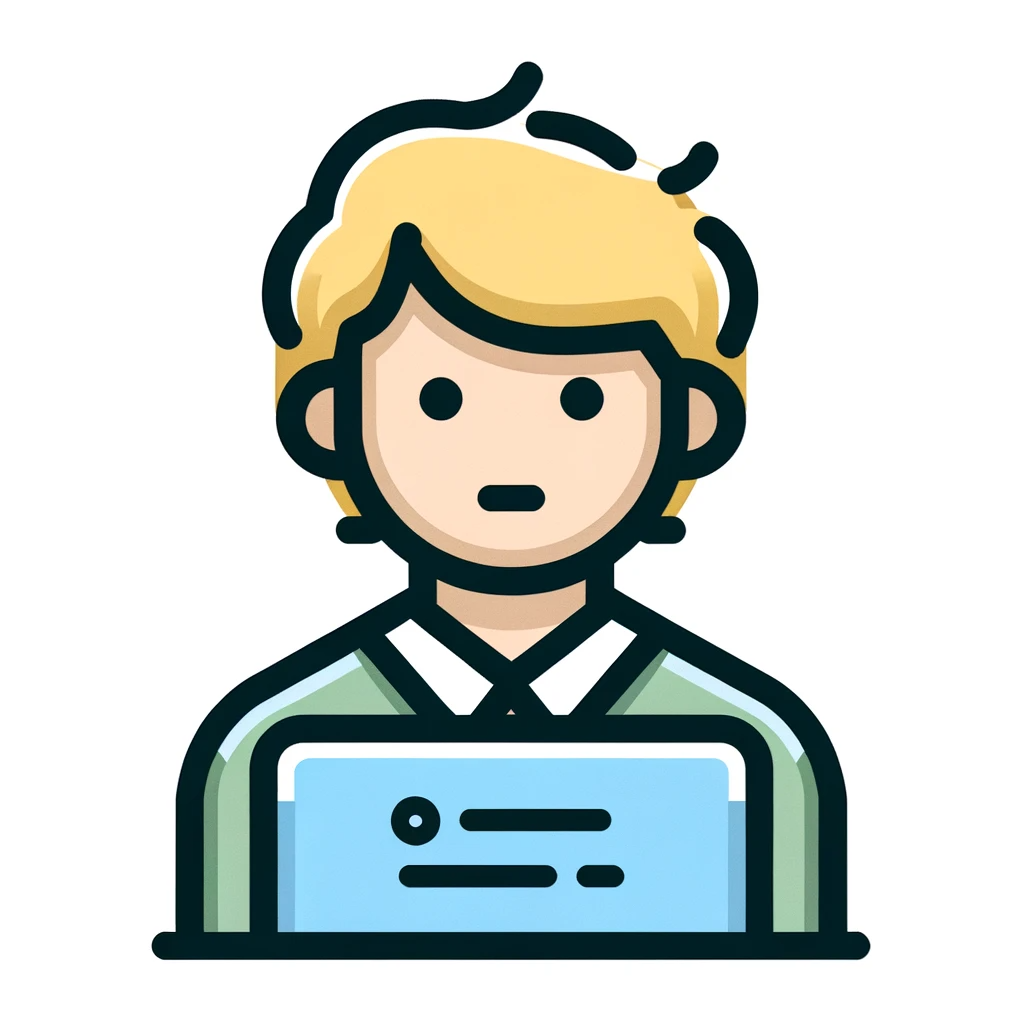
How can I process arrays with forEach?
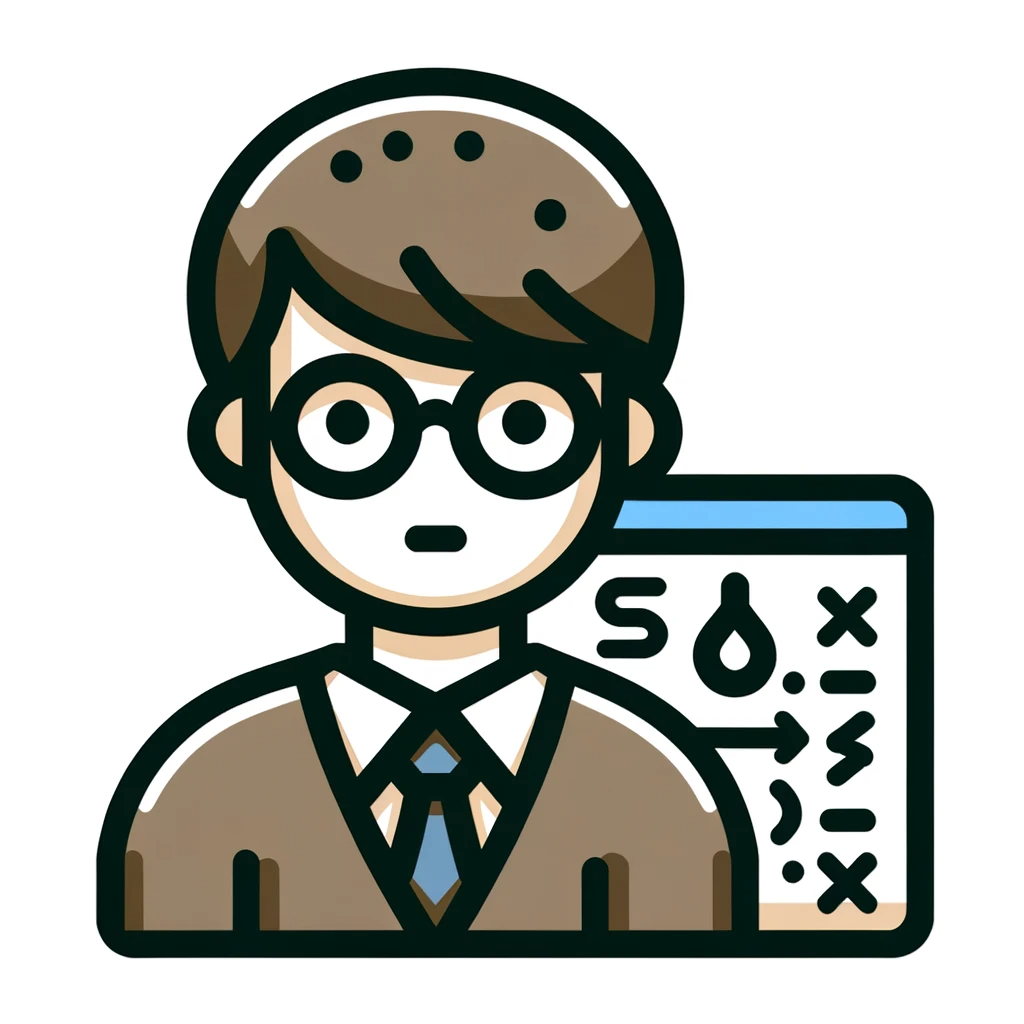
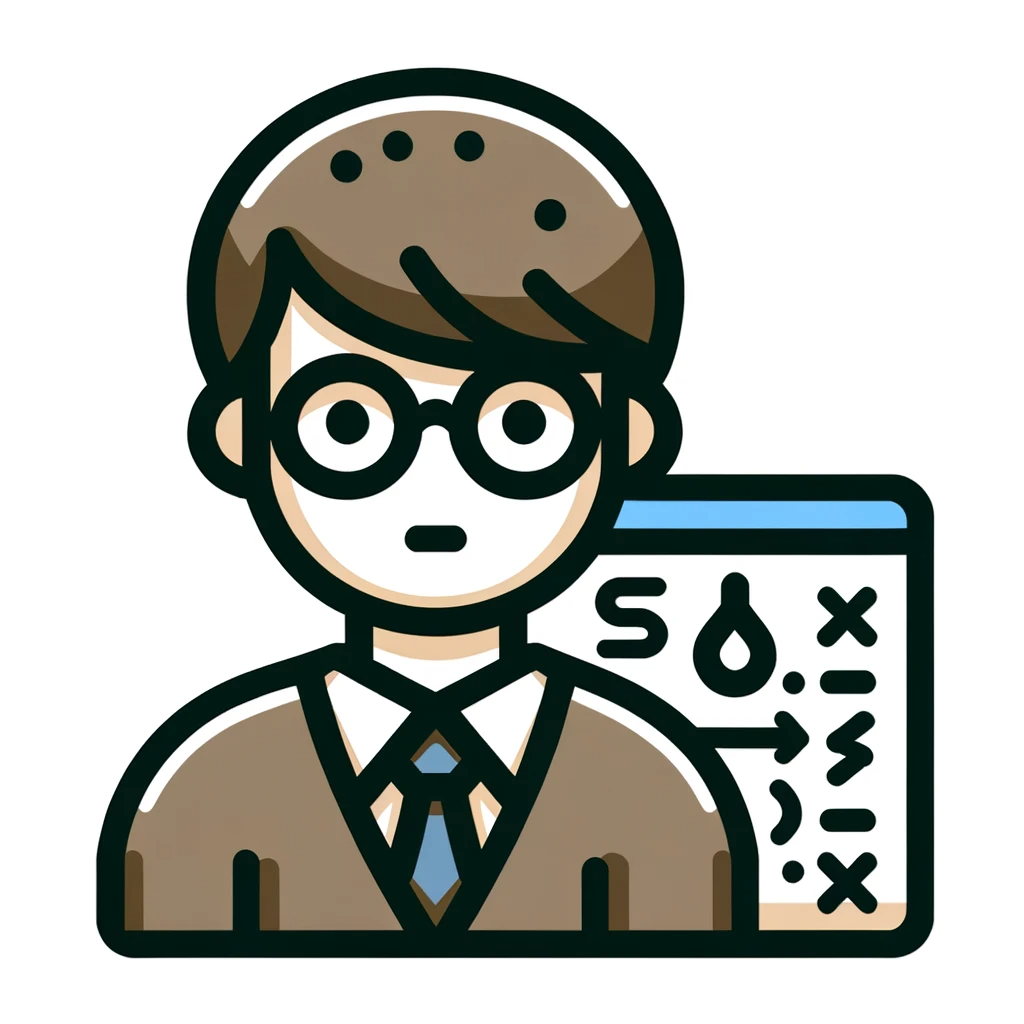
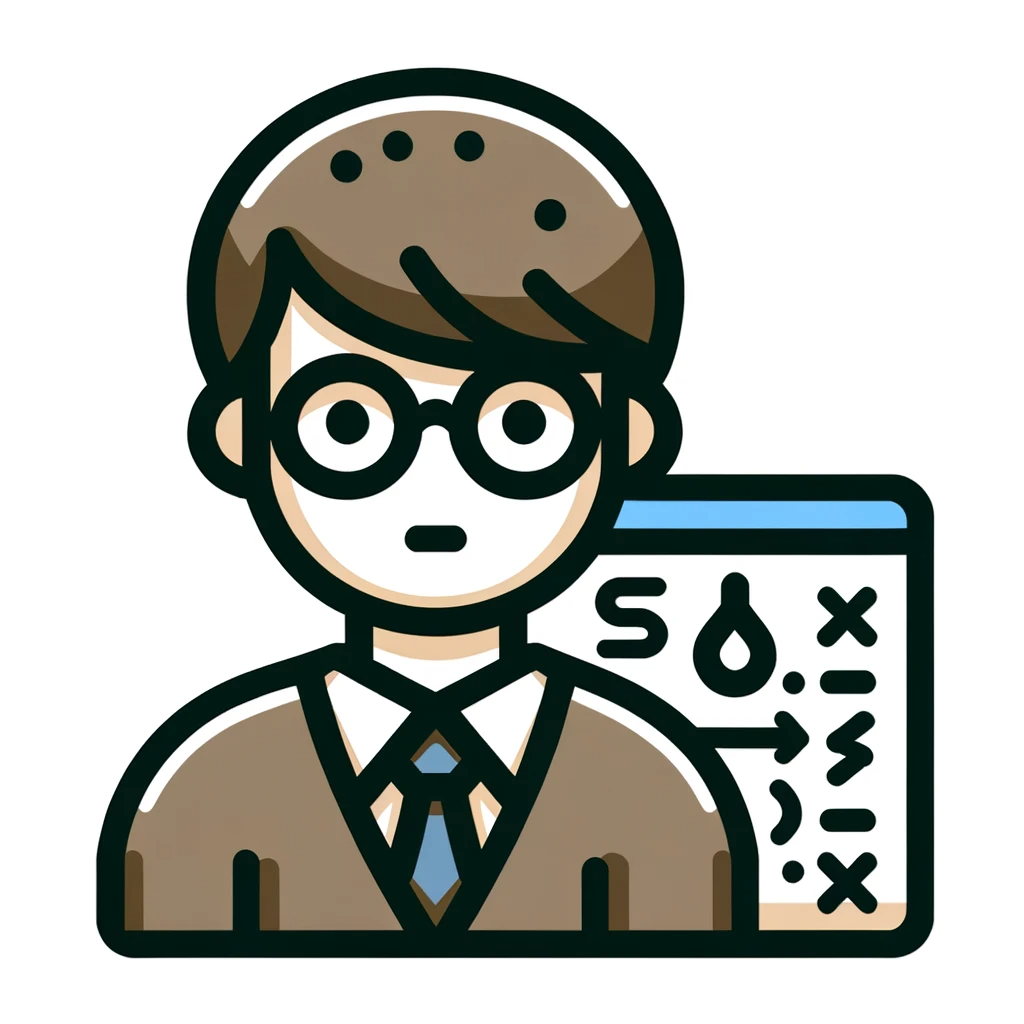
It is easy to use forEach to loop through an array.
Using forEach, you can repeat the process for each element of the array.
How to process arrays using forEach
forEach is used to iterate over each element of an array .
Below is a sample using four forEachs.
Output array elements one by one with forEach
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
This sample uses forEach to print the elements of the array numbers one after the other. For the first argument of forEach, pass a function that receives the elements of the array. This function receives the elements of an array and processes them.
In the above example, the received elements are output as is using console.log().
Output array elements with index using forEach
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number, index) {
console.log(index + ": " + number);
});
This sample uses forEach to print the indexed elements of the array numbers. For the first argument of forEach, pass a function that receives the array element and index.
This function takes an array element and an index and operates on them. In the above example, the index and element are concatenated as a string and output to console.log()
.
Add and output array elements with forEach
let numbers = [1, 2, 3, 4, 5];
let sum = 0;
numbers.forEach(function(number) {
sum += number;
});
console.log(sum);
This sample uses forEach to add and print the elements of the array numbers. For the first argument of forEach, pass a function that receives the elements of the array. This function receives the elements of an array and processes them.
In the above example, the received elements are added to the sum variable. Finally, after the forEach process is finished, the sum variable is output to console.log
.
Update array elements with forEach
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number, index, array) {
array[index] = number * 2;
});
console.log(numbers);
This sample uses forEach to update the elements of the array numbers. The first argument to forEach is a function that takes an array element, an index, and the array itself.
This function receives the elements of an array and processes them. In the above example, the value obtained by doubling the received element is assigned to the position specified by the index.
Finally, after the forEach process is finished, the numbers array is output to console.log.
Summary of this article
I explained how to use forEach.
- By using forEach, you can process the elements of an array in order.
- Pass a function that receives the array element, index, and the array itself as the first argument of forEach.
- Updating an array element within forEach is achieved by assigning it to the position specified by the index.
By using forEach, you can easily process arrays.
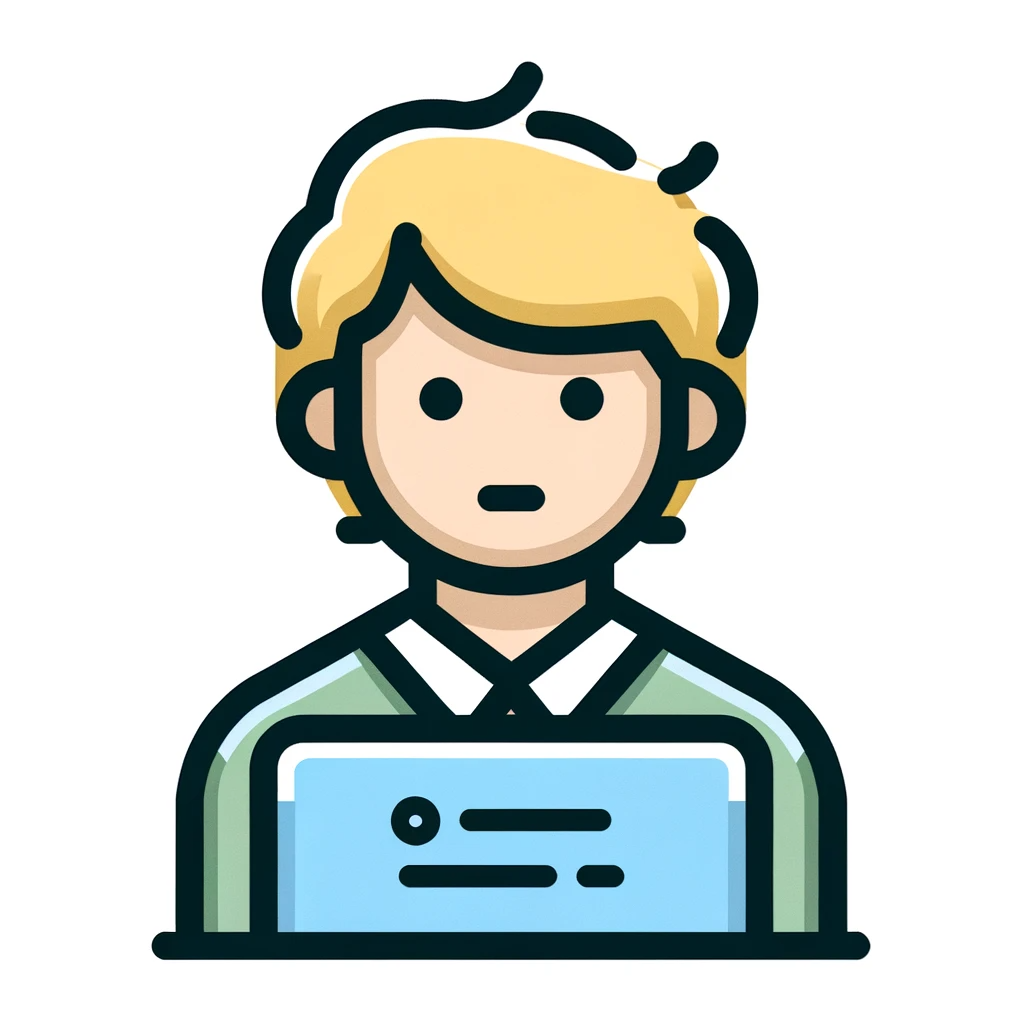
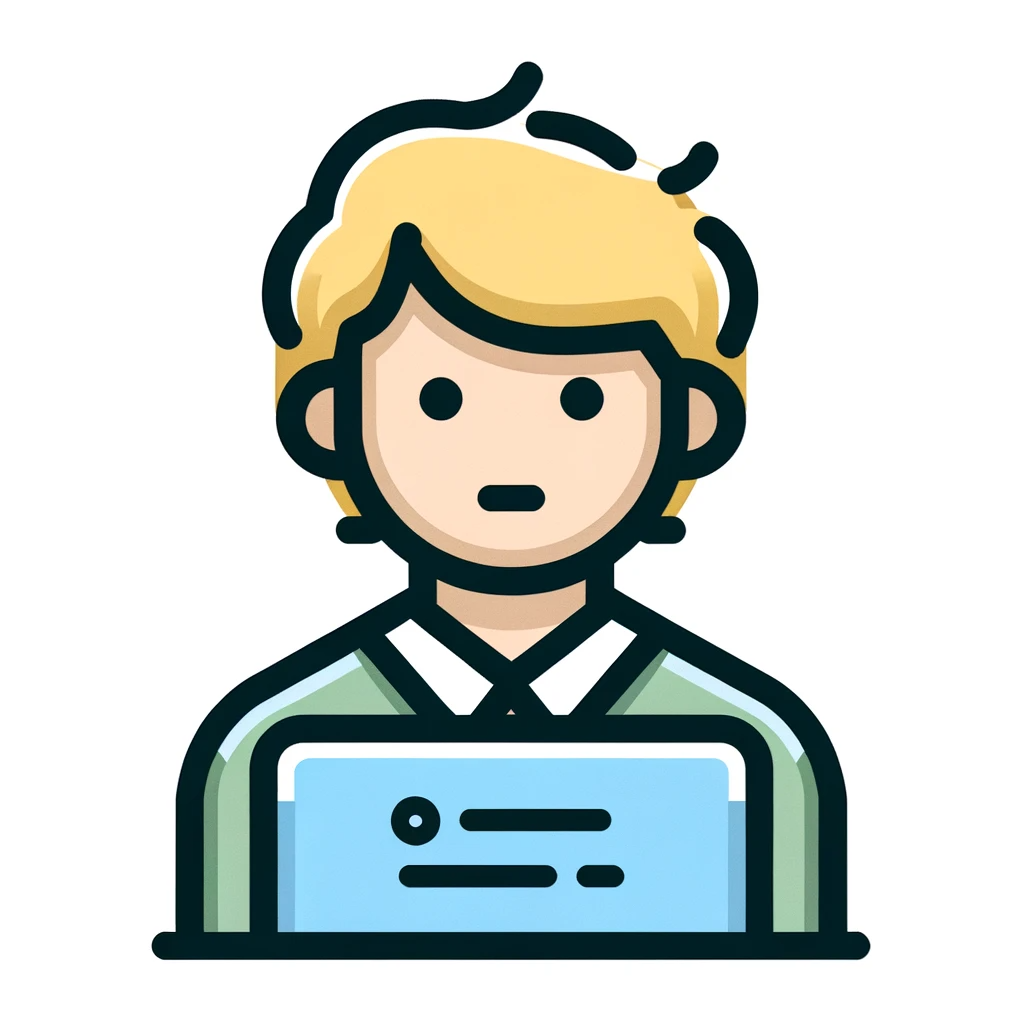
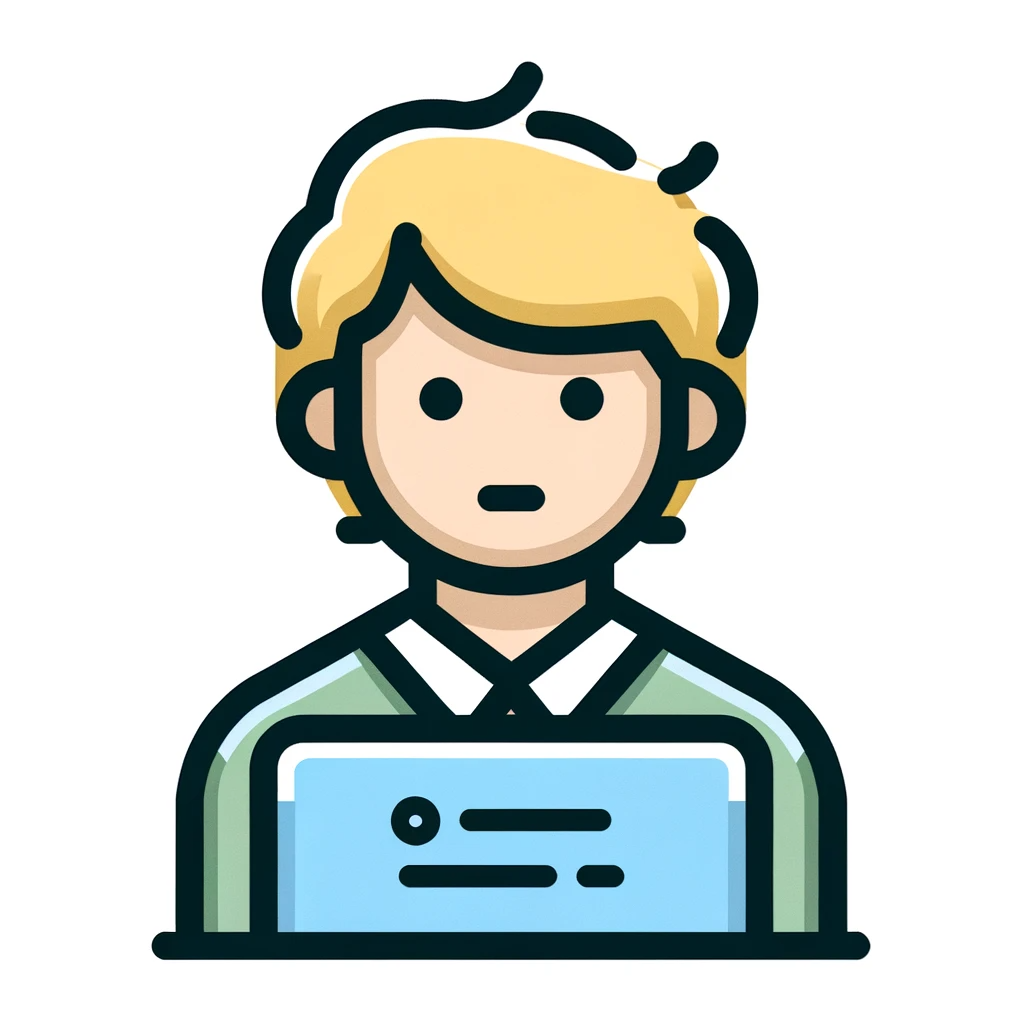
I was able to use forEach to process the elements of an array in order!
Comments