We will explain how to escape URIs in JavaScript and introduce a method using the encodeURI function and encodeURIComponent function.
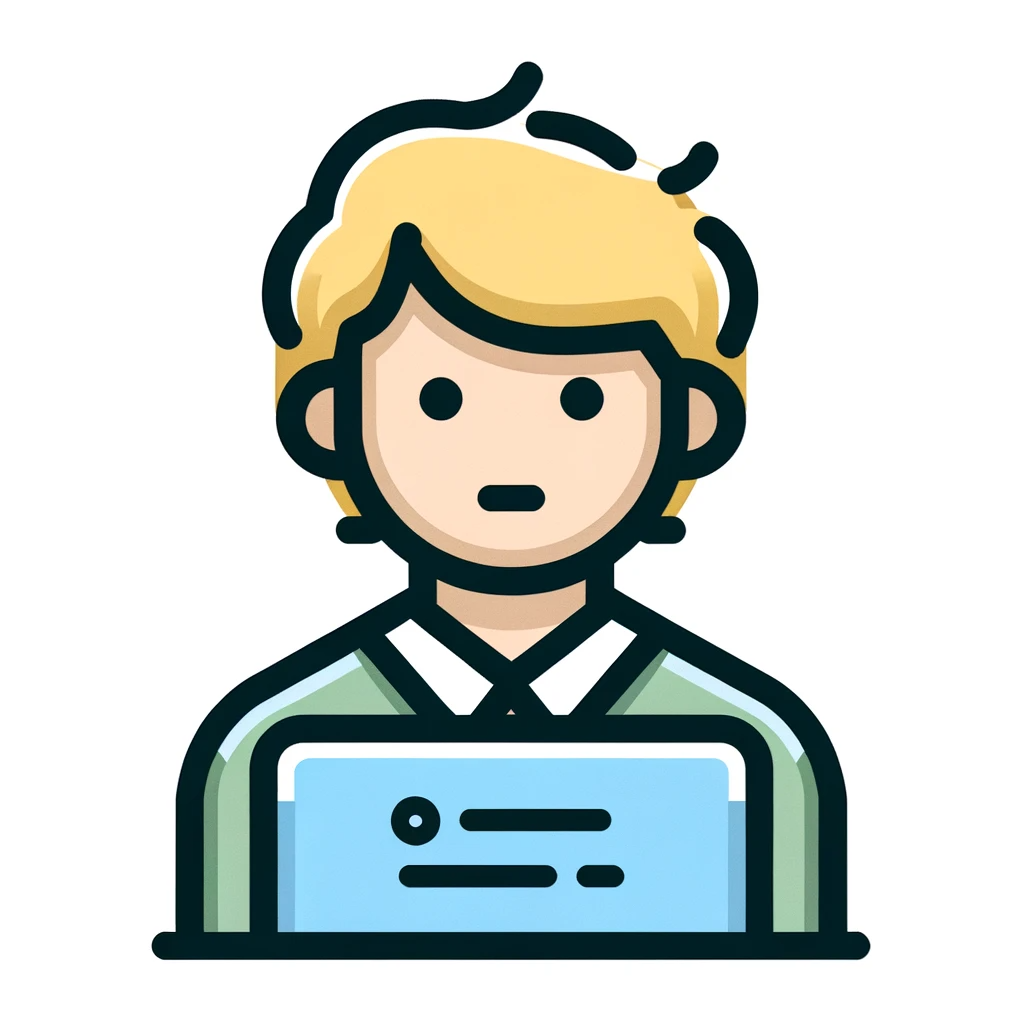
How can I escape the URI?
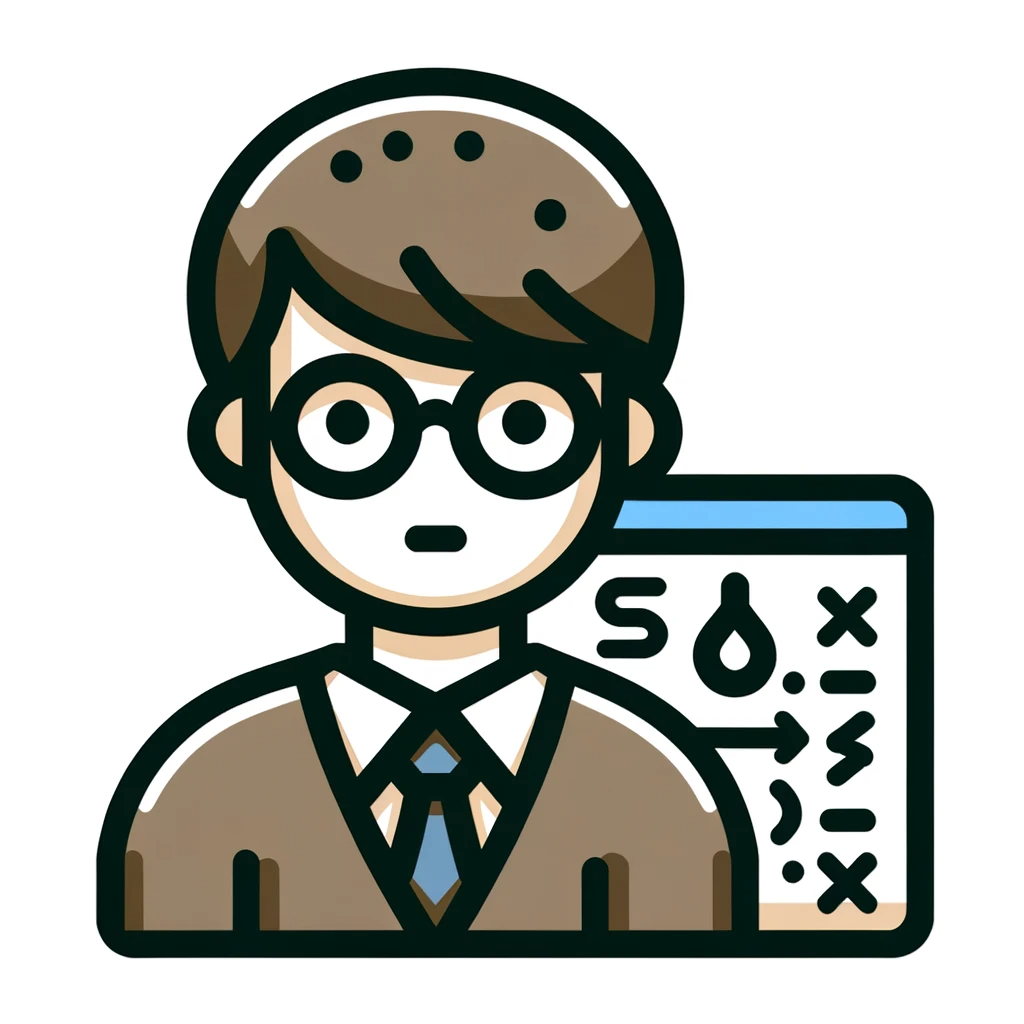
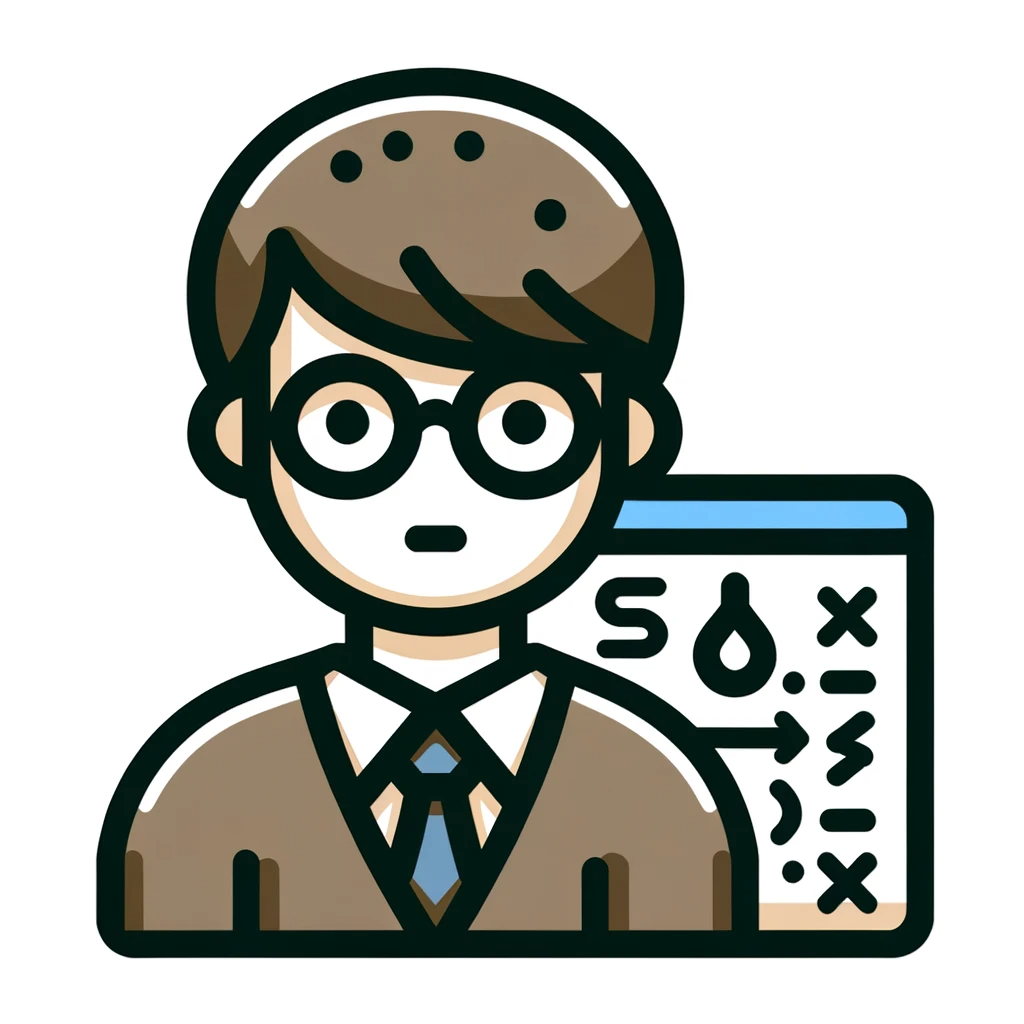
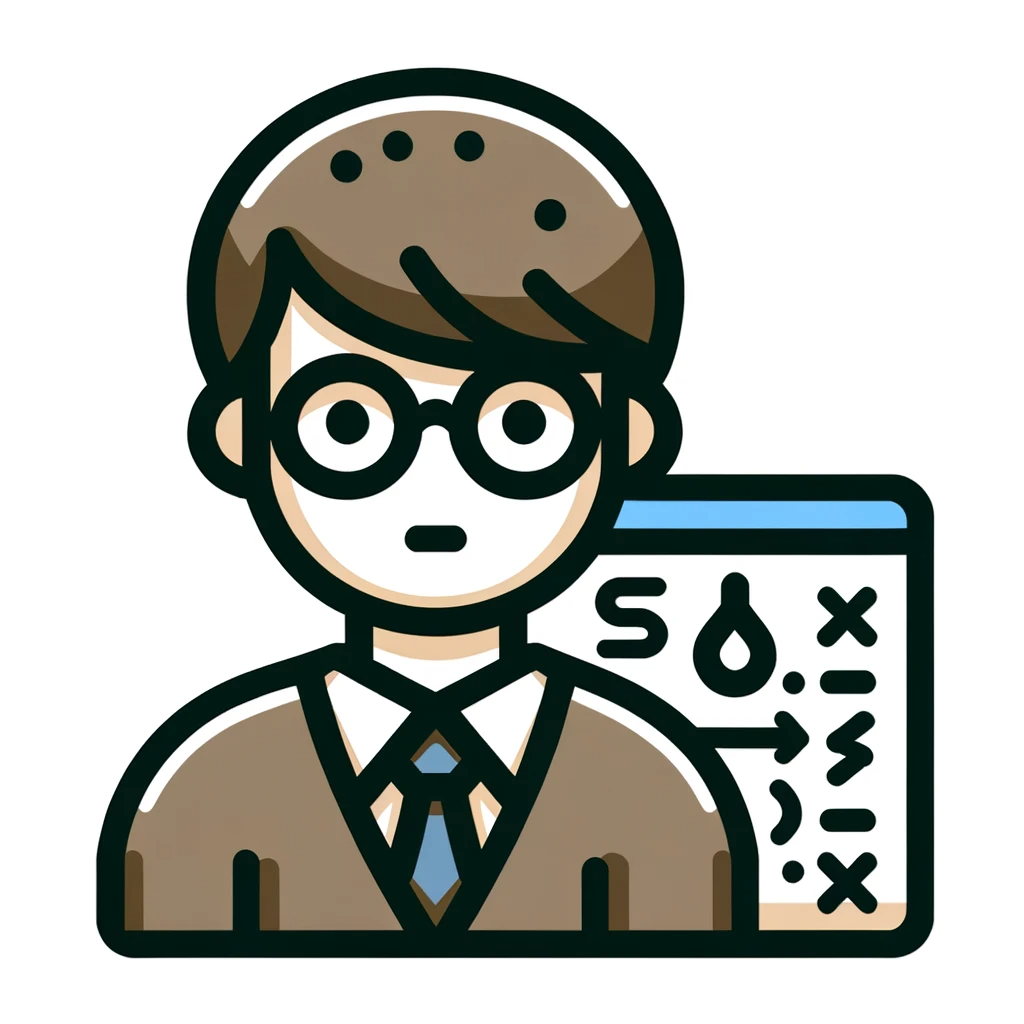
You can convert by using the encodeURI function or encodeURIComponent function.
How to escape URI using encodeURI function
There are two functions available to escape URIs: encodeURI and encodeURIComponent.
The encodeURI function is provided for use in URL encoding. This function converts valid URL characters and converts other characters to %encoded form.
Below is a sample program using the encodeURI function.
const url = "https://example.com?name=John Doe&age=20";
const encodedUrl = encodeURI(url);
console.log(encodedUrl); // "https://example.com?name=John%20Doe&age=20"
In this program, the string “https://example.com?name=John Doe&age=20” is assigned to the variable url, and the string encoded using the encodeURI function is assigned to encodedUrl.
This way, the encodeURI function converts spaces to %20 and escapes special characters.
Use encodeURIComponent if query information contains special characters
If the query information contains special characters, you can convert them using the encodeURIComponent function.
This function converts all characters to %encoded format.
Below is a sample program using the encodeURIComponent function.
const query = "name=John+Doe&age=20";
const encodedQuery = encodeURIComponent(query);
console.log(encodedQuery); // "name=John%2BDoe&age=20"
In general, it is best to use the encodeURI function to encode the entire URL, and the encodeURIComponent function to encode just the query string.
We recommend that you avoid using the traditional escape method, as its results tend to vary depending on the environment and character code, and it is now deprecated.
How to restore escaped characters
There are two functions available to return escaped characters: decodeURI and decodeURIComponent .
The decodeURI function is provided for use in URL decoding. This function decodes valid URL characters expressed in %encoded format .
Below is a sample program using the decodeURI function.
const encodedUrl = "https://example.com?name=John%20Doe";
const decodedUrl = decodeURI(encodedUrl);
console.log(decodedUrl); // "https://example.com?name=John Doe"
The decodeURIComponent function is provided to decode %encoded formats of query strings. This function decodes characters represented in %encoded format .
Below is a sample program using the decodeURIComponent function.
const encodedQuery = "name=John%2BDoe&age=20";
const decodedQuery = decodeURIComponent(encodedQuery);
console.log(decodedQuery); // "name=John+Doe&age=20"
In general, it is best to use the decodeURI function when decoding the entire URL, and the decodeURIComponent function when decoding just the query string.
Summary
“How to escape URI with encodeURI/encodeURIComponent” is summarized below.
- There are two functions available to escape URIs in JavaScript: encodeURI and encodeURIComponent.
- The encodeURI function is used for URL encoding, converting valid URL characters and converting other characters to %encoded format.
- The encodeURIComponent function encodes the query string, converting all characters to %encoded format.
- In general, it is best to use the encodeURI function to encode the entire URL, and the encodeURIComponent function to encode just the query string.
- There are two functions available to return escaped characters in JavaScript: decodeURI and decodeURIComponent.
- The decodeURI function is used for URL decoding and decodes valid URL characters expressed in %encoded format.
- The decodeURIComponent function decodes the %encoded form of the query string and decodes the characters represented in the %encoded form.
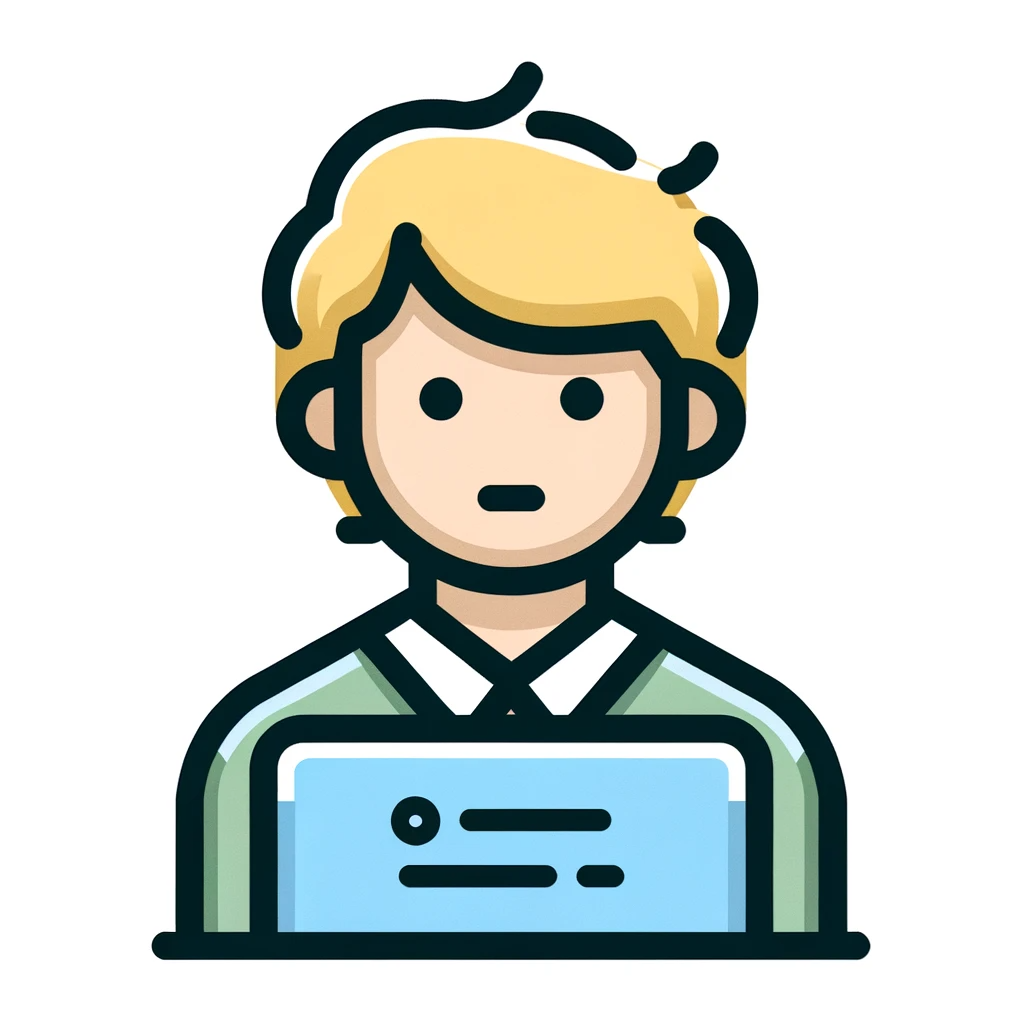
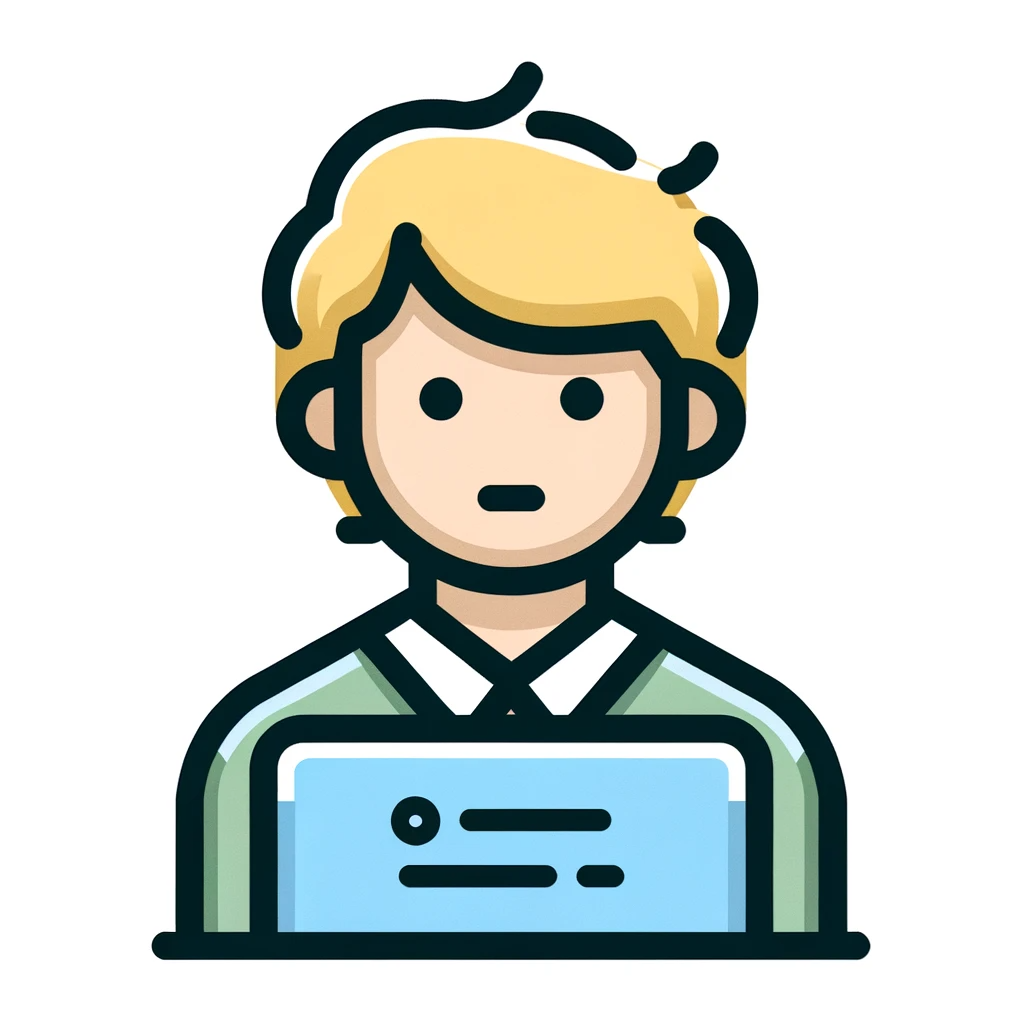
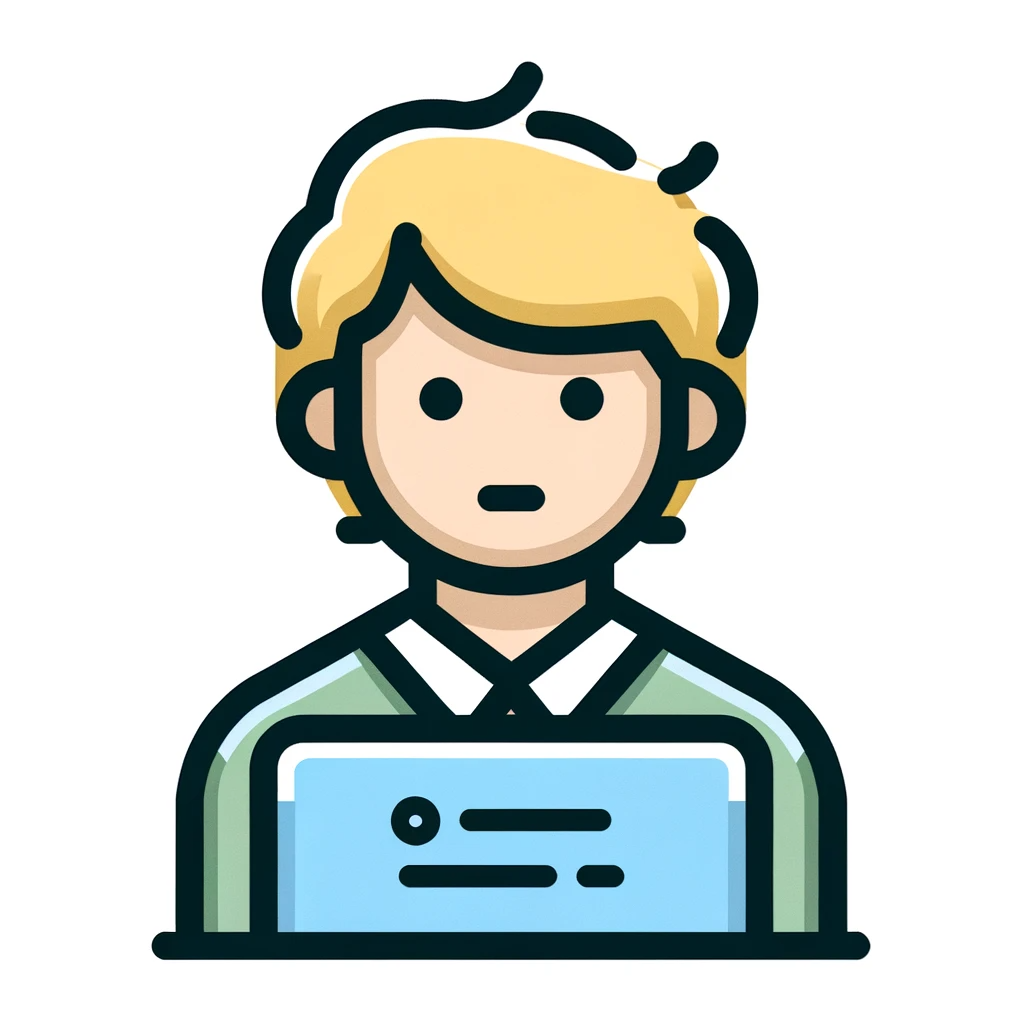
You now know how to escape URIs in JavaScript using the encodeURI and encodeURIComponent functions.
It would be nice to know what to do when the query information contains special characters.
Comments