We will explain in detail how to inherit classes using extends in JavaScript.
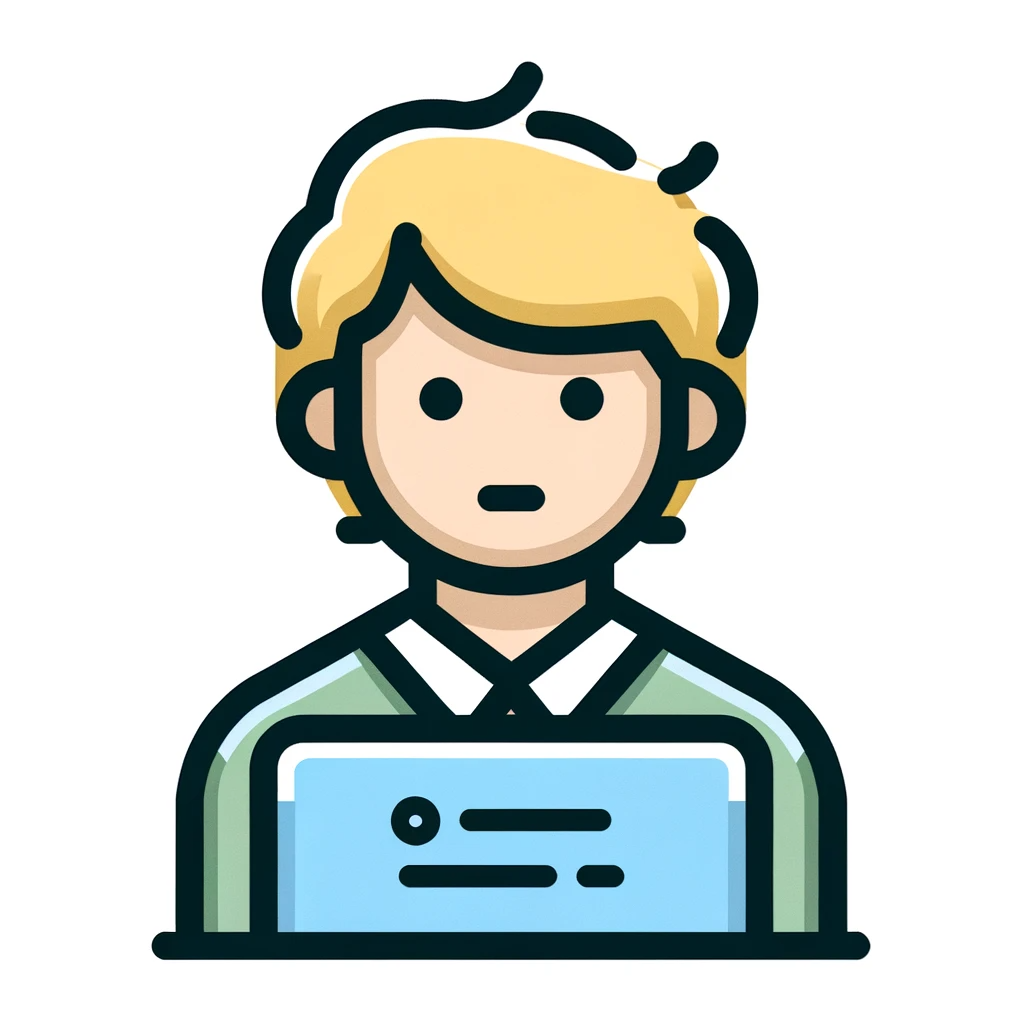
I don’t really understand how to inherit classes, so I’d like some help!
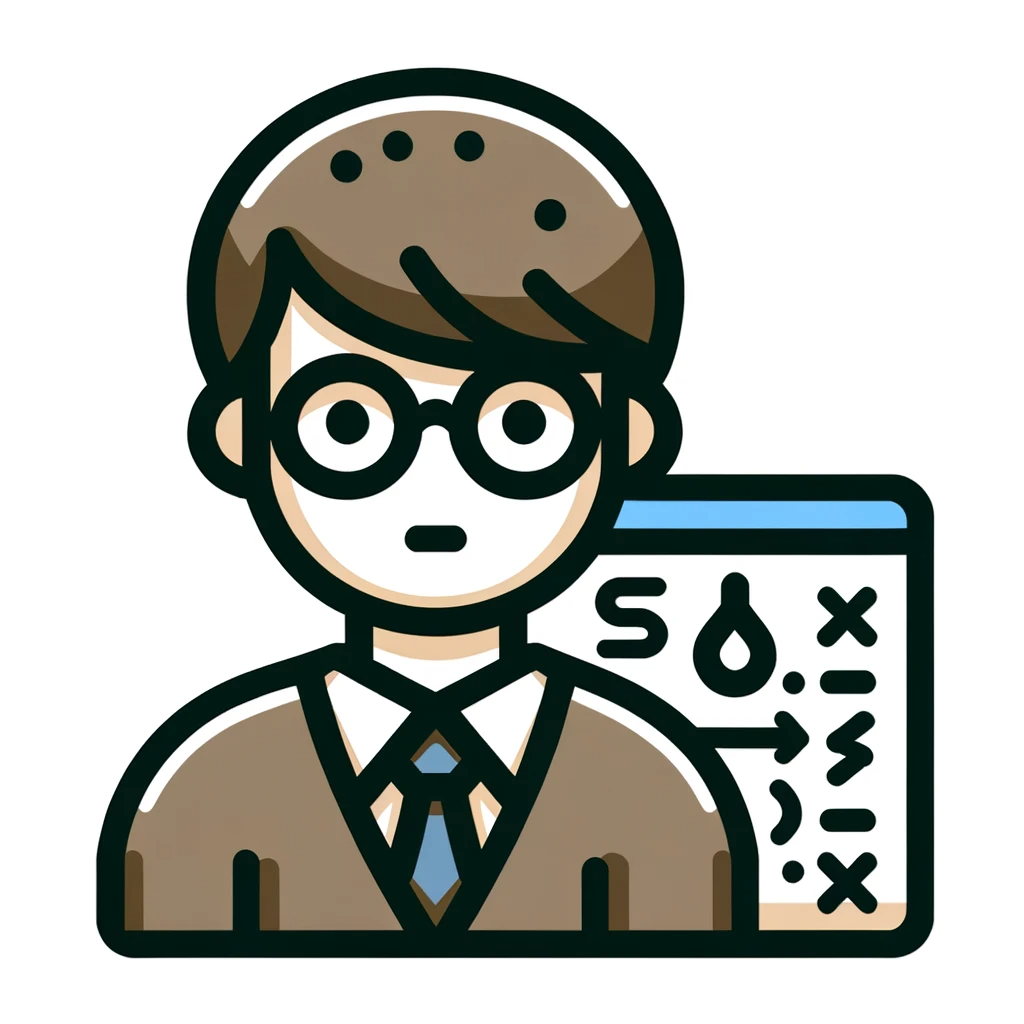
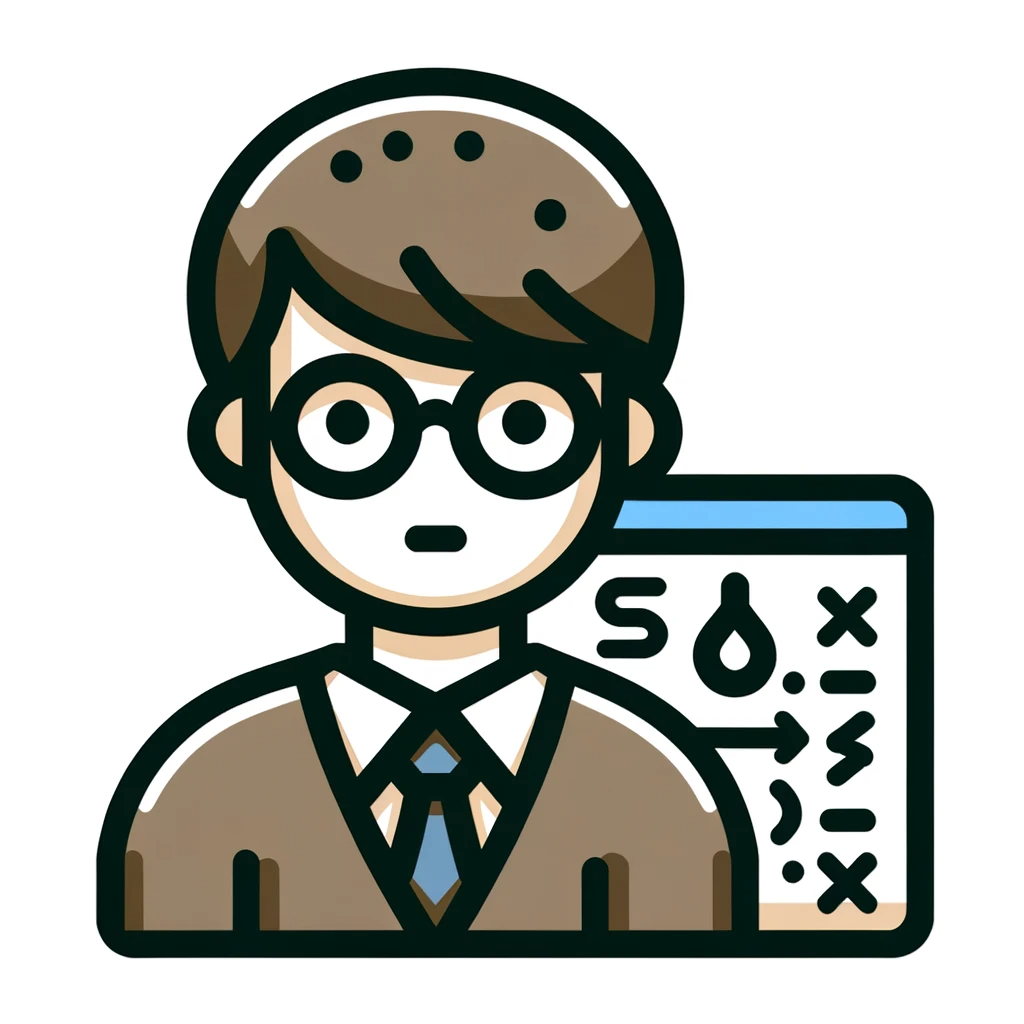
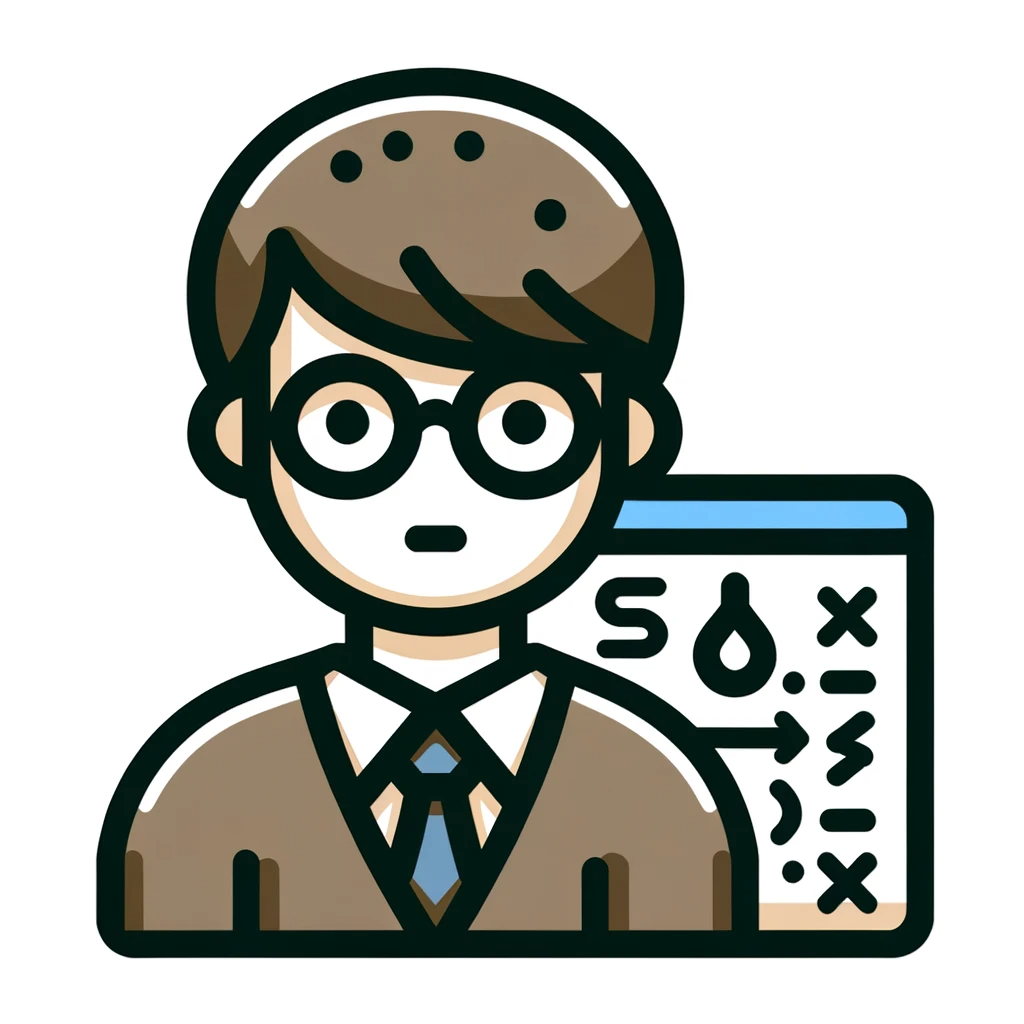
of course.
Let’s explain in detail how to inherit classes in JavaScript.
Basic concepts in class inheritance
Understanding the following concepts will deepen your understanding of class inheritance in JavaScript.
prototype chain
A prototype chain is a mechanism that represents the inheritance relationship of objects. Each object has a reference to its parent object that you can follow to find the information you need as you explore its properties and methods.
constructor method
The constructor method is a special method that is called when creating a class. This method performs the initial configuration necessary to create a new instance.
super keyword
The super keyword is used to call the functionality of the parent class. It can be used when calling parent class functionality from a child class. You can also call the parent class’s constructor method in your constructor method.
How to do class inheritance
The method for class inheritance in JavaScript is as follows.
Use the extends keyword
Starting with ES2015, when inheriting a class, you can define child classes by using the extends keyword. Define the child class as follows.
class ChildClass extends ParentClass {
// ChildClassに固有のプロパティやメソッドを定義する
}
Manipulate and inherit the prototype chain
Class inheritance can be achieved by manipulating the prototype chain. Although this method is a lower-level method than using the extends keyword, it is also used as an advanced technique in JavaScript.
In this method, an inheritance relationship is created by assigning the parent class’s prototype object to the child class’s prototype object.
Below is sample code that uses this method to inherit a class.
function ParentClass() {
// 親クラスのコンストラクタ処理
}
ParentClass.prototype.method1 = function() {
// 親クラスのメソッド1
};
function ChildClass() {
// 子クラスのコンストラクタ処理
}
ChildClass.prototype = Object.create(ParentClass.prototype);
ChildClass.prototype.constructor = ChildClass;
ChildClass.prototype.method2 = function() {
// 子クラスのメソッド2
};
Example using extend to understand inheritance
To understand inheritance, let’s look at a sample program that uses the extends keyword.
First, define the parent class. Below is the sample code for the parent class.
class ParentClass {
constructor(name) {
this.name = name;
}
sayHello() {
console.log(`Hello, I'm ${this.name}`);
}
}
Next, define a child class that inherits from this parent class. Below is the sample code for the child class.
class ChildClass extends ParentClass {
constructor(name, age) {
super(name);
this.age = age;
}
sayHello() {
console.log(`Hello, I'm ${this.name} and ${this.age} years old`);
}
}
Here, the child class uses the super keyword to call the constructor function of the parent class. In addition, the child class defines a child class-specific sayHello function by overwriting the parent class’s sayHello function.
Finally, let’s instantiate the child class and call the sayHello function.
const child = new ChildClass("John", 30);
child.sayHello(); // Hello, I'm John and 30 years old
In this way, we can easily inherit classes by using the extends keyword.
Summary of this article
We explained how to inherit a class using extends.
- To perform class inheritance in JavaScript, use the extends keyword.
- A child class that inherits from a parent class uses the super keyword to call the parent class’s constructor function.
- Inheritance is based on a mechanism called prototype chaining.
- Prototype chains allow methods and properties to be inherited from child classes to parent classes.
- A child class can define its own methods by overwriting the parent class’s methods.
- A child class inherits the properties of its parent class.
- Inheritance allows you to reuse classes that perform similar tasks, making your code more efficient.
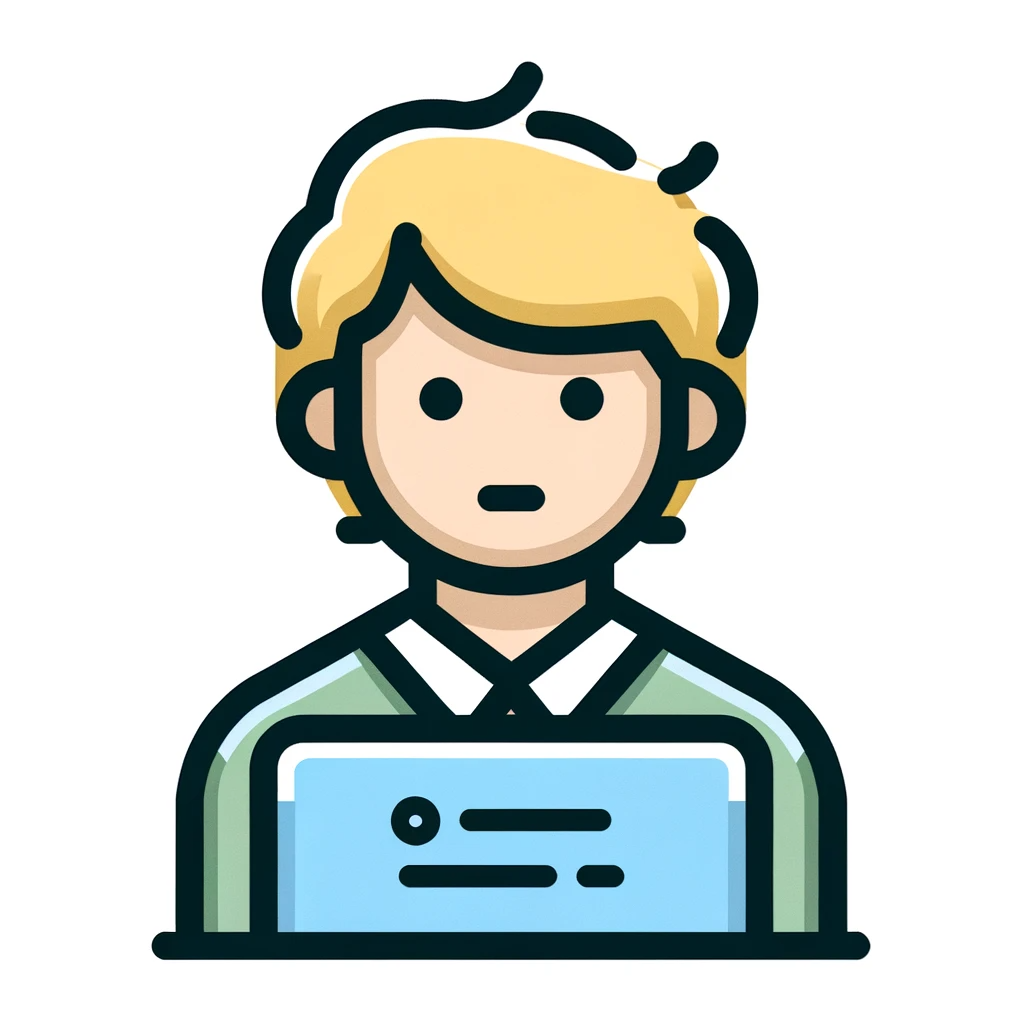
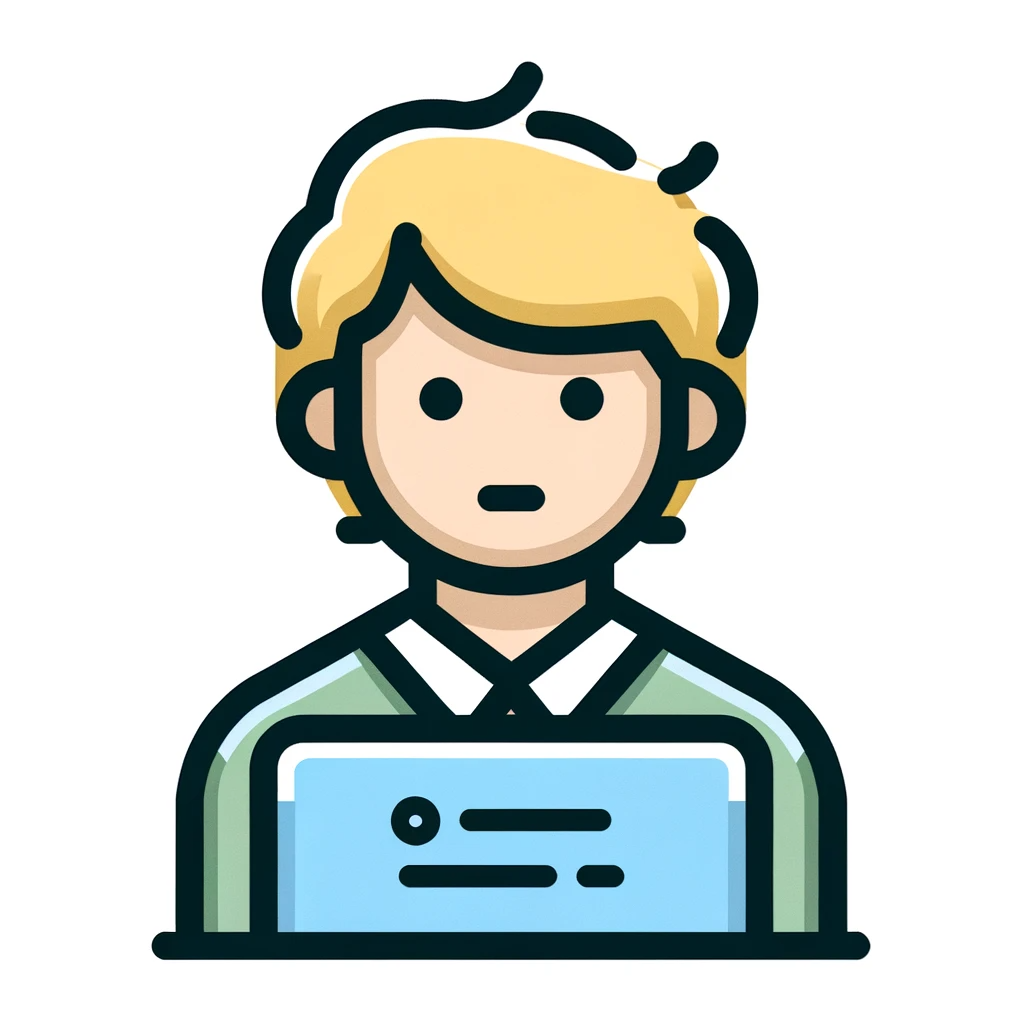
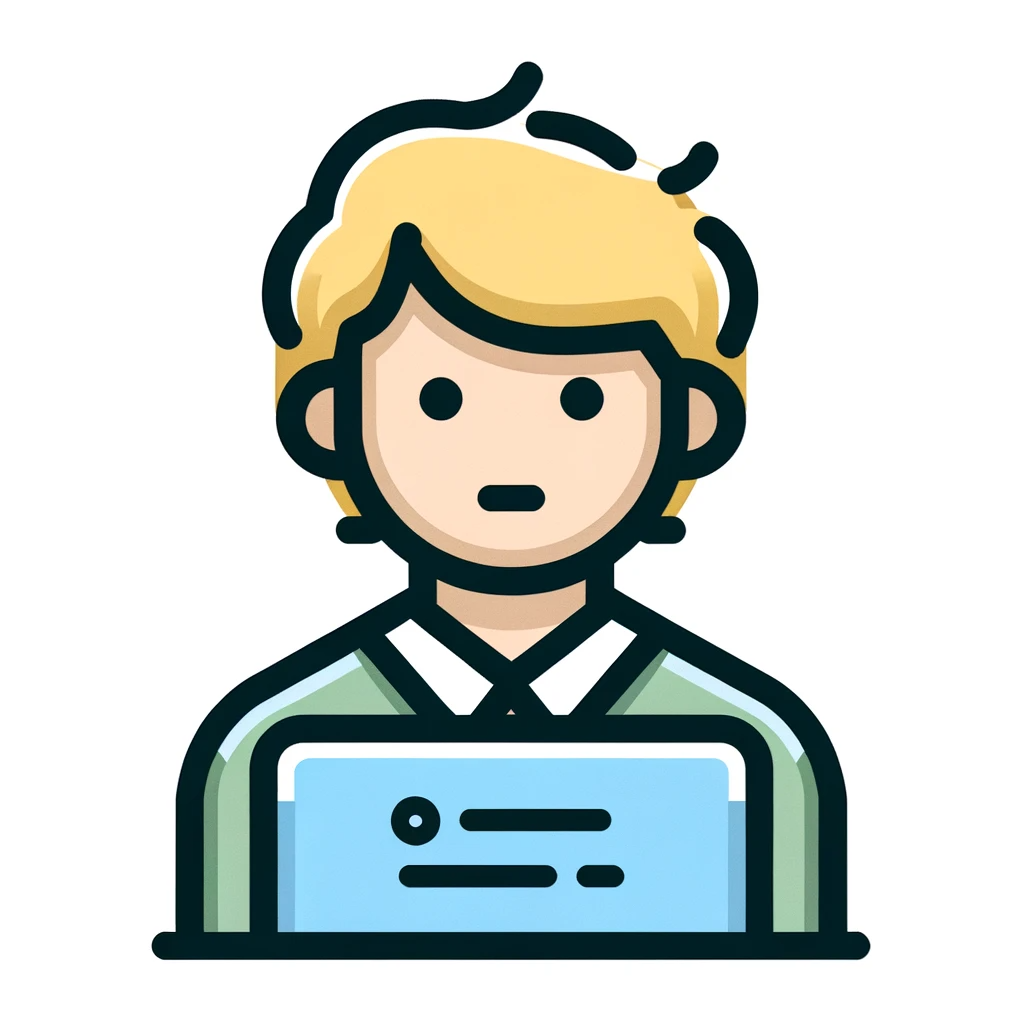
I now have a good understanding of how classes are inherited!
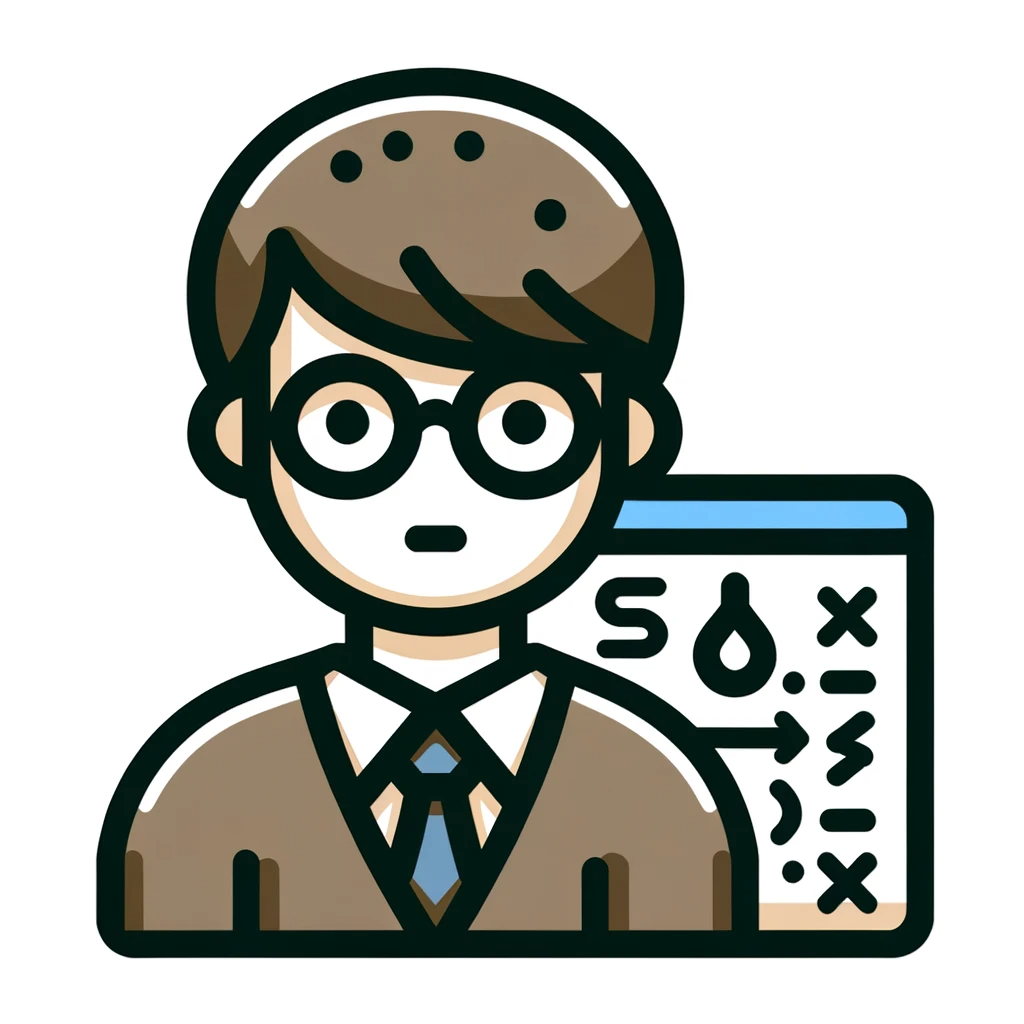
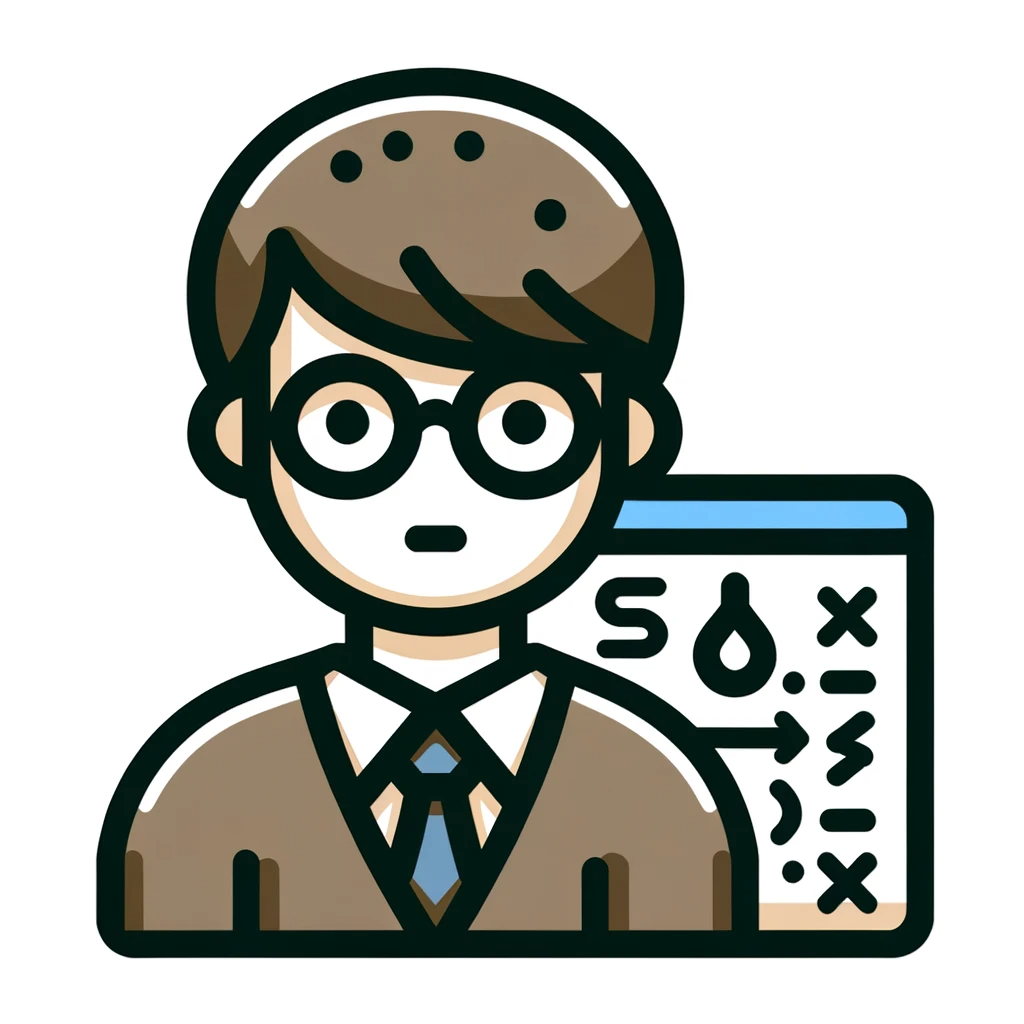
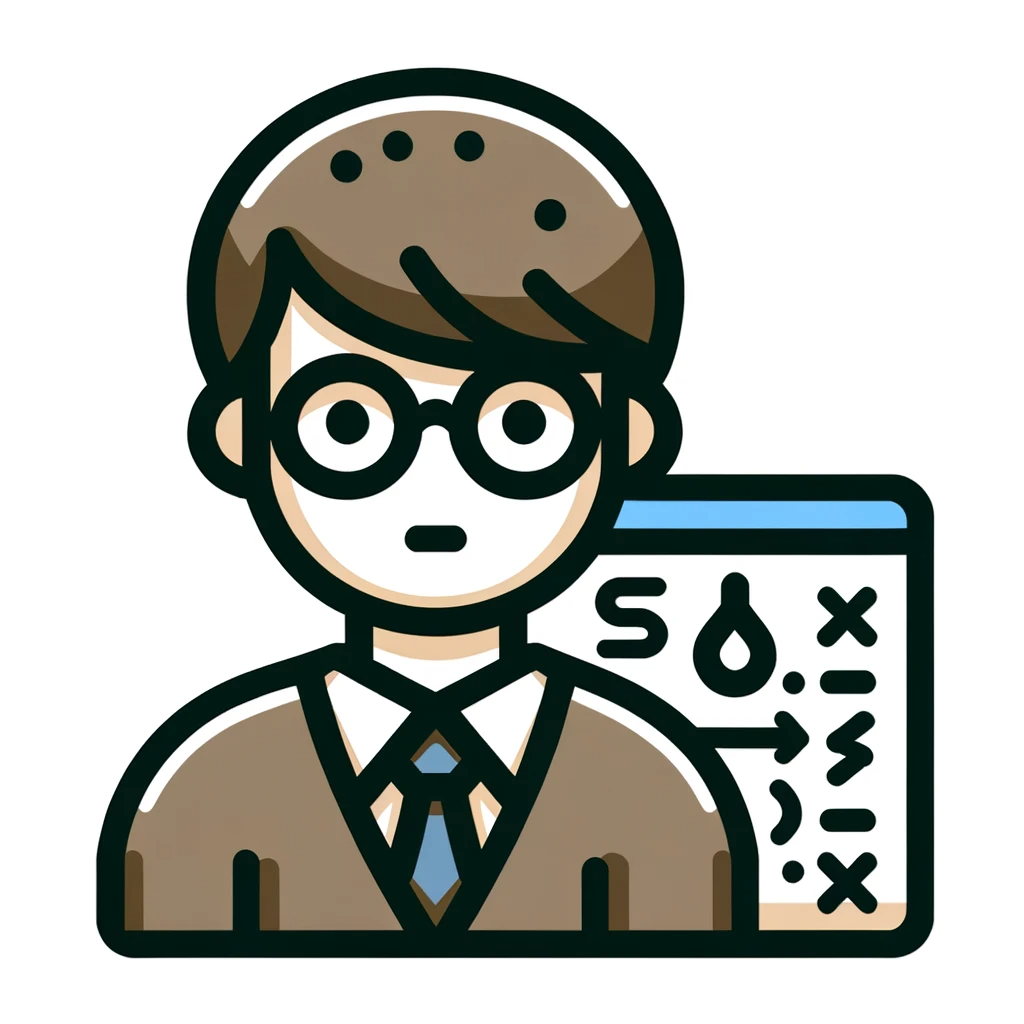
When using inheritance, be careful to inherit only the minimum amount necessary.
Proper use of inheritance is expected to improve readability and maintainability.
Comments