This section explains in detail how to use JavaScript to obtain information about a file selected with an HTML input element.
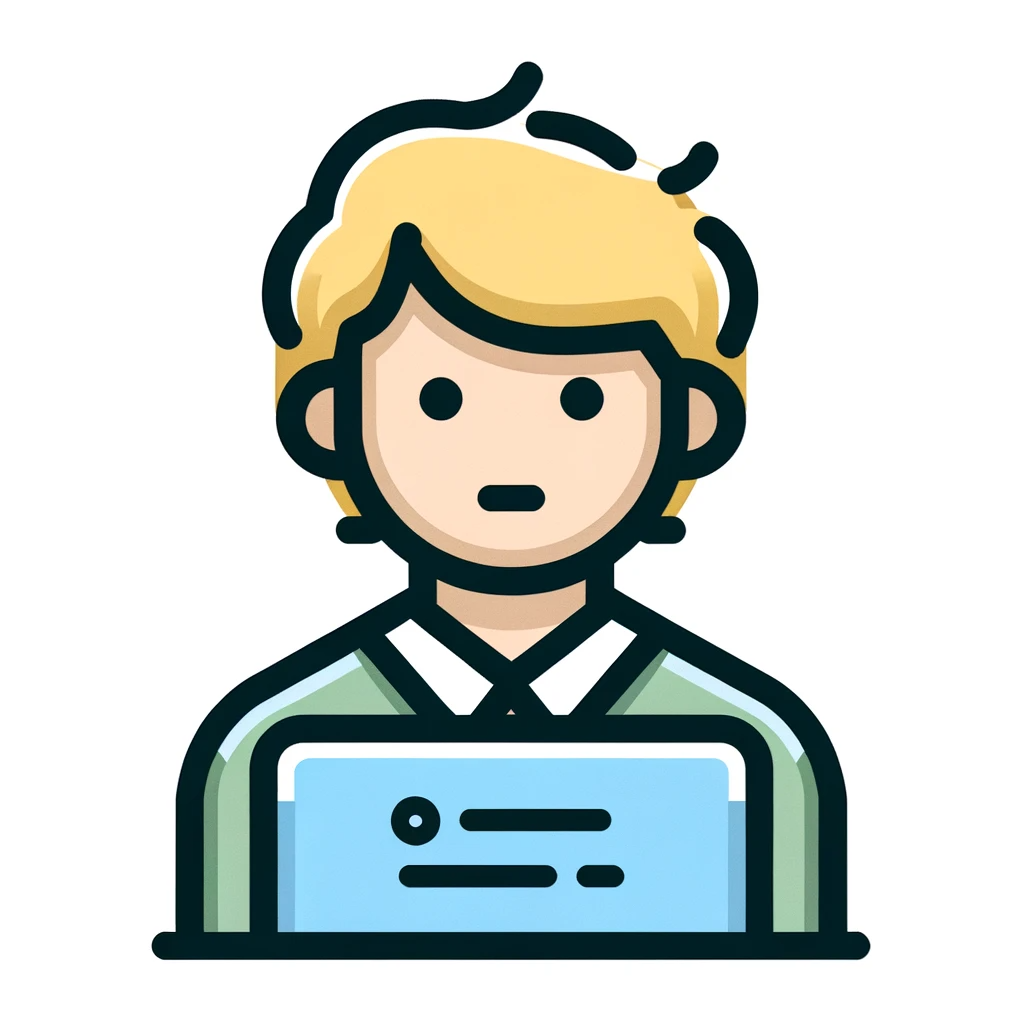
How can I get information about the selected file in an HTML input element?
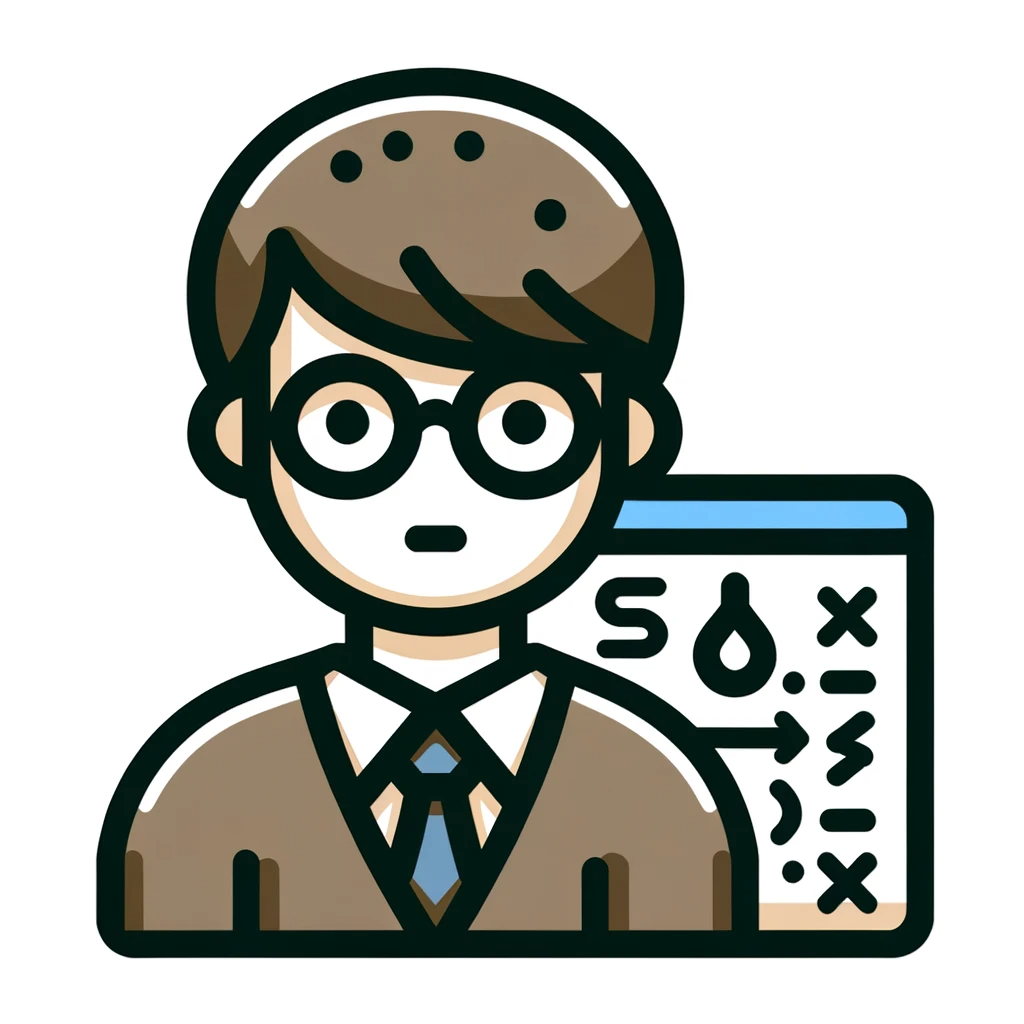
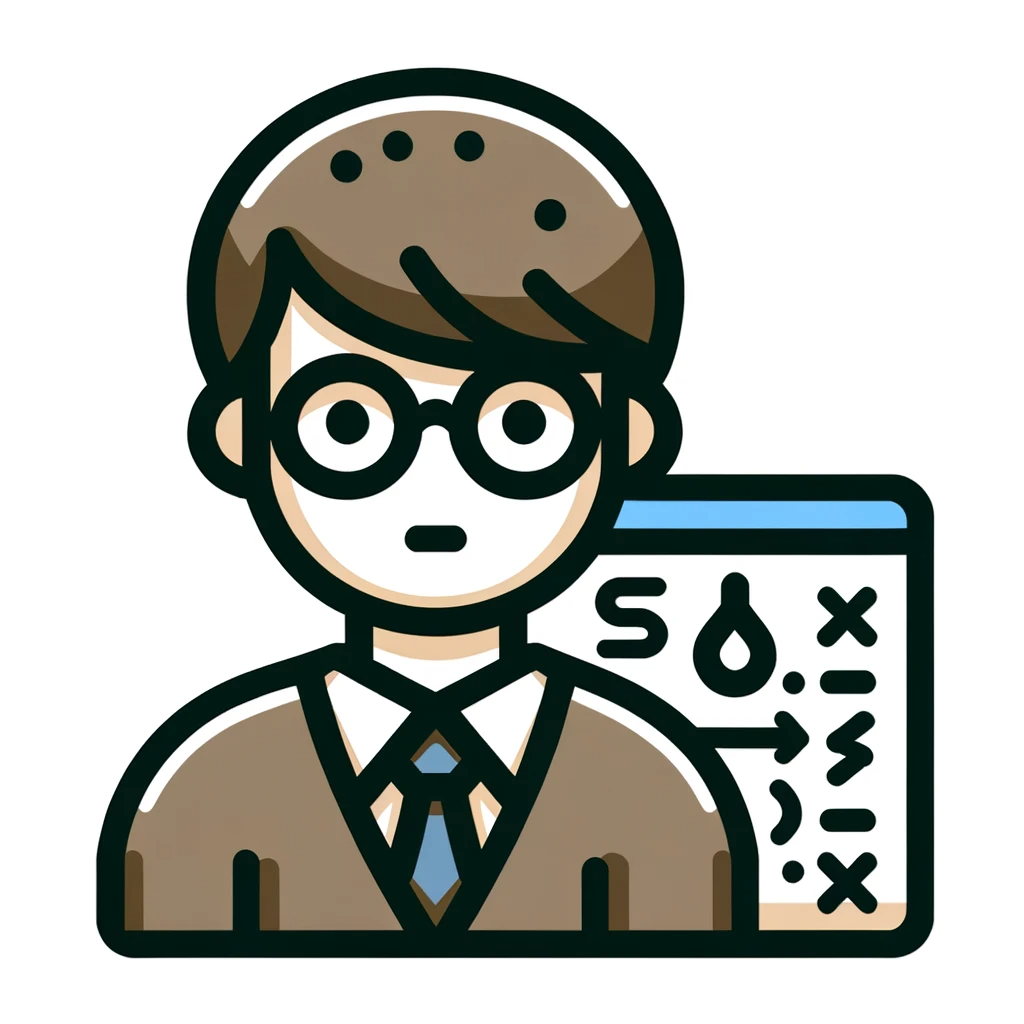
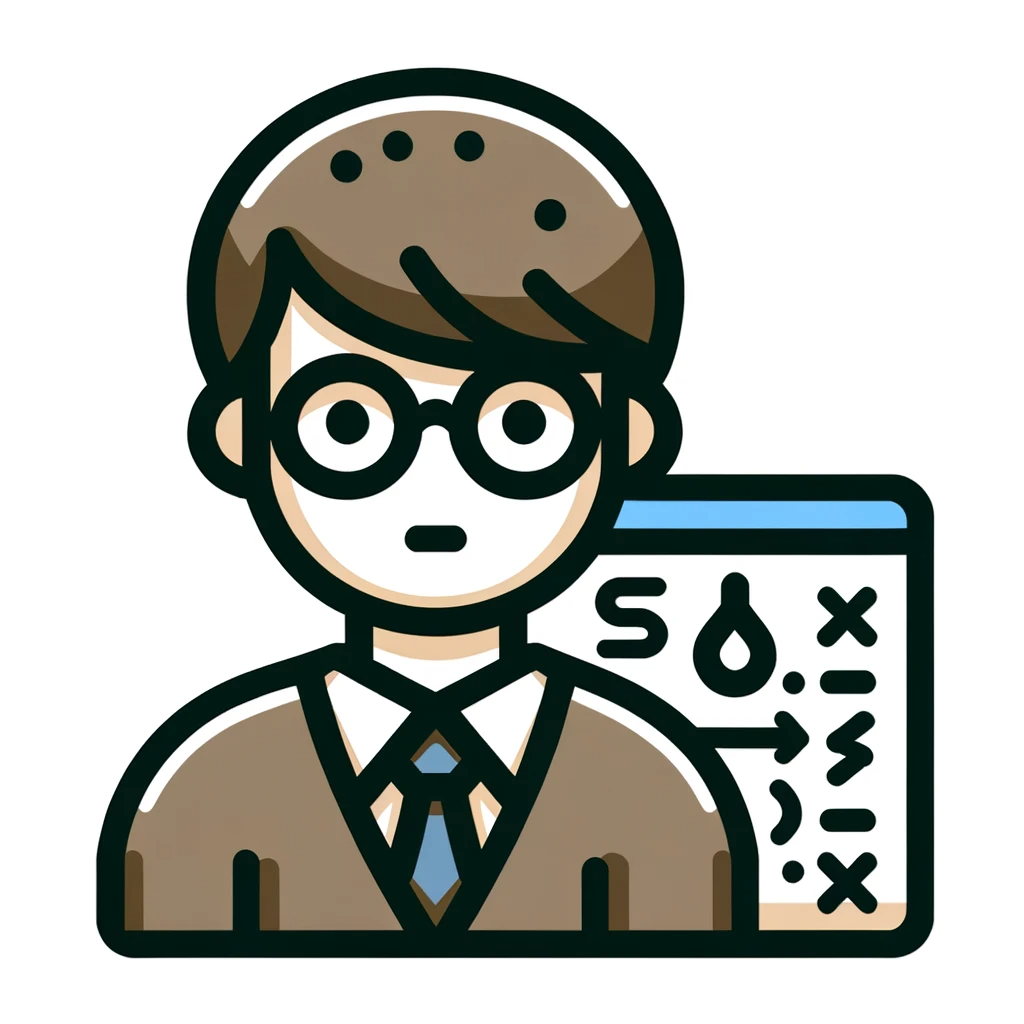
It can be obtained using the file property.
How to obtain file information using the file property
The file property is a JavaScript property for manipulating the information of the file object selected in the HTML input element.
To obtain file information using the file property, obtain the selected file object from the files property of the input element , and use the file property to obtain the file information.
Sample to get file information
The following is a sample program that retrieves information about the selected file from the input element.
<input type="file" id="fileInput">
<script>
const input = document.getElementById("fileInput");
input.addEventListener("change", function() {
const file = input.files[0];
console.log("File name: ", file.name);
console.log("File size: ", file.size + "bytes");
console.log("File type: ", file.type);
});
</script>
This sample program uses the HTML input element to select a file and retrieve information about that file.
An input element is created in HTML and the id attribute is set to “fileInput”. This input element has “file” specified in the type attribute. This provides an interface for the user to select files.
First, we are getting the input element. This is document.getElementById("fileInput")
accomplished with. This allows you to configure event listeners that you add later.
After that, we add a “change” event listener to the input element. This event occurs when the user selects a file. Inside the listener function, information about the selected file is obtained from the files property of the input element. files[0]
By doing this, you can get information about the first selected file.
Information about the retrieved files is retrieved from each property. I am getting name
the file name from the property, size
the file size (in bytes) from the property, and the file type from the property. type
Finally, the values obtained from each property are output to the log using the console.log function.
Summary of this article
We explained how to obtain information about the file selected using the HTML input element.
- The file property is a JavaScript property for manipulating the information of the file selected in the HTML input element.
files
Get the selected file object from the properties of the input element , andfile
use the properties to get the file information.file
Properties store information such as file name, size, and type.
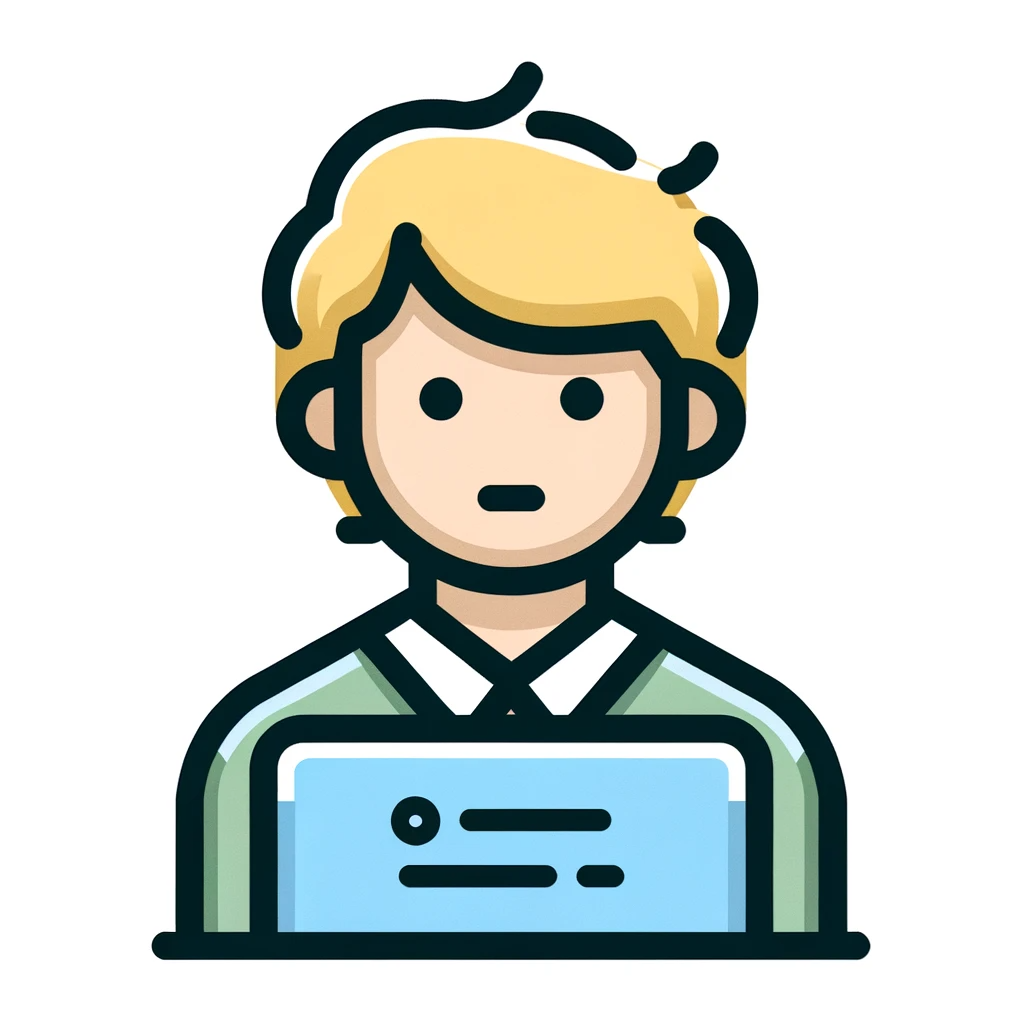
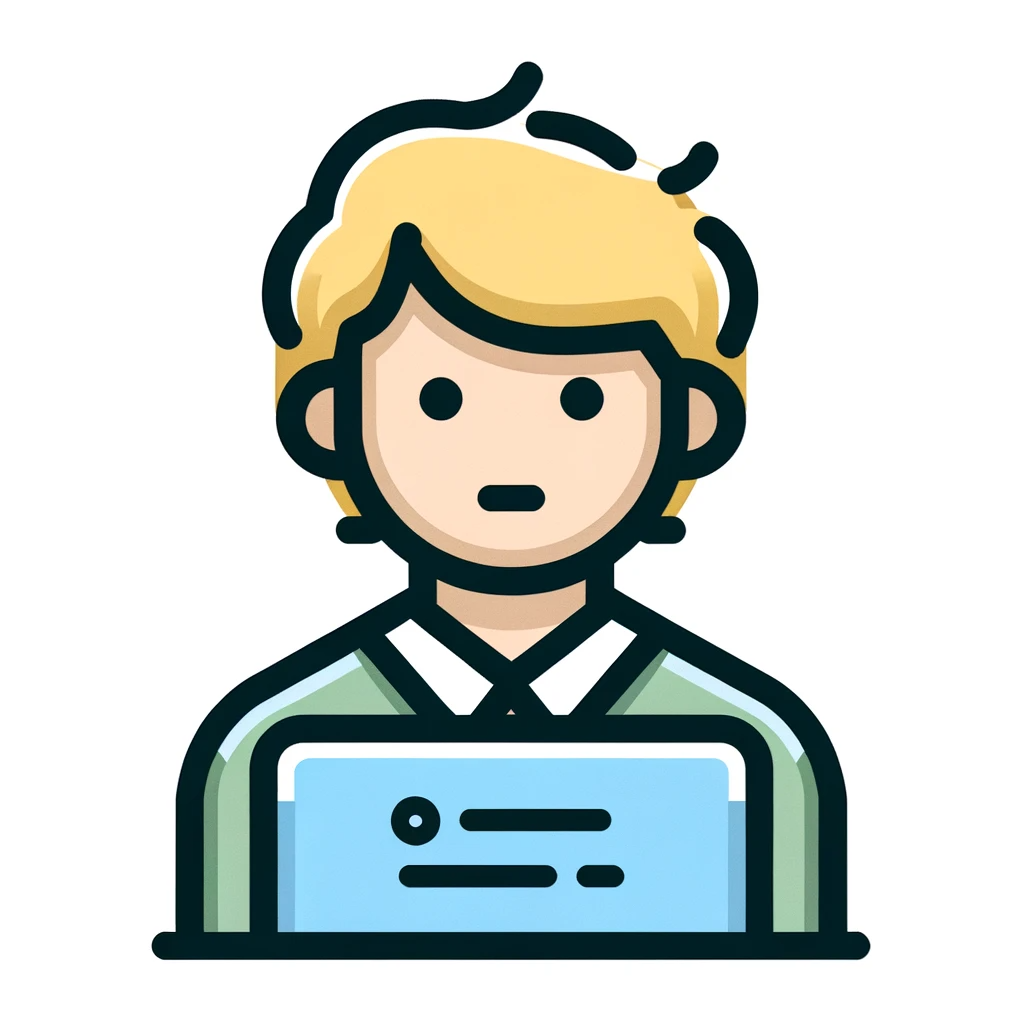
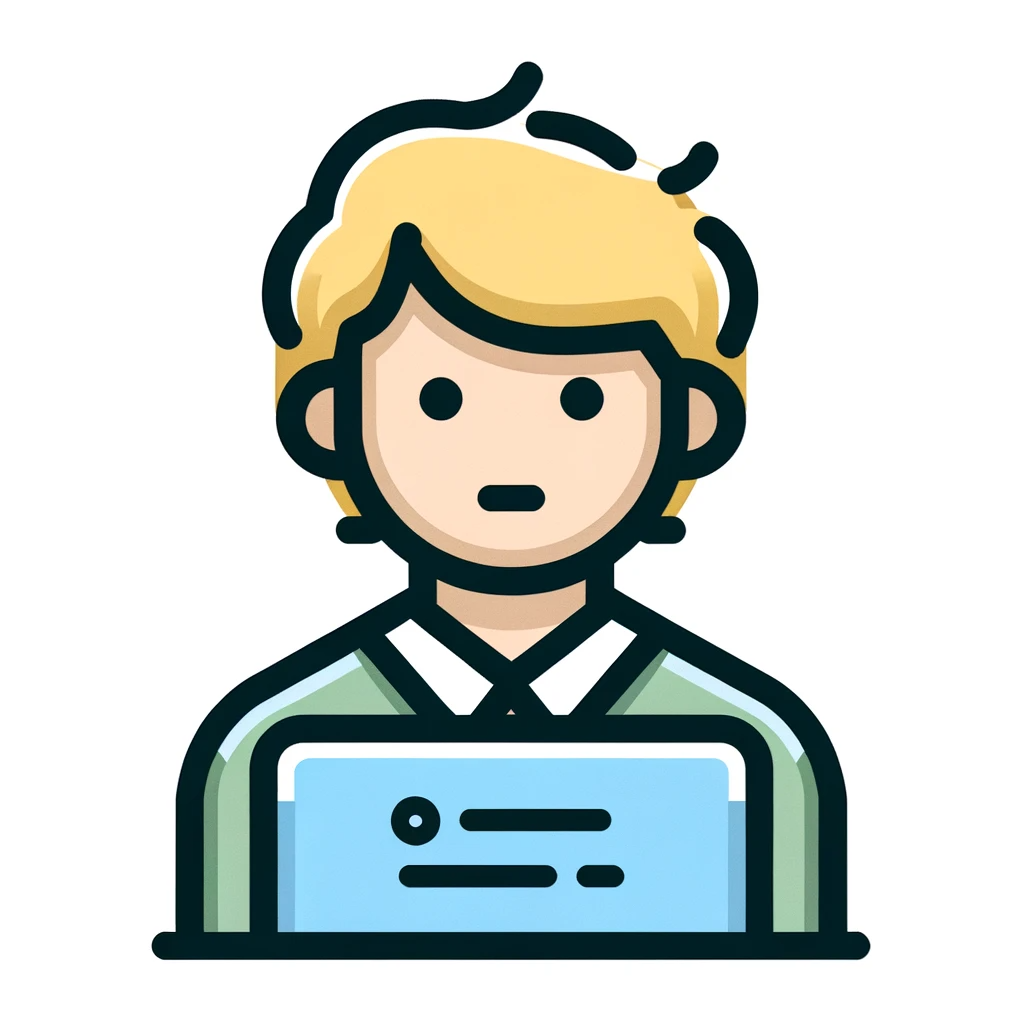
The method using the file property is simple and easy to remember!
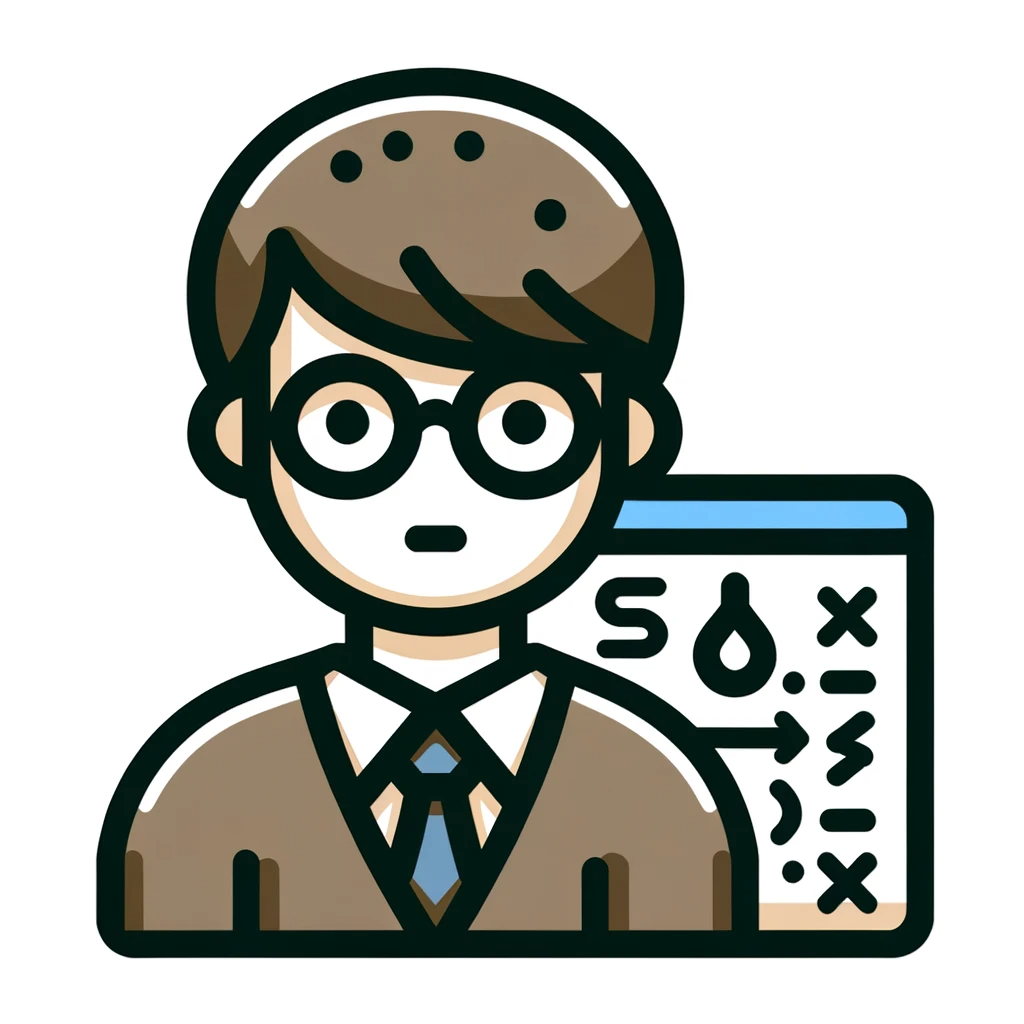
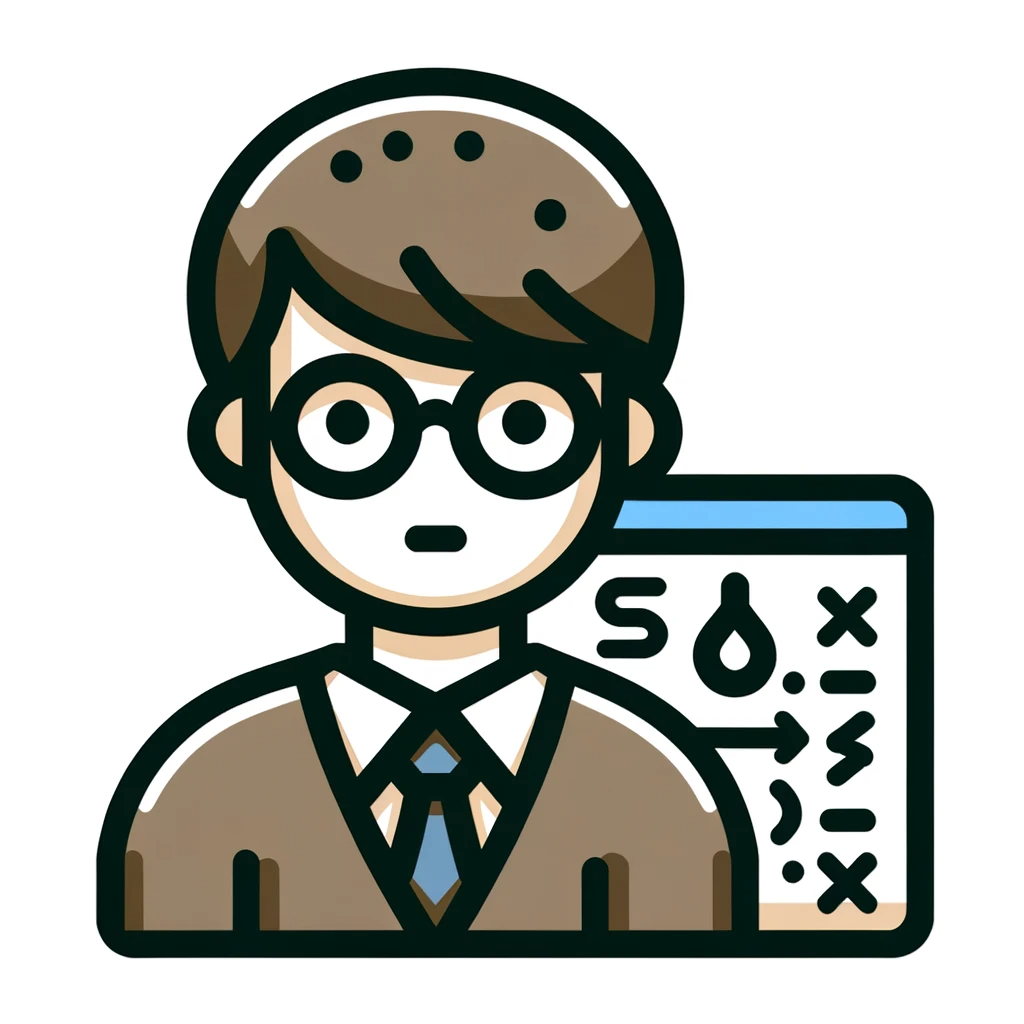
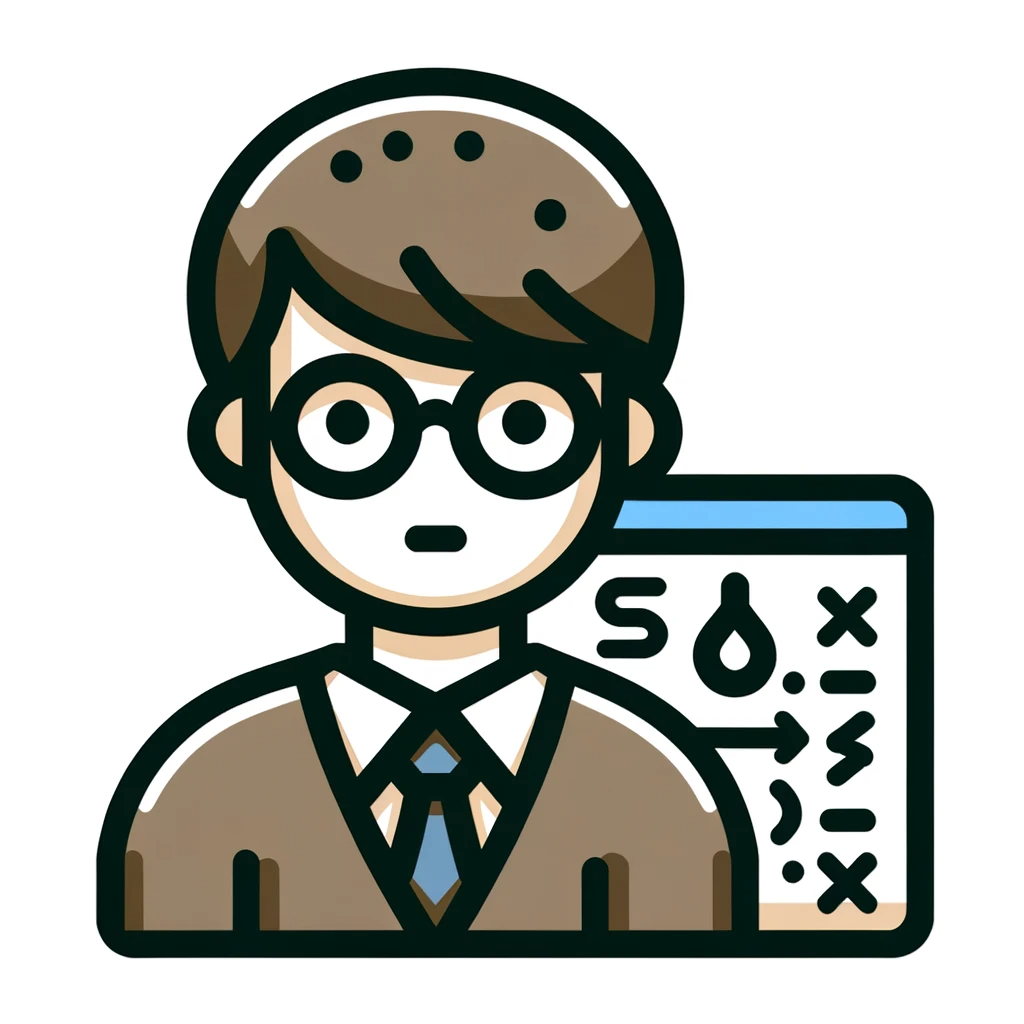
By using the “change” event of the input element, you can perform processing when the user selects a file.
This can be used in the same way as an input form, so it is a very useful technique in web application development.
Comments