We will introduce explanations and sample codes that are easy for even beginners to understand about events that can be used on the browser with JavaScript.
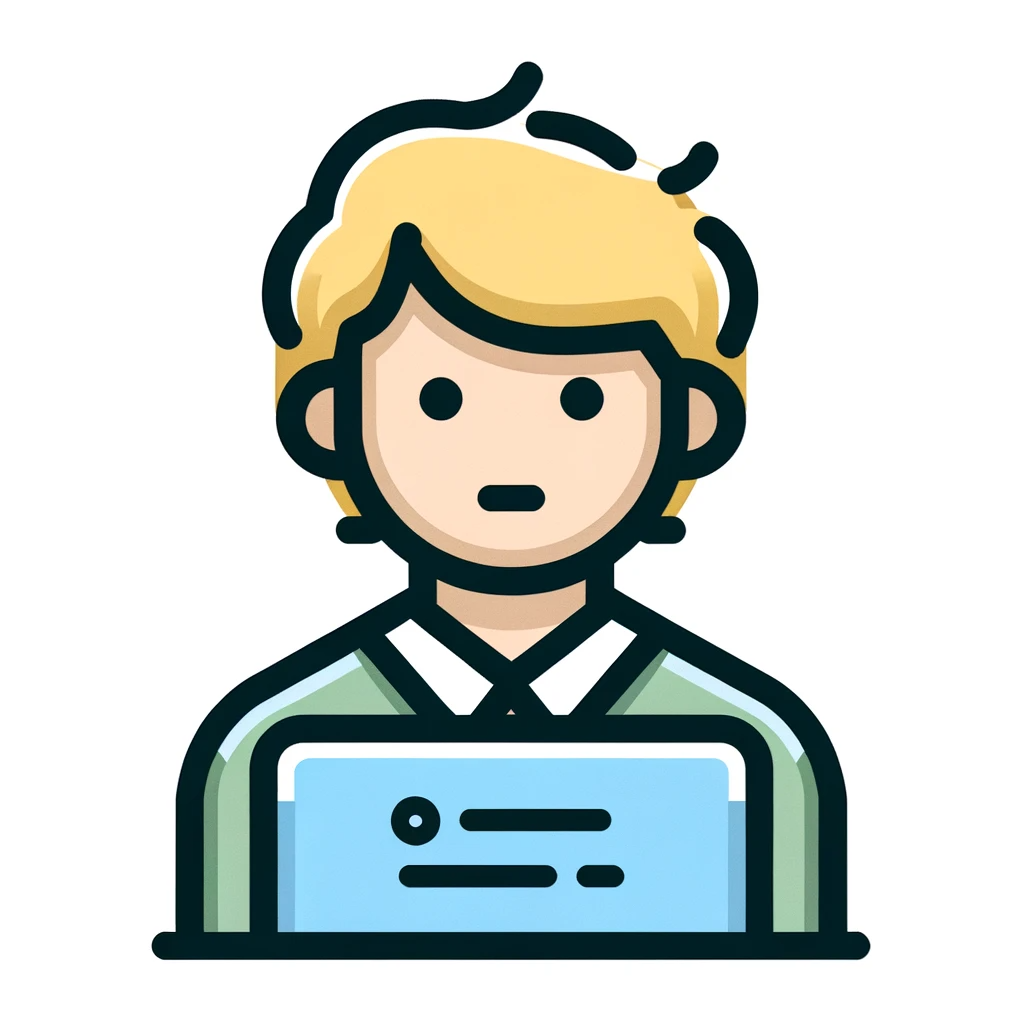
What types of JavaScript events are available on the browser?
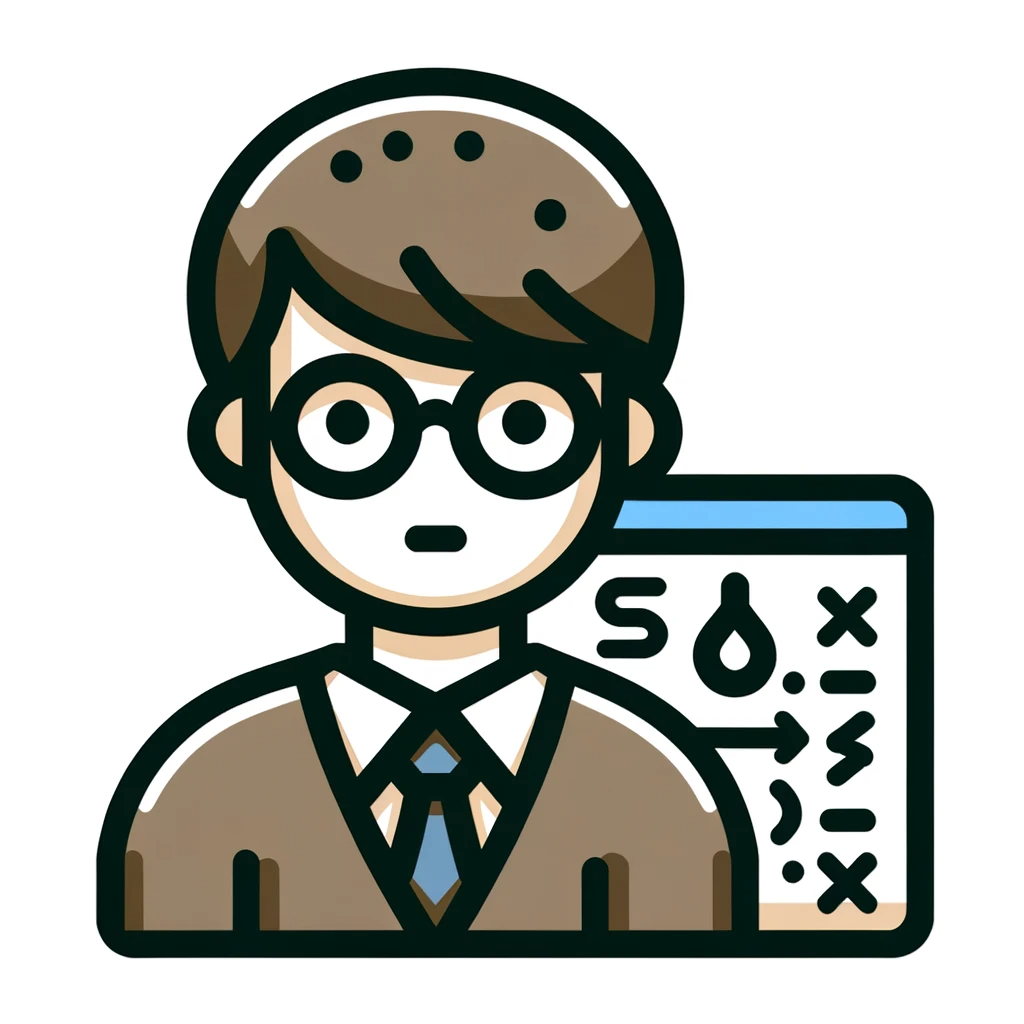
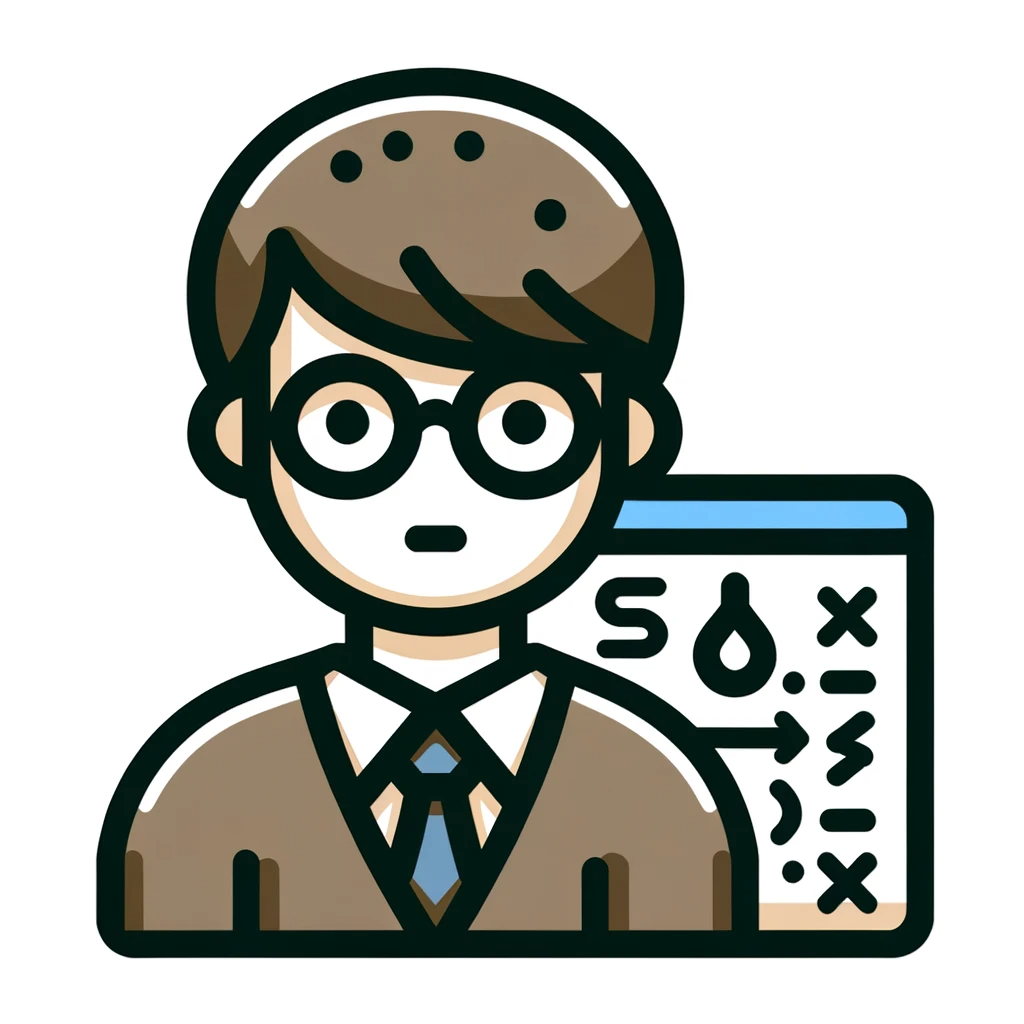
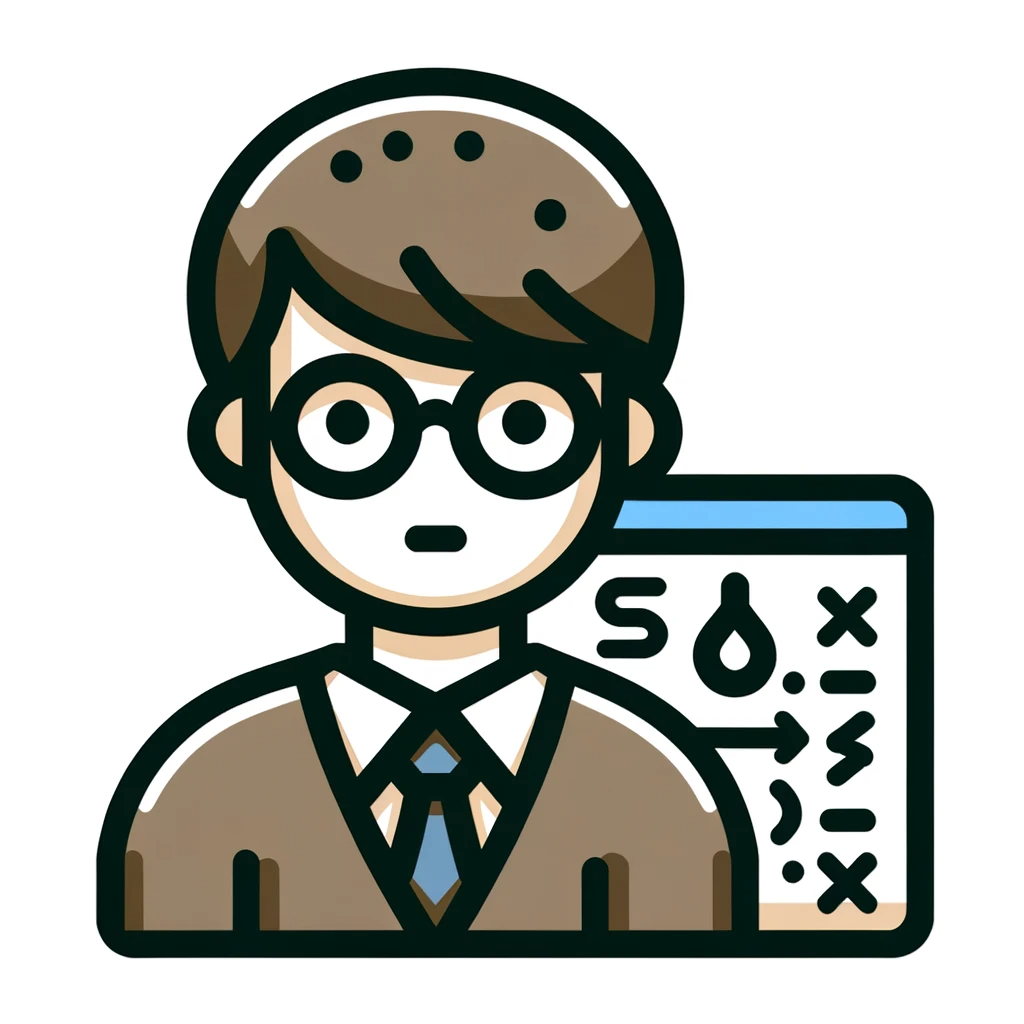
These include mouse click events, keyboard input events, and form operation events.
Summary of events available on the browser
Events occur in response to user actions or page state changes. The main events available on the browser are as follows.
classification | event name | Occurrence timing |
---|---|---|
mouse | click | when a mouse button is clicked |
mouse | mouseover | when the mouse cursor hovers over an element |
mouse | mouseout | When the mouse cursor leaves the element |
mouse | mousedown | when a mouse button is pressed |
mouse | mouse up | when the mouse button is released |
mouse | mousemove | when the mouse cursor moves over an element |
mouse | mouseenter | When the mouse cursor enters the element |
mouse | mouse leave | When the mouse cursor leaves the element |
Key | key down | when a key on the keyboard is pressed |
Key | keyup | when a key on the keyboard is released |
Key | keypress | When a character is entered while a key on the keyboard is pressed |
form | submit | when the form is submitted |
form | reset | when the form is reset |
form | change | When a form value changes |
form | focus | When a form element has focus |
form | blur | When a form element loses focus |
form | focusin | When a form element is focused (with bubbling) |
form | focus out | When the focus is removed from the form element (with bubbling) |
others | load | When a page, image, etc. is loaded |
others | unload | when the page is unloaded |
others | resize | when the window is resized |
others | scroll | when scrolled |
Click event explanation and sample
A click event is an event that occurs when a user clicks an element . You can set click events using the addEventListener() method.
document.getElementById("btn").addEventListener("click", function(){
alert("button has been clicked!");
});
Explanation and sample of mouseover event
A mouseover event is an event that occurs when the mouse cursor hovers over an element . You can set mouseover events using the addEventListener() method.
document.getElementById("btn").addEventListener("mouseover", function(){
document.getElementById("btn").style.backgroundColor = "yellow";
});
In the above code, when the mouse cursor hovers over the element with id “btn”, the background color of the element will be changed to yellow.
Explanation and samples of keyboard events
A keyboard event is an event that occurs when a key on the keyboard is pressed . You can set keyboard events using the addEventListener() method.
document.addEventListener("keydown", function(event){
console.log("Key pressed! Key Code:" + event.keyCode);
});
In the above code, when a key is pressed, the console will display “Key pressed! Key code: [code of the pressed key]”.
The mouseenter and mouseleave events are explained.
mouseenter event that occurs when the mouse cursor enters an element
The mouseenter event is fired when the mouse cursor enters an element. However, it does not occur when the mouse cursor enters a child element of the element. Also, unlike the mouseover event, it does not occur when the mouse moves within the element.
The mouseenter event occurs when the mouse cursor enters an element. However, it does not occur when the mouse cursor enters a child element of the element. Also, unlike the mouseover event, it does not occur even if the mouse moves within the element.
mouseleave event fired when the mouse cursor leaves an element
The mouseleave event is fired when the mouse cursor leaves an element. It is also fired when the mouse leaves a child element within an element. Unlike the mouseout event, it is not fired when the mouse moves within an element.
The mouseleave event is used to detect when a user leaves an element. For example, if you have a menu that displays submenus, you can set the mouseleave event on the main menu to hide the submenus when the mouse leaves the main menu.
sample of mouseenter and mouseleave events
Let’s check the behavior of the mouseenter and mouseleave events using sample code.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>mouse event</title>
</head>
<body>
<div id="target" style="width: 200px; height: 200px; background-color: #f00;"></div>
<script>
const target = document.getElementById('target');
target.addEventListener('mouseenter', function() {
target.style.backgroundColor = '#0f0';
});
target.addEventListener('mouseleave', function() {
target.style.backgroundColor = '#f00';
});
</script>
</body>
</html>
In the above code, the mouseenter and mouseleave events are set on a div element with an ID of target. When the mouse cursor enters the div element, the background color changes to green, and when it leaves the div element, the background color changes to red.
Difference between mouseenter/mouseleave and mouseover/mouseout
The mouseenter and mouseleave events and the mouseover and mouseout events are sometimes confused because they are similar events, but there is a difference between them.
The mouseover event is fired when the mouse cursor hovers over an element. Each time the mouse moves within an element, the mouseover event is fired even if the cursor hovers over a child element within the element.
The mouseout event is fired when the mouse cursor leaves the element. The mouseout event is fired even if the mouse moves from within the element to a child element.
On the other hand, the mouseenter event is fired when the mouse cursor hovers over an element. Even if the cursor hovers over a child element within an element each time it moves within the element, the mouseenter event is not fired.
Similarly, the mouseleave event is fired when the mouse cursor leaves the element. The mouseleave event is also fired when the mouse moves from within an element to a child element.
In other words, the mouseover and mouseout events fire even when the mouse cursor is over a child element within an element, while the mouseenter and mouseleave events do not fire when the mouse cursor is over a child element within an element. The difference is that the mouseenter and mouseleave events do not fire when the mouse cursor is over a child element within an element.
Difference between focus/blur and focusin/focusout
Form elements have two states: focused and unfocused. Focused means that keyboard and mouse actions are applied directly to the form element. A form element that is in focus is often in a state that can be manipulated by the user; for example, to enter text in a text box, it must first be in focus.
The focus and blur events are used to detect the focused and unfocused states. These events are fired when a form element gains or loses focus.
On the other hand, the focusin and focusout events are similar to the focus and blur events, but with a difference. Unlike the focus and blur events, the focusin and focusout events respond to focus movement from a parent element to a child element when the elements are nested.
For example, if HTML elements are nested as shown below, the focus event is fired when the child element has the focus, but the focusin event is fired even when the parent element has the focus.
<div id="parent">
<input type="text" id="child">
</div>
In other words, the difference is that the focus and blur events are events that react to the focus movement of a form element, while the focusin and focusout events are events that also react to the focus movement of elements in a parent-child relationship.
To confirm the above differences, try executing the sample code below and you will see that the timing at which the focus and blur events and the focusin and focusout events are fired is different.
summary
I explained the events that can be used on the browser with JavaScript.
- Events occur in response to user actions or page state changes.
- Mouse events include click, mousedown, mouseup, mousemove, mouseenter, mouseleave, etc.
- Keyboard events include keydown, keyup, and keypress.
- Form events include submit, reset, focus, blur, change, and select.
- Other events include load, unload, resize, and scroll.
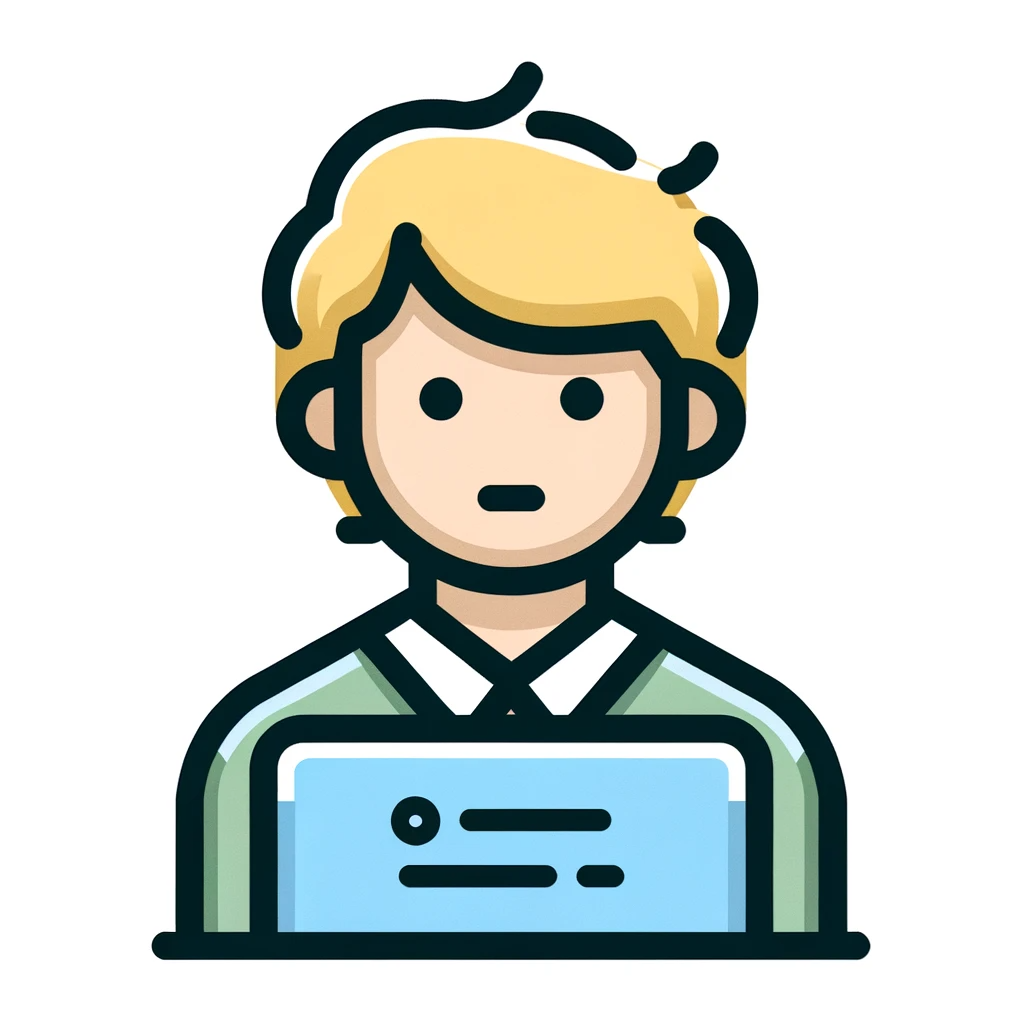
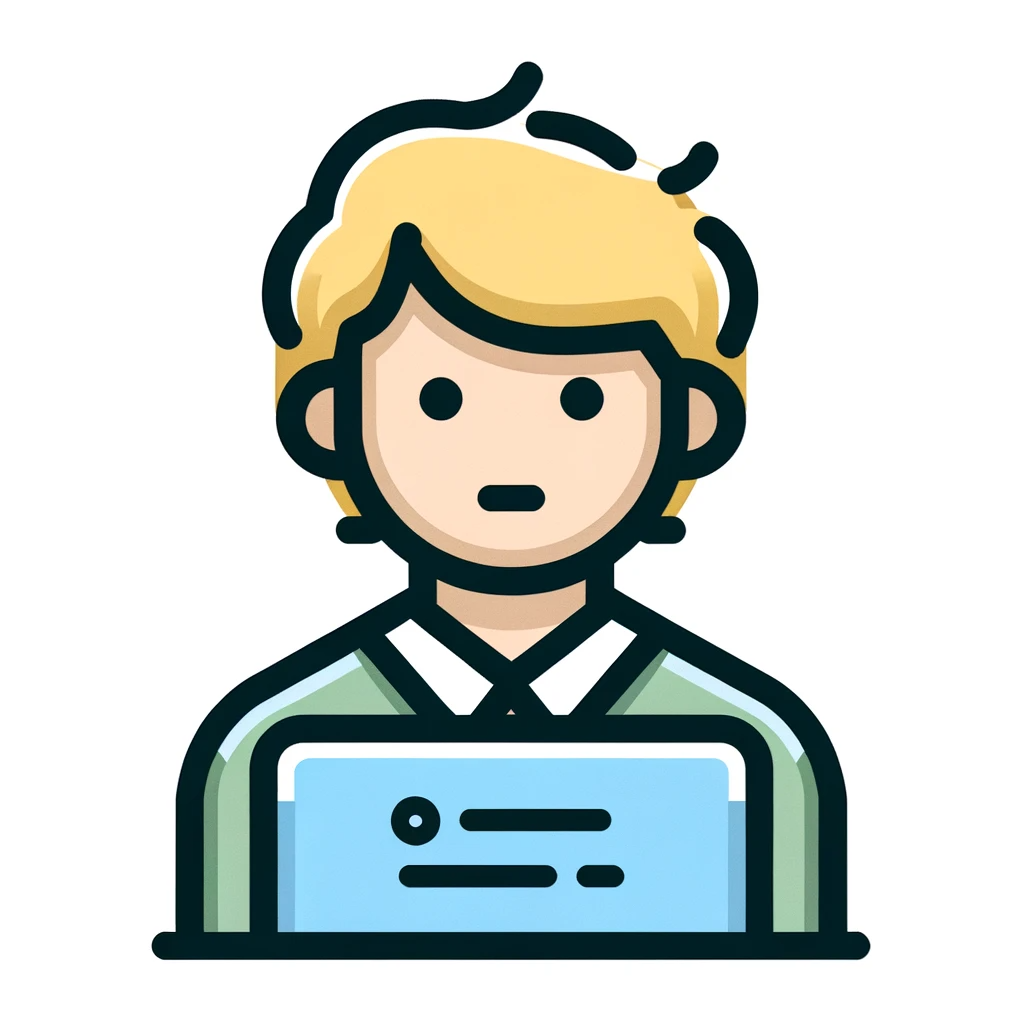
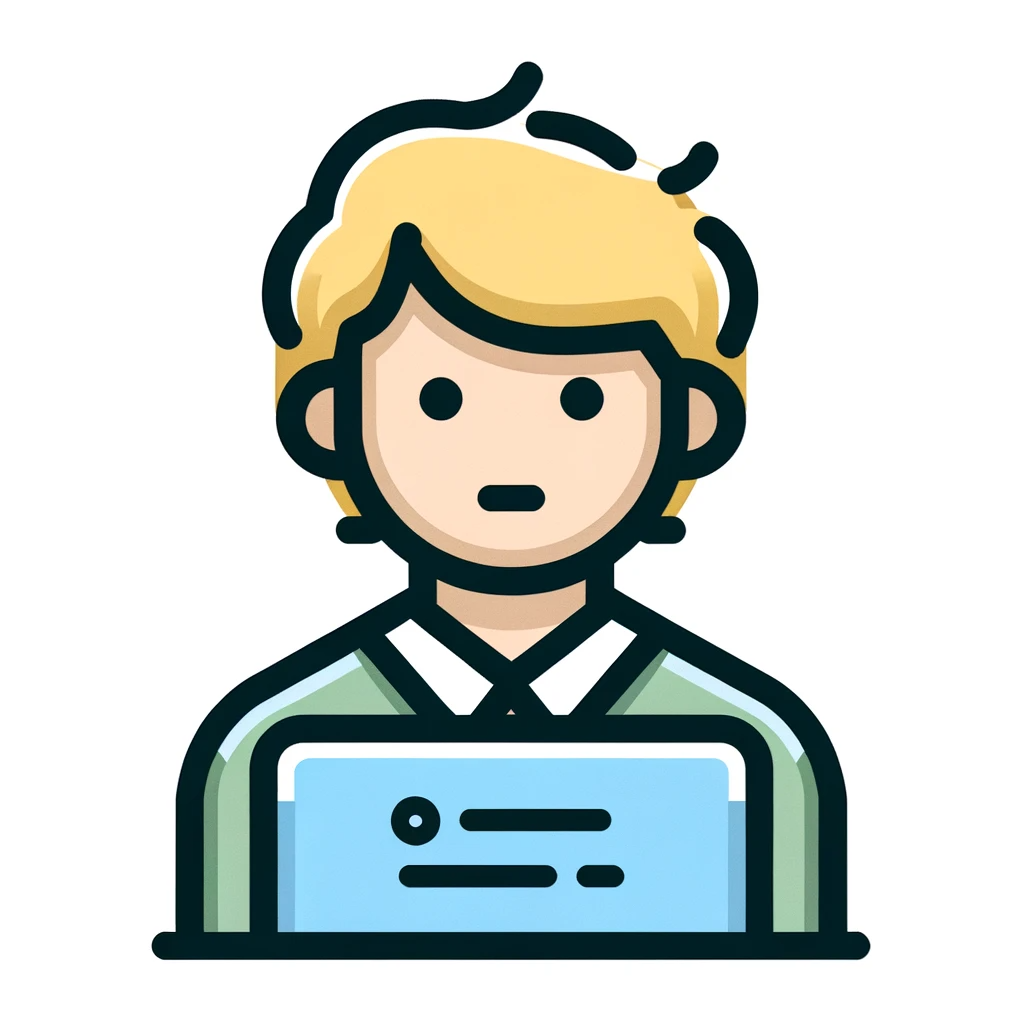
I now understand what kind of events there are and how to use them!
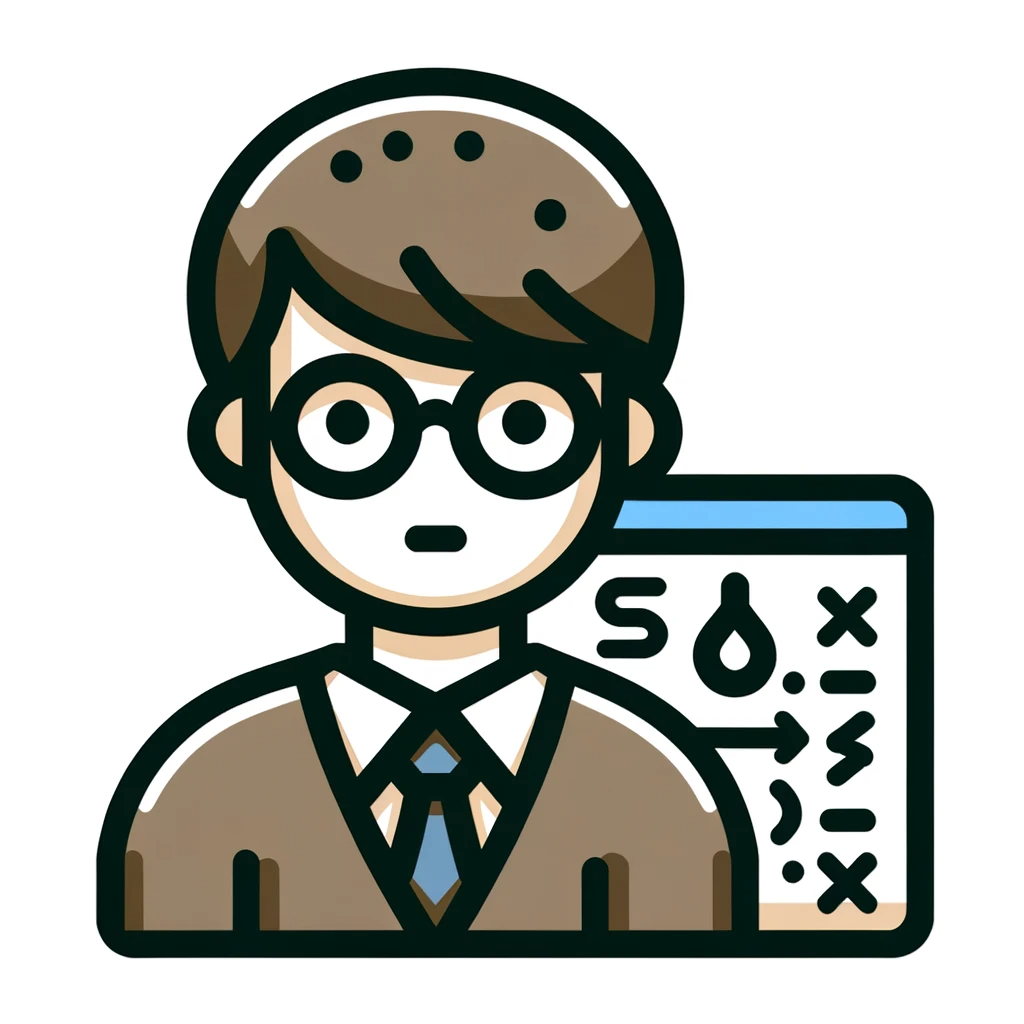
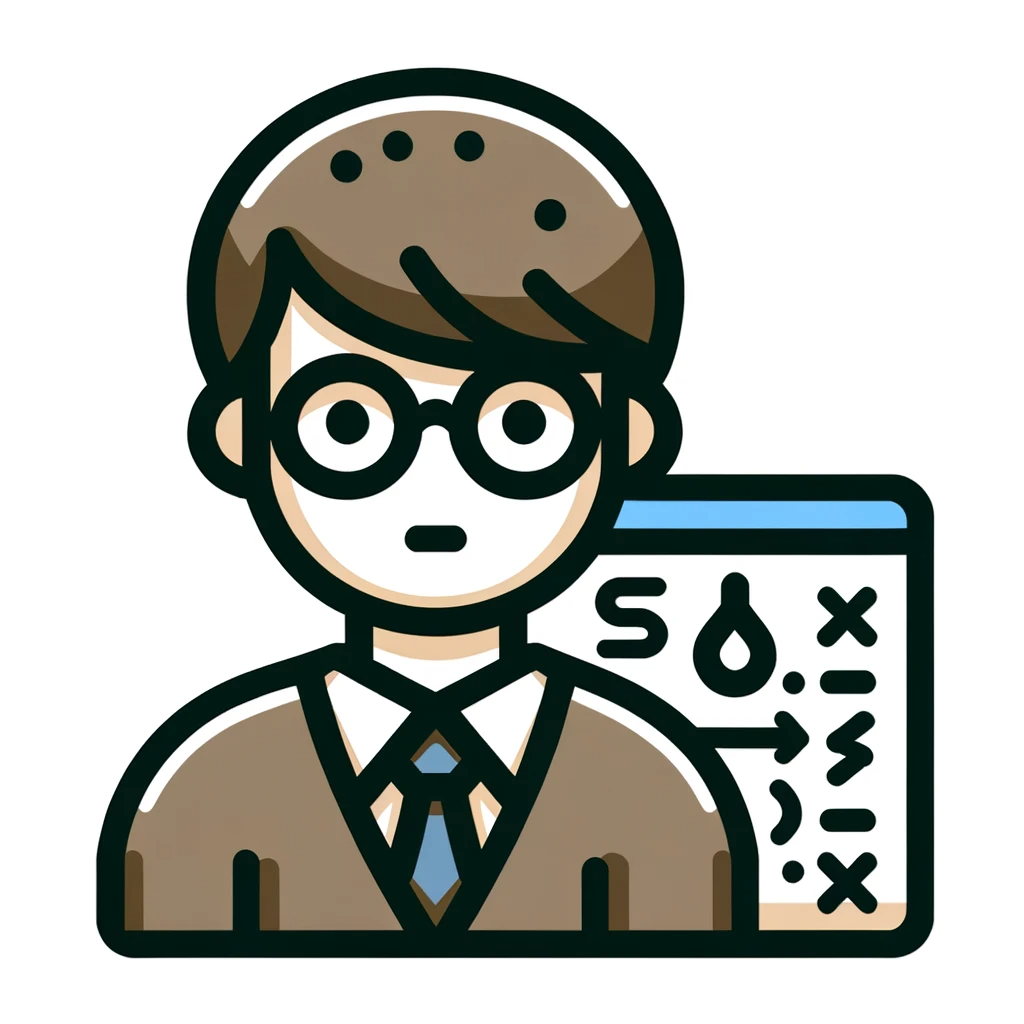
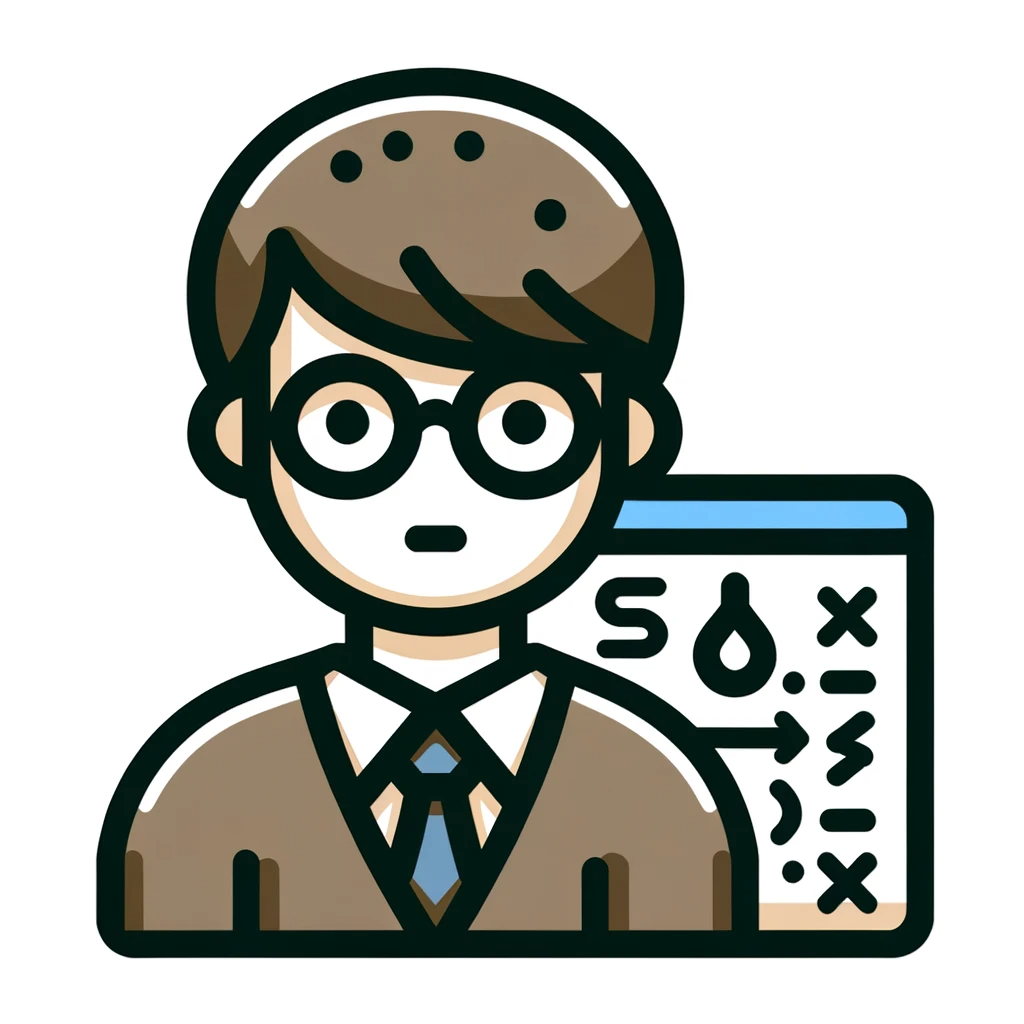
Understanding JavaScript events is important for creating dynamic content for web pages.
By understanding when events occur and how to set up event handlers, you can develop advanced web applications.
Also, be sure to consider accessibility when using the event.
Comments