This article explains how to conditionally extract elements of an array using JavaScript.
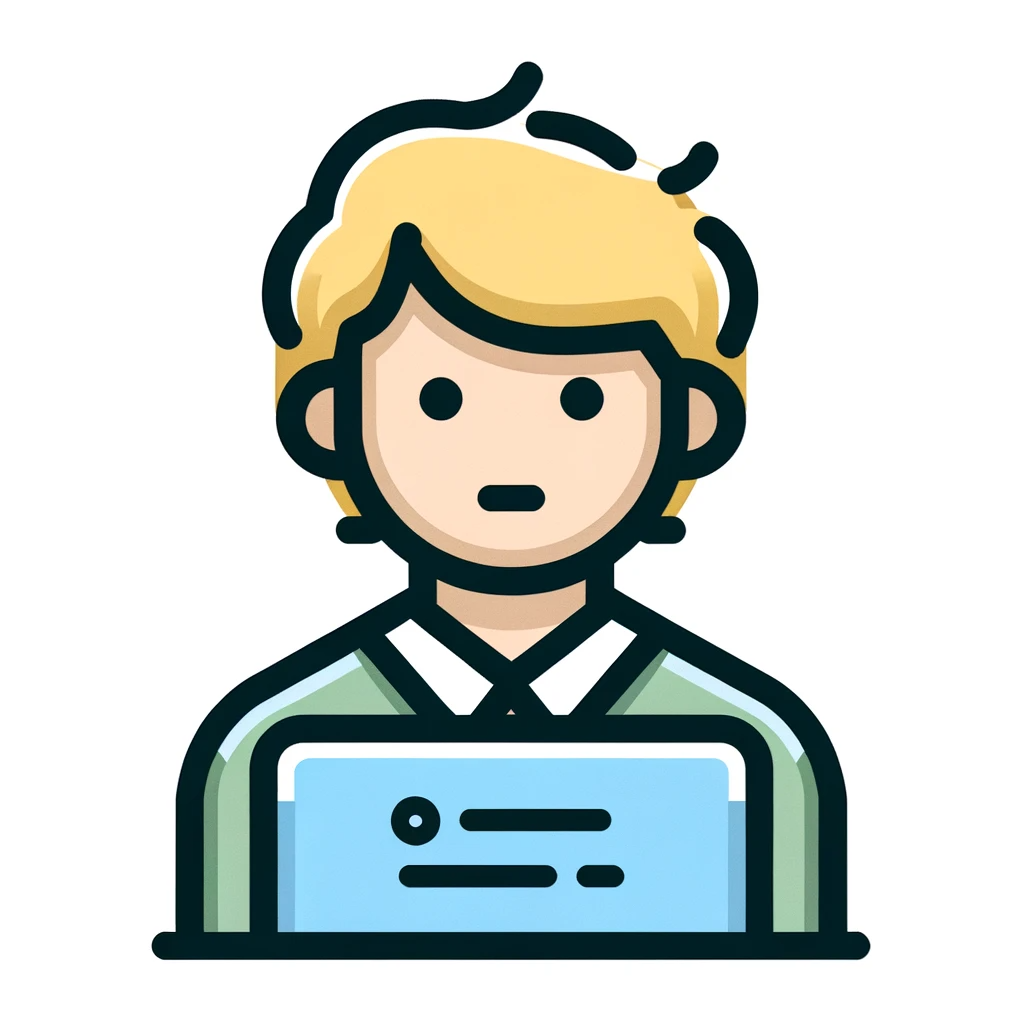
Is there a way to conditionally extract elements of an array?
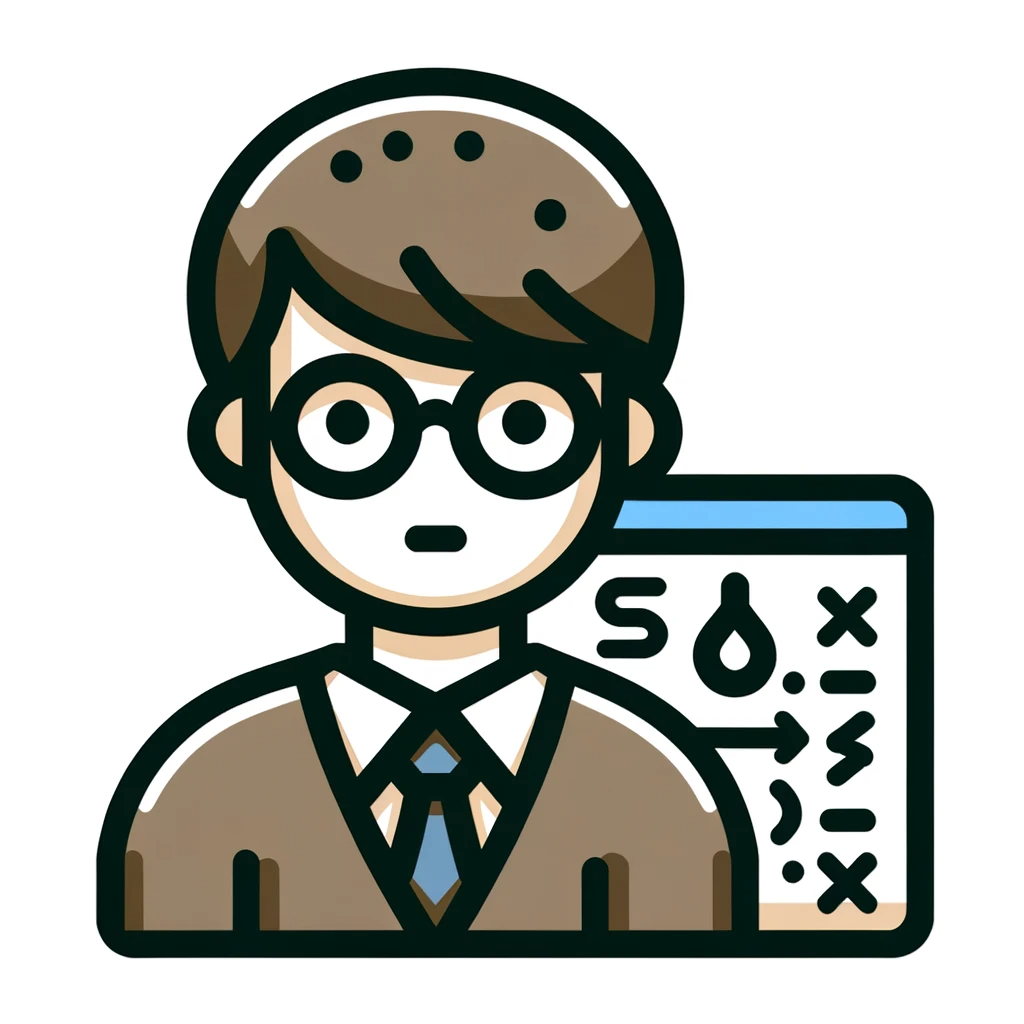
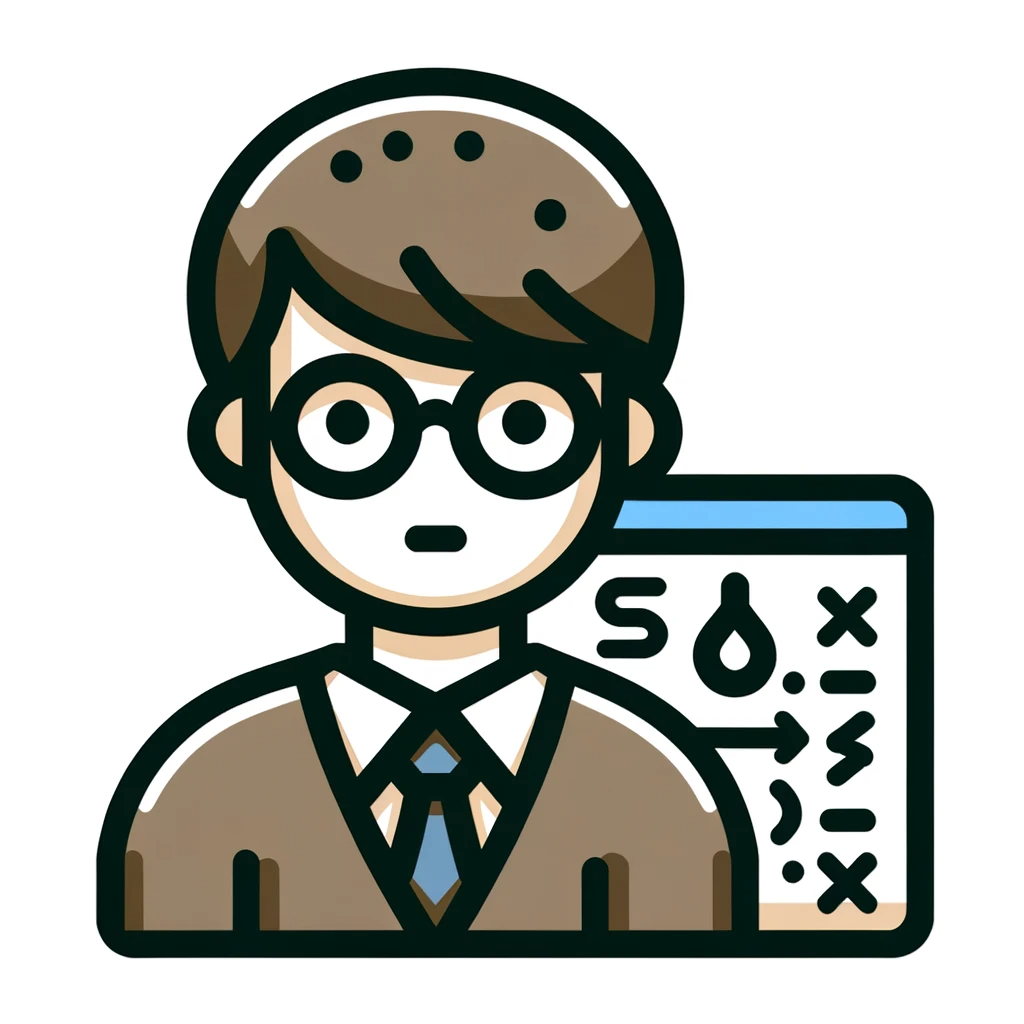
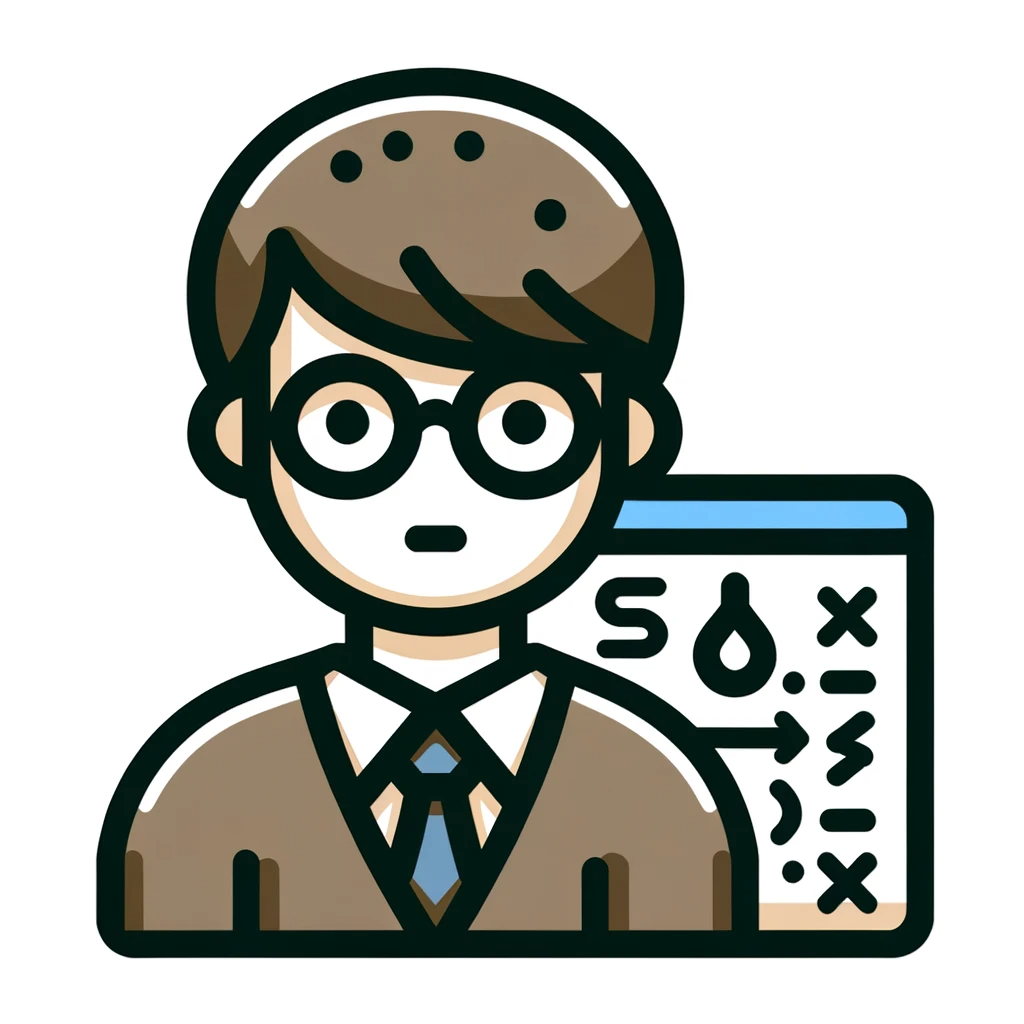
You can easily extract the elements of an array by using the filter method.
How to extract conditions using the filter method
The filter method uses a callback function to determine the elements of an array and stores only those elements that meet the criteria into a new array.
The filter method passes each element of an array to a function and returns a new array containing only those elements for which the result is true.
Three arguments are passed to the function: “each element of the array,” “its index,” and “the original array.”
array.filter(function(element value, index, original array){ ..Processing to determine true/false… } [, thisValue])
The following is a sample program that extracts only elements that meet specified conditions.
// array
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
// Extract elements that match the criteria
const evenNumbers = numbers.filter(function(number) {
return number % 2 === 0;
});
console.log(evenNumbers); // [2, 4, 6, 8, 10]
In the above sample, the filter method is used to extract only even numbers from the numbers array and store them in the evenNumbers array. The function passed to the filter method determines whether the number received as an argument is divisible by 2, and only if the result is true is it stored in the array evenNumbers.
How to specify the second argument (thisValue) of the filter method
You can also specify a parameter called thisValue as the second argument to this filter method. By specifying this, you can specify a value that can be referenced as this in the function used inside the filter method.
const obj={a:2};
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = numbers.filter(function(number) {
return number % this.a === 0;
}, obj);
console.log(evenNumbers); // [2, 4, 6, 8, 10]
In this sample program, only even numbers are extracted from the numbers array and stored in the evenNumbers array. Pass a function to process the array elements as the first argument of the filter function. This function is passed an element, which is used to evaluate the condition.
The function in the first argument determines the element based on the condition “number % this.a === 0”.
Pass the object to be used as this in the second argument. In this example, we are passing an object named obj. Since obj.a is 2, this.a in the first argument function will be 2, and only even numbers in the numbers array will be stored in the evenNumbers array.
Determining the condition of array elements used by some method
The some method is used to determine whether there are any elements in the array that match the specified conditions.
Pass a callback function that describes the processing to be performed on the array elements as an argument.
array.some(function(element value, index, original array) , [, thisValue])
Summary of this article
We explained how to extract elements of an array based on conditions using the filter method.
- You can use the filter method to extract elements of an array based on specific conditions.
- Pass the function that describes the extraction conditions to the first argument.
- By specifying thisValue as the second argument, it will be used as this in the extraction condition function.
- The filter method returns a new array, leaving the original array unchanged.
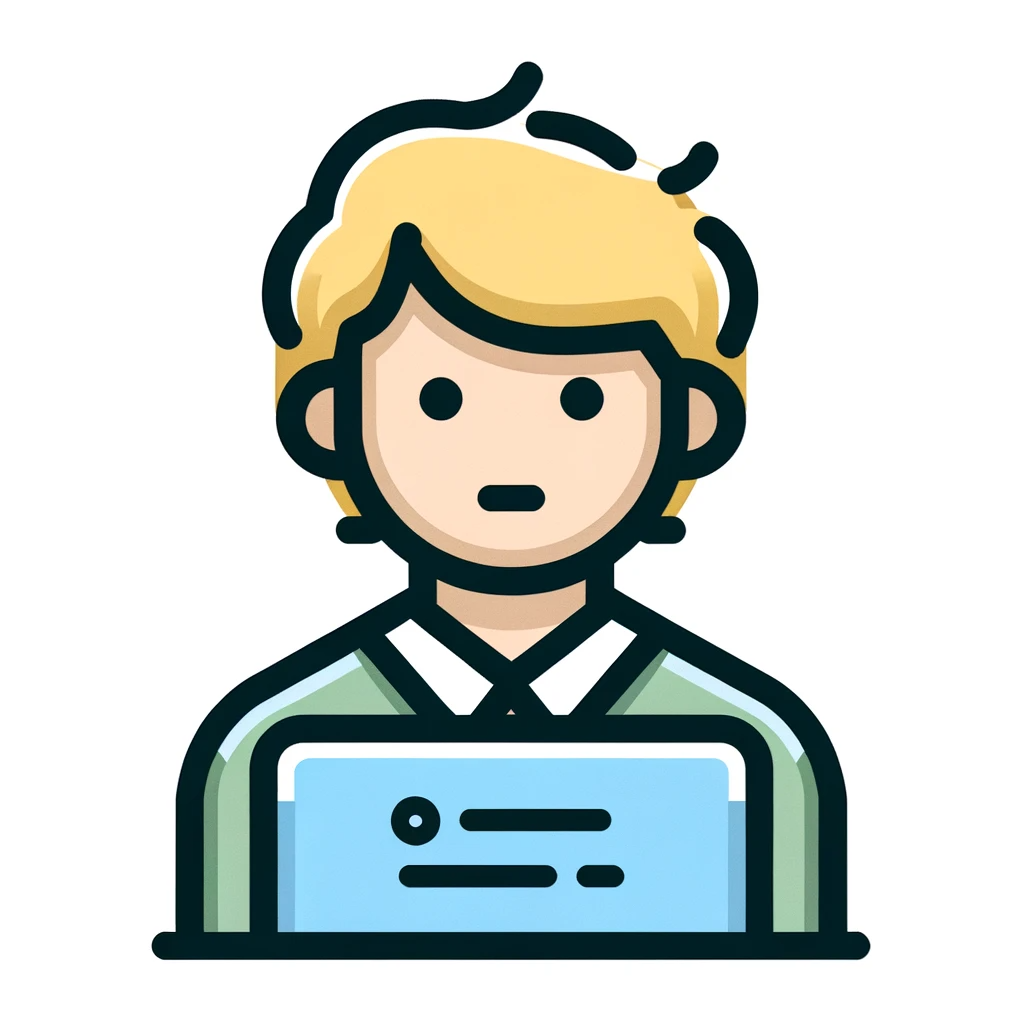
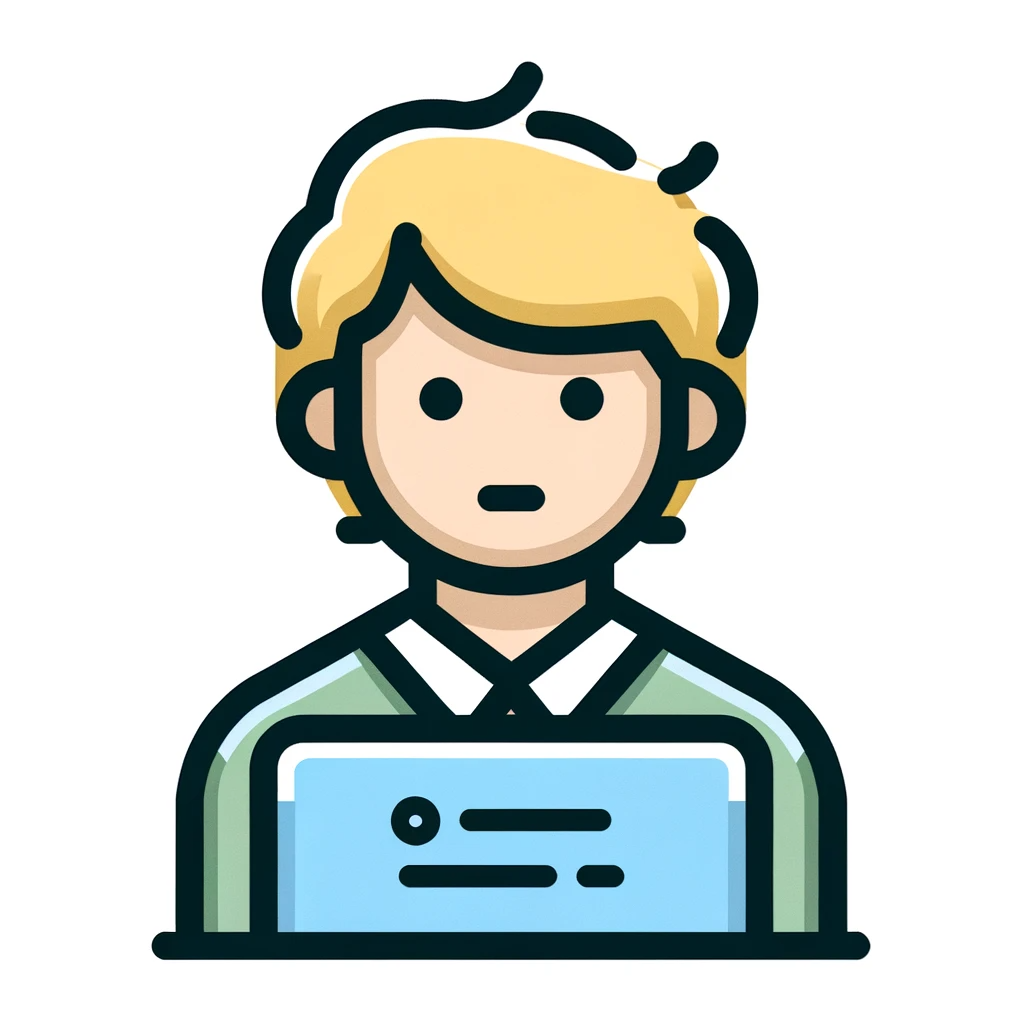
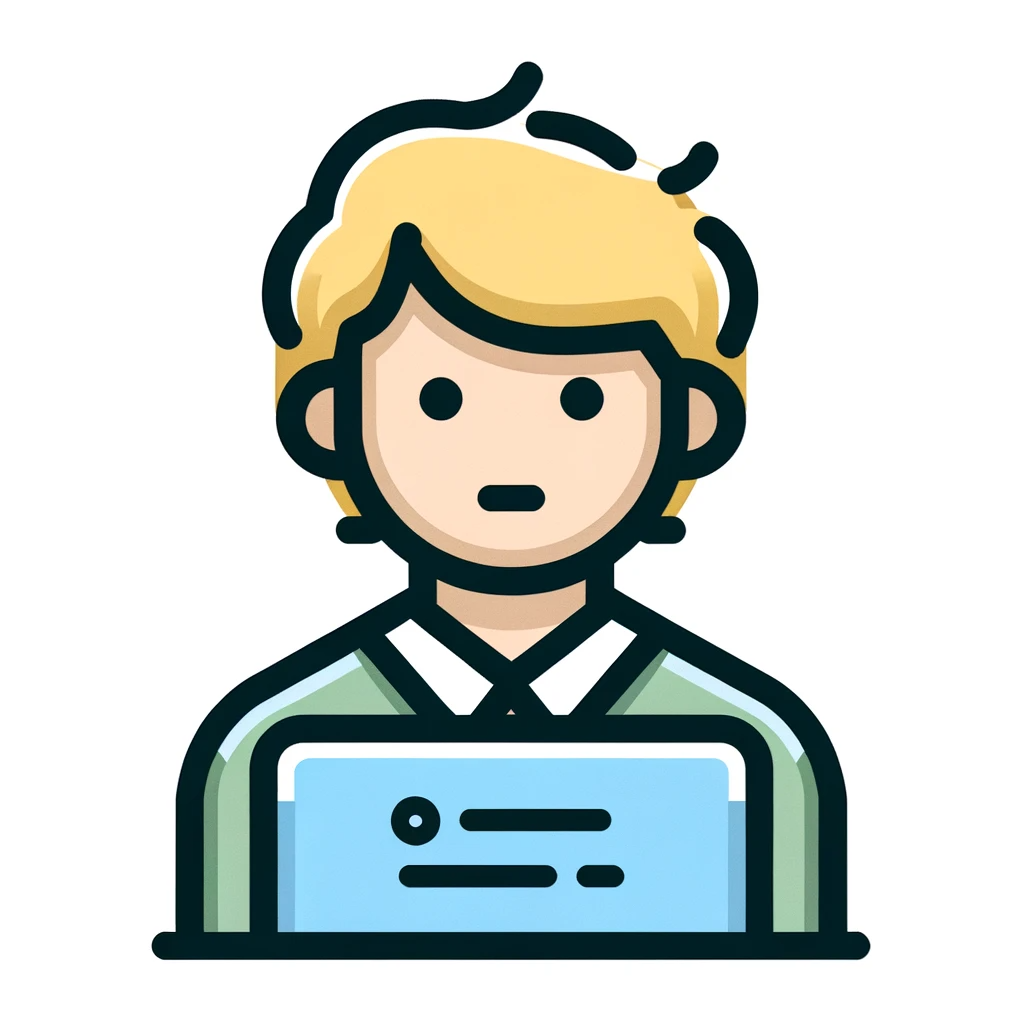
The filter method is easy to use and useful for extracting elements that meet certain conditions from an array!
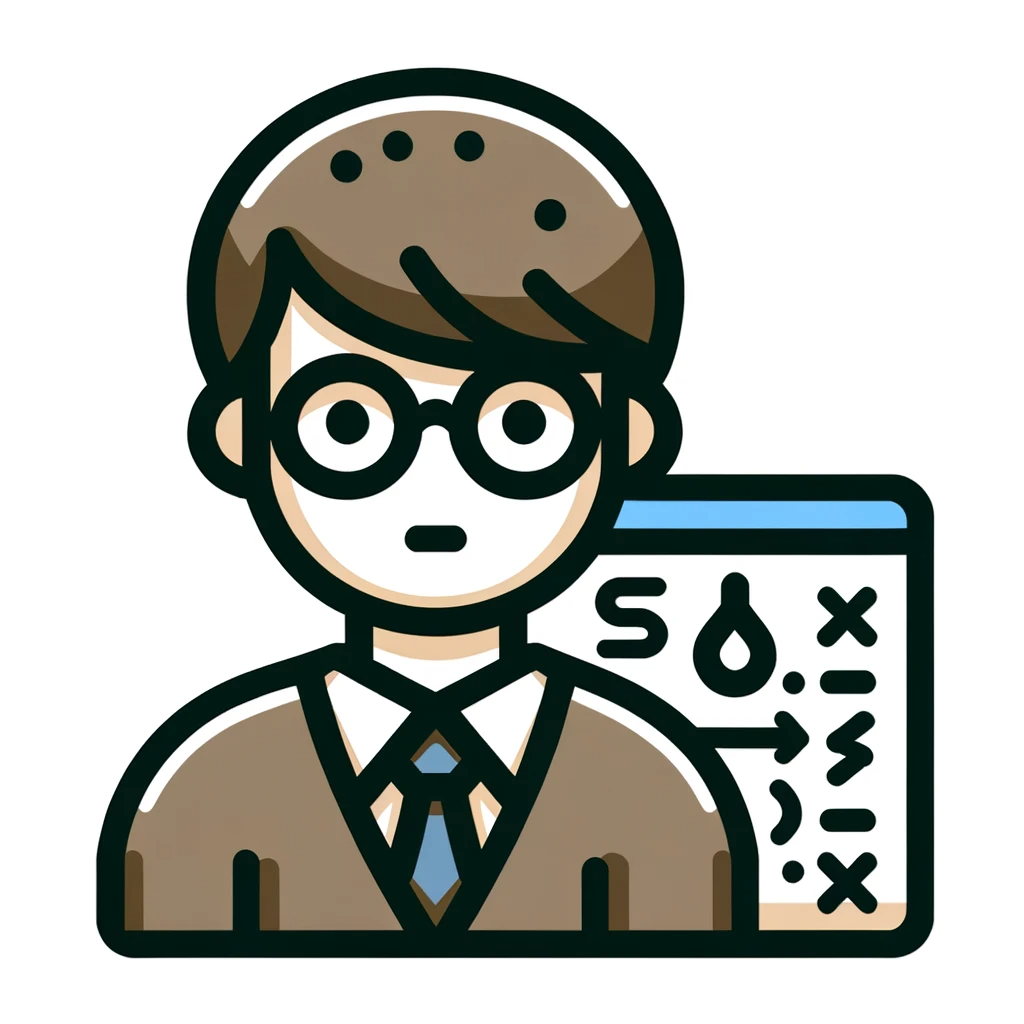
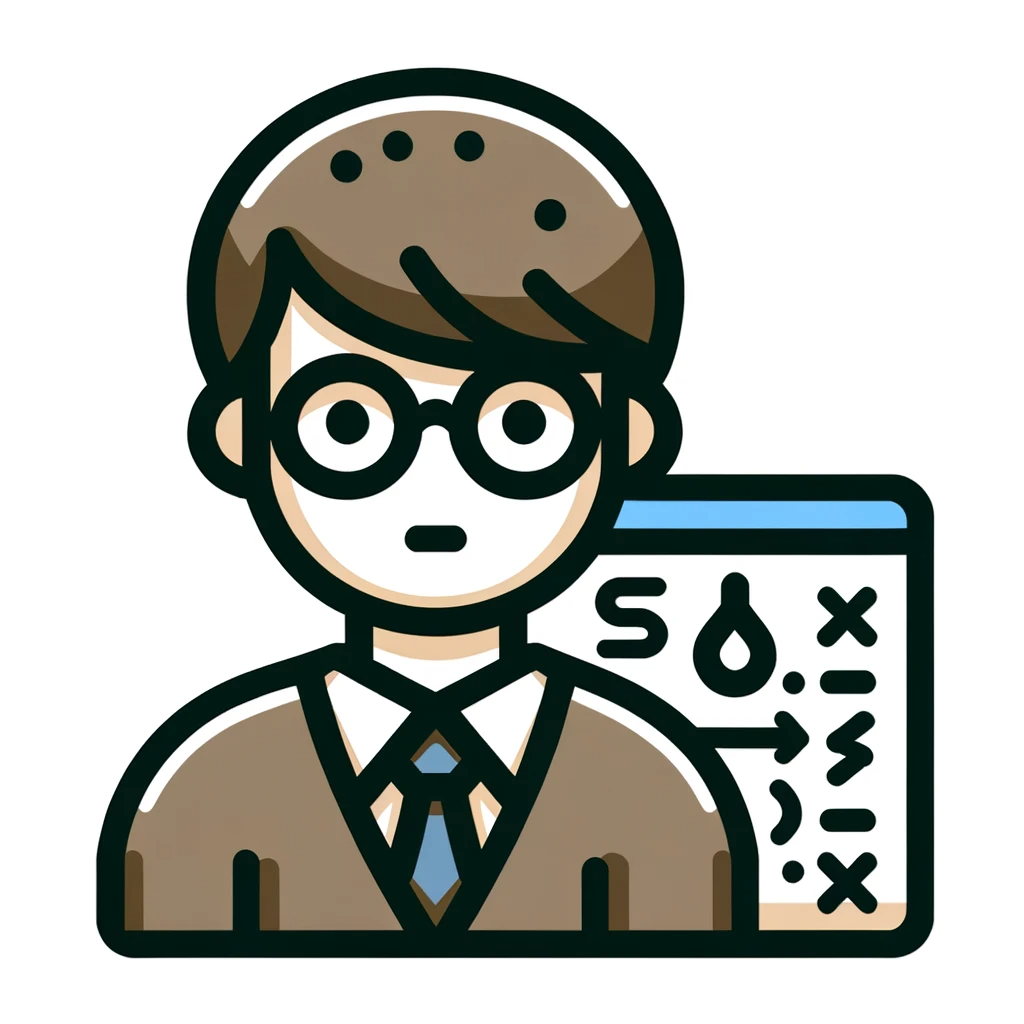
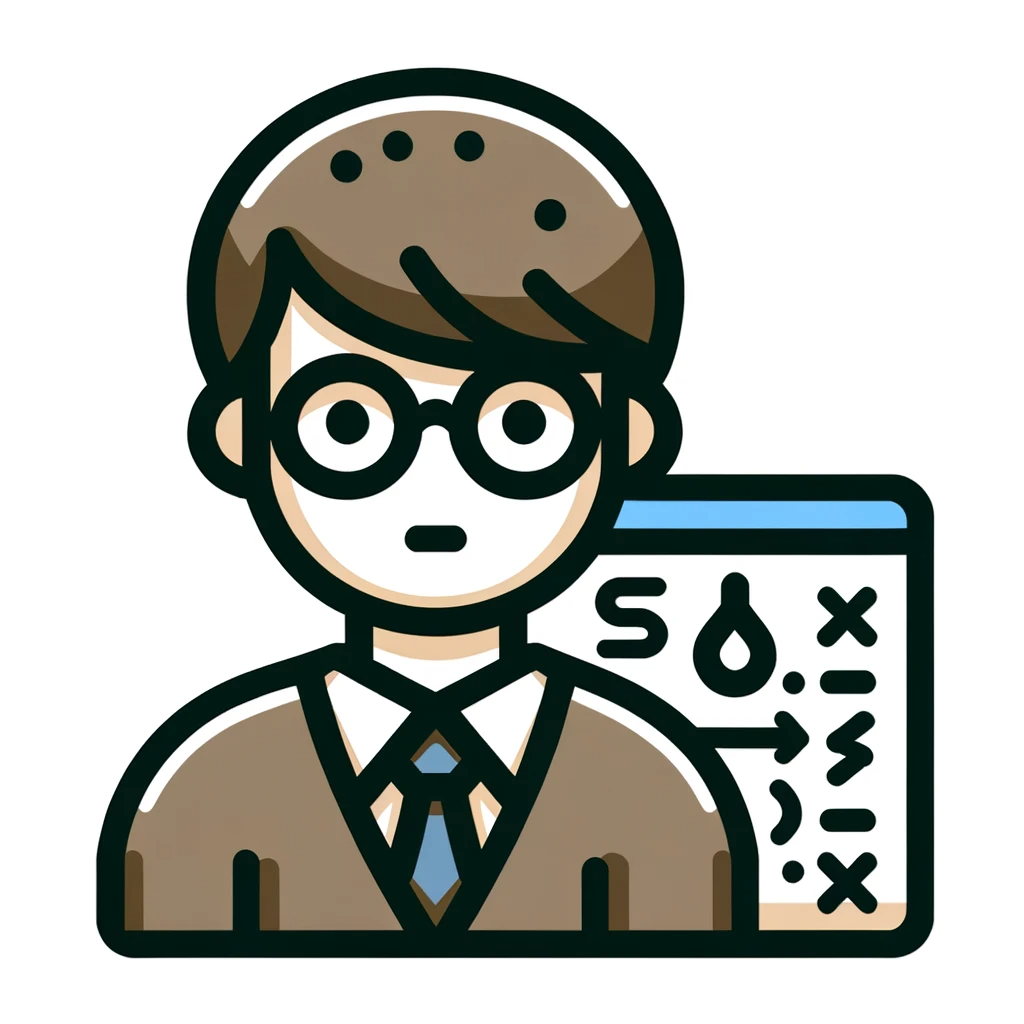
When using the filter method, be careful to appropriately set this used in the function that describes the extraction conditions.
By specifying thisValue, it will be used as this in the criteria function, allowing you to write more concise code.
Comments