This article explains how to use generators in JavaScript.
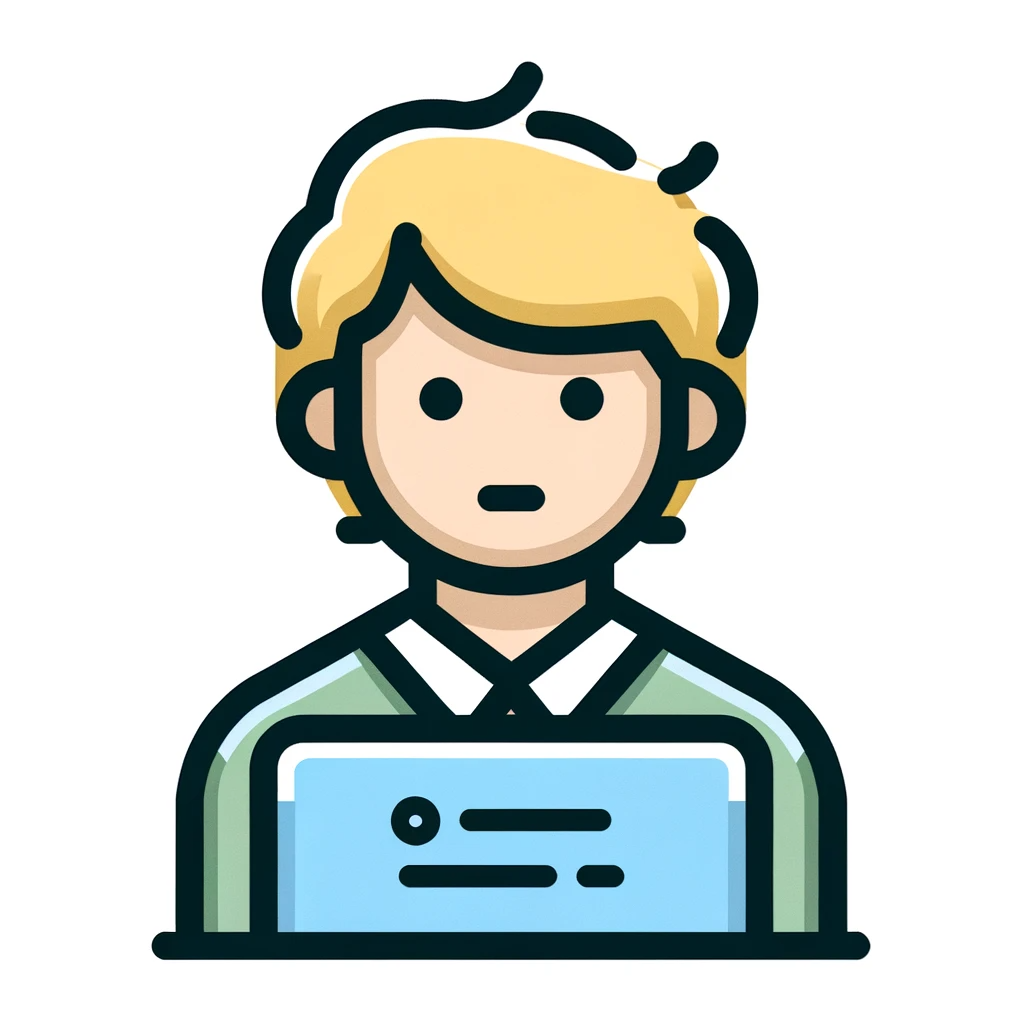
Please tell me about enumeration using generators.
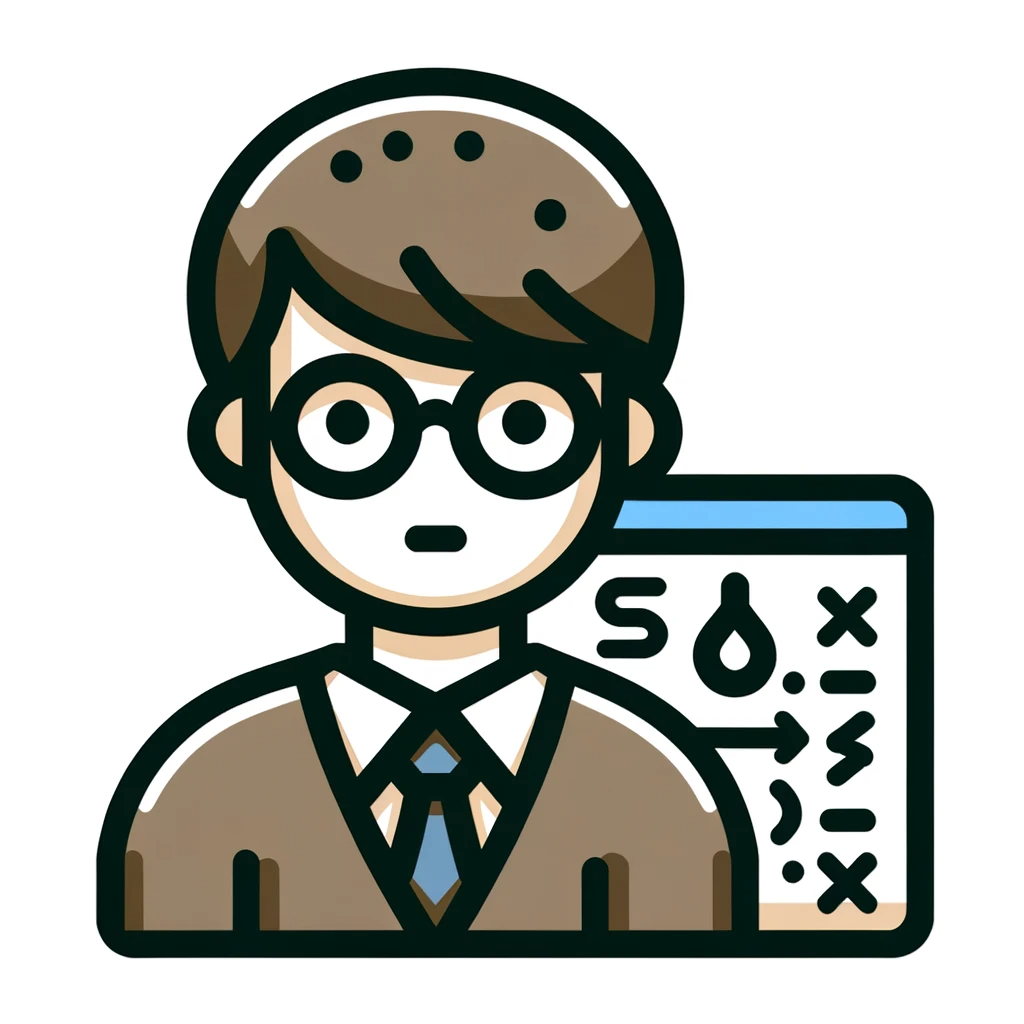
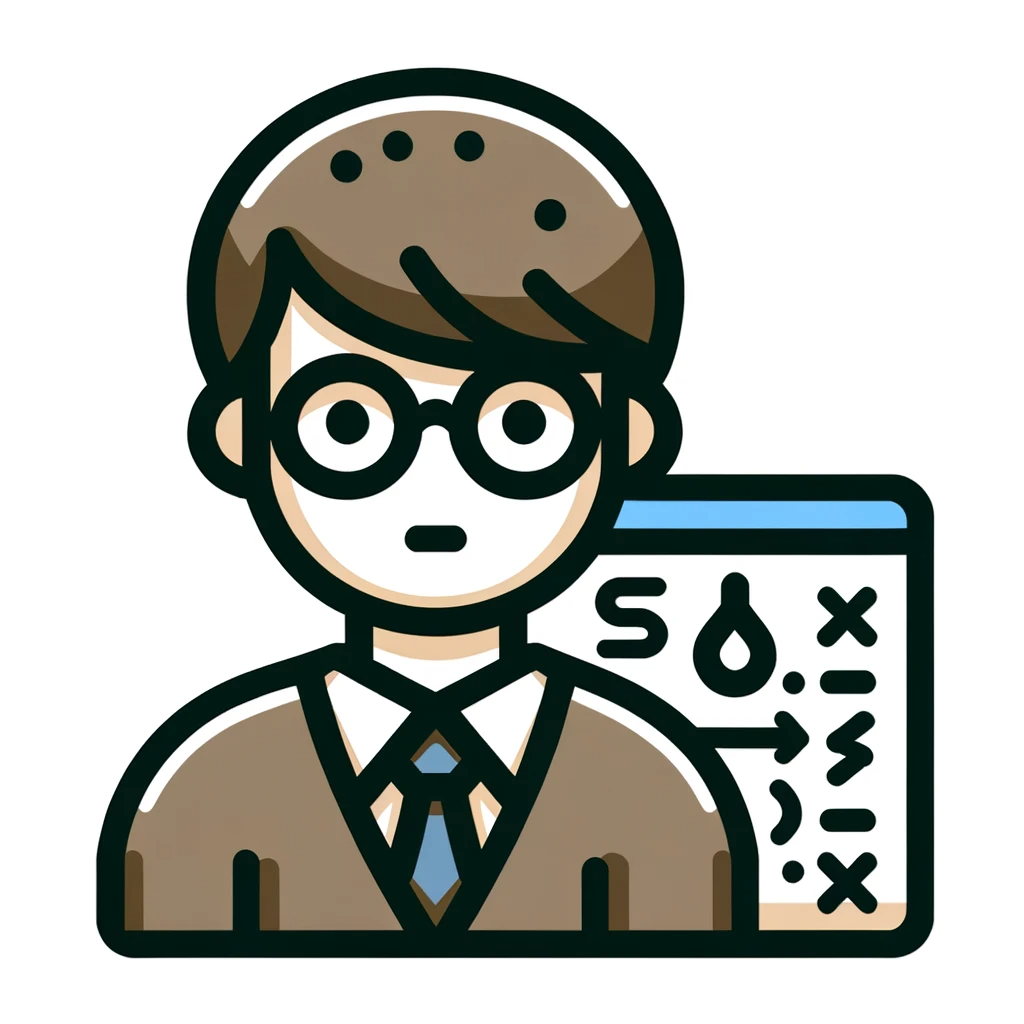
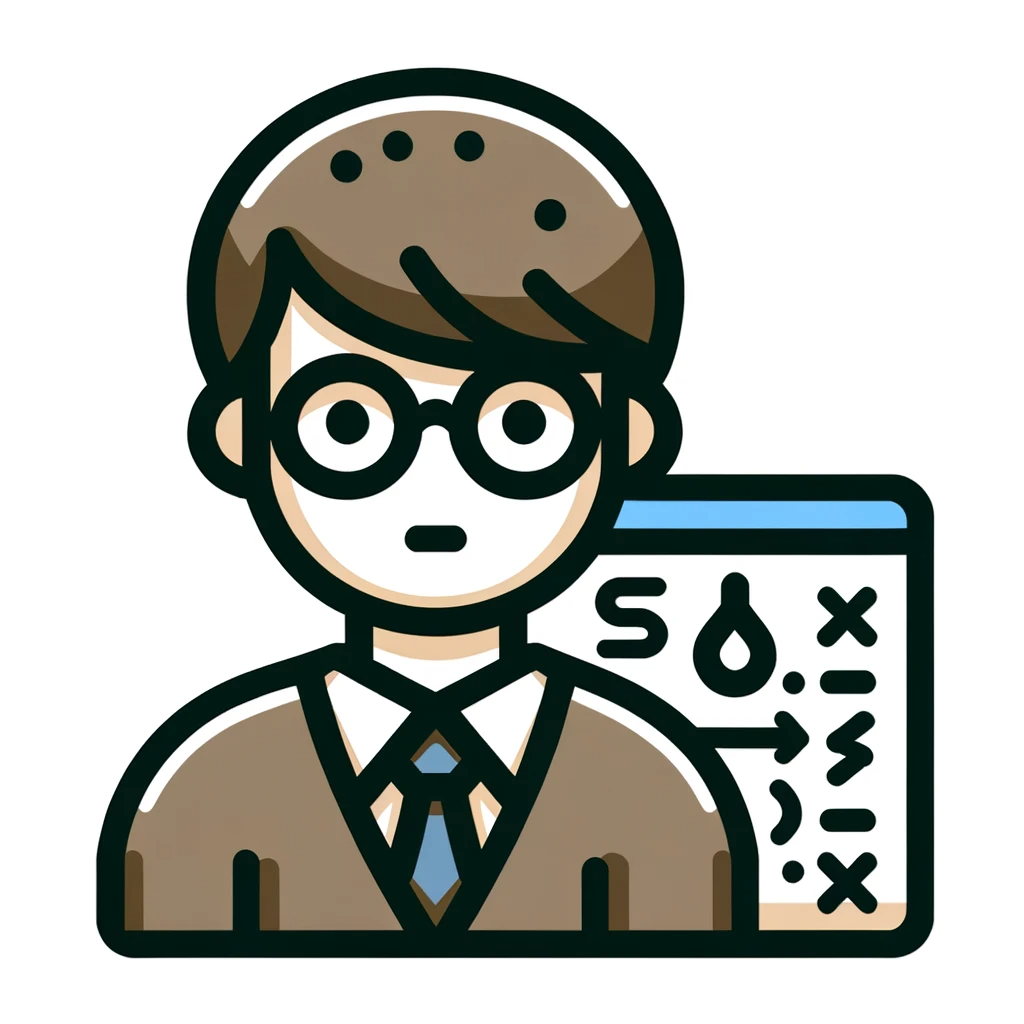
Generators allow you to easily generate enumerable values using for…of loops.
What is a generator?
In JavaScript, a generator is a function that generates an iterator. Generators allow you to easily generate enumerable values using for…of loops.
An iterator is an object whose elements can be accessed “in order”. In JavaScript, iterators have an internal “next()” method that returns the next element when this method is called.
How to enumerate with for…of
I will explain how to enumerate with for…of using a generator . Define a generator function and use a yield statement within it to generate a value. You can then enumerate its values using for…of.
Below is a sample program that uses a generator function to enumerate with for…of.
function* generator() {
yield 1;
yield 2;
yield 3;
}
for (const value of generator()) {
console.log(value);
}
Also, below is a sample generator function that takes start and end numbers as arguments and outputs the value between them.
function* generator(start, end) {
for (let i = start; i <= end; i++) {
yield i;
}
}
for (const value of generator(5, 10)) {
console.log(value);
}
About yield
”yield” is a keyword used within the generator function. Generator functions can generate values using “yield” within the function.
When called within a generator function, ‘yield’ suspends execution of the generator function at that point and returns the generated value to the caller. The next time you call the generator function, it will pick up where it left off on the previous call. By repeating these movements, the generator function can generate values in sequence.
The sample generator function below generates numbers from 1 to 5.
function* generator() {
yield 1;
yield 2;
yield 3;
yield 4;
yield 5;
}
const iterator = generator();
console.log(iterator.next().value); // 1
console.log(iterator.next().value); // 2
console.log(iterator.next().value); // 3
console.log(iterator.next().value); // 4
console.log(iterator.next().value); // 5
Summary of this article
I explained how to use the generator.
- In JavaScript, a generator is a function that generates an iterator.
- You can use for…of to enumerate the values generated by a generator function.
- “yield” is a keyword used within the generator function.
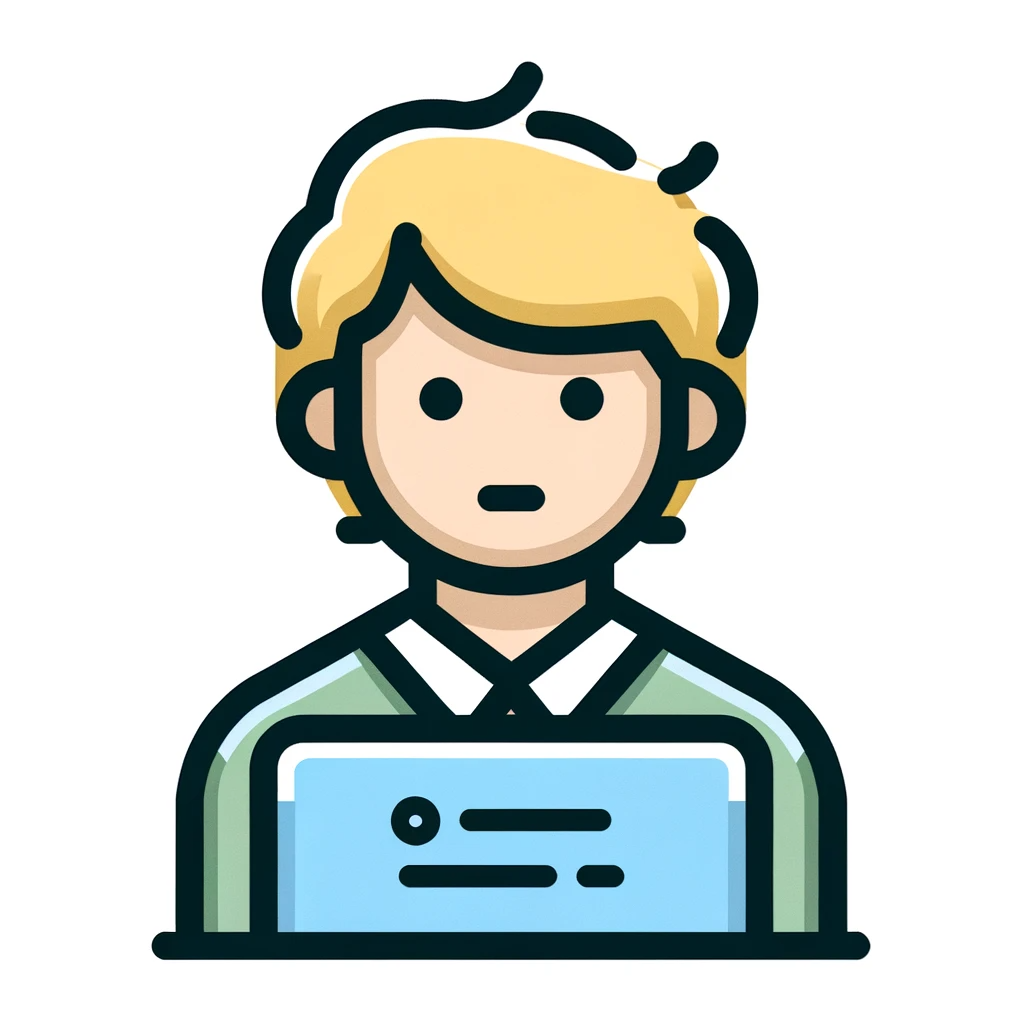
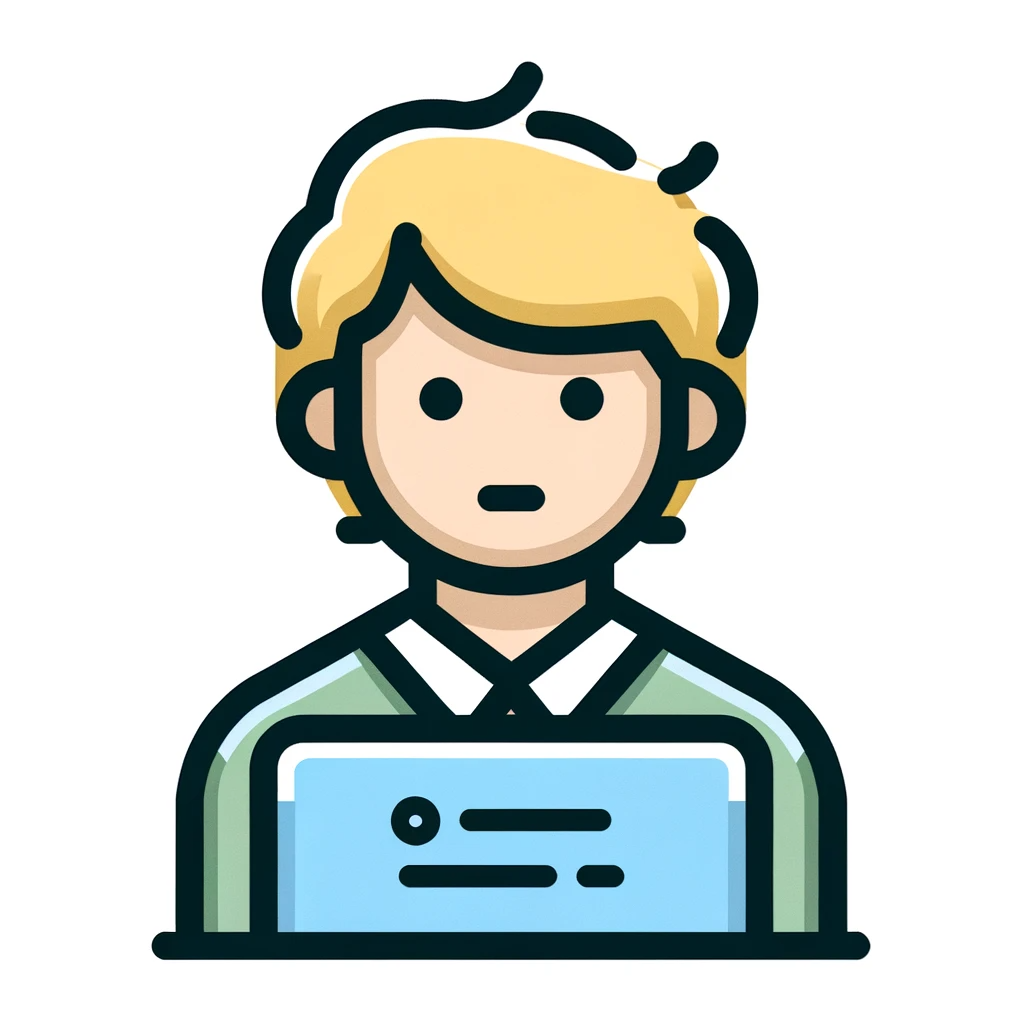
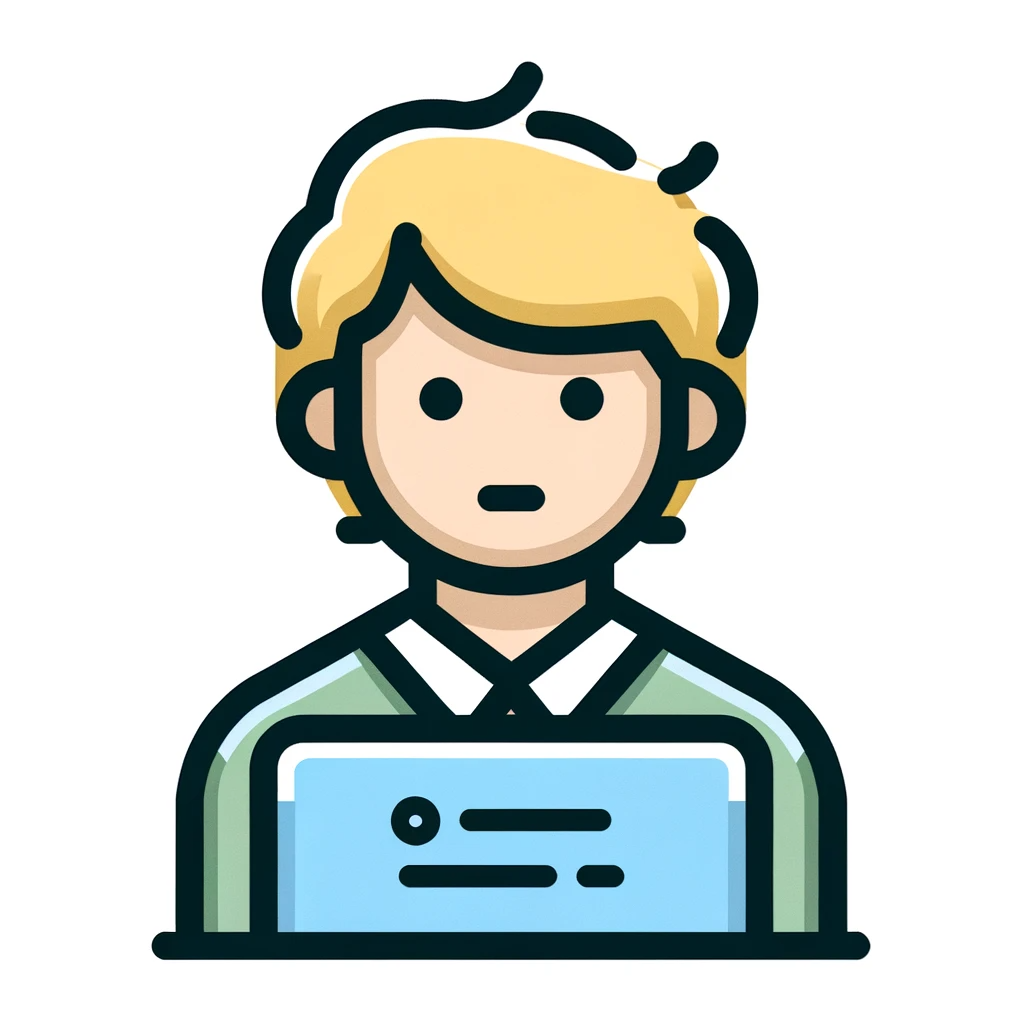
I was able to learn about generator functions and how to use “yield”!
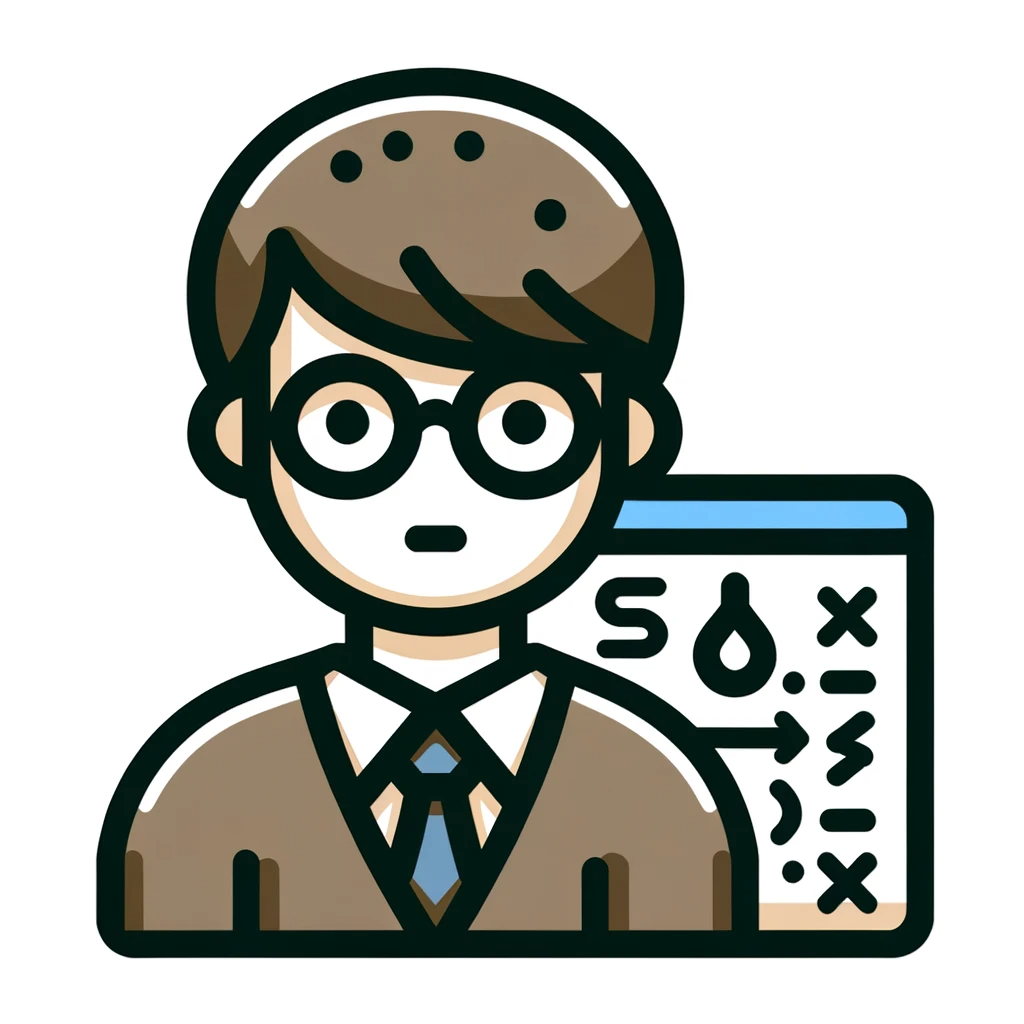
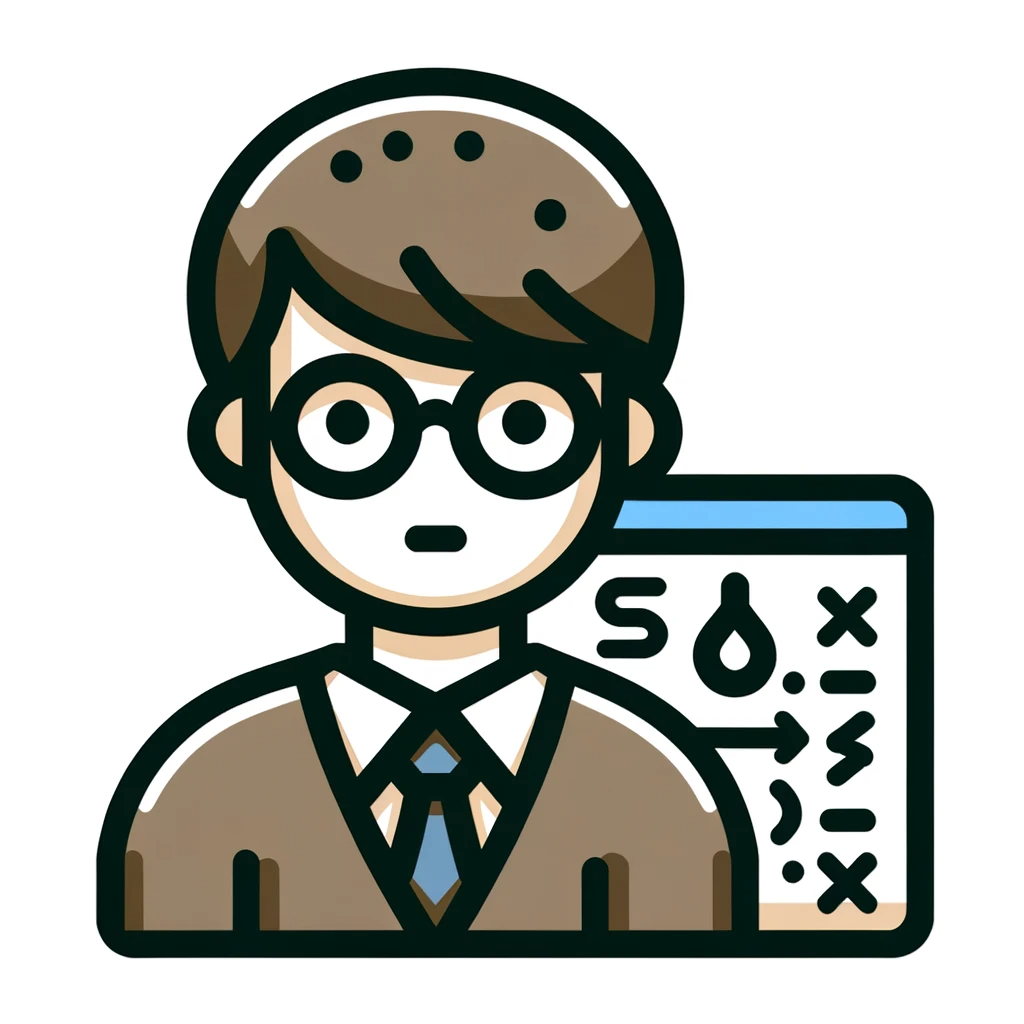
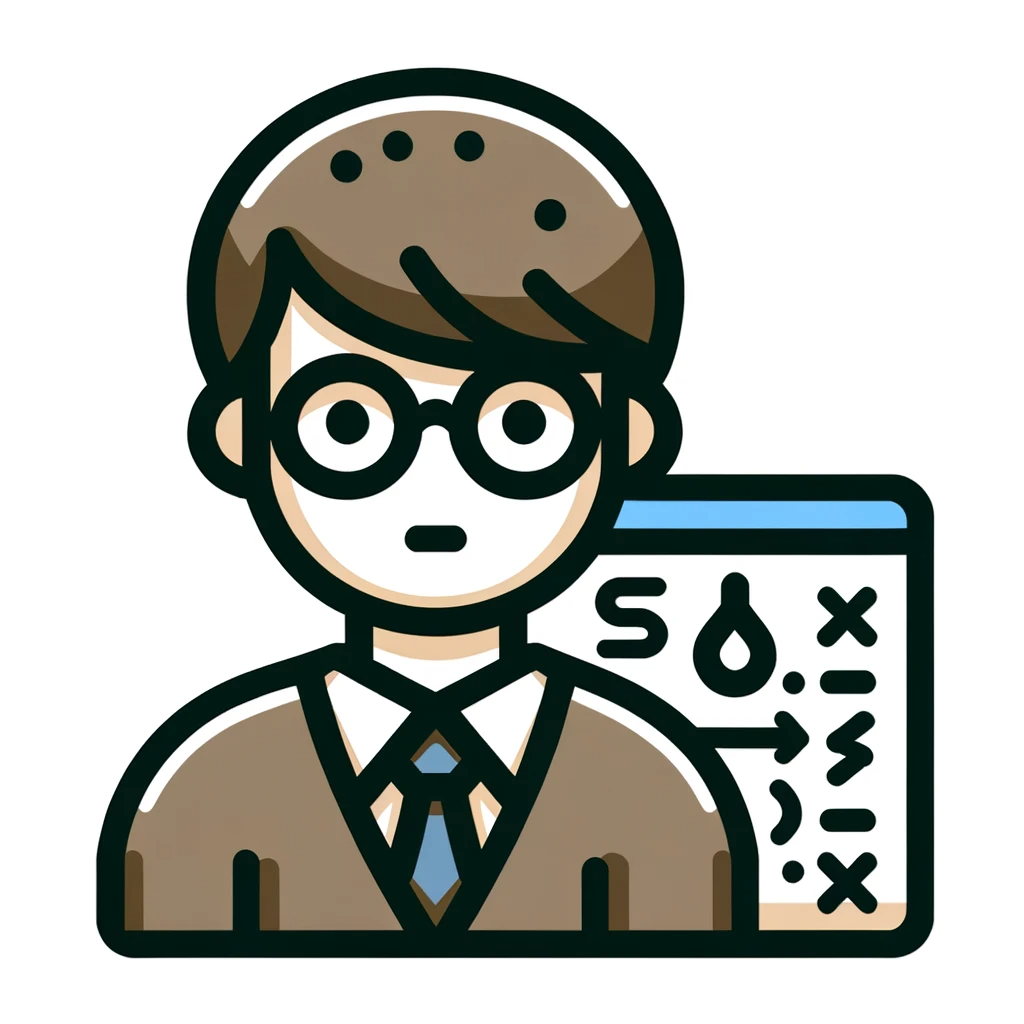
Try it out and find out how you can use it conveniently.
Comments